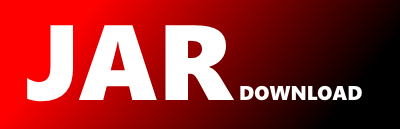
software.amazon.awssdk.services.tnb.TnbClient Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.tnb;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkPublicApi;
import software.amazon.awssdk.annotations.ThreadSafe;
import software.amazon.awssdk.awscore.AwsClient;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.core.exception.SdkClientException;
import software.amazon.awssdk.regions.ServiceMetadata;
import software.amazon.awssdk.services.tnb.model.AccessDeniedException;
import software.amazon.awssdk.services.tnb.model.CancelSolNetworkOperationRequest;
import software.amazon.awssdk.services.tnb.model.CancelSolNetworkOperationResponse;
import software.amazon.awssdk.services.tnb.model.CreateSolFunctionPackageRequest;
import software.amazon.awssdk.services.tnb.model.CreateSolFunctionPackageResponse;
import software.amazon.awssdk.services.tnb.model.CreateSolNetworkInstanceRequest;
import software.amazon.awssdk.services.tnb.model.CreateSolNetworkInstanceResponse;
import software.amazon.awssdk.services.tnb.model.CreateSolNetworkPackageRequest;
import software.amazon.awssdk.services.tnb.model.CreateSolNetworkPackageResponse;
import software.amazon.awssdk.services.tnb.model.DeleteSolFunctionPackageRequest;
import software.amazon.awssdk.services.tnb.model.DeleteSolFunctionPackageResponse;
import software.amazon.awssdk.services.tnb.model.DeleteSolNetworkInstanceRequest;
import software.amazon.awssdk.services.tnb.model.DeleteSolNetworkInstanceResponse;
import software.amazon.awssdk.services.tnb.model.DeleteSolNetworkPackageRequest;
import software.amazon.awssdk.services.tnb.model.DeleteSolNetworkPackageResponse;
import software.amazon.awssdk.services.tnb.model.GetSolFunctionInstanceRequest;
import software.amazon.awssdk.services.tnb.model.GetSolFunctionInstanceResponse;
import software.amazon.awssdk.services.tnb.model.GetSolFunctionPackageContentRequest;
import software.amazon.awssdk.services.tnb.model.GetSolFunctionPackageContentResponse;
import software.amazon.awssdk.services.tnb.model.GetSolFunctionPackageDescriptorRequest;
import software.amazon.awssdk.services.tnb.model.GetSolFunctionPackageDescriptorResponse;
import software.amazon.awssdk.services.tnb.model.GetSolFunctionPackageRequest;
import software.amazon.awssdk.services.tnb.model.GetSolFunctionPackageResponse;
import software.amazon.awssdk.services.tnb.model.GetSolNetworkInstanceRequest;
import software.amazon.awssdk.services.tnb.model.GetSolNetworkInstanceResponse;
import software.amazon.awssdk.services.tnb.model.GetSolNetworkOperationRequest;
import software.amazon.awssdk.services.tnb.model.GetSolNetworkOperationResponse;
import software.amazon.awssdk.services.tnb.model.GetSolNetworkPackageContentRequest;
import software.amazon.awssdk.services.tnb.model.GetSolNetworkPackageContentResponse;
import software.amazon.awssdk.services.tnb.model.GetSolNetworkPackageDescriptorRequest;
import software.amazon.awssdk.services.tnb.model.GetSolNetworkPackageDescriptorResponse;
import software.amazon.awssdk.services.tnb.model.GetSolNetworkPackageRequest;
import software.amazon.awssdk.services.tnb.model.GetSolNetworkPackageResponse;
import software.amazon.awssdk.services.tnb.model.InstantiateSolNetworkInstanceRequest;
import software.amazon.awssdk.services.tnb.model.InstantiateSolNetworkInstanceResponse;
import software.amazon.awssdk.services.tnb.model.InternalServerException;
import software.amazon.awssdk.services.tnb.model.ListSolFunctionInstancesRequest;
import software.amazon.awssdk.services.tnb.model.ListSolFunctionInstancesResponse;
import software.amazon.awssdk.services.tnb.model.ListSolFunctionPackagesRequest;
import software.amazon.awssdk.services.tnb.model.ListSolFunctionPackagesResponse;
import software.amazon.awssdk.services.tnb.model.ListSolNetworkInstancesRequest;
import software.amazon.awssdk.services.tnb.model.ListSolNetworkInstancesResponse;
import software.amazon.awssdk.services.tnb.model.ListSolNetworkOperationsRequest;
import software.amazon.awssdk.services.tnb.model.ListSolNetworkOperationsResponse;
import software.amazon.awssdk.services.tnb.model.ListSolNetworkPackagesRequest;
import software.amazon.awssdk.services.tnb.model.ListSolNetworkPackagesResponse;
import software.amazon.awssdk.services.tnb.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.tnb.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.tnb.model.PutSolFunctionPackageContentRequest;
import software.amazon.awssdk.services.tnb.model.PutSolFunctionPackageContentResponse;
import software.amazon.awssdk.services.tnb.model.PutSolNetworkPackageContentRequest;
import software.amazon.awssdk.services.tnb.model.PutSolNetworkPackageContentResponse;
import software.amazon.awssdk.services.tnb.model.ResourceNotFoundException;
import software.amazon.awssdk.services.tnb.model.ServiceQuotaExceededException;
import software.amazon.awssdk.services.tnb.model.TagResourceRequest;
import software.amazon.awssdk.services.tnb.model.TagResourceResponse;
import software.amazon.awssdk.services.tnb.model.TerminateSolNetworkInstanceRequest;
import software.amazon.awssdk.services.tnb.model.TerminateSolNetworkInstanceResponse;
import software.amazon.awssdk.services.tnb.model.ThrottlingException;
import software.amazon.awssdk.services.tnb.model.TnbException;
import software.amazon.awssdk.services.tnb.model.UntagResourceRequest;
import software.amazon.awssdk.services.tnb.model.UntagResourceResponse;
import software.amazon.awssdk.services.tnb.model.UpdateSolFunctionPackageRequest;
import software.amazon.awssdk.services.tnb.model.UpdateSolFunctionPackageResponse;
import software.amazon.awssdk.services.tnb.model.UpdateSolNetworkInstanceRequest;
import software.amazon.awssdk.services.tnb.model.UpdateSolNetworkInstanceResponse;
import software.amazon.awssdk.services.tnb.model.UpdateSolNetworkPackageRequest;
import software.amazon.awssdk.services.tnb.model.UpdateSolNetworkPackageResponse;
import software.amazon.awssdk.services.tnb.model.ValidateSolFunctionPackageContentRequest;
import software.amazon.awssdk.services.tnb.model.ValidateSolFunctionPackageContentResponse;
import software.amazon.awssdk.services.tnb.model.ValidateSolNetworkPackageContentRequest;
import software.amazon.awssdk.services.tnb.model.ValidateSolNetworkPackageContentResponse;
import software.amazon.awssdk.services.tnb.model.ValidationException;
import software.amazon.awssdk.services.tnb.paginators.ListSolFunctionInstancesIterable;
import software.amazon.awssdk.services.tnb.paginators.ListSolFunctionPackagesIterable;
import software.amazon.awssdk.services.tnb.paginators.ListSolNetworkInstancesIterable;
import software.amazon.awssdk.services.tnb.paginators.ListSolNetworkOperationsIterable;
import software.amazon.awssdk.services.tnb.paginators.ListSolNetworkPackagesIterable;
/**
* Service client for accessing AWS Telco Network Builder. This can be created using the static {@link #builder()}
* method.
*
*
* Amazon Web Services Telco Network Builder (TNB) is a network automation service that helps you deploy and manage
* telecom networks. AWS TNB helps you with the lifecycle management of your telecommunication network functions
* throughout planning, deployment, and post-deployment activities.
*
*/
@Generated("software.amazon.awssdk:codegen")
@SdkPublicApi
@ThreadSafe
public interface TnbClient extends AwsClient {
String SERVICE_NAME = "tnb";
/**
* Value for looking up the service's metadata from the
* {@link software.amazon.awssdk.regions.ServiceMetadataProvider}.
*/
String SERVICE_METADATA_ID = "tnb";
/**
*
* Cancels a network operation.
*
*
* A network operation is any operation that is done to your network, such as network instance instantiation or
* termination.
*
*
* @param cancelSolNetworkOperationRequest
* @return Result of the CancelSolNetworkOperation operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws ResourceNotFoundException
* Request references a resource that doesn't exist.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TnbException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TnbClient.CancelSolNetworkOperation
* @see AWS
* API Documentation
*/
default CancelSolNetworkOperationResponse cancelSolNetworkOperation(
CancelSolNetworkOperationRequest cancelSolNetworkOperationRequest) throws InternalServerException,
ThrottlingException, ValidationException, ResourceNotFoundException, AccessDeniedException, AwsServiceException,
SdkClientException, TnbException {
throw new UnsupportedOperationException();
}
/**
*
* Cancels a network operation.
*
*
* A network operation is any operation that is done to your network, such as network instance instantiation or
* termination.
*
*
*
* This is a convenience which creates an instance of the {@link CancelSolNetworkOperationRequest.Builder} avoiding
* the need to create one manually via {@link CancelSolNetworkOperationRequest#builder()}
*
*
* @param cancelSolNetworkOperationRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.tnb.model.CancelSolNetworkOperationRequest.Builder} to create a
* request.
* @return Result of the CancelSolNetworkOperation operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws ResourceNotFoundException
* Request references a resource that doesn't exist.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TnbException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TnbClient.CancelSolNetworkOperation
* @see AWS
* API Documentation
*/
default CancelSolNetworkOperationResponse cancelSolNetworkOperation(
Consumer cancelSolNetworkOperationRequest) throws InternalServerException,
ThrottlingException, ValidationException, ResourceNotFoundException, AccessDeniedException, AwsServiceException,
SdkClientException, TnbException {
return cancelSolNetworkOperation(CancelSolNetworkOperationRequest.builder()
.applyMutation(cancelSolNetworkOperationRequest).build());
}
/**
*
* Creates a function package.
*
*
* A function package is a .zip file in CSAR (Cloud Service Archive) format that contains a network function (an
* ETSI standard telecommunication application) and function package descriptor that uses the TOSCA standard to
* describe how the network functions should run on your network. For more information, see Function packages in the Amazon
* Web Services Telco Network Builder User Guide.
*
*
* Creating a function package is the first step for creating a network in AWS TNB. This request creates an empty
* container with an ID. The next step is to upload the actual CSAR zip file into that empty container. To upload
* function package content, see PutSolFunctionPackageContent.
*
*
* @param createSolFunctionPackageRequest
* @return Result of the CreateSolFunctionPackage operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ServiceQuotaExceededException
* Service quotas have been exceeded.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TnbException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TnbClient.CreateSolFunctionPackage
* @see AWS
* API Documentation
*/
default CreateSolFunctionPackageResponse createSolFunctionPackage(
CreateSolFunctionPackageRequest createSolFunctionPackageRequest) throws InternalServerException,
ServiceQuotaExceededException, ThrottlingException, ValidationException, AccessDeniedException, AwsServiceException,
SdkClientException, TnbException {
throw new UnsupportedOperationException();
}
/**
*
* Creates a function package.
*
*
* A function package is a .zip file in CSAR (Cloud Service Archive) format that contains a network function (an
* ETSI standard telecommunication application) and function package descriptor that uses the TOSCA standard to
* describe how the network functions should run on your network. For more information, see Function packages in the Amazon
* Web Services Telco Network Builder User Guide.
