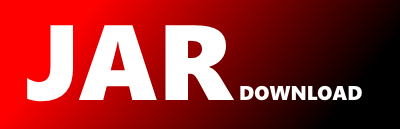
software.amazon.awssdk.services.transcribe.TranscribeClient Maven / Gradle / Ivy
Show all versions of transcribe Show documentation
/*
* Copyright 2014-2019 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.transcribe;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.core.SdkClient;
import software.amazon.awssdk.core.exception.SdkClientException;
import software.amazon.awssdk.regions.ServiceMetadata;
import software.amazon.awssdk.services.transcribe.model.BadRequestException;
import software.amazon.awssdk.services.transcribe.model.ConflictException;
import software.amazon.awssdk.services.transcribe.model.CreateVocabularyRequest;
import software.amazon.awssdk.services.transcribe.model.CreateVocabularyResponse;
import software.amazon.awssdk.services.transcribe.model.DeleteTranscriptionJobRequest;
import software.amazon.awssdk.services.transcribe.model.DeleteTranscriptionJobResponse;
import software.amazon.awssdk.services.transcribe.model.DeleteVocabularyRequest;
import software.amazon.awssdk.services.transcribe.model.DeleteVocabularyResponse;
import software.amazon.awssdk.services.transcribe.model.GetTranscriptionJobRequest;
import software.amazon.awssdk.services.transcribe.model.GetTranscriptionJobResponse;
import software.amazon.awssdk.services.transcribe.model.GetVocabularyRequest;
import software.amazon.awssdk.services.transcribe.model.GetVocabularyResponse;
import software.amazon.awssdk.services.transcribe.model.InternalFailureException;
import software.amazon.awssdk.services.transcribe.model.LimitExceededException;
import software.amazon.awssdk.services.transcribe.model.ListTranscriptionJobsRequest;
import software.amazon.awssdk.services.transcribe.model.ListTranscriptionJobsResponse;
import software.amazon.awssdk.services.transcribe.model.ListVocabulariesRequest;
import software.amazon.awssdk.services.transcribe.model.ListVocabulariesResponse;
import software.amazon.awssdk.services.transcribe.model.NotFoundException;
import software.amazon.awssdk.services.transcribe.model.StartTranscriptionJobRequest;
import software.amazon.awssdk.services.transcribe.model.StartTranscriptionJobResponse;
import software.amazon.awssdk.services.transcribe.model.TranscribeException;
import software.amazon.awssdk.services.transcribe.model.UpdateVocabularyRequest;
import software.amazon.awssdk.services.transcribe.model.UpdateVocabularyResponse;
import software.amazon.awssdk.services.transcribe.paginators.ListTranscriptionJobsIterable;
import software.amazon.awssdk.services.transcribe.paginators.ListVocabulariesIterable;
/**
* Service client for accessing Amazon Transcribe Service. This can be created using the static {@link #builder()}
* method.
*
*
* Operations and objects for transcribing speech to text.
*
*/
@Generated("software.amazon.awssdk:codegen")
public interface TranscribeClient extends SdkClient {
String SERVICE_NAME = "transcribe";
/**
* Create a {@link TranscribeClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static TranscribeClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link TranscribeClient}.
*/
static TranscribeClientBuilder builder() {
return new DefaultTranscribeClientBuilder();
}
/**
*
* Creates a new custom vocabulary that you can use to change the way Amazon Transcribe handles transcription of an
* audio file.
*
*
* @param createVocabularyRequest
* @return Result of the CreateVocabulary operation returned by the service.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the transcription you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws ConflictException
* When you are using the CreateVocabulary
operation, the JobName
field is a
* duplicate of a previously entered job name. Resend your request with a different name.
*
* When you are using the UpdateVocabulary
operation, there are two jobs running at the same
* time. Resend the second request later.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.CreateVocabulary
* @see AWS
* API Documentation
*/
default CreateVocabularyResponse createVocabulary(CreateVocabularyRequest createVocabularyRequest)
throws BadRequestException, LimitExceededException, InternalFailureException, ConflictException, AwsServiceException,
SdkClientException, TranscribeException {
throw new UnsupportedOperationException();
}
/**
*
* Creates a new custom vocabulary that you can use to change the way Amazon Transcribe handles transcription of an
* audio file.
