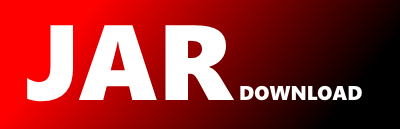
software.amazon.awssdk.services.transcribe.model.StartMedicalTranscriptionJobRequest Maven / Gradle / Ivy
Show all versions of transcribe Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.transcribe.model;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class StartMedicalTranscriptionJobRequest extends TranscribeRequest implements
ToCopyableBuilder {
private static final SdkField MEDICAL_TRANSCRIPTION_JOB_NAME_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("MedicalTranscriptionJobName")
.getter(getter(StartMedicalTranscriptionJobRequest::medicalTranscriptionJobName))
.setter(setter(Builder::medicalTranscriptionJobName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MedicalTranscriptionJobName")
.build()).build();
private static final SdkField LANGUAGE_CODE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("LanguageCode").getter(getter(StartMedicalTranscriptionJobRequest::languageCodeAsString))
.setter(setter(Builder::languageCode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LanguageCode").build()).build();
private static final SdkField MEDIA_SAMPLE_RATE_HERTZ_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("MediaSampleRateHertz").getter(getter(StartMedicalTranscriptionJobRequest::mediaSampleRateHertz))
.setter(setter(Builder::mediaSampleRateHertz))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MediaSampleRateHertz").build())
.build();
private static final SdkField MEDIA_FORMAT_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("MediaFormat").getter(getter(StartMedicalTranscriptionJobRequest::mediaFormatAsString))
.setter(setter(Builder::mediaFormat))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MediaFormat").build()).build();
private static final SdkField MEDIA_FIELD = SdkField. builder(MarshallingType.SDK_POJO).memberName("Media")
.getter(getter(StartMedicalTranscriptionJobRequest::media)).setter(setter(Builder::media))
.constructor(Media::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Media").build()).build();
private static final SdkField OUTPUT_BUCKET_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("OutputBucketName").getter(getter(StartMedicalTranscriptionJobRequest::outputBucketName))
.setter(setter(Builder::outputBucketName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OutputBucketName").build()).build();
private static final SdkField OUTPUT_KEY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("OutputKey").getter(getter(StartMedicalTranscriptionJobRequest::outputKey))
.setter(setter(Builder::outputKey))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OutputKey").build()).build();
private static final SdkField OUTPUT_ENCRYPTION_KMS_KEY_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("OutputEncryptionKMSKeyId").getter(getter(StartMedicalTranscriptionJobRequest::outputEncryptionKMSKeyId))
.setter(setter(Builder::outputEncryptionKMSKeyId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OutputEncryptionKMSKeyId").build())
.build();
private static final SdkField SETTINGS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("Settings")
.getter(getter(StartMedicalTranscriptionJobRequest::settings)).setter(setter(Builder::settings))
.constructor(MedicalTranscriptionSetting::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Settings").build()).build();
private static final SdkField SPECIALTY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Specialty").getter(getter(StartMedicalTranscriptionJobRequest::specialtyAsString))
.setter(setter(Builder::specialty))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Specialty").build()).build();
private static final SdkField TYPE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Type")
.getter(getter(StartMedicalTranscriptionJobRequest::typeAsString)).setter(setter(Builder::type))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Type").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(
MEDICAL_TRANSCRIPTION_JOB_NAME_FIELD, LANGUAGE_CODE_FIELD, MEDIA_SAMPLE_RATE_HERTZ_FIELD, MEDIA_FORMAT_FIELD,
MEDIA_FIELD, OUTPUT_BUCKET_NAME_FIELD, OUTPUT_KEY_FIELD, OUTPUT_ENCRYPTION_KMS_KEY_ID_FIELD, SETTINGS_FIELD,
SPECIALTY_FIELD, TYPE_FIELD));
private final String medicalTranscriptionJobName;
private final String languageCode;
private final Integer mediaSampleRateHertz;
private final String mediaFormat;
private final Media media;
private final String outputBucketName;
private final String outputKey;
private final String outputEncryptionKMSKeyId;
private final MedicalTranscriptionSetting settings;
private final String specialty;
private final String type;
private StartMedicalTranscriptionJobRequest(BuilderImpl builder) {
super(builder);
this.medicalTranscriptionJobName = builder.medicalTranscriptionJobName;
this.languageCode = builder.languageCode;
this.mediaSampleRateHertz = builder.mediaSampleRateHertz;
this.mediaFormat = builder.mediaFormat;
this.media = builder.media;
this.outputBucketName = builder.outputBucketName;
this.outputKey = builder.outputKey;
this.outputEncryptionKMSKeyId = builder.outputEncryptionKMSKeyId;
this.settings = builder.settings;
this.specialty = builder.specialty;
this.type = builder.type;
}
/**
*
* The name of the medical transcription job. You can't use the strings ".
