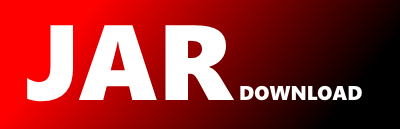
software.amazon.awssdk.services.transcribe.model.CreateVocabularyRequest Maven / Gradle / Ivy
Show all versions of transcribe Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.transcribe.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class CreateVocabularyRequest extends TranscribeRequest implements
ToCopyableBuilder {
private static final SdkField VOCABULARY_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("VocabularyName").getter(getter(CreateVocabularyRequest::vocabularyName))
.setter(setter(Builder::vocabularyName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("VocabularyName").build()).build();
private static final SdkField LANGUAGE_CODE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("LanguageCode").getter(getter(CreateVocabularyRequest::languageCodeAsString))
.setter(setter(Builder::languageCode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LanguageCode").build()).build();
private static final SdkField> PHRASES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Phrases")
.getter(getter(CreateVocabularyRequest::phrases))
.setter(setter(Builder::phrases))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Phrases").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField VOCABULARY_FILE_URI_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("VocabularyFileUri").getter(getter(CreateVocabularyRequest::vocabularyFileUri))
.setter(setter(Builder::vocabularyFileUri))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("VocabularyFileUri").build()).build();
private static final SdkField> TAGS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Tags")
.getter(getter(CreateVocabularyRequest::tags))
.setter(setter(Builder::tags))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Tags").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Tag::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField DATA_ACCESS_ROLE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("DataAccessRoleArn").getter(getter(CreateVocabularyRequest::dataAccessRoleArn))
.setter(setter(Builder::dataAccessRoleArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DataAccessRoleArn").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(VOCABULARY_NAME_FIELD,
LANGUAGE_CODE_FIELD, PHRASES_FIELD, VOCABULARY_FILE_URI_FIELD, TAGS_FIELD, DATA_ACCESS_ROLE_ARN_FIELD));
private final String vocabularyName;
private final String languageCode;
private final List phrases;
private final String vocabularyFileUri;
private final List tags;
private final String dataAccessRoleArn;
private CreateVocabularyRequest(BuilderImpl builder) {
super(builder);
this.vocabularyName = builder.vocabularyName;
this.languageCode = builder.languageCode;
this.phrases = builder.phrases;
this.vocabularyFileUri = builder.vocabularyFileUri;
this.tags = builder.tags;
this.dataAccessRoleArn = builder.dataAccessRoleArn;
}
/**
*
* A unique name, chosen by you, for your new custom vocabulary.
*
*
* This name is case sensitive, cannot contain spaces, and must be unique within an Amazon Web Services account. If
* you try to create a new custom vocabulary with the same name as an existing custom vocabulary, you get a
* ConflictException
error.
*
*
* @return A unique name, chosen by you, for your new custom vocabulary.
*
* This name is case sensitive, cannot contain spaces, and must be unique within an Amazon Web Services
* account. If you try to create a new custom vocabulary with the same name as an existing custom
* vocabulary, you get a ConflictException
error.
*/
public final String vocabularyName() {
return vocabularyName;
}
/**
*
* The language code that represents the language of the entries in your custom vocabulary. Each custom vocabulary
* must contain terms in only one language.
*
*
* A custom vocabulary can only be used to transcribe files in the same language as the custom vocabulary. For
* example, if you create a custom vocabulary using US English (en-US
), you can only apply this custom
* vocabulary to files that contain English audio.
*
*
* For a list of supported languages and their associated language codes, refer to the Supported languages table.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #languageCode} will
* return {@link LanguageCode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #languageCodeAsString}.
*
*
* @return The language code that represents the language of the entries in your custom vocabulary. Each custom
* vocabulary must contain terms in only one language.
*
* A custom vocabulary can only be used to transcribe files in the same language as the custom vocabulary.
* For example, if you create a custom vocabulary using US English (en-US
), you can only apply
* this custom vocabulary to files that contain English audio.
*
*
* For a list of supported languages and their associated language codes, refer to the Supported languages
* table.
