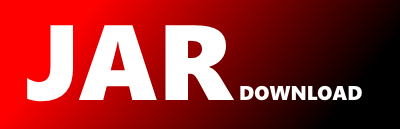
software.amazon.awssdk.services.transcribe.TranscribeClient Maven / Gradle / Ivy
Show all versions of transcribe Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.transcribe;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.core.SdkClient;
import software.amazon.awssdk.core.exception.SdkClientException;
import software.amazon.awssdk.regions.ServiceMetadata;
import software.amazon.awssdk.services.transcribe.model.BadRequestException;
import software.amazon.awssdk.services.transcribe.model.ConflictException;
import software.amazon.awssdk.services.transcribe.model.CreateLanguageModelRequest;
import software.amazon.awssdk.services.transcribe.model.CreateLanguageModelResponse;
import software.amazon.awssdk.services.transcribe.model.CreateMedicalVocabularyRequest;
import software.amazon.awssdk.services.transcribe.model.CreateMedicalVocabularyResponse;
import software.amazon.awssdk.services.transcribe.model.CreateVocabularyFilterRequest;
import software.amazon.awssdk.services.transcribe.model.CreateVocabularyFilterResponse;
import software.amazon.awssdk.services.transcribe.model.CreateVocabularyRequest;
import software.amazon.awssdk.services.transcribe.model.CreateVocabularyResponse;
import software.amazon.awssdk.services.transcribe.model.DeleteLanguageModelRequest;
import software.amazon.awssdk.services.transcribe.model.DeleteLanguageModelResponse;
import software.amazon.awssdk.services.transcribe.model.DeleteMedicalTranscriptionJobRequest;
import software.amazon.awssdk.services.transcribe.model.DeleteMedicalTranscriptionJobResponse;
import software.amazon.awssdk.services.transcribe.model.DeleteMedicalVocabularyRequest;
import software.amazon.awssdk.services.transcribe.model.DeleteMedicalVocabularyResponse;
import software.amazon.awssdk.services.transcribe.model.DeleteTranscriptionJobRequest;
import software.amazon.awssdk.services.transcribe.model.DeleteTranscriptionJobResponse;
import software.amazon.awssdk.services.transcribe.model.DeleteVocabularyFilterRequest;
import software.amazon.awssdk.services.transcribe.model.DeleteVocabularyFilterResponse;
import software.amazon.awssdk.services.transcribe.model.DeleteVocabularyRequest;
import software.amazon.awssdk.services.transcribe.model.DeleteVocabularyResponse;
import software.amazon.awssdk.services.transcribe.model.DescribeLanguageModelRequest;
import software.amazon.awssdk.services.transcribe.model.DescribeLanguageModelResponse;
import software.amazon.awssdk.services.transcribe.model.GetMedicalTranscriptionJobRequest;
import software.amazon.awssdk.services.transcribe.model.GetMedicalTranscriptionJobResponse;
import software.amazon.awssdk.services.transcribe.model.GetMedicalVocabularyRequest;
import software.amazon.awssdk.services.transcribe.model.GetMedicalVocabularyResponse;
import software.amazon.awssdk.services.transcribe.model.GetTranscriptionJobRequest;
import software.amazon.awssdk.services.transcribe.model.GetTranscriptionJobResponse;
import software.amazon.awssdk.services.transcribe.model.GetVocabularyFilterRequest;
import software.amazon.awssdk.services.transcribe.model.GetVocabularyFilterResponse;
import software.amazon.awssdk.services.transcribe.model.GetVocabularyRequest;
import software.amazon.awssdk.services.transcribe.model.GetVocabularyResponse;
import software.amazon.awssdk.services.transcribe.model.InternalFailureException;
import software.amazon.awssdk.services.transcribe.model.LimitExceededException;
import software.amazon.awssdk.services.transcribe.model.ListLanguageModelsRequest;
import software.amazon.awssdk.services.transcribe.model.ListLanguageModelsResponse;
import software.amazon.awssdk.services.transcribe.model.ListMedicalTranscriptionJobsRequest;
import software.amazon.awssdk.services.transcribe.model.ListMedicalTranscriptionJobsResponse;
import software.amazon.awssdk.services.transcribe.model.ListMedicalVocabulariesRequest;
import software.amazon.awssdk.services.transcribe.model.ListMedicalVocabulariesResponse;
import software.amazon.awssdk.services.transcribe.model.ListTranscriptionJobsRequest;
import software.amazon.awssdk.services.transcribe.model.ListTranscriptionJobsResponse;
import software.amazon.awssdk.services.transcribe.model.ListVocabulariesRequest;
import software.amazon.awssdk.services.transcribe.model.ListVocabulariesResponse;
import software.amazon.awssdk.services.transcribe.model.ListVocabularyFiltersRequest;
import software.amazon.awssdk.services.transcribe.model.ListVocabularyFiltersResponse;
import software.amazon.awssdk.services.transcribe.model.NotFoundException;
import software.amazon.awssdk.services.transcribe.model.StartMedicalTranscriptionJobRequest;
import software.amazon.awssdk.services.transcribe.model.StartMedicalTranscriptionJobResponse;
import software.amazon.awssdk.services.transcribe.model.StartTranscriptionJobRequest;
import software.amazon.awssdk.services.transcribe.model.StartTranscriptionJobResponse;
import software.amazon.awssdk.services.transcribe.model.TranscribeException;
import software.amazon.awssdk.services.transcribe.model.UpdateMedicalVocabularyRequest;
import software.amazon.awssdk.services.transcribe.model.UpdateMedicalVocabularyResponse;
import software.amazon.awssdk.services.transcribe.model.UpdateVocabularyFilterRequest;
import software.amazon.awssdk.services.transcribe.model.UpdateVocabularyFilterResponse;
import software.amazon.awssdk.services.transcribe.model.UpdateVocabularyRequest;
import software.amazon.awssdk.services.transcribe.model.UpdateVocabularyResponse;
import software.amazon.awssdk.services.transcribe.paginators.ListLanguageModelsIterable;
import software.amazon.awssdk.services.transcribe.paginators.ListMedicalTranscriptionJobsIterable;
import software.amazon.awssdk.services.transcribe.paginators.ListMedicalVocabulariesIterable;
import software.amazon.awssdk.services.transcribe.paginators.ListTranscriptionJobsIterable;
import software.amazon.awssdk.services.transcribe.paginators.ListVocabulariesIterable;
import software.amazon.awssdk.services.transcribe.paginators.ListVocabularyFiltersIterable;
/**
* Service client for accessing Amazon Transcribe Service. This can be created using the static {@link #builder()}
* method.
*
*
* Operations and objects for transcribing speech to text.
*
*/
@Generated("software.amazon.awssdk:codegen")
public interface TranscribeClient extends SdkClient {
String SERVICE_NAME = "transcribe";
/**
* Create a {@link TranscribeClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static TranscribeClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link TranscribeClient}.
*/
static TranscribeClientBuilder builder() {
return new DefaultTranscribeClientBuilder();
}
/**
*
* Creates a new custom language model. Use Amazon S3 prefixes to provide the location of your input files. The time
* it takes to create your model depends on the size of your training data.
*
*
* @param createLanguageModelRequest
* @return Result of the CreateLanguageModel operation returned by the service.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the entity that you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws ConflictException
* There is already a resource with that name.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.CreateLanguageModel
* @see AWS
* API Documentation
*/
default CreateLanguageModelResponse createLanguageModel(CreateLanguageModelRequest createLanguageModelRequest)
throws BadRequestException, LimitExceededException, InternalFailureException, ConflictException, AwsServiceException,
SdkClientException, TranscribeException {
throw new UnsupportedOperationException();
}
/**
*
* Creates a new custom language model. Use Amazon S3 prefixes to provide the location of your input files. The time
* it takes to create your model depends on the size of your training data.
*
*
*
* This is a convenience which creates an instance of the {@link CreateLanguageModelRequest.Builder} avoiding the
* need to create one manually via {@link CreateLanguageModelRequest#builder()}
*
*
* @param createLanguageModelRequest
* A {@link Consumer} that will call methods on {@link CreateLanguageModelRequest.Builder} to create a
* request.
* @return Result of the CreateLanguageModel operation returned by the service.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the entity that you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws ConflictException
* There is already a resource with that name.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.CreateLanguageModel
* @see AWS
* API Documentation
*/
default CreateLanguageModelResponse createLanguageModel(
Consumer createLanguageModelRequest) throws BadRequestException,
LimitExceededException, InternalFailureException, ConflictException, AwsServiceException, SdkClientException,
TranscribeException {
return createLanguageModel(CreateLanguageModelRequest.builder().applyMutation(createLanguageModelRequest).build());
}
/**
*
* Creates a new custom vocabulary that you can use to change how Amazon Transcribe Medical transcribes your audio
* file.
