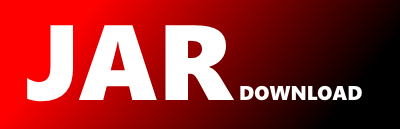
software.amazon.awssdk.services.swf.model.PollForDecisionTaskRequest Maven / Gradle / Ivy
Show all versions of swf Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.swf.model;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class PollForDecisionTaskRequest extends SwfRequest implements
ToCopyableBuilder {
private static final SdkField DOMAIN_FIELD = SdkField. builder(MarshallingType.STRING).memberName("domain")
.getter(getter(PollForDecisionTaskRequest::domain)).setter(setter(Builder::domain))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("domain").build()).build();
private static final SdkField TASK_LIST_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("taskList").getter(getter(PollForDecisionTaskRequest::taskList)).setter(setter(Builder::taskList))
.constructor(TaskList::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("taskList").build()).build();
private static final SdkField IDENTITY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("identity").getter(getter(PollForDecisionTaskRequest::identity)).setter(setter(Builder::identity))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("identity").build()).build();
private static final SdkField NEXT_PAGE_TOKEN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("nextPageToken").getter(getter(PollForDecisionTaskRequest::nextPageToken))
.setter(setter(Builder::nextPageToken))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("nextPageToken").build()).build();
private static final SdkField MAXIMUM_PAGE_SIZE_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("maximumPageSize").getter(getter(PollForDecisionTaskRequest::maximumPageSize))
.setter(setter(Builder::maximumPageSize))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("maximumPageSize").build()).build();
private static final SdkField REVERSE_ORDER_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("reverseOrder").getter(getter(PollForDecisionTaskRequest::reverseOrder))
.setter(setter(Builder::reverseOrder))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("reverseOrder").build()).build();
private static final SdkField START_AT_PREVIOUS_STARTED_EVENT_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("startAtPreviousStartedEvent")
.getter(getter(PollForDecisionTaskRequest::startAtPreviousStartedEvent))
.setter(setter(Builder::startAtPreviousStartedEvent))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("startAtPreviousStartedEvent")
.build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(DOMAIN_FIELD, TASK_LIST_FIELD,
IDENTITY_FIELD, NEXT_PAGE_TOKEN_FIELD, MAXIMUM_PAGE_SIZE_FIELD, REVERSE_ORDER_FIELD,
START_AT_PREVIOUS_STARTED_EVENT_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("domain", DOMAIN_FIELD);
put("taskList", TASK_LIST_FIELD);
put("identity", IDENTITY_FIELD);
put("nextPageToken", NEXT_PAGE_TOKEN_FIELD);
put("maximumPageSize", MAXIMUM_PAGE_SIZE_FIELD);
put("reverseOrder", REVERSE_ORDER_FIELD);
put("startAtPreviousStartedEvent", START_AT_PREVIOUS_STARTED_EVENT_FIELD);
}
});
private final String domain;
private final TaskList taskList;
private final String identity;
private final String nextPageToken;
private final Integer maximumPageSize;
private final Boolean reverseOrder;
private final Boolean startAtPreviousStartedEvent;
private PollForDecisionTaskRequest(BuilderImpl builder) {
super(builder);
this.domain = builder.domain;
this.taskList = builder.taskList;
this.identity = builder.identity;
this.nextPageToken = builder.nextPageToken;
this.maximumPageSize = builder.maximumPageSize;
this.reverseOrder = builder.reverseOrder;
this.startAtPreviousStartedEvent = builder.startAtPreviousStartedEvent;
}
/**
*
* The name of the domain containing the task lists to poll.
*
*
* @return The name of the domain containing the task lists to poll.
*/
public final String domain() {
return domain;
}
/**
*
* Specifies the task list to poll for decision tasks.
*
*
* The specified string must not contain a :
(colon), /
(slash), |
(vertical
* bar), or any control characters (\u0000-\u001f
| \u007f-\u009f
). Also, it must
* not be the literal string arn
.
*
*
* @return Specifies the task list to poll for decision tasks.
*
* The specified string must not contain a :
(colon), /
(slash), |
* (vertical bar), or any control characters (\u0000-\u001f
| \u007f-\u009f
).
* Also, it must not be the literal string arn
.
*/
public final TaskList taskList() {
return taskList;
}
/**
*
* Identity of the decider making the request, which is recorded in the DecisionTaskStarted event in the workflow
* history. This enables diagnostic tracing when problems arise. The form of this identity is user defined.
*
*
* @return Identity of the decider making the request, which is recorded in the DecisionTaskStarted event in the
* workflow history. This enables diagnostic tracing when problems arise. The form of this identity is user
* defined.
