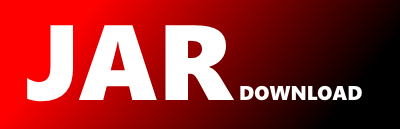
software.amazon.awssdk.services.transcribe.model.StartTranscriptionJobRequest Maven / Gradle / Ivy
Show all versions of transcribe Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.transcribe.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class StartTranscriptionJobRequest extends TranscribeRequest implements
ToCopyableBuilder {
private static final SdkField TRANSCRIPTION_JOB_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TranscriptionJobName").getter(getter(StartTranscriptionJobRequest::transcriptionJobName))
.setter(setter(Builder::transcriptionJobName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TranscriptionJobName").build())
.build();
private static final SdkField LANGUAGE_CODE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("LanguageCode").getter(getter(StartTranscriptionJobRequest::languageCodeAsString))
.setter(setter(Builder::languageCode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LanguageCode").build()).build();
private static final SdkField MEDIA_SAMPLE_RATE_HERTZ_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("MediaSampleRateHertz").getter(getter(StartTranscriptionJobRequest::mediaSampleRateHertz))
.setter(setter(Builder::mediaSampleRateHertz))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MediaSampleRateHertz").build())
.build();
private static final SdkField MEDIA_FORMAT_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("MediaFormat").getter(getter(StartTranscriptionJobRequest::mediaFormatAsString))
.setter(setter(Builder::mediaFormat))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MediaFormat").build()).build();
private static final SdkField MEDIA_FIELD = SdkField. builder(MarshallingType.SDK_POJO).memberName("Media")
.getter(getter(StartTranscriptionJobRequest::media)).setter(setter(Builder::media)).constructor(Media::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Media").build()).build();
private static final SdkField OUTPUT_BUCKET_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("OutputBucketName").getter(getter(StartTranscriptionJobRequest::outputBucketName))
.setter(setter(Builder::outputBucketName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OutputBucketName").build()).build();
private static final SdkField OUTPUT_KEY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("OutputKey").getter(getter(StartTranscriptionJobRequest::outputKey)).setter(setter(Builder::outputKey))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OutputKey").build()).build();
private static final SdkField OUTPUT_ENCRYPTION_KMS_KEY_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("OutputEncryptionKMSKeyId").getter(getter(StartTranscriptionJobRequest::outputEncryptionKMSKeyId))
.setter(setter(Builder::outputEncryptionKMSKeyId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OutputEncryptionKMSKeyId").build())
.build();
private static final SdkField SETTINGS_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("Settings").getter(getter(StartTranscriptionJobRequest::settings)).setter(setter(Builder::settings))
.constructor(Settings::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Settings").build()).build();
private static final SdkField MODEL_SETTINGS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("ModelSettings")
.getter(getter(StartTranscriptionJobRequest::modelSettings)).setter(setter(Builder::modelSettings))
.constructor(ModelSettings::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ModelSettings").build()).build();
private static final SdkField JOB_EXECUTION_SETTINGS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("JobExecutionSettings")
.getter(getter(StartTranscriptionJobRequest::jobExecutionSettings)).setter(setter(Builder::jobExecutionSettings))
.constructor(JobExecutionSettings::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("JobExecutionSettings").build())
.build();
private static final SdkField CONTENT_REDACTION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("ContentRedaction")
.getter(getter(StartTranscriptionJobRequest::contentRedaction)).setter(setter(Builder::contentRedaction))
.constructor(ContentRedaction::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ContentRedaction").build()).build();
private static final SdkField IDENTIFY_LANGUAGE_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("IdentifyLanguage").getter(getter(StartTranscriptionJobRequest::identifyLanguage))
.setter(setter(Builder::identifyLanguage))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IdentifyLanguage").build()).build();
private static final SdkField> LANGUAGE_OPTIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("LanguageOptions")
.getter(getter(StartTranscriptionJobRequest::languageOptionsAsStrings))
.setter(setter(Builder::languageOptionsWithStrings))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LanguageOptions").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(TRANSCRIPTION_JOB_NAME_FIELD,