*
*
* @param createMedicalVocabularyRequest
* @return Result of the CreateMedicalVocabulary operation returned by the service.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the entity that you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws ConflictException
* There is already a resource with that name.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.CreateMedicalVocabulary
* @see AWS API Documentation
*/
default CreateMedicalVocabularyResponse createMedicalVocabulary(CreateMedicalVocabularyRequest createMedicalVocabularyRequest)
throws BadRequestException, LimitExceededException, InternalFailureException, ConflictException, AwsServiceException,
SdkClientException, TranscribeException {
throw new UnsupportedOperationException();
}
/**
*
* Creates a new custom vocabulary that you can use to change how Amazon Transcribe Medical transcribes your audio
* file.
*
*
*
* This is a convenience which creates an instance of the {@link CreateMedicalVocabularyRequest.Builder} avoiding
* the need to create one manually via {@link CreateMedicalVocabularyRequest#builder()}
*
*
* @param createMedicalVocabularyRequest
* A {@link Consumer} that will call methods on {@link CreateMedicalVocabularyRequest.Builder} to create a
* request.
* @return Result of the CreateMedicalVocabulary operation returned by the service.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the entity that you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws ConflictException
* There is already a resource with that name.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.CreateMedicalVocabulary
* @see AWS API Documentation
*/
default CreateMedicalVocabularyResponse createMedicalVocabulary(
Consumer createMedicalVocabularyRequest) throws BadRequestException,
LimitExceededException, InternalFailureException, ConflictException, AwsServiceException, SdkClientException,
TranscribeException {
return createMedicalVocabulary(CreateMedicalVocabularyRequest.builder().applyMutation(createMedicalVocabularyRequest)
.build());
}
/**
*
* Creates a new custom vocabulary that you can use to change the way Amazon Transcribe handles transcription of an
* audio file.
*
*
* @param createVocabularyRequest
* @return Result of the CreateVocabulary operation returned by the service.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the entity that you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws ConflictException
* There is already a resource with that name.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.CreateVocabulary
* @see AWS
* API Documentation
*/
default CreateVocabularyResponse createVocabulary(CreateVocabularyRequest createVocabularyRequest)
throws BadRequestException, LimitExceededException, InternalFailureException, ConflictException, AwsServiceException,
SdkClientException, TranscribeException {
throw new UnsupportedOperationException();
}
/**
*
* Creates a new custom vocabulary that you can use to change the way Amazon Transcribe handles transcription of an
* audio file.
*
*
*
* This is a convenience which creates an instance of the {@link CreateVocabularyRequest.Builder} avoiding the need
* to create one manually via {@link CreateVocabularyRequest#builder()}
*
*
* @param createVocabularyRequest
* A {@link Consumer} that will call methods on {@link CreateVocabularyRequest.Builder} to create a request.
* @return Result of the CreateVocabulary operation returned by the service.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the entity that you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws ConflictException
* There is already a resource with that name.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.CreateVocabulary
* @see AWS
* API Documentation
*/
default CreateVocabularyResponse createVocabulary(Consumer createVocabularyRequest)
throws BadRequestException, LimitExceededException, InternalFailureException, ConflictException, AwsServiceException,
SdkClientException, TranscribeException {
return createVocabulary(CreateVocabularyRequest.builder().applyMutation(createVocabularyRequest).build());
}
/**
*
* Creates a new vocabulary filter that you can use to filter words, such as profane words, from the output of a
* transcription job.
*
*
* @param createVocabularyFilterRequest
* @return Result of the CreateVocabularyFilter operation returned by the service.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the entity that you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws ConflictException
* There is already a resource with that name.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.CreateVocabularyFilter
* @see AWS API Documentation
*/
default CreateVocabularyFilterResponse createVocabularyFilter(CreateVocabularyFilterRequest createVocabularyFilterRequest)
throws BadRequestException, LimitExceededException, InternalFailureException, ConflictException, AwsServiceException,
SdkClientException, TranscribeException {
throw new UnsupportedOperationException();
}
/**
*
* Creates a new vocabulary filter that you can use to filter words, such as profane words, from the output of a
* transcription job.
*
*
*
* This is a convenience which creates an instance of the {@link CreateVocabularyFilterRequest.Builder} avoiding the
* need to create one manually via {@link CreateVocabularyFilterRequest#builder()}
*
*
* @param createVocabularyFilterRequest
* A {@link Consumer} that will call methods on {@link CreateVocabularyFilterRequest.Builder} to create a
* request.
* @return Result of the CreateVocabularyFilter operation returned by the service.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the entity that you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws ConflictException
* There is already a resource with that name.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.CreateVocabularyFilter
* @see AWS API Documentation
*/
default CreateVocabularyFilterResponse createVocabularyFilter(
Consumer createVocabularyFilterRequest) throws BadRequestException,
LimitExceededException, InternalFailureException, ConflictException, AwsServiceException, SdkClientException,
TranscribeException {
return createVocabularyFilter(CreateVocabularyFilterRequest.builder().applyMutation(createVocabularyFilterRequest)
.build());
}
/**
*
* Deletes a custom language model using its name.
*
*
* @param deleteLanguageModelRequest
* @return Result of the DeleteLanguageModel operation returned by the service.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the entity that you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.DeleteLanguageModel
* @see AWS
* API Documentation
*/
default DeleteLanguageModelResponse deleteLanguageModel(DeleteLanguageModelRequest deleteLanguageModelRequest)
throws BadRequestException, LimitExceededException, InternalFailureException, AwsServiceException,
SdkClientException, TranscribeException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a custom language model using its name.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteLanguageModelRequest.Builder} avoiding the
* need to create one manually via {@link DeleteLanguageModelRequest#builder()}
*
*
* @param deleteLanguageModelRequest
* A {@link Consumer} that will call methods on {@link DeleteLanguageModelRequest.Builder} to create a
* request.
* @return Result of the DeleteLanguageModel operation returned by the service.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the entity that you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.DeleteLanguageModel
* @see AWS
* API Documentation
*/
default DeleteLanguageModelResponse deleteLanguageModel(
Consumer deleteLanguageModelRequest) throws BadRequestException,
LimitExceededException, InternalFailureException, AwsServiceException, SdkClientException, TranscribeException {
return deleteLanguageModel(DeleteLanguageModelRequest.builder().applyMutation(deleteLanguageModelRequest).build());
}
/**
*
* Deletes a transcription job generated by Amazon Transcribe Medical and any related information.
*
*
* @param deleteMedicalTranscriptionJobRequest
* @return Result of the DeleteMedicalTranscriptionJob operation returned by the service.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the entity that you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.DeleteMedicalTranscriptionJob
* @see AWS API Documentation
*/
default DeleteMedicalTranscriptionJobResponse deleteMedicalTranscriptionJob(
DeleteMedicalTranscriptionJobRequest deleteMedicalTranscriptionJobRequest) throws LimitExceededException,
BadRequestException, InternalFailureException, AwsServiceException, SdkClientException, TranscribeException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a transcription job generated by Amazon Transcribe Medical and any related information.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteMedicalTranscriptionJobRequest.Builder}
* avoiding the need to create one manually via {@link DeleteMedicalTranscriptionJobRequest#builder()}
*
*
* @param deleteMedicalTranscriptionJobRequest
* A {@link Consumer} that will call methods on {@link DeleteMedicalTranscriptionJobRequest.Builder} to
* create a request.
* @return Result of the DeleteMedicalTranscriptionJob operation returned by the service.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the entity that you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.DeleteMedicalTranscriptionJob
* @see AWS API Documentation
*/
default DeleteMedicalTranscriptionJobResponse deleteMedicalTranscriptionJob(
Consumer deleteMedicalTranscriptionJobRequest)
throws LimitExceededException, BadRequestException, InternalFailureException, AwsServiceException,
SdkClientException, TranscribeException {
return deleteMedicalTranscriptionJob(DeleteMedicalTranscriptionJobRequest.builder()
.applyMutation(deleteMedicalTranscriptionJobRequest).build());
}
/**
*
* Deletes a vocabulary from Amazon Transcribe Medical.
*
*
* @param deleteMedicalVocabularyRequest
* @return Result of the DeleteMedicalVocabulary operation returned by the service.
* @throws NotFoundException
* We can't find the requested resource. Check the name and try your request again.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the entity that you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.DeleteMedicalVocabulary
* @see AWS API Documentation
*/
default DeleteMedicalVocabularyResponse deleteMedicalVocabulary(DeleteMedicalVocabularyRequest deleteMedicalVocabularyRequest)
throws NotFoundException, LimitExceededException, BadRequestException, InternalFailureException, AwsServiceException,
SdkClientException, TranscribeException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a vocabulary from Amazon Transcribe Medical.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteMedicalVocabularyRequest.Builder} avoiding
* the need to create one manually via {@link DeleteMedicalVocabularyRequest#builder()}
*
*
* @param deleteMedicalVocabularyRequest
* A {@link Consumer} that will call methods on {@link DeleteMedicalVocabularyRequest.Builder} to create a
* request.
* @return Result of the DeleteMedicalVocabulary operation returned by the service.
* @throws NotFoundException
* We can't find the requested resource. Check the name and try your request again.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the entity that you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.DeleteMedicalVocabulary
* @see AWS API Documentation
*/
default DeleteMedicalVocabularyResponse deleteMedicalVocabulary(
Consumer deleteMedicalVocabularyRequest) throws NotFoundException,
LimitExceededException, BadRequestException, InternalFailureException, AwsServiceException, SdkClientException,
TranscribeException {
return deleteMedicalVocabulary(DeleteMedicalVocabularyRequest.builder().applyMutation(deleteMedicalVocabularyRequest)
.build());
}
/**
*
* Deletes a previously submitted transcription job along with any other generated results such as the
* transcription, models, and so on.
