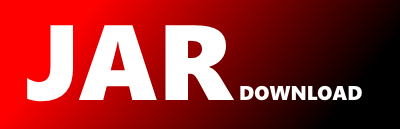
software.amazon.awssdk.services.sts.model.AssumeRoleWithWebIdentityResponse Maven / Gradle / Ivy
Show all versions of sts Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.sts.model;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Contains the response to a successful AssumeRoleWithWebIdentity request, including temporary AWS credentials
* that can be used to make AWS requests.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class AssumeRoleWithWebIdentityResponse extends StsResponse implements
ToCopyableBuilder {
private static final SdkField CREDENTIALS_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("Credentials").getter(getter(AssumeRoleWithWebIdentityResponse::credentials))
.setter(setter(Builder::credentials)).constructor(Credentials::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Credentials").build()).build();
private static final SdkField SUBJECT_FROM_WEB_IDENTITY_TOKEN_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("SubjectFromWebIdentityToken")
.getter(getter(AssumeRoleWithWebIdentityResponse::subjectFromWebIdentityToken))
.setter(setter(Builder::subjectFromWebIdentityToken))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SubjectFromWebIdentityToken")
.build()).build();
private static final SdkField ASSUMED_ROLE_USER_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("AssumedRoleUser")
.getter(getter(AssumeRoleWithWebIdentityResponse::assumedRoleUser)).setter(setter(Builder::assumedRoleUser))
.constructor(AssumedRoleUser::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AssumedRoleUser").build()).build();
private static final SdkField PACKED_POLICY_SIZE_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("PackedPolicySize").getter(getter(AssumeRoleWithWebIdentityResponse::packedPolicySize))
.setter(setter(Builder::packedPolicySize))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PackedPolicySize").build()).build();
private static final SdkField PROVIDER_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Provider").getter(getter(AssumeRoleWithWebIdentityResponse::provider)).setter(setter(Builder::provider))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Provider").build()).build();
private static final SdkField AUDIENCE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Audience").getter(getter(AssumeRoleWithWebIdentityResponse::audience)).setter(setter(Builder::audience))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Audience").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(CREDENTIALS_FIELD,
SUBJECT_FROM_WEB_IDENTITY_TOKEN_FIELD, ASSUMED_ROLE_USER_FIELD, PACKED_POLICY_SIZE_FIELD, PROVIDER_FIELD,
AUDIENCE_FIELD));
private final Credentials credentials;
private final String subjectFromWebIdentityToken;
private final AssumedRoleUser assumedRoleUser;
private final Integer packedPolicySize;
private final String provider;
private final String audience;
private AssumeRoleWithWebIdentityResponse(BuilderImpl builder) {
super(builder);
this.credentials = builder.credentials;
this.subjectFromWebIdentityToken = builder.subjectFromWebIdentityToken;
this.assumedRoleUser = builder.assumedRoleUser;
this.packedPolicySize = builder.packedPolicySize;
this.provider = builder.provider;
this.audience = builder.audience;
}
/**
*
* The temporary security credentials, which include an access key ID, a secret access key, and a security token.
*
*
*
* The size of the security token that STS API operations return is not fixed. We strongly recommend that you make
* no assumptions about the maximum size.
*
*
*
* @return The temporary security credentials, which include an access key ID, a secret access key, and a security
* token.
*
* The size of the security token that STS API operations return is not fixed. We strongly recommend that
* you make no assumptions about the maximum size.
*
*/
public Credentials credentials() {
return credentials;
}
/**
*
* The unique user identifier that is returned by the identity provider. This identifier is associated with the
* WebIdentityToken
that was submitted with the AssumeRoleWithWebIdentity
call. The
* identifier is typically unique to the user and the application that acquired the WebIdentityToken
* (pairwise identifier). For OpenID Connect ID tokens, this field contains the value returned by the identity
* provider as the token's sub
(Subject) claim.
*
*
* @return The unique user identifier that is returned by the identity provider. This identifier is associated with
* the WebIdentityToken
that was submitted with the AssumeRoleWithWebIdentity
* call. The identifier is typically unique to the user and the application that acquired the
* WebIdentityToken
(pairwise identifier). For OpenID Connect ID tokens, this field contains
* the value returned by the identity provider as the token's sub
(Subject) claim.