* @see LanguageCode
*/
public final LanguageCode languageCode() {
return LanguageCode.fromValue(languageCode);
}
/**
*
* The language code that represents the language of the entries in your custom vocabulary. Each custom vocabulary
* must contain terms in only one language.
*
*
* A custom vocabulary can only be used to transcribe files in the same language as the custom vocabulary. For
* example, if you create a custom vocabulary using US English (en-US
), you can only apply this custom
* vocabulary to files that contain English audio.
*
*
* For a list of supported languages and their associated language codes, refer to the Supported languages table.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #languageCode} will
* return {@link LanguageCode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #languageCodeAsString}.
*
*
* @return The language code that represents the language of the entries in your custom vocabulary. Each custom
* vocabulary must contain terms in only one language.
*
* A custom vocabulary can only be used to transcribe files in the same language as the custom vocabulary.
* For example, if you create a custom vocabulary using US English (en-US
), you can only apply
* this custom vocabulary to files that contain English audio.
*
*
* For a list of supported languages and their associated language codes, refer to the Supported languages
* table.
* @see LanguageCode
*/
public final String languageCodeAsString() {
return languageCode;
}
/**
* For responses, this returns true if the service returned a value for the Phrases property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasPhrases() {
return phrases != null && !(phrases instanceof SdkAutoConstructList);
}
/**
*
* Use this parameter if you want to create your custom vocabulary by including all desired terms, as
* comma-separated values, within your request. The other option for creating your custom vocabulary is to save your
* entries in a text file and upload them to an Amazon S3 bucket, then specify the location of your file using the
* VocabularyFileUri
parameter.
*
*
* Note that if you include Phrases
in your request, you cannot use VocabularyFileUri
; you
* must choose one or the other.
*
*
* Each language has a character set that contains all allowed characters for that specific language. If you use
* unsupported characters, your custom vocabulary filter request fails. Refer to Character Sets for Custom Vocabularies
* to get the character set for your language.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasPhrases} method.
*
*
* @return Use this parameter if you want to create your custom vocabulary by including all desired terms, as
* comma-separated values, within your request. The other option for creating your custom vocabulary is to
* save your entries in a text file and upload them to an Amazon S3 bucket, then specify the location of
* your file using the VocabularyFileUri
parameter.
*
* Note that if you include Phrases
in your request, you cannot use
* VocabularyFileUri
; you must choose one or the other.
*
*
* Each language has a character set that contains all allowed characters for that specific language. If you
* use unsupported characters, your custom vocabulary filter request fails. Refer to Character Sets for Custom
* Vocabularies to get the character set for your language.
*/
public final List phrases() {
return phrases;
}
/**
*
* The Amazon S3 location of the text file that contains your custom vocabulary. The URI must be located in the same
* Amazon Web Services Region as the resource you're calling.
*
*
* Here's an example URI path: s3://DOC-EXAMPLE-BUCKET/my-vocab-file.txt
*
*
* Note that if you include VocabularyFileUri
in your request, you cannot use the Phrases
* flag; you must choose one or the other.
*
*
* @return The Amazon S3 location of the text file that contains your custom vocabulary. The URI must be located in
* the same Amazon Web Services Region as the resource you're calling.
*
* Here's an example URI path: s3://DOC-EXAMPLE-BUCKET/my-vocab-file.txt
*
*
* Note that if you include VocabularyFileUri
in your request, you cannot use the
* Phrases
flag; you must choose one or the other.
*/
public final String vocabularyFileUri() {
return vocabularyFileUri;
}
/**
* For responses, this returns true if the service returned a value for the Tags property. This DOES NOT check that
* the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is useful
* because the SDK will never return a null collection or map, but you may need to differentiate between the service
* returning nothing (or null) and the service returning an empty collection or map. For requests, this returns true
* if a value for the property was specified in the request builder, and false if a value was not specified.
*/
public final boolean hasTags() {
return tags != null && !(tags instanceof SdkAutoConstructList);
}
/**
*
* Adds one or more custom tags, each in the form of a key:value pair, to a new custom vocabulary at the time you
* create this new custom vocabulary.
