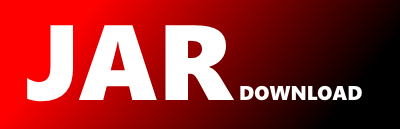
software.amazon.awssdk.services.transcribe.model.CreateLanguageModelRequest Maven / Gradle / Ivy
Show all versions of transcribe Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.transcribe.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class CreateLanguageModelRequest extends TranscribeRequest implements
ToCopyableBuilder {
private static final SdkField LANGUAGE_CODE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("LanguageCode").getter(getter(CreateLanguageModelRequest::languageCodeAsString))
.setter(setter(Builder::languageCode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LanguageCode").build()).build();
private static final SdkField BASE_MODEL_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("BaseModelName").getter(getter(CreateLanguageModelRequest::baseModelNameAsString))
.setter(setter(Builder::baseModelName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("BaseModelName").build()).build();
private static final SdkField MODEL_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ModelName").getter(getter(CreateLanguageModelRequest::modelName)).setter(setter(Builder::modelName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ModelName").build()).build();
private static final SdkField INPUT_DATA_CONFIG_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("InputDataConfig")
.getter(getter(CreateLanguageModelRequest::inputDataConfig)).setter(setter(Builder::inputDataConfig))
.constructor(InputDataConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InputDataConfig").build()).build();
private static final SdkField> TAGS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Tags")
.getter(getter(CreateLanguageModelRequest::tags))
.setter(setter(Builder::tags))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Tags").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Tag::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(LANGUAGE_CODE_FIELD,
BASE_MODEL_NAME_FIELD, MODEL_NAME_FIELD, INPUT_DATA_CONFIG_FIELD, TAGS_FIELD));
private final String languageCode;
private final String baseModelName;
private final String modelName;
private final InputDataConfig inputDataConfig;
private final List tags;
private CreateLanguageModelRequest(BuilderImpl builder) {
super(builder);
this.languageCode = builder.languageCode;
this.baseModelName = builder.baseModelName;
this.modelName = builder.modelName;
this.inputDataConfig = builder.inputDataConfig;
this.tags = builder.tags;
}
/**
*
* The language code that represents the language of your model. Each language model must contain terms in only one
* language, and the language you select for your model must match the language of your training and tuning data.
*
*
* For a list of supported languages and their associated language codes, refer to the Supported languages table.
* Note that U.S. English (en-US
) is the only language supported with Amazon Transcribe Medical.
*
*
* A custom language model can only be used to transcribe files in the same language as the model. For example, if
* you create a language model using US English (en-US
), you can only apply this model to files that
* contain English audio.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #languageCode} will
* return {@link CLMLanguageCode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #languageCodeAsString}.
*
*
* @return The language code that represents the language of your model. Each language model must contain terms in
* only one language, and the language you select for your model must match the language of your training
* and tuning data.
*
* For a list of supported languages and their associated language codes, refer to the Supported languages
* table. Note that U.S. English (en-US
) is the only language supported with Amazon Transcribe
* Medical.
*
*
* A custom language model can only be used to transcribe files in the same language as the model. For
* example, if you create a language model using US English (en-US
), you can only apply this
* model to files that contain English audio.
* @see CLMLanguageCode
*/
public final CLMLanguageCode languageCode() {
return CLMLanguageCode.fromValue(languageCode);
}
/**
*
* The language code that represents the language of your model. Each language model must contain terms in only one
* language, and the language you select for your model must match the language of your training and tuning data.
*
*
* For a list of supported languages and their associated language codes, refer to the Supported languages table.
* Note that U.S. English (en-US
) is the only language supported with Amazon Transcribe Medical.
*
*
* A custom language model can only be used to transcribe files in the same language as the model. For example, if
* you create a language model using US English (en-US
), you can only apply this model to files that
* contain English audio.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #languageCode} will
* return {@link CLMLanguageCode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #languageCodeAsString}.
*
*
* @return The language code that represents the language of your model. Each language model must contain terms in
* only one language, and the language you select for your model must match the language of your training
* and tuning data.
*
* For a list of supported languages and their associated language codes, refer to the Supported languages
* table. Note that U.S. English (en-US
) is the only language supported with Amazon Transcribe
* Medical.
*
*
* A custom language model can only be used to transcribe files in the same language as the model. For
* example, if you create a language model using US English (en-US
), you can only apply this
* model to files that contain English audio.
