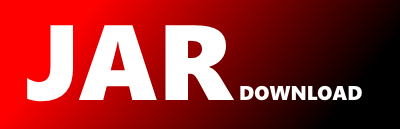
software.amazon.awssdk.services.timestreaminfluxdb.model.DeleteDbInstanceResponse Maven / Gradle / Ivy
Show all versions of timestreaminfluxdb Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.timestreaminfluxdb.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class DeleteDbInstanceResponse extends TimestreamInfluxDbResponse implements
ToCopyableBuilder {
private static final SdkField ID_FIELD = SdkField. builder(MarshallingType.STRING).memberName("id")
.getter(getter(DeleteDbInstanceResponse::id)).setter(setter(Builder::id))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("id").build()).build();
private static final SdkField NAME_FIELD = SdkField. builder(MarshallingType.STRING).memberName("name")
.getter(getter(DeleteDbInstanceResponse::name)).setter(setter(Builder::name))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("name").build()).build();
private static final SdkField ARN_FIELD = SdkField. builder(MarshallingType.STRING).memberName("arn")
.getter(getter(DeleteDbInstanceResponse::arn)).setter(setter(Builder::arn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("arn").build()).build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("status")
.getter(getter(DeleteDbInstanceResponse::statusAsString)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("status").build()).build();
private static final SdkField ENDPOINT_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("endpoint").getter(getter(DeleteDbInstanceResponse::endpoint)).setter(setter(Builder::endpoint))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("endpoint").build()).build();
private static final SdkField PORT_FIELD = SdkField. builder(MarshallingType.INTEGER).memberName("port")
.getter(getter(DeleteDbInstanceResponse::port)).setter(setter(Builder::port))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("port").build()).build();
private static final SdkField DB_INSTANCE_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("dbInstanceType").getter(getter(DeleteDbInstanceResponse::dbInstanceTypeAsString))
.setter(setter(Builder::dbInstanceType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("dbInstanceType").build()).build();
private static final SdkField DB_STORAGE_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("dbStorageType").getter(getter(DeleteDbInstanceResponse::dbStorageTypeAsString))
.setter(setter(Builder::dbStorageType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("dbStorageType").build()).build();
private static final SdkField ALLOCATED_STORAGE_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("allocatedStorage").getter(getter(DeleteDbInstanceResponse::allocatedStorage))
.setter(setter(Builder::allocatedStorage))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("allocatedStorage").build()).build();
private static final SdkField DEPLOYMENT_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("deploymentType").getter(getter(DeleteDbInstanceResponse::deploymentTypeAsString))
.setter(setter(Builder::deploymentType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("deploymentType").build()).build();
private static final SdkField> VPC_SUBNET_IDS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("vpcSubnetIds")
.getter(getter(DeleteDbInstanceResponse::vpcSubnetIds))
.setter(setter(Builder::vpcSubnetIds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("vpcSubnetIds").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField PUBLICLY_ACCESSIBLE_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("publiclyAccessible").getter(getter(DeleteDbInstanceResponse::publiclyAccessible))
.setter(setter(Builder::publiclyAccessible))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("publiclyAccessible").build())
.build();
private static final SdkField> VPC_SECURITY_GROUP_IDS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("vpcSecurityGroupIds")
.getter(getter(DeleteDbInstanceResponse::vpcSecurityGroupIds))
.setter(setter(Builder::vpcSecurityGroupIds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("vpcSecurityGroupIds").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField DB_PARAMETER_GROUP_IDENTIFIER_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("dbParameterGroupIdentifier")
.getter(getter(DeleteDbInstanceResponse::dbParameterGroupIdentifier))
.setter(setter(Builder::dbParameterGroupIdentifier))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("dbParameterGroupIdentifier").build())