*
*
*
* This is a convenience which creates an instance of the {@link CreateVocabularyRequest.Builder} avoiding the need
* to create one manually via {@link CreateVocabularyRequest#builder()}
*
*
* @param createVocabularyRequest
* A {@link Consumer} that will call methods on {@link CreateVocabularyRequest.Builder} to create a request.
* @return Result of the CreateVocabulary operation returned by the service.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the transcription you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws ConflictException
* When you are using the CreateVocabulary
operation, the JobName
field is a
* duplicate of a previously entered job name. Resend your request with a different name.
*
* When you are using the UpdateVocabulary
operation, there are two jobs running at the same
* time. Resend the second request later.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.CreateVocabulary
* @see AWS
* API Documentation
*/
default CreateVocabularyResponse createVocabulary(Consumer createVocabularyRequest)
throws BadRequestException, LimitExceededException, InternalFailureException, ConflictException, AwsServiceException,
SdkClientException, TranscribeException {
return createVocabulary(CreateVocabularyRequest.builder().applyMutation(createVocabularyRequest).build());
}
/**
*
* Deletes a previously submitted transcription job along with any other generated results such as the
* transcription, models, and so on.
*
*
* @param deleteTranscriptionJobRequest
* @return Result of the DeleteTranscriptionJob operation returned by the service.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the transcription you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.DeleteTranscriptionJob
* @see AWS API Documentation
*/
default DeleteTranscriptionJobResponse deleteTranscriptionJob(DeleteTranscriptionJobRequest deleteTranscriptionJobRequest)
throws LimitExceededException, BadRequestException, InternalFailureException, AwsServiceException,
SdkClientException, TranscribeException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a previously submitted transcription job along with any other generated results such as the
* transcription, models, and so on.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteTranscriptionJobRequest.Builder} avoiding the
* need to create one manually via {@link DeleteTranscriptionJobRequest#builder()}
*
*
* @param deleteTranscriptionJobRequest
* A {@link Consumer} that will call methods on {@link DeleteTranscriptionJobRequest.Builder} to create a
* request.
* @return Result of the DeleteTranscriptionJob operation returned by the service.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the transcription you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.DeleteTranscriptionJob
* @see AWS API Documentation
*/
default DeleteTranscriptionJobResponse deleteTranscriptionJob(
Consumer deleteTranscriptionJobRequest) throws LimitExceededException,
BadRequestException, InternalFailureException, AwsServiceException, SdkClientException, TranscribeException {
return deleteTranscriptionJob(DeleteTranscriptionJobRequest.builder().applyMutation(deleteTranscriptionJobRequest)
.build());
}
/**
*
* Deletes a vocabulary from Amazon Transcribe.
*
*
* @param deleteVocabularyRequest
* @return Result of the DeleteVocabulary operation returned by the service.
* @throws NotFoundException
* We can't find the requested resource. Check the name and try your request again.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the transcription you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.DeleteVocabulary
* @see AWS
* API Documentation
*/
default DeleteVocabularyResponse deleteVocabulary(DeleteVocabularyRequest deleteVocabularyRequest) throws NotFoundException,
LimitExceededException, BadRequestException, InternalFailureException, AwsServiceException, SdkClientException,
TranscribeException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a vocabulary from Amazon Transcribe.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteVocabularyRequest.Builder} avoiding the need
* to create one manually via {@link DeleteVocabularyRequest#builder()}
*
*
* @param deleteVocabularyRequest
* A {@link Consumer} that will call methods on {@link DeleteVocabularyRequest.Builder} to create a request.
* @return Result of the DeleteVocabulary operation returned by the service.
