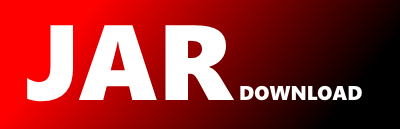
software.amazon.awssdk.services.swf.model.StartWorkflowExecutionRequest Maven / Gradle / Ivy
Show all versions of swf Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.swf.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class StartWorkflowExecutionRequest extends SwfRequest implements
ToCopyableBuilder {
private static final SdkField DOMAIN_FIELD = SdkField. builder(MarshallingType.STRING).memberName("domain")
.getter(getter(StartWorkflowExecutionRequest::domain)).setter(setter(Builder::domain))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("domain").build()).build();
private static final SdkField WORKFLOW_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("workflowId").getter(getter(StartWorkflowExecutionRequest::workflowId))
.setter(setter(Builder::workflowId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("workflowId").build()).build();
private static final SdkField WORKFLOW_TYPE_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("workflowType").getter(getter(StartWorkflowExecutionRequest::workflowType))
.setter(setter(Builder::workflowType)).constructor(WorkflowType::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("workflowType").build()).build();
private static final SdkField TASK_LIST_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("taskList").getter(getter(StartWorkflowExecutionRequest::taskList)).setter(setter(Builder::taskList))
.constructor(TaskList::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("taskList").build()).build();
private static final SdkField TASK_PRIORITY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("taskPriority").getter(getter(StartWorkflowExecutionRequest::taskPriority))
.setter(setter(Builder::taskPriority))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("taskPriority").build()).build();
private static final SdkField INPUT_FIELD = SdkField. builder(MarshallingType.STRING).memberName("input")
.getter(getter(StartWorkflowExecutionRequest::input)).setter(setter(Builder::input))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("input").build()).build();
private static final SdkField EXECUTION_START_TO_CLOSE_TIMEOUT_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("executionStartToCloseTimeout")
.getter(getter(StartWorkflowExecutionRequest::executionStartToCloseTimeout))
.setter(setter(Builder::executionStartToCloseTimeout))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("executionStartToCloseTimeout")
.build()).build();
private static final SdkField> TAG_LIST_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("tagList")
.getter(getter(StartWorkflowExecutionRequest::tagList))
.setter(setter(Builder::tagList))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("tagList").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField TASK_START_TO_CLOSE_TIMEOUT_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("taskStartToCloseTimeout").getter(getter(StartWorkflowExecutionRequest::taskStartToCloseTimeout))
.setter(setter(Builder::taskStartToCloseTimeout))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("taskStartToCloseTimeout").build())
.build();
private static final SdkField CHILD_POLICY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("childPolicy").getter(getter(StartWorkflowExecutionRequest::childPolicyAsString))
.setter(setter(Builder::childPolicy))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("childPolicy").build()).build();
private static final SdkField LAMBDA_ROLE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("lambdaRole").getter(getter(StartWorkflowExecutionRequest::lambdaRole))
.setter(setter(Builder::lambdaRole))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("lambdaRole").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(DOMAIN_FIELD,
WORKFLOW_ID_FIELD, WORKFLOW_TYPE_FIELD, TASK_LIST_FIELD, TASK_PRIORITY_FIELD, INPUT_FIELD,
EXECUTION_START_TO_CLOSE_TIMEOUT_FIELD, TAG_LIST_FIELD, TASK_START_TO_CLOSE_TIMEOUT_FIELD, CHILD_POLICY_FIELD,
LAMBDA_ROLE_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("domain", DOMAIN_FIELD);
put("workflowId", WORKFLOW_ID_FIELD);
put("workflowType", WORKFLOW_TYPE_FIELD);
put("taskList", TASK_LIST_FIELD);
put("taskPriority", TASK_PRIORITY_FIELD);
put("input", INPUT_FIELD);
put("executionStartToCloseTimeout", EXECUTION_START_TO_CLOSE_TIMEOUT_FIELD);
put("tagList", TAG_LIST_FIELD);
put("taskStartToCloseTimeout", TASK_START_TO_CLOSE_TIMEOUT_FIELD);
put("childPolicy", CHILD_POLICY_FIELD);
put("lambdaRole", LAMBDA_ROLE_FIELD);
}
});
private final String domain;
private final String workflowId;
private final WorkflowType workflowType;
private final TaskList taskList;
private final String taskPriority;
private final String input;
private final String executionStartToCloseTimeout;
private final List tagList;
private final String taskStartToCloseTimeout;
private final String childPolicy;
private final String lambdaRole;
private StartWorkflowExecutionRequest(BuilderImpl builder) {
super(builder);
this.domain = builder.domain;
this.workflowId = builder.workflowId;
this.workflowType = builder.workflowType;
this.taskList = builder.taskList;
this.taskPriority = builder.taskPriority;
this.input = builder.input;
this.executionStartToCloseTimeout = builder.executionStartToCloseTimeout;
this.tagList = builder.tagList;
this.taskStartToCloseTimeout = builder.taskStartToCloseTimeout;
this.childPolicy = builder.childPolicy;
this.lambdaRole = builder.lambdaRole;
}
/**
*
* The name of the domain in which the workflow execution is created.