*
*
* Creating a function package is the first step for creating a network in AWS TNB. This request creates an empty
* container with an ID. The next step is to upload the actual CSAR zip file into that empty container. To upload
* function package content, see PutSolFunctionPackageContent.
*
*
*
* This is a convenience which creates an instance of the {@link CreateSolFunctionPackageRequest.Builder} avoiding
* the need to create one manually via {@link CreateSolFunctionPackageRequest#builder()}
*
*
* @param createSolFunctionPackageRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.tnb.model.CreateSolFunctionPackageRequest.Builder} to create a
* request.
* @return Result of the CreateSolFunctionPackage operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ServiceQuotaExceededException
* Service quotas have been exceeded.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TnbException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TnbClient.CreateSolFunctionPackage
* @see AWS
* API Documentation
*/
default CreateSolFunctionPackageResponse createSolFunctionPackage(
Consumer createSolFunctionPackageRequest) throws InternalServerException,
ServiceQuotaExceededException, ThrottlingException, ValidationException, AccessDeniedException, AwsServiceException,
SdkClientException, TnbException {
return createSolFunctionPackage(CreateSolFunctionPackageRequest.builder().applyMutation(createSolFunctionPackageRequest)
.build());
}
/**
*
* Creates a network instance.
*
*
* A network instance is a single network created in Amazon Web Services TNB that can be deployed and on which
* life-cycle operations (like terminate, update, and delete) can be performed. Creating a network instance is the
* third step after creating a network package. For more information about network instances, Network instances in the Amazon
* Web Services Telco Network Builder User Guide.
*
*
* Once you create a network instance, you can instantiate it. To instantiate a network, see InstantiateSolNetworkInstance.
*
*
* @param createSolNetworkInstanceRequest
* @return Result of the CreateSolNetworkInstance operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ServiceQuotaExceededException
* Service quotas have been exceeded.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws ResourceNotFoundException
* Request references a resource that doesn't exist.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TnbException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TnbClient.CreateSolNetworkInstance
* @see AWS
* API Documentation
*/
default CreateSolNetworkInstanceResponse createSolNetworkInstance(
CreateSolNetworkInstanceRequest createSolNetworkInstanceRequest) throws InternalServerException,
ServiceQuotaExceededException, ThrottlingException, ValidationException, ResourceNotFoundException,
AccessDeniedException, AwsServiceException, SdkClientException, TnbException {
throw new UnsupportedOperationException();
}
/**
*
* Creates a network instance.
*
*
* A network instance is a single network created in Amazon Web Services TNB that can be deployed and on which
* life-cycle operations (like terminate, update, and delete) can be performed. Creating a network instance is the
* third step after creating a network package. For more information about network instances, Network instances in the Amazon
* Web Services Telco Network Builder User Guide.
*
*
* Once you create a network instance, you can instantiate it. To instantiate a network, see InstantiateSolNetworkInstance.
*
*
*
* This is a convenience which creates an instance of the {@link CreateSolNetworkInstanceRequest.Builder} avoiding
* the need to create one manually via {@link CreateSolNetworkInstanceRequest#builder()}
*
*
* @param createSolNetworkInstanceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.tnb.model.CreateSolNetworkInstanceRequest.Builder} to create a
* request.
* @return Result of the CreateSolNetworkInstance operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ServiceQuotaExceededException
* Service quotas have been exceeded.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws ResourceNotFoundException
* Request references a resource that doesn't exist.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TnbException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TnbClient.CreateSolNetworkInstance
* @see AWS
* API Documentation
*/
default CreateSolNetworkInstanceResponse createSolNetworkInstance(
Consumer createSolNetworkInstanceRequest) throws InternalServerException,
ServiceQuotaExceededException, ThrottlingException, ValidationException, ResourceNotFoundException,
AccessDeniedException, AwsServiceException, SdkClientException, TnbException {
return createSolNetworkInstance(CreateSolNetworkInstanceRequest.builder().applyMutation(createSolNetworkInstanceRequest)
.build());
}
/**
*
* Creates a network package.
*
*
* A network package is a .zip file in CSAR (Cloud Service Archive) format defines the function packages you want to
* deploy and the Amazon Web Services infrastructure you want to deploy them on. For more information, see Network instances in the Amazon
* Web Services Telco Network Builder User Guide.
*
*
* A network package consists of a network service descriptor (NSD) file (required) and any additional files
* (optional), such as scripts specific to your needs. For example, if you have multiple function packages in your
* network package, you can use the NSD to define which network functions should run in certain VPCs, subnets, or
* EKS clusters.
*
*
* This request creates an empty network package container with an ID. Once you create a network package, you can
* upload the network package content using PutSolNetworkPackageContent.
*
*
* @param createSolNetworkPackageRequest
* @return Result of the CreateSolNetworkPackage operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ServiceQuotaExceededException
* Service quotas have been exceeded.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TnbException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TnbClient.CreateSolNetworkPackage
* @see AWS
* API Documentation
*/
default CreateSolNetworkPackageResponse createSolNetworkPackage(CreateSolNetworkPackageRequest createSolNetworkPackageRequest)
throws InternalServerException, ServiceQuotaExceededException, ThrottlingException, ValidationException,
AccessDeniedException, AwsServiceException, SdkClientException, TnbException {
throw new UnsupportedOperationException();
}
/**
*
* Creates a network package.
*
*
* A network package is a .zip file in CSAR (Cloud Service Archive) format defines the function packages you want to
* deploy and the Amazon Web Services infrastructure you want to deploy them on. For more information, see Network instances in the Amazon
* Web Services Telco Network Builder User Guide.
*
*
* A network package consists of a network service descriptor (NSD) file (required) and any additional files
* (optional), such as scripts specific to your needs. For example, if you have multiple function packages in your
* network package, you can use the NSD to define which network functions should run in certain VPCs, subnets, or
* EKS clusters.
*
*
* This request creates an empty network package container with an ID. Once you create a network package, you can
* upload the network package content using PutSolNetworkPackageContent.
*
*
*
* This is a convenience which creates an instance of the {@link CreateSolNetworkPackageRequest.Builder} avoiding
* the need to create one manually via {@link CreateSolNetworkPackageRequest#builder()}
*
*
* @param createSolNetworkPackageRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.tnb.model.CreateSolNetworkPackageRequest.Builder} to create a
* request.
* @return Result of the CreateSolNetworkPackage operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ServiceQuotaExceededException
* Service quotas have been exceeded.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TnbException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TnbClient.CreateSolNetworkPackage
* @see AWS
* API Documentation
*/
default CreateSolNetworkPackageResponse createSolNetworkPackage(
Consumer createSolNetworkPackageRequest) throws InternalServerException,
ServiceQuotaExceededException, ThrottlingException, ValidationException, AccessDeniedException, AwsServiceException,
SdkClientException, TnbException {
return createSolNetworkPackage(CreateSolNetworkPackageRequest.builder().applyMutation(createSolNetworkPackageRequest)
.build());
}
/**
*
* Deletes a function package.
*
*
* A function package is a .zip file in CSAR (Cloud Service Archive) format that contains a network function (an
* ETSI standard telecommunication application) and function package descriptor that uses the TOSCA standard to
* describe how the network functions should run on your network.
*
*
* To delete a function package, the package must be in a disabled state. To disable a function package, see UpdateSolFunctionPackage
* .
*
*
* @param deleteSolFunctionPackageRequest
* @return Result of the DeleteSolFunctionPackage operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws ResourceNotFoundException
* Request references a resource that doesn't exist.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TnbException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TnbClient.DeleteSolFunctionPackage
* @see AWS
* API Documentation
*/
default DeleteSolFunctionPackageResponse deleteSolFunctionPackage(
DeleteSolFunctionPackageRequest deleteSolFunctionPackageRequest) throws InternalServerException, ThrottlingException,
ValidationException, ResourceNotFoundException, AccessDeniedException, AwsServiceException, SdkClientException,
TnbException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a function package.
*
*
* A function package is a .zip file in CSAR (Cloud Service Archive) format that contains a network function (an
* ETSI standard telecommunication application) and function package descriptor that uses the TOSCA standard to
* describe how the network functions should run on your network.
*
*
* To delete a function package, the package must be in a disabled state. To disable a function package, see UpdateSolFunctionPackage
* .
*
*
*
* This is a convenience which creates an instance of the {@link DeleteSolFunctionPackageRequest.Builder} avoiding
* the need to create one manually via {@link DeleteSolFunctionPackageRequest#builder()}
*
*
* @param deleteSolFunctionPackageRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.tnb.model.DeleteSolFunctionPackageRequest.Builder} to create a
* request.
* @return Result of the DeleteSolFunctionPackage operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws ResourceNotFoundException
* Request references a resource that doesn't exist.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TnbException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TnbClient.DeleteSolFunctionPackage
* @see AWS
* API Documentation
*/
default DeleteSolFunctionPackageResponse deleteSolFunctionPackage(
Consumer deleteSolFunctionPackageRequest) throws InternalServerException,
ThrottlingException, ValidationException, ResourceNotFoundException, AccessDeniedException, AwsServiceException,
SdkClientException, TnbException {
return deleteSolFunctionPackage(DeleteSolFunctionPackageRequest.builder().applyMutation(deleteSolFunctionPackageRequest)
.build());
}
/**
*
* Deletes a network instance.
*
*
* A network instance is a single network created in Amazon Web Services TNB that can be deployed and on which
* life-cycle operations (like terminate, update, and delete) can be performed.
*
*
* To delete a network instance, the instance must be in a stopped or terminated state. To terminate a network
* instance, see
* TerminateSolNetworkInstance.
*
*
* @param deleteSolNetworkInstanceRequest
* @return Result of the DeleteSolNetworkInstance operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws ResourceNotFoundException
* Request references a resource that doesn't exist.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TnbException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TnbClient.DeleteSolNetworkInstance
* @see AWS
* API Documentation
*/
default DeleteSolNetworkInstanceResponse deleteSolNetworkInstance(
DeleteSolNetworkInstanceRequest deleteSolNetworkInstanceRequest) throws InternalServerException, ThrottlingException,
ValidationException, ResourceNotFoundException, AccessDeniedException, AwsServiceException, SdkClientException,
TnbException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a network instance.
*
*
* A network instance is a single network created in Amazon Web Services TNB that can be deployed and on which
* life-cycle operations (like terminate, update, and delete) can be performed.
*
*
* To delete a network instance, the instance must be in a stopped or terminated state. To terminate a network
* instance, see
* TerminateSolNetworkInstance.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteSolNetworkInstanceRequest.Builder} avoiding
* the need to create one manually via {@link DeleteSolNetworkInstanceRequest#builder()}
*
*
* @param deleteSolNetworkInstanceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.tnb.model.DeleteSolNetworkInstanceRequest.Builder} to create a
* request.
* @return Result of the DeleteSolNetworkInstance operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws ResourceNotFoundException
* Request references a resource that doesn't exist.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TnbException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TnbClient.DeleteSolNetworkInstance
* @see AWS
* API Documentation
*/
default DeleteSolNetworkInstanceResponse deleteSolNetworkInstance(
Consumer deleteSolNetworkInstanceRequest) throws InternalServerException,
ThrottlingException, ValidationException, ResourceNotFoundException, AccessDeniedException, AwsServiceException,
SdkClientException, TnbException {
return deleteSolNetworkInstance(DeleteSolNetworkInstanceRequest.builder().applyMutation(deleteSolNetworkInstanceRequest)
.build());
}
/**
*
* Deletes network package.
