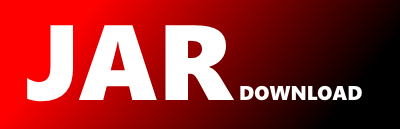
software.amazon.awssdk.services.transcribe.model.LanguageModel Maven / Gradle / Ivy
Show all versions of transcribe Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.transcribe.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The structure used to describe a custom language model.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class LanguageModel implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField MODEL_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ModelName").getter(getter(LanguageModel::modelName)).setter(setter(Builder::modelName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ModelName").build()).build();
private static final SdkField CREATE_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("CreateTime").getter(getter(LanguageModel::createTime)).setter(setter(Builder::createTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CreateTime").build()).build();
private static final SdkField LAST_MODIFIED_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("LastModifiedTime").getter(getter(LanguageModel::lastModifiedTime))
.setter(setter(Builder::lastModifiedTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LastModifiedTime").build()).build();
private static final SdkField LANGUAGE_CODE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("LanguageCode").getter(getter(LanguageModel::languageCodeAsString)).setter(setter(Builder::languageCode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LanguageCode").build()).build();
private static final SdkField BASE_MODEL_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("BaseModelName").getter(getter(LanguageModel::baseModelNameAsString))
.setter(setter(Builder::baseModelName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("BaseModelName").build()).build();
private static final SdkField MODEL_STATUS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ModelStatus").getter(getter(LanguageModel::modelStatusAsString)).setter(setter(Builder::modelStatus))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ModelStatus").build()).build();
private static final SdkField UPGRADE_AVAILABILITY_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("UpgradeAvailability").getter(getter(LanguageModel::upgradeAvailability))
.setter(setter(Builder::upgradeAvailability))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("UpgradeAvailability").build())
.build();
private static final SdkField FAILURE_REASON_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("FailureReason").getter(getter(LanguageModel::failureReason)).setter(setter(Builder::failureReason))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("FailureReason").build()).build();
private static final SdkField INPUT_DATA_CONFIG_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("InputDataConfig")
.getter(getter(LanguageModel::inputDataConfig)).setter(setter(Builder::inputDataConfig))
.constructor(InputDataConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InputDataConfig").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(MODEL_NAME_FIELD,
CREATE_TIME_FIELD, LAST_MODIFIED_TIME_FIELD, LANGUAGE_CODE_FIELD, BASE_MODEL_NAME_FIELD, MODEL_STATUS_FIELD,
UPGRADE_AVAILABILITY_FIELD, FAILURE_REASON_FIELD, INPUT_DATA_CONFIG_FIELD));
private static final long serialVersionUID = 1L;
private final String modelName;
private final Instant createTime;
private final Instant lastModifiedTime;
private final String languageCode;
private final String baseModelName;
private final String modelStatus;
private final Boolean upgradeAvailability;
private final String failureReason;
private final InputDataConfig inputDataConfig;
private LanguageModel(BuilderImpl builder) {
this.modelName = builder.modelName;
this.createTime = builder.createTime;
this.lastModifiedTime = builder.lastModifiedTime;
this.languageCode = builder.languageCode;
this.baseModelName = builder.baseModelName;
this.modelStatus = builder.modelStatus;
this.upgradeAvailability = builder.upgradeAvailability;
this.failureReason = builder.failureReason;
this.inputDataConfig = builder.inputDataConfig;
}
/**
*
* The name of the custom language model.
*
*
* @return The name of the custom language model.
*/
public String modelName() {
return modelName;
}
/**
*
* The time the custom language model was created.
*
*
* @return The time the custom language model was created.
*/
public Instant createTime() {
return createTime;
}
/**
*
* The most recent time the custom language model was modified.
*
*
* @return The most recent time the custom language model was modified.
*/
public Instant lastModifiedTime() {
return lastModifiedTime;
}
/**
*
* The language code you used to create your custom language model.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #languageCode} will
* return {@link CLMLanguageCode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #languageCodeAsString}.
*
*
* @return The language code you used to create your custom language model.
* @see CLMLanguageCode
*/
public CLMLanguageCode languageCode() {
return CLMLanguageCode.fromValue(languageCode);
}
/**
*
* The language code you used to create your custom language model.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #languageCode} will
* return {@link CLMLanguageCode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #languageCodeAsString}.
*
*
* @return The language code you used to create your custom language model.
* @see CLMLanguageCode
*/
public String languageCodeAsString() {
return languageCode;
}
/**
*
* The Amazon Transcribe standard language model, or base model used to create the custom language model.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #baseModelName}
* will return {@link BaseModelName#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #baseModelNameAsString}.
*
*
* @return The Amazon Transcribe standard language model, or base model used to create the custom language model.