*
*
* The specified string must not contain a :
(colon), /
(slash), |
(vertical
* bar), or any control characters (\u0000-\u001f
| \u007f-\u009f
). Also, it must
* not be the literal string arn
.
*
*
* @return The name of the domain in which the workflow execution is created.
*
* The specified string must not contain a :
(colon), /
(slash), |
* (vertical bar), or any control characters (\u0000-\u001f
| \u007f-\u009f
).
* Also, it must not be the literal string arn
.
*/
public final String domain() {
return domain;
}
/**
*
* The user defined identifier associated with the workflow execution. You can use this to associate a custom
* identifier with the workflow execution. You may specify the same identifier if a workflow execution is logically
* a restart of a previous execution. You cannot have two open workflow executions with the same
* workflowId
at the same time within the same domain.
*
*
* The specified string must not contain a :
(colon), /
(slash), |
(vertical
* bar), or any control characters (\u0000-\u001f
| \u007f-\u009f
). Also, it must
* not be the literal string arn
.
*
*
* @return The user defined identifier associated with the workflow execution. You can use this to associate a
* custom identifier with the workflow execution. You may specify the same identifier if a workflow
* execution is logically a restart of a previous execution. You cannot have two open workflow
* executions with the same workflowId
at the same time within the same domain.
*
* The specified string must not contain a :
(colon), /
(slash), |
* (vertical bar), or any control characters (\u0000-\u001f
| \u007f-\u009f
).
* Also, it must not be the literal string arn
.
*/
public final String workflowId() {
return workflowId;
}
/**
*
* The type of the workflow to start.
*
*
* @return The type of the workflow to start.
*/
public final WorkflowType workflowType() {
return workflowType;
}
/**
*
* The task list to use for the decision tasks generated for this workflow execution. This overrides the
* defaultTaskList
specified when registering the workflow type.
*
*
*
* A task list for this workflow execution must be specified either as a default for the workflow type or through
* this parameter. If neither this parameter is set nor a default task list was specified at registration time then
* a fault is returned.
*
*
*
* The specified string must not contain a :
(colon), /
(slash), |
(vertical
* bar), or any control characters (\u0000-\u001f
| \u007f-\u009f
). Also, it must
* not be the literal string arn
.
*
*
* @return The task list to use for the decision tasks generated for this workflow execution. This overrides the
* defaultTaskList
specified when registering the workflow type.
*
* A task list for this workflow execution must be specified either as a default for the workflow type or
* through this parameter. If neither this parameter is set nor a default task list was specified at
* registration time then a fault is returned.
*
*
*
* The specified string must not contain a :
(colon), /
(slash), |
* (vertical bar), or any control characters (\u0000-\u001f
| \u007f-\u009f
).
* Also, it must not be the literal string arn
.
*/
public final TaskList taskList() {
return taskList;
}
/**
*
* The task priority to use for this workflow execution. This overrides any default priority that was assigned when
* the workflow type was registered. If not set, then the default task priority for the workflow type is used. Valid
* values are integers that range from Java's Integer.MIN_VALUE
(-2147483648) to
* Integer.MAX_VALUE
(2147483647). Higher numbers indicate higher priority.