*
*
* A network package is a .zip file in CSAR (Cloud Service Archive) format defines the function packages you want to
* deploy and the Amazon Web Services infrastructure you want to deploy them on.
*
*
* To delete a network package, the package must be in a disable state. To disable a network package, see UpdateSolNetworkPackage.
*
*
* @param deleteSolNetworkPackageRequest
* @return Result of the DeleteSolNetworkPackage operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws ResourceNotFoundException
* Request references a resource that doesn't exist.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TnbException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TnbClient.DeleteSolNetworkPackage
* @see AWS
* API Documentation
*/
default DeleteSolNetworkPackageResponse deleteSolNetworkPackage(DeleteSolNetworkPackageRequest deleteSolNetworkPackageRequest)
throws InternalServerException, ThrottlingException, ValidationException, ResourceNotFoundException,
AccessDeniedException, AwsServiceException, SdkClientException, TnbException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes network package.
*
*
* A network package is a .zip file in CSAR (Cloud Service Archive) format defines the function packages you want to
* deploy and the Amazon Web Services infrastructure you want to deploy them on.
*
*
* To delete a network package, the package must be in a disable state. To disable a network package, see UpdateSolNetworkPackage.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteSolNetworkPackageRequest.Builder} avoiding
* the need to create one manually via {@link DeleteSolNetworkPackageRequest#builder()}
*
*
* @param deleteSolNetworkPackageRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.tnb.model.DeleteSolNetworkPackageRequest.Builder} to create a
* request.
* @return Result of the DeleteSolNetworkPackage operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws ResourceNotFoundException
* Request references a resource that doesn't exist.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TnbException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TnbClient.DeleteSolNetworkPackage
* @see AWS
* API Documentation
*/
default DeleteSolNetworkPackageResponse deleteSolNetworkPackage(
Consumer deleteSolNetworkPackageRequest) throws InternalServerException,
ThrottlingException, ValidationException, ResourceNotFoundException, AccessDeniedException, AwsServiceException,
SdkClientException, TnbException {
return deleteSolNetworkPackage(DeleteSolNetworkPackageRequest.builder().applyMutation(deleteSolNetworkPackageRequest)
.build());
}
/**
*
* Gets the details of a network function instance, including the instantiation state and metadata from the function
* package descriptor in the network function package.
*
*
* A network function instance is a function in a function package .
*
*
* @param getSolFunctionInstanceRequest
* @return Result of the GetSolFunctionInstance operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws ResourceNotFoundException
* Request references a resource that doesn't exist.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TnbException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TnbClient.GetSolFunctionInstance
* @see AWS
* API Documentation
*/
default GetSolFunctionInstanceResponse getSolFunctionInstance(GetSolFunctionInstanceRequest getSolFunctionInstanceRequest)
throws InternalServerException, ThrottlingException, ValidationException, ResourceNotFoundException,
AccessDeniedException, AwsServiceException, SdkClientException, TnbException {
throw new UnsupportedOperationException();
}
/**
*
* Gets the details of a network function instance, including the instantiation state and metadata from the function
* package descriptor in the network function package.
*
*
* A network function instance is a function in a function package .
*
*
*
* This is a convenience which creates an instance of the {@link GetSolFunctionInstanceRequest.Builder} avoiding the
* need to create one manually via {@link GetSolFunctionInstanceRequest#builder()}
*
*
* @param getSolFunctionInstanceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.tnb.model.GetSolFunctionInstanceRequest.Builder} to create a
* request.
* @return Result of the GetSolFunctionInstance operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws ResourceNotFoundException
* Request references a resource that doesn't exist.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TnbException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TnbClient.GetSolFunctionInstance
* @see AWS
* API Documentation
*/
default GetSolFunctionInstanceResponse getSolFunctionInstance(
Consumer getSolFunctionInstanceRequest) throws InternalServerException,
ThrottlingException, ValidationException, ResourceNotFoundException, AccessDeniedException, AwsServiceException,
SdkClientException, TnbException {
return getSolFunctionInstance(GetSolFunctionInstanceRequest.builder().applyMutation(getSolFunctionInstanceRequest)
.build());
}
/**
*
* Gets the details of an individual function package, such as the operational state and whether the package is in
* use.
*
*
* A function package is a .zip file in CSAR (Cloud Service Archive) format that contains a network function (an
* ETSI standard telecommunication application) and function package descriptor that uses the TOSCA standard to
* describe how the network functions should run on your network..
*
*
* @param getSolFunctionPackageRequest
* @return Result of the GetSolFunctionPackage operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws ResourceNotFoundException
* Request references a resource that doesn't exist.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TnbException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TnbClient.GetSolFunctionPackage
* @see AWS API
* Documentation
*/
default GetSolFunctionPackageResponse getSolFunctionPackage(GetSolFunctionPackageRequest getSolFunctionPackageRequest)
throws InternalServerException, ThrottlingException, ValidationException, ResourceNotFoundException,
AccessDeniedException, AwsServiceException, SdkClientException, TnbException {
throw new UnsupportedOperationException();
}
/**
*
* Gets the details of an individual function package, such as the operational state and whether the package is in
* use.
*
*
* A function package is a .zip file in CSAR (Cloud Service Archive) format that contains a network function (an
* ETSI standard telecommunication application) and function package descriptor that uses the TOSCA standard to
* describe how the network functions should run on your network..
*
*
*
* This is a convenience which creates an instance of the {@link GetSolFunctionPackageRequest.Builder} avoiding the
* need to create one manually via {@link GetSolFunctionPackageRequest#builder()}
*
*
* @param getSolFunctionPackageRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.tnb.model.GetSolFunctionPackageRequest.Builder} to create a
* request.
* @return Result of the GetSolFunctionPackage operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws ResourceNotFoundException
* Request references a resource that doesn't exist.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TnbException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TnbClient.GetSolFunctionPackage
* @see AWS API
* Documentation
*/
default GetSolFunctionPackageResponse getSolFunctionPackage(
Consumer getSolFunctionPackageRequest) throws InternalServerException,
ThrottlingException, ValidationException, ResourceNotFoundException, AccessDeniedException, AwsServiceException,
SdkClientException, TnbException {
return getSolFunctionPackage(GetSolFunctionPackageRequest.builder().applyMutation(getSolFunctionPackageRequest).build());
}
/**
*
* Gets the contents of a function package.
*
*
* A function package is a .zip file in CSAR (Cloud Service Archive) format that contains a network function (an
* ETSI standard telecommunication application) and function package descriptor that uses the TOSCA standard to
* describe how the network functions should run on your network.
*
*
* @param getSolFunctionPackageContentRequest
* @return Result of the GetSolFunctionPackageContent operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws ResourceNotFoundException
* Request references a resource that doesn't exist.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TnbException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TnbClient.GetSolFunctionPackageContent
* @see AWS API Documentation
*/
default GetSolFunctionPackageContentResponse getSolFunctionPackageContent(
GetSolFunctionPackageContentRequest getSolFunctionPackageContentRequest) throws InternalServerException,
ThrottlingException, ValidationException, ResourceNotFoundException, AccessDeniedException, AwsServiceException,
SdkClientException, TnbException {
throw new UnsupportedOperationException();
}
/**
*
* Gets the contents of a function package.
*
*
* A function package is a .zip file in CSAR (Cloud Service Archive) format that contains a network function (an
* ETSI standard telecommunication application) and function package descriptor that uses the TOSCA standard to
* describe how the network functions should run on your network.
*
*
*
* This is a convenience which creates an instance of the {@link GetSolFunctionPackageContentRequest.Builder}
* avoiding the need to create one manually via {@link GetSolFunctionPackageContentRequest#builder()}
*
*
* @param getSolFunctionPackageContentRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.tnb.model.GetSolFunctionPackageContentRequest.Builder} to create a
* request.
* @return Result of the GetSolFunctionPackageContent operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws ResourceNotFoundException
* Request references a resource that doesn't exist.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TnbException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TnbClient.GetSolFunctionPackageContent
* @see AWS API Documentation
*/
default GetSolFunctionPackageContentResponse getSolFunctionPackageContent(
Consumer getSolFunctionPackageContentRequest)
throws InternalServerException, ThrottlingException, ValidationException, ResourceNotFoundException,
AccessDeniedException, AwsServiceException, SdkClientException, TnbException {
return getSolFunctionPackageContent(GetSolFunctionPackageContentRequest.builder()
.applyMutation(getSolFunctionPackageContentRequest).build());
}
/**
*
* Gets a function package descriptor in a function package.
*
*
* A function package descriptor is a .yaml file in a function package that uses the TOSCA standard to describe how
* the network function in the function package should run on your network.
*
*
* A function package is a .zip file in CSAR (Cloud Service Archive) format that contains a network function (an
* ETSI standard telecommunication application) and function package descriptor that uses the TOSCA standard to
* describe how the network functions should run on your network.
*
*
* @param getSolFunctionPackageDescriptorRequest
* @return Result of the GetSolFunctionPackageDescriptor operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws ResourceNotFoundException
* Request references a resource that doesn't exist.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TnbException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TnbClient.GetSolFunctionPackageDescriptor
* @see AWS API Documentation
*/
default GetSolFunctionPackageDescriptorResponse getSolFunctionPackageDescriptor(
GetSolFunctionPackageDescriptorRequest getSolFunctionPackageDescriptorRequest) throws InternalServerException,
ThrottlingException, ValidationException, ResourceNotFoundException, AccessDeniedException, AwsServiceException,
SdkClientException, TnbException {
throw new UnsupportedOperationException();
}
/**
*
* Gets a function package descriptor in a function package.
*
*
* A function package descriptor is a .yaml file in a function package that uses the TOSCA standard to describe how
* the network function in the function package should run on your network.
*
*
* A function package is a .zip file in CSAR (Cloud Service Archive) format that contains a network function (an
* ETSI standard telecommunication application) and function package descriptor that uses the TOSCA standard to
* describe how the network functions should run on your network.
*
*
*
* This is a convenience which creates an instance of the {@link GetSolFunctionPackageDescriptorRequest.Builder}
* avoiding the need to create one manually via {@link GetSolFunctionPackageDescriptorRequest#builder()}
*
*
* @param getSolFunctionPackageDescriptorRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.tnb.model.GetSolFunctionPackageDescriptorRequest.Builder} to create
* a request.
* @return Result of the GetSolFunctionPackageDescriptor operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws ResourceNotFoundException
* Request references a resource that doesn't exist.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TnbException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TnbClient.GetSolFunctionPackageDescriptor
* @see AWS API Documentation
*/
default GetSolFunctionPackageDescriptorResponse getSolFunctionPackageDescriptor(
Consumer getSolFunctionPackageDescriptorRequest)
throws InternalServerException, ThrottlingException, ValidationException, ResourceNotFoundException,
AccessDeniedException, AwsServiceException, SdkClientException, TnbException {
return getSolFunctionPackageDescriptor(GetSolFunctionPackageDescriptorRequest.builder()
.applyMutation(getSolFunctionPackageDescriptorRequest).build());
}
/**
*
* Gets the details of the network instance.
*
*
* A network instance is a single network created in Amazon Web Services TNB that can be deployed and on which
* life-cycle operations (like terminate, update, and delete) can be performed.
*
*
* @param getSolNetworkInstanceRequest
* @return Result of the GetSolNetworkInstance operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws ResourceNotFoundException
* Request references a resource that doesn't exist.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TnbException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TnbClient.GetSolNetworkInstance
* @see AWS API
* Documentation
*/
default GetSolNetworkInstanceResponse getSolNetworkInstance(GetSolNetworkInstanceRequest getSolNetworkInstanceRequest)
throws InternalServerException, ThrottlingException, ValidationException, ResourceNotFoundException,
AccessDeniedException, AwsServiceException, SdkClientException, TnbException {
throw new UnsupportedOperationException();
}
/**
*
* Gets the details of the network instance.