*
*
* @param deleteTranscriptionJobRequest
* @return Result of the DeleteTranscriptionJob operation returned by the service.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the entity that you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.DeleteTranscriptionJob
* @see AWS API Documentation
*/
default DeleteTranscriptionJobResponse deleteTranscriptionJob(DeleteTranscriptionJobRequest deleteTranscriptionJobRequest)
throws LimitExceededException, BadRequestException, InternalFailureException, AwsServiceException,
SdkClientException, TranscribeException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a previously submitted transcription job along with any other generated results such as the
* transcription, models, and so on.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteTranscriptionJobRequest.Builder} avoiding the
* need to create one manually via {@link DeleteTranscriptionJobRequest#builder()}
*
*
* @param deleteTranscriptionJobRequest
* A {@link Consumer} that will call methods on {@link DeleteTranscriptionJobRequest.Builder} to create a
* request.
* @return Result of the DeleteTranscriptionJob operation returned by the service.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the entity that you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.DeleteTranscriptionJob
* @see AWS API Documentation
*/
default DeleteTranscriptionJobResponse deleteTranscriptionJob(
Consumer deleteTranscriptionJobRequest) throws LimitExceededException,
BadRequestException, InternalFailureException, AwsServiceException, SdkClientException, TranscribeException {
return deleteTranscriptionJob(DeleteTranscriptionJobRequest.builder().applyMutation(deleteTranscriptionJobRequest)
.build());
}
/**
*
* Deletes a vocabulary from Amazon Transcribe.
*
*
* @param deleteVocabularyRequest
* @return Result of the DeleteVocabulary operation returned by the service.
* @throws NotFoundException
* We can't find the requested resource. Check the name and try your request again.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the entity that you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.DeleteVocabulary
* @see AWS
* API Documentation
*/
default DeleteVocabularyResponse deleteVocabulary(DeleteVocabularyRequest deleteVocabularyRequest) throws NotFoundException,
LimitExceededException, BadRequestException, InternalFailureException, AwsServiceException, SdkClientException,
TranscribeException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a vocabulary from Amazon Transcribe.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteVocabularyRequest.Builder} avoiding the need
* to create one manually via {@link DeleteVocabularyRequest#builder()}
*
*
* @param deleteVocabularyRequest
* A {@link Consumer} that will call methods on {@link DeleteVocabularyRequest.Builder} to create a request.
* @return Result of the DeleteVocabulary operation returned by the service.
* @throws NotFoundException
* We can't find the requested resource. Check the name and try your request again.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the entity that you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.DeleteVocabulary
* @see AWS
* API Documentation
*/
default DeleteVocabularyResponse deleteVocabulary(Consumer deleteVocabularyRequest)
throws NotFoundException, LimitExceededException, BadRequestException, InternalFailureException, AwsServiceException,
SdkClientException, TranscribeException {
return deleteVocabulary(DeleteVocabularyRequest.builder().applyMutation(deleteVocabularyRequest).build());
}
/**
*
* Removes a vocabulary filter.
*
*
* @param deleteVocabularyFilterRequest
* @return Result of the DeleteVocabularyFilter operation returned by the service.
* @throws NotFoundException
* We can't find the requested resource. Check the name and try your request again.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the entity that you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.DeleteVocabularyFilter
* @see AWS API Documentation
*/
default DeleteVocabularyFilterResponse deleteVocabularyFilter(DeleteVocabularyFilterRequest deleteVocabularyFilterRequest)
throws NotFoundException, LimitExceededException, BadRequestException, InternalFailureException, AwsServiceException,
SdkClientException, TranscribeException {
throw new UnsupportedOperationException();
}
/**
*
* Removes a vocabulary filter.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteVocabularyFilterRequest.Builder} avoiding the
* need to create one manually via {@link DeleteVocabularyFilterRequest#builder()}
*
*
* @param deleteVocabularyFilterRequest
* A {@link Consumer} that will call methods on {@link DeleteVocabularyFilterRequest.Builder} to create a
* request.
* @return Result of the DeleteVocabularyFilter operation returned by the service.
* @throws NotFoundException
* We can't find the requested resource. Check the name and try your request again.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the entity that you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.DeleteVocabularyFilter
* @see AWS API Documentation
*/
default DeleteVocabularyFilterResponse deleteVocabularyFilter(
Consumer deleteVocabularyFilterRequest) throws NotFoundException,
LimitExceededException, BadRequestException, InternalFailureException, AwsServiceException, SdkClientException,
TranscribeException {
return deleteVocabularyFilter(DeleteVocabularyFilterRequest.builder().applyMutation(deleteVocabularyFilterRequest)
.build());
}
/**
*
* Gets information about a single custom language model. Use this information to see details about the language
* model in your AWS account. You can also see whether the base language model used to create your custom language
* model has been updated. If Amazon Transcribe has updated the base model, you can create a new custom language
* model using the updated base model. If the language model wasn't created, you can use this operation to
* understand why Amazon Transcribe couldn't create it.
*
*
* @param describeLanguageModelRequest
* @return Result of the DescribeLanguageModel operation returned by the service.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the entity that you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws NotFoundException
* We can't find the requested resource. Check the name and try your request again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.DescribeLanguageModel
* @see AWS API Documentation
*/
default DescribeLanguageModelResponse describeLanguageModel(DescribeLanguageModelRequest describeLanguageModelRequest)
throws BadRequestException, LimitExceededException, InternalFailureException, NotFoundException, AwsServiceException,
SdkClientException, TranscribeException {
throw new UnsupportedOperationException();
}
/**
*
* Gets information about a single custom language model. Use this information to see details about the language
* model in your AWS account. You can also see whether the base language model used to create your custom language
* model has been updated. If Amazon Transcribe has updated the base model, you can create a new custom language
* model using the updated base model. If the language model wasn't created, you can use this operation to
* understand why Amazon Transcribe couldn't create it.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeLanguageModelRequest.Builder} avoiding the
* need to create one manually via {@link DescribeLanguageModelRequest#builder()}
*
*
* @param describeLanguageModelRequest
* A {@link Consumer} that will call methods on {@link DescribeLanguageModelRequest.Builder} to create a
* request.
* @return Result of the DescribeLanguageModel operation returned by the service.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the entity that you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws NotFoundException
* We can't find the requested resource. Check the name and try your request again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.DescribeLanguageModel
* @see AWS API Documentation
*/
default DescribeLanguageModelResponse describeLanguageModel(
Consumer describeLanguageModelRequest) throws BadRequestException,
LimitExceededException, InternalFailureException, NotFoundException, AwsServiceException, SdkClientException,
TranscribeException {
return describeLanguageModel(DescribeLanguageModelRequest.builder().applyMutation(describeLanguageModelRequest).build());
}
/**
*
* Returns information about a transcription job from Amazon Transcribe Medical. To see the status of the job, check
* the TranscriptionJobStatus
field. If the status is COMPLETED
, the job is finished. You
* find the results of the completed job in the TranscriptFileUri
field.
*
*
* @param getMedicalTranscriptionJobRequest
* @return Result of the GetMedicalTranscriptionJob operation returned by the service.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the entity that you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws NotFoundException
* We can't find the requested resource. Check the name and try your request again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.GetMedicalTranscriptionJob
* @see AWS API Documentation
*/
default GetMedicalTranscriptionJobResponse getMedicalTranscriptionJob(
GetMedicalTranscriptionJobRequest getMedicalTranscriptionJobRequest) throws BadRequestException,
LimitExceededException, InternalFailureException, NotFoundException, AwsServiceException, SdkClientException,
TranscribeException {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about a transcription job from Amazon Transcribe Medical. To see the status of the job, check
* the TranscriptionJobStatus
field. If the status is COMPLETED
, the job is finished. You
* find the results of the completed job in the TranscriptFileUri
field.
*
*
*
* This is a convenience which creates an instance of the {@link GetMedicalTranscriptionJobRequest.Builder} avoiding
* the need to create one manually via {@link GetMedicalTranscriptionJobRequest#builder()}
*
*
* @param getMedicalTranscriptionJobRequest
* A {@link Consumer} that will call methods on {@link GetMedicalTranscriptionJobRequest.Builder} to create a
* request.
* @return Result of the GetMedicalTranscriptionJob operation returned by the service.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the entity that you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws NotFoundException
* We can't find the requested resource. Check the name and try your request again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.GetMedicalTranscriptionJob
* @see AWS API Documentation
*/
default GetMedicalTranscriptionJobResponse getMedicalTranscriptionJob(
Consumer getMedicalTranscriptionJobRequest) throws BadRequestException,
LimitExceededException, InternalFailureException, NotFoundException, AwsServiceException, SdkClientException,
TranscribeException {
return getMedicalTranscriptionJob(GetMedicalTranscriptionJobRequest.builder()
.applyMutation(getMedicalTranscriptionJobRequest).build());
}
/**
*
* Retrieves information about a medical vocabulary.