* @see BaseModelName
*/
public BaseModelName baseModelName() {
return BaseModelName.fromValue(baseModelName);
}
/**
*
* The Amazon Transcribe standard language model, or base model used to create the custom language model.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #baseModelName}
* will return {@link BaseModelName#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #baseModelNameAsString}.
*
*
* @return The Amazon Transcribe standard language model, or base model used to create the custom language model.
* @see BaseModelName
*/
public String baseModelNameAsString() {
return baseModelName;
}
/**
*
* The creation status of a custom language model. When the status is COMPLETED
the model is ready for
* use.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #modelStatus} will
* return {@link ModelStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #modelStatusAsString}.
*
*
* @return The creation status of a custom language model. When the status is COMPLETED
the model is
* ready for use.
* @see ModelStatus
*/
public ModelStatus modelStatus() {
return ModelStatus.fromValue(modelStatus);
}
/**
*
* The creation status of a custom language model. When the status is COMPLETED
the model is ready for
* use.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #modelStatus} will
* return {@link ModelStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #modelStatusAsString}.
*
*
* @return The creation status of a custom language model. When the status is COMPLETED
the model is
* ready for use.
* @see ModelStatus
*/
public String modelStatusAsString() {
return modelStatus;
}
/**
*
* Whether the base model used for the custom language model is up to date. If this field is true
then
* you are running the most up-to-date version of the base model in your custom language model.
*
*
* @return Whether the base model used for the custom language model is up to date. If this field is
* true
then you are running the most up-to-date version of the base model in your custom
* language model.
*/
public Boolean upgradeAvailability() {
return upgradeAvailability;
}
/**
*
* The reason why the custom language model couldn't be created.
*
*
* @return The reason why the custom language model couldn't be created.
*/
public String failureReason() {
return failureReason;
}
/**
*
* The data access role and Amazon S3 prefixes for the input files used to train the custom language model.
*
*
* @return The data access role and Amazon S3 prefixes for the input files used to train the custom language model.
*/
public InputDataConfig inputDataConfig() {
return inputDataConfig;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(modelName());
hashCode = 31 * hashCode + Objects.hashCode(createTime());
hashCode = 31 * hashCode + Objects.hashCode(lastModifiedTime());
hashCode = 31 * hashCode + Objects.hashCode(languageCodeAsString());
hashCode = 31 * hashCode + Objects.hashCode(baseModelNameAsString());
hashCode = 31 * hashCode + Objects.hashCode(modelStatusAsString());
hashCode = 31 * hashCode + Objects.hashCode(upgradeAvailability());
hashCode = 31 * hashCode + Objects.hashCode(failureReason());
hashCode = 31 * hashCode + Objects.hashCode(inputDataConfig());
return hashCode;
}
@Override
public boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof LanguageModel)) {
return false;
}
LanguageModel other = (LanguageModel) obj;
return Objects.equals(modelName(), other.modelName()) && Objects.equals(createTime(), other.createTime())
&& Objects.equals(lastModifiedTime(), other.lastModifiedTime())
&& Objects.equals(languageCodeAsString(), other.languageCodeAsString())
&& Objects.equals(baseModelNameAsString(), other.baseModelNameAsString())
&& Objects.equals(modelStatusAsString(), other.modelStatusAsString())
&& Objects.equals(upgradeAvailability(), other.upgradeAvailability())
&& Objects.equals(failureReason(), other.failureReason())
&& Objects.equals(inputDataConfig(), other.inputDataConfig());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("LanguageModel").add("ModelName", modelName()).add("CreateTime", createTime())
.add("LastModifiedTime", lastModifiedTime()).add("LanguageCode", languageCodeAsString())
.add("BaseModelName", baseModelNameAsString()).add("ModelStatus", modelStatusAsString())
.add("UpgradeAvailability", upgradeAvailability()).add("FailureReason", failureReason())
.add("InputDataConfig", inputDataConfig()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ModelName":
return Optional.ofNullable(clazz.cast(modelName()));
case "CreateTime":
return Optional.ofNullable(clazz.cast(createTime()));
case "LastModifiedTime":
return Optional.ofNullable(clazz.cast(lastModifiedTime()));
case "LanguageCode":
return Optional.ofNullable(clazz.cast(languageCodeAsString()));
case "BaseModelName":
return Optional.ofNullable(clazz.cast(baseModelNameAsString()));
case "ModelStatus":
return Optional.ofNullable(clazz.cast(modelStatusAsString()));
case "UpgradeAvailability":
return Optional.ofNullable(clazz.cast(upgradeAvailability()));
case "FailureReason":
return Optional.ofNullable(clazz.cast(failureReason()));
case "InputDataConfig":
return Optional.ofNullable(clazz.cast(inputDataConfig()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function