* @throws NotFoundException
* We can't find the requested resource. Check the name and try your request again.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the transcription you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.DeleteVocabulary
* @see AWS
* API Documentation
*/
default DeleteVocabularyResponse deleteVocabulary(Consumer deleteVocabularyRequest)
throws NotFoundException, LimitExceededException, BadRequestException, InternalFailureException, AwsServiceException,
SdkClientException, TranscribeException {
return deleteVocabulary(DeleteVocabularyRequest.builder().applyMutation(deleteVocabularyRequest).build());
}
/**
*
* Returns information about a transcription job. To see the status of the job, check the
* TranscriptionJobStatus
field. If the status is COMPLETED
, the job is finished and you
* can find the results at the location specified in the TranscriptionFileUri
field.
*
*
* @param getTranscriptionJobRequest
* @return Result of the GetTranscriptionJob operation returned by the service.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the transcription you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws NotFoundException
* We can't find the requested resource. Check the name and try your request again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.GetTranscriptionJob
* @see AWS
* API Documentation
*/
default GetTranscriptionJobResponse getTranscriptionJob(GetTranscriptionJobRequest getTranscriptionJobRequest)
throws BadRequestException, LimitExceededException, InternalFailureException, NotFoundException, AwsServiceException,
SdkClientException, TranscribeException {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about a transcription job. To see the status of the job, check the
* TranscriptionJobStatus
field. If the status is COMPLETED
, the job is finished and you
* can find the results at the location specified in the TranscriptionFileUri
field.
*
*
*
* This is a convenience which creates an instance of the {@link GetTranscriptionJobRequest.Builder} avoiding the
* need to create one manually via {@link GetTranscriptionJobRequest#builder()}
*
*
* @param getTranscriptionJobRequest
* A {@link Consumer} that will call methods on {@link GetTranscriptionJobRequest.Builder} to create a
* request.
* @return Result of the GetTranscriptionJob operation returned by the service.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the transcription you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws NotFoundException
* We can't find the requested resource. Check the name and try your request again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.GetTranscriptionJob
* @see AWS
* API Documentation
*/
default GetTranscriptionJobResponse getTranscriptionJob(
Consumer getTranscriptionJobRequest) throws BadRequestException,
LimitExceededException, InternalFailureException, NotFoundException, AwsServiceException, SdkClientException,
TranscribeException {
return getTranscriptionJob(GetTranscriptionJobRequest.builder().applyMutation(getTranscriptionJobRequest).build());
}
/**
*
* Gets information about a vocabulary.
*
*
* @param getVocabularyRequest
* @return Result of the GetVocabulary operation returned by the service.
* @throws NotFoundException
* We can't find the requested resource. Check the name and try your request again.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the transcription you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.GetVocabulary
* @see AWS API
* Documentation
*/
default GetVocabularyResponse getVocabulary(GetVocabularyRequest getVocabularyRequest) throws NotFoundException,
LimitExceededException, InternalFailureException, BadRequestException, AwsServiceException, SdkClientException,
TranscribeException {
throw new UnsupportedOperationException();
}
/**
*
* Gets information about a vocabulary.
*
*
*
* This is a convenience which creates an instance of the {@link GetVocabularyRequest.Builder} avoiding the need to
* create one manually via {@link GetVocabularyRequest#builder()}
*
*
* @param getVocabularyRequest
* A {@link Consumer} that will call methods on {@link GetVocabularyRequest.Builder} to create a request.
* @return Result of the GetVocabulary operation returned by the service.
* @throws NotFoundException
* We can't find the requested resource. Check the name and try your request again.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the transcription you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.GetVocabulary
* @see AWS API
* Documentation
*/
default GetVocabularyResponse getVocabulary(Consumer getVocabularyRequest)
throws NotFoundException, LimitExceededException, InternalFailureException, BadRequestException, AwsServiceException,
SdkClientException, TranscribeException {
return getVocabulary(GetVocabularyRequest.builder().applyMutation(getVocabularyRequest).build());
}
/**
*
* Lists transcription jobs with the specified status.
