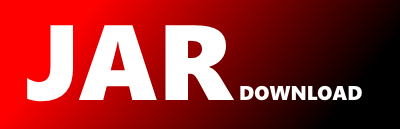
software.amazon.awssdk.services.transcribe.model.TranscriptionJob Maven / Gradle / Ivy
Show all versions of transcribe Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.transcribe.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Describes an asynchronous transcription job that was created with the StartTranscriptionJob
operation.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class TranscriptionJob implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField TRANSCRIPTION_JOB_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TranscriptionJobName").getter(getter(TranscriptionJob::transcriptionJobName))
.setter(setter(Builder::transcriptionJobName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TranscriptionJobName").build())
.build();
private static final SdkField TRANSCRIPTION_JOB_STATUS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TranscriptionJobStatus").getter(getter(TranscriptionJob::transcriptionJobStatusAsString))
.setter(setter(Builder::transcriptionJobStatus))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TranscriptionJobStatus").build())
.build();
private static final SdkField LANGUAGE_CODE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("LanguageCode").getter(getter(TranscriptionJob::languageCodeAsString))
.setter(setter(Builder::languageCode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LanguageCode").build()).build();
private static final SdkField MEDIA_SAMPLE_RATE_HERTZ_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("MediaSampleRateHertz").getter(getter(TranscriptionJob::mediaSampleRateHertz))
.setter(setter(Builder::mediaSampleRateHertz))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MediaSampleRateHertz").build())
.build();
private static final SdkField MEDIA_FORMAT_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("MediaFormat").getter(getter(TranscriptionJob::mediaFormatAsString)).setter(setter(Builder::mediaFormat))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MediaFormat").build()).build();
private static final SdkField MEDIA_FIELD = SdkField. builder(MarshallingType.SDK_POJO).memberName("Media")
.getter(getter(TranscriptionJob::media)).setter(setter(Builder::media)).constructor(Media::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Media").build()).build();
private static final SdkField TRANSCRIPT_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("Transcript").getter(getter(TranscriptionJob::transcript)).setter(setter(Builder::transcript))
.constructor(Transcript::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Transcript").build()).build();
private static final SdkField START_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("StartTime").getter(getter(TranscriptionJob::startTime)).setter(setter(Builder::startTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StartTime").build()).build();
private static final SdkField CREATION_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("CreationTime").getter(getter(TranscriptionJob::creationTime)).setter(setter(Builder::creationTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CreationTime").build()).build();
private static final SdkField COMPLETION_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("CompletionTime").getter(getter(TranscriptionJob::completionTime))
.setter(setter(Builder::completionTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CompletionTime").build()).build();
private static final SdkField FAILURE_REASON_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("FailureReason").getter(getter(TranscriptionJob::failureReason)).setter(setter(Builder::failureReason))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("FailureReason").build()).build();
private static final SdkField SETTINGS_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("Settings").getter(getter(TranscriptionJob::settings)).setter(setter(Builder::settings))
.constructor(Settings::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Settings").build()).build();
private static final SdkField MODEL_SETTINGS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("ModelSettings")
.getter(getter(TranscriptionJob::modelSettings)).setter(setter(Builder::modelSettings))
.constructor(ModelSettings::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ModelSettings").build()).build();
private static final SdkField JOB_EXECUTION_SETTINGS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("JobExecutionSettings")
.getter(getter(TranscriptionJob::jobExecutionSettings)).setter(setter(Builder::jobExecutionSettings))
.constructor(JobExecutionSettings::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("JobExecutionSettings").build())
.build();
private static final SdkField CONTENT_REDACTION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("ContentRedaction")
.getter(getter(TranscriptionJob::contentRedaction)).setter(setter(Builder::contentRedaction))
.constructor(ContentRedaction::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ContentRedaction").build()).build();