*
*
* To learn more about using tags with Amazon Transcribe, refer to Tagging resources.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasTags} method.
*
*
* @return Adds one or more custom tags, each in the form of a key:value pair, to a new custom vocabulary at the
* time you create this new custom vocabulary.
*
* To learn more about using tags with Amazon Transcribe, refer to Tagging resources.
*/
public final List tags() {
return tags;
}
/**
*
* The Amazon Resource Name (ARN) of an IAM role that has permissions to access the Amazon S3 bucket that contains
* your input files (in this case, your custom vocabulary). If the role that you specify doesn’t have the
* appropriate permissions to access the specified Amazon S3 location, your request fails.
*
*
* IAM role ARNs have the format arn:partition:iam::account:role/role-name-with-path
. For example:
* arn:aws:iam::111122223333:role/Admin
.
*
*
* For more information, see IAM ARNs.
*
*
* @return The Amazon Resource Name (ARN) of an IAM role that has permissions to access the Amazon S3 bucket that
* contains your input files (in this case, your custom vocabulary). If the role that you specify doesn’t
* have the appropriate permissions to access the specified Amazon S3 location, your request fails.
*
* IAM role ARNs have the format arn:partition:iam::account:role/role-name-with-path
. For
* example: arn:aws:iam::111122223333:role/Admin
.
*
*
* For more information, see IAM
* ARNs.
*/
public final String dataAccessRoleArn() {
return dataAccessRoleArn;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(vocabularyName());
hashCode = 31 * hashCode + Objects.hashCode(languageCodeAsString());
hashCode = 31 * hashCode + Objects.hashCode(hasPhrases() ? phrases() : null);
hashCode = 31 * hashCode + Objects.hashCode(vocabularyFileUri());
hashCode = 31 * hashCode + Objects.hashCode(hasTags() ? tags() : null);
hashCode = 31 * hashCode + Objects.hashCode(dataAccessRoleArn());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CreateVocabularyRequest)) {
return false;
}
CreateVocabularyRequest other = (CreateVocabularyRequest) obj;
return Objects.equals(vocabularyName(), other.vocabularyName())
&& Objects.equals(languageCodeAsString(), other.languageCodeAsString()) && hasPhrases() == other.hasPhrases()
&& Objects.equals(phrases(), other.phrases()) && Objects.equals(vocabularyFileUri(), other.vocabularyFileUri())
&& hasTags() == other.hasTags() && Objects.equals(tags(), other.tags())
&& Objects.equals(dataAccessRoleArn(), other.dataAccessRoleArn());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("CreateVocabularyRequest").add("VocabularyName", vocabularyName())
.add("LanguageCode", languageCodeAsString()).add("Phrases", hasPhrases() ? phrases() : null)
.add("VocabularyFileUri", vocabularyFileUri()).add("Tags", hasTags() ? tags() : null)
.add("DataAccessRoleArn", dataAccessRoleArn()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "VocabularyName":
return Optional.ofNullable(clazz.cast(vocabularyName()));
case "LanguageCode":
return Optional.ofNullable(clazz.cast(languageCodeAsString()));
case "Phrases":
return Optional.ofNullable(clazz.cast(phrases()));
case "VocabularyFileUri":
return Optional.ofNullable(clazz.cast(vocabularyFileUri()));
case "Tags":
return Optional.ofNullable(clazz.cast(tags()));
case "DataAccessRoleArn":
return Optional.ofNullable(clazz.cast(dataAccessRoleArn()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
*
* This name is case sensitive, cannot contain spaces, and must be unique within an Amazon Web Services
* account. If you try to create a new custom vocabulary with the same name as an existing custom
* vocabulary, you get a ConflictException
error.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder vocabularyName(String vocabularyName);
/**
*
* The language code that represents the language of the entries in your custom vocabulary. Each custom
* vocabulary must contain terms in only one language.