*
*
* A network instance is a single network created in Amazon Web Services TNB that can be deployed and on which
* life-cycle operations (like terminate, update, and delete) can be performed.
*
*
*
* This is a convenience which creates an instance of the {@link GetSolNetworkInstanceRequest.Builder} avoiding the
* need to create one manually via {@link GetSolNetworkInstanceRequest#builder()}
*
*
* @param getSolNetworkInstanceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.tnb.model.GetSolNetworkInstanceRequest.Builder} to create a
* request.
* @return Result of the GetSolNetworkInstance operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws ResourceNotFoundException
* Request references a resource that doesn't exist.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TnbException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TnbClient.GetSolNetworkInstance
* @see AWS API
* Documentation
*/
default GetSolNetworkInstanceResponse getSolNetworkInstance(
Consumer getSolNetworkInstanceRequest) throws InternalServerException,
ThrottlingException, ValidationException, ResourceNotFoundException, AccessDeniedException, AwsServiceException,
SdkClientException, TnbException {
return getSolNetworkInstance(GetSolNetworkInstanceRequest.builder().applyMutation(getSolNetworkInstanceRequest).build());
}
/**
*
* Gets the details of a network operation, including the tasks involved in the network operation and the status of
* the tasks.
*
*
* A network operation is any operation that is done to your network, such as network instance instantiation or
* termination.
*
*
* @param getSolNetworkOperationRequest
* @return Result of the GetSolNetworkOperation operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws ResourceNotFoundException
* Request references a resource that doesn't exist.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TnbException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TnbClient.GetSolNetworkOperation
* @see AWS
* API Documentation
*/
default GetSolNetworkOperationResponse getSolNetworkOperation(GetSolNetworkOperationRequest getSolNetworkOperationRequest)
throws InternalServerException, ThrottlingException, ValidationException, ResourceNotFoundException,
AccessDeniedException, AwsServiceException, SdkClientException, TnbException {
throw new UnsupportedOperationException();
}
/**
*
* Gets the details of a network operation, including the tasks involved in the network operation and the status of
* the tasks.
*
*
* A network operation is any operation that is done to your network, such as network instance instantiation or
* termination.
*
*
*
* This is a convenience which creates an instance of the {@link GetSolNetworkOperationRequest.Builder} avoiding the
* need to create one manually via {@link GetSolNetworkOperationRequest#builder()}
*
*
* @param getSolNetworkOperationRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.tnb.model.GetSolNetworkOperationRequest.Builder} to create a
* request.
* @return Result of the GetSolNetworkOperation operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws ResourceNotFoundException
* Request references a resource that doesn't exist.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TnbException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TnbClient.GetSolNetworkOperation
* @see AWS
* API Documentation
*/
default GetSolNetworkOperationResponse getSolNetworkOperation(
Consumer getSolNetworkOperationRequest) throws InternalServerException,
ThrottlingException, ValidationException, ResourceNotFoundException, AccessDeniedException, AwsServiceException,
SdkClientException, TnbException {
return getSolNetworkOperation(GetSolNetworkOperationRequest.builder().applyMutation(getSolNetworkOperationRequest)
.build());
}
/**
*
* Gets the details of a network package.
*
*
* A network package is a .zip file in CSAR (Cloud Service Archive) format defines the function packages you want to
* deploy and the Amazon Web Services infrastructure you want to deploy them on.
*
*
* @param getSolNetworkPackageRequest
* @return Result of the GetSolNetworkPackage operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws ResourceNotFoundException
* Request references a resource that doesn't exist.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TnbException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TnbClient.GetSolNetworkPackage
* @see AWS API
* Documentation
*/
default GetSolNetworkPackageResponse getSolNetworkPackage(GetSolNetworkPackageRequest getSolNetworkPackageRequest)
throws InternalServerException, ThrottlingException, ValidationException, ResourceNotFoundException,
AccessDeniedException, AwsServiceException, SdkClientException, TnbException {
throw new UnsupportedOperationException();
}
/**
*
* Gets the details of a network package.
*
*
* A network package is a .zip file in CSAR (Cloud Service Archive) format defines the function packages you want to
* deploy and the Amazon Web Services infrastructure you want to deploy them on.
*
*
*
* This is a convenience which creates an instance of the {@link GetSolNetworkPackageRequest.Builder} avoiding the
* need to create one manually via {@link GetSolNetworkPackageRequest#builder()}
*
*
* @param getSolNetworkPackageRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.tnb.model.GetSolNetworkPackageRequest.Builder} to create a request.
* @return Result of the GetSolNetworkPackage operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws ResourceNotFoundException
* Request references a resource that doesn't exist.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TnbException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TnbClient.GetSolNetworkPackage
* @see AWS API
* Documentation
*/
default GetSolNetworkPackageResponse getSolNetworkPackage(
Consumer getSolNetworkPackageRequest) throws InternalServerException,
ThrottlingException, ValidationException, ResourceNotFoundException, AccessDeniedException, AwsServiceException,
SdkClientException, TnbException {
return getSolNetworkPackage(GetSolNetworkPackageRequest.builder().applyMutation(getSolNetworkPackageRequest).build());
}
/**
*
* Gets the contents of a network package.
*
*
* A network package is a .zip file in CSAR (Cloud Service Archive) format defines the function packages you want to
* deploy and the Amazon Web Services infrastructure you want to deploy them on.
*
*
* @param getSolNetworkPackageContentRequest
* @return Result of the GetSolNetworkPackageContent operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws ResourceNotFoundException
* Request references a resource that doesn't exist.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TnbException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TnbClient.GetSolNetworkPackageContent
* @see AWS API Documentation
*/
default GetSolNetworkPackageContentResponse getSolNetworkPackageContent(
GetSolNetworkPackageContentRequest getSolNetworkPackageContentRequest) throws InternalServerException,
ThrottlingException, ValidationException, ResourceNotFoundException, AccessDeniedException, AwsServiceException,
SdkClientException, TnbException {
throw new UnsupportedOperationException();
}
/**
*
* Gets the contents of a network package.
*
*
* A network package is a .zip file in CSAR (Cloud Service Archive) format defines the function packages you want to
* deploy and the Amazon Web Services infrastructure you want to deploy them on.
*
*
*
* This is a convenience which creates an instance of the {@link GetSolNetworkPackageContentRequest.Builder}
* avoiding the need to create one manually via {@link GetSolNetworkPackageContentRequest#builder()}
*
*
* @param getSolNetworkPackageContentRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.tnb.model.GetSolNetworkPackageContentRequest.Builder} to create a
* request.
* @return Result of the GetSolNetworkPackageContent operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws ResourceNotFoundException
* Request references a resource that doesn't exist.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TnbException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TnbClient.GetSolNetworkPackageContent
* @see AWS API Documentation
*/
default GetSolNetworkPackageContentResponse getSolNetworkPackageContent(
Consumer getSolNetworkPackageContentRequest)
throws InternalServerException, ThrottlingException, ValidationException, ResourceNotFoundException,
AccessDeniedException, AwsServiceException, SdkClientException, TnbException {
return getSolNetworkPackageContent(GetSolNetworkPackageContentRequest.builder()
.applyMutation(getSolNetworkPackageContentRequest).build());
}
/**
*
* Gets the content of the network service descriptor.
*
*
* A network service descriptor is a .yaml file in a network package that uses the TOSCA standard to describe the
* network functions you want to deploy and the Amazon Web Services infrastructure you want to deploy the network
* functions on.
*
*
* @param getSolNetworkPackageDescriptorRequest
* @return Result of the GetSolNetworkPackageDescriptor operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws ResourceNotFoundException
* Request references a resource that doesn't exist.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TnbException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TnbClient.GetSolNetworkPackageDescriptor
* @see AWS API Documentation
*/
default GetSolNetworkPackageDescriptorResponse getSolNetworkPackageDescriptor(
GetSolNetworkPackageDescriptorRequest getSolNetworkPackageDescriptorRequest) throws InternalServerException,
ThrottlingException, ValidationException, ResourceNotFoundException, AccessDeniedException, AwsServiceException,
SdkClientException, TnbException {
throw new UnsupportedOperationException();
}
/**
*
* Gets the content of the network service descriptor.
*
*
* A network service descriptor is a .yaml file in a network package that uses the TOSCA standard to describe the
* network functions you want to deploy and the Amazon Web Services infrastructure you want to deploy the network
* functions on.
*
*
*
* This is a convenience which creates an instance of the {@link GetSolNetworkPackageDescriptorRequest.Builder}
* avoiding the need to create one manually via {@link GetSolNetworkPackageDescriptorRequest#builder()}
*
*
* @param getSolNetworkPackageDescriptorRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.tnb.model.GetSolNetworkPackageDescriptorRequest.Builder} to create
* a request.
* @return Result of the GetSolNetworkPackageDescriptor operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws ResourceNotFoundException
* Request references a resource that doesn't exist.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TnbException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TnbClient.GetSolNetworkPackageDescriptor
* @see AWS API Documentation
*/
default GetSolNetworkPackageDescriptorResponse getSolNetworkPackageDescriptor(
Consumer getSolNetworkPackageDescriptorRequest)
throws InternalServerException, ThrottlingException, ValidationException, ResourceNotFoundException,
AccessDeniedException, AwsServiceException, SdkClientException, TnbException {
return getSolNetworkPackageDescriptor(GetSolNetworkPackageDescriptorRequest.builder()
.applyMutation(getSolNetworkPackageDescriptorRequest).build());
}
/**
*
* Instantiates a network instance.
*
*
* A network instance is a single network created in Amazon Web Services TNB that can be deployed and on which
* life-cycle operations (like terminate, update, and delete) can be performed.
*
*
* Before you can instantiate a network instance, you have to create a network instance. For more information, see
*
* CreateSolNetworkInstance.
*
*
* @param instantiateSolNetworkInstanceRequest
* @return Result of the InstantiateSolNetworkInstance operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ServiceQuotaExceededException
* Service quotas have been exceeded.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws ResourceNotFoundException
* Request references a resource that doesn't exist.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TnbException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TnbClient.InstantiateSolNetworkInstance
* @see AWS API Documentation
*/
default InstantiateSolNetworkInstanceResponse instantiateSolNetworkInstance(
InstantiateSolNetworkInstanceRequest instantiateSolNetworkInstanceRequest) throws InternalServerException,
ServiceQuotaExceededException, ThrottlingException, ValidationException, ResourceNotFoundException,
AccessDeniedException, AwsServiceException, SdkClientException, TnbException {
throw new UnsupportedOperationException();
}
/**
*
* Instantiates a network instance.
*
*
* A network instance is a single network created in Amazon Web Services TNB that can be deployed and on which
* life-cycle operations (like terminate, update, and delete) can be performed.
*
*
* Before you can instantiate a network instance, you have to create a network instance. For more information, see
*
* CreateSolNetworkInstance.
*
*
*
* This is a convenience which creates an instance of the {@link InstantiateSolNetworkInstanceRequest.Builder}
* avoiding the need to create one manually via {@link InstantiateSolNetworkInstanceRequest#builder()}
*
*
* @param instantiateSolNetworkInstanceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.tnb.model.InstantiateSolNetworkInstanceRequest.Builder} to create a
* request.