" or "..
" by
* themselves as the job name. The name must also be unique within an AWS account. If you try to create a medical
* transcription job with the same name as a previous medical transcription job, you get a
* ConflictException
error.
*
*
* @return The name of the medical transcription job. You can't use the strings ".
" or "..
* " by themselves as the job name. The name must also be unique within an AWS account. If you try to create
* a medical transcription job with the same name as a previous medical transcription job, you get a
* ConflictException
error.
*/
public String medicalTranscriptionJobName() {
return medicalTranscriptionJobName;
}
/**
*
* The language code for the language spoken in the input media file. US English (en-US) is the valid value for
* medical transcription jobs. Any other value you enter for language code results in a
* BadRequestException
error.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #languageCode} will
* return {@link LanguageCode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #languageCodeAsString}.
*
*
* @return The language code for the language spoken in the input media file. US English (en-US) is the valid value
* for medical transcription jobs. Any other value you enter for language code results in a
* BadRequestException
error.
* @see LanguageCode
*/
public LanguageCode languageCode() {
return LanguageCode.fromValue(languageCode);
}
/**
*
* The language code for the language spoken in the input media file. US English (en-US) is the valid value for
* medical transcription jobs. Any other value you enter for language code results in a
* BadRequestException
error.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #languageCode} will
* return {@link LanguageCode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #languageCodeAsString}.
*
*
* @return The language code for the language spoken in the input media file. US English (en-US) is the valid value
* for medical transcription jobs. Any other value you enter for language code results in a
* BadRequestException
error.
* @see LanguageCode
*/
public String languageCodeAsString() {
return languageCode;
}
/**
*
* The sample rate, in Hertz, of the audio track in the input media file.
*
*
* If you do not specify the media sample rate, Amazon Transcribe Medical determines the sample rate. If you specify
* the sample rate, it must match the rate detected by Amazon Transcribe Medical. In most cases, you should leave
* the MediaSampleRateHertz
field blank and let Amazon Transcribe Medical determine the sample rate.
*
*
* @return The sample rate, in Hertz, of the audio track in the input media file.
*
* If you do not specify the media sample rate, Amazon Transcribe Medical determines the sample rate. If you
* specify the sample rate, it must match the rate detected by Amazon Transcribe Medical. In most cases, you
* should leave the MediaSampleRateHertz
field blank and let Amazon Transcribe Medical
* determine the sample rate.
*/
public Integer mediaSampleRateHertz() {
return mediaSampleRateHertz;
}
/**
*
* The audio format of the input media file.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #mediaFormat} will
* return {@link MediaFormat#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #mediaFormatAsString}.
*
*
* @return The audio format of the input media file.
* @see MediaFormat
*/
public MediaFormat mediaFormat() {
return MediaFormat.fromValue(mediaFormat);
}
/**
*
* The audio format of the input media file.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #mediaFormat} will
* return {@link MediaFormat#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #mediaFormatAsString}.
*
*
* @return The audio format of the input media file.
* @see MediaFormat
*/
public String mediaFormatAsString() {
return mediaFormat;
}
/**
* Returns the value of the Media property for this object.
*
* @return The value of the Media property for this object.
*/
public Media media() {
return media;
}
/**
*
* The Amazon S3 location where the transcription is stored.
*
*
* You must set OutputBucketName
for Amazon Transcribe Medical to store the transcription results. Your
* transcript appears in the S3 location you specify. When you call the GetMedicalTranscriptionJob, the
* operation returns this location in the TranscriptFileUri
field. The S3 bucket must have permissions
* that allow Amazon Transcribe Medical to put files in the bucket. For more information, see Permissions Required for IAM User Roles.
*
*
* You can specify an AWS Key Management Service (KMS) key to encrypt the output of your transcription using the
* OutputEncryptionKMSKeyId
parameter. If you don't specify a KMS key, Amazon Transcribe Medical uses
* the default Amazon S3 key for server-side encryption of transcripts that are placed in your S3 bucket.
*
*
* @return The Amazon S3 location where the transcription is stored.
*
* You must set OutputBucketName
for Amazon Transcribe Medical to store the transcription
* results. Your transcript appears in the S3 location you specify. When you call the
* GetMedicalTranscriptionJob, the operation returns this location in the
* TranscriptFileUri
field. The S3 bucket must have permissions that allow Amazon Transcribe
* Medical to put files in the bucket. For more information, see Permissions Required for IAM User Roles.
*
*
* You can specify an AWS Key Management Service (KMS) key to encrypt the output of your transcription using
* the OutputEncryptionKMSKeyId
parameter. If you don't specify a KMS key, Amazon Transcribe
* Medical uses the default Amazon S3 key for server-side encryption of transcripts that are placed in your
* S3 bucket.