.build();
private static final SdkField AVAILABILITY_ZONE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("availabilityZone").getter(getter(DeleteDbInstanceResponse::availabilityZone))
.setter(setter(Builder::availabilityZone))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("availabilityZone").build()).build();
private static final SdkField SECONDARY_AVAILABILITY_ZONE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("secondaryAvailabilityZone").getter(getter(DeleteDbInstanceResponse::secondaryAvailabilityZone))
.setter(setter(Builder::secondaryAvailabilityZone))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("secondaryAvailabilityZone").build())
.build();
private static final SdkField LOG_DELIVERY_CONFIGURATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("logDeliveryConfiguration")
.getter(getter(DeleteDbInstanceResponse::logDeliveryConfiguration)).setter(setter(Builder::logDeliveryConfiguration))
.constructor(LogDeliveryConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("logDeliveryConfiguration").build())
.build();
private static final SdkField INFLUX_AUTH_PARAMETERS_SECRET_ARN_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("influxAuthParametersSecretArn")
.getter(getter(DeleteDbInstanceResponse::influxAuthParametersSecretArn))
.setter(setter(Builder::influxAuthParametersSecretArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("influxAuthParametersSecretArn")
.build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ID_FIELD, NAME_FIELD,
ARN_FIELD, STATUS_FIELD, ENDPOINT_FIELD, PORT_FIELD, DB_INSTANCE_TYPE_FIELD, DB_STORAGE_TYPE_FIELD,
ALLOCATED_STORAGE_FIELD, DEPLOYMENT_TYPE_FIELD, VPC_SUBNET_IDS_FIELD, PUBLICLY_ACCESSIBLE_FIELD,
VPC_SECURITY_GROUP_IDS_FIELD, DB_PARAMETER_GROUP_IDENTIFIER_FIELD, AVAILABILITY_ZONE_FIELD,
SECONDARY_AVAILABILITY_ZONE_FIELD, LOG_DELIVERY_CONFIGURATION_FIELD, INFLUX_AUTH_PARAMETERS_SECRET_ARN_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("id", ID_FIELD);
put("name", NAME_FIELD);
put("arn", ARN_FIELD);
put("status", STATUS_FIELD);
put("endpoint", ENDPOINT_FIELD);
put("port", PORT_FIELD);
put("dbInstanceType", DB_INSTANCE_TYPE_FIELD);
put("dbStorageType", DB_STORAGE_TYPE_FIELD);
put("allocatedStorage", ALLOCATED_STORAGE_FIELD);
put("deploymentType", DEPLOYMENT_TYPE_FIELD);
put("vpcSubnetIds", VPC_SUBNET_IDS_FIELD);
put("publiclyAccessible", PUBLICLY_ACCESSIBLE_FIELD);
put("vpcSecurityGroupIds", VPC_SECURITY_GROUP_IDS_FIELD);
put("dbParameterGroupIdentifier", DB_PARAMETER_GROUP_IDENTIFIER_FIELD);
put("availabilityZone", AVAILABILITY_ZONE_FIELD);
put("secondaryAvailabilityZone", SECONDARY_AVAILABILITY_ZONE_FIELD);
put("logDeliveryConfiguration", LOG_DELIVERY_CONFIGURATION_FIELD);
put("influxAuthParametersSecretArn", INFLUX_AUTH_PARAMETERS_SECRET_ARN_FIELD);
}
});
private final String id;
private final String name;
private final String arn;
private final String status;
private final String endpoint;
private final Integer port;
private final String dbInstanceType;
private final String dbStorageType;
private final Integer allocatedStorage;
private final String deploymentType;
private final List vpcSubnetIds;
private final Boolean publiclyAccessible;
private final List vpcSecurityGroupIds;
private final String dbParameterGroupIdentifier;
private final String availabilityZone;
private final String secondaryAvailabilityZone;
private final LogDeliveryConfiguration logDeliveryConfiguration;
private final String influxAuthParametersSecretArn;
private DeleteDbInstanceResponse(BuilderImpl builder) {
super(builder);
this.id = builder.id;
this.name = builder.name;
this.arn = builder.arn;
this.status = builder.status;
this.endpoint = builder.endpoint;
this.port = builder.port;
this.dbInstanceType = builder.dbInstanceType;
this.dbStorageType = builder.dbStorageType;
this.allocatedStorage = builder.allocatedStorage;
this.deploymentType = builder.deploymentType;
this.vpcSubnetIds = builder.vpcSubnetIds;
this.publiclyAccessible = builder.publiclyAccessible;
this.vpcSecurityGroupIds = builder.vpcSecurityGroupIds;
this.dbParameterGroupIdentifier = builder.dbParameterGroupIdentifier;
this.availabilityZone = builder.availabilityZone;
this.secondaryAvailabilityZone = builder.secondaryAvailabilityZone;
this.logDeliveryConfiguration = builder.logDeliveryConfiguration;
this.influxAuthParametersSecretArn = builder.influxAuthParametersSecretArn;
}
/**
*
* A service-generated unique identifier.
