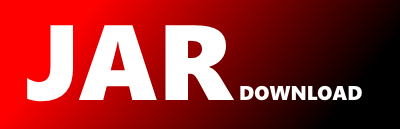
software.amazon.awssdk.services.swf.model.StartChildWorkflowExecutionFailedEventAttributes Maven / Gradle / Ivy
Show all versions of swf Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.swf.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Provides the details of the StartChildWorkflowExecutionFailed
event.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class StartChildWorkflowExecutionFailedEventAttributes
implements
SdkPojo,
Serializable,
ToCopyableBuilder {
private static final SdkField WORKFLOW_TYPE_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("workflowType").getter(getter(StartChildWorkflowExecutionFailedEventAttributes::workflowType))
.setter(setter(Builder::workflowType)).constructor(WorkflowType::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("workflowType").build()).build();
private static final SdkField CAUSE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("cause")
.getter(getter(StartChildWorkflowExecutionFailedEventAttributes::causeAsString)).setter(setter(Builder::cause))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("cause").build()).build();
private static final SdkField WORKFLOW_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("workflowId").getter(getter(StartChildWorkflowExecutionFailedEventAttributes::workflowId))
.setter(setter(Builder::workflowId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("workflowId").build()).build();
private static final SdkField INITIATED_EVENT_ID_FIELD = SdkField. builder(MarshallingType.LONG)
.memberName("initiatedEventId").getter(getter(StartChildWorkflowExecutionFailedEventAttributes::initiatedEventId))
.setter(setter(Builder::initiatedEventId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("initiatedEventId").build()).build();
private static final SdkField DECISION_TASK_COMPLETED_EVENT_ID_FIELD = SdkField
. builder(MarshallingType.LONG)
.memberName("decisionTaskCompletedEventId")
.getter(getter(StartChildWorkflowExecutionFailedEventAttributes::decisionTaskCompletedEventId))
.setter(setter(Builder::decisionTaskCompletedEventId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("decisionTaskCompletedEventId")
.build()).build();
private static final SdkField CONTROL_FIELD = SdkField. builder(MarshallingType.STRING).memberName("control")
.getter(getter(StartChildWorkflowExecutionFailedEventAttributes::control)).setter(setter(Builder::control))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("control").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(WORKFLOW_TYPE_FIELD,
CAUSE_FIELD, WORKFLOW_ID_FIELD, INITIATED_EVENT_ID_FIELD, DECISION_TASK_COMPLETED_EVENT_ID_FIELD, CONTROL_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("workflowType", WORKFLOW_TYPE_FIELD);
put("cause", CAUSE_FIELD);
put("workflowId", WORKFLOW_ID_FIELD);
put("initiatedEventId", INITIATED_EVENT_ID_FIELD);
put("decisionTaskCompletedEventId", DECISION_TASK_COMPLETED_EVENT_ID_FIELD);
put("control", CONTROL_FIELD);
}
});
private static final long serialVersionUID = 1L;
private final WorkflowType workflowType;
private final String causeValue;
private final String workflowId;
private final Long initiatedEventId;
private final Long decisionTaskCompletedEventId;
private final String control;
private StartChildWorkflowExecutionFailedEventAttributes(BuilderImpl builder) {
this.workflowType = builder.workflowType;
this.causeValue = builder.causeValue;
this.workflowId = builder.workflowId;
this.initiatedEventId = builder.initiatedEventId;
this.decisionTaskCompletedEventId = builder.decisionTaskCompletedEventId;
this.control = builder.control;
}
/**
*
* The workflow type provided in the StartChildWorkflowExecution
Decision that failed.
*
*
* @return The workflow type provided in the StartChildWorkflowExecution
Decision that failed.
*/
public final WorkflowType workflowType() {
return workflowType;
}
/**
*
* The cause of the failure. This information is generated by the system and can be useful for diagnostic purposes.
*
*
*
* When cause
is set to OPERATION_NOT_PERMITTED
, the decision fails because it lacks
* sufficient permissions. For details and example IAM policies, see Using IAM to Manage Access
* to Amazon SWF Workflows in the Amazon SWF Developer Guide.
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #cause} will return
* {@link StartChildWorkflowExecutionFailedCause#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #causeAsString}.
*
*
* @return The cause of the failure. This information is generated by the system and can be useful for diagnostic
* purposes.
*
* When cause
is set to OPERATION_NOT_PERMITTED
, the decision fails because it
* lacks sufficient permissions. For details and example IAM policies, see Using IAM to Manage
* Access to Amazon SWF Workflows in the Amazon SWF Developer Guide.
*
* @see StartChildWorkflowExecutionFailedCause
*/
public final StartChildWorkflowExecutionFailedCause cause() {
return StartChildWorkflowExecutionFailedCause.fromValue(causeValue);
}
/**
*
* The cause of the failure. This information is generated by the system and can be useful for diagnostic purposes.