LANGUAGE_CODE_FIELD, MEDIA_SAMPLE_RATE_HERTZ_FIELD, MEDIA_FORMAT_FIELD, MEDIA_FIELD, OUTPUT_BUCKET_NAME_FIELD,
OUTPUT_KEY_FIELD, OUTPUT_ENCRYPTION_KMS_KEY_ID_FIELD, SETTINGS_FIELD, MODEL_SETTINGS_FIELD,
JOB_EXECUTION_SETTINGS_FIELD, CONTENT_REDACTION_FIELD, IDENTIFY_LANGUAGE_FIELD, LANGUAGE_OPTIONS_FIELD));
private final String transcriptionJobName;
private final String languageCode;
private final Integer mediaSampleRateHertz;
private final String mediaFormat;
private final Media media;
private final String outputBucketName;
private final String outputKey;
private final String outputEncryptionKMSKeyId;
private final Settings settings;
private final ModelSettings modelSettings;
private final JobExecutionSettings jobExecutionSettings;
private final ContentRedaction contentRedaction;
private final Boolean identifyLanguage;
private final List languageOptions;
private StartTranscriptionJobRequest(BuilderImpl builder) {
super(builder);
this.transcriptionJobName = builder.transcriptionJobName;
this.languageCode = builder.languageCode;
this.mediaSampleRateHertz = builder.mediaSampleRateHertz;
this.mediaFormat = builder.mediaFormat;
this.media = builder.media;
this.outputBucketName = builder.outputBucketName;
this.outputKey = builder.outputKey;
this.outputEncryptionKMSKeyId = builder.outputEncryptionKMSKeyId;
this.settings = builder.settings;
this.modelSettings = builder.modelSettings;
this.jobExecutionSettings = builder.jobExecutionSettings;
this.contentRedaction = builder.contentRedaction;
this.identifyLanguage = builder.identifyLanguage;
this.languageOptions = builder.languageOptions;
}
/**
*
* The name of the job. You can't use the strings ".
" or "..
" by themselves as the job
* name. The name must also be unique within an AWS account. If you try to create a transcription job with the same
* name as a previous transcription job, you get a ConflictException
error.
*
*
* @return The name of the job. You can't use the strings ".
" or "..
" by themselves as the
* job name. The name must also be unique within an AWS account. If you try to create a transcription job
* with the same name as a previous transcription job, you get a ConflictException
error.
*/
public String transcriptionJobName() {
return transcriptionJobName;
}
/**
*
* The language code for the language used in the input media file.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #languageCode} will
* return {@link LanguageCode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #languageCodeAsString}.
*
*
* @return The language code for the language used in the input media file.
* @see LanguageCode
*/
public LanguageCode languageCode() {
return LanguageCode.fromValue(languageCode);
}
/**
*
* The language code for the language used in the input media file.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #languageCode} will
* return {@link LanguageCode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #languageCodeAsString}.
*
*
* @return The language code for the language used in the input media file.
* @see LanguageCode
*/
public String languageCodeAsString() {
return languageCode;
}
/**
*
* The sample rate, in Hertz, of the audio track in the input media file.
*
*
* If you do not specify the media sample rate, Amazon Transcribe determines the sample rate. If you specify the
* sample rate, it must match the sample rate detected by Amazon Transcribe. In most cases, you should leave the
* MediaSampleRateHertz
field blank and let Amazon Transcribe determine the sample rate.
*
*
* @return The sample rate, in Hertz, of the audio track in the input media file.
*
* If you do not specify the media sample rate, Amazon Transcribe determines the sample rate. If you specify
* the sample rate, it must match the sample rate detected by Amazon Transcribe. In most cases, you should
* leave the MediaSampleRateHertz
field blank and let Amazon Transcribe determine the sample
* rate.
*/
public Integer mediaSampleRateHertz() {
return mediaSampleRateHertz;
}
/**
*
* The format of the input media file.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #mediaFormat} will
* return {@link MediaFormat#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #mediaFormatAsString}.
*
*
* @return The format of the input media file.
* @see MediaFormat
*/
public MediaFormat mediaFormat() {
return MediaFormat.fromValue(mediaFormat);
}
/**
*
* The format of the input media file.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #mediaFormat} will
* return {@link MediaFormat#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #mediaFormatAsString}.
*
*
* @return The format of the input media file.
* @see MediaFormat
*/
public String mediaFormatAsString() {
return mediaFormat;
}
/**
*
* An object that describes the input media for a transcription job.
*
*
* @return An object that describes the input media for a transcription job.
*/
public Media media() {
return media;
}
/**
*
* The location where the transcription is stored.
*
*
* If you set the OutputBucketName
, Amazon Transcribe puts the transcript in the specified S3 bucket.