*
*
* @return A service-generated unique identifier.
*/
public final String id() {
return id;
}
/**
*
* The customer-supplied name that uniquely identifies the DB instance when interacting with the Amazon Timestream
* for InfluxDB API and CLI commands.
*
*
* @return The customer-supplied name that uniquely identifies the DB instance when interacting with the Amazon
* Timestream for InfluxDB API and CLI commands.
*/
public final String name() {
return name;
}
/**
*
* The Amazon Resource Name (ARN) of the DB instance.
*
*
* @return The Amazon Resource Name (ARN) of the DB instance.
*/
public final String arn() {
return arn;
}
/**
*
* The status of the DB instance.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link Status#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statusAsString}.
*
*
* @return The status of the DB instance.
* @see Status
*/
public final Status status() {
return Status.fromValue(status);
}
/**
*
* The status of the DB instance.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link Status#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statusAsString}.
*
*
* @return The status of the DB instance.
* @see Status
*/
public final String statusAsString() {
return status;
}
/**
*
* The endpoint used to connect to InfluxDB. The default InfluxDB port is 8086.
*
*
* @return The endpoint used to connect to InfluxDB. The default InfluxDB port is 8086.
*/
public final String endpoint() {
return endpoint;
}
/**
*
* The port number on which InfluxDB accepts connections.
*
*
* @return The port number on which InfluxDB accepts connections.
*/
public final Integer port() {
return port;
}
/**
*
* The Timestream for InfluxDB instance type that InfluxDB runs on.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #dbInstanceType}
* will return {@link DbInstanceType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #dbInstanceTypeAsString}.
*
*
* @return The Timestream for InfluxDB instance type that InfluxDB runs on.
* @see DbInstanceType
*/
public final DbInstanceType dbInstanceType() {
return DbInstanceType.fromValue(dbInstanceType);
}
/**
*
* The Timestream for InfluxDB instance type that InfluxDB runs on.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #dbInstanceType}
* will return {@link DbInstanceType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #dbInstanceTypeAsString}.
*
*
* @return The Timestream for InfluxDB instance type that InfluxDB runs on.
* @see DbInstanceType
*/
public final String dbInstanceTypeAsString() {
return dbInstanceType;
}
/**
*
* The Timestream for InfluxDB DB storage type that InfluxDB stores data on.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #dbStorageType}
* will return {@link DbStorageType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #dbStorageTypeAsString}.
*
*
* @return The Timestream for InfluxDB DB storage type that InfluxDB stores data on.
* @see DbStorageType
*/
public final DbStorageType dbStorageType() {
return DbStorageType.fromValue(dbStorageType);
}
/**
*
* The Timestream for InfluxDB DB storage type that InfluxDB stores data on.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #dbStorageType}
* will return {@link DbStorageType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #dbStorageTypeAsString}.
*
*
* @return The Timestream for InfluxDB DB storage type that InfluxDB stores data on.
* @see DbStorageType
*/
public final String dbStorageTypeAsString() {
return dbStorageType;
}
/**
*
* The amount of storage allocated for your DB storage type (in gibibytes).
*
*
* @return The amount of storage allocated for your DB storage type (in gibibytes).
*/
public final Integer allocatedStorage() {
return allocatedStorage;
}
/**
*
* Specifies whether the Timestream for InfluxDB is deployed as Single-AZ or with a MultiAZ Standby for High
* availability.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #deploymentType}
* will return {@link DeploymentType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #deploymentTypeAsString}.
*
*
* @return Specifies whether the Timestream for InfluxDB is deployed as Single-AZ or with a MultiAZ Standby for High
* availability.
* @see DeploymentType
*/
public final DeploymentType deploymentType() {
return DeploymentType.fromValue(deploymentType);
}
/**
*
* Specifies whether the Timestream for InfluxDB is deployed as Single-AZ or with a MultiAZ Standby for High
* availability.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #deploymentType}
* will return {@link DeploymentType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #deploymentTypeAsString}.
*
*
* @return Specifies whether the Timestream for InfluxDB is deployed as Single-AZ or with a MultiAZ Standby for High
* availability.