private static final SdkField IDENTIFY_LANGUAGE_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("IdentifyLanguage").getter(getter(TranscriptionJob::identifyLanguage))
.setter(setter(Builder::identifyLanguage))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IdentifyLanguage").build()).build();
private static final SdkField> LANGUAGE_OPTIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("LanguageOptions")
.getter(getter(TranscriptionJob::languageOptionsAsStrings))
.setter(setter(Builder::languageOptionsWithStrings))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LanguageOptions").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField IDENTIFIED_LANGUAGE_SCORE_FIELD = SdkField. builder(MarshallingType.FLOAT)
.memberName("IdentifiedLanguageScore").getter(getter(TranscriptionJob::identifiedLanguageScore))
.setter(setter(Builder::identifiedLanguageScore))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IdentifiedLanguageScore").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(TRANSCRIPTION_JOB_NAME_FIELD,
TRANSCRIPTION_JOB_STATUS_FIELD, LANGUAGE_CODE_FIELD, MEDIA_SAMPLE_RATE_HERTZ_FIELD, MEDIA_FORMAT_FIELD, MEDIA_FIELD,
TRANSCRIPT_FIELD, START_TIME_FIELD, CREATION_TIME_FIELD, COMPLETION_TIME_FIELD, FAILURE_REASON_FIELD, SETTINGS_FIELD,
MODEL_SETTINGS_FIELD, JOB_EXECUTION_SETTINGS_FIELD, CONTENT_REDACTION_FIELD, IDENTIFY_LANGUAGE_FIELD,
LANGUAGE_OPTIONS_FIELD, IDENTIFIED_LANGUAGE_SCORE_FIELD));
private static final long serialVersionUID = 1L;
private final String transcriptionJobName;
private final String transcriptionJobStatus;
private final String languageCode;
private final Integer mediaSampleRateHertz;
private final String mediaFormat;
private final Media media;
private final Transcript transcript;
private final Instant startTime;
private final Instant creationTime;
private final Instant completionTime;
private final String failureReason;
private final Settings settings;
private final ModelSettings modelSettings;
private final JobExecutionSettings jobExecutionSettings;
private final ContentRedaction contentRedaction;
private final Boolean identifyLanguage;
private final List languageOptions;
private final Float identifiedLanguageScore;
private TranscriptionJob(BuilderImpl builder) {
this.transcriptionJobName = builder.transcriptionJobName;
this.transcriptionJobStatus = builder.transcriptionJobStatus;
this.languageCode = builder.languageCode;
this.mediaSampleRateHertz = builder.mediaSampleRateHertz;
this.mediaFormat = builder.mediaFormat;
this.media = builder.media;
this.transcript = builder.transcript;
this.startTime = builder.startTime;
this.creationTime = builder.creationTime;
this.completionTime = builder.completionTime;
this.failureReason = builder.failureReason;
this.settings = builder.settings;
this.modelSettings = builder.modelSettings;
this.jobExecutionSettings = builder.jobExecutionSettings;
this.contentRedaction = builder.contentRedaction;
this.identifyLanguage = builder.identifyLanguage;
this.languageOptions = builder.languageOptions;
this.identifiedLanguageScore = builder.identifiedLanguageScore;
}
/**
*
* The name of the transcription job.
*
*
* @return The name of the transcription job.
*/
public final String transcriptionJobName() {
return transcriptionJobName;
}
/**
*
* The status of the transcription job.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #transcriptionJobStatus} will return {@link TranscriptionJobStatus#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #transcriptionJobStatusAsString}.
*
*
* @return The status of the transcription job.
* @see TranscriptionJobStatus
*/
public final TranscriptionJobStatus transcriptionJobStatus() {
return TranscriptionJobStatus.fromValue(transcriptionJobStatus);
}
/**
*
* The status of the transcription job.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #transcriptionJobStatus} will return {@link TranscriptionJobStatus#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #transcriptionJobStatusAsString}.
*
*
* @return The status of the transcription job.
* @see TranscriptionJobStatus
*/
public final String transcriptionJobStatusAsString() {
return transcriptionJobStatus;
}
/**
*
* The language code for the input speech.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #languageCode} will
* return {@link LanguageCode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #languageCodeAsString}.
*
*
* @return The language code for the input speech.
* @see LanguageCode
*/
public final LanguageCode languageCode() {
return LanguageCode.fromValue(languageCode);
}
/**
*
* The language code for the input speech.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #languageCode} will
* return {@link LanguageCode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #languageCodeAsString}.
*
*
* @return The language code for the input speech.
* @see LanguageCode
*/
public final String languageCodeAsString() {
return languageCode;
}
/**
*
* The sample rate, in Hertz, of the audio track in the input media file.