* @return Result of the InstantiateSolNetworkInstance operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ServiceQuotaExceededException
* Service quotas have been exceeded.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws ResourceNotFoundException
* Request references a resource that doesn't exist.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TnbException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TnbClient.InstantiateSolNetworkInstance
* @see AWS API Documentation
*/
default InstantiateSolNetworkInstanceResponse instantiateSolNetworkInstance(
Consumer instantiateSolNetworkInstanceRequest)
throws InternalServerException, ServiceQuotaExceededException, ThrottlingException, ValidationException,
ResourceNotFoundException, AccessDeniedException, AwsServiceException, SdkClientException, TnbException {
return instantiateSolNetworkInstance(InstantiateSolNetworkInstanceRequest.builder()
.applyMutation(instantiateSolNetworkInstanceRequest).build());
}
/**
*
* Lists network function instances.
*
*
* A network function instance is a function in a function package .
*
*
* @param listSolFunctionInstancesRequest
* @return Result of the ListSolFunctionInstances operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TnbException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TnbClient.ListSolFunctionInstances
* @see AWS
* API Documentation
*/
default ListSolFunctionInstancesResponse listSolFunctionInstances(
ListSolFunctionInstancesRequest listSolFunctionInstancesRequest) throws InternalServerException, ThrottlingException,
ValidationException, AccessDeniedException, AwsServiceException, SdkClientException, TnbException {
throw new UnsupportedOperationException();
}
/**
*
* Lists network function instances.
*
*
* A network function instance is a function in a function package .
*
*
*
* This is a convenience which creates an instance of the {@link ListSolFunctionInstancesRequest.Builder} avoiding
* the need to create one manually via {@link ListSolFunctionInstancesRequest#builder()}
*
*
* @param listSolFunctionInstancesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.tnb.model.ListSolFunctionInstancesRequest.Builder} to create a
* request.
* @return Result of the ListSolFunctionInstances operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TnbException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TnbClient.ListSolFunctionInstances
* @see AWS
* API Documentation
*/
default ListSolFunctionInstancesResponse listSolFunctionInstances(
Consumer listSolFunctionInstancesRequest) throws InternalServerException,
ThrottlingException, ValidationException, AccessDeniedException, AwsServiceException, SdkClientException,
TnbException {
return listSolFunctionInstances(ListSolFunctionInstancesRequest.builder().applyMutation(listSolFunctionInstancesRequest)
.build());
}
/**
*
* This is a variant of
* {@link #listSolFunctionInstances(software.amazon.awssdk.services.tnb.model.ListSolFunctionInstancesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.tnb.paginators.ListSolFunctionInstancesIterable responses = client.listSolFunctionInstancesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.tnb.paginators.ListSolFunctionInstancesIterable responses = client
* .listSolFunctionInstancesPaginator(request);
* for (software.amazon.awssdk.services.tnb.model.ListSolFunctionInstancesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.tnb.paginators.ListSolFunctionInstancesIterable responses = client.listSolFunctionInstancesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listSolFunctionInstances(software.amazon.awssdk.services.tnb.model.ListSolFunctionInstancesRequest)}
* operation.
*
*
* @param listSolFunctionInstancesRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TnbException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TnbClient.ListSolFunctionInstances
* @see AWS
* API Documentation
*/
default ListSolFunctionInstancesIterable listSolFunctionInstancesPaginator(
ListSolFunctionInstancesRequest listSolFunctionInstancesRequest) throws InternalServerException, ThrottlingException,
ValidationException, AccessDeniedException, AwsServiceException, SdkClientException, TnbException {
return new ListSolFunctionInstancesIterable(this, listSolFunctionInstancesRequest);
}
/**
*
* This is a variant of
* {@link #listSolFunctionInstances(software.amazon.awssdk.services.tnb.model.ListSolFunctionInstancesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.tnb.paginators.ListSolFunctionInstancesIterable responses = client.listSolFunctionInstancesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.tnb.paginators.ListSolFunctionInstancesIterable responses = client
* .listSolFunctionInstancesPaginator(request);
* for (software.amazon.awssdk.services.tnb.model.ListSolFunctionInstancesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.tnb.paginators.ListSolFunctionInstancesIterable responses = client.listSolFunctionInstancesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listSolFunctionInstances(software.amazon.awssdk.services.tnb.model.ListSolFunctionInstancesRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListSolFunctionInstancesRequest.Builder} avoiding
* the need to create one manually via {@link ListSolFunctionInstancesRequest#builder()}
*
*
* @param listSolFunctionInstancesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.tnb.model.ListSolFunctionInstancesRequest.Builder} to create a
* request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TnbException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TnbClient.ListSolFunctionInstances
* @see AWS
* API Documentation
*/
default ListSolFunctionInstancesIterable listSolFunctionInstancesPaginator(
Consumer listSolFunctionInstancesRequest) throws InternalServerException,
ThrottlingException, ValidationException, AccessDeniedException, AwsServiceException, SdkClientException,
TnbException {
return listSolFunctionInstancesPaginator(ListSolFunctionInstancesRequest.builder()
.applyMutation(listSolFunctionInstancesRequest).build());
}
/**
*
* Lists information about function packages.
*
*
* A function package is a .zip file in CSAR (Cloud Service Archive) format that contains a network function (an
* ETSI standard telecommunication application) and function package descriptor that uses the TOSCA standard to
* describe how the network functions should run on your network.
*
*
* @param listSolFunctionPackagesRequest
* @return Result of the ListSolFunctionPackages operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TnbException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TnbClient.ListSolFunctionPackages
* @see AWS
* API Documentation
*/
default ListSolFunctionPackagesResponse listSolFunctionPackages(ListSolFunctionPackagesRequest listSolFunctionPackagesRequest)
throws InternalServerException, ThrottlingException, ValidationException, AccessDeniedException, AwsServiceException,
SdkClientException, TnbException {
throw new UnsupportedOperationException();
}
/**
*
* Lists information about function packages.
*
*
* A function package is a .zip file in CSAR (Cloud Service Archive) format that contains a network function (an
* ETSI standard telecommunication application) and function package descriptor that uses the TOSCA standard to
* describe how the network functions should run on your network.
*
*
*
* This is a convenience which creates an instance of the {@link ListSolFunctionPackagesRequest.Builder} avoiding
* the need to create one manually via {@link ListSolFunctionPackagesRequest#builder()}
*
*
* @param listSolFunctionPackagesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.tnb.model.ListSolFunctionPackagesRequest.Builder} to create a
* request.
* @return Result of the ListSolFunctionPackages operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TnbException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TnbClient.ListSolFunctionPackages
* @see AWS
* API Documentation
*/
default ListSolFunctionPackagesResponse listSolFunctionPackages(
Consumer listSolFunctionPackagesRequest) throws InternalServerException,
ThrottlingException, ValidationException, AccessDeniedException, AwsServiceException, SdkClientException,
TnbException {
return listSolFunctionPackages(ListSolFunctionPackagesRequest.builder().applyMutation(listSolFunctionPackagesRequest)
.build());
}
/**
*
* This is a variant of
* {@link #listSolFunctionPackages(software.amazon.awssdk.services.tnb.model.ListSolFunctionPackagesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.tnb.paginators.ListSolFunctionPackagesIterable responses = client.listSolFunctionPackagesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.tnb.paginators.ListSolFunctionPackagesIterable responses = client
* .listSolFunctionPackagesPaginator(request);
* for (software.amazon.awssdk.services.tnb.model.ListSolFunctionPackagesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.tnb.paginators.ListSolFunctionPackagesIterable responses = client.listSolFunctionPackagesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listSolFunctionPackages(software.amazon.awssdk.services.tnb.model.ListSolFunctionPackagesRequest)}
* operation.
*
*
* @param listSolFunctionPackagesRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TnbException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TnbClient.ListSolFunctionPackages
* @see AWS
* API Documentation
*/
default ListSolFunctionPackagesIterable listSolFunctionPackagesPaginator(
ListSolFunctionPackagesRequest listSolFunctionPackagesRequest) throws InternalServerException, ThrottlingException,
ValidationException, AccessDeniedException, AwsServiceException, SdkClientException, TnbException {
return new ListSolFunctionPackagesIterable(this, listSolFunctionPackagesRequest);
}
/**
*
* This is a variant of
* {@link #listSolFunctionPackages(software.amazon.awssdk.services.tnb.model.ListSolFunctionPackagesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.tnb.paginators.ListSolFunctionPackagesIterable responses = client.listSolFunctionPackagesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.tnb.paginators.ListSolFunctionPackagesIterable responses = client
* .listSolFunctionPackagesPaginator(request);
* for (software.amazon.awssdk.services.tnb.model.ListSolFunctionPackagesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.tnb.paginators.ListSolFunctionPackagesIterable responses = client.listSolFunctionPackagesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listSolFunctionPackages(software.amazon.awssdk.services.tnb.model.ListSolFunctionPackagesRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListSolFunctionPackagesRequest.Builder} avoiding
* the need to create one manually via {@link ListSolFunctionPackagesRequest#builder()}
*
*
* @param listSolFunctionPackagesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.tnb.model.ListSolFunctionPackagesRequest.Builder} to create a
* request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TnbException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TnbClient.ListSolFunctionPackages
* @see AWS
* API Documentation
*/
default ListSolFunctionPackagesIterable listSolFunctionPackagesPaginator(
Consumer listSolFunctionPackagesRequest) throws InternalServerException,
ThrottlingException, ValidationException, AccessDeniedException, AwsServiceException, SdkClientException,
TnbException {
return listSolFunctionPackagesPaginator(ListSolFunctionPackagesRequest.builder()
.applyMutation(listSolFunctionPackagesRequest).build());
}
/**
*
* Lists your network instances.
*
*
* A network instance is a single network created in Amazon Web Services TNB that can be deployed and on which
* life-cycle operations (like terminate, update, and delete) can be performed.
*
*
* @param listSolNetworkInstancesRequest
* @return Result of the ListSolNetworkInstances operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TnbException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TnbClient.ListSolNetworkInstances
* @see AWS
* API Documentation
*/
default ListSolNetworkInstancesResponse listSolNetworkInstances(ListSolNetworkInstancesRequest listSolNetworkInstancesRequest)
throws InternalServerException, ThrottlingException, ValidationException, AccessDeniedException, AwsServiceException,
SdkClientException, TnbException {
throw new UnsupportedOperationException();
}
/**
*
* Lists your network instances.
*
*
* A network instance is a single network created in Amazon Web Services TNB that can be deployed and on which
* life-cycle operations (like terminate, update, and delete) can be performed.
*
*
*
* This is a convenience which creates an instance of the {@link ListSolNetworkInstancesRequest.Builder} avoiding
* the need to create one manually via {@link ListSolNetworkInstancesRequest#builder()}
*
*
* @param listSolNetworkInstancesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.tnb.model.ListSolNetworkInstancesRequest.Builder} to create a
* request.
* @return Result of the ListSolNetworkInstances operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TnbException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TnbClient.ListSolNetworkInstances
* @see AWS
* API Documentation
*/
default ListSolNetworkInstancesResponse listSolNetworkInstances(
Consumer listSolNetworkInstancesRequest) throws InternalServerException,
ThrottlingException, ValidationException, AccessDeniedException, AwsServiceException, SdkClientException,
TnbException {
return listSolNetworkInstances(ListSolNetworkInstancesRequest.builder().applyMutation(listSolNetworkInstancesRequest)
.build());
}
/**
*
* This is a variant of
* {@link #listSolNetworkInstances(software.amazon.awssdk.services.tnb.model.ListSolNetworkInstancesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.tnb.paginators.ListSolNetworkInstancesIterable responses = client.listSolNetworkInstancesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.tnb.paginators.ListSolNetworkInstancesIterable responses = client
* .listSolNetworkInstancesPaginator(request);
* for (software.amazon.awssdk.services.tnb.model.ListSolNetworkInstancesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.tnb.paginators.ListSolNetworkInstancesIterable responses = client.listSolNetworkInstancesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listSolNetworkInstances(software.amazon.awssdk.services.tnb.model.ListSolNetworkInstancesRequest)}
* operation.