*/
public String outputBucketName() {
return outputBucketName;
}
/**
*
* You can specify a location in an Amazon S3 bucket to store the output of your medical transcription job.
*
*
* If you don't specify an output key, Amazon Transcribe Medical stores the output of your transcription job in the
* Amazon S3 bucket you specified. By default, the object key is "your-transcription-job-name.json".
*
*
* You can use output keys to specify the Amazon S3 prefix and file name of the transcription output. For example,
* specifying the Amazon S3 prefix, "folder1/folder2/", as an output key would lead to the output being stored as
* "folder1/folder2/your-transcription-job-name.json". If you specify "my-other-job-name.json" as the output key,
* the object key is changed to "my-other-job-name.json". You can use an output key to change both the prefix and
* the file name, for example "folder/my-other-job-name.json".
*
*
* If you specify an output key, you must also specify an S3 bucket in the OutputBucketName
parameter.
*
*
* @return You can specify a location in an Amazon S3 bucket to store the output of your medical transcription
* job.
*
* If you don't specify an output key, Amazon Transcribe Medical stores the output of your transcription job
* in the Amazon S3 bucket you specified. By default, the object key is "your-transcription-job-name.json".
*
*
* You can use output keys to specify the Amazon S3 prefix and file name of the transcription output. For
* example, specifying the Amazon S3 prefix, "folder1/folder2/", as an output key would lead to the output
* being stored as "folder1/folder2/your-transcription-job-name.json". If you specify
* "my-other-job-name.json" as the output key, the object key is changed to "my-other-job-name.json". You
* can use an output key to change both the prefix and the file name, for example
* "folder/my-other-job-name.json".
*
*
* If you specify an output key, you must also specify an S3 bucket in the OutputBucketName
* parameter.
*/
public String outputKey() {
return outputKey;
}
/**
*
* The Amazon Resource Name (ARN) of the AWS Key Management Service (KMS) key used to encrypt the output of the
* transcription job. The user calling the StartMedicalTranscriptionJob operation must have permission to use
* the specified KMS key.
*
*
* You use either of the following to identify a KMS key in the current account:
*
*
* -
*
* KMS Key ID: "1234abcd-12ab-34cd-56ef-1234567890ab"
*
*
* -
*
* KMS Key Alias: "alias/ExampleAlias"
*
*
*
*
* You can use either of the following to identify a KMS key in the current account or another account:
*
*
* -
*
* Amazon Resource Name (ARN) of a KMS key in the current account or another account:
* "arn:aws:kms:region:account ID:key/1234abcd-12ab-34cd-56ef-1234567890ab"
*
*
* -
*
* ARN of a KMS Key Alias: "arn:aws:kms:region:account ID:alias/ExampleAlias"
*
*
*
*
* If you don't specify an encryption key, the output of the medical transcription job is encrypted with the default
* Amazon S3 key (SSE-S3).
*
*
* If you specify a KMS key to encrypt your output, you must also specify an output location in the
* OutputBucketName
parameter.
*
*
* @return The Amazon Resource Name (ARN) of the AWS Key Management Service (KMS) key used to encrypt the output of
* the transcription job. The user calling the StartMedicalTranscriptionJob operation must have
* permission to use the specified KMS key.
*
* You use either of the following to identify a KMS key in the current account:
*
*
* -
*
* KMS Key ID: "1234abcd-12ab-34cd-56ef-1234567890ab"
*
*
* -
*
* KMS Key Alias: "alias/ExampleAlias"
*
*
*
*
* You can use either of the following to identify a KMS key in the current account or another account:
*
*
* -
*
* Amazon Resource Name (ARN) of a KMS key in the current account or another account:
* "arn:aws:kms:region:account ID:key/1234abcd-12ab-34cd-56ef-1234567890ab"
*
*
* -
*
* ARN of a KMS Key Alias: "arn:aws:kms:region:account ID:alias/ExampleAlias"
*
*
*
*
* If you don't specify an encryption key, the output of the medical transcription job is encrypted with the
* default Amazon S3 key (SSE-S3).
*
*
* If you specify a KMS key to encrypt your output, you must also specify an output location in the
* OutputBucketName
parameter.
*/
public String outputEncryptionKMSKeyId() {
return outputEncryptionKMSKeyId;
}
/**
*
* Optional settings for the medical transcription job.
*
*
* @return Optional settings for the medical transcription job.
*/
public MedicalTranscriptionSetting settings() {
return settings;
}
/**
*
* The medical specialty of any clinician speaking in the input media.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #specialty} will
* return {@link Specialty#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #specialtyAsString}.
*
*
* @return The medical specialty of any clinician speaking in the input media.
* @see Specialty
*/
public Specialty specialty() {
return Specialty.fromValue(specialty);
}
/**
*
* The medical specialty of any clinician speaking in the input media.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #specialty} will
* return {@link Specialty#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #specialtyAsString}.
