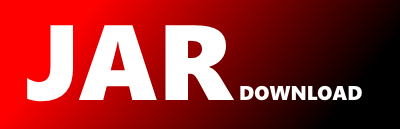
software.amazon.awssdk.services.support.model.CaseDetails Maven / Gradle / Ivy
Show all versions of support Show documentation
/*
* Copyright 2014-2019 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.support.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* A JSON-formatted object that contains the metadata for a support case. It is contained the response from a
* DescribeCases request. CaseDetails contains the following fields:
*
*
* -
*
* caseId. The AWS Support case ID requested or returned in the call. The case ID is an alphanumeric string
* formatted as shown in this example: case-12345678910-2013-c4c1d2bf33c5cf47.
*
*
* -
*
* categoryCode. The category of problem for the AWS Support case. Corresponds to the CategoryCode values
* returned by a call to DescribeServices.
*
*
* -
*
* displayId. The identifier for the case on pages in the AWS Support Center.
*
*
* -
*
* language. The ISO 639-1 code for the language in which AWS provides support. AWS Support currently supports
* English ("en") and Japanese ("ja"). Language parameters must be passed explicitly for operations that take them.
*
*
* -
*
* recentCommunications. One or more Communication objects. Fields of these objects are
* attachments
, body
, caseId
, submittedBy
, and
* timeCreated
.
*
*
* -
*
* nextToken. A resumption point for pagination.
*
*
* -
*
* serviceCode. The identifier for the AWS service that corresponds to the service code defined in the call to
* DescribeServices.
*
*
* -
*
* severityCode. The severity code assigned to the case. Contains one of the values returned by the call to
* DescribeSeverityLevels.
*
*
* -
*
* status. The status of the case in the AWS Support Center.
*
*
* -
*
* subject. The subject line of the case.
*
*
* -
*
* submittedBy. The email address of the account that submitted the case.
*
*
* -
*
* timeCreated. The time the case was created, in ISO-8601 format.
*
*
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class CaseDetails implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField CASE_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(CaseDetails::caseId)).setter(setter(Builder::caseId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("caseId").build()).build();
private static final SdkField DISPLAY_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(CaseDetails::displayId)).setter(setter(Builder::displayId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("displayId").build()).build();
private static final SdkField SUBJECT_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(CaseDetails::subject)).setter(setter(Builder::subject))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("subject").build()).build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(CaseDetails::status)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("status").build()).build();
private static final SdkField SERVICE_CODE_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(CaseDetails::serviceCode)).setter(setter(Builder::serviceCode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("serviceCode").build()).build();
private static final SdkField CATEGORY_CODE_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(CaseDetails::categoryCode)).setter(setter(Builder::categoryCode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("categoryCode").build()).build();
private static final SdkField SEVERITY_CODE_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(CaseDetails::severityCode)).setter(setter(Builder::severityCode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("severityCode").build()).build();
private static final SdkField SUBMITTED_BY_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(CaseDetails::submittedBy)).setter(setter(Builder::submittedBy))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("submittedBy").build()).build();
private static final SdkField TIME_CREATED_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(CaseDetails::timeCreated)).setter(setter(Builder::timeCreated))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("timeCreated").build()).build();
private static final SdkField RECENT_COMMUNICATIONS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).getter(getter(CaseDetails::recentCommunications))
.setter(setter(Builder::recentCommunications)).constructor(RecentCaseCommunications::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("recentCommunications").build())
.build();
private static final SdkField> CC_EMAIL_ADDRESSES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(CaseDetails::ccEmailAddresses))
.setter(setter(Builder::ccEmailAddresses))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ccEmailAddresses").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField LANGUAGE_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(CaseDetails::language)).setter(setter(Builder::language))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("language").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(CASE_ID_FIELD,
DISPLAY_ID_FIELD, SUBJECT_FIELD, STATUS_FIELD, SERVICE_CODE_FIELD, CATEGORY_CODE_FIELD, SEVERITY_CODE_FIELD,
SUBMITTED_BY_FIELD, TIME_CREATED_FIELD, RECENT_COMMUNICATIONS_FIELD, CC_EMAIL_ADDRESSES_FIELD, LANGUAGE_FIELD));
private static final long serialVersionUID = 1L;
private final String caseId;
private final String displayId;
private final String subject;
private final String status;
private final String serviceCode;
private final String categoryCode;
private final String severityCode;
private final String submittedBy;
private final String timeCreated;
private final RecentCaseCommunications recentCommunications;
private final List ccEmailAddresses;
private final String language;
private CaseDetails(BuilderImpl builder) {
this.caseId = builder.caseId;
this.displayId = builder.displayId;
this.subject = builder.subject;
this.status = builder.status;
this.serviceCode = builder.serviceCode;
this.categoryCode = builder.categoryCode;
this.severityCode = builder.severityCode;
this.submittedBy = builder.submittedBy;
this.timeCreated = builder.timeCreated;
this.recentCommunications = builder.recentCommunications;
this.ccEmailAddresses = builder.ccEmailAddresses;
this.language = builder.language;
}
/**
*
* The AWS Support case ID requested or returned in the call. The case ID is an alphanumeric string formatted as
* shown in this example: case-12345678910-2013-c4c1d2bf33c5cf47
*
*
* @return The AWS Support case ID requested or returned in the call. The case ID is an alphanumeric string
* formatted as shown in this example: case-12345678910-2013-c4c1d2bf33c5cf47
*/
public String caseId() {
return caseId;
}
/**
*
* The ID displayed for the case in the AWS Support Center. This is a numeric string.
