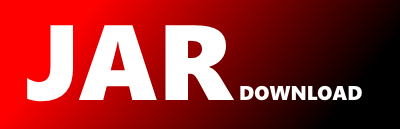
software.amazon.awssdk.services.swf.model.DecisionTaskScheduledEventAttributes Maven / Gradle / Ivy
Show all versions of swf Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.swf.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Provides details about the DecisionTaskScheduled
event.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class DecisionTaskScheduledEventAttributes implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField TASK_LIST_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("taskList").getter(getter(DecisionTaskScheduledEventAttributes::taskList))
.setter(setter(Builder::taskList)).constructor(TaskList::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("taskList").build()).build();
private static final SdkField TASK_PRIORITY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("taskPriority").getter(getter(DecisionTaskScheduledEventAttributes::taskPriority))
.setter(setter(Builder::taskPriority))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("taskPriority").build()).build();
private static final SdkField START_TO_CLOSE_TIMEOUT_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("startToCloseTimeout").getter(getter(DecisionTaskScheduledEventAttributes::startToCloseTimeout))
.setter(setter(Builder::startToCloseTimeout))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("startToCloseTimeout").build())
.build();
private static final SdkField SCHEDULE_TO_START_TIMEOUT_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("scheduleToStartTimeout").getter(getter(DecisionTaskScheduledEventAttributes::scheduleToStartTimeout))
.setter(setter(Builder::scheduleToStartTimeout))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("scheduleToStartTimeout").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(TASK_LIST_FIELD,
TASK_PRIORITY_FIELD, START_TO_CLOSE_TIMEOUT_FIELD, SCHEDULE_TO_START_TIMEOUT_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("taskList", TASK_LIST_FIELD);
put("taskPriority", TASK_PRIORITY_FIELD);
put("startToCloseTimeout", START_TO_CLOSE_TIMEOUT_FIELD);
put("scheduleToStartTimeout", SCHEDULE_TO_START_TIMEOUT_FIELD);
}
});
private static final long serialVersionUID = 1L;
private final TaskList taskList;
private final String taskPriority;
private final String startToCloseTimeout;
private final String scheduleToStartTimeout;
private DecisionTaskScheduledEventAttributes(BuilderImpl builder) {
this.taskList = builder.taskList;
this.taskPriority = builder.taskPriority;
this.startToCloseTimeout = builder.startToCloseTimeout;
this.scheduleToStartTimeout = builder.scheduleToStartTimeout;
}
/**
*
* The name of the task list in which the decision task was scheduled.
*
*
* @return The name of the task list in which the decision task was scheduled.
*/
public final TaskList taskList() {
return taskList;
}
/**
*
* A task priority that, if set, specifies the priority for this decision task. Valid values are integers that range
* from Java's Integer.MIN_VALUE
(-2147483648) to Integer.MAX_VALUE
(2147483647). Higher
* numbers indicate higher priority.
*
*
* For more information about setting task priority, see Setting Task
* Priority in the Amazon SWF Developer Guide.
*
*
* @return A task priority that, if set, specifies the priority for this decision task. Valid values are integers
* that range from Java's Integer.MIN_VALUE
(-2147483648) to Integer.MAX_VALUE
* (2147483647). Higher numbers indicate higher priority.
*
* For more information about setting task priority, see Setting Task
* Priority in the Amazon SWF Developer Guide.
*/
public final String taskPriority() {
return taskPriority;
}
/**
*
* The maximum duration for this decision task. The task is considered timed out if it doesn't completed within this
* duration.
*
*
* The duration is specified in seconds, an integer greater than or equal to 0
. You can use
* NONE
to specify unlimited duration.
*
*
* @return The maximum duration for this decision task. The task is considered timed out if it doesn't completed
* within this duration.
*
* The duration is specified in seconds, an integer greater than or equal to 0
. You can use
* NONE
to specify unlimited duration.
*/
public final String startToCloseTimeout() {
return startToCloseTimeout;
}
/**
*
* The maximum amount of time the decision task can wait to be assigned to a worker.
*
*
* @return The maximum amount of time the decision task can wait to be assigned to a worker.
*/
public final String scheduleToStartTimeout() {
return scheduleToStartTimeout;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(taskList());
hashCode = 31 * hashCode + Objects.hashCode(taskPriority());
hashCode = 31 * hashCode + Objects.hashCode(startToCloseTimeout());
hashCode = 31 * hashCode + Objects.hashCode(scheduleToStartTimeout());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof DecisionTaskScheduledEventAttributes)) {
return false;
}
DecisionTaskScheduledEventAttributes other = (DecisionTaskScheduledEventAttributes) obj;
return Objects.equals(taskList(), other.taskList()) && Objects.equals(taskPriority(), other.taskPriority())
&& Objects.equals(startToCloseTimeout(), other.startToCloseTimeout())
&& Objects.equals(scheduleToStartTimeout(), other.scheduleToStartTimeout());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("DecisionTaskScheduledEventAttributes").add("TaskList", taskList())
.add("TaskPriority", taskPriority()).add("StartToCloseTimeout", startToCloseTimeout())
.add("ScheduleToStartTimeout", scheduleToStartTimeout()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "taskList":
return Optional.ofNullable(clazz.cast(taskList()));
case "taskPriority":
return Optional.ofNullable(clazz.cast(taskPriority()));
case "startToCloseTimeout":
return Optional.ofNullable(clazz.cast(startToCloseTimeout()));
case "scheduleToStartTimeout":
return Optional.ofNullable(clazz.cast(scheduleToStartTimeout()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function