*
*
* @return Result of the ListTranscriptionJobs operation returned by the service.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the transcription you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.ListTranscriptionJobs
* @see #listTranscriptionJobs(ListTranscriptionJobsRequest)
* @see AWS API Documentation
*/
default ListTranscriptionJobsResponse listTranscriptionJobs() throws BadRequestException, LimitExceededException,
InternalFailureException, AwsServiceException, SdkClientException, TranscribeException {
return listTranscriptionJobs(ListTranscriptionJobsRequest.builder().build());
}
/**
*
* Lists transcription jobs with the specified status.
*
*
* @param listTranscriptionJobsRequest
* @return Result of the ListTranscriptionJobs operation returned by the service.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the transcription you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.ListTranscriptionJobs
* @see AWS API Documentation
*/
default ListTranscriptionJobsResponse listTranscriptionJobs(ListTranscriptionJobsRequest listTranscriptionJobsRequest)
throws BadRequestException, LimitExceededException, InternalFailureException, AwsServiceException,
SdkClientException, TranscribeException {
throw new UnsupportedOperationException();
}
/**
*
* Lists transcription jobs with the specified status.
*
*
*
* This is a convenience which creates an instance of the {@link ListTranscriptionJobsRequest.Builder} avoiding the
* need to create one manually via {@link ListTranscriptionJobsRequest#builder()}
*
*
* @param listTranscriptionJobsRequest
* A {@link Consumer} that will call methods on {@link ListTranscriptionJobsRequest.Builder} to create a
* request.
* @return Result of the ListTranscriptionJobs operation returned by the service.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the transcription you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.ListTranscriptionJobs
* @see AWS API Documentation
*/
default ListTranscriptionJobsResponse listTranscriptionJobs(
Consumer listTranscriptionJobsRequest) throws BadRequestException,
LimitExceededException, InternalFailureException, AwsServiceException, SdkClientException, TranscribeException {
return listTranscriptionJobs(ListTranscriptionJobsRequest.builder().applyMutation(listTranscriptionJobsRequest).build());
}
/**
*
* Lists transcription jobs with the specified status.
*
*
*
* This is a variant of
* {@link #listTranscriptionJobs(software.amazon.awssdk.services.transcribe.model.ListTranscriptionJobsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.transcribe.paginators.ListTranscriptionJobsIterable responses = client.listTranscriptionJobsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.transcribe.paginators.ListTranscriptionJobsIterable responses = client
* .listTranscriptionJobsPaginator(request);
* for (software.amazon.awssdk.services.transcribe.model.ListTranscriptionJobsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.transcribe.paginators.ListTranscriptionJobsIterable responses = client.listTranscriptionJobsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listTranscriptionJobs(software.amazon.awssdk.services.transcribe.model.ListTranscriptionJobsRequest)}
* operation.
*
*
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the transcription you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.ListTranscriptionJobs
* @see #listTranscriptionJobsPaginator(ListTranscriptionJobsRequest)
* @see AWS API Documentation
*/
default ListTranscriptionJobsIterable listTranscriptionJobsPaginator() throws BadRequestException, LimitExceededException,
InternalFailureException, AwsServiceException, SdkClientException, TranscribeException {
return listTranscriptionJobsPaginator(ListTranscriptionJobsRequest.builder().build());
}
/**
*
* Lists transcription jobs with the specified status.
*
*
*
* This is a variant of
* {@link #listTranscriptionJobs(software.amazon.awssdk.services.transcribe.model.ListTranscriptionJobsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.transcribe.paginators.ListTranscriptionJobsIterable responses = client.listTranscriptionJobsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.transcribe.paginators.ListTranscriptionJobsIterable responses = client
* .listTranscriptionJobsPaginator(request);
* for (software.amazon.awssdk.services.transcribe.model.ListTranscriptionJobsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.transcribe.paginators.ListTranscriptionJobsIterable responses = client.listTranscriptionJobsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listTranscriptionJobs(software.amazon.awssdk.services.transcribe.model.ListTranscriptionJobsRequest)}
* operation.