* @see CLMLanguageCode
*/
public final String languageCodeAsString() {
return languageCode;
}
/**
*
* The Amazon Transcribe standard language model, or base model, used to create your custom language model. Amazon
* Transcribe offers two options for base models: Wideband and Narrowband.
*
*
* If the audio you want to transcribe has a sample rate of 16,000 Hz or greater, choose WideBand
. To
* transcribe audio with a sample rate less than 16,000 Hz, choose NarrowBand
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #baseModelName}
* will return {@link BaseModelName#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #baseModelNameAsString}.
*
*
* @return The Amazon Transcribe standard language model, or base model, used to create your custom language model.
* Amazon Transcribe offers two options for base models: Wideband and Narrowband.
*
* If the audio you want to transcribe has a sample rate of 16,000 Hz or greater, choose
* WideBand
. To transcribe audio with a sample rate less than 16,000 Hz, choose
* NarrowBand
.
* @see BaseModelName
*/
public final BaseModelName baseModelName() {
return BaseModelName.fromValue(baseModelName);
}
/**
*
* The Amazon Transcribe standard language model, or base model, used to create your custom language model. Amazon
* Transcribe offers two options for base models: Wideband and Narrowband.
*
*
* If the audio you want to transcribe has a sample rate of 16,000 Hz or greater, choose WideBand
. To
* transcribe audio with a sample rate less than 16,000 Hz, choose NarrowBand
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #baseModelName}
* will return {@link BaseModelName#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #baseModelNameAsString}.
*
*
* @return The Amazon Transcribe standard language model, or base model, used to create your custom language model.
* Amazon Transcribe offers two options for base models: Wideband and Narrowband.
*
* If the audio you want to transcribe has a sample rate of 16,000 Hz or greater, choose
* WideBand
. To transcribe audio with a sample rate less than 16,000 Hz, choose
* NarrowBand
.
* @see BaseModelName
*/
public final String baseModelNameAsString() {
return baseModelName;
}
/**
*
* A unique name, chosen by you, for your custom language model.
*
*
* This name is case sensitive, cannot contain spaces, and must be unique within an Amazon Web Services account. If
* you try to create a new language model with the same name as an existing language model, you get a
* ConflictException
error.
*
*
* @return A unique name, chosen by you, for your custom language model.
*
* This name is case sensitive, cannot contain spaces, and must be unique within an Amazon Web Services
* account. If you try to create a new language model with the same name as an existing language model, you
* get a ConflictException
error.
*/
public final String modelName() {
return modelName;
}
/**
*
* Contains the Amazon S3 location of the training data you want to use to create a new custom language model, and
* permissions to access this location.
*
*
* When using InputDataConfig
, you must include these sub-parameters: S3Uri
, which is the
* Amazon S3 location of your training data, and DataAccessRoleArn
, which is the Amazon Resource Name
* (ARN) of the role that has permission to access your specified Amazon S3 location. You can optionally include
* TuningDataS3Uri
, which is the Amazon S3 location of your tuning data. If you specify different
* Amazon S3 locations for training and tuning data, the ARN you use must have permissions to access both locations.
*
*
* @return Contains the Amazon S3 location of the training data you want to use to create a new custom language
* model, and permissions to access this location.
*
* When using InputDataConfig
, you must include these sub-parameters: S3Uri
, which
* is the Amazon S3 location of your training data, and DataAccessRoleArn
, which is the Amazon
* Resource Name (ARN) of the role that has permission to access your specified Amazon S3 location. You can
* optionally include TuningDataS3Uri
, which is the Amazon S3 location of your tuning data. If
* you specify different Amazon S3 locations for training and tuning data, the ARN you use must have
* permissions to access both locations.
*/
public final InputDataConfig inputDataConfig() {
return inputDataConfig;
}
/**
* For responses, this returns true if the service returned a value for the Tags property. This DOES NOT check that
* the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is useful
* because the SDK will never return a null collection or map, but you may need to differentiate between the service
* returning nothing (or null) and the service returning an empty collection or map. For requests, this returns true
* if a value for the property was specified in the request builder, and false if a value was not specified.
*/
public final boolean hasTags() {
return tags != null && !(tags instanceof SdkAutoConstructList);
}
/**
*
* Adds one or more custom tags, each in the form of a key:value pair, to a new custom language model at the time
* you create this new model.
*
*
* To learn more about using tags with Amazon Transcribe, refer to Tagging resources.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasTags} method.