*/
public String subjectFromWebIdentityToken() {
return subjectFromWebIdentityToken;
}
/**
*
* The Amazon Resource Name (ARN) and the assumed role ID, which are identifiers that you can use to refer to the
* resulting temporary security credentials. For example, you can reference these credentials as a principal in a
* resource-based policy by using the ARN or assumed role ID. The ARN and ID include the
* RoleSessionName
that you specified when you called AssumeRole
.
*
*
* @return The Amazon Resource Name (ARN) and the assumed role ID, which are identifiers that you can use to refer
* to the resulting temporary security credentials. For example, you can reference these credentials as a
* principal in a resource-based policy by using the ARN or assumed role ID. The ARN and ID include the
* RoleSessionName
that you specified when you called AssumeRole
.
*/
public AssumedRoleUser assumedRoleUser() {
return assumedRoleUser;
}
/**
*
* A percentage value that indicates the packed size of the session policies and session tags combined passed in the
* request. The request fails if the packed size is greater than 100 percent, which means the policies and tags
* exceeded the allowed space.
*
*
* @return A percentage value that indicates the packed size of the session policies and session tags combined
* passed in the request. The request fails if the packed size is greater than 100 percent, which means the
* policies and tags exceeded the allowed space.
*/
public Integer packedPolicySize() {
return packedPolicySize;
}
/**
*
* The issuing authority of the web identity token presented. For OpenID Connect ID tokens, this contains the value
* of the iss
field. For OAuth 2.0 access tokens, this contains the value of the
* ProviderId
parameter that was passed in the AssumeRoleWithWebIdentity
request.
*
*
* @return The issuing authority of the web identity token presented. For OpenID Connect ID tokens, this contains
* the value of the iss
field. For OAuth 2.0 access tokens, this contains the value of the
* ProviderId
parameter that was passed in the AssumeRoleWithWebIdentity
request.
*/
public String provider() {
return provider;
}
/**
*
* The intended audience (also known as client ID) of the web identity token. This is traditionally the client
* identifier issued to the application that requested the web identity token.
*
*
* @return The intended audience (also known as client ID) of the web identity token. This is traditionally the
* client identifier issued to the application that requested the web identity token.
*/
public String audience() {
return audience;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(credentials());
hashCode = 31 * hashCode + Objects.hashCode(subjectFromWebIdentityToken());
hashCode = 31 * hashCode + Objects.hashCode(assumedRoleUser());
hashCode = 31 * hashCode + Objects.hashCode(packedPolicySize());
hashCode = 31 * hashCode + Objects.hashCode(provider());
hashCode = 31 * hashCode + Objects.hashCode(audience());
return hashCode;
}
@Override
public boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof AssumeRoleWithWebIdentityResponse)) {
return false;
}
AssumeRoleWithWebIdentityResponse other = (AssumeRoleWithWebIdentityResponse) obj;
return Objects.equals(credentials(), other.credentials())
&& Objects.equals(subjectFromWebIdentityToken(), other.subjectFromWebIdentityToken())
&& Objects.equals(assumedRoleUser(), other.assumedRoleUser())
&& Objects.equals(packedPolicySize(), other.packedPolicySize()) && Objects.equals(provider(), other.provider())
&& Objects.equals(audience(), other.audience());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("AssumeRoleWithWebIdentityResponse").add("Credentials", credentials())
.add("SubjectFromWebIdentityToken", subjectFromWebIdentityToken()).add("AssumedRoleUser", assumedRoleUser())
.add("PackedPolicySize", packedPolicySize()).add("Provider", provider()).add("Audience", audience()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Credentials":
return Optional.ofNullable(clazz.cast(credentials()));
case "SubjectFromWebIdentityToken":
return Optional.ofNullable(clazz.cast(subjectFromWebIdentityToken()));
case "AssumedRoleUser":
return Optional.ofNullable(clazz.cast(assumedRoleUser()));
case "PackedPolicySize":
return Optional.ofNullable(clazz.cast(packedPolicySize()));
case "Provider":
return Optional.ofNullable(clazz.cast(provider()));
case "Audience":
return Optional.ofNullable(clazz.cast(audience()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function