*
*
* @param listTranscriptionJobsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the transcription you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.ListTranscriptionJobs
* @see AWS API Documentation
*/
default ListTranscriptionJobsIterable listTranscriptionJobsPaginator(ListTranscriptionJobsRequest listTranscriptionJobsRequest)
throws BadRequestException, LimitExceededException, InternalFailureException, AwsServiceException,
SdkClientException, TranscribeException {
throw new UnsupportedOperationException();
}
/**
*
* Lists transcription jobs with the specified status.
*
*
*
* This is a variant of
* {@link #listTranscriptionJobs(software.amazon.awssdk.services.transcribe.model.ListTranscriptionJobsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.transcribe.paginators.ListTranscriptionJobsIterable responses = client.listTranscriptionJobsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.transcribe.paginators.ListTranscriptionJobsIterable responses = client
* .listTranscriptionJobsPaginator(request);
* for (software.amazon.awssdk.services.transcribe.model.ListTranscriptionJobsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.transcribe.paginators.ListTranscriptionJobsIterable responses = client.listTranscriptionJobsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listTranscriptionJobs(software.amazon.awssdk.services.transcribe.model.ListTranscriptionJobsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListTranscriptionJobsRequest.Builder} avoiding the
* need to create one manually via {@link ListTranscriptionJobsRequest#builder()}
*
*
* @param listTranscriptionJobsRequest
* A {@link Consumer} that will call methods on {@link ListTranscriptionJobsRequest.Builder} to create a
* request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the transcription you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.ListTranscriptionJobs
* @see AWS API Documentation
*/
default ListTranscriptionJobsIterable listTranscriptionJobsPaginator(
Consumer listTranscriptionJobsRequest) throws BadRequestException,
LimitExceededException, InternalFailureException, AwsServiceException, SdkClientException, TranscribeException {
return listTranscriptionJobsPaginator(ListTranscriptionJobsRequest.builder().applyMutation(listTranscriptionJobsRequest)
.build());
}
/**
*
* Returns a list of vocabularies that match the specified criteria. If no criteria are specified, returns the
* entire list of vocabularies.
*
*
* @return Result of the ListVocabularies operation returned by the service.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the transcription you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.ListVocabularies
* @see #listVocabularies(ListVocabulariesRequest)
* @see AWS
* API Documentation
*/
default ListVocabulariesResponse listVocabularies() throws BadRequestException, LimitExceededException,
InternalFailureException, AwsServiceException, SdkClientException, TranscribeException {
return listVocabularies(ListVocabulariesRequest.builder().build());
}
/**
*
* Returns a list of vocabularies that match the specified criteria. If no criteria are specified, returns the
* entire list of vocabularies.
*
*
* @param listVocabulariesRequest
* @return Result of the ListVocabularies operation returned by the service.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the transcription you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.ListVocabularies
* @see AWS
* API Documentation
*/
default ListVocabulariesResponse listVocabularies(ListVocabulariesRequest listVocabulariesRequest)
throws BadRequestException, LimitExceededException, InternalFailureException, AwsServiceException,
SdkClientException, TranscribeException {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of vocabularies that match the specified criteria. If no criteria are specified, returns the
* entire list of vocabularies.
*
*
*
* This is a convenience which creates an instance of the {@link ListVocabulariesRequest.Builder} avoiding the need
* to create one manually via {@link ListVocabulariesRequest#builder()}
*
*
* @param listVocabulariesRequest
* A {@link Consumer} that will call methods on {@link ListVocabulariesRequest.Builder} to create a request.
* @return Result of the ListVocabularies operation returned by the service.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the transcription you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.ListVocabularies
* @see AWS
* API Documentation
*/
default ListVocabulariesResponse listVocabularies(Consumer listVocabulariesRequest)
throws BadRequestException, LimitExceededException, InternalFailureException, AwsServiceException,
SdkClientException, TranscribeException {
return listVocabularies(ListVocabulariesRequest.builder().applyMutation(listVocabulariesRequest).build());
}
/**
*
* Returns a list of vocabularies that match the specified criteria. If no criteria are specified, returns the
* entire list of vocabularies.