*
*
* @return The sample rate, in Hertz, of the audio track in the input media file.
*/
public final Integer mediaSampleRateHertz() {
return mediaSampleRateHertz;
}
/**
*
* The format of the input media file.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #mediaFormat} will
* return {@link MediaFormat#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #mediaFormatAsString}.
*
*
* @return The format of the input media file.
* @see MediaFormat
*/
public final MediaFormat mediaFormat() {
return MediaFormat.fromValue(mediaFormat);
}
/**
*
* The format of the input media file.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #mediaFormat} will
* return {@link MediaFormat#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #mediaFormatAsString}.
*
*
* @return The format of the input media file.
* @see MediaFormat
*/
public final String mediaFormatAsString() {
return mediaFormat;
}
/**
*
* An object that describes the input media for the transcription job.
*
*
* @return An object that describes the input media for the transcription job.
*/
public final Media media() {
return media;
}
/**
*
* An object that describes the output of the transcription job.
*
*
* @return An object that describes the output of the transcription job.
*/
public final Transcript transcript() {
return transcript;
}
/**
*
* A timestamp that shows with the job was started processing.
*
*
* @return A timestamp that shows with the job was started processing.
*/
public final Instant startTime() {
return startTime;
}
/**
*
* A timestamp that shows when the job was created.
*
*
* @return A timestamp that shows when the job was created.
*/
public final Instant creationTime() {
return creationTime;
}
/**
*
* A timestamp that shows when the job was completed.
*
*
* @return A timestamp that shows when the job was completed.
*/
public final Instant completionTime() {
return completionTime;
}
/**
*
* If the TranscriptionJobStatus
field is FAILED
, this field contains information about
* why the job failed.
*
*
* The FailureReason
field can contain one of the following values:
*
*
* -
*
* Unsupported media format
- The media format specified in the MediaFormat
field of the
* request isn't valid. See the description of the MediaFormat
field for a list of valid values.
*
*
* -
*
* The media format provided does not match the detected media format
- The media format of the audio
* file doesn't match the format specified in the MediaFormat
field in the request. Check the media
* format of your media file and make sure that the two values match.
*
*
* -
*
* Invalid sample rate for audio file
- The sample rate specified in the
* MediaSampleRateHertz
of the request isn't valid. The sample rate must be between 8000 and 48000
* Hertz.
*
*
* -
*
* The sample rate provided does not match the detected sample rate
- The sample rate in the audio file
* doesn't match the sample rate specified in the MediaSampleRateHertz
field in the request. Check the
* sample rate of your media file and make sure that the two values match.
*
*
* -
*
* Invalid file size: file size too large
- The size of your audio file is larger than Amazon
* Transcribe can process. For more information, see Limits in the Amazon
* Transcribe Developer Guide.
*
*
* -
*
* Invalid number of channels: number of channels too large
- Your audio contains more channels than
* Amazon Transcribe is configured to process. To request additional channels, see Amazon
* Transcribe Limits in the Amazon Web Services General Reference.
*
*
*
*
* @return If the TranscriptionJobStatus
field is FAILED
, this field contains information
* about why the job failed.
*
* The FailureReason
field can contain one of the following values:
*
*
* -
*
* Unsupported media format
- The media format specified in the MediaFormat
field
* of the request isn't valid. See the description of the MediaFormat
field for a list of valid
* values.
*
*
* -
*
* The media format provided does not match the detected media format
- The media format of the
* audio file doesn't match the format specified in the MediaFormat
field in the request. Check
* the media format of your media file and make sure that the two values match.
*
*
* -
*
* Invalid sample rate for audio file
- The sample rate specified in the
* MediaSampleRateHertz
of the request isn't valid. The sample rate must be between 8000 and
* 48000 Hertz.
*
*
* -
*
* The sample rate provided does not match the detected sample rate
- The sample rate in the
* audio file doesn't match the sample rate specified in the MediaSampleRateHertz
field in the
* request. Check the sample rate of your media file and make sure that the two values match.
*
*
* -
*
* Invalid file size: file size too large
- The size of your audio file is larger than Amazon
* Transcribe can process. For more information, see Limits in the
* Amazon Transcribe Developer Guide.