* When you call the GetTranscriptionJob operation, the operation returns this location in the
* TranscriptFileUri
field. If you enable content redaction, the redacted transcript appears in
* RedactedTranscriptFileUri
. If you enable content redaction and choose to output an unredacted
* transcript, that transcript's location still appears in the TranscriptFileUri
. The S3 bucket must
* have permissions that allow Amazon Transcribe to put files in the bucket. For more information, see Permissions Required for IAM User Roles.
*
*
* You can specify an AWS Key Management Service (KMS) key to encrypt the output of your transcription using the
* OutputEncryptionKMSKeyId
parameter. If you don't specify a KMS key, Amazon Transcribe uses the
* default Amazon S3 key for server-side encryption of transcripts that are placed in your S3 bucket.
*
*
* If you don't set the OutputBucketName
, Amazon Transcribe generates a pre-signed URL, a shareable URL
* that provides secure access to your transcription, and returns it in the TranscriptFileUri
field.
* Use this URL to download the transcription.
*
*
* @return The location where the transcription is stored.
*
* If you set the OutputBucketName
, Amazon Transcribe puts the transcript in the specified S3
* bucket. When you call the GetTranscriptionJob operation, the operation returns this location in
* the TranscriptFileUri
field. If you enable content redaction, the redacted transcript
* appears in RedactedTranscriptFileUri
. If you enable content redaction and choose to output
* an unredacted transcript, that transcript's location still appears in the TranscriptFileUri
.
* The S3 bucket must have permissions that allow Amazon Transcribe to put files in the bucket. For more
* information, see Permissions Required for IAM User Roles.
*
*
* You can specify an AWS Key Management Service (KMS) key to encrypt the output of your transcription using
* the OutputEncryptionKMSKeyId
parameter. If you don't specify a KMS key, Amazon Transcribe
* uses the default Amazon S3 key for server-side encryption of transcripts that are placed in your S3
* bucket.
*
*
* If you don't set the OutputBucketName
, Amazon Transcribe generates a pre-signed URL, a
* shareable URL that provides secure access to your transcription, and returns it in the
* TranscriptFileUri
field. Use this URL to download the transcription.
*/
public String outputBucketName() {
return outputBucketName;
}
/**
*
* You can specify a location in an Amazon S3 bucket to store the output of your transcription job.
*
*
* If you don't specify an output key, Amazon Transcribe stores the output of your transcription job in the Amazon
* S3 bucket you specified. By default, the object key is "your-transcription-job-name.json".
*
*
* You can use output keys to specify the Amazon S3 prefix and file name of the transcription output. For example,
* specifying the Amazon S3 prefix, "folder1/folder2/", as an output key would lead to the output being stored as
* "folder1/folder2/your-transcription-job-name.json". If you specify "my-other-job-name.json" as the output key,
* the object key is changed to "my-other-job-name.json". You can use an output key to change both the prefix and
* the file name, for example "folder/my-other-job-name.json".
*
*
* If you specify an output key, you must also specify an S3 bucket in the OutputBucketName
parameter.
*
*
* @return You can specify a location in an Amazon S3 bucket to store the output of your transcription job.
*
* If you don't specify an output key, Amazon Transcribe stores the output of your transcription job in the
* Amazon S3 bucket you specified. By default, the object key is "your-transcription-job-name.json".
*
*
* You can use output keys to specify the Amazon S3 prefix and file name of the transcription output. For
* example, specifying the Amazon S3 prefix, "folder1/folder2/", as an output key would lead to the output
* being stored as "folder1/folder2/your-transcription-job-name.json". If you specify
* "my-other-job-name.json" as the output key, the object key is changed to "my-other-job-name.json". You
* can use an output key to change both the prefix and the file name, for example
* "folder/my-other-job-name.json".
*
*
* If you specify an output key, you must also specify an S3 bucket in the OutputBucketName
* parameter.
*/
public String outputKey() {
return outputKey;
}
/**
*
* The Amazon Resource Name (ARN) of the AWS Key Management Service (KMS) key used to encrypt the output of the
* transcription job. The user calling the StartTranscriptionJob
operation must have permission to use
* the specified KMS key.
*
*
* You can use either of the following to identify a KMS key in the current account:
*
*
* -
*
* KMS Key ID: "1234abcd-12ab-34cd-56ef-1234567890ab"
*
*
* -
*
* KMS Key Alias: "alias/ExampleAlias"
*
*
*
*
* You can use either of the following to identify a KMS key in the current account or another account:
*
*
* -
*
* Amazon Resource Name (ARN) of a KMS Key: "arn:aws:kms:region:account ID:key/1234abcd-12ab-34cd-56ef-1234567890ab"
*
*
* -
*
* ARN of a KMS Key Alias: "arn:aws:kms:region:account ID:alias/ExampleAlias"
*
*
*
*
* If you don't specify an encryption key, the output of the transcription job is encrypted with the default Amazon
* S3 key (SSE-S3).