*
*
* For more information about setting task priority, see Setting Task
* Priority in the Amazon SWF Developer Guide.
*
*
* @return The task priority to use for this workflow execution. This overrides any default priority that was
* assigned when the workflow type was registered. If not set, then the default task priority for the
* workflow type is used. Valid values are integers that range from Java's Integer.MIN_VALUE
* (-2147483648) to Integer.MAX_VALUE
(2147483647). Higher numbers indicate higher
* priority.
*
* For more information about setting task priority, see Setting Task
* Priority in the Amazon SWF Developer Guide.
*/
public final String taskPriority() {
return taskPriority;
}
/**
*
* The input for the workflow execution. This is a free form string which should be meaningful to the workflow you
* are starting. This input
is made available to the new workflow execution in the
* WorkflowExecutionStarted
history event.
*
*
* @return The input for the workflow execution. This is a free form string which should be meaningful to the
* workflow you are starting. This input
is made available to the new workflow execution in the
* WorkflowExecutionStarted
history event.
*/
public final String input() {
return input;
}
/**
*
* The total duration for this workflow execution. This overrides the defaultExecutionStartToCloseTimeout specified
* when registering the workflow type.
*
*
* The duration is specified in seconds; an integer greater than or equal to 0
. Exceeding this limit
* causes the workflow execution to time out. Unlike some of the other timeout parameters in Amazon SWF, you cannot
* specify a value of "NONE" for this timeout; there is a one-year max limit on the time that a workflow execution
* can run.
*
*
*
* An execution start-to-close timeout must be specified either through this parameter or as a default when the
* workflow type is registered. If neither this parameter nor a default execution start-to-close timeout is
* specified, a fault is returned.
*
*
*
* @return The total duration for this workflow execution. This overrides the defaultExecutionStartToCloseTimeout
* specified when registering the workflow type.
*
* The duration is specified in seconds; an integer greater than or equal to 0
. Exceeding this
* limit causes the workflow execution to time out. Unlike some of the other timeout parameters in Amazon
* SWF, you cannot specify a value of "NONE" for this timeout; there is a one-year max limit on the time
* that a workflow execution can run.
*
*
*
* An execution start-to-close timeout must be specified either through this parameter or as a default when
* the workflow type is registered. If neither this parameter nor a default execution start-to-close timeout
* is specified, a fault is returned.
*
*/
public final String executionStartToCloseTimeout() {
return executionStartToCloseTimeout;
}
/**
* For responses, this returns true if the service returned a value for the TagList property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasTagList() {
return tagList != null && !(tagList instanceof SdkAutoConstructList);
}
/**
*
* The list of tags to associate with the workflow execution. You can specify a maximum of 5 tags. You can list
* workflow executions with a specific tag by calling ListOpenWorkflowExecutions or
* ListClosedWorkflowExecutions and specifying a TagFilter.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasTagList} method.
*
*
* @return The list of tags to associate with the workflow execution. You can specify a maximum of 5 tags. You can
* list workflow executions with a specific tag by calling ListOpenWorkflowExecutions or
* ListClosedWorkflowExecutions and specifying a TagFilter.
*/
public final List tagList() {
return tagList;
}
/**
*
* Specifies the maximum duration of decision tasks for this workflow execution. This parameter overrides the
* defaultTaskStartToCloseTimout
specified when registering the workflow type using
* RegisterWorkflowType.
*
*
* The duration is specified in seconds, an integer greater than or equal to 0
. You can use
* NONE
to specify unlimited duration.
*
*
*
* A task start-to-close timeout for this workflow execution must be specified either as a default for the workflow
* type or through this parameter. If neither this parameter is set nor a default task start-to-close timeout was
* specified at registration time then a fault is returned.
*
*
*
* @return Specifies the maximum duration of decision tasks for this workflow execution. This parameter overrides
* the defaultTaskStartToCloseTimout
specified when registering the workflow type using
* RegisterWorkflowType.
*
* The duration is specified in seconds, an integer greater than or equal to 0
. You can use
* NONE
to specify unlimited duration.
*
*
*
* A task start-to-close timeout for this workflow execution must be specified either as a default for the
* workflow type or through this parameter. If neither this parameter is set nor a default task
* start-to-close timeout was specified at registration time then a fault is returned.