*
*
* @return The ID displayed for the case in the AWS Support Center. This is a numeric string.
*/
public String displayId() {
return displayId;
}
/**
*
* The subject line for the case in the AWS Support Center.
*
*
* @return The subject line for the case in the AWS Support Center.
*/
public String subject() {
return subject;
}
/**
*
* The status of the case.
*
*
* @return The status of the case.
*/
public String status() {
return status;
}
/**
*
* The code for the AWS service returned by the call to DescribeServices.
*
*
* @return The code for the AWS service returned by the call to DescribeServices.
*/
public String serviceCode() {
return serviceCode;
}
/**
*
* The category of problem for the AWS Support case.
*
*
* @return The category of problem for the AWS Support case.
*/
public String categoryCode() {
return categoryCode;
}
/**
*
* The code for the severity level returned by the call to DescribeSeverityLevels.
*
*
* @return The code for the severity level returned by the call to DescribeSeverityLevels.
*/
public String severityCode() {
return severityCode;
}
/**
*
* The email address of the account that submitted the case.
*
*
* @return The email address of the account that submitted the case.
*/
public String submittedBy() {
return submittedBy;
}
/**
*
* The time that the case was case created in the AWS Support Center.
*
*
* @return The time that the case was case created in the AWS Support Center.
*/
public String timeCreated() {
return timeCreated;
}
/**
*
* The five most recent communications between you and AWS Support Center, including the IDs of any attachments to
* the communications. Also includes a nextToken
that you can use to retrieve earlier communications.
*
*
* @return The five most recent communications between you and AWS Support Center, including the IDs of any
* attachments to the communications. Also includes a nextToken
that you can use to retrieve
* earlier communications.
*/
public RecentCaseCommunications recentCommunications() {
return recentCommunications;
}
/**
*
* The email addresses that receive copies of communication about the case.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return The email addresses that receive copies of communication about the case.
*/
public List ccEmailAddresses() {
return ccEmailAddresses;
}
/**
*
* The ISO 639-1 code for the language in which AWS provides support. AWS Support currently supports English ("en")
* and Japanese ("ja"). Language parameters must be passed explicitly for operations that take them.
*
*
* @return The ISO 639-1 code for the language in which AWS provides support. AWS Support currently supports English
* ("en") and Japanese ("ja"). Language parameters must be passed explicitly for operations that take them.
*/
public String language() {
return language;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(caseId());
hashCode = 31 * hashCode + Objects.hashCode(displayId());
hashCode = 31 * hashCode + Objects.hashCode(subject());
hashCode = 31 * hashCode + Objects.hashCode(status());
hashCode = 31 * hashCode + Objects.hashCode(serviceCode());
hashCode = 31 * hashCode + Objects.hashCode(categoryCode());
hashCode = 31 * hashCode + Objects.hashCode(severityCode());
hashCode = 31 * hashCode + Objects.hashCode(submittedBy());
hashCode = 31 * hashCode + Objects.hashCode(timeCreated());
hashCode = 31 * hashCode + Objects.hashCode(recentCommunications());
hashCode = 31 * hashCode + Objects.hashCode(ccEmailAddresses());
hashCode = 31 * hashCode + Objects.hashCode(language());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CaseDetails)) {
return false;
}
CaseDetails other = (CaseDetails) obj;
return Objects.equals(caseId(), other.caseId()) && Objects.equals(displayId(), other.displayId())
&& Objects.equals(subject(), other.subject()) && Objects.equals(status(), other.status())
&& Objects.equals(serviceCode(), other.serviceCode()) && Objects.equals(categoryCode(), other.categoryCode())
&& Objects.equals(severityCode(), other.severityCode()) && Objects.equals(submittedBy(), other.submittedBy())
&& Objects.equals(timeCreated(), other.timeCreated())
&& Objects.equals(recentCommunications(), other.recentCommunications())
&& Objects.equals(ccEmailAddresses(), other.ccEmailAddresses()) && Objects.equals(language(), other.language());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("CaseDetails").add("CaseId", caseId()).add("DisplayId", displayId()).add("Subject", subject())
.add("Status", status()).add("ServiceCode", serviceCode()).add("CategoryCode", categoryCode())
.add("SeverityCode", severityCode()).add("SubmittedBy", submittedBy()).add("TimeCreated", timeCreated())
.add("RecentCommunications", recentCommunications()).add("CcEmailAddresses", ccEmailAddresses())
.add("Language", language()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "caseId":
return Optional.ofNullable(clazz.cast(caseId()));
case "displayId":
return Optional.ofNullable(clazz.cast(displayId()));
case "subject":
return Optional.ofNullable(clazz.cast(subject()));
case "status":
return Optional.ofNullable(clazz.cast(status()));
case "serviceCode":
return Optional.ofNullable(clazz.cast(serviceCode()));
case "categoryCode":
return Optional.ofNullable(clazz.cast(categoryCode()));
case "severityCode":
return Optional.ofNullable(clazz.cast(severityCode()));
case "submittedBy":
return Optional.ofNullable(clazz.cast(submittedBy()));
case "timeCreated":
return Optional.ofNullable(clazz.cast(timeCreated()));
case "recentCommunications":
return Optional.ofNullable(clazz.cast(recentCommunications()));
case "ccEmailAddresses":
return Optional.ofNullable(clazz.cast(ccEmailAddresses()));
case "language":
return Optional.ofNullable(clazz.cast(language()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function