* @see DeploymentType
*/
public final String deploymentTypeAsString() {
return deploymentType;
}
/**
* For responses, this returns true if the service returned a value for the VpcSubnetIds property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasVpcSubnetIds() {
return vpcSubnetIds != null && !(vpcSubnetIds instanceof SdkAutoConstructList);
}
/**
*
* A list of VPC subnet IDs associated with the DB instance.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasVpcSubnetIds} method.
*
*
* @return A list of VPC subnet IDs associated with the DB instance.
*/
public final List vpcSubnetIds() {
return vpcSubnetIds;
}
/**
*
* Indicates if the DB instance has a public IP to facilitate access.
*
*
* @return Indicates if the DB instance has a public IP to facilitate access.
*/
public final Boolean publiclyAccessible() {
return publiclyAccessible;
}
/**
* For responses, this returns true if the service returned a value for the VpcSecurityGroupIds property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasVpcSecurityGroupIds() {
return vpcSecurityGroupIds != null && !(vpcSecurityGroupIds instanceof SdkAutoConstructList);
}
/**
*
* A list of VPC security group IDs associated with the DB instance.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasVpcSecurityGroupIds} method.
*
*
* @return A list of VPC security group IDs associated with the DB instance.
*/
public final List vpcSecurityGroupIds() {
return vpcSecurityGroupIds;
}
/**
*
* The id of the DB parameter group assigned to your DB instance.
*
*
* @return The id of the DB parameter group assigned to your DB instance.
*/
public final String dbParameterGroupIdentifier() {
return dbParameterGroupIdentifier;
}
/**
*
* The Availability Zone in which the DB instance resides.
*
*
* @return The Availability Zone in which the DB instance resides.
*/
public final String availabilityZone() {
return availabilityZone;
}
/**
*
* The Availability Zone in which the standby instance is located when deploying with a MultiAZ standby instance.
*
*
* @return The Availability Zone in which the standby instance is located when deploying with a MultiAZ standby
* instance.
*/
public final String secondaryAvailabilityZone() {
return secondaryAvailabilityZone;
}
/**
*
* Configuration for sending InfluxDB engine logs to send to specified S3 bucket.
*
*
* @return Configuration for sending InfluxDB engine logs to send to specified S3 bucket.
*/
public final LogDeliveryConfiguration logDeliveryConfiguration() {
return logDeliveryConfiguration;
}
/**
*
* The Amazon Resource Name (ARN) of the AWS Secrets Manager secret containing the initial InfluxDB authorization
* parameters. The secret value is a JSON formatted key-value pair holding InfluxDB authorization values:
* organization, bucket, username, and password.
*
*
* @return The Amazon Resource Name (ARN) of the AWS Secrets Manager secret containing the initial InfluxDB
* authorization parameters. The secret value is a JSON formatted key-value pair holding InfluxDB
* authorization values: organization, bucket, username, and password.
*/
public final String influxAuthParametersSecretArn() {
return influxAuthParametersSecretArn;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(id());
hashCode = 31 * hashCode + Objects.hashCode(name());
hashCode = 31 * hashCode + Objects.hashCode(arn());
hashCode = 31 * hashCode + Objects.hashCode(statusAsString());
hashCode = 31 * hashCode + Objects.hashCode(endpoint());
hashCode = 31 * hashCode + Objects.hashCode(port());
hashCode = 31 * hashCode + Objects.hashCode(dbInstanceTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(dbStorageTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(allocatedStorage());
hashCode = 31 * hashCode + Objects.hashCode(deploymentTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(hasVpcSubnetIds() ? vpcSubnetIds() : null);
hashCode = 31 * hashCode + Objects.hashCode(publiclyAccessible());
hashCode = 31 * hashCode + Objects.hashCode(hasVpcSecurityGroupIds() ? vpcSecurityGroupIds() : null);
hashCode = 31 * hashCode + Objects.hashCode(dbParameterGroupIdentifier());
hashCode = 31 * hashCode + Objects.hashCode(availabilityZone());