*
*
* @param listSolNetworkInstancesRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TnbException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TnbClient.ListSolNetworkInstances
* @see AWS
* API Documentation
*/
default ListSolNetworkInstancesIterable listSolNetworkInstancesPaginator(
ListSolNetworkInstancesRequest listSolNetworkInstancesRequest) throws InternalServerException, ThrottlingException,
ValidationException, AccessDeniedException, AwsServiceException, SdkClientException, TnbException {
return new ListSolNetworkInstancesIterable(this, listSolNetworkInstancesRequest);
}
/**
*
* This is a variant of
* {@link #listSolNetworkInstances(software.amazon.awssdk.services.tnb.model.ListSolNetworkInstancesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.tnb.paginators.ListSolNetworkInstancesIterable responses = client.listSolNetworkInstancesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.tnb.paginators.ListSolNetworkInstancesIterable responses = client
* .listSolNetworkInstancesPaginator(request);
* for (software.amazon.awssdk.services.tnb.model.ListSolNetworkInstancesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.tnb.paginators.ListSolNetworkInstancesIterable responses = client.listSolNetworkInstancesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listSolNetworkInstances(software.amazon.awssdk.services.tnb.model.ListSolNetworkInstancesRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListSolNetworkInstancesRequest.Builder} avoiding
* the need to create one manually via {@link ListSolNetworkInstancesRequest#builder()}
*
*
* @param listSolNetworkInstancesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.tnb.model.ListSolNetworkInstancesRequest.Builder} to create a
* request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TnbException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TnbClient.ListSolNetworkInstances
* @see AWS
* API Documentation
*/
default ListSolNetworkInstancesIterable listSolNetworkInstancesPaginator(
Consumer listSolNetworkInstancesRequest) throws InternalServerException,
ThrottlingException, ValidationException, AccessDeniedException, AwsServiceException, SdkClientException,
TnbException {
return listSolNetworkInstancesPaginator(ListSolNetworkInstancesRequest.builder()
.applyMutation(listSolNetworkInstancesRequest).build());
}
/**
*
* Lists details for a network operation, including when the operation started and the status of the operation.
*
*
* A network operation is any operation that is done to your network, such as network instance instantiation or
* termination.
*
*
* @param listSolNetworkOperationsRequest
* @return Result of the ListSolNetworkOperations operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TnbException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TnbClient.ListSolNetworkOperations
* @see AWS
* API Documentation
*/
default ListSolNetworkOperationsResponse listSolNetworkOperations(
ListSolNetworkOperationsRequest listSolNetworkOperationsRequest) throws InternalServerException, ThrottlingException,
ValidationException, AccessDeniedException, AwsServiceException, SdkClientException, TnbException {
throw new UnsupportedOperationException();
}
/**
*
* Lists details for a network operation, including when the operation started and the status of the operation.
*
*
* A network operation is any operation that is done to your network, such as network instance instantiation or
* termination.
*
*
*
* This is a convenience which creates an instance of the {@link ListSolNetworkOperationsRequest.Builder} avoiding
* the need to create one manually via {@link ListSolNetworkOperationsRequest#builder()}
*
*
* @param listSolNetworkOperationsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.tnb.model.ListSolNetworkOperationsRequest.Builder} to create a
* request.
* @return Result of the ListSolNetworkOperations operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TnbException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TnbClient.ListSolNetworkOperations
* @see AWS
* API Documentation
*/
default ListSolNetworkOperationsResponse listSolNetworkOperations(
Consumer listSolNetworkOperationsRequest) throws InternalServerException,
ThrottlingException, ValidationException, AccessDeniedException, AwsServiceException, SdkClientException,
TnbException {
return listSolNetworkOperations(ListSolNetworkOperationsRequest.builder().applyMutation(listSolNetworkOperationsRequest)
.build());
}
/**
*
* This is a variant of
* {@link #listSolNetworkOperations(software.amazon.awssdk.services.tnb.model.ListSolNetworkOperationsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.tnb.paginators.ListSolNetworkOperationsIterable responses = client.listSolNetworkOperationsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.tnb.paginators.ListSolNetworkOperationsIterable responses = client
* .listSolNetworkOperationsPaginator(request);
* for (software.amazon.awssdk.services.tnb.model.ListSolNetworkOperationsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.tnb.paginators.ListSolNetworkOperationsIterable responses = client.listSolNetworkOperationsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listSolNetworkOperations(software.amazon.awssdk.services.tnb.model.ListSolNetworkOperationsRequest)}
* operation.
*
*
* @param listSolNetworkOperationsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TnbException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TnbClient.ListSolNetworkOperations
* @see AWS
* API Documentation
*/
default ListSolNetworkOperationsIterable listSolNetworkOperationsPaginator(
ListSolNetworkOperationsRequest listSolNetworkOperationsRequest) throws InternalServerException, ThrottlingException,
ValidationException, AccessDeniedException, AwsServiceException, SdkClientException, TnbException {
return new ListSolNetworkOperationsIterable(this, listSolNetworkOperationsRequest);
}
/**
*
* This is a variant of
* {@link #listSolNetworkOperations(software.amazon.awssdk.services.tnb.model.ListSolNetworkOperationsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.tnb.paginators.ListSolNetworkOperationsIterable responses = client.listSolNetworkOperationsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.tnb.paginators.ListSolNetworkOperationsIterable responses = client
* .listSolNetworkOperationsPaginator(request);
* for (software.amazon.awssdk.services.tnb.model.ListSolNetworkOperationsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.tnb.paginators.ListSolNetworkOperationsIterable responses = client.listSolNetworkOperationsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listSolNetworkOperations(software.amazon.awssdk.services.tnb.model.ListSolNetworkOperationsRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListSolNetworkOperationsRequest.Builder} avoiding
* the need to create one manually via {@link ListSolNetworkOperationsRequest#builder()}
*
*
* @param listSolNetworkOperationsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.tnb.model.ListSolNetworkOperationsRequest.Builder} to create a
* request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TnbException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TnbClient.ListSolNetworkOperations
* @see AWS
* API Documentation
*/
default ListSolNetworkOperationsIterable listSolNetworkOperationsPaginator(
Consumer listSolNetworkOperationsRequest) throws InternalServerException,
ThrottlingException, ValidationException, AccessDeniedException, AwsServiceException, SdkClientException,
TnbException {
return listSolNetworkOperationsPaginator(ListSolNetworkOperationsRequest.builder()
.applyMutation(listSolNetworkOperationsRequest).build());
}
/**
*
* Lists network packages.
*
*
* A network package is a .zip file in CSAR (Cloud Service Archive) format defines the function packages you want to
* deploy and the Amazon Web Services infrastructure you want to deploy them on.
*
*
* @param listSolNetworkPackagesRequest
* @return Result of the ListSolNetworkPackages operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TnbException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TnbClient.ListSolNetworkPackages
* @see AWS
* API Documentation
*/
default ListSolNetworkPackagesResponse listSolNetworkPackages(ListSolNetworkPackagesRequest listSolNetworkPackagesRequest)
throws InternalServerException, ThrottlingException, ValidationException, AccessDeniedException, AwsServiceException,
SdkClientException, TnbException {
throw new UnsupportedOperationException();
}
/**
*
* Lists network packages.
*
*
* A network package is a .zip file in CSAR (Cloud Service Archive) format defines the function packages you want to
* deploy and the Amazon Web Services infrastructure you want to deploy them on.
*
*
*
* This is a convenience which creates an instance of the {@link ListSolNetworkPackagesRequest.Builder} avoiding the
* need to create one manually via {@link ListSolNetworkPackagesRequest#builder()}
*
*
* @param listSolNetworkPackagesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.tnb.model.ListSolNetworkPackagesRequest.Builder} to create a
* request.
* @return Result of the ListSolNetworkPackages operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TnbException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TnbClient.ListSolNetworkPackages
* @see AWS
* API Documentation
*/
default ListSolNetworkPackagesResponse listSolNetworkPackages(
Consumer listSolNetworkPackagesRequest) throws InternalServerException,
ThrottlingException, ValidationException, AccessDeniedException, AwsServiceException, SdkClientException,
TnbException {
return listSolNetworkPackages(ListSolNetworkPackagesRequest.builder().applyMutation(listSolNetworkPackagesRequest)
.build());
}
/**
*
* This is a variant of
* {@link #listSolNetworkPackages(software.amazon.awssdk.services.tnb.model.ListSolNetworkPackagesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.tnb.paginators.ListSolNetworkPackagesIterable responses = client.listSolNetworkPackagesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.tnb.paginators.ListSolNetworkPackagesIterable responses = client
* .listSolNetworkPackagesPaginator(request);
* for (software.amazon.awssdk.services.tnb.model.ListSolNetworkPackagesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.tnb.paginators.ListSolNetworkPackagesIterable responses = client.listSolNetworkPackagesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listSolNetworkPackages(software.amazon.awssdk.services.tnb.model.ListSolNetworkPackagesRequest)}
* operation.
*
*
* @param listSolNetworkPackagesRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TnbException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TnbClient.ListSolNetworkPackages
* @see AWS
* API Documentation
*/
default ListSolNetworkPackagesIterable listSolNetworkPackagesPaginator(
ListSolNetworkPackagesRequest listSolNetworkPackagesRequest) throws InternalServerException, ThrottlingException,
ValidationException, AccessDeniedException, AwsServiceException, SdkClientException, TnbException {
return new ListSolNetworkPackagesIterable(this, listSolNetworkPackagesRequest);
}
/**
*
* This is a variant of
* {@link #listSolNetworkPackages(software.amazon.awssdk.services.tnb.model.ListSolNetworkPackagesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.tnb.paginators.ListSolNetworkPackagesIterable responses = client.listSolNetworkPackagesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.tnb.paginators.ListSolNetworkPackagesIterable responses = client
* .listSolNetworkPackagesPaginator(request);
* for (software.amazon.awssdk.services.tnb.model.ListSolNetworkPackagesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.tnb.paginators.ListSolNetworkPackagesIterable responses = client.listSolNetworkPackagesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listSolNetworkPackages(software.amazon.awssdk.services.tnb.model.ListSolNetworkPackagesRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListSolNetworkPackagesRequest.Builder} avoiding the
* need to create one manually via {@link ListSolNetworkPackagesRequest#builder()}
*
*
* @param listSolNetworkPackagesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.tnb.model.ListSolNetworkPackagesRequest.Builder} to create a
* request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TnbException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TnbClient.ListSolNetworkPackages
* @see AWS
* API Documentation
*/
default ListSolNetworkPackagesIterable listSolNetworkPackagesPaginator(
Consumer listSolNetworkPackagesRequest) throws InternalServerException,
ThrottlingException, ValidationException, AccessDeniedException, AwsServiceException, SdkClientException,
TnbException {
return listSolNetworkPackagesPaginator(ListSolNetworkPackagesRequest.builder()
.applyMutation(listSolNetworkPackagesRequest).build());
}
/**
*
* Lists tags for AWS TNB resources.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws ResourceNotFoundException
* Request references a resource that doesn't exist.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TnbException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TnbClient.ListTagsForResource
* @see AWS API
* Documentation
*/
default ListTagsForResourceResponse listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest)
throws InternalServerException, ThrottlingException, ValidationException, ResourceNotFoundException,
AccessDeniedException, AwsServiceException, SdkClientException, TnbException {
throw new UnsupportedOperationException();
}
/**
*
* Lists tags for AWS TNB resources.
