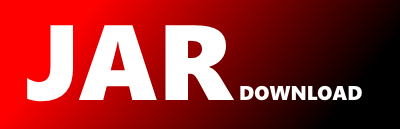
software.amazon.awssdk.services.transcribe.TranscribeAsyncClient Maven / Gradle / Ivy
Show all versions of transcribe Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.transcribe;
import java.util.concurrent.CompletableFuture;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkPublicApi;
import software.amazon.awssdk.annotations.ThreadSafe;
import software.amazon.awssdk.awscore.AwsClient;
import software.amazon.awssdk.services.transcribe.model.CreateCallAnalyticsCategoryRequest;
import software.amazon.awssdk.services.transcribe.model.CreateCallAnalyticsCategoryResponse;
import software.amazon.awssdk.services.transcribe.model.CreateLanguageModelRequest;
import software.amazon.awssdk.services.transcribe.model.CreateLanguageModelResponse;
import software.amazon.awssdk.services.transcribe.model.CreateMedicalVocabularyRequest;
import software.amazon.awssdk.services.transcribe.model.CreateMedicalVocabularyResponse;
import software.amazon.awssdk.services.transcribe.model.CreateVocabularyFilterRequest;
import software.amazon.awssdk.services.transcribe.model.CreateVocabularyFilterResponse;
import software.amazon.awssdk.services.transcribe.model.CreateVocabularyRequest;
import software.amazon.awssdk.services.transcribe.model.CreateVocabularyResponse;
import software.amazon.awssdk.services.transcribe.model.DeleteCallAnalyticsCategoryRequest;
import software.amazon.awssdk.services.transcribe.model.DeleteCallAnalyticsCategoryResponse;
import software.amazon.awssdk.services.transcribe.model.DeleteCallAnalyticsJobRequest;
import software.amazon.awssdk.services.transcribe.model.DeleteCallAnalyticsJobResponse;
import software.amazon.awssdk.services.transcribe.model.DeleteLanguageModelRequest;
import software.amazon.awssdk.services.transcribe.model.DeleteLanguageModelResponse;
import software.amazon.awssdk.services.transcribe.model.DeleteMedicalScribeJobRequest;
import software.amazon.awssdk.services.transcribe.model.DeleteMedicalScribeJobResponse;
import software.amazon.awssdk.services.transcribe.model.DeleteMedicalTranscriptionJobRequest;
import software.amazon.awssdk.services.transcribe.model.DeleteMedicalTranscriptionJobResponse;
import software.amazon.awssdk.services.transcribe.model.DeleteMedicalVocabularyRequest;
import software.amazon.awssdk.services.transcribe.model.DeleteMedicalVocabularyResponse;
import software.amazon.awssdk.services.transcribe.model.DeleteTranscriptionJobRequest;
import software.amazon.awssdk.services.transcribe.model.DeleteTranscriptionJobResponse;
import software.amazon.awssdk.services.transcribe.model.DeleteVocabularyFilterRequest;
import software.amazon.awssdk.services.transcribe.model.DeleteVocabularyFilterResponse;
import software.amazon.awssdk.services.transcribe.model.DeleteVocabularyRequest;
import software.amazon.awssdk.services.transcribe.model.DeleteVocabularyResponse;
import software.amazon.awssdk.services.transcribe.model.DescribeLanguageModelRequest;
import software.amazon.awssdk.services.transcribe.model.DescribeLanguageModelResponse;
import software.amazon.awssdk.services.transcribe.model.GetCallAnalyticsCategoryRequest;
import software.amazon.awssdk.services.transcribe.model.GetCallAnalyticsCategoryResponse;
import software.amazon.awssdk.services.transcribe.model.GetCallAnalyticsJobRequest;
import software.amazon.awssdk.services.transcribe.model.GetCallAnalyticsJobResponse;
import software.amazon.awssdk.services.transcribe.model.GetMedicalScribeJobRequest;
import software.amazon.awssdk.services.transcribe.model.GetMedicalScribeJobResponse;
import software.amazon.awssdk.services.transcribe.model.GetMedicalTranscriptionJobRequest;
import software.amazon.awssdk.services.transcribe.model.GetMedicalTranscriptionJobResponse;
import software.amazon.awssdk.services.transcribe.model.GetMedicalVocabularyRequest;
import software.amazon.awssdk.services.transcribe.model.GetMedicalVocabularyResponse;
import software.amazon.awssdk.services.transcribe.model.GetTranscriptionJobRequest;
import software.amazon.awssdk.services.transcribe.model.GetTranscriptionJobResponse;
import software.amazon.awssdk.services.transcribe.model.GetVocabularyFilterRequest;
import software.amazon.awssdk.services.transcribe.model.GetVocabularyFilterResponse;
import software.amazon.awssdk.services.transcribe.model.GetVocabularyRequest;
import software.amazon.awssdk.services.transcribe.model.GetVocabularyResponse;
import software.amazon.awssdk.services.transcribe.model.ListCallAnalyticsCategoriesRequest;
import software.amazon.awssdk.services.transcribe.model.ListCallAnalyticsCategoriesResponse;
import software.amazon.awssdk.services.transcribe.model.ListCallAnalyticsJobsRequest;
import software.amazon.awssdk.services.transcribe.model.ListCallAnalyticsJobsResponse;
import software.amazon.awssdk.services.transcribe.model.ListLanguageModelsRequest;
import software.amazon.awssdk.services.transcribe.model.ListLanguageModelsResponse;
import software.amazon.awssdk.services.transcribe.model.ListMedicalScribeJobsRequest;
import software.amazon.awssdk.services.transcribe.model.ListMedicalScribeJobsResponse;
import software.amazon.awssdk.services.transcribe.model.ListMedicalTranscriptionJobsRequest;
import software.amazon.awssdk.services.transcribe.model.ListMedicalTranscriptionJobsResponse;
import software.amazon.awssdk.services.transcribe.model.ListMedicalVocabulariesRequest;
import software.amazon.awssdk.services.transcribe.model.ListMedicalVocabulariesResponse;
import software.amazon.awssdk.services.transcribe.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.transcribe.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.transcribe.model.ListTranscriptionJobsRequest;
import software.amazon.awssdk.services.transcribe.model.ListTranscriptionJobsResponse;
import software.amazon.awssdk.services.transcribe.model.ListVocabulariesRequest;
import software.amazon.awssdk.services.transcribe.model.ListVocabulariesResponse;
import software.amazon.awssdk.services.transcribe.model.ListVocabularyFiltersRequest;
import software.amazon.awssdk.services.transcribe.model.ListVocabularyFiltersResponse;
import software.amazon.awssdk.services.transcribe.model.StartCallAnalyticsJobRequest;
import software.amazon.awssdk.services.transcribe.model.StartCallAnalyticsJobResponse;
import software.amazon.awssdk.services.transcribe.model.StartMedicalScribeJobRequest;
import software.amazon.awssdk.services.transcribe.model.StartMedicalScribeJobResponse;
import software.amazon.awssdk.services.transcribe.model.StartMedicalTranscriptionJobRequest;
import software.amazon.awssdk.services.transcribe.model.StartMedicalTranscriptionJobResponse;
import software.amazon.awssdk.services.transcribe.model.StartTranscriptionJobRequest;
import software.amazon.awssdk.services.transcribe.model.StartTranscriptionJobResponse;
import software.amazon.awssdk.services.transcribe.model.TagResourceRequest;
import software.amazon.awssdk.services.transcribe.model.TagResourceResponse;
import software.amazon.awssdk.services.transcribe.model.UntagResourceRequest;
import software.amazon.awssdk.services.transcribe.model.UntagResourceResponse;
import software.amazon.awssdk.services.transcribe.model.UpdateCallAnalyticsCategoryRequest;
import software.amazon.awssdk.services.transcribe.model.UpdateCallAnalyticsCategoryResponse;
import software.amazon.awssdk.services.transcribe.model.UpdateMedicalVocabularyRequest;
import software.amazon.awssdk.services.transcribe.model.UpdateMedicalVocabularyResponse;
import software.amazon.awssdk.services.transcribe.model.UpdateVocabularyFilterRequest;
import software.amazon.awssdk.services.transcribe.model.UpdateVocabularyFilterResponse;
import software.amazon.awssdk.services.transcribe.model.UpdateVocabularyRequest;
import software.amazon.awssdk.services.transcribe.model.UpdateVocabularyResponse;
import software.amazon.awssdk.services.transcribe.paginators.ListCallAnalyticsCategoriesPublisher;
import software.amazon.awssdk.services.transcribe.paginators.ListCallAnalyticsJobsPublisher;
import software.amazon.awssdk.services.transcribe.paginators.ListLanguageModelsPublisher;
import software.amazon.awssdk.services.transcribe.paginators.ListMedicalScribeJobsPublisher;
import software.amazon.awssdk.services.transcribe.paginators.ListMedicalTranscriptionJobsPublisher;
import software.amazon.awssdk.services.transcribe.paginators.ListMedicalVocabulariesPublisher;
import software.amazon.awssdk.services.transcribe.paginators.ListTranscriptionJobsPublisher;
import software.amazon.awssdk.services.transcribe.paginators.ListVocabulariesPublisher;
import software.amazon.awssdk.services.transcribe.paginators.ListVocabularyFiltersPublisher;
/**
* Service client for accessing Amazon Transcribe Service asynchronously. This can be created using the static
* {@link #builder()} method.
