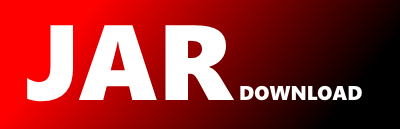
software.amazon.awssdk.services.transcribe.model.UpdateMedicalVocabularyRequest Maven / Gradle / Ivy
Show all versions of transcribe Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.transcribe.model;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class UpdateMedicalVocabularyRequest extends TranscribeRequest implements
ToCopyableBuilder {
private static final SdkField VOCABULARY_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("VocabularyName").getter(getter(UpdateMedicalVocabularyRequest::vocabularyName))
.setter(setter(Builder::vocabularyName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("VocabularyName").build()).build();
private static final SdkField LANGUAGE_CODE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("LanguageCode").getter(getter(UpdateMedicalVocabularyRequest::languageCodeAsString))
.setter(setter(Builder::languageCode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LanguageCode").build()).build();
private static final SdkField VOCABULARY_FILE_URI_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("VocabularyFileUri").getter(getter(UpdateMedicalVocabularyRequest::vocabularyFileUri))
.setter(setter(Builder::vocabularyFileUri))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("VocabularyFileUri").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(VOCABULARY_NAME_FIELD,
LANGUAGE_CODE_FIELD, VOCABULARY_FILE_URI_FIELD));
private final String vocabularyName;
private final String languageCode;
private final String vocabularyFileUri;
private UpdateMedicalVocabularyRequest(BuilderImpl builder) {
super(builder);
this.vocabularyName = builder.vocabularyName;
this.languageCode = builder.languageCode;
this.vocabularyFileUri = builder.vocabularyFileUri;
}
/**
*
* The name of the custom medical vocabulary you want to update. Vocabulary names are case sensitive.
*
*
* @return The name of the custom medical vocabulary you want to update. Vocabulary names are case sensitive.
*/
public final String vocabularyName() {
return vocabularyName;
}
/**
*
* The language code that represents the language of the entries in the custom vocabulary you want to update. US
* English (en-US
) is the only language supported with Amazon Transcribe Medical.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #languageCode} will
* return {@link LanguageCode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #languageCodeAsString}.
*
*
* @return The language code that represents the language of the entries in the custom vocabulary you want to
* update. US English (en-US
) is the only language supported with Amazon Transcribe Medical.
* @see LanguageCode
*/
public final LanguageCode languageCode() {
return LanguageCode.fromValue(languageCode);
}
/**
*
* The language code that represents the language of the entries in the custom vocabulary you want to update. US
* English (en-US
) is the only language supported with Amazon Transcribe Medical.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #languageCode} will
* return {@link LanguageCode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #languageCodeAsString}.
*
*
* @return The language code that represents the language of the entries in the custom vocabulary you want to
* update. US English (en-US
) is the only language supported with Amazon Transcribe Medical.
* @see LanguageCode
*/
public final String languageCodeAsString() {
return languageCode;
}
/**
*
* The Amazon S3 location of the text file that contains your custom medical vocabulary. The URI must be located in
* the same Amazon Web Services Region as the resource you're calling.
*
*
* Here's an example URI path: s3://DOC-EXAMPLE-BUCKET/my-vocab-file.txt
*
*
* @return The Amazon S3 location of the text file that contains your custom medical vocabulary. The URI must be
* located in the same Amazon Web Services Region as the resource you're calling.
*
* Here's an example URI path: s3://DOC-EXAMPLE-BUCKET/my-vocab-file.txt
*/
public final String vocabularyFileUri() {
return vocabularyFileUri;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(vocabularyName());
hashCode = 31 * hashCode + Objects.hashCode(languageCodeAsString());
hashCode = 31 * hashCode + Objects.hashCode(vocabularyFileUri());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof UpdateMedicalVocabularyRequest)) {
return false;
}
UpdateMedicalVocabularyRequest other = (UpdateMedicalVocabularyRequest) obj;
return Objects.equals(vocabularyName(), other.vocabularyName())
&& Objects.equals(languageCodeAsString(), other.languageCodeAsString())
&& Objects.equals(vocabularyFileUri(), other.vocabularyFileUri());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("UpdateMedicalVocabularyRequest").add("VocabularyName", vocabularyName())
.add("LanguageCode", languageCodeAsString()).add("VocabularyFileUri", vocabularyFileUri()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "VocabularyName":
return Optional.ofNullable(clazz.cast(vocabularyName()));
case "LanguageCode":
return Optional.ofNullable(clazz.cast(languageCodeAsString()));
case "VocabularyFileUri":
return Optional.ofNullable(clazz.cast(vocabularyFileUri()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
*
* Here's an example URI path: s3://DOC-EXAMPLE-BUCKET/my-vocab-file.txt
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder vocabularyFileUri(String vocabularyFileUri);
@Override
Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration);
@Override
Builder overrideConfiguration(Consumer builderConsumer);
}
static final class BuilderImpl extends TranscribeRequest.BuilderImpl implements Builder {
private String vocabularyName;
private String languageCode;
private String vocabularyFileUri;
private BuilderImpl() {
}
private BuilderImpl(UpdateMedicalVocabularyRequest model) {
super(model);
vocabularyName(model.vocabularyName);
languageCode(model.languageCode);
vocabularyFileUri(model.vocabularyFileUri);
}
public final String getVocabularyName() {
return vocabularyName;
}
public final void setVocabularyName(String vocabularyName) {
this.vocabularyName = vocabularyName;
}
@Override
public final Builder vocabularyName(String vocabularyName) {
this.vocabularyName = vocabularyName;
return this;
}
public final String getLanguageCode() {
return languageCode;
}
public final void setLanguageCode(String languageCode) {
this.languageCode = languageCode;
}
@Override
public final Builder languageCode(String languageCode) {
this.languageCode = languageCode;
return this;
}
@Override
public final Builder languageCode(LanguageCode languageCode) {
this.languageCode(languageCode == null ? null : languageCode.toString());
return this;
}
public final String getVocabularyFileUri() {
return vocabularyFileUri;
}
public final void setVocabularyFileUri(String vocabularyFileUri) {
this.vocabularyFileUri = vocabularyFileUri;
}
@Override
public final Builder vocabularyFileUri(String vocabularyFileUri) {
this.vocabularyFileUri = vocabularyFileUri;
return this;
}
@Override
public Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration) {
super.overrideConfiguration(overrideConfiguration);
return this;
}
@Override
public Builder overrideConfiguration(Consumer builderConsumer) {
super.overrideConfiguration(builderConsumer);
return this;
}
@Override
public UpdateMedicalVocabularyRequest build() {
return new UpdateMedicalVocabularyRequest(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
}
}