*
*
* @param getMedicalVocabularyRequest
* @return Result of the GetMedicalVocabulary operation returned by the service.
* @throws NotFoundException
* We can't find the requested resource. Check the name and try your request again.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the entity that you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.GetMedicalVocabulary
* @see AWS API Documentation
*/
default GetMedicalVocabularyResponse getMedicalVocabulary(GetMedicalVocabularyRequest getMedicalVocabularyRequest)
throws NotFoundException, LimitExceededException, InternalFailureException, BadRequestException, AwsServiceException,
SdkClientException, TranscribeException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves information about a medical vocabulary.
*
*
*
* This is a convenience which creates an instance of the {@link GetMedicalVocabularyRequest.Builder} avoiding the
* need to create one manually via {@link GetMedicalVocabularyRequest#builder()}
*
*
* @param getMedicalVocabularyRequest
* A {@link Consumer} that will call methods on {@link GetMedicalVocabularyRequest.Builder} to create a
* request.
* @return Result of the GetMedicalVocabulary operation returned by the service.
* @throws NotFoundException
* We can't find the requested resource. Check the name and try your request again.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the entity that you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.GetMedicalVocabulary
* @see AWS API Documentation
*/
default GetMedicalVocabularyResponse getMedicalVocabulary(
Consumer getMedicalVocabularyRequest) throws NotFoundException,
LimitExceededException, InternalFailureException, BadRequestException, AwsServiceException, SdkClientException,
TranscribeException {
return getMedicalVocabulary(GetMedicalVocabularyRequest.builder().applyMutation(getMedicalVocabularyRequest).build());
}
/**
*
* Returns information about a transcription job. To see the status of the job, check the
* TranscriptionJobStatus
field. If the status is COMPLETED
, the job is finished and you
* can find the results at the location specified in the TranscriptFileUri
field. If you enable content
* redaction, the redacted transcript appears in RedactedTranscriptFileUri
.
*
*
* @param getTranscriptionJobRequest
* @return Result of the GetTranscriptionJob operation returned by the service.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the entity that you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws NotFoundException
* We can't find the requested resource. Check the name and try your request again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.GetTranscriptionJob
* @see AWS
* API Documentation
*/
default GetTranscriptionJobResponse getTranscriptionJob(GetTranscriptionJobRequest getTranscriptionJobRequest)
throws BadRequestException, LimitExceededException, InternalFailureException, NotFoundException, AwsServiceException,
SdkClientException, TranscribeException {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about a transcription job. To see the status of the job, check the
* TranscriptionJobStatus
field. If the status is COMPLETED
, the job is finished and you
* can find the results at the location specified in the TranscriptFileUri
field. If you enable content
* redaction, the redacted transcript appears in RedactedTranscriptFileUri
.
*
*
*
* This is a convenience which creates an instance of the {@link GetTranscriptionJobRequest.Builder} avoiding the
* need to create one manually via {@link GetTranscriptionJobRequest#builder()}
*
*
* @param getTranscriptionJobRequest
* A {@link Consumer} that will call methods on {@link GetTranscriptionJobRequest.Builder} to create a
* request.
* @return Result of the GetTranscriptionJob operation returned by the service.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the entity that you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws NotFoundException
* We can't find the requested resource. Check the name and try your request again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.GetTranscriptionJob
* @see AWS
* API Documentation
*/
default GetTranscriptionJobResponse getTranscriptionJob(
Consumer getTranscriptionJobRequest) throws BadRequestException,
LimitExceededException, InternalFailureException, NotFoundException, AwsServiceException, SdkClientException,
TranscribeException {
return getTranscriptionJob(GetTranscriptionJobRequest.builder().applyMutation(getTranscriptionJobRequest).build());
}
/**
*
* Gets information about a vocabulary.
*
*
* @param getVocabularyRequest
* @return Result of the GetVocabulary operation returned by the service.
* @throws NotFoundException
* We can't find the requested resource. Check the name and try your request again.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the entity that you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.GetVocabulary
* @see AWS API
* Documentation
*/
default GetVocabularyResponse getVocabulary(GetVocabularyRequest getVocabularyRequest) throws NotFoundException,
LimitExceededException, InternalFailureException, BadRequestException, AwsServiceException, SdkClientException,
TranscribeException {
throw new UnsupportedOperationException();
}
/**
*
* Gets information about a vocabulary.
*
*
*
* This is a convenience which creates an instance of the {@link GetVocabularyRequest.Builder} avoiding the need to
* create one manually via {@link GetVocabularyRequest#builder()}
*
*
* @param getVocabularyRequest
* A {@link Consumer} that will call methods on {@link GetVocabularyRequest.Builder} to create a request.
* @return Result of the GetVocabulary operation returned by the service.
* @throws NotFoundException
* We can't find the requested resource. Check the name and try your request again.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the entity that you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.GetVocabulary
* @see AWS API
* Documentation
*/
default GetVocabularyResponse getVocabulary(Consumer getVocabularyRequest)
throws NotFoundException, LimitExceededException, InternalFailureException, BadRequestException, AwsServiceException,
SdkClientException, TranscribeException {
return getVocabulary(GetVocabularyRequest.builder().applyMutation(getVocabularyRequest).build());
}
/**
*
* Returns information about a vocabulary filter.
*
*
* @param getVocabularyFilterRequest
* @return Result of the GetVocabularyFilter operation returned by the service.
* @throws NotFoundException
* We can't find the requested resource. Check the name and try your request again.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the entity that you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.GetVocabularyFilter
* @see AWS
* API Documentation
*/
default GetVocabularyFilterResponse getVocabularyFilter(GetVocabularyFilterRequest getVocabularyFilterRequest)
throws NotFoundException, LimitExceededException, InternalFailureException, BadRequestException, AwsServiceException,
SdkClientException, TranscribeException {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about a vocabulary filter.
*
*
*
* This is a convenience which creates an instance of the {@link GetVocabularyFilterRequest.Builder} avoiding the
* need to create one manually via {@link GetVocabularyFilterRequest#builder()}
*
*
* @param getVocabularyFilterRequest
* A {@link Consumer} that will call methods on {@link GetVocabularyFilterRequest.Builder} to create a
* request.
* @return Result of the GetVocabularyFilter operation returned by the service.
* @throws NotFoundException
* We can't find the requested resource. Check the name and try your request again.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the entity that you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.GetVocabularyFilter
* @see AWS
* API Documentation
*/
default GetVocabularyFilterResponse getVocabularyFilter(
Consumer getVocabularyFilterRequest) throws NotFoundException,
LimitExceededException, InternalFailureException, BadRequestException, AwsServiceException, SdkClientException,
TranscribeException {
return getVocabularyFilter(GetVocabularyFilterRequest.builder().applyMutation(getVocabularyFilterRequest).build());
}
/**
*
* Provides more information about the custom language models you've created. You can use the information in this
* list to find a specific custom language model. You can then use the operation to get more information about it.
*
*
* @param listLanguageModelsRequest
* @return Result of the ListLanguageModels operation returned by the service.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the entity that you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.ListLanguageModels
* @see AWS
* API Documentation
*/
default ListLanguageModelsResponse listLanguageModels(ListLanguageModelsRequest listLanguageModelsRequest)
throws BadRequestException, LimitExceededException, InternalFailureException, AwsServiceException,
SdkClientException, TranscribeException {
throw new UnsupportedOperationException();
}
/**
*
* Provides more information about the custom language models you've created. You can use the information in this
* list to find a specific custom language model. You can then use the operation to get more information about it.
*
*
*
* This is a convenience which creates an instance of the {@link ListLanguageModelsRequest.Builder} avoiding the
* need to create one manually via {@link ListLanguageModelsRequest#builder()}
*
*
* @param listLanguageModelsRequest
* A {@link Consumer} that will call methods on {@link ListLanguageModelsRequest.Builder} to create a
* request.
* @return Result of the ListLanguageModels operation returned by the service.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the entity that you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.ListLanguageModels
* @see AWS
* API Documentation
*/
default ListLanguageModelsResponse listLanguageModels(Consumer listLanguageModelsRequest)
throws BadRequestException, LimitExceededException, InternalFailureException, AwsServiceException,
SdkClientException, TranscribeException {
return listLanguageModels(ListLanguageModelsRequest.builder().applyMutation(listLanguageModelsRequest).build());
}
/**
*
* Provides more information about the custom language models you've created. You can use the information in this
* list to find a specific custom language model. You can then use the operation to get more information about it.
*
*
*
* This is a variant of
* {@link #listLanguageModels(software.amazon.awssdk.services.transcribe.model.ListLanguageModelsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.transcribe.paginators.ListLanguageModelsIterable responses = client.listLanguageModelsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.transcribe.paginators.ListLanguageModelsIterable responses = client
* .listLanguageModelsPaginator(request);
* for (software.amazon.awssdk.services.transcribe.model.ListLanguageModelsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.transcribe.paginators.ListLanguageModelsIterable responses = client.listLanguageModelsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listLanguageModels(software.amazon.awssdk.services.transcribe.model.ListLanguageModelsRequest)}
* operation.
