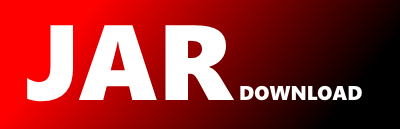
software.amazon.awssdk.services.transcribe.model.ContentRedaction Maven / Gradle / Ivy
Show all versions of transcribe Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.transcribe.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Allows you to redact or flag specified personally identifiable information (PII) in your transcript. If you use
* ContentRedaction
, you must also include the sub-parameters: PiiEntityTypes
,
* RedactionOutput
, and RedactionType
.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ContentRedaction implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField REDACTION_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("RedactionType").getter(getter(ContentRedaction::redactionTypeAsString))
.setter(setter(Builder::redactionType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RedactionType").build()).build();
private static final SdkField REDACTION_OUTPUT_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("RedactionOutput").getter(getter(ContentRedaction::redactionOutputAsString))
.setter(setter(Builder::redactionOutput))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RedactionOutput").build()).build();
private static final SdkField> PII_ENTITY_TYPES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("PiiEntityTypes")
.getter(getter(ContentRedaction::piiEntityTypesAsStrings))
.setter(setter(Builder::piiEntityTypesWithStrings))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PiiEntityTypes").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(REDACTION_TYPE_FIELD,
REDACTION_OUTPUT_FIELD, PII_ENTITY_TYPES_FIELD));
private static final long serialVersionUID = 1L;
private final String redactionType;
private final String redactionOutput;
private final List piiEntityTypes;
private ContentRedaction(BuilderImpl builder) {
this.redactionType = builder.redactionType;
this.redactionOutput = builder.redactionOutput;
this.piiEntityTypes = builder.piiEntityTypes;
}
/**
*
* Specify the category of information you want to redact; PII
(personally identifiable information) is
* the only valid value. You can use PiiEntityTypes
to choose which types of PII you want to redact.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #redactionType}
* will return {@link RedactionType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #redactionTypeAsString}.
*
*
* @return Specify the category of information you want to redact; PII
(personally identifiable
* information) is the only valid value. You can use PiiEntityTypes
to choose which types of
* PII you want to redact.
* @see RedactionType
*/
public final RedactionType redactionType() {
return RedactionType.fromValue(redactionType);
}
/**
*
* Specify the category of information you want to redact; PII
(personally identifiable information) is
* the only valid value. You can use PiiEntityTypes
to choose which types of PII you want to redact.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #redactionType}
* will return {@link RedactionType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #redactionTypeAsString}.
*
*
* @return Specify the category of information you want to redact; PII
(personally identifiable
* information) is the only valid value. You can use PiiEntityTypes
to choose which types of
* PII you want to redact.
* @see RedactionType
*/
public final String redactionTypeAsString() {
return redactionType;
}
/**
*
* Specify if you want only a redacted transcript, or if you want a redacted and an unredacted transcript.
*
*
* When you choose redacted
Amazon Transcribe creates only a redacted transcript.
*
*
* When you choose redacted_and_unredacted
Amazon Transcribe creates a redacted and an unredacted
* transcript (as two separate files).
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #redactionOutput}
* will return {@link RedactionOutput#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #redactionOutputAsString}.
*
*
* @return Specify if you want only a redacted transcript, or if you want a redacted and an unredacted
* transcript.
*
* When you choose redacted
Amazon Transcribe creates only a redacted transcript.
*
*
* When you choose redacted_and_unredacted
Amazon Transcribe creates a redacted and an
* unredacted transcript (as two separate files).
* @see RedactionOutput
*/
public final RedactionOutput redactionOutput() {
return RedactionOutput.fromValue(redactionOutput);
}
/**
*
* Specify if you want only a redacted transcript, or if you want a redacted and an unredacted transcript.
*
*
* When you choose redacted
Amazon Transcribe creates only a redacted transcript.
*
*
* When you choose redacted_and_unredacted
Amazon Transcribe creates a redacted and an unredacted
* transcript (as two separate files).
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #redactionOutput}
* will return {@link RedactionOutput#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #redactionOutputAsString}.
*
*
* @return Specify if you want only a redacted transcript, or if you want a redacted and an unredacted
* transcript.
*
* When you choose redacted
Amazon Transcribe creates only a redacted transcript.
*
*
* When you choose redacted_and_unredacted
Amazon Transcribe creates a redacted and an
* unredacted transcript (as two separate files).
* @see RedactionOutput
*/
public final String redactionOutputAsString() {
return redactionOutput;
}
/**
*
* Specify which types of personally identifiable information (PII) you want to redact in your transcript. You can
* include as many types as you'd like, or you can select ALL
.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasPiiEntityTypes} method.
*
*
* @return Specify which types of personally identifiable information (PII) you want to redact in your transcript.
* You can include as many types as you'd like, or you can select ALL
.
*/
public final List piiEntityTypes() {
return PiiEntityTypesCopier.copyStringToEnum(piiEntityTypes);
}
/**
* For responses, this returns true if the service returned a value for the PiiEntityTypes property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasPiiEntityTypes() {
return piiEntityTypes != null && !(piiEntityTypes instanceof SdkAutoConstructList);
}
/**
*
* Specify which types of personally identifiable information (PII) you want to redact in your transcript. You can
* include as many types as you'd like, or you can select ALL
.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasPiiEntityTypes} method.
*
*
* @return Specify which types of personally identifiable information (PII) you want to redact in your transcript.
* You can include as many types as you'd like, or you can select ALL
.
*/
public final List piiEntityTypesAsStrings() {
return piiEntityTypes;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(redactionTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(redactionOutputAsString());
hashCode = 31 * hashCode + Objects.hashCode(hasPiiEntityTypes() ? piiEntityTypesAsStrings() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ContentRedaction)) {
return false;
}
ContentRedaction other = (ContentRedaction) obj;
return Objects.equals(redactionTypeAsString(), other.redactionTypeAsString())
&& Objects.equals(redactionOutputAsString(), other.redactionOutputAsString())
&& hasPiiEntityTypes() == other.hasPiiEntityTypes()
&& Objects.equals(piiEntityTypesAsStrings(), other.piiEntityTypesAsStrings());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ContentRedaction").add("RedactionType", redactionTypeAsString())
.add("RedactionOutput", redactionOutputAsString())
.add("PiiEntityTypes", hasPiiEntityTypes() ? piiEntityTypesAsStrings() : null).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "RedactionType":
return Optional.ofNullable(clazz.cast(redactionTypeAsString()));
case "RedactionOutput":
return Optional.ofNullable(clazz.cast(redactionOutputAsString()));
case "PiiEntityTypes":
return Optional.ofNullable(clazz.cast(piiEntityTypesAsStrings()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function