*
*
* A custom vocabulary can only be used to transcribe files in the same language as the custom vocabulary. For
* example, if you create a custom vocabulary using US English (en-US
), you can only apply this
* custom vocabulary to files that contain English audio.
*
*
* For a list of supported languages and their associated language codes, refer to the Supported languages
* table.
*
*
* @param languageCode
* The language code that represents the language of the entries in your custom vocabulary. Each custom
* vocabulary must contain terms in only one language.
*
* A custom vocabulary can only be used to transcribe files in the same language as the custom
* vocabulary. For example, if you create a custom vocabulary using US English (en-US
), you
* can only apply this custom vocabulary to files that contain English audio.
*
*
* For a list of supported languages and their associated language codes, refer to the Supported
* languages table.
* @see LanguageCode
* @return Returns a reference to this object so that method calls can be chained together.
* @see LanguageCode
*/
Builder languageCode(String languageCode);
/**
*
* The language code that represents the language of the entries in your custom vocabulary. Each custom
* vocabulary must contain terms in only one language.
*
*
* A custom vocabulary can only be used to transcribe files in the same language as the custom vocabulary. For
* example, if you create a custom vocabulary using US English (en-US
), you can only apply this
* custom vocabulary to files that contain English audio.
*
*
* For a list of supported languages and their associated language codes, refer to the Supported languages
* table.
*
*
* @param languageCode
* The language code that represents the language of the entries in your custom vocabulary. Each custom
* vocabulary must contain terms in only one language.
*
* A custom vocabulary can only be used to transcribe files in the same language as the custom
* vocabulary. For example, if you create a custom vocabulary using US English (en-US
), you
* can only apply this custom vocabulary to files that contain English audio.
*
*
* For a list of supported languages and their associated language codes, refer to the Supported
* languages table.
* @see LanguageCode
* @return Returns a reference to this object so that method calls can be chained together.
* @see LanguageCode
*/
Builder languageCode(LanguageCode languageCode);
/**
*
* Use this parameter if you want to create your custom vocabulary by including all desired terms, as
* comma-separated values, within your request. The other option for creating your custom vocabulary is to save
* your entries in a text file and upload them to an Amazon S3 bucket, then specify the location of your file
* using the VocabularyFileUri
parameter.
*
*
* Note that if you include Phrases
in your request, you cannot use VocabularyFileUri
;
* you must choose one or the other.
*
*
* Each language has a character set that contains all allowed characters for that specific language. If you use
* unsupported characters, your custom vocabulary filter request fails. Refer to Character Sets for Custom
* Vocabularies to get the character set for your language.
*
*
* @param phrases
* Use this parameter if you want to create your custom vocabulary by including all desired terms, as
* comma-separated values, within your request. The other option for creating your custom vocabulary is
* to save your entries in a text file and upload them to an Amazon S3 bucket, then specify the location
* of your file using the VocabularyFileUri
parameter.
*
* Note that if you include Phrases
in your request, you cannot use
* VocabularyFileUri
; you must choose one or the other.
*
*
* Each language has a character set that contains all allowed characters for that specific language. If
* you use unsupported characters, your custom vocabulary filter request fails. Refer to Character Sets for Custom
* Vocabularies to get the character set for your language.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder phrases(Collection phrases);
/**
*
* Use this parameter if you want to create your custom vocabulary by including all desired terms, as
* comma-separated values, within your request. The other option for creating your custom vocabulary is to save
* your entries in a text file and upload them to an Amazon S3 bucket, then specify the location of your file
* using the VocabularyFileUri
parameter.
*
*
* Note that if you include Phrases
in your request, you cannot use VocabularyFileUri
;
* you must choose one or the other.
*
*
* Each language has a character set that contains all allowed characters for that specific language. If you use
* unsupported characters, your custom vocabulary filter request fails. Refer to Character Sets for Custom
* Vocabularies to get the character set for your language.
*
*
* @param phrases
* Use this parameter if you want to create your custom vocabulary by including all desired terms, as
* comma-separated values, within your request. The other option for creating your custom vocabulary is
* to save your entries in a text file and upload them to an Amazon S3 bucket, then specify the location
* of your file using the VocabularyFileUri
parameter.