*
*
* @param listLanguageModelsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the entity that you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.ListLanguageModels
* @see AWS
* API Documentation
*/
default ListLanguageModelsIterable listLanguageModelsPaginator(ListLanguageModelsRequest listLanguageModelsRequest)
throws BadRequestException, LimitExceededException, InternalFailureException, AwsServiceException,
SdkClientException, TranscribeException {
throw new UnsupportedOperationException();
}
/**
*
* Provides more information about the custom language models you've created. You can use the information in this
* list to find a specific custom language model. You can then use the operation to get more information about it.
*
*
*
* This is a variant of
* {@link #listLanguageModels(software.amazon.awssdk.services.transcribe.model.ListLanguageModelsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.transcribe.paginators.ListLanguageModelsIterable responses = client.listLanguageModelsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.transcribe.paginators.ListLanguageModelsIterable responses = client
* .listLanguageModelsPaginator(request);
* for (software.amazon.awssdk.services.transcribe.model.ListLanguageModelsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.transcribe.paginators.ListLanguageModelsIterable responses = client.listLanguageModelsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listLanguageModels(software.amazon.awssdk.services.transcribe.model.ListLanguageModelsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListLanguageModelsRequest.Builder} avoiding the
* need to create one manually via {@link ListLanguageModelsRequest#builder()}
*
*
* @param listLanguageModelsRequest
* A {@link Consumer} that will call methods on {@link ListLanguageModelsRequest.Builder} to create a
* request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the entity that you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.ListLanguageModels
* @see AWS
* API Documentation
*/
default ListLanguageModelsIterable listLanguageModelsPaginator(
Consumer listLanguageModelsRequest) throws BadRequestException,
LimitExceededException, InternalFailureException, AwsServiceException, SdkClientException, TranscribeException {
return listLanguageModelsPaginator(ListLanguageModelsRequest.builder().applyMutation(listLanguageModelsRequest).build());
}
/**
*
* Lists medical transcription jobs with a specified status or substring that matches their names.
*
*
* @param listMedicalTranscriptionJobsRequest
* @return Result of the ListMedicalTranscriptionJobs operation returned by the service.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the entity that you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.ListMedicalTranscriptionJobs
* @see AWS API Documentation
*/
default ListMedicalTranscriptionJobsResponse listMedicalTranscriptionJobs(
ListMedicalTranscriptionJobsRequest listMedicalTranscriptionJobsRequest) throws BadRequestException,
LimitExceededException, InternalFailureException, AwsServiceException, SdkClientException, TranscribeException {
throw new UnsupportedOperationException();
}
/**
*
* Lists medical transcription jobs with a specified status or substring that matches their names.
*
*
*
* This is a convenience which creates an instance of the {@link ListMedicalTranscriptionJobsRequest.Builder}
* avoiding the need to create one manually via {@link ListMedicalTranscriptionJobsRequest#builder()}
*
*
* @param listMedicalTranscriptionJobsRequest
* A {@link Consumer} that will call methods on {@link ListMedicalTranscriptionJobsRequest.Builder} to create
* a request.
* @return Result of the ListMedicalTranscriptionJobs operation returned by the service.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the entity that you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.ListMedicalTranscriptionJobs
* @see AWS API Documentation
*/
default ListMedicalTranscriptionJobsResponse listMedicalTranscriptionJobs(
Consumer listMedicalTranscriptionJobsRequest)
throws BadRequestException, LimitExceededException, InternalFailureException, AwsServiceException,
SdkClientException, TranscribeException {
return listMedicalTranscriptionJobs(ListMedicalTranscriptionJobsRequest.builder()
.applyMutation(listMedicalTranscriptionJobsRequest).build());
}
/**
*
* Lists medical transcription jobs with a specified status or substring that matches their names.
*
*
*
* This is a variant of
* {@link #listMedicalTranscriptionJobs(software.amazon.awssdk.services.transcribe.model.ListMedicalTranscriptionJobsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.transcribe.paginators.ListMedicalTranscriptionJobsIterable responses = client.listMedicalTranscriptionJobsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.transcribe.paginators.ListMedicalTranscriptionJobsIterable responses = client
* .listMedicalTranscriptionJobsPaginator(request);
* for (software.amazon.awssdk.services.transcribe.model.ListMedicalTranscriptionJobsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.transcribe.paginators.ListMedicalTranscriptionJobsIterable responses = client.listMedicalTranscriptionJobsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listMedicalTranscriptionJobs(software.amazon.awssdk.services.transcribe.model.ListMedicalTranscriptionJobsRequest)}
* operation.
*
*
* @param listMedicalTranscriptionJobsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the entity that you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.ListMedicalTranscriptionJobs
* @see AWS API Documentation
*/
default ListMedicalTranscriptionJobsIterable listMedicalTranscriptionJobsPaginator(
ListMedicalTranscriptionJobsRequest listMedicalTranscriptionJobsRequest) throws BadRequestException,
LimitExceededException, InternalFailureException, AwsServiceException, SdkClientException, TranscribeException {
throw new UnsupportedOperationException();
}
/**
*
* Lists medical transcription jobs with a specified status or substring that matches their names.
*
*
*
* This is a variant of
* {@link #listMedicalTranscriptionJobs(software.amazon.awssdk.services.transcribe.model.ListMedicalTranscriptionJobsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.transcribe.paginators.ListMedicalTranscriptionJobsIterable responses = client.listMedicalTranscriptionJobsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.transcribe.paginators.ListMedicalTranscriptionJobsIterable responses = client
* .listMedicalTranscriptionJobsPaginator(request);
* for (software.amazon.awssdk.services.transcribe.model.ListMedicalTranscriptionJobsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.transcribe.paginators.ListMedicalTranscriptionJobsIterable responses = client.listMedicalTranscriptionJobsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listMedicalTranscriptionJobs(software.amazon.awssdk.services.transcribe.model.ListMedicalTranscriptionJobsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListMedicalTranscriptionJobsRequest.Builder}
* avoiding the need to create one manually via {@link ListMedicalTranscriptionJobsRequest#builder()}
*
*
* @param listMedicalTranscriptionJobsRequest
* A {@link Consumer} that will call methods on {@link ListMedicalTranscriptionJobsRequest.Builder} to create
* a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the entity that you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.ListMedicalTranscriptionJobs
* @see AWS API Documentation
*/
default ListMedicalTranscriptionJobsIterable listMedicalTranscriptionJobsPaginator(
Consumer listMedicalTranscriptionJobsRequest)
throws BadRequestException, LimitExceededException, InternalFailureException, AwsServiceException,
SdkClientException, TranscribeException {
return listMedicalTranscriptionJobsPaginator(ListMedicalTranscriptionJobsRequest.builder()
.applyMutation(listMedicalTranscriptionJobsRequest).build());
}
/**
*
* Returns a list of vocabularies that match the specified criteria. If you don't enter a value in any of the
* request parameters, returns the entire list of vocabularies.
*
*
* @param listMedicalVocabulariesRequest
* @return Result of the ListMedicalVocabularies operation returned by the service.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the entity that you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.ListMedicalVocabularies
* @see AWS API Documentation
*/
default ListMedicalVocabulariesResponse listMedicalVocabularies(ListMedicalVocabulariesRequest listMedicalVocabulariesRequest)
throws BadRequestException, LimitExceededException, InternalFailureException, AwsServiceException,
SdkClientException, TranscribeException {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of vocabularies that match the specified criteria. If you don't enter a value in any of the
* request parameters, returns the entire list of vocabularies.
*
*
*
* This is a convenience which creates an instance of the {@link ListMedicalVocabulariesRequest.Builder} avoiding
* the need to create one manually via {@link ListMedicalVocabulariesRequest#builder()}
*
*
* @param listMedicalVocabulariesRequest
* A {@link Consumer} that will call methods on {@link ListMedicalVocabulariesRequest.Builder} to create a
* request.
* @return Result of the ListMedicalVocabularies operation returned by the service.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the entity that you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.ListMedicalVocabularies
* @see AWS API Documentation
*/
default ListMedicalVocabulariesResponse listMedicalVocabularies(
Consumer listMedicalVocabulariesRequest) throws BadRequestException,
LimitExceededException, InternalFailureException, AwsServiceException, SdkClientException, TranscribeException {
return listMedicalVocabularies(ListMedicalVocabulariesRequest.builder().applyMutation(listMedicalVocabulariesRequest)
.build());
}
/**
*
* Returns a list of vocabularies that match the specified criteria. If you don't enter a value in any of the
* request parameters, returns the entire list of vocabularies.
*
*
*
* This is a variant of
* {@link #listMedicalVocabularies(software.amazon.awssdk.services.transcribe.model.ListMedicalVocabulariesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.transcribe.paginators.ListMedicalVocabulariesIterable responses = client.listMedicalVocabulariesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.transcribe.paginators.ListMedicalVocabulariesIterable responses = client
* .listMedicalVocabulariesPaginator(request);
* for (software.amazon.awssdk.services.transcribe.model.ListMedicalVocabulariesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.transcribe.paginators.ListMedicalVocabulariesIterable responses = client.listMedicalVocabulariesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listMedicalVocabularies(software.amazon.awssdk.services.transcribe.model.ListMedicalVocabulariesRequest)}
* operation.