*
*
*
* This is a variant of
* {@link #listVocabularies(software.amazon.awssdk.services.transcribe.model.ListVocabulariesRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.transcribe.paginators.ListVocabulariesIterable responses = client.listVocabulariesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.transcribe.paginators.ListVocabulariesIterable responses = client
* .listVocabulariesPaginator(request);
* for (software.amazon.awssdk.services.transcribe.model.ListVocabulariesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.transcribe.paginators.ListVocabulariesIterable responses = client.listVocabulariesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listVocabularies(software.amazon.awssdk.services.transcribe.model.ListVocabulariesRequest)}
* operation.
*
*
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the transcription you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.ListVocabularies
* @see #listVocabulariesPaginator(ListVocabulariesRequest)
* @see AWS
* API Documentation
*/
default ListVocabulariesIterable listVocabulariesPaginator() throws BadRequestException, LimitExceededException,
InternalFailureException, AwsServiceException, SdkClientException, TranscribeException {
return listVocabulariesPaginator(ListVocabulariesRequest.builder().build());
}
/**
*
* Returns a list of vocabularies that match the specified criteria. If no criteria are specified, returns the
* entire list of vocabularies.
*
*
*
* This is a variant of
* {@link #listVocabularies(software.amazon.awssdk.services.transcribe.model.ListVocabulariesRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.transcribe.paginators.ListVocabulariesIterable responses = client.listVocabulariesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.transcribe.paginators.ListVocabulariesIterable responses = client
* .listVocabulariesPaginator(request);
* for (software.amazon.awssdk.services.transcribe.model.ListVocabulariesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.transcribe.paginators.ListVocabulariesIterable responses = client.listVocabulariesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listVocabularies(software.amazon.awssdk.services.transcribe.model.ListVocabulariesRequest)}
* operation.
*
*
* @param listVocabulariesRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the transcription you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.ListVocabularies
* @see AWS
* API Documentation
*/
default ListVocabulariesIterable listVocabulariesPaginator(ListVocabulariesRequest listVocabulariesRequest)
throws BadRequestException, LimitExceededException, InternalFailureException, AwsServiceException,
SdkClientException, TranscribeException {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of vocabularies that match the specified criteria. If no criteria are specified, returns the
* entire list of vocabularies.
*
*
*
* This is a variant of
* {@link #listVocabularies(software.amazon.awssdk.services.transcribe.model.ListVocabulariesRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.transcribe.paginators.ListVocabulariesIterable responses = client.listVocabulariesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.transcribe.paginators.ListVocabulariesIterable responses = client
* .listVocabulariesPaginator(request);
* for (software.amazon.awssdk.services.transcribe.model.ListVocabulariesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.transcribe.paginators.ListVocabulariesIterable responses = client.listVocabulariesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listVocabularies(software.amazon.awssdk.services.transcribe.model.ListVocabulariesRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListVocabulariesRequest.Builder} avoiding the need
* to create one manually via {@link ListVocabulariesRequest#builder()}
*
*
* @param listVocabulariesRequest
* A {@link Consumer} that will call methods on {@link ListVocabulariesRequest.Builder} to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the transcription you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.ListVocabularies
* @see AWS
* API Documentation
*/
default ListVocabulariesIterable listVocabulariesPaginator(Consumer listVocabulariesRequest)
throws BadRequestException, LimitExceededException, InternalFailureException, AwsServiceException,
SdkClientException, TranscribeException {
return listVocabulariesPaginator(ListVocabulariesRequest.builder().applyMutation(listVocabulariesRequest).build());
}
/**
*
* Starts an asynchronous job to transcribe speech to text.
*
*
* @param startTranscriptionJobRequest
* @return Result of the StartTranscriptionJob operation returned by the service.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the transcription you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws ConflictException
* When you are using the CreateVocabulary
operation, the JobName
field is a
* duplicate of a previously entered job name. Resend your request with a different name.