*/
public final String identity() {
return identity;
}
/**
*
* If NextPageToken
is returned there are more results available. The value of
* NextPageToken
is a unique pagination token for each page. Make the call again using the returned
* token to retrieve the next page. Keep all other arguments unchanged. Each pagination token expires after 24
* hours. Using an expired pagination token will return a 400
error: "
* Specified token has exceeded its maximum lifetime
".
*
*
* The configured maximumPageSize
determines how many results can be returned in a single call.
*
*
*
* The nextPageToken
returned by this action cannot be used with GetWorkflowExecutionHistory to
* get the next page. You must call PollForDecisionTask again (with the nextPageToken
) to
* retrieve the next page of history records. Calling PollForDecisionTask with a nextPageToken
* doesn't return a new decision task.
*
*
*
* @return If NextPageToken
is returned there are more results available. The value of
* NextPageToken
is a unique pagination token for each page. Make the call again using the
* returned token to retrieve the next page. Keep all other arguments unchanged. Each pagination token
* expires after 24 hours. Using an expired pagination token will return a 400
error: "
* Specified token has exceeded its maximum lifetime
".
*
* The configured maximumPageSize
determines how many results can be returned in a single call.
*
*
*
* The nextPageToken
returned by this action cannot be used with
* GetWorkflowExecutionHistory to get the next page. You must call PollForDecisionTask again
* (with the nextPageToken
) to retrieve the next page of history records. Calling
* PollForDecisionTask with a nextPageToken
doesn't return a new decision task.
*
*/
public final String nextPageToken() {
return nextPageToken;
}
/**
*
* The maximum number of results that are returned per call. Use nextPageToken
to obtain further pages
* of results.
*
*
* This is an upper limit only; the actual number of results returned per call may be fewer than the specified
* maximum.
*
*
* @return The maximum number of results that are returned per call. Use nextPageToken
to obtain
* further pages of results.
*
* This is an upper limit only; the actual number of results returned per call may be fewer than the
* specified maximum.
*/
public final Integer maximumPageSize() {
return maximumPageSize;
}
/**
*
* When set to true
, returns the events in reverse order. By default the results are returned in
* ascending order of the eventTimestamp
of the events.
*
*
* @return When set to true
, returns the events in reverse order. By default the results are returned
* in ascending order of the eventTimestamp
of the events.
*/
public final Boolean reverseOrder() {
return reverseOrder;
}
/**
*
* When set to true
, returns the events with eventTimestamp
greater than or equal to
* eventTimestamp
of the most recent DecisionTaskStarted
event. By default, this parameter
* is set to false
.
*
*
* @return When set to true
, returns the events with eventTimestamp
greater than or equal
* to eventTimestamp
of the most recent DecisionTaskStarted
event. By default,
* this parameter is set to false
.
*/
public final Boolean startAtPreviousStartedEvent() {
return startAtPreviousStartedEvent;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(domain());
hashCode = 31 * hashCode + Objects.hashCode(taskList());
hashCode = 31 * hashCode + Objects.hashCode(identity());
hashCode = 31 * hashCode + Objects.hashCode(nextPageToken());
hashCode = 31 * hashCode + Objects.hashCode(maximumPageSize());
hashCode = 31 * hashCode + Objects.hashCode(reverseOrder());
hashCode = 31 * hashCode + Objects.hashCode(startAtPreviousStartedEvent());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof PollForDecisionTaskRequest)) {
return false;
}
PollForDecisionTaskRequest other = (PollForDecisionTaskRequest) obj;
return Objects.equals(domain(), other.domain()) && Objects.equals(taskList(), other.taskList())
&& Objects.equals(identity(), other.identity()) && Objects.equals(nextPageToken(), other.nextPageToken())
&& Objects.equals(maximumPageSize(), other.maximumPageSize())
&& Objects.equals(reverseOrder(), other.reverseOrder())
&& Objects.equals(startAtPreviousStartedEvent(), other.startAtPreviousStartedEvent());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("PollForDecisionTaskRequest").add("Domain", domain()).add("TaskList", taskList())
.add("Identity", identity()).add("NextPageToken", nextPageToken()).add("MaximumPageSize", maximumPageSize())
.add("ReverseOrder", reverseOrder()).add("StartAtPreviousStartedEvent", startAtPreviousStartedEvent()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "domain":
return Optional.ofNullable(clazz.cast(domain()));
case "taskList":
return Optional.ofNullable(clazz.cast(taskList()));
case "identity":
return Optional.ofNullable(clazz.cast(identity()));
case "nextPageToken":
return Optional.ofNullable(clazz.cast(nextPageToken()));
case "maximumPageSize":
return Optional.ofNullable(clazz.cast(maximumPageSize()));
case "reverseOrder":
return Optional.ofNullable(clazz.cast(reverseOrder()));
case "startAtPreviousStartedEvent":
return Optional.ofNullable(clazz.cast(startAtPreviousStartedEvent()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function