*
*
* @param listMedicalVocabulariesRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the entity that you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.ListMedicalVocabularies
* @see AWS API Documentation
*/
default ListMedicalVocabulariesIterable listMedicalVocabulariesPaginator(
ListMedicalVocabulariesRequest listMedicalVocabulariesRequest) throws BadRequestException, LimitExceededException,
InternalFailureException, AwsServiceException, SdkClientException, TranscribeException {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of vocabularies that match the specified criteria. If you don't enter a value in any of the
* request parameters, returns the entire list of vocabularies.
*
*
*
* This is a variant of
* {@link #listMedicalVocabularies(software.amazon.awssdk.services.transcribe.model.ListMedicalVocabulariesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.transcribe.paginators.ListMedicalVocabulariesIterable responses = client.listMedicalVocabulariesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.transcribe.paginators.ListMedicalVocabulariesIterable responses = client
* .listMedicalVocabulariesPaginator(request);
* for (software.amazon.awssdk.services.transcribe.model.ListMedicalVocabulariesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.transcribe.paginators.ListMedicalVocabulariesIterable responses = client.listMedicalVocabulariesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listMedicalVocabularies(software.amazon.awssdk.services.transcribe.model.ListMedicalVocabulariesRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListMedicalVocabulariesRequest.Builder} avoiding
* the need to create one manually via {@link ListMedicalVocabulariesRequest#builder()}
*
*
* @param listMedicalVocabulariesRequest
* A {@link Consumer} that will call methods on {@link ListMedicalVocabulariesRequest.Builder} to create a
* request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the entity that you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.ListMedicalVocabularies
* @see AWS API Documentation
*/
default ListMedicalVocabulariesIterable listMedicalVocabulariesPaginator(
Consumer listMedicalVocabulariesRequest) throws BadRequestException,
LimitExceededException, InternalFailureException, AwsServiceException, SdkClientException, TranscribeException {
return listMedicalVocabulariesPaginator(ListMedicalVocabulariesRequest.builder()
.applyMutation(listMedicalVocabulariesRequest).build());
}
/**
*
* Lists transcription jobs with the specified status.
*
*
* @return Result of the ListTranscriptionJobs operation returned by the service.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the entity that you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.ListTranscriptionJobs
* @see #listTranscriptionJobs(ListTranscriptionJobsRequest)
* @see AWS API Documentation
*/
default ListTranscriptionJobsResponse listTranscriptionJobs() throws BadRequestException, LimitExceededException,
InternalFailureException, AwsServiceException, SdkClientException, TranscribeException {
return listTranscriptionJobs(ListTranscriptionJobsRequest.builder().build());
}
/**
*
* Lists transcription jobs with the specified status.
*
*
* @param listTranscriptionJobsRequest
* @return Result of the ListTranscriptionJobs operation returned by the service.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the entity that you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.ListTranscriptionJobs
* @see AWS API Documentation
*/
default ListTranscriptionJobsResponse listTranscriptionJobs(ListTranscriptionJobsRequest listTranscriptionJobsRequest)
throws BadRequestException, LimitExceededException, InternalFailureException, AwsServiceException,
SdkClientException, TranscribeException {
throw new UnsupportedOperationException();
}
/**
*
* Lists transcription jobs with the specified status.
*
*
*
* This is a convenience which creates an instance of the {@link ListTranscriptionJobsRequest.Builder} avoiding the
* need to create one manually via {@link ListTranscriptionJobsRequest#builder()}
*
*
* @param listTranscriptionJobsRequest
* A {@link Consumer} that will call methods on {@link ListTranscriptionJobsRequest.Builder} to create a
* request.
* @return Result of the ListTranscriptionJobs operation returned by the service.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the entity that you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.ListTranscriptionJobs
* @see AWS API Documentation
*/
default ListTranscriptionJobsResponse listTranscriptionJobs(
Consumer listTranscriptionJobsRequest) throws BadRequestException,
LimitExceededException, InternalFailureException, AwsServiceException, SdkClientException, TranscribeException {
return listTranscriptionJobs(ListTranscriptionJobsRequest.builder().applyMutation(listTranscriptionJobsRequest).build());
}
/**
*
* Lists transcription jobs with the specified status.
*
*
*
* This is a variant of
* {@link #listTranscriptionJobs(software.amazon.awssdk.services.transcribe.model.ListTranscriptionJobsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.transcribe.paginators.ListTranscriptionJobsIterable responses = client.listTranscriptionJobsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.transcribe.paginators.ListTranscriptionJobsIterable responses = client
* .listTranscriptionJobsPaginator(request);
* for (software.amazon.awssdk.services.transcribe.model.ListTranscriptionJobsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.transcribe.paginators.ListTranscriptionJobsIterable responses = client.listTranscriptionJobsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listTranscriptionJobs(software.amazon.awssdk.services.transcribe.model.ListTranscriptionJobsRequest)}
* operation.
*
*
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the entity that you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.ListTranscriptionJobs
* @see #listTranscriptionJobsPaginator(ListTranscriptionJobsRequest)
* @see AWS API Documentation
*/
default ListTranscriptionJobsIterable listTranscriptionJobsPaginator() throws BadRequestException, LimitExceededException,
InternalFailureException, AwsServiceException, SdkClientException, TranscribeException {
return listTranscriptionJobsPaginator(ListTranscriptionJobsRequest.builder().build());
}
/**
*
* Lists transcription jobs with the specified status.
*
*
*
* This is a variant of
* {@link #listTranscriptionJobs(software.amazon.awssdk.services.transcribe.model.ListTranscriptionJobsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.transcribe.paginators.ListTranscriptionJobsIterable responses = client.listTranscriptionJobsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.transcribe.paginators.ListTranscriptionJobsIterable responses = client
* .listTranscriptionJobsPaginator(request);
* for (software.amazon.awssdk.services.transcribe.model.ListTranscriptionJobsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.transcribe.paginators.ListTranscriptionJobsIterable responses = client.listTranscriptionJobsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listTranscriptionJobs(software.amazon.awssdk.services.transcribe.model.ListTranscriptionJobsRequest)}
* operation.
*
*
* @param listTranscriptionJobsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the entity that you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.ListTranscriptionJobs
* @see AWS API Documentation
*/
default ListTranscriptionJobsIterable listTranscriptionJobsPaginator(ListTranscriptionJobsRequest listTranscriptionJobsRequest)
throws BadRequestException, LimitExceededException, InternalFailureException, AwsServiceException,
SdkClientException, TranscribeException {
throw new UnsupportedOperationException();
}
/**
*
* Lists transcription jobs with the specified status.
*
*
*
* This is a variant of
* {@link #listTranscriptionJobs(software.amazon.awssdk.services.transcribe.model.ListTranscriptionJobsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.transcribe.paginators.ListTranscriptionJobsIterable responses = client.listTranscriptionJobsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.transcribe.paginators.ListTranscriptionJobsIterable responses = client
* .listTranscriptionJobsPaginator(request);
* for (software.amazon.awssdk.services.transcribe.model.ListTranscriptionJobsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.transcribe.paginators.ListTranscriptionJobsIterable responses = client.listTranscriptionJobsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listTranscriptionJobs(software.amazon.awssdk.services.transcribe.model.ListTranscriptionJobsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListTranscriptionJobsRequest.Builder} avoiding the
* need to create one manually via {@link ListTranscriptionJobsRequest#builder()}
*
*
* @param listTranscriptionJobsRequest
* A {@link Consumer} that will call methods on {@link ListTranscriptionJobsRequest.Builder} to create a
* request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the entity that you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.ListTranscriptionJobs
* @see AWS API Documentation
*/
default ListTranscriptionJobsIterable listTranscriptionJobsPaginator(
Consumer listTranscriptionJobsRequest) throws BadRequestException,
LimitExceededException, InternalFailureException, AwsServiceException, SdkClientException, TranscribeException {
return listTranscriptionJobsPaginator(ListTranscriptionJobsRequest.builder().applyMutation(listTranscriptionJobsRequest)
.build());
}
/**
*
* Returns a list of vocabularies that match the specified criteria. If no criteria are specified, returns the
* entire list of vocabularies.
*
*
* @return Result of the ListVocabularies operation returned by the service.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the entity that you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.ListVocabularies
* @see #listVocabularies(ListVocabulariesRequest)
* @see AWS
* API Documentation
*/
default ListVocabulariesResponse listVocabularies() throws BadRequestException, LimitExceededException,
InternalFailureException, AwsServiceException, SdkClientException, TranscribeException {
return listVocabularies(ListVocabulariesRequest.builder().build());
}
/**
*
* Returns a list of vocabularies that match the specified criteria. If no criteria are specified, returns the
* entire list of vocabularies.
*
*
* @param listVocabulariesRequest
* @return Result of the ListVocabularies operation returned by the service.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the entity that you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.ListVocabularies
* @see AWS
* API Documentation
*/
default ListVocabulariesResponse listVocabularies(ListVocabulariesRequest listVocabulariesRequest)
throws BadRequestException, LimitExceededException, InternalFailureException, AwsServiceException,
SdkClientException, TranscribeException {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of vocabularies that match the specified criteria. If no criteria are specified, returns the
* entire list of vocabularies.