hashCode = 31 * hashCode + Objects.hashCode(secondaryAvailabilityZone());
hashCode = 31 * hashCode + Objects.hashCode(logDeliveryConfiguration());
hashCode = 31 * hashCode + Objects.hashCode(influxAuthParametersSecretArn());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof DeleteDbInstanceResponse)) {
return false;
}
DeleteDbInstanceResponse other = (DeleteDbInstanceResponse) obj;
return Objects.equals(id(), other.id()) && Objects.equals(name(), other.name()) && Objects.equals(arn(), other.arn())
&& Objects.equals(statusAsString(), other.statusAsString()) && Objects.equals(endpoint(), other.endpoint())
&& Objects.equals(port(), other.port())
&& Objects.equals(dbInstanceTypeAsString(), other.dbInstanceTypeAsString())
&& Objects.equals(dbStorageTypeAsString(), other.dbStorageTypeAsString())
&& Objects.equals(allocatedStorage(), other.allocatedStorage())
&& Objects.equals(deploymentTypeAsString(), other.deploymentTypeAsString())
&& hasVpcSubnetIds() == other.hasVpcSubnetIds() && Objects.equals(vpcSubnetIds(), other.vpcSubnetIds())
&& Objects.equals(publiclyAccessible(), other.publiclyAccessible())
&& hasVpcSecurityGroupIds() == other.hasVpcSecurityGroupIds()
&& Objects.equals(vpcSecurityGroupIds(), other.vpcSecurityGroupIds())
&& Objects.equals(dbParameterGroupIdentifier(), other.dbParameterGroupIdentifier())
&& Objects.equals(availabilityZone(), other.availabilityZone())
&& Objects.equals(secondaryAvailabilityZone(), other.secondaryAvailabilityZone())
&& Objects.equals(logDeliveryConfiguration(), other.logDeliveryConfiguration())
&& Objects.equals(influxAuthParametersSecretArn(), other.influxAuthParametersSecretArn());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("DeleteDbInstanceResponse").add("Id", id()).add("Name", name()).add("Arn", arn())
.add("Status", statusAsString()).add("Endpoint", endpoint()).add("Port", port())
.add("DbInstanceType", dbInstanceTypeAsString()).add("DbStorageType", dbStorageTypeAsString())
.add("AllocatedStorage", allocatedStorage()).add("DeploymentType", deploymentTypeAsString())
.add("VpcSubnetIds", hasVpcSubnetIds() ? vpcSubnetIds() : null).add("PubliclyAccessible", publiclyAccessible())
.add("VpcSecurityGroupIds", hasVpcSecurityGroupIds() ? vpcSecurityGroupIds() : null)
.add("DbParameterGroupIdentifier", dbParameterGroupIdentifier()).add("AvailabilityZone", availabilityZone())
.add("SecondaryAvailabilityZone", secondaryAvailabilityZone())
.add("LogDeliveryConfiguration", logDeliveryConfiguration())
.add("InfluxAuthParametersSecretArn", influxAuthParametersSecretArn()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "id":
return Optional.ofNullable(clazz.cast(id()));
case "name":
return Optional.ofNullable(clazz.cast(name()));
case "arn":
return Optional.ofNullable(clazz.cast(arn()));
case "status":
return Optional.ofNullable(clazz.cast(statusAsString()));
case "endpoint":
return Optional.ofNullable(clazz.cast(endpoint()));
case "port":
return Optional.ofNullable(clazz.cast(port()));
case "dbInstanceType":
return Optional.ofNullable(clazz.cast(dbInstanceTypeAsString()));
case "dbStorageType":
return Optional.ofNullable(clazz.cast(dbStorageTypeAsString()));
case "allocatedStorage":
return Optional.ofNullable(clazz.cast(allocatedStorage()));
case "deploymentType":
return Optional.ofNullable(clazz.cast(deploymentTypeAsString()));
case "vpcSubnetIds":
return Optional.ofNullable(clazz.cast(vpcSubnetIds()));
case "publiclyAccessible":
return Optional.ofNullable(clazz.cast(publiclyAccessible()));
case "vpcSecurityGroupIds":
return Optional.ofNullable(clazz.cast(vpcSecurityGroupIds()));
case "dbParameterGroupIdentifier":
return Optional.ofNullable(clazz.cast(dbParameterGroupIdentifier()));
case "availabilityZone":
return Optional.ofNullable(clazz.cast(availabilityZone()));
case "secondaryAvailabilityZone":
return Optional.ofNullable(clazz.cast(secondaryAvailabilityZone()));
case "logDeliveryConfiguration":
return Optional.ofNullable(clazz.cast(logDeliveryConfiguration()));
case "influxAuthParametersSecretArn":
return Optional.ofNullable(clazz.cast(influxAuthParametersSecretArn()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function