*
* When you are using the UpdateVocabulary
operation, there are two jobs running at the same
* time. Resend the second request later.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.StartTranscriptionJob
* @see AWS API Documentation
*/
default StartTranscriptionJobResponse startTranscriptionJob(StartTranscriptionJobRequest startTranscriptionJobRequest)
throws BadRequestException, LimitExceededException, InternalFailureException, ConflictException, AwsServiceException,
SdkClientException, TranscribeException {
throw new UnsupportedOperationException();
}
/**
*
* Starts an asynchronous job to transcribe speech to text.
*
*
*
* This is a convenience which creates an instance of the {@link StartTranscriptionJobRequest.Builder} avoiding the
* need to create one manually via {@link StartTranscriptionJobRequest#builder()}
*
*
* @param startTranscriptionJobRequest
* A {@link Consumer} that will call methods on {@link StartTranscriptionJobRequest.Builder} to create a
* request.
* @return Result of the StartTranscriptionJob operation returned by the service.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the transcription you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws ConflictException
* When you are using the CreateVocabulary
operation, the JobName
field is a
* duplicate of a previously entered job name. Resend your request with a different name.
*
* When you are using the UpdateVocabulary
operation, there are two jobs running at the same
* time. Resend the second request later.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.StartTranscriptionJob
* @see AWS API Documentation
*/
default StartTranscriptionJobResponse startTranscriptionJob(
Consumer startTranscriptionJobRequest) throws BadRequestException,
LimitExceededException, InternalFailureException, ConflictException, AwsServiceException, SdkClientException,
TranscribeException {
return startTranscriptionJob(StartTranscriptionJobRequest.builder().applyMutation(startTranscriptionJobRequest).build());
}
/**
*
* Updates an existing vocabulary with new values. The UpdateVocabulary
operation overwrites all of the
* existing information with the values that you provide in the request.
*
*
* @param updateVocabularyRequest
* @return Result of the UpdateVocabulary operation returned by the service.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the transcription you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws NotFoundException
* We can't find the requested resource. Check the name and try your request again.
* @throws ConflictException
* When you are using the CreateVocabulary
operation, the JobName
field is a
* duplicate of a previously entered job name. Resend your request with a different name.
*
* When you are using the UpdateVocabulary
operation, there are two jobs running at the same
* time. Resend the second request later.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.UpdateVocabulary
* @see AWS
* API Documentation
*/
default UpdateVocabularyResponse updateVocabulary(UpdateVocabularyRequest updateVocabularyRequest)
throws BadRequestException, LimitExceededException, InternalFailureException, NotFoundException, ConflictException,
AwsServiceException, SdkClientException, TranscribeException {
throw new UnsupportedOperationException();
}
/**
*
* Updates an existing vocabulary with new values. The UpdateVocabulary
operation overwrites all of the
* existing information with the values that you provide in the request.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateVocabularyRequest.Builder} avoiding the need
* to create one manually via {@link UpdateVocabularyRequest#builder()}
*
*
* @param updateVocabularyRequest
* A {@link Consumer} that will call methods on {@link UpdateVocabularyRequest.Builder} to create a request.
* @return Result of the UpdateVocabulary operation returned by the service.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the transcription you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws NotFoundException
* We can't find the requested resource. Check the name and try your request again.
* @throws ConflictException
* When you are using the CreateVocabulary
operation, the JobName
field is a
* duplicate of a previously entered job name. Resend your request with a different name.
*
* When you are using the UpdateVocabulary
operation, there are two jobs running at the same
* time. Resend the second request later.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.UpdateVocabulary
* @see AWS
* API Documentation
*/
default UpdateVocabularyResponse updateVocabulary(Consumer updateVocabularyRequest)
throws BadRequestException, LimitExceededException, InternalFailureException, NotFoundException, ConflictException,
AwsServiceException, SdkClientException, TranscribeException {
return updateVocabulary(UpdateVocabularyRequest.builder().applyMutation(updateVocabularyRequest).build());
}
static ServiceMetadata serviceMetadata() {
return ServiceMetadata.of("transcribe");
}
}