*
*
* @return The medical specialty of any clinician speaking in the input media.
* @see Specialty
*/
public String specialtyAsString() {
return specialty;
}
/**
*
* The type of speech in the input audio. CONVERSATION
refers to conversations between two or more
* speakers, e.g., a conversations between doctors and patients. DICTATION
refers to single-speaker
* dictated speech, e.g., for clinical notes.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #type} will return
* {@link Type#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #typeAsString}.
*
*
* @return The type of speech in the input audio. CONVERSATION
refers to conversations between two or
* more speakers, e.g., a conversations between doctors and patients. DICTATION
refers to
* single-speaker dictated speech, e.g., for clinical notes.
* @see Type
*/
public Type type() {
return Type.fromValue(type);
}
/**
*
* The type of speech in the input audio. CONVERSATION
refers to conversations between two or more
* speakers, e.g., a conversations between doctors and patients. DICTATION
refers to single-speaker
* dictated speech, e.g., for clinical notes.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #type} will return
* {@link Type#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #typeAsString}.
*
*
* @return The type of speech in the input audio. CONVERSATION
refers to conversations between two or
* more speakers, e.g., a conversations between doctors and patients. DICTATION
refers to
* single-speaker dictated speech, e.g., for clinical notes.
* @see Type
*/
public String typeAsString() {
return type;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(medicalTranscriptionJobName());
hashCode = 31 * hashCode + Objects.hashCode(languageCodeAsString());
hashCode = 31 * hashCode + Objects.hashCode(mediaSampleRateHertz());
hashCode = 31 * hashCode + Objects.hashCode(mediaFormatAsString());
hashCode = 31 * hashCode + Objects.hashCode(media());
hashCode = 31 * hashCode + Objects.hashCode(outputBucketName());
hashCode = 31 * hashCode + Objects.hashCode(outputKey());
hashCode = 31 * hashCode + Objects.hashCode(outputEncryptionKMSKeyId());
hashCode = 31 * hashCode + Objects.hashCode(settings());
hashCode = 31 * hashCode + Objects.hashCode(specialtyAsString());
hashCode = 31 * hashCode + Objects.hashCode(typeAsString());
return hashCode;
}
@Override
public boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof StartMedicalTranscriptionJobRequest)) {
return false;
}
StartMedicalTranscriptionJobRequest other = (StartMedicalTranscriptionJobRequest) obj;
return Objects.equals(medicalTranscriptionJobName(), other.medicalTranscriptionJobName())
&& Objects.equals(languageCodeAsString(), other.languageCodeAsString())
&& Objects.equals(mediaSampleRateHertz(), other.mediaSampleRateHertz())
&& Objects.equals(mediaFormatAsString(), other.mediaFormatAsString()) && Objects.equals(media(), other.media())
&& Objects.equals(outputBucketName(), other.outputBucketName()) && Objects.equals(outputKey(), other.outputKey())
&& Objects.equals(outputEncryptionKMSKeyId(), other.outputEncryptionKMSKeyId())
&& Objects.equals(settings(), other.settings()) && Objects.equals(specialtyAsString(), other.specialtyAsString())
&& Objects.equals(typeAsString(), other.typeAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("StartMedicalTranscriptionJobRequest")
.add("MedicalTranscriptionJobName", medicalTranscriptionJobName()).add("LanguageCode", languageCodeAsString())
.add("MediaSampleRateHertz", mediaSampleRateHertz()).add("MediaFormat", mediaFormatAsString())
.add("Media", media()).add("OutputBucketName", outputBucketName()).add("OutputKey", outputKey())
.add("OutputEncryptionKMSKeyId", outputEncryptionKMSKeyId()).add("Settings", settings())
.add("Specialty", specialtyAsString()).add("Type", typeAsString()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "MedicalTranscriptionJobName":
return Optional.ofNullable(clazz.cast(medicalTranscriptionJobName()));
case "LanguageCode":
return Optional.ofNullable(clazz.cast(languageCodeAsString()));
case "MediaSampleRateHertz":
return Optional.ofNullable(clazz.cast(mediaSampleRateHertz()));
case "MediaFormat":
return Optional.ofNullable(clazz.cast(mediaFormatAsString()));
case "Media":
return Optional.ofNullable(clazz.cast(media()));
case "OutputBucketName":
return Optional.ofNullable(clazz.cast(outputBucketName()));
case "OutputKey":
return Optional.ofNullable(clazz.cast(outputKey()));
case "OutputEncryptionKMSKeyId":
return Optional.ofNullable(clazz.cast(outputEncryptionKMSKeyId()));
case "Settings":
return Optional.ofNullable(clazz.cast(settings()));
case "Specialty":
return Optional.ofNullable(clazz.cast(specialtyAsString()));
case "Type":
return Optional.ofNullable(clazz.cast(typeAsString()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function