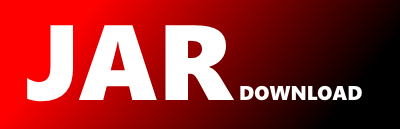
software.amazon.awssdk.services.transcribe.model.MedicalTranscriptionSetting Maven / Gradle / Ivy
Show all versions of transcribe Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.transcribe.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Optional settings for the StartMedicalTranscriptionJob operation.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class MedicalTranscriptionSetting implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField SHOW_SPEAKER_LABELS_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("ShowSpeakerLabels").getter(getter(MedicalTranscriptionSetting::showSpeakerLabels))
.setter(setter(Builder::showSpeakerLabels))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ShowSpeakerLabels").build()).build();
private static final SdkField MAX_SPEAKER_LABELS_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("MaxSpeakerLabels").getter(getter(MedicalTranscriptionSetting::maxSpeakerLabels))
.setter(setter(Builder::maxSpeakerLabels))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MaxSpeakerLabels").build()).build();
private static final SdkField CHANNEL_IDENTIFICATION_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("ChannelIdentification").getter(getter(MedicalTranscriptionSetting::channelIdentification))
.setter(setter(Builder::channelIdentification))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ChannelIdentification").build())
.build();
private static final SdkField SHOW_ALTERNATIVES_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("ShowAlternatives").getter(getter(MedicalTranscriptionSetting::showAlternatives))
.setter(setter(Builder::showAlternatives))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ShowAlternatives").build()).build();
private static final SdkField MAX_ALTERNATIVES_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("MaxAlternatives").getter(getter(MedicalTranscriptionSetting::maxAlternatives))
.setter(setter(Builder::maxAlternatives))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MaxAlternatives").build()).build();
private static final SdkField VOCABULARY_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("VocabularyName").getter(getter(MedicalTranscriptionSetting::vocabularyName))
.setter(setter(Builder::vocabularyName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("VocabularyName").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(SHOW_SPEAKER_LABELS_FIELD,
MAX_SPEAKER_LABELS_FIELD, CHANNEL_IDENTIFICATION_FIELD, SHOW_ALTERNATIVES_FIELD, MAX_ALTERNATIVES_FIELD,
VOCABULARY_NAME_FIELD));
private static final long serialVersionUID = 1L;
private final Boolean showSpeakerLabels;
private final Integer maxSpeakerLabels;
private final Boolean channelIdentification;
private final Boolean showAlternatives;
private final Integer maxAlternatives;
private final String vocabularyName;
private MedicalTranscriptionSetting(BuilderImpl builder) {
this.showSpeakerLabels = builder.showSpeakerLabels;
this.maxSpeakerLabels = builder.maxSpeakerLabels;
this.channelIdentification = builder.channelIdentification;
this.showAlternatives = builder.showAlternatives;
this.maxAlternatives = builder.maxAlternatives;
this.vocabularyName = builder.vocabularyName;
}
/**
*
* Determines whether the transcription job uses speaker recognition to identify different speakers in the input
* audio. Speaker recognition labels individual speakers in the audio file. If you set the
* ShowSpeakerLabels
field to true, you must also set the maximum number of speaker labels in the
* MaxSpeakerLabels
field.
*
*
* You can't set both ShowSpeakerLabels
and ChannelIdentification
in the same request. If
* you set both, your request returns a BadRequestException
.
*
*
* @return Determines whether the transcription job uses speaker recognition to identify different speakers in the
* input audio. Speaker recognition labels individual speakers in the audio file. If you set the
* ShowSpeakerLabels
field to true, you must also set the maximum number of speaker labels in
* the MaxSpeakerLabels
field.
*
* You can't set both ShowSpeakerLabels
and ChannelIdentification
in the same
* request. If you set both, your request returns a BadRequestException
.
*/
public final Boolean showSpeakerLabels() {
return showSpeakerLabels;
}
/**
*
* The maximum number of speakers to identify in the input audio. If there are more speakers in the audio than this
* number, multiple speakers are identified as a single speaker. If you specify the MaxSpeakerLabels
* field, you must set the ShowSpeakerLabels
field to true.
*
*
* @return The maximum number of speakers to identify in the input audio. If there are more speakers in the audio
* than this number, multiple speakers are identified as a single speaker. If you specify the
* MaxSpeakerLabels
field, you must set the ShowSpeakerLabels
field to true.