*
*/
public final String taskStartToCloseTimeout() {
return taskStartToCloseTimeout;
}
/**
*
* If set, specifies the policy to use for the child workflow executions of this workflow execution if it is
* terminated, by calling the TerminateWorkflowExecution action explicitly or due to an expired timeout. This
* policy overrides the default child policy specified when registering the workflow type using
* RegisterWorkflowType.
*
*
* The supported child policies are:
*
*
* -
*
* TERMINATE
– The child executions are terminated.
*
*
* -
*
* REQUEST_CANCEL
– A request to cancel is attempted for each child execution by recording a
* WorkflowExecutionCancelRequested
event in its history. It is up to the decider to take appropriate
* actions when it receives an execution history with this event.
*
*
* -
*
* ABANDON
– No action is taken. The child executions continue to run.
*
*
*
*
*
* A child policy for this workflow execution must be specified either as a default for the workflow type or through
* this parameter. If neither this parameter is set nor a default child policy was specified at registration time
* then a fault is returned.
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #childPolicy} will
* return {@link ChildPolicy#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #childPolicyAsString}.
*
*
* @return If set, specifies the policy to use for the child workflow executions of this workflow execution if it is
* terminated, by calling the TerminateWorkflowExecution action explicitly or due to an expired
* timeout. This policy overrides the default child policy specified when registering the workflow type
* using RegisterWorkflowType.
*
* The supported child policies are:
*
*
* -
*
* TERMINATE
– The child executions are terminated.
*
*
* -
*
* REQUEST_CANCEL
– A request to cancel is attempted for each child execution by recording a
* WorkflowExecutionCancelRequested
event in its history. It is up to the decider to take
* appropriate actions when it receives an execution history with this event.
*
*
* -
*
* ABANDON
– No action is taken. The child executions continue to run.
*
*
*
*
*
* A child policy for this workflow execution must be specified either as a default for the workflow type or
* through this parameter. If neither this parameter is set nor a default child policy was specified at
* registration time then a fault is returned.
*
* @see ChildPolicy
*/
public final ChildPolicy childPolicy() {
return ChildPolicy.fromValue(childPolicy);
}
/**
*
* If set, specifies the policy to use for the child workflow executions of this workflow execution if it is
* terminated, by calling the TerminateWorkflowExecution action explicitly or due to an expired timeout. This
* policy overrides the default child policy specified when registering the workflow type using
* RegisterWorkflowType.
*
*
* The supported child policies are:
*
*
* -
*
* TERMINATE
– The child executions are terminated.
*
*
* -
*
* REQUEST_CANCEL
– A request to cancel is attempted for each child execution by recording a
* WorkflowExecutionCancelRequested
event in its history. It is up to the decider to take appropriate
* actions when it receives an execution history with this event.
*
*
* -
*
* ABANDON
– No action is taken. The child executions continue to run.
*
*
*
*
*
* A child policy for this workflow execution must be specified either as a default for the workflow type or through
* this parameter. If neither this parameter is set nor a default child policy was specified at registration time
* then a fault is returned.
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #childPolicy} will
* return {@link ChildPolicy#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #childPolicyAsString}.
*
*
* @return If set, specifies the policy to use for the child workflow executions of this workflow execution if it is
* terminated, by calling the TerminateWorkflowExecution action explicitly or due to an expired
* timeout. This policy overrides the default child policy specified when registering the workflow type
* using RegisterWorkflowType.
*
* The supported child policies are:
*
*
* -
*
* TERMINATE
– The child executions are terminated.
*
*
* -
*
* REQUEST_CANCEL
– A request to cancel is attempted for each child execution by recording a
* WorkflowExecutionCancelRequested
event in its history. It is up to the decider to take
* appropriate actions when it receives an execution history with this event.
*
*
* -
*
* ABANDON
– No action is taken. The child executions continue to run.
*
*
*
*
*
* A child policy for this workflow execution must be specified either as a default for the workflow type or
* through this parameter. If neither this parameter is set nor a default child policy was specified at
* registration time then a fault is returned.
*
* @see ChildPolicy
*/
public final String childPolicyAsString() {
return childPolicy;
}
/**
*
* The IAM role to attach to this workflow execution.