*
*
* Amazon Transcribe offers three main types of batch transcription: Standard, Medical, and Call
* Analytics.
*
*
* -
*
* Standard transcriptions are the most common option. Refer to for details.
*
*
* -
*
* Medical transcriptions are tailored to medical professionals and incorporate medical terms. A common use case
* for this service is transcribing doctor-patient dialogue into after-visit notes. Refer to for details.
*
*
* -
*
* Call Analytics transcriptions are designed for use with call center audio on two different channels; if you're
* looking for insight into customer service calls, use this option. Refer to for details.
*
*
*
*/
@Generated("software.amazon.awssdk:codegen")
@SdkPublicApi
@ThreadSafe
public interface TranscribeAsyncClient extends AwsClient {
String SERVICE_NAME = "transcribe";
/**
* Value for looking up the service's metadata from the
* {@link software.amazon.awssdk.regions.ServiceMetadataProvider}.
*/
String SERVICE_METADATA_ID = "transcribe";
/**
*
* Creates a new Call Analytics category.
*
*
* All categories are automatically applied to your Call Analytics transcriptions. Note that in order to apply
* categories to your transcriptions, you must create them before submitting your transcription request, as
* categories cannot be applied retroactively.
*
*
* When creating a new category, you can use the InputType
parameter to label the category as a
* POST_CALL
or a REAL_TIME
category. POST_CALL
categories can only be
* applied to post-call transcriptions and REAL_TIME
categories can only be applied to real-time
* transcriptions. If you do not include InputType
, your category is created as a
* POST_CALL
category by default.
*
*
* Call Analytics categories are composed of rules. For each category, you must create between 1 and 20 rules. Rules
* can include these parameters: , , , and .
*
*
* To update an existing category, see .
*
*
* To learn more about Call Analytics categories, see Creating categories for
* post-call transcriptions and Creating categories for
* real-time transcriptions.
*
*
* @param createCallAnalyticsCategoryRequest
* @return A Java Future containing the result of the CreateCallAnalyticsCategory operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - ConflictException A resource already exists with this name. Resource names must be unique within an
* Amazon Web Services account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.CreateCallAnalyticsCategory
* @see AWS API Documentation
*/
default CompletableFuture createCallAnalyticsCategory(
CreateCallAnalyticsCategoryRequest createCallAnalyticsCategoryRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a new Call Analytics category.
*
*
* All categories are automatically applied to your Call Analytics transcriptions. Note that in order to apply
* categories to your transcriptions, you must create them before submitting your transcription request, as
* categories cannot be applied retroactively.
*
*
* When creating a new category, you can use the InputType
parameter to label the category as a
* POST_CALL
or a REAL_TIME
category. POST_CALL
categories can only be
* applied to post-call transcriptions and REAL_TIME
categories can only be applied to real-time
* transcriptions. If you do not include InputType
, your category is created as a
* POST_CALL
category by default.
*
*
* Call Analytics categories are composed of rules. For each category, you must create between 1 and 20 rules. Rules
* can include these parameters: , , , and .
*
*
* To update an existing category, see .
*
*
* To learn more about Call Analytics categories, see Creating categories for
* post-call transcriptions and Creating categories for
* real-time transcriptions.
*
*
*
* This is a convenience which creates an instance of the {@link CreateCallAnalyticsCategoryRequest.Builder}
* avoiding the need to create one manually via {@link CreateCallAnalyticsCategoryRequest#builder()}
*
*
* @param createCallAnalyticsCategoryRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transcribe.model.CreateCallAnalyticsCategoryRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the CreateCallAnalyticsCategory operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - ConflictException A resource already exists with this name. Resource names must be unique within an
* Amazon Web Services account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.CreateCallAnalyticsCategory
* @see AWS API Documentation
*/
default CompletableFuture createCallAnalyticsCategory(
Consumer createCallAnalyticsCategoryRequest) {
return createCallAnalyticsCategory(CreateCallAnalyticsCategoryRequest.builder()
.applyMutation(createCallAnalyticsCategoryRequest).build());
}
/**
*
* Creates a new custom language model.
*
*
* When creating a new custom language model, you must specify:
*
*
* -
*
* If you want a Wideband (audio sample rates over 16,000 Hz) or Narrowband (audio sample rates under 16,000 Hz)
* base model
*
*
* -
*
* The location of your training and tuning files (this must be an Amazon S3 URI)
*
*
* -
*
* The language of your model
*
*
* -
*
* A unique name for your model
*
*
*
*
* @param createLanguageModelRequest
* @return A Java Future containing the result of the CreateLanguageModel operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - ConflictException A resource already exists with this name. Resource names must be unique within an
* Amazon Web Services account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.CreateLanguageModel
* @see AWS API Documentation
*/
default CompletableFuture createLanguageModel(
CreateLanguageModelRequest createLanguageModelRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a new custom language model.
*
*
* When creating a new custom language model, you must specify:
*
*
* -
*
* If you want a Wideband (audio sample rates over 16,000 Hz) or Narrowband (audio sample rates under 16,000 Hz)
* base model
*
*
* -
*
* The location of your training and tuning files (this must be an Amazon S3 URI)
*
*
* -
*
* The language of your model
*
*
* -
*
* A unique name for your model
*
*
*
*
*
* This is a convenience which creates an instance of the {@link CreateLanguageModelRequest.Builder} avoiding the
* need to create one manually via {@link CreateLanguageModelRequest#builder()}
*
*
* @param createLanguageModelRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transcribe.model.CreateLanguageModelRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the CreateLanguageModel operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - ConflictException A resource already exists with this name. Resource names must be unique within an
* Amazon Web Services account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.CreateLanguageModel
* @see AWS API Documentation
*/
default CompletableFuture createLanguageModel(
Consumer createLanguageModelRequest) {
return createLanguageModel(CreateLanguageModelRequest.builder().applyMutation(createLanguageModelRequest).build());
}
/**
*
* Creates a new custom medical vocabulary.
*
*
* Before creating a new custom medical vocabulary, you must first upload a text file that contains your vocabulary
* table into an Amazon S3 bucket. Note that this differs from , where you can include a list of terms within your
* request using the Phrases
flag; CreateMedicalVocabulary
does not support the
* Phrases
flag and only accepts vocabularies in table format.
*
*
* Each language has a character set that contains all allowed characters for that specific language. If you use
* unsupported characters, your custom vocabulary request fails. Refer to Character Sets for Custom Vocabularies
* to get the character set for your language.
*
*
* For more information, see Custom vocabularies.
*
*
* @param createMedicalVocabularyRequest
* @return A Java Future containing the result of the CreateMedicalVocabulary operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - ConflictException A resource already exists with this name. Resource names must be unique within an
* Amazon Web Services account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.CreateMedicalVocabulary
* @see AWS API Documentation
*/
default CompletableFuture createMedicalVocabulary(
CreateMedicalVocabularyRequest createMedicalVocabularyRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a new custom medical vocabulary.
*
*
* Before creating a new custom medical vocabulary, you must first upload a text file that contains your vocabulary
* table into an Amazon S3 bucket. Note that this differs from , where you can include a list of terms within your
* request using the Phrases
flag; CreateMedicalVocabulary
does not support the
* Phrases
flag and only accepts vocabularies in table format.
*
*
* Each language has a character set that contains all allowed characters for that specific language. If you use
* unsupported characters, your custom vocabulary request fails. Refer to Character Sets for Custom Vocabularies
* to get the character set for your language.
*
*
* For more information, see Custom vocabularies.
*
*
*
* This is a convenience which creates an instance of the {@link CreateMedicalVocabularyRequest.Builder} avoiding
* the need to create one manually via {@link CreateMedicalVocabularyRequest#builder()}
*
*
* @param createMedicalVocabularyRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transcribe.model.CreateMedicalVocabularyRequest.Builder} to create
* a request.