*
*
*
* This is a convenience which creates an instance of the {@link ListTagsForResourceRequest.Builder} avoiding the
* need to create one manually via {@link ListTagsForResourceRequest#builder()}
*
*
* @param listTagsForResourceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.tnb.model.ListTagsForResourceRequest.Builder} to create a request.
* @return Result of the ListTagsForResource operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws ResourceNotFoundException
* Request references a resource that doesn't exist.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TnbException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TnbClient.ListTagsForResource
* @see AWS API
* Documentation
*/
default ListTagsForResourceResponse listTagsForResource(
Consumer listTagsForResourceRequest) throws InternalServerException,
ThrottlingException, ValidationException, ResourceNotFoundException, AccessDeniedException, AwsServiceException,
SdkClientException, TnbException {
return listTagsForResource(ListTagsForResourceRequest.builder().applyMutation(listTagsForResourceRequest).build());
}
/**
*
* Uploads the contents of a function package.
*
*
* A function package is a .zip file in CSAR (Cloud Service Archive) format that contains a network function (an
* ETSI standard telecommunication application) and function package descriptor that uses the TOSCA standard to
* describe how the network functions should run on your network.
*
*
* @param putSolFunctionPackageContentRequest
* @return Result of the PutSolFunctionPackageContent operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws ResourceNotFoundException
* Request references a resource that doesn't exist.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TnbException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TnbClient.PutSolFunctionPackageContent
* @see AWS API Documentation
*/
default PutSolFunctionPackageContentResponse putSolFunctionPackageContent(
PutSolFunctionPackageContentRequest putSolFunctionPackageContentRequest) throws InternalServerException,
ThrottlingException, ValidationException, ResourceNotFoundException, AccessDeniedException, AwsServiceException,
SdkClientException, TnbException {
throw new UnsupportedOperationException();
}
/**
*
* Uploads the contents of a function package.
*
*
* A function package is a .zip file in CSAR (Cloud Service Archive) format that contains a network function (an
* ETSI standard telecommunication application) and function package descriptor that uses the TOSCA standard to
* describe how the network functions should run on your network.
*
*
*
* This is a convenience which creates an instance of the {@link PutSolFunctionPackageContentRequest.Builder}
* avoiding the need to create one manually via {@link PutSolFunctionPackageContentRequest#builder()}
*
*
* @param putSolFunctionPackageContentRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.tnb.model.PutSolFunctionPackageContentRequest.Builder} to create a
* request.
* @return Result of the PutSolFunctionPackageContent operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws ResourceNotFoundException
* Request references a resource that doesn't exist.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TnbException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TnbClient.PutSolFunctionPackageContent
* @see AWS API Documentation
*/
default PutSolFunctionPackageContentResponse putSolFunctionPackageContent(
Consumer putSolFunctionPackageContentRequest)
throws InternalServerException, ThrottlingException, ValidationException, ResourceNotFoundException,
AccessDeniedException, AwsServiceException, SdkClientException, TnbException {
return putSolFunctionPackageContent(PutSolFunctionPackageContentRequest.builder()
.applyMutation(putSolFunctionPackageContentRequest).build());
}
/**
*
* Uploads the contents of a network package.
*
*
* A network package is a .zip file in CSAR (Cloud Service Archive) format defines the function packages you want to
* deploy and the Amazon Web Services infrastructure you want to deploy them on.
*
*
* @param putSolNetworkPackageContentRequest
* @return Result of the PutSolNetworkPackageContent operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws ResourceNotFoundException
* Request references a resource that doesn't exist.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TnbException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TnbClient.PutSolNetworkPackageContent
* @see AWS API Documentation
*/
default PutSolNetworkPackageContentResponse putSolNetworkPackageContent(
PutSolNetworkPackageContentRequest putSolNetworkPackageContentRequest) throws InternalServerException,
ThrottlingException, ValidationException, ResourceNotFoundException, AccessDeniedException, AwsServiceException,
SdkClientException, TnbException {
throw new UnsupportedOperationException();
}
/**
*
* Uploads the contents of a network package.
*
*
* A network package is a .zip file in CSAR (Cloud Service Archive) format defines the function packages you want to
* deploy and the Amazon Web Services infrastructure you want to deploy them on.
*
*
*
* This is a convenience which creates an instance of the {@link PutSolNetworkPackageContentRequest.Builder}
* avoiding the need to create one manually via {@link PutSolNetworkPackageContentRequest#builder()}
*
*
* @param putSolNetworkPackageContentRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.tnb.model.PutSolNetworkPackageContentRequest.Builder} to create a
* request.
* @return Result of the PutSolNetworkPackageContent operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws ResourceNotFoundException
* Request references a resource that doesn't exist.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TnbException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TnbClient.PutSolNetworkPackageContent
* @see AWS API Documentation
*/
default PutSolNetworkPackageContentResponse putSolNetworkPackageContent(
Consumer putSolNetworkPackageContentRequest)
throws InternalServerException, ThrottlingException, ValidationException, ResourceNotFoundException,
AccessDeniedException, AwsServiceException, SdkClientException, TnbException {
return putSolNetworkPackageContent(PutSolNetworkPackageContentRequest.builder()
.applyMutation(putSolNetworkPackageContentRequest).build());
}
/**
*
* Tags an AWS TNB resource.
*
*
* A tag is a label that you assign to an Amazon Web Services resource. Each tag consists of a key and an optional
* value. You can use tags to search and filter your resources or track your Amazon Web Services costs.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws ResourceNotFoundException
* Request references a resource that doesn't exist.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TnbException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TnbClient.TagResource
* @see AWS API
* Documentation
*/
default TagResourceResponse tagResource(TagResourceRequest tagResourceRequest) throws InternalServerException,
ThrottlingException, ValidationException, ResourceNotFoundException, AccessDeniedException, AwsServiceException,
SdkClientException, TnbException {
throw new UnsupportedOperationException();
}
/**
*
* Tags an AWS TNB resource.
*
*
* A tag is a label that you assign to an Amazon Web Services resource. Each tag consists of a key and an optional
* value. You can use tags to search and filter your resources or track your Amazon Web Services costs.
*
*
*
* This is a convenience which creates an instance of the {@link TagResourceRequest.Builder} avoiding the need to
* create one manually via {@link TagResourceRequest#builder()}
*
*
* @param tagResourceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.tnb.model.TagResourceRequest.Builder} to create a request.
* @return Result of the TagResource operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws ResourceNotFoundException
* Request references a resource that doesn't exist.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TnbException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TnbClient.TagResource
* @see AWS API
* Documentation
*/
default TagResourceResponse tagResource(Consumer tagResourceRequest)
throws InternalServerException, ThrottlingException, ValidationException, ResourceNotFoundException,
AccessDeniedException, AwsServiceException, SdkClientException, TnbException {
return tagResource(TagResourceRequest.builder().applyMutation(tagResourceRequest).build());
}
/**
*
* Terminates a network instance.
*
*
* A network instance is a single network created in Amazon Web Services TNB that can be deployed and on which
* life-cycle operations (like terminate, update, and delete) can be performed.
*
*
* You must terminate a network instance before you can delete it.
*
*
* @param terminateSolNetworkInstanceRequest
* @return Result of the TerminateSolNetworkInstance operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ServiceQuotaExceededException
* Service quotas have been exceeded.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws ResourceNotFoundException
* Request references a resource that doesn't exist.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TnbException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TnbClient.TerminateSolNetworkInstance
* @see AWS API Documentation
*/
default TerminateSolNetworkInstanceResponse terminateSolNetworkInstance(
TerminateSolNetworkInstanceRequest terminateSolNetworkInstanceRequest) throws InternalServerException,
ServiceQuotaExceededException, ThrottlingException, ValidationException, ResourceNotFoundException,
AccessDeniedException, AwsServiceException, SdkClientException, TnbException {
throw new UnsupportedOperationException();
}
/**
*
* Terminates a network instance.
*
*
* A network instance is a single network created in Amazon Web Services TNB that can be deployed and on which
* life-cycle operations (like terminate, update, and delete) can be performed.
*
*
* You must terminate a network instance before you can delete it.
*
*
*
* This is a convenience which creates an instance of the {@link TerminateSolNetworkInstanceRequest.Builder}
* avoiding the need to create one manually via {@link TerminateSolNetworkInstanceRequest#builder()}
*
*
* @param terminateSolNetworkInstanceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.tnb.model.TerminateSolNetworkInstanceRequest.Builder} to create a
* request.
* @return Result of the TerminateSolNetworkInstance operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ServiceQuotaExceededException
* Service quotas have been exceeded.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws ResourceNotFoundException
* Request references a resource that doesn't exist.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TnbException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TnbClient.TerminateSolNetworkInstance
* @see AWS API Documentation
*/
default TerminateSolNetworkInstanceResponse terminateSolNetworkInstance(
Consumer terminateSolNetworkInstanceRequest)
throws InternalServerException, ServiceQuotaExceededException, ThrottlingException, ValidationException,
ResourceNotFoundException, AccessDeniedException, AwsServiceException, SdkClientException, TnbException {
return terminateSolNetworkInstance(TerminateSolNetworkInstanceRequest.builder()
.applyMutation(terminateSolNetworkInstanceRequest).build());
}
/**
*
* Untags an AWS TNB resource.
*
*
* A tag is a label that you assign to an Amazon Web Services resource. Each tag consists of a key and an optional
* value. You can use tags to search and filter your resources or track your Amazon Web Services costs.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws ResourceNotFoundException
* Request references a resource that doesn't exist.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TnbException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TnbClient.UntagResource
* @see AWS API
* Documentation
*/
default UntagResourceResponse untagResource(UntagResourceRequest untagResourceRequest) throws InternalServerException,
ThrottlingException, ValidationException, ResourceNotFoundException, AccessDeniedException, AwsServiceException,
SdkClientException, TnbException {
throw new UnsupportedOperationException();
}
/**
*
* Untags an AWS TNB resource.
*
*
* A tag is a label that you assign to an Amazon Web Services resource. Each tag consists of a key and an optional
* value. You can use tags to search and filter your resources or track your Amazon Web Services costs.
*
*
*
* This is a convenience which creates an instance of the {@link UntagResourceRequest.Builder} avoiding the need to
* create one manually via {@link UntagResourceRequest#builder()}
*
*
* @param untagResourceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.tnb.model.UntagResourceRequest.Builder} to create a request.
* @return Result of the UntagResource operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws ResourceNotFoundException
* Request references a resource that doesn't exist.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TnbException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TnbClient.UntagResource
* @see AWS API
* Documentation
*/
default UntagResourceResponse untagResource(Consumer untagResourceRequest)
throws InternalServerException, ThrottlingException, ValidationException, ResourceNotFoundException,
AccessDeniedException, AwsServiceException, SdkClientException, TnbException {
return untagResource(UntagResourceRequest.builder().applyMutation(untagResourceRequest).build());
}
/**
*
* Updates the operational state of function package.
*
*
* A function package is a .zip file in CSAR (Cloud Service Archive) format that contains a network function (an
* ETSI standard telecommunication application) and function package descriptor that uses the TOSCA standard to
* describe how the network functions should run on your network.