*
*
*
* This is a convenience which creates an instance of the {@link ListVocabulariesRequest.Builder} avoiding the need
* to create one manually via {@link ListVocabulariesRequest#builder()}
*
*
* @param listVocabulariesRequest
* A {@link Consumer} that will call methods on {@link ListVocabulariesRequest.Builder} to create a request.
* @return Result of the ListVocabularies operation returned by the service.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the entity that you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.ListVocabularies
* @see AWS
* API Documentation
*/
default ListVocabulariesResponse listVocabularies(Consumer listVocabulariesRequest)
throws BadRequestException, LimitExceededException, InternalFailureException, AwsServiceException,
SdkClientException, TranscribeException {
return listVocabularies(ListVocabulariesRequest.builder().applyMutation(listVocabulariesRequest).build());
}
/**
*
* Returns a list of vocabularies that match the specified criteria. If no criteria are specified, returns the
* entire list of vocabularies.
*
*
*
* This is a variant of
* {@link #listVocabularies(software.amazon.awssdk.services.transcribe.model.ListVocabulariesRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.transcribe.paginators.ListVocabulariesIterable responses = client.listVocabulariesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.transcribe.paginators.ListVocabulariesIterable responses = client
* .listVocabulariesPaginator(request);
* for (software.amazon.awssdk.services.transcribe.model.ListVocabulariesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.transcribe.paginators.ListVocabulariesIterable responses = client.listVocabulariesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listVocabularies(software.amazon.awssdk.services.transcribe.model.ListVocabulariesRequest)}
* operation.
*
*
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the entity that you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.ListVocabularies
* @see #listVocabulariesPaginator(ListVocabulariesRequest)
* @see AWS
* API Documentation
*/
default ListVocabulariesIterable listVocabulariesPaginator() throws BadRequestException, LimitExceededException,
InternalFailureException, AwsServiceException, SdkClientException, TranscribeException {
return listVocabulariesPaginator(ListVocabulariesRequest.builder().build());
}
/**
*
* Returns a list of vocabularies that match the specified criteria. If no criteria are specified, returns the
* entire list of vocabularies.
*
*
*
* This is a variant of
* {@link #listVocabularies(software.amazon.awssdk.services.transcribe.model.ListVocabulariesRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.transcribe.paginators.ListVocabulariesIterable responses = client.listVocabulariesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.transcribe.paginators.ListVocabulariesIterable responses = client
* .listVocabulariesPaginator(request);
* for (software.amazon.awssdk.services.transcribe.model.ListVocabulariesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.transcribe.paginators.ListVocabulariesIterable responses = client.listVocabulariesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listVocabularies(software.amazon.awssdk.services.transcribe.model.ListVocabulariesRequest)}
* operation.
*
*
* @param listVocabulariesRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the entity that you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.ListVocabularies
* @see AWS
* API Documentation
*/
default ListVocabulariesIterable listVocabulariesPaginator(ListVocabulariesRequest listVocabulariesRequest)
throws BadRequestException, LimitExceededException, InternalFailureException, AwsServiceException,
SdkClientException, TranscribeException {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of vocabularies that match the specified criteria. If no criteria are specified, returns the
* entire list of vocabularies.
*
*
*
* This is a variant of
* {@link #listVocabularies(software.amazon.awssdk.services.transcribe.model.ListVocabulariesRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.transcribe.paginators.ListVocabulariesIterable responses = client.listVocabulariesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.transcribe.paginators.ListVocabulariesIterable responses = client
* .listVocabulariesPaginator(request);
* for (software.amazon.awssdk.services.transcribe.model.ListVocabulariesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.transcribe.paginators.ListVocabulariesIterable responses = client.listVocabulariesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listVocabularies(software.amazon.awssdk.services.transcribe.model.ListVocabulariesRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListVocabulariesRequest.Builder} avoiding the need
* to create one manually via {@link ListVocabulariesRequest#builder()}
*
*
* @param listVocabulariesRequest
* A {@link Consumer} that will call methods on {@link ListVocabulariesRequest.Builder} to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the entity that you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.ListVocabularies
* @see AWS
* API Documentation
*/
default ListVocabulariesIterable listVocabulariesPaginator(Consumer listVocabulariesRequest)
throws BadRequestException, LimitExceededException, InternalFailureException, AwsServiceException,
SdkClientException, TranscribeException {
return listVocabulariesPaginator(ListVocabulariesRequest.builder().applyMutation(listVocabulariesRequest).build());
}
/**
*
* Gets information about vocabulary filters.
*
*
* @param listVocabularyFiltersRequest
* @return Result of the ListVocabularyFilters operation returned by the service.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the entity that you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.ListVocabularyFilters
* @see AWS API Documentation
*/
default ListVocabularyFiltersResponse listVocabularyFilters(ListVocabularyFiltersRequest listVocabularyFiltersRequest)
throws BadRequestException, LimitExceededException, InternalFailureException, AwsServiceException,
SdkClientException, TranscribeException {
throw new UnsupportedOperationException();
}
/**
*
* Gets information about vocabulary filters.
*
*
*
* This is a convenience which creates an instance of the {@link ListVocabularyFiltersRequest.Builder} avoiding the
* need to create one manually via {@link ListVocabularyFiltersRequest#builder()}
*
*
* @param listVocabularyFiltersRequest
* A {@link Consumer} that will call methods on {@link ListVocabularyFiltersRequest.Builder} to create a
* request.
* @return Result of the ListVocabularyFilters operation returned by the service.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the entity that you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.ListVocabularyFilters
* @see AWS API Documentation
*/
default ListVocabularyFiltersResponse listVocabularyFilters(
Consumer listVocabularyFiltersRequest) throws BadRequestException,
LimitExceededException, InternalFailureException, AwsServiceException, SdkClientException, TranscribeException {
return listVocabularyFilters(ListVocabularyFiltersRequest.builder().applyMutation(listVocabularyFiltersRequest).build());
}
/**
*
* Gets information about vocabulary filters.
*
*
*
* This is a variant of
* {@link #listVocabularyFilters(software.amazon.awssdk.services.transcribe.model.ListVocabularyFiltersRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.transcribe.paginators.ListVocabularyFiltersIterable responses = client.listVocabularyFiltersPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.transcribe.paginators.ListVocabularyFiltersIterable responses = client
* .listVocabularyFiltersPaginator(request);
* for (software.amazon.awssdk.services.transcribe.model.ListVocabularyFiltersResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.transcribe.paginators.ListVocabularyFiltersIterable responses = client.listVocabularyFiltersPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listVocabularyFilters(software.amazon.awssdk.services.transcribe.model.ListVocabularyFiltersRequest)}
* operation.
*
*
* @param listVocabularyFiltersRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the entity that you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.ListVocabularyFilters
* @see AWS API Documentation
*/
default ListVocabularyFiltersIterable listVocabularyFiltersPaginator(ListVocabularyFiltersRequest listVocabularyFiltersRequest)
throws BadRequestException, LimitExceededException, InternalFailureException, AwsServiceException,
SdkClientException, TranscribeException {
throw new UnsupportedOperationException();
}
/**
*
* Gets information about vocabulary filters.
*
*
*
* This is a variant of
* {@link #listVocabularyFilters(software.amazon.awssdk.services.transcribe.model.ListVocabularyFiltersRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.transcribe.paginators.ListVocabularyFiltersIterable responses = client.listVocabularyFiltersPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.transcribe.paginators.ListVocabularyFiltersIterable responses = client
* .listVocabularyFiltersPaginator(request);
* for (software.amazon.awssdk.services.transcribe.model.ListVocabularyFiltersResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.transcribe.paginators.ListVocabularyFiltersIterable responses = client.listVocabularyFiltersPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listVocabularyFilters(software.amazon.awssdk.services.transcribe.model.ListVocabularyFiltersRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListVocabularyFiltersRequest.Builder} avoiding the
* need to create one manually via {@link ListVocabularyFiltersRequest#builder()}
*
*
* @param listVocabularyFiltersRequest
* A {@link Consumer} that will call methods on {@link ListVocabularyFiltersRequest.Builder} to create a
* request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the entity that you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.ListVocabularyFilters
* @see AWS API Documentation
*/
default ListVocabularyFiltersIterable listVocabularyFiltersPaginator(
Consumer listVocabularyFiltersRequest) throws BadRequestException,
LimitExceededException, InternalFailureException, AwsServiceException, SdkClientException, TranscribeException {
return listVocabularyFiltersPaginator(ListVocabularyFiltersRequest.builder().applyMutation(listVocabularyFiltersRequest)
.build());
}
/**
*
* Starts a batch job to transcribe medical speech to text.
*
*
* @param startMedicalTranscriptionJobRequest
* @return Result of the StartMedicalTranscriptionJob operation returned by the service.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the entity that you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws ConflictException
* There is already a resource with that name.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.StartMedicalTranscriptionJob
* @see AWS API Documentation
*/
default StartMedicalTranscriptionJobResponse startMedicalTranscriptionJob(
StartMedicalTranscriptionJobRequest startMedicalTranscriptionJobRequest) throws BadRequestException,
LimitExceededException, InternalFailureException, ConflictException, AwsServiceException, SdkClientException,
TranscribeException {
throw new UnsupportedOperationException();
}
/**
*
* Starts a batch job to transcribe medical speech to text.