*
*
* If you specify a KMS key to encrypt your output, you must also specify an output location in the
* OutputBucketName
parameter.
*
*
* @return The Amazon Resource Name (ARN) of the AWS Key Management Service (KMS) key used to encrypt the output of
* the transcription job. The user calling the StartTranscriptionJob
operation must have
* permission to use the specified KMS key.
*
* You can use either of the following to identify a KMS key in the current account:
*
*
* -
*
* KMS Key ID: "1234abcd-12ab-34cd-56ef-1234567890ab"
*
*
* -
*
* KMS Key Alias: "alias/ExampleAlias"
*
*
*
*
* You can use either of the following to identify a KMS key in the current account or another account:
*
*
* -
*
* Amazon Resource Name (ARN) of a KMS Key:
* "arn:aws:kms:region:account ID:key/1234abcd-12ab-34cd-56ef-1234567890ab"
*
*
* -
*
* ARN of a KMS Key Alias: "arn:aws:kms:region:account ID:alias/ExampleAlias"
*
*
*
*
* If you don't specify an encryption key, the output of the transcription job is encrypted with the default
* Amazon S3 key (SSE-S3).
*
*
* If you specify a KMS key to encrypt your output, you must also specify an output location in the
* OutputBucketName
parameter.
*/
public String outputEncryptionKMSKeyId() {
return outputEncryptionKMSKeyId;
}
/**
*
* A Settings
object that provides optional settings for a transcription job.
*
*
* @return A Settings
object that provides optional settings for a transcription job.
*/
public Settings settings() {
return settings;
}
/**
*
* Choose the custom language model you use for your transcription job in this parameter.
*
*
* @return Choose the custom language model you use for your transcription job in this parameter.
*/
public ModelSettings modelSettings() {
return modelSettings;
}
/**
*
* Provides information about how a transcription job is executed. Use this field to indicate that the job can be
* queued for deferred execution if the concurrency limit is reached and there are no slots available to immediately
* run the job.
*
*
* @return Provides information about how a transcription job is executed. Use this field to indicate that the job
* can be queued for deferred execution if the concurrency limit is reached and there are no slots available
* to immediately run the job.
*/
public JobExecutionSettings jobExecutionSettings() {
return jobExecutionSettings;
}
/**
*
* An object that contains the request parameters for content redaction.
*
*
* @return An object that contains the request parameters for content redaction.
*/
public ContentRedaction contentRedaction() {
return contentRedaction;
}
/**
*
* Set this field to true
to enable automatic language identification. Automatic language
* identification is disabled by default. You receive a BadRequestException
error if you enter a value
* for a LanguageCode
.
*
*
* @return Set this field to true
to enable automatic language identification. Automatic language
* identification is disabled by default. You receive a BadRequestException
error if you enter
* a value for a LanguageCode
.
*/
public Boolean identifyLanguage() {
return identifyLanguage;
}
/**
*
* An object containing a list of languages that might be present in your collection of audio files. Automatic
* language identification chooses a language that best matches the source audio from that list.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasLanguageOptions()} to see if a value was sent in this field.
*
*
* @return An object containing a list of languages that might be present in your collection of audio files.
* Automatic language identification chooses a language that best matches the source audio from that list.
*/
public List languageOptions() {
return LanguageOptionsCopier.copyStringToEnum(languageOptions);
}
/**
* Returns true if the LanguageOptions property was specified by the sender (it may be empty), or false if the
* sender did not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS
* service.
*/
public boolean hasLanguageOptions() {
return languageOptions != null && !(languageOptions instanceof SdkAutoConstructList);
}
/**
*
* An object containing a list of languages that might be present in your collection of audio files. Automatic
* language identification chooses a language that best matches the source audio from that list.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasLanguageOptions()} to see if a value was sent in this field.
*
*
* @return An object containing a list of languages that might be present in your collection of audio files.
* Automatic language identification chooses a language that best matches the source audio from that list.