*
*
* @param updateSolFunctionPackageRequest
* @return Result of the UpdateSolFunctionPackage operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws ResourceNotFoundException
* Request references a resource that doesn't exist.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TnbException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TnbClient.UpdateSolFunctionPackage
* @see AWS
* API Documentation
*/
default UpdateSolFunctionPackageResponse updateSolFunctionPackage(
UpdateSolFunctionPackageRequest updateSolFunctionPackageRequest) throws InternalServerException, ThrottlingException,
ValidationException, ResourceNotFoundException, AccessDeniedException, AwsServiceException, SdkClientException,
TnbException {
throw new UnsupportedOperationException();
}
/**
*
* Updates the operational state of function package.
*
*
* A function package is a .zip file in CSAR (Cloud Service Archive) format that contains a network function (an
* ETSI standard telecommunication application) and function package descriptor that uses the TOSCA standard to
* describe how the network functions should run on your network.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateSolFunctionPackageRequest.Builder} avoiding
* the need to create one manually via {@link UpdateSolFunctionPackageRequest#builder()}
*
*
* @param updateSolFunctionPackageRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.tnb.model.UpdateSolFunctionPackageRequest.Builder} to create a
* request.
* @return Result of the UpdateSolFunctionPackage operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws ResourceNotFoundException
* Request references a resource that doesn't exist.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TnbException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TnbClient.UpdateSolFunctionPackage
* @see AWS
* API Documentation
*/
default UpdateSolFunctionPackageResponse updateSolFunctionPackage(
Consumer updateSolFunctionPackageRequest) throws InternalServerException,
ThrottlingException, ValidationException, ResourceNotFoundException, AccessDeniedException, AwsServiceException,
SdkClientException, TnbException {
return updateSolFunctionPackage(UpdateSolFunctionPackageRequest.builder().applyMutation(updateSolFunctionPackageRequest)
.build());
}
/**
*
* Update a network instance.
*
*
* A network instance is a single network created in Amazon Web Services TNB that can be deployed and on which
* life-cycle operations (like terminate, update, and delete) can be performed.
*
*
* Choose the updateType parameter to target the necessary update of the network instance.
*
*
* @param updateSolNetworkInstanceRequest
* @return Result of the UpdateSolNetworkInstance operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ServiceQuotaExceededException
* Service quotas have been exceeded.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws ResourceNotFoundException
* Request references a resource that doesn't exist.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TnbException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TnbClient.UpdateSolNetworkInstance
* @see AWS
* API Documentation
*/
default UpdateSolNetworkInstanceResponse updateSolNetworkInstance(
UpdateSolNetworkInstanceRequest updateSolNetworkInstanceRequest) throws InternalServerException,
ServiceQuotaExceededException, ThrottlingException, ValidationException, ResourceNotFoundException,
AccessDeniedException, AwsServiceException, SdkClientException, TnbException {
throw new UnsupportedOperationException();
}
/**
*
* Update a network instance.
*
*
* A network instance is a single network created in Amazon Web Services TNB that can be deployed and on which
* life-cycle operations (like terminate, update, and delete) can be performed.
*
*
* Choose the updateType parameter to target the necessary update of the network instance.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateSolNetworkInstanceRequest.Builder} avoiding
* the need to create one manually via {@link UpdateSolNetworkInstanceRequest#builder()}
*
*
* @param updateSolNetworkInstanceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.tnb.model.UpdateSolNetworkInstanceRequest.Builder} to create a
* request.
* @return Result of the UpdateSolNetworkInstance operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ServiceQuotaExceededException
* Service quotas have been exceeded.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws ResourceNotFoundException
* Request references a resource that doesn't exist.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TnbException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TnbClient.UpdateSolNetworkInstance
* @see AWS
* API Documentation
*/
default UpdateSolNetworkInstanceResponse updateSolNetworkInstance(
Consumer updateSolNetworkInstanceRequest) throws InternalServerException,
ServiceQuotaExceededException, ThrottlingException, ValidationException, ResourceNotFoundException,
AccessDeniedException, AwsServiceException, SdkClientException, TnbException {
return updateSolNetworkInstance(UpdateSolNetworkInstanceRequest.builder().applyMutation(updateSolNetworkInstanceRequest)
.build());
}
/**
*
* Updates the operational state of a network package.
*
*
* A network package is a .zip file in CSAR (Cloud Service Archive) format defines the function packages you want to
* deploy and the Amazon Web Services infrastructure you want to deploy them on.
*
*
* A network service descriptor is a .yaml file in a network package that uses the TOSCA standard to describe the
* network functions you want to deploy and the Amazon Web Services infrastructure you want to deploy the network
* functions on.
*
*
* @param updateSolNetworkPackageRequest
* @return Result of the UpdateSolNetworkPackage operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws ResourceNotFoundException
* Request references a resource that doesn't exist.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TnbException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TnbClient.UpdateSolNetworkPackage
* @see AWS
* API Documentation
*/
default UpdateSolNetworkPackageResponse updateSolNetworkPackage(UpdateSolNetworkPackageRequest updateSolNetworkPackageRequest)
throws InternalServerException, ThrottlingException, ValidationException, ResourceNotFoundException,
AccessDeniedException, AwsServiceException, SdkClientException, TnbException {
throw new UnsupportedOperationException();
}
/**
*
* Updates the operational state of a network package.
*
*
* A network package is a .zip file in CSAR (Cloud Service Archive) format defines the function packages you want to
* deploy and the Amazon Web Services infrastructure you want to deploy them on.
*
*
* A network service descriptor is a .yaml file in a network package that uses the TOSCA standard to describe the
* network functions you want to deploy and the Amazon Web Services infrastructure you want to deploy the network
* functions on.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateSolNetworkPackageRequest.Builder} avoiding
* the need to create one manually via {@link UpdateSolNetworkPackageRequest#builder()}
*
*
* @param updateSolNetworkPackageRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.tnb.model.UpdateSolNetworkPackageRequest.Builder} to create a
* request.
* @return Result of the UpdateSolNetworkPackage operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws ResourceNotFoundException
* Request references a resource that doesn't exist.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TnbException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TnbClient.UpdateSolNetworkPackage
* @see AWS
* API Documentation
*/
default UpdateSolNetworkPackageResponse updateSolNetworkPackage(
Consumer updateSolNetworkPackageRequest) throws InternalServerException,
ThrottlingException, ValidationException, ResourceNotFoundException, AccessDeniedException, AwsServiceException,
SdkClientException, TnbException {
return updateSolNetworkPackage(UpdateSolNetworkPackageRequest.builder().applyMutation(updateSolNetworkPackageRequest)
.build());
}
/**
*
* Validates function package content. This can be used as a dry run before uploading function package content with
*
* PutSolFunctionPackageContent.
*
*
* A function package is a .zip file in CSAR (Cloud Service Archive) format that contains a network function (an
* ETSI standard telecommunication application) and function package descriptor that uses the TOSCA standard to
* describe how the network functions should run on your network.
*
*
* @param validateSolFunctionPackageContentRequest
* @return Result of the ValidateSolFunctionPackageContent operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws ResourceNotFoundException
* Request references a resource that doesn't exist.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TnbException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TnbClient.ValidateSolFunctionPackageContent
* @see AWS API Documentation
*/
default ValidateSolFunctionPackageContentResponse validateSolFunctionPackageContent(
ValidateSolFunctionPackageContentRequest validateSolFunctionPackageContentRequest) throws InternalServerException,
ThrottlingException, ValidationException, ResourceNotFoundException, AccessDeniedException, AwsServiceException,
SdkClientException, TnbException {
throw new UnsupportedOperationException();
}
/**
*
* Validates function package content. This can be used as a dry run before uploading function package content with
*
* PutSolFunctionPackageContent.
*
*
* A function package is a .zip file in CSAR (Cloud Service Archive) format that contains a network function (an
* ETSI standard telecommunication application) and function package descriptor that uses the TOSCA standard to
* describe how the network functions should run on your network.
*
*
*
* This is a convenience which creates an instance of the {@link ValidateSolFunctionPackageContentRequest.Builder}
* avoiding the need to create one manually via {@link ValidateSolFunctionPackageContentRequest#builder()}
*
*
* @param validateSolFunctionPackageContentRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.tnb.model.ValidateSolFunctionPackageContentRequest.Builder} to
* create a request.
* @return Result of the ValidateSolFunctionPackageContent operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws ResourceNotFoundException
* Request references a resource that doesn't exist.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TnbException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TnbClient.ValidateSolFunctionPackageContent
* @see AWS API Documentation
*/
default ValidateSolFunctionPackageContentResponse validateSolFunctionPackageContent(
Consumer validateSolFunctionPackageContentRequest)
throws InternalServerException, ThrottlingException, ValidationException, ResourceNotFoundException,
AccessDeniedException, AwsServiceException, SdkClientException, TnbException {
return validateSolFunctionPackageContent(ValidateSolFunctionPackageContentRequest.builder()
.applyMutation(validateSolFunctionPackageContentRequest).build());
}
/**
*
* Validates network package content. This can be used as a dry run before uploading network package content with
* PutSolNetworkPackageContent.
*
*
* A network package is a .zip file in CSAR (Cloud Service Archive) format defines the function packages you want to
* deploy and the Amazon Web Services infrastructure you want to deploy them on.
*
*
* @param validateSolNetworkPackageContentRequest
* @return Result of the ValidateSolNetworkPackageContent operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws ResourceNotFoundException
* Request references a resource that doesn't exist.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TnbException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TnbClient.ValidateSolNetworkPackageContent
* @see AWS API Documentation
*/
default ValidateSolNetworkPackageContentResponse validateSolNetworkPackageContent(
ValidateSolNetworkPackageContentRequest validateSolNetworkPackageContentRequest) throws InternalServerException,
ThrottlingException, ValidationException, ResourceNotFoundException, AccessDeniedException, AwsServiceException,
SdkClientException, TnbException {
throw new UnsupportedOperationException();
}
/**
*
* Validates network package content. This can be used as a dry run before uploading network package content with
* PutSolNetworkPackageContent.
*
*
* A network package is a .zip file in CSAR (Cloud Service Archive) format defines the function packages you want to
* deploy and the Amazon Web Services infrastructure you want to deploy them on.
*
*
*
* This is a convenience which creates an instance of the {@link ValidateSolNetworkPackageContentRequest.Builder}
* avoiding the need to create one manually via {@link ValidateSolNetworkPackageContentRequest#builder()}
*
*
* @param validateSolNetworkPackageContentRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.tnb.model.ValidateSolNetworkPackageContentRequest.Builder} to
* create a request.
* @return Result of the ValidateSolNetworkPackageContent operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws ResourceNotFoundException
* Request references a resource that doesn't exist.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TnbException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TnbClient.ValidateSolNetworkPackageContent
* @see AWS API Documentation
*/
default ValidateSolNetworkPackageContentResponse validateSolNetworkPackageContent(
Consumer validateSolNetworkPackageContentRequest)
throws InternalServerException, ThrottlingException, ValidationException, ResourceNotFoundException,
AccessDeniedException, AwsServiceException, SdkClientException, TnbException {
return validateSolNetworkPackageContent(ValidateSolNetworkPackageContentRequest.builder()
.applyMutation(validateSolNetworkPackageContentRequest).build());
}
/**
* Create a {@link TnbClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static TnbClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link TnbClient}.
*/
static TnbClientBuilder builder() {
return new DefaultTnbClientBuilder();
}
static ServiceMetadata serviceMetadata() {
return ServiceMetadata.of(SERVICE_METADATA_ID);
}
@Override
default TnbServiceClientConfiguration serviceClientConfiguration() {
throw new UnsupportedOperationException();
}
}