* @return A Java Future containing the result of the CreateMedicalVocabulary operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - ConflictException A resource already exists with this name. Resource names must be unique within an
* Amazon Web Services account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.CreateMedicalVocabulary
* @see AWS API Documentation
*/
default CompletableFuture createMedicalVocabulary(
Consumer createMedicalVocabularyRequest) {
return createMedicalVocabulary(CreateMedicalVocabularyRequest.builder().applyMutation(createMedicalVocabularyRequest)
.build());
}
/**
*
* Creates a new custom vocabulary.
*
*
* When creating a new custom vocabulary, you can either upload a text file that contains your new entries, phrases,
* and terms into an Amazon S3 bucket and include the URI in your request. Or you can include a list of terms
* directly in your request using the Phrases
flag.
*
*
* Each language has a character set that contains all allowed characters for that specific language. If you use
* unsupported characters, your custom vocabulary request fails. Refer to Character Sets for Custom Vocabularies
* to get the character set for your language.
*
*
* For more information, see Custom vocabularies.
*
*
* @param createVocabularyRequest
* @return A Java Future containing the result of the CreateVocabulary operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - ConflictException A resource already exists with this name. Resource names must be unique within an
* Amazon Web Services account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.CreateVocabulary
* @see AWS
* API Documentation
*/
default CompletableFuture createVocabulary(CreateVocabularyRequest createVocabularyRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a new custom vocabulary.
*
*
* When creating a new custom vocabulary, you can either upload a text file that contains your new entries, phrases,
* and terms into an Amazon S3 bucket and include the URI in your request. Or you can include a list of terms
* directly in your request using the Phrases
flag.
*
*
* Each language has a character set that contains all allowed characters for that specific language. If you use
* unsupported characters, your custom vocabulary request fails. Refer to Character Sets for Custom Vocabularies
* to get the character set for your language.
*
*
* For more information, see Custom vocabularies.
*
*
*
* This is a convenience which creates an instance of the {@link CreateVocabularyRequest.Builder} avoiding the need
* to create one manually via {@link CreateVocabularyRequest#builder()}
*
*
* @param createVocabularyRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transcribe.model.CreateVocabularyRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the CreateVocabulary operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - ConflictException A resource already exists with this name. Resource names must be unique within an
* Amazon Web Services account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.CreateVocabulary
* @see AWS
* API Documentation
*/
default CompletableFuture createVocabulary(
Consumer createVocabularyRequest) {
return createVocabulary(CreateVocabularyRequest.builder().applyMutation(createVocabularyRequest).build());
}
/**
*
* Creates a new custom vocabulary filter.
*
*
* You can use custom vocabulary filters to mask, delete, or flag specific words from your transcript. Custom
* vocabulary filters are commonly used to mask profanity in transcripts.
*
*
* Each language has a character set that contains all allowed characters for that specific language. If you use
* unsupported characters, your custom vocabulary filter request fails. Refer to Character Sets for Custom Vocabularies
* to get the character set for your language.
*
*
* For more information, see Vocabulary filtering.
*
*
* @param createVocabularyFilterRequest
* @return A Java Future containing the result of the CreateVocabularyFilter operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - ConflictException A resource already exists with this name. Resource names must be unique within an
* Amazon Web Services account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.CreateVocabularyFilter
* @see AWS API Documentation
*/
default CompletableFuture createVocabularyFilter(
CreateVocabularyFilterRequest createVocabularyFilterRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a new custom vocabulary filter.
*
*
* You can use custom vocabulary filters to mask, delete, or flag specific words from your transcript. Custom
* vocabulary filters are commonly used to mask profanity in transcripts.
*
*
* Each language has a character set that contains all allowed characters for that specific language. If you use
* unsupported characters, your custom vocabulary filter request fails. Refer to Character Sets for Custom Vocabularies
* to get the character set for your language.
*
*
* For more information, see Vocabulary filtering.
*
*
*
* This is a convenience which creates an instance of the {@link CreateVocabularyFilterRequest.Builder} avoiding the
* need to create one manually via {@link CreateVocabularyFilterRequest#builder()}
*
*
* @param createVocabularyFilterRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transcribe.model.CreateVocabularyFilterRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the CreateVocabularyFilter operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - ConflictException A resource already exists with this name. Resource names must be unique within an
* Amazon Web Services account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.CreateVocabularyFilter
* @see AWS API Documentation
*/
default CompletableFuture createVocabularyFilter(
Consumer createVocabularyFilterRequest) {
return createVocabularyFilter(CreateVocabularyFilterRequest.builder().applyMutation(createVocabularyFilterRequest)
.build());
}
/**
*
* Deletes a Call Analytics category. To use this operation, specify the name of the category you want to delete
* using CategoryName
. Category names are case sensitive.
*
*
* @param deleteCallAnalyticsCategoryRequest
* @return A Java Future containing the result of the DeleteCallAnalyticsCategory operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException We can't find the requested resource. Check that the specified name is correct and
* try your request again.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.DeleteCallAnalyticsCategory
* @see AWS API Documentation
*/
default CompletableFuture deleteCallAnalyticsCategory(
DeleteCallAnalyticsCategoryRequest deleteCallAnalyticsCategoryRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a Call Analytics category. To use this operation, specify the name of the category you want to delete
* using CategoryName
. Category names are case sensitive.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteCallAnalyticsCategoryRequest.Builder}
* avoiding the need to create one manually via {@link DeleteCallAnalyticsCategoryRequest#builder()}
*
*
* @param deleteCallAnalyticsCategoryRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transcribe.model.DeleteCallAnalyticsCategoryRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the DeleteCallAnalyticsCategory operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException We can't find the requested resource. Check that the specified name is correct and
* try your request again.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.DeleteCallAnalyticsCategory
* @see AWS API Documentation
*/
default CompletableFuture deleteCallAnalyticsCategory(
Consumer deleteCallAnalyticsCategoryRequest) {
return deleteCallAnalyticsCategory(DeleteCallAnalyticsCategoryRequest.builder()
.applyMutation(deleteCallAnalyticsCategoryRequest).build());
}
/**
*
* Deletes a Call Analytics job. To use this operation, specify the name of the job you want to delete using
* CallAnalyticsJobName
. Job names are case sensitive.
*
*
* @param deleteCallAnalyticsJobRequest
* @return A Java Future containing the result of the DeleteCallAnalyticsJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.DeleteCallAnalyticsJob
* @see AWS API Documentation
*/
default CompletableFuture deleteCallAnalyticsJob(
DeleteCallAnalyticsJobRequest deleteCallAnalyticsJobRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a Call Analytics job. To use this operation, specify the name of the job you want to delete using
* CallAnalyticsJobName
. Job names are case sensitive.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteCallAnalyticsJobRequest.Builder} avoiding the
* need to create one manually via {@link DeleteCallAnalyticsJobRequest#builder()}
*
*
* @param deleteCallAnalyticsJobRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transcribe.model.DeleteCallAnalyticsJobRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DeleteCallAnalyticsJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.DeleteCallAnalyticsJob
* @see AWS API Documentation
*/
default CompletableFuture deleteCallAnalyticsJob(
Consumer deleteCallAnalyticsJobRequest) {
return deleteCallAnalyticsJob(DeleteCallAnalyticsJobRequest.builder().applyMutation(deleteCallAnalyticsJobRequest)
.build());
}
/**
*
* Deletes a custom language model. To use this operation, specify the name of the language model you want to delete
* using ModelName
. custom language model names are case sensitive.
*
*
* @param deleteLanguageModelRequest
* @return A Java Future containing the result of the DeleteLanguageModel operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.DeleteLanguageModel
* @see AWS API Documentation
*/
default CompletableFuture deleteLanguageModel(
DeleteLanguageModelRequest deleteLanguageModelRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a custom language model. To use this operation, specify the name of the language model you want to delete
* using ModelName
. custom language model names are case sensitive.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteLanguageModelRequest.Builder} avoiding the
* need to create one manually via {@link DeleteLanguageModelRequest#builder()}
*
*
* @param deleteLanguageModelRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transcribe.model.DeleteLanguageModelRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DeleteLanguageModel operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.DeleteLanguageModel
* @see AWS API Documentation
*/
default CompletableFuture deleteLanguageModel(
Consumer deleteLanguageModelRequest) {
return deleteLanguageModel(DeleteLanguageModelRequest.builder().applyMutation(deleteLanguageModelRequest).build());
}
/**
*
* Deletes a Medical Scribe job. To use this operation, specify the name of the job you want to delete using
* MedicalScribeJobName
. Job names are case sensitive.