*/
public List languageOptionsAsStrings() {
return languageOptions;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(transcriptionJobName());
hashCode = 31 * hashCode + Objects.hashCode(languageCodeAsString());
hashCode = 31 * hashCode + Objects.hashCode(mediaSampleRateHertz());
hashCode = 31 * hashCode + Objects.hashCode(mediaFormatAsString());
hashCode = 31 * hashCode + Objects.hashCode(media());
hashCode = 31 * hashCode + Objects.hashCode(outputBucketName());
hashCode = 31 * hashCode + Objects.hashCode(outputKey());
hashCode = 31 * hashCode + Objects.hashCode(outputEncryptionKMSKeyId());
hashCode = 31 * hashCode + Objects.hashCode(settings());
hashCode = 31 * hashCode + Objects.hashCode(modelSettings());
hashCode = 31 * hashCode + Objects.hashCode(jobExecutionSettings());
hashCode = 31 * hashCode + Objects.hashCode(contentRedaction());
hashCode = 31 * hashCode + Objects.hashCode(identifyLanguage());
hashCode = 31 * hashCode + Objects.hashCode(hasLanguageOptions() ? languageOptionsAsStrings() : null);
return hashCode;
}
@Override
public boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof StartTranscriptionJobRequest)) {
return false;
}
StartTranscriptionJobRequest other = (StartTranscriptionJobRequest) obj;
return Objects.equals(transcriptionJobName(), other.transcriptionJobName())
&& Objects.equals(languageCodeAsString(), other.languageCodeAsString())
&& Objects.equals(mediaSampleRateHertz(), other.mediaSampleRateHertz())
&& Objects.equals(mediaFormatAsString(), other.mediaFormatAsString()) && Objects.equals(media(), other.media())
&& Objects.equals(outputBucketName(), other.outputBucketName()) && Objects.equals(outputKey(), other.outputKey())
&& Objects.equals(outputEncryptionKMSKeyId(), other.outputEncryptionKMSKeyId())
&& Objects.equals(settings(), other.settings()) && Objects.equals(modelSettings(), other.modelSettings())
&& Objects.equals(jobExecutionSettings(), other.jobExecutionSettings())
&& Objects.equals(contentRedaction(), other.contentRedaction())
&& Objects.equals(identifyLanguage(), other.identifyLanguage())
&& hasLanguageOptions() == other.hasLanguageOptions()
&& Objects.equals(languageOptionsAsStrings(), other.languageOptionsAsStrings());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("StartTranscriptionJobRequest").add("TranscriptionJobName", transcriptionJobName())
.add("LanguageCode", languageCodeAsString()).add("MediaSampleRateHertz", mediaSampleRateHertz())
.add("MediaFormat", mediaFormatAsString()).add("Media", media()).add("OutputBucketName", outputBucketName())
.add("OutputKey", outputKey()).add("OutputEncryptionKMSKeyId", outputEncryptionKMSKeyId())
.add("Settings", settings()).add("ModelSettings", modelSettings())
.add("JobExecutionSettings", jobExecutionSettings()).add("ContentRedaction", contentRedaction())
.add("IdentifyLanguage", identifyLanguage())
.add("LanguageOptions", hasLanguageOptions() ? languageOptionsAsStrings() : null).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "TranscriptionJobName":
return Optional.ofNullable(clazz.cast(transcriptionJobName()));
case "LanguageCode":
return Optional.ofNullable(clazz.cast(languageCodeAsString()));
case "MediaSampleRateHertz":
return Optional.ofNullable(clazz.cast(mediaSampleRateHertz()));
case "MediaFormat":
return Optional.ofNullable(clazz.cast(mediaFormatAsString()));
case "Media":
return Optional.ofNullable(clazz.cast(media()));
case "OutputBucketName":
return Optional.ofNullable(clazz.cast(outputBucketName()));
case "OutputKey":
return Optional.ofNullable(clazz.cast(outputKey()));
case "OutputEncryptionKMSKeyId":
return Optional.ofNullable(clazz.cast(outputEncryptionKMSKeyId()));
case "Settings":
return Optional.ofNullable(clazz.cast(settings()));
case "ModelSettings":
return Optional.ofNullable(clazz.cast(modelSettings()));
case "JobExecutionSettings":
return Optional.ofNullable(clazz.cast(jobExecutionSettings()));
case "ContentRedaction":
return Optional.ofNullable(clazz.cast(contentRedaction()));
case "IdentifyLanguage":
return Optional.ofNullable(clazz.cast(identifyLanguage()));
case "LanguageOptions":
return Optional.ofNullable(clazz.cast(languageOptionsAsStrings()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function