*
*
*
* When cause
is set to OPERATION_NOT_PERMITTED
, the decision fails because it lacks
* sufficient permissions. For details and example IAM policies, see Using IAM to Manage Access
* to Amazon SWF Workflows in the Amazon SWF Developer Guide.
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #cause} will return
* {@link StartChildWorkflowExecutionFailedCause#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #causeAsString}.
*
*
* @return The cause of the failure. This information is generated by the system and can be useful for diagnostic
* purposes.
*
* When cause
is set to OPERATION_NOT_PERMITTED
, the decision fails because it
* lacks sufficient permissions. For details and example IAM policies, see Using IAM to Manage
* Access to Amazon SWF Workflows in the Amazon SWF Developer Guide.
*
* @see StartChildWorkflowExecutionFailedCause
*/
public final String causeAsString() {
return causeValue;
}
/**
*
* The workflowId
of the child workflow execution.
*
*
* @return The workflowId
of the child workflow execution.
*/
public final String workflowId() {
return workflowId;
}
/**
*
* When the cause
is WORKFLOW_ALREADY_RUNNING
, initiatedEventId
is the ID of
* the StartChildWorkflowExecutionInitiated
event that corresponds to the
* StartChildWorkflowExecution
Decision to start the workflow execution. You can use this
* information to diagnose problems by tracing back the chain of events leading up to this event.
*
*
* When the cause
isn't WORKFLOW_ALREADY_RUNNING
, initiatedEventId
is set to
* 0
because the StartChildWorkflowExecutionInitiated
event doesn't exist.
*
*
* @return When the cause
is WORKFLOW_ALREADY_RUNNING
, initiatedEventId
is
* the ID of the StartChildWorkflowExecutionInitiated
event that corresponds to the
* StartChildWorkflowExecution
Decision to start the workflow execution. You can use
* this information to diagnose problems by tracing back the chain of events leading up to this event.
*
* When the cause
isn't WORKFLOW_ALREADY_RUNNING
, initiatedEventId
is
* set to 0
because the StartChildWorkflowExecutionInitiated
event doesn't exist.
*/
public final Long initiatedEventId() {
return initiatedEventId;
}
/**
*
* The ID of the DecisionTaskCompleted
event corresponding to the decision task that resulted in the
* StartChildWorkflowExecution
Decision to request this child workflow execution. This
* information can be useful for diagnosing problems by tracing back the chain of events.
*
*
* @return The ID of the DecisionTaskCompleted
event corresponding to the decision task that resulted
* in the StartChildWorkflowExecution
Decision to request this child workflow execution.
* This information can be useful for diagnosing problems by tracing back the chain of events.
*/
public final Long decisionTaskCompletedEventId() {
return decisionTaskCompletedEventId;
}
/**
*
* The data attached to the event that the decider can use in subsequent workflow tasks. This data isn't sent to the
* child workflow execution.
*
*
* @return The data attached to the event that the decider can use in subsequent workflow tasks. This data isn't
* sent to the child workflow execution.
*/
public final String control() {
return control;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(workflowType());
hashCode = 31 * hashCode + Objects.hashCode(causeAsString());
hashCode = 31 * hashCode + Objects.hashCode(workflowId());
hashCode = 31 * hashCode + Objects.hashCode(initiatedEventId());
hashCode = 31 * hashCode + Objects.hashCode(decisionTaskCompletedEventId());
hashCode = 31 * hashCode + Objects.hashCode(control());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof StartChildWorkflowExecutionFailedEventAttributes)) {
return false;
}
StartChildWorkflowExecutionFailedEventAttributes other = (StartChildWorkflowExecutionFailedEventAttributes) obj;
return Objects.equals(workflowType(), other.workflowType()) && Objects.equals(causeAsString(), other.causeAsString())
&& Objects.equals(workflowId(), other.workflowId())
&& Objects.equals(initiatedEventId(), other.initiatedEventId())
&& Objects.equals(decisionTaskCompletedEventId(), other.decisionTaskCompletedEventId())
&& Objects.equals(control(), other.control());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("StartChildWorkflowExecutionFailedEventAttributes").add("WorkflowType", workflowType())
.add("Cause", causeAsString()).add("WorkflowId", workflowId()).add("InitiatedEventId", initiatedEventId())
.add("DecisionTaskCompletedEventId", decisionTaskCompletedEventId()).add("Control", control()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "workflowType":
return Optional.ofNullable(clazz.cast(workflowType()));
case "cause":
return Optional.ofNullable(clazz.cast(causeAsString()));
case "workflowId":
return Optional.ofNullable(clazz.cast(workflowId()));
case "initiatedEventId":
return Optional.ofNullable(clazz.cast(initiatedEventId()));
case "decisionTaskCompletedEventId":
return Optional.ofNullable(clazz.cast(decisionTaskCompletedEventId()));
case "control":
return Optional.ofNullable(clazz.cast(control()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function