*
*
* @param deleteMedicalScribeJobRequest
* @return A Java Future containing the result of the DeleteMedicalScribeJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.DeleteMedicalScribeJob
* @see AWS API Documentation
*/
default CompletableFuture deleteMedicalScribeJob(
DeleteMedicalScribeJobRequest deleteMedicalScribeJobRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a Medical Scribe job. To use this operation, specify the name of the job you want to delete using
* MedicalScribeJobName
. Job names are case sensitive.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteMedicalScribeJobRequest.Builder} avoiding the
* need to create one manually via {@link DeleteMedicalScribeJobRequest#builder()}
*
*
* @param deleteMedicalScribeJobRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transcribe.model.DeleteMedicalScribeJobRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DeleteMedicalScribeJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.DeleteMedicalScribeJob
* @see AWS API Documentation
*/
default CompletableFuture deleteMedicalScribeJob(
Consumer deleteMedicalScribeJobRequest) {
return deleteMedicalScribeJob(DeleteMedicalScribeJobRequest.builder().applyMutation(deleteMedicalScribeJobRequest)
.build());
}
/**
*
* Deletes a medical transcription job. To use this operation, specify the name of the job you want to delete using
* MedicalTranscriptionJobName
. Job names are case sensitive.
*
*
* @param deleteMedicalTranscriptionJobRequest
* @return A Java Future containing the result of the DeleteMedicalTranscriptionJob operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.DeleteMedicalTranscriptionJob
* @see AWS API Documentation
*/
default CompletableFuture deleteMedicalTranscriptionJob(
DeleteMedicalTranscriptionJobRequest deleteMedicalTranscriptionJobRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a medical transcription job. To use this operation, specify the name of the job you want to delete using
* MedicalTranscriptionJobName
. Job names are case sensitive.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteMedicalTranscriptionJobRequest.Builder}
* avoiding the need to create one manually via {@link DeleteMedicalTranscriptionJobRequest#builder()}
*
*
* @param deleteMedicalTranscriptionJobRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transcribe.model.DeleteMedicalTranscriptionJobRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the DeleteMedicalTranscriptionJob operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.DeleteMedicalTranscriptionJob
* @see AWS API Documentation
*/
default CompletableFuture deleteMedicalTranscriptionJob(
Consumer deleteMedicalTranscriptionJobRequest) {
return deleteMedicalTranscriptionJob(DeleteMedicalTranscriptionJobRequest.builder()
.applyMutation(deleteMedicalTranscriptionJobRequest).build());
}
/**
*
* Deletes a custom medical vocabulary. To use this operation, specify the name of the custom vocabulary you want to
* delete using VocabularyName
. Custom vocabulary names are case sensitive.
*
*
* @param deleteMedicalVocabularyRequest
* @return A Java Future containing the result of the DeleteMedicalVocabulary operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException We can't find the requested resource. Check that the specified name is correct and
* try your request again.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.DeleteMedicalVocabulary
* @see AWS API Documentation
*/
default CompletableFuture deleteMedicalVocabulary(
DeleteMedicalVocabularyRequest deleteMedicalVocabularyRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a custom medical vocabulary. To use this operation, specify the name of the custom vocabulary you want to
* delete using VocabularyName
. Custom vocabulary names are case sensitive.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteMedicalVocabularyRequest.Builder} avoiding
* the need to create one manually via {@link DeleteMedicalVocabularyRequest#builder()}
*
*
* @param deleteMedicalVocabularyRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transcribe.model.DeleteMedicalVocabularyRequest.Builder} to create
* a request.
* @return A Java Future containing the result of the DeleteMedicalVocabulary operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException We can't find the requested resource. Check that the specified name is correct and
* try your request again.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.DeleteMedicalVocabulary
* @see AWS API Documentation
*/
default CompletableFuture deleteMedicalVocabulary(
Consumer deleteMedicalVocabularyRequest) {
return deleteMedicalVocabulary(DeleteMedicalVocabularyRequest.builder().applyMutation(deleteMedicalVocabularyRequest)
.build());
}
/**
*
* Deletes a transcription job. To use this operation, specify the name of the job you want to delete using
* TranscriptionJobName
. Job names are case sensitive.
*
*
* @param deleteTranscriptionJobRequest
* @return A Java Future containing the result of the DeleteTranscriptionJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.DeleteTranscriptionJob
* @see AWS API Documentation
*/
default CompletableFuture deleteTranscriptionJob(
DeleteTranscriptionJobRequest deleteTranscriptionJobRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a transcription job. To use this operation, specify the name of the job you want to delete using
* TranscriptionJobName
. Job names are case sensitive.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteTranscriptionJobRequest.Builder} avoiding the
* need to create one manually via {@link DeleteTranscriptionJobRequest#builder()}
*
*
* @param deleteTranscriptionJobRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transcribe.model.DeleteTranscriptionJobRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DeleteTranscriptionJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.DeleteTranscriptionJob
* @see AWS API Documentation
*/
default CompletableFuture deleteTranscriptionJob(
Consumer deleteTranscriptionJobRequest) {
return deleteTranscriptionJob(DeleteTranscriptionJobRequest.builder().applyMutation(deleteTranscriptionJobRequest)
.build());
}
/**
*
* Deletes a custom vocabulary. To use this operation, specify the name of the custom vocabulary you want to delete
* using VocabularyName
. Custom vocabulary names are case sensitive.
*
*
* @param deleteVocabularyRequest
* @return A Java Future containing the result of the DeleteVocabulary operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException We can't find the requested resource. Check that the specified name is correct and
* try your request again.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.DeleteVocabulary
* @see AWS
* API Documentation
*/
default CompletableFuture deleteVocabulary(DeleteVocabularyRequest deleteVocabularyRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a custom vocabulary. To use this operation, specify the name of the custom vocabulary you want to delete
* using VocabularyName
. Custom vocabulary names are case sensitive.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteVocabularyRequest.Builder} avoiding the need
* to create one manually via {@link DeleteVocabularyRequest#builder()}
*
*
* @param deleteVocabularyRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transcribe.model.DeleteVocabularyRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DeleteVocabulary operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException We can't find the requested resource. Check that the specified name is correct and
* try your request again.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.DeleteVocabulary
* @see AWS
* API Documentation
*/
default CompletableFuture deleteVocabulary(
Consumer deleteVocabularyRequest) {
return deleteVocabulary(DeleteVocabularyRequest.builder().applyMutation(deleteVocabularyRequest).build());
}
/**
*
* Deletes a custom vocabulary filter. To use this operation, specify the name of the custom vocabulary filter you
* want to delete using VocabularyFilterName
. Custom vocabulary filter names are case sensitive.
*
*
* @param deleteVocabularyFilterRequest
* @return A Java Future containing the result of the DeleteVocabularyFilter operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException We can't find the requested resource. Check that the specified name is correct and
* try your request again.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.DeleteVocabularyFilter
* @see AWS API Documentation
*/
default CompletableFuture deleteVocabularyFilter(
DeleteVocabularyFilterRequest deleteVocabularyFilterRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a custom vocabulary filter. To use this operation, specify the name of the custom vocabulary filter you
* want to delete using VocabularyFilterName
. Custom vocabulary filter names are case sensitive.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteVocabularyFilterRequest.Builder} avoiding the
* need to create one manually via {@link DeleteVocabularyFilterRequest#builder()}
*
*
* @param deleteVocabularyFilterRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transcribe.model.DeleteVocabularyFilterRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DeleteVocabularyFilter operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException We can't find the requested resource. Check that the specified name is correct and
* try your request again.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.DeleteVocabularyFilter
* @see AWS API Documentation
*/
default CompletableFuture deleteVocabularyFilter(
Consumer deleteVocabularyFilterRequest) {
return deleteVocabularyFilter(DeleteVocabularyFilterRequest.builder().applyMutation(deleteVocabularyFilterRequest)
.build());
}
/**
*
* Provides information about the specified custom language model.
*
*
* This operation also shows if the base language model that you used to create your custom language model has been
* updated. If Amazon Transcribe has updated the base model, you can create a new custom language model using the
* updated base model.
*
*
* If you tried to create a new custom language model and the request wasn't successful, you can use
* DescribeLanguageModel
to help identify the reason for this failure.
*
*
* @param describeLanguageModelRequest
* @return A Java Future containing the result of the DescribeLanguageModel operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - NotFoundException We can't find the requested resource. Check that the specified name is correct and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.DescribeLanguageModel
* @see AWS API Documentation
*/
default CompletableFuture describeLanguageModel(
DescribeLanguageModelRequest describeLanguageModelRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Provides information about the specified custom language model.
*
*
* This operation also shows if the base language model that you used to create your custom language model has been
* updated. If Amazon Transcribe has updated the base model, you can create a new custom language model using the
* updated base model.
*
*
* If you tried to create a new custom language model and the request wasn't successful, you can use
* DescribeLanguageModel
to help identify the reason for this failure.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeLanguageModelRequest.Builder} avoiding the
* need to create one manually via {@link DescribeLanguageModelRequest#builder()}
*
*
* @param describeLanguageModelRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transcribe.model.DescribeLanguageModelRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DescribeLanguageModel operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - NotFoundException We can't find the requested resource. Check that the specified name is correct and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.DescribeLanguageModel
* @see AWS API Documentation
*/
default CompletableFuture describeLanguageModel(
Consumer describeLanguageModelRequest) {
return describeLanguageModel(DescribeLanguageModelRequest.builder().applyMutation(describeLanguageModelRequest).build());
}
/**
*
* Provides information about the specified Call Analytics category.
*
*
* To get a list of your Call Analytics categories, use the operation.
*
*
* @param getCallAnalyticsCategoryRequest
* @return A Java Future containing the result of the GetCallAnalyticsCategory operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException We can't find the requested resource. Check that the specified name is correct and
* try your request again.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.GetCallAnalyticsCategory
* @see AWS API Documentation
*/
default CompletableFuture getCallAnalyticsCategory(
GetCallAnalyticsCategoryRequest getCallAnalyticsCategoryRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Provides information about the specified Call Analytics category.
*
*
* To get a list of your Call Analytics categories, use the operation.
*
*
*
* This is a convenience which creates an instance of the {@link GetCallAnalyticsCategoryRequest.Builder} avoiding
* the need to create one manually via {@link GetCallAnalyticsCategoryRequest#builder()}
*
*
* @param getCallAnalyticsCategoryRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transcribe.model.GetCallAnalyticsCategoryRequest.Builder} to create
* a request.
* @return A Java Future containing the result of the GetCallAnalyticsCategory operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException We can't find the requested resource. Check that the specified name is correct and
* try your request again.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.GetCallAnalyticsCategory
* @see AWS API Documentation
*/
default CompletableFuture getCallAnalyticsCategory(
Consumer getCallAnalyticsCategoryRequest) {
return getCallAnalyticsCategory(GetCallAnalyticsCategoryRequest.builder().applyMutation(getCallAnalyticsCategoryRequest)
.build());
}
/**
*
* Provides information about the specified Call Analytics job.
*
*
* To view the job's status, refer to CallAnalyticsJobStatus
. If the status is COMPLETED
,
* the job is finished. You can find your completed transcript at the URI specified in
* TranscriptFileUri
. If the status is FAILED
, FailureReason
provides details
* on why your transcription job failed.
*
*
* If you enabled personally identifiable information (PII) redaction, the redacted transcript appears at the
* location specified in RedactedTranscriptFileUri
.
*
*
* If you chose to redact the audio in your media file, you can find your redacted media file at the location
* specified in RedactedMediaFileUri
.
*
*
* To get a list of your Call Analytics jobs, use the operation.
*
*
* @param getCallAnalyticsJobRequest
* @return A Java Future containing the result of the GetCallAnalyticsJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - NotFoundException We can't find the requested resource. Check that the specified name is correct and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.GetCallAnalyticsJob
* @see AWS API Documentation
*/
default CompletableFuture getCallAnalyticsJob(
GetCallAnalyticsJobRequest getCallAnalyticsJobRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Provides information about the specified Call Analytics job.
*
*
* To view the job's status, refer to CallAnalyticsJobStatus
. If the status is COMPLETED
,
* the job is finished. You can find your completed transcript at the URI specified in
* TranscriptFileUri
. If the status is FAILED
, FailureReason
provides details
* on why your transcription job failed.
*
*
* If you enabled personally identifiable information (PII) redaction, the redacted transcript appears at the
* location specified in RedactedTranscriptFileUri
.
*
*
* If you chose to redact the audio in your media file, you can find your redacted media file at the location
* specified in RedactedMediaFileUri
.
*
*
* To get a list of your Call Analytics jobs, use the operation.
*
*
*
* This is a convenience which creates an instance of the {@link GetCallAnalyticsJobRequest.Builder} avoiding the
* need to create one manually via {@link GetCallAnalyticsJobRequest#builder()}
*
*
* @param getCallAnalyticsJobRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transcribe.model.GetCallAnalyticsJobRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetCallAnalyticsJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - NotFoundException We can't find the requested resource. Check that the specified name is correct and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.GetCallAnalyticsJob
* @see AWS API Documentation
*/
default CompletableFuture getCallAnalyticsJob(
Consumer getCallAnalyticsJobRequest) {
return getCallAnalyticsJob(GetCallAnalyticsJobRequest.builder().applyMutation(getCallAnalyticsJobRequest).build());
}
/**
*
* Provides information about the specified Medical Scribe job.
*
*
* To view the status of the specified medical transcription job, check the MedicalScribeJobStatus
* field. If the status is COMPLETED
, the job is finished. You can find the results at the location
* specified in MedicalScribeOutput
. If the status is FAILED
, FailureReason
* provides details on why your Medical Scribe job failed.
*
*
* To get a list of your Medical Scribe jobs, use the operation.
*
*
* @param getMedicalScribeJobRequest
* @return A Java Future containing the result of the GetMedicalScribeJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - NotFoundException We can't find the requested resource. Check that the specified name is correct and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.GetMedicalScribeJob
* @see AWS API Documentation
*/
default CompletableFuture getMedicalScribeJob(
GetMedicalScribeJobRequest getMedicalScribeJobRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Provides information about the specified Medical Scribe job.
*
*
* To view the status of the specified medical transcription job, check the MedicalScribeJobStatus
* field. If the status is COMPLETED
, the job is finished. You can find the results at the location
* specified in MedicalScribeOutput
. If the status is FAILED
, FailureReason
* provides details on why your Medical Scribe job failed.
*
*
* To get a list of your Medical Scribe jobs, use the operation.
*
*
*
* This is a convenience which creates an instance of the {@link GetMedicalScribeJobRequest.Builder} avoiding the
* need to create one manually via {@link GetMedicalScribeJobRequest#builder()}
*
*
* @param getMedicalScribeJobRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transcribe.model.GetMedicalScribeJobRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetMedicalScribeJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - NotFoundException We can't find the requested resource. Check that the specified name is correct and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.GetMedicalScribeJob
* @see AWS API Documentation
*/
default CompletableFuture getMedicalScribeJob(
Consumer getMedicalScribeJobRequest) {
return getMedicalScribeJob(GetMedicalScribeJobRequest.builder().applyMutation(getMedicalScribeJobRequest).build());
}
/**
*
* Provides information about the specified medical transcription job.
*
*
* To view the status of the specified medical transcription job, check the TranscriptionJobStatus
* field. If the status is COMPLETED
, the job is finished. You can find the results at the location
* specified in TranscriptFileUri
. If the status is FAILED
, FailureReason
* provides details on why your transcription job failed.
*
*
* To get a list of your medical transcription jobs, use the operation.
*
*
* @param getMedicalTranscriptionJobRequest
* @return A Java Future containing the result of the GetMedicalTranscriptionJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - NotFoundException We can't find the requested resource. Check that the specified name is correct and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.GetMedicalTranscriptionJob
* @see AWS API Documentation
*/
default CompletableFuture getMedicalTranscriptionJob(
GetMedicalTranscriptionJobRequest getMedicalTranscriptionJobRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Provides information about the specified medical transcription job.
*
*
* To view the status of the specified medical transcription job, check the TranscriptionJobStatus
* field. If the status is COMPLETED
, the job is finished. You can find the results at the location
* specified in TranscriptFileUri
. If the status is FAILED
, FailureReason
* provides details on why your transcription job failed.
*
*
* To get a list of your medical transcription jobs, use the operation.
*
*
*
* This is a convenience which creates an instance of the {@link GetMedicalTranscriptionJobRequest.Builder} avoiding
* the need to create one manually via {@link GetMedicalTranscriptionJobRequest#builder()}
*
*
* @param getMedicalTranscriptionJobRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transcribe.model.GetMedicalTranscriptionJobRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the GetMedicalTranscriptionJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - NotFoundException We can't find the requested resource. Check that the specified name is correct and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.GetMedicalTranscriptionJob
* @see AWS API Documentation
*/
default CompletableFuture getMedicalTranscriptionJob(
Consumer getMedicalTranscriptionJobRequest) {
return getMedicalTranscriptionJob(GetMedicalTranscriptionJobRequest.builder()
.applyMutation(getMedicalTranscriptionJobRequest).build());
}
/**
*
* Provides information about the specified custom medical vocabulary.
*
*
* To view the status of the specified custom medical vocabulary, check the VocabularyState
field. If
* the status is READY
, your custom vocabulary is available to use. If the status is
* FAILED
, FailureReason
provides details on why your vocabulary failed.
*
*
* To get a list of your custom medical vocabularies, use the operation.
*
*
* @param getMedicalVocabularyRequest
* @return A Java Future containing the result of the GetMedicalVocabulary operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException We can't find the requested resource. Check that the specified name is correct and
* try your request again.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.GetMedicalVocabulary
* @see AWS API Documentation
*/
default CompletableFuture getMedicalVocabulary(
GetMedicalVocabularyRequest getMedicalVocabularyRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Provides information about the specified custom medical vocabulary.
*
*
* To view the status of the specified custom medical vocabulary, check the VocabularyState
field. If
* the status is READY
, your custom vocabulary is available to use. If the status is
* FAILED
, FailureReason
provides details on why your vocabulary failed.
*
*
* To get a list of your custom medical vocabularies, use the operation.
*
*
*
* This is a convenience which creates an instance of the {@link GetMedicalVocabularyRequest.Builder} avoiding the
* need to create one manually via {@link GetMedicalVocabularyRequest#builder()}
*
*
* @param getMedicalVocabularyRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transcribe.model.GetMedicalVocabularyRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetMedicalVocabulary operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException We can't find the requested resource. Check that the specified name is correct and
* try your request again.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.GetMedicalVocabulary
* @see AWS API Documentation
*/
default CompletableFuture getMedicalVocabulary(
Consumer getMedicalVocabularyRequest) {
return getMedicalVocabulary(GetMedicalVocabularyRequest.builder().applyMutation(getMedicalVocabularyRequest).build());
}
/**
*
* Provides information about the specified transcription job.
*
*
* To view the status of the specified transcription job, check the TranscriptionJobStatus
field. If
* the status is COMPLETED
, the job is finished. You can find the results at the location specified in
* TranscriptFileUri
. If the status is FAILED
, FailureReason
provides details
* on why your transcription job failed.
*
*
* If you enabled content redaction, the redacted transcript can be found at the location specified in
* RedactedTranscriptFileUri
.
*
*
* To get a list of your transcription jobs, use the operation.
*
*
* @param getTranscriptionJobRequest
* @return A Java Future containing the result of the GetTranscriptionJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - NotFoundException We can't find the requested resource. Check that the specified name is correct and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.GetTranscriptionJob
* @see AWS API Documentation
*/
default CompletableFuture getTranscriptionJob(
GetTranscriptionJobRequest getTranscriptionJobRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Provides information about the specified transcription job.
*
*
* To view the status of the specified transcription job, check the TranscriptionJobStatus
field. If
* the status is COMPLETED
, the job is finished. You can find the results at the location specified in
* TranscriptFileUri
. If the status is FAILED
, FailureReason
provides details
* on why your transcription job failed.
*
*
* If you enabled content redaction, the redacted transcript can be found at the location specified in
* RedactedTranscriptFileUri
.
*
*
* To get a list of your transcription jobs, use the operation.
*
*
*
* This is a convenience which creates an instance of the {@link GetTranscriptionJobRequest.Builder} avoiding the
* need to create one manually via {@link GetTranscriptionJobRequest#builder()}
*
*
* @param getTranscriptionJobRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transcribe.model.GetTranscriptionJobRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetTranscriptionJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - NotFoundException We can't find the requested resource. Check that the specified name is correct and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.GetTranscriptionJob
* @see AWS API Documentation
*/
default CompletableFuture getTranscriptionJob(
Consumer getTranscriptionJobRequest) {
return getTranscriptionJob(GetTranscriptionJobRequest.builder().applyMutation(getTranscriptionJobRequest).build());
}
/**
*
* Provides information about the specified custom vocabulary.
*
*
* To view the status of the specified custom vocabulary, check the VocabularyState
field. If the
* status is READY
, your custom vocabulary is available to use. If the status is FAILED
,
* FailureReason
provides details on why your custom vocabulary failed.
*
*
* To get a list of your custom vocabularies, use the operation.
*
*
* @param getVocabularyRequest
* @return A Java Future containing the result of the GetVocabulary operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException We can't find the requested resource. Check that the specified name is correct and
* try your request again.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.GetVocabulary
* @see AWS API
* Documentation
*/
default CompletableFuture getVocabulary(GetVocabularyRequest getVocabularyRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Provides information about the specified custom vocabulary.
*
*
* To view the status of the specified custom vocabulary, check the VocabularyState
field. If the
* status is READY
, your custom vocabulary is available to use. If the status is FAILED
,
* FailureReason
provides details on why your custom vocabulary failed.
*
*
* To get a list of your custom vocabularies, use the operation.
*
*
*
* This is a convenience which creates an instance of the {@link GetVocabularyRequest.Builder} avoiding the need to
* create one manually via {@link GetVocabularyRequest#builder()}
*
*
* @param getVocabularyRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transcribe.model.GetVocabularyRequest.Builder} to create a request.
* @return A Java Future containing the result of the GetVocabulary operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException We can't find the requested resource. Check that the specified name is correct and
* try your request again.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.GetVocabulary
* @see AWS API
* Documentation
*/
default CompletableFuture getVocabulary(Consumer getVocabularyRequest) {
return getVocabulary(GetVocabularyRequest.builder().applyMutation(getVocabularyRequest).build());
}
/**
*
* Provides information about the specified custom vocabulary filter.
*
*
* To get a list of your custom vocabulary filters, use the operation.
*
*
* @param getVocabularyFilterRequest
* @return A Java Future containing the result of the GetVocabularyFilter operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException We can't find the requested resource. Check that the specified name is correct and
* try your request again.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.GetVocabularyFilter
* @see AWS API Documentation
*/
default CompletableFuture getVocabularyFilter(
GetVocabularyFilterRequest getVocabularyFilterRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Provides information about the specified custom vocabulary filter.
*
*
* To get a list of your custom vocabulary filters, use the operation.
*
*
*
* This is a convenience which creates an instance of the {@link GetVocabularyFilterRequest.Builder} avoiding the
* need to create one manually via {@link GetVocabularyFilterRequest#builder()}
*
*
* @param getVocabularyFilterRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transcribe.model.GetVocabularyFilterRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetVocabularyFilter operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException We can't find the requested resource. Check that the specified name is correct and
* try your request again.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.GetVocabularyFilter
* @see AWS API Documentation
*/
default CompletableFuture getVocabularyFilter(
Consumer getVocabularyFilterRequest) {
return getVocabularyFilter(GetVocabularyFilterRequest.builder().applyMutation(getVocabularyFilterRequest).build());
}
/**
*
* Provides a list of Call Analytics categories, including all rules that make up each category.
*
*
* To get detailed information about a specific Call Analytics category, use the operation.
*
*
* @param listCallAnalyticsCategoriesRequest
* @return A Java Future containing the result of the ListCallAnalyticsCategories operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.ListCallAnalyticsCategories
* @see AWS API Documentation
*/
default CompletableFuture listCallAnalyticsCategories(
ListCallAnalyticsCategoriesRequest listCallAnalyticsCategoriesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Provides a list of Call Analytics categories, including all rules that make up each category.
*
*
* To get detailed information about a specific Call Analytics category, use the operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListCallAnalyticsCategoriesRequest.Builder}
* avoiding the need to create one manually via {@link ListCallAnalyticsCategoriesRequest#builder()}
*
*
* @param listCallAnalyticsCategoriesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transcribe.model.ListCallAnalyticsCategoriesRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the ListCallAnalyticsCategories operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.ListCallAnalyticsCategories
* @see AWS API Documentation
*/
default CompletableFuture listCallAnalyticsCategories(
Consumer listCallAnalyticsCategoriesRequest) {
return listCallAnalyticsCategories(ListCallAnalyticsCategoriesRequest.builder()
.applyMutation(listCallAnalyticsCategoriesRequest).build());
}
/**
*
* This is a variant of
* {@link #listCallAnalyticsCategories(software.amazon.awssdk.services.transcribe.model.ListCallAnalyticsCategoriesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.transcribe.paginators.ListCallAnalyticsCategoriesPublisher publisher = client.listCallAnalyticsCategoriesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.transcribe.paginators.ListCallAnalyticsCategoriesPublisher publisher = client.listCallAnalyticsCategoriesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.transcribe.model.ListCallAnalyticsCategoriesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listCallAnalyticsCategories(software.amazon.awssdk.services.transcribe.model.ListCallAnalyticsCategoriesRequest)}
* operation.
*
*
* @param listCallAnalyticsCategoriesRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.ListCallAnalyticsCategories
* @see AWS API Documentation
*/
default ListCallAnalyticsCategoriesPublisher listCallAnalyticsCategoriesPaginator(
ListCallAnalyticsCategoriesRequest listCallAnalyticsCategoriesRequest) {
return new ListCallAnalyticsCategoriesPublisher(this, listCallAnalyticsCategoriesRequest);
}
/**
*
* This is a variant of
* {@link #listCallAnalyticsCategories(software.amazon.awssdk.services.transcribe.model.ListCallAnalyticsCategoriesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.transcribe.paginators.ListCallAnalyticsCategoriesPublisher publisher = client.listCallAnalyticsCategoriesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.transcribe.paginators.ListCallAnalyticsCategoriesPublisher publisher = client.listCallAnalyticsCategoriesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.transcribe.model.ListCallAnalyticsCategoriesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listCallAnalyticsCategories(software.amazon.awssdk.services.transcribe.model.ListCallAnalyticsCategoriesRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListCallAnalyticsCategoriesRequest.Builder}
* avoiding the need to create one manually via {@link ListCallAnalyticsCategoriesRequest#builder()}
*
*
* @param listCallAnalyticsCategoriesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transcribe.model.ListCallAnalyticsCategoriesRequest.Builder} to
* create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.ListCallAnalyticsCategories
* @see AWS API Documentation
*/
default ListCallAnalyticsCategoriesPublisher listCallAnalyticsCategoriesPaginator(
Consumer listCallAnalyticsCategoriesRequest) {
return listCallAnalyticsCategoriesPaginator(ListCallAnalyticsCategoriesRequest.builder()
.applyMutation(listCallAnalyticsCategoriesRequest).build());
}
/**
*
* Provides a list of Call Analytics jobs that match the specified criteria. If no criteria are specified, all Call
* Analytics jobs are returned.
*
*
* To get detailed information about a specific Call Analytics job, use the operation.
*
*
* @param listCallAnalyticsJobsRequest
* @return A Java Future containing the result of the ListCallAnalyticsJobs operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.ListCallAnalyticsJobs
* @see AWS API Documentation
*/
default CompletableFuture listCallAnalyticsJobs(
ListCallAnalyticsJobsRequest listCallAnalyticsJobsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Provides a list of Call Analytics jobs that match the specified criteria. If no criteria are specified, all Call
* Analytics jobs are returned.
*
*
* To get detailed information about a specific Call Analytics job, use the operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListCallAnalyticsJobsRequest.Builder} avoiding the
* need to create one manually via {@link ListCallAnalyticsJobsRequest#builder()}
*
*
* @param listCallAnalyticsJobsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transcribe.model.ListCallAnalyticsJobsRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListCallAnalyticsJobs operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.ListCallAnalyticsJobs
* @see AWS API Documentation
*/
default CompletableFuture listCallAnalyticsJobs(
Consumer listCallAnalyticsJobsRequest) {
return listCallAnalyticsJobs(ListCallAnalyticsJobsRequest.builder().applyMutation(listCallAnalyticsJobsRequest).build());
}
/**
*
* This is a variant of
* {@link #listCallAnalyticsJobs(software.amazon.awssdk.services.transcribe.model.ListCallAnalyticsJobsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.transcribe.paginators.ListCallAnalyticsJobsPublisher publisher = client.listCallAnalyticsJobsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.transcribe.paginators.ListCallAnalyticsJobsPublisher publisher = client.listCallAnalyticsJobsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.transcribe.model.ListCallAnalyticsJobsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listCallAnalyticsJobs(software.amazon.awssdk.services.transcribe.model.ListCallAnalyticsJobsRequest)}
* operation.
*
*
* @param listCallAnalyticsJobsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.ListCallAnalyticsJobs
* @see AWS API Documentation
*/
default ListCallAnalyticsJobsPublisher listCallAnalyticsJobsPaginator(
ListCallAnalyticsJobsRequest listCallAnalyticsJobsRequest) {
return new ListCallAnalyticsJobsPublisher(this, listCallAnalyticsJobsRequest);
}
/**
*
* This is a variant of
* {@link #listCallAnalyticsJobs(software.amazon.awssdk.services.transcribe.model.ListCallAnalyticsJobsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.transcribe.paginators.ListCallAnalyticsJobsPublisher publisher = client.listCallAnalyticsJobsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.transcribe.paginators.ListCallAnalyticsJobsPublisher publisher = client.listCallAnalyticsJobsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.transcribe.model.ListCallAnalyticsJobsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listCallAnalyticsJobs(software.amazon.awssdk.services.transcribe.model.ListCallAnalyticsJobsRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListCallAnalyticsJobsRequest.Builder} avoiding the
* need to create one manually via {@link ListCallAnalyticsJobsRequest#builder()}
*
*
* @param listCallAnalyticsJobsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transcribe.model.ListCallAnalyticsJobsRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.ListCallAnalyticsJobs
* @see AWS API Documentation
*/
default ListCallAnalyticsJobsPublisher listCallAnalyticsJobsPaginator(
Consumer listCallAnalyticsJobsRequest) {
return listCallAnalyticsJobsPaginator(ListCallAnalyticsJobsRequest.builder().applyMutation(listCallAnalyticsJobsRequest)
.build());
}
/**
*
* Provides a list of custom language models that match the specified criteria. If no criteria are specified, all
* custom language models are returned.
*
*
* To get detailed information about a specific custom language model, use the operation.
*
*
* @param listLanguageModelsRequest
* @return A Java Future containing the result of the ListLanguageModels operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.ListLanguageModels
* @see AWS
* API Documentation
*/
default CompletableFuture listLanguageModels(ListLanguageModelsRequest listLanguageModelsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Provides a list of custom language models that match the specified criteria. If no criteria are specified, all
* custom language models are returned.
*
*
* To get detailed information about a specific custom language model, use the operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListLanguageModelsRequest.Builder} avoiding the
* need to create one manually via {@link ListLanguageModelsRequest#builder()}
*
*
* @param listLanguageModelsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transcribe.model.ListLanguageModelsRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListLanguageModels operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.ListLanguageModels
* @see AWS
* API Documentation
*/
default CompletableFuture listLanguageModels(
Consumer listLanguageModelsRequest) {
return listLanguageModels(ListLanguageModelsRequest.builder().applyMutation(listLanguageModelsRequest).build());
}
/**
*
* This is a variant of
* {@link #listLanguageModels(software.amazon.awssdk.services.transcribe.model.ListLanguageModelsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.transcribe.paginators.ListLanguageModelsPublisher publisher = client.listLanguageModelsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.transcribe.paginators.ListLanguageModelsPublisher publisher = client.listLanguageModelsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.transcribe.model.ListLanguageModelsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listLanguageModels(software.amazon.awssdk.services.transcribe.model.ListLanguageModelsRequest)}
* operation.
*
*
* @param listLanguageModelsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.ListLanguageModels
* @see AWS
* API Documentation
*/
default ListLanguageModelsPublisher listLanguageModelsPaginator(ListLanguageModelsRequest listLanguageModelsRequest) {
return new ListLanguageModelsPublisher(this, listLanguageModelsRequest);
}
/**
*
* This is a variant of
* {@link #listLanguageModels(software.amazon.awssdk.services.transcribe.model.ListLanguageModelsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.transcribe.paginators.ListLanguageModelsPublisher publisher = client.listLanguageModelsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.transcribe.paginators.ListLanguageModelsPublisher publisher = client.listLanguageModelsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.transcribe.model.ListLanguageModelsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listLanguageModels(software.amazon.awssdk.services.transcribe.model.ListLanguageModelsRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListLanguageModelsRequest.Builder} avoiding the
* need to create one manually via {@link ListLanguageModelsRequest#builder()}
*
*
* @param listLanguageModelsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transcribe.model.ListLanguageModelsRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.ListLanguageModels
* @see AWS
* API Documentation
*/
default ListLanguageModelsPublisher listLanguageModelsPaginator(
Consumer listLanguageModelsRequest) {
return listLanguageModelsPaginator(ListLanguageModelsRequest.builder().applyMutation(listLanguageModelsRequest).build());
}
/**
*
* Provides a list of Medical Scribe jobs that match the specified criteria. If no criteria are specified, all
* Medical Scribe jobs are returned.
*
*
* To get detailed information about a specific Medical Scribe job, use the operation.
*
*
* @param listMedicalScribeJobsRequest
* @return A Java Future containing the result of the ListMedicalScribeJobs operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.ListMedicalScribeJobs
* @see AWS API Documentation
*/
default CompletableFuture listMedicalScribeJobs(
ListMedicalScribeJobsRequest listMedicalScribeJobsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Provides a list of Medical Scribe jobs that match the specified criteria. If no criteria are specified, all
* Medical Scribe jobs are returned.
*
*
* To get detailed information about a specific Medical Scribe job, use the operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListMedicalScribeJobsRequest.Builder} avoiding the
* need to create one manually via {@link ListMedicalScribeJobsRequest#builder()}
*
*
* @param listMedicalScribeJobsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transcribe.model.ListMedicalScribeJobsRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListMedicalScribeJobs operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.ListMedicalScribeJobs
* @see AWS API Documentation
*/
default CompletableFuture listMedicalScribeJobs(
Consumer listMedicalScribeJobsRequest) {
return listMedicalScribeJobs(ListMedicalScribeJobsRequest.builder().applyMutation(listMedicalScribeJobsRequest).build());
}
/**
*
* This is a variant of
* {@link #listMedicalScribeJobs(software.amazon.awssdk.services.transcribe.model.ListMedicalScribeJobsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.transcribe.paginators.ListMedicalScribeJobsPublisher publisher = client.listMedicalScribeJobsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.transcribe.paginators.ListMedicalScribeJobsPublisher publisher = client.listMedicalScribeJobsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.transcribe.model.ListMedicalScribeJobsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listMedicalScribeJobs(software.amazon.awssdk.services.transcribe.model.ListMedicalScribeJobsRequest)}
* operation.
*
*
* @param listMedicalScribeJobsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.ListMedicalScribeJobs
* @see AWS API Documentation
*/
default ListMedicalScribeJobsPublisher listMedicalScribeJobsPaginator(
ListMedicalScribeJobsRequest listMedicalScribeJobsRequest) {
return new ListMedicalScribeJobsPublisher(this, listMedicalScribeJobsRequest);
}
/**
*
* This is a variant of
* {@link #listMedicalScribeJobs(software.amazon.awssdk.services.transcribe.model.ListMedicalScribeJobsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.transcribe.paginators.ListMedicalScribeJobsPublisher publisher = client.listMedicalScribeJobsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.transcribe.paginators.ListMedicalScribeJobsPublisher publisher = client.listMedicalScribeJobsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.transcribe.model.ListMedicalScribeJobsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listMedicalScribeJobs(software.amazon.awssdk.services.transcribe.model.ListMedicalScribeJobsRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListMedicalScribeJobsRequest.Builder} avoiding the
* need to create one manually via {@link ListMedicalScribeJobsRequest#builder()}
*
*
* @param listMedicalScribeJobsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transcribe.model.ListMedicalScribeJobsRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.ListMedicalScribeJobs
* @see AWS API Documentation
*/
default ListMedicalScribeJobsPublisher listMedicalScribeJobsPaginator(
Consumer listMedicalScribeJobsRequest) {
return listMedicalScribeJobsPaginator(ListMedicalScribeJobsRequest.builder().applyMutation(listMedicalScribeJobsRequest)
.build());
}
/**
*
* Provides a list of medical transcription jobs that match the specified criteria. If no criteria are specified,
* all medical transcription jobs are returned.
*
*
* To get detailed information about a specific medical transcription job, use the operation.
*
*
* @param listMedicalTranscriptionJobsRequest
* @return A Java Future containing the result of the ListMedicalTranscriptionJobs operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.ListMedicalTranscriptionJobs
* @see AWS API Documentation
*/
default CompletableFuture listMedicalTranscriptionJobs(
ListMedicalTranscriptionJobsRequest listMedicalTranscriptionJobsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Provides a list of medical transcription jobs that match the specified criteria. If no criteria are specified,
* all medical transcription jobs are returned.
*
*
* To get detailed information about a specific medical transcription job, use the operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListMedicalTranscriptionJobsRequest.Builder}
* avoiding the need to create one manually via {@link ListMedicalTranscriptionJobsRequest#builder()}
*
*
* @param listMedicalTranscriptionJobsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transcribe.model.ListMedicalTranscriptionJobsRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the ListMedicalTranscriptionJobs operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.ListMedicalTranscriptionJobs
* @see AWS API Documentation
*/
default CompletableFuture listMedicalTranscriptionJobs(
Consumer listMedicalTranscriptionJobsRequest) {
return listMedicalTranscriptionJobs(ListMedicalTranscriptionJobsRequest.builder()
.applyMutation(listMedicalTranscriptionJobsRequest).build());
}
/**
*
* This is a variant of
* {@link #listMedicalTranscriptionJobs(software.amazon.awssdk.services.transcribe.model.ListMedicalTranscriptionJobsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.transcribe.paginators.ListMedicalTranscriptionJobsPublisher publisher = client.listMedicalTranscriptionJobsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.transcribe.paginators.ListMedicalTranscriptionJobsPublisher publisher = client.listMedicalTranscriptionJobsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.transcribe.model.ListMedicalTranscriptionJobsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listMedicalTranscriptionJobs(software.amazon.awssdk.services.transcribe.model.ListMedicalTranscriptionJobsRequest)}
* operation.
*
*
* @param listMedicalTranscriptionJobsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.ListMedicalTranscriptionJobs
* @see AWS API Documentation
*/
default ListMedicalTranscriptionJobsPublisher listMedicalTranscriptionJobsPaginator(
ListMedicalTranscriptionJobsRequest listMedicalTranscriptionJobsRequest) {
return new ListMedicalTranscriptionJobsPublisher(this, listMedicalTranscriptionJobsRequest);
}
/**
*
* This is a variant of
* {@link #listMedicalTranscriptionJobs(software.amazon.awssdk.services.transcribe.model.ListMedicalTranscriptionJobsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.transcribe.paginators.ListMedicalTranscriptionJobsPublisher publisher = client.listMedicalTranscriptionJobsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.transcribe.paginators.ListMedicalTranscriptionJobsPublisher publisher = client.listMedicalTranscriptionJobsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.transcribe.model.ListMedicalTranscriptionJobsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listMedicalTranscriptionJobs(software.amazon.awssdk.services.transcribe.model.ListMedicalTranscriptionJobsRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListMedicalTranscriptionJobsRequest.Builder}
* avoiding the need to create one manually via {@link ListMedicalTranscriptionJobsRequest#builder()}
*
*
* @param listMedicalTranscriptionJobsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transcribe.model.ListMedicalTranscriptionJobsRequest.Builder} to
* create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.ListMedicalTranscriptionJobs
* @see AWS API Documentation
*/
default ListMedicalTranscriptionJobsPublisher listMedicalTranscriptionJobsPaginator(
Consumer listMedicalTranscriptionJobsRequest) {
return listMedicalTranscriptionJobsPaginator(ListMedicalTranscriptionJobsRequest.builder()
.applyMutation(listMedicalTranscriptionJobsRequest).build());
}
/**
*
* Provides a list of custom medical vocabularies that match the specified criteria. If no criteria are specified,
* all custom medical vocabularies are returned.
*
*
* To get detailed information about a specific custom medical vocabulary, use the operation.
*
*
* @param listMedicalVocabulariesRequest
* @return A Java Future containing the result of the ListMedicalVocabularies operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.ListMedicalVocabularies
* @see AWS API Documentation
*/
default CompletableFuture listMedicalVocabularies(
ListMedicalVocabulariesRequest listMedicalVocabulariesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Provides a list of custom medical vocabularies that match the specified criteria. If no criteria are specified,
* all custom medical vocabularies are returned.
*
*
* To get detailed information about a specific custom medical vocabulary, use the operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListMedicalVocabulariesRequest.Builder} avoiding
* the need to create one manually via {@link ListMedicalVocabulariesRequest#builder()}
*
*
* @param listMedicalVocabulariesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transcribe.model.ListMedicalVocabulariesRequest.Builder} to create
* a request.
* @return A Java Future containing the result of the ListMedicalVocabularies operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.ListMedicalVocabularies
* @see AWS API Documentation
*/
default CompletableFuture listMedicalVocabularies(
Consumer listMedicalVocabulariesRequest) {
return listMedicalVocabularies(ListMedicalVocabulariesRequest.builder().applyMutation(listMedicalVocabulariesRequest)
.build());