*/
public final Integer maxSpeakerLabels() {
return maxSpeakerLabels;
}
/**
*
* Instructs Amazon Transcribe Medical to process each audio channel separately and then merge the transcription
* output of each channel into a single transcription.
*
*
* Amazon Transcribe Medical also produces a transcription of each item detected on an audio channel, including the
* start time and end time of the item and alternative transcriptions of item. The alternative transcriptions also
* come with confidence scores provided by Amazon Transcribe Medical.
*
*
* You can't set both ShowSpeakerLabels
and ChannelIdentification
in the same request. If
* you set both, your request returns a BadRequestException
*
*
* @return Instructs Amazon Transcribe Medical to process each audio channel separately and then merge the
* transcription output of each channel into a single transcription.
*
* Amazon Transcribe Medical also produces a transcription of each item detected on an audio channel,
* including the start time and end time of the item and alternative transcriptions of item. The alternative
* transcriptions also come with confidence scores provided by Amazon Transcribe Medical.
*
*
* You can't set both ShowSpeakerLabels
and ChannelIdentification
in the same
* request. If you set both, your request returns a BadRequestException
*/
public final Boolean channelIdentification() {
return channelIdentification;
}
/**
*
* Determines whether alternative transcripts are generated along with the transcript that has the highest
* confidence. If you set ShowAlternatives
field to true, you must also set the maximum number of
* alternatives to return in the MaxAlternatives
field.
*
*
* @return Determines whether alternative transcripts are generated along with the transcript that has the highest
* confidence. If you set ShowAlternatives
field to true, you must also set the maximum number
* of alternatives to return in the MaxAlternatives
field.
*/
public final Boolean showAlternatives() {
return showAlternatives;
}
/**
*
* The maximum number of alternatives that you tell the service to return. If you specify the
* MaxAlternatives
field, you must set the ShowAlternatives
field to true.
*
*
* @return The maximum number of alternatives that you tell the service to return. If you specify the
* MaxAlternatives
field, you must set the ShowAlternatives
field to true.
*/
public final Integer maxAlternatives() {
return maxAlternatives;
}
/**
*
* The name of the vocabulary to use when processing a medical transcription job.
*
*
* @return The name of the vocabulary to use when processing a medical transcription job.
*/
public final String vocabularyName() {
return vocabularyName;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(showSpeakerLabels());
hashCode = 31 * hashCode + Objects.hashCode(maxSpeakerLabels());
hashCode = 31 * hashCode + Objects.hashCode(channelIdentification());
hashCode = 31 * hashCode + Objects.hashCode(showAlternatives());
hashCode = 31 * hashCode + Objects.hashCode(maxAlternatives());
hashCode = 31 * hashCode + Objects.hashCode(vocabularyName());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof MedicalTranscriptionSetting)) {
return false;
}
MedicalTranscriptionSetting other = (MedicalTranscriptionSetting) obj;
return Objects.equals(showSpeakerLabels(), other.showSpeakerLabels())
&& Objects.equals(maxSpeakerLabels(), other.maxSpeakerLabels())
&& Objects.equals(channelIdentification(), other.channelIdentification())
&& Objects.equals(showAlternatives(), other.showAlternatives())
&& Objects.equals(maxAlternatives(), other.maxAlternatives())
&& Objects.equals(vocabularyName(), other.vocabularyName());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("MedicalTranscriptionSetting").add("ShowSpeakerLabels", showSpeakerLabels())
.add("MaxSpeakerLabels", maxSpeakerLabels()).add("ChannelIdentification", channelIdentification())
.add("ShowAlternatives", showAlternatives()).add("MaxAlternatives", maxAlternatives())
.add("VocabularyName", vocabularyName()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ShowSpeakerLabels":
return Optional.ofNullable(clazz.cast(showSpeakerLabels()));
case "MaxSpeakerLabels":
return Optional.ofNullable(clazz.cast(maxSpeakerLabels()));
case "ChannelIdentification":
return Optional.ofNullable(clazz.cast(channelIdentification()));
case "ShowAlternatives":
return Optional.ofNullable(clazz.cast(showAlternatives()));
case "MaxAlternatives":
return Optional.ofNullable(clazz.cast(maxAlternatives()));
case "VocabularyName":
return Optional.ofNullable(clazz.cast(vocabularyName()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function