*
*
*
* Executions of this workflow type need IAM roles to invoke Lambda functions. If you don't attach an IAM role, any
* attempt to schedule a Lambda task fails. This results in a ScheduleLambdaFunctionFailed
history
* event. For more information, see https://docs.aws.amazon.com/amazonswf/latest/developerguide/lambda-task.html in the Amazon SWF Developer
* Guide.
*
*
*
* @return The IAM role to attach to this workflow execution.
*
* Executions of this workflow type need IAM roles to invoke Lambda functions. If you don't attach an IAM
* role, any attempt to schedule a Lambda task fails. This results in a
* ScheduleLambdaFunctionFailed
history event. For more information, see https://docs.aws.amazon.com/amazonswf/latest/developerguide/lambda-task.html in the Amazon SWF
* Developer Guide.
*
*/
public final String lambdaRole() {
return lambdaRole;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(domain());
hashCode = 31 * hashCode + Objects.hashCode(workflowId());
hashCode = 31 * hashCode + Objects.hashCode(workflowType());
hashCode = 31 * hashCode + Objects.hashCode(taskList());
hashCode = 31 * hashCode + Objects.hashCode(taskPriority());
hashCode = 31 * hashCode + Objects.hashCode(input());
hashCode = 31 * hashCode + Objects.hashCode(executionStartToCloseTimeout());
hashCode = 31 * hashCode + Objects.hashCode(hasTagList() ? tagList() : null);
hashCode = 31 * hashCode + Objects.hashCode(taskStartToCloseTimeout());
hashCode = 31 * hashCode + Objects.hashCode(childPolicyAsString());
hashCode = 31 * hashCode + Objects.hashCode(lambdaRole());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof StartWorkflowExecutionRequest)) {
return false;
}
StartWorkflowExecutionRequest other = (StartWorkflowExecutionRequest) obj;
return Objects.equals(domain(), other.domain()) && Objects.equals(workflowId(), other.workflowId())
&& Objects.equals(workflowType(), other.workflowType()) && Objects.equals(taskList(), other.taskList())
&& Objects.equals(taskPriority(), other.taskPriority()) && Objects.equals(input(), other.input())
&& Objects.equals(executionStartToCloseTimeout(), other.executionStartToCloseTimeout())
&& hasTagList() == other.hasTagList() && Objects.equals(tagList(), other.tagList())
&& Objects.equals(taskStartToCloseTimeout(), other.taskStartToCloseTimeout())
&& Objects.equals(childPolicyAsString(), other.childPolicyAsString())
&& Objects.equals(lambdaRole(), other.lambdaRole());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("StartWorkflowExecutionRequest").add("Domain", domain()).add("WorkflowId", workflowId())
.add("WorkflowType", workflowType()).add("TaskList", taskList()).add("TaskPriority", taskPriority())
.add("Input", input()).add("ExecutionStartToCloseTimeout", executionStartToCloseTimeout())
.add("TagList", hasTagList() ? tagList() : null).add("TaskStartToCloseTimeout", taskStartToCloseTimeout())
.add("ChildPolicy", childPolicyAsString()).add("LambdaRole", lambdaRole()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "domain":
return Optional.ofNullable(clazz.cast(domain()));
case "workflowId":
return Optional.ofNullable(clazz.cast(workflowId()));
case "workflowType":
return Optional.ofNullable(clazz.cast(workflowType()));
case "taskList":
return Optional.ofNullable(clazz.cast(taskList()));
case "taskPriority":
return Optional.ofNullable(clazz.cast(taskPriority()));
case "input":
return Optional.ofNullable(clazz.cast(input()));
case "executionStartToCloseTimeout":
return Optional.ofNullable(clazz.cast(executionStartToCloseTimeout()));
case "tagList":
return Optional.ofNullable(clazz.cast(tagList()));
case "taskStartToCloseTimeout":
return Optional.ofNullable(clazz.cast(taskStartToCloseTimeout()));
case "childPolicy":
return Optional.ofNullable(clazz.cast(childPolicyAsString()));
case "lambdaRole":
return Optional.ofNullable(clazz.cast(lambdaRole()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function