*
* Note that if you include Phrases
in your request, you cannot use
* VocabularyFileUri
; you must choose one or the other.
*
*
* Each language has a character set that contains all allowed characters for that specific language. If
* you use unsupported characters, your custom vocabulary filter request fails. Refer to Character Sets for Custom
* Vocabularies to get the character set for your language.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder phrases(String... phrases);
/**
*
* The Amazon S3 location of the text file that contains your custom vocabulary. The URI must be located in the
* same Amazon Web Services Region as the resource you're calling.
*
*
* Here's an example URI path: s3://DOC-EXAMPLE-BUCKET/my-vocab-file.txt
*
*
* Note that if you include VocabularyFileUri
in your request, you cannot use the
* Phrases
flag; you must choose one or the other.
*
*
* @param vocabularyFileUri
* The Amazon S3 location of the text file that contains your custom vocabulary. The URI must be located
* in the same Amazon Web Services Region as the resource you're calling.
*
* Here's an example URI path: s3://DOC-EXAMPLE-BUCKET/my-vocab-file.txt
*
*
* Note that if you include VocabularyFileUri
in your request, you cannot use the
* Phrases
flag; you must choose one or the other.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder vocabularyFileUri(String vocabularyFileUri);
/**
*
* Adds one or more custom tags, each in the form of a key:value pair, to a new custom vocabulary at the time
* you create this new custom vocabulary.
*
*
* To learn more about using tags with Amazon Transcribe, refer to Tagging resources.
*
*
* @param tags
* Adds one or more custom tags, each in the form of a key:value pair, to a new custom vocabulary at the
* time you create this new custom vocabulary.
*
* To learn more about using tags with Amazon Transcribe, refer to Tagging resources.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder tags(Collection tags);
/**
*
* Adds one or more custom tags, each in the form of a key:value pair, to a new custom vocabulary at the time
* you create this new custom vocabulary.
*
*
* To learn more about using tags with Amazon Transcribe, refer to Tagging resources.
*
*
* @param tags
* Adds one or more custom tags, each in the form of a key:value pair, to a new custom vocabulary at the
* time you create this new custom vocabulary.
*
* To learn more about using tags with Amazon Transcribe, refer to Tagging resources.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder tags(Tag... tags);
/**
*
* Adds one or more custom tags, each in the form of a key:value pair, to a new custom vocabulary at the time
* you create this new custom vocabulary.
*
*
* To learn more about using tags with Amazon Transcribe, refer to Tagging resources.
*
* This is a convenience method that creates an instance of the
* {@link software.amazon.awssdk.services.transcribe.model.Tag.Builder} avoiding the need to create one manually
* via {@link software.amazon.awssdk.services.transcribe.model.Tag#builder()}.
*
*
* When the {@link Consumer} completes,
* {@link software.amazon.awssdk.services.transcribe.model.Tag.Builder#build()} is called immediately and its
* result is passed to {@link #tags(List)}.
*
* @param tags
* a consumer that will call methods on
* {@link software.amazon.awssdk.services.transcribe.model.Tag.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #tags(java.util.Collection)
*/
Builder tags(Consumer... tags);
/**
*
* The Amazon Resource Name (ARN) of an IAM role that has permissions to access the Amazon S3 bucket that
* contains your input files (in this case, your custom vocabulary). If the role that you specify doesn’t have
* the appropriate permissions to access the specified Amazon S3 location, your request fails.
*
*
* IAM role ARNs have the format arn:partition:iam::account:role/role-name-with-path
. For example:
* arn:aws:iam::111122223333:role/Admin
.
*
*
* For more information, see IAM
* ARNs.
*
*
* @param dataAccessRoleArn
* The Amazon Resource Name (ARN) of an IAM role that has permissions to access the Amazon S3 bucket that
* contains your input files (in this case, your custom vocabulary). If the role that you specify doesn’t
* have the appropriate permissions to access the specified Amazon S3 location, your request fails.