*
*
*
* This is a convenience which creates an instance of the {@link StartMedicalTranscriptionJobRequest.Builder}
* avoiding the need to create one manually via {@link StartMedicalTranscriptionJobRequest#builder()}
*
*
* @param startMedicalTranscriptionJobRequest
* A {@link Consumer} that will call methods on {@link StartMedicalTranscriptionJobRequest.Builder} to create
* a request.
* @return Result of the StartMedicalTranscriptionJob operation returned by the service.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the entity that you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws ConflictException
* There is already a resource with that name.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.StartMedicalTranscriptionJob
* @see AWS API Documentation
*/
default StartMedicalTranscriptionJobResponse startMedicalTranscriptionJob(
Consumer startMedicalTranscriptionJobRequest)
throws BadRequestException, LimitExceededException, InternalFailureException, ConflictException, AwsServiceException,
SdkClientException, TranscribeException {
return startMedicalTranscriptionJob(StartMedicalTranscriptionJobRequest.builder()
.applyMutation(startMedicalTranscriptionJobRequest).build());
}
/**
*
* Starts an asynchronous job to transcribe speech to text.
*
*
* @param startTranscriptionJobRequest
* @return Result of the StartTranscriptionJob operation returned by the service.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the entity that you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws ConflictException
* There is already a resource with that name.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.StartTranscriptionJob
* @see AWS API Documentation
*/
default StartTranscriptionJobResponse startTranscriptionJob(StartTranscriptionJobRequest startTranscriptionJobRequest)
throws BadRequestException, LimitExceededException, InternalFailureException, ConflictException, AwsServiceException,
SdkClientException, TranscribeException {
throw new UnsupportedOperationException();
}
/**
*
* Starts an asynchronous job to transcribe speech to text.
*
*
*
* This is a convenience which creates an instance of the {@link StartTranscriptionJobRequest.Builder} avoiding the
* need to create one manually via {@link StartTranscriptionJobRequest#builder()}
*
*
* @param startTranscriptionJobRequest
* A {@link Consumer} that will call methods on {@link StartTranscriptionJobRequest.Builder} to create a
* request.
* @return Result of the StartTranscriptionJob operation returned by the service.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the entity that you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws ConflictException
* There is already a resource with that name.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.StartTranscriptionJob
* @see AWS API Documentation
*/
default StartTranscriptionJobResponse startTranscriptionJob(
Consumer startTranscriptionJobRequest) throws BadRequestException,
LimitExceededException, InternalFailureException, ConflictException, AwsServiceException, SdkClientException,
TranscribeException {
return startTranscriptionJob(StartTranscriptionJobRequest.builder().applyMutation(startTranscriptionJobRequest).build());
}
/**
*
* Updates a vocabulary with new values that you provide in a different text file from the one you used to create
* the vocabulary. The UpdateMedicalVocabulary
operation overwrites all of the existing information
* with the values that you provide in the request.
*
*
* @param updateMedicalVocabularyRequest
* @return Result of the UpdateMedicalVocabulary operation returned by the service.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the entity that you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws NotFoundException
* We can't find the requested resource. Check the name and try your request again.
* @throws ConflictException
* There is already a resource with that name.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.UpdateMedicalVocabulary
* @see AWS API Documentation
*/
default UpdateMedicalVocabularyResponse updateMedicalVocabulary(UpdateMedicalVocabularyRequest updateMedicalVocabularyRequest)
throws BadRequestException, LimitExceededException, InternalFailureException, NotFoundException, ConflictException,
AwsServiceException, SdkClientException, TranscribeException {
throw new UnsupportedOperationException();
}
/**
*
* Updates a vocabulary with new values that you provide in a different text file from the one you used to create
* the vocabulary. The UpdateMedicalVocabulary
operation overwrites all of the existing information
* with the values that you provide in the request.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateMedicalVocabularyRequest.Builder} avoiding
* the need to create one manually via {@link UpdateMedicalVocabularyRequest#builder()}
*
*
* @param updateMedicalVocabularyRequest
* A {@link Consumer} that will call methods on {@link UpdateMedicalVocabularyRequest.Builder} to create a
* request.
* @return Result of the UpdateMedicalVocabulary operation returned by the service.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the entity that you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws NotFoundException
* We can't find the requested resource. Check the name and try your request again.
* @throws ConflictException
* There is already a resource with that name.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.UpdateMedicalVocabulary
* @see AWS API Documentation
*/
default UpdateMedicalVocabularyResponse updateMedicalVocabulary(
Consumer updateMedicalVocabularyRequest) throws BadRequestException,
LimitExceededException, InternalFailureException, NotFoundException, ConflictException, AwsServiceException,
SdkClientException, TranscribeException {
return updateMedicalVocabulary(UpdateMedicalVocabularyRequest.builder().applyMutation(updateMedicalVocabularyRequest)
.build());
}
/**
*
* Updates an existing vocabulary with new values. The UpdateVocabulary
operation overwrites all of the
* existing information with the values that you provide in the request.
*
*
* @param updateVocabularyRequest
* @return Result of the UpdateVocabulary operation returned by the service.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the entity that you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws NotFoundException
* We can't find the requested resource. Check the name and try your request again.
* @throws ConflictException
* There is already a resource with that name.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.UpdateVocabulary
* @see AWS
* API Documentation
*/
default UpdateVocabularyResponse updateVocabulary(UpdateVocabularyRequest updateVocabularyRequest)
throws BadRequestException, LimitExceededException, InternalFailureException, NotFoundException, ConflictException,
AwsServiceException, SdkClientException, TranscribeException {
throw new UnsupportedOperationException();
}
/**
*
* Updates an existing vocabulary with new values. The UpdateVocabulary
operation overwrites all of the
* existing information with the values that you provide in the request.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateVocabularyRequest.Builder} avoiding the need
* to create one manually via {@link UpdateVocabularyRequest#builder()}
*
*
* @param updateVocabularyRequest
* A {@link Consumer} that will call methods on {@link UpdateVocabularyRequest.Builder} to create a request.
* @return Result of the UpdateVocabulary operation returned by the service.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the entity that you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws NotFoundException
* We can't find the requested resource. Check the name and try your request again.
* @throws ConflictException
* There is already a resource with that name.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.UpdateVocabulary
* @see AWS
* API Documentation
*/
default UpdateVocabularyResponse updateVocabulary(Consumer updateVocabularyRequest)
throws BadRequestException, LimitExceededException, InternalFailureException, NotFoundException, ConflictException,
AwsServiceException, SdkClientException, TranscribeException {
return updateVocabulary(UpdateVocabularyRequest.builder().applyMutation(updateVocabularyRequest).build());
}
/**
*
* Updates a vocabulary filter with a new list of filtered words.
*
*
* @param updateVocabularyFilterRequest
* @return Result of the UpdateVocabularyFilter operation returned by the service.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the entity that you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws NotFoundException
* We can't find the requested resource. Check the name and try your request again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.UpdateVocabularyFilter
* @see AWS API Documentation
*/
default UpdateVocabularyFilterResponse updateVocabularyFilter(UpdateVocabularyFilterRequest updateVocabularyFilterRequest)
throws BadRequestException, LimitExceededException, InternalFailureException, NotFoundException, AwsServiceException,
SdkClientException, TranscribeException {
throw new UnsupportedOperationException();
}
/**
*
* Updates a vocabulary filter with a new list of filtered words.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateVocabularyFilterRequest.Builder} avoiding the
* need to create one manually via {@link UpdateVocabularyFilterRequest#builder()}
*
*
* @param updateVocabularyFilterRequest
* A {@link Consumer} that will call methods on {@link UpdateVocabularyFilterRequest.Builder} to create a
* request.
* @return Result of the UpdateVocabularyFilter operation returned by the service.
* @throws BadRequestException
* Your request didn't pass one or more validation tests. For example, if the entity that you're trying to
* delete doesn't exist or if it is in a non-terminal state (for example, it's "in progress"). See the
* exception Message
field for more information.
* @throws LimitExceededException
* Either you have sent too many requests or your input file is too long. Wait before you resend your
* request, or use a smaller file and resend the request.
* @throws InternalFailureException
* There was an internal error. Check the error message and try your request again.
* @throws NotFoundException
* We can't find the requested resource. Check the name and try your request again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranscribeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranscribeClient.UpdateVocabularyFilter
* @see AWS API Documentation
*/
default UpdateVocabularyFilterResponse updateVocabularyFilter(
Consumer updateVocabularyFilterRequest) throws BadRequestException,
LimitExceededException, InternalFailureException, NotFoundException, AwsServiceException, SdkClientException,
TranscribeException {
return updateVocabularyFilter(UpdateVocabularyFilterRequest.builder().applyMutation(updateVocabularyFilterRequest)
.build());
}
static ServiceMetadata serviceMetadata() {
return ServiceMetadata.of("transcribe");
}
}