*
*
* -
*
* Invalid number of channels: number of channels too large
- Your audio contains more channels
* than Amazon Transcribe is configured to process. To request additional channels, see Amazon Transcribe Limits in the Amazon Web Services General Reference.
*
*
*/
public final String failureReason() {
return failureReason;
}
/**
*
* Optional settings for the transcription job. Use these settings to turn on speaker recognition, to set the
* maximum number of speakers that should be identified and to specify a custom vocabulary to use when processing
* the transcription job.
*
*
* @return Optional settings for the transcription job. Use these settings to turn on speaker recognition, to set
* the maximum number of speakers that should be identified and to specify a custom vocabulary to use when
* processing the transcription job.
*/
public final Settings settings() {
return settings;
}
/**
*
* An object containing the details of your custom language model.
*
*
* @return An object containing the details of your custom language model.
*/
public final ModelSettings modelSettings() {
return modelSettings;
}
/**
*
* Provides information about how a transcription job is executed.
*
*
* @return Provides information about how a transcription job is executed.
*/
public final JobExecutionSettings jobExecutionSettings() {
return jobExecutionSettings;
}
/**
*
* An object that describes content redaction settings for the transcription job.
*
*
* @return An object that describes content redaction settings for the transcription job.
*/
public final ContentRedaction contentRedaction() {
return contentRedaction;
}
/**
*
* A value that shows if automatic language identification was enabled for a transcription job.
*
*
* @return A value that shows if automatic language identification was enabled for a transcription job.
*/
public final Boolean identifyLanguage() {
return identifyLanguage;
}
/**
*
* An object that shows the optional array of languages inputted for transcription jobs with automatic language
* identification enabled.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasLanguageOptions()} to see if a value was sent in this field.
*
*
* @return An object that shows the optional array of languages inputted for transcription jobs with automatic
* language identification enabled.
*/
public final List languageOptions() {
return LanguageOptionsCopier.copyStringToEnum(languageOptions);
}
/**
* Returns true if the LanguageOptions property was specified by the sender (it may be empty), or false if the
* sender did not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS
* service.
*/
public final boolean hasLanguageOptions() {
return languageOptions != null && !(languageOptions instanceof SdkAutoConstructList);
}
/**
*
* An object that shows the optional array of languages inputted for transcription jobs with automatic language
* identification enabled.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasLanguageOptions()} to see if a value was sent in this field.
*
*
* @return An object that shows the optional array of languages inputted for transcription jobs with automatic
* language identification enabled.
*/
public final List languageOptionsAsStrings() {
return languageOptions;
}
/**
*
* A value between zero and one that Amazon Transcribe assigned to the language that it identified in the source
* audio. Larger values indicate that Amazon Transcribe has higher confidence in the language it identified.
*
*
* @return A value between zero and one that Amazon Transcribe assigned to the language that it identified in the
* source audio. Larger values indicate that Amazon Transcribe has higher confidence in the language it
* identified.
*/
public final Float identifiedLanguageScore() {
return identifiedLanguageScore;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(transcriptionJobName());
hashCode = 31 * hashCode + Objects.hashCode(transcriptionJobStatusAsString());
hashCode = 31 * hashCode + Objects.hashCode(languageCodeAsString());
hashCode = 31 * hashCode + Objects.hashCode(mediaSampleRateHertz());
hashCode = 31 * hashCode + Objects.hashCode(mediaFormatAsString());
hashCode = 31 * hashCode + Objects.hashCode(media());
hashCode = 31 * hashCode + Objects.hashCode(transcript());
hashCode = 31 * hashCode + Objects.hashCode(startTime());
hashCode = 31 * hashCode + Objects.hashCode(creationTime());
hashCode = 31 * hashCode + Objects.hashCode(completionTime());
hashCode = 31 * hashCode + Objects.hashCode(failureReason());
hashCode = 31 * hashCode + Objects.hashCode(settings());
hashCode = 31 * hashCode + Objects.hashCode(modelSettings());
hashCode = 31 * hashCode + Objects.hashCode(jobExecutionSettings());
hashCode = 31 * hashCode + Objects.hashCode(contentRedaction());
hashCode = 31 * hashCode + Objects.hashCode(identifyLanguage());
hashCode = 31 * hashCode + Objects.hashCode(hasLanguageOptions() ? languageOptionsAsStrings() : null);
hashCode = 31 * hashCode + Objects.hashCode(identifiedLanguageScore());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof TranscriptionJob)) {
return false;
}
TranscriptionJob other = (TranscriptionJob) obj;
return Objects.equals(transcriptionJobName(), other.transcriptionJobName())
&& Objects.equals(transcriptionJobStatusAsString(), other.transcriptionJobStatusAsString())
&& Objects.equals(languageCodeAsString(), other.languageCodeAsString())
&& Objects.equals(mediaSampleRateHertz(), other.mediaSampleRateHertz())
&& Objects.equals(mediaFormatAsString(), other.mediaFormatAsString()) && Objects.equals(media(), other.media())
&& Objects.equals(transcript(), other.transcript()) && Objects.equals(startTime(), other.startTime())
&& Objects.equals(creationTime(), other.creationTime())
&& Objects.equals(completionTime(), other.completionTime())
&& Objects.equals(failureReason(), other.failureReason()) && Objects.equals(settings(), other.settings())
&& Objects.equals(modelSettings(), other.modelSettings())
&& Objects.equals(jobExecutionSettings(), other.jobExecutionSettings())
&& Objects.equals(contentRedaction(), other.contentRedaction())
&& Objects.equals(identifyLanguage(), other.identifyLanguage())
&& hasLanguageOptions() == other.hasLanguageOptions()
&& Objects.equals(languageOptionsAsStrings(), other.languageOptionsAsStrings())
&& Objects.equals(identifiedLanguageScore(), other.identifiedLanguageScore());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("TranscriptionJob").add("TranscriptionJobName", transcriptionJobName())
.add("TranscriptionJobStatus", transcriptionJobStatusAsString()).add("LanguageCode", languageCodeAsString())
.add("MediaSampleRateHertz", mediaSampleRateHertz()).add("MediaFormat", mediaFormatAsString())
.add("Media", media()).add("Transcript", transcript()).add("StartTime", startTime())
.add("CreationTime", creationTime()).add("CompletionTime", completionTime())
.add("FailureReason", failureReason()).add("Settings", settings()).add("ModelSettings", modelSettings())
.add("JobExecutionSettings", jobExecutionSettings()).add("ContentRedaction", contentRedaction())
.add("IdentifyLanguage", identifyLanguage())
.add("LanguageOptions", hasLanguageOptions() ? languageOptionsAsStrings() : null)
.add("IdentifiedLanguageScore", identifiedLanguageScore()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "TranscriptionJobName":
return Optional.ofNullable(clazz.cast(transcriptionJobName()));
case "TranscriptionJobStatus":
return Optional.ofNullable(clazz.cast(transcriptionJobStatusAsString()));
case "LanguageCode":
return Optional.ofNullable(clazz.cast(languageCodeAsString()));
case "MediaSampleRateHertz":
return Optional.ofNullable(clazz.cast(mediaSampleRateHertz()));
case "MediaFormat":
return Optional.ofNullable(clazz.cast(mediaFormatAsString()));
case "Media":
return Optional.ofNullable(clazz.cast(media()));
case "Transcript":
return Optional.ofNullable(clazz.cast(transcript()));
case "StartTime":
return Optional.ofNullable(clazz.cast(startTime()));
case "CreationTime":
return Optional.ofNullable(clazz.cast(creationTime()));
case "CompletionTime":
return Optional.ofNullable(clazz.cast(completionTime()));
case "FailureReason":
return Optional.ofNullable(clazz.cast(failureReason()));
case "Settings":
return Optional.ofNullable(clazz.cast(settings()));
case "ModelSettings":
return Optional.ofNullable(clazz.cast(modelSettings()));
case "JobExecutionSettings":
return Optional.ofNullable(clazz.cast(jobExecutionSettings()));
case "ContentRedaction":
return Optional.ofNullable(clazz.cast(contentRedaction()));
case "IdentifyLanguage":
return Optional.ofNullable(clazz.cast(identifyLanguage()));
case "LanguageOptions":
return Optional.ofNullable(clazz.cast(languageOptionsAsStrings()));
case "IdentifiedLanguageScore":
return Optional.ofNullable(clazz.cast(identifiedLanguageScore()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function