*
* IAM role ARNs have the format arn:partition:iam::account:role/role-name-with-path
. For
* example: arn:aws:iam::111122223333:role/Admin
.
*
*
* For more information, see IAM ARNs.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder dataAccessRoleArn(String dataAccessRoleArn);
@Override
Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration);
@Override
Builder overrideConfiguration(Consumer builderConsumer);
}
static final class BuilderImpl extends TranscribeRequest.BuilderImpl implements Builder {
private String vocabularyName;
private String languageCode;
private List phrases = DefaultSdkAutoConstructList.getInstance();
private String vocabularyFileUri;
private List tags = DefaultSdkAutoConstructList.getInstance();
private String dataAccessRoleArn;
private BuilderImpl() {
}
private BuilderImpl(CreateVocabularyRequest model) {
super(model);
vocabularyName(model.vocabularyName);
languageCode(model.languageCode);
phrases(model.phrases);
vocabularyFileUri(model.vocabularyFileUri);
tags(model.tags);
dataAccessRoleArn(model.dataAccessRoleArn);
}
public final String getVocabularyName() {
return vocabularyName;
}
public final void setVocabularyName(String vocabularyName) {
this.vocabularyName = vocabularyName;
}
@Override
public final Builder vocabularyName(String vocabularyName) {
this.vocabularyName = vocabularyName;
return this;
}
public final String getLanguageCode() {
return languageCode;
}
public final void setLanguageCode(String languageCode) {
this.languageCode = languageCode;
}
@Override
public final Builder languageCode(String languageCode) {
this.languageCode = languageCode;
return this;
}
@Override
public final Builder languageCode(LanguageCode languageCode) {
this.languageCode(languageCode == null ? null : languageCode.toString());
return this;
}
public final Collection getPhrases() {
if (phrases instanceof SdkAutoConstructList) {
return null;
}
return phrases;
}
public final void setPhrases(Collection phrases) {
this.phrases = PhrasesCopier.copy(phrases);
}
@Override
public final Builder phrases(Collection phrases) {
this.phrases = PhrasesCopier.copy(phrases);
return this;
}
@Override
@SafeVarargs
public final Builder phrases(String... phrases) {
phrases(Arrays.asList(phrases));
return this;
}
public final String getVocabularyFileUri() {
return vocabularyFileUri;
}
public final void setVocabularyFileUri(String vocabularyFileUri) {
this.vocabularyFileUri = vocabularyFileUri;
}
@Override
public final Builder vocabularyFileUri(String vocabularyFileUri) {
this.vocabularyFileUri = vocabularyFileUri;
return this;
}
public final List getTags() {
List result = TagListCopier.copyToBuilder(this.tags);
if (result instanceof SdkAutoConstructList) {
return null;
}
return result;
}
public final void setTags(Collection tags) {
this.tags = TagListCopier.copyFromBuilder(tags);
}
@Override
public final Builder tags(Collection tags) {
this.tags = TagListCopier.copy(tags);
return this;
}
@Override
@SafeVarargs
public final Builder tags(Tag... tags) {
tags(Arrays.asList(tags));
return this;
}
@Override
@SafeVarargs
public final Builder tags(Consumer... tags) {
tags(Stream.of(tags).map(c -> Tag.builder().applyMutation(c).build()).collect(Collectors.toList()));
return this;
}
public final String getDataAccessRoleArn() {
return dataAccessRoleArn;
}
public final void setDataAccessRoleArn(String dataAccessRoleArn) {
this.dataAccessRoleArn = dataAccessRoleArn;
}
@Override
public final Builder dataAccessRoleArn(String dataAccessRoleArn) {
this.dataAccessRoleArn = dataAccessRoleArn;
return this;
}
@Override
public Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration) {
super.overrideConfiguration(overrideConfiguration);
return this;
}
@Override
public Builder overrideConfiguration(Consumer builderConsumer) {
super.overrideConfiguration(builderConsumer);
return this;
}
@Override
public CreateVocabularyRequest build() {
return new CreateVocabularyRequest(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
}
}