*
*
* @return Adds one or more custom tags, each in the form of a key:value pair, to a new custom language model at the
* time you create this new model.
*
* To learn more about using tags with Amazon Transcribe, refer to Tagging resources.
*/
public final List tags() {
return tags;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(languageCodeAsString());
hashCode = 31 * hashCode + Objects.hashCode(baseModelNameAsString());
hashCode = 31 * hashCode + Objects.hashCode(modelName());
hashCode = 31 * hashCode + Objects.hashCode(inputDataConfig());
hashCode = 31 * hashCode + Objects.hashCode(hasTags() ? tags() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CreateLanguageModelRequest)) {
return false;
}
CreateLanguageModelRequest other = (CreateLanguageModelRequest) obj;
return Objects.equals(languageCodeAsString(), other.languageCodeAsString())
&& Objects.equals(baseModelNameAsString(), other.baseModelNameAsString())
&& Objects.equals(modelName(), other.modelName()) && Objects.equals(inputDataConfig(), other.inputDataConfig())
&& hasTags() == other.hasTags() && Objects.equals(tags(), other.tags());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("CreateLanguageModelRequest").add("LanguageCode", languageCodeAsString())
.add("BaseModelName", baseModelNameAsString()).add("ModelName", modelName())
.add("InputDataConfig", inputDataConfig()).add("Tags", hasTags() ? tags() : null).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "LanguageCode":
return Optional.ofNullable(clazz.cast(languageCodeAsString()));
case "BaseModelName":
return Optional.ofNullable(clazz.cast(baseModelNameAsString()));
case "ModelName":
return Optional.ofNullable(clazz.cast(modelName()));
case "InputDataConfig":
return Optional.ofNullable(clazz.cast(inputDataConfig()));
case "Tags":
return Optional.ofNullable(clazz.cast(tags()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
*
* For a list of supported languages and their associated language codes, refer to the Supported
* languages table. Note that U.S. English (en-US
) is the only language supported with
* Amazon Transcribe Medical.
*
*
* A custom language model can only be used to transcribe files in the same language as the model. For
* example, if you create a language model using US English (en-US
), you can only apply this
* model to files that contain English audio.
* @see CLMLanguageCode
* @return Returns a reference to this object so that method calls can be chained together.
* @see CLMLanguageCode
*/
Builder languageCode(String languageCode);
/**
*
* The language code that represents the language of your model. Each language model must contain terms in only
* one language, and the language you select for your model must match the language of your training and tuning
* data.
*
*
* For a list of supported languages and their associated language codes, refer to the Supported languages
* table. Note that U.S. English (en-US
) is the only language supported with Amazon Transcribe
* Medical.
*
*
* A custom language model can only be used to transcribe files in the same language as the model. For example,
* if you create a language model using US English (en-US
), you can only apply this model to files
* that contain English audio.
*
*
* @param languageCode
* The language code that represents the language of your model. Each language model must contain terms
* in only one language, and the language you select for your model must match the language of your
* training and tuning data.
*
* For a list of supported languages and their associated language codes, refer to the Supported
* languages table. Note that U.S. English (en-US
) is the only language supported with
* Amazon Transcribe Medical.
*
*
* A custom language model can only be used to transcribe files in the same language as the model. For
* example, if you create a language model using US English (en-US
), you can only apply this
* model to files that contain English audio.
* @see CLMLanguageCode
* @return Returns a reference to this object so that method calls can be chained together.
* @see CLMLanguageCode
*/
Builder languageCode(CLMLanguageCode languageCode);
/**
*
* The Amazon Transcribe standard language model, or base model, used to create your custom language model.
* Amazon Transcribe offers two options for base models: Wideband and Narrowband.
*
*
* If the audio you want to transcribe has a sample rate of 16,000 Hz or greater, choose WideBand
.
* To transcribe audio with a sample rate less than 16,000 Hz, choose NarrowBand
.
*
*
* @param baseModelName
* The Amazon Transcribe standard language model, or base model, used to create your custom language
* model. Amazon Transcribe offers two options for base models: Wideband and Narrowband.
*
* If the audio you want to transcribe has a sample rate of 16,000 Hz or greater, choose
* WideBand
. To transcribe audio with a sample rate less than 16,000 Hz, choose
* NarrowBand
.
* @see BaseModelName
* @return Returns a reference to this object so that method calls can be chained together.
* @see BaseModelName
*/
Builder baseModelName(String baseModelName);
/**
*
* The Amazon Transcribe standard language model, or base model, used to create your custom language model.
* Amazon Transcribe offers two options for base models: Wideband and Narrowband.
*
*
* If the audio you want to transcribe has a sample rate of 16,000 Hz or greater, choose WideBand
.
* To transcribe audio with a sample rate less than 16,000 Hz, choose NarrowBand
.
*
*
* @param baseModelName
* The Amazon Transcribe standard language model, or base model, used to create your custom language
* model. Amazon Transcribe offers two options for base models: Wideband and Narrowband.
*
* If the audio you want to transcribe has a sample rate of 16,000 Hz or greater, choose
* WideBand
. To transcribe audio with a sample rate less than 16,000 Hz, choose
* NarrowBand
.
* @see BaseModelName
* @return Returns a reference to this object so that method calls can be chained together.
* @see BaseModelName
*/
Builder baseModelName(BaseModelName baseModelName);
/**
*
* A unique name, chosen by you, for your custom language model.
*
*
* This name is case sensitive, cannot contain spaces, and must be unique within an Amazon Web Services account.
* If you try to create a new language model with the same name as an existing language model, you get a
* ConflictException
error.
*
*
* @param modelName
* A unique name, chosen by you, for your custom language model.
*
* This name is case sensitive, cannot contain spaces, and must be unique within an Amazon Web Services
* account. If you try to create a new language model with the same name as an existing language model,
* you get a ConflictException
error.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder modelName(String modelName);
/**
*
* Contains the Amazon S3 location of the training data you want to use to create a new custom language model,
* and permissions to access this location.
*
*
* When using InputDataConfig
, you must include these sub-parameters: S3Uri
, which is
* the Amazon S3 location of your training data, and DataAccessRoleArn
, which is the Amazon
* Resource Name (ARN) of the role that has permission to access your specified Amazon S3 location. You can
* optionally include TuningDataS3Uri
, which is the Amazon S3 location of your tuning data. If you
* specify different Amazon S3 locations for training and tuning data, the ARN you use must have permissions to
* access both locations.
*
*
* @param inputDataConfig
* Contains the Amazon S3 location of the training data you want to use to create a new custom language
* model, and permissions to access this location.
*
* When using InputDataConfig
, you must include these sub-parameters: S3Uri
,
* which is the Amazon S3 location of your training data, and DataAccessRoleArn
, which is
* the Amazon Resource Name (ARN) of the role that has permission to access your specified Amazon S3
* location. You can optionally include TuningDataS3Uri
, which is the Amazon S3 location of
* your tuning data. If you specify different Amazon S3 locations for training and tuning data, the ARN
* you use must have permissions to access both locations.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder inputDataConfig(InputDataConfig inputDataConfig);
/**
*
* Contains the Amazon S3 location of the training data you want to use to create a new custom language model,
* and permissions to access this location.
*
*
* When using InputDataConfig
, you must include these sub-parameters: S3Uri
, which is
* the Amazon S3 location of your training data, and DataAccessRoleArn
, which is the Amazon
* Resource Name (ARN) of the role that has permission to access your specified Amazon S3 location. You can
* optionally include TuningDataS3Uri
, which is the Amazon S3 location of your tuning data. If you
* specify different Amazon S3 locations for training and tuning data, the ARN you use must have permissions to
* access both locations.
*
* This is a convenience method that creates an instance of the {@link InputDataConfig.Builder} avoiding the
* need to create one manually via {@link InputDataConfig#builder()}.
*
* When the {@link Consumer} completes, {@link InputDataConfig.Builder#build()} is called immediately and its
* result is passed to {@link #inputDataConfig(InputDataConfig)}.
*
* @param inputDataConfig
* a consumer that will call methods on {@link InputDataConfig.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #inputDataConfig(InputDataConfig)
*/
default Builder inputDataConfig(Consumer inputDataConfig) {
return inputDataConfig(InputDataConfig.builder().applyMutation(inputDataConfig).build());
}
/**
*
* Adds one or more custom tags, each in the form of a key:value pair, to a new custom language model at the
* time you create this new model.
*
*
* To learn more about using tags with Amazon Transcribe, refer to Tagging resources.
*
*
* @param tags
* Adds one or more custom tags, each in the form of a key:value pair, to a new custom language model at
* the time you create this new model.
*
* To learn more about using tags with Amazon Transcribe, refer to Tagging resources.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder tags(Collection tags);
/**
*
* Adds one or more custom tags, each in the form of a key:value pair, to a new custom language model at the
* time you create this new model.
*
*
* To learn more about using tags with Amazon Transcribe, refer to Tagging resources.
*
*
* @param tags
* Adds one or more custom tags, each in the form of a key:value pair, to a new custom language model at
* the time you create this new model.
*
* To learn more about using tags with Amazon Transcribe, refer to Tagging resources.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder tags(Tag... tags);
/**
*
* Adds one or more custom tags, each in the form of a key:value pair, to a new custom language model at the
* time you create this new model.
*
*
* To learn more about using tags with Amazon Transcribe, refer to Tagging resources.
*
* This is a convenience method that creates an instance of the {@link List.Builder} avoiding the need to
* create one manually via {@link List#builder()}.
*
* When the {@link Consumer} completes, {@link List.Builder#build()} is called immediately and its result
* is passed to {@link #tags(List)}.
*
* @param tags
* a consumer that will call methods on {@link List.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #tags(List)
*/
Builder tags(Consumer... tags);
@Override
Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration);
@Override
Builder overrideConfiguration(Consumer builderConsumer);
}
static final class BuilderImpl extends TranscribeRequest.BuilderImpl implements Builder {
private String languageCode;
private String baseModelName;
private String modelName;
private InputDataConfig inputDataConfig;
private List tags = DefaultSdkAutoConstructList.getInstance();
private BuilderImpl() {
}
private BuilderImpl(CreateLanguageModelRequest model) {
super(model);
languageCode(model.languageCode);
baseModelName(model.baseModelName);
modelName(model.modelName);
inputDataConfig(model.inputDataConfig);
tags(model.tags);
}
public final String getLanguageCode() {
return languageCode;
}
public final void setLanguageCode(String languageCode) {
this.languageCode = languageCode;
}
@Override
public final Builder languageCode(String languageCode) {
this.languageCode = languageCode;
return this;
}
@Override
public final Builder languageCode(CLMLanguageCode languageCode) {
this.languageCode(languageCode == null ? null : languageCode.toString());
return this;
}
public final String getBaseModelName() {
return baseModelName;
}
public final void setBaseModelName(String baseModelName) {
this.baseModelName = baseModelName;
}
@Override
public final Builder baseModelName(String baseModelName) {
this.baseModelName = baseModelName;
return this;
}
@Override
public final Builder baseModelName(BaseModelName baseModelName) {
this.baseModelName(baseModelName == null ? null : baseModelName.toString());
return this;
}
public final String getModelName() {
return modelName;
}
public final void setModelName(String modelName) {
this.modelName = modelName;
}
@Override
public final Builder modelName(String modelName) {
this.modelName = modelName;
return this;
}
public final InputDataConfig.Builder getInputDataConfig() {
return inputDataConfig != null ? inputDataConfig.toBuilder() : null;
}
public final void setInputDataConfig(InputDataConfig.BuilderImpl inputDataConfig) {
this.inputDataConfig = inputDataConfig != null ? inputDataConfig.build() : null;
}
@Override
public final Builder inputDataConfig(InputDataConfig inputDataConfig) {
this.inputDataConfig = inputDataConfig;
return this;
}
public final List getTags() {
List result = TagListCopier.copyToBuilder(this.tags);
if (result instanceof SdkAutoConstructList) {
return null;
}
return result;
}
public final void setTags(Collection tags) {
this.tags = TagListCopier.copyFromBuilder(tags);
}
@Override
public final Builder tags(Collection tags) {
this.tags = TagListCopier.copy(tags);
return this;
}
@Override
@SafeVarargs
public final Builder tags(Tag... tags) {
tags(Arrays.asList(tags));
return this;
}
@Override
@SafeVarargs
public final Builder tags(Consumer... tags) {
tags(Stream.of(tags).map(c -> Tag.builder().applyMutation(c).build()).collect(Collectors.toList()));
return this;
}
@Override
public Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration) {
super.overrideConfiguration(overrideConfiguration);
return this;
}
@Override
public Builder overrideConfiguration(Consumer builderConsumer) {
super.overrideConfiguration(builderConsumer);
return this;
}
@Override
public CreateLanguageModelRequest build() {
return new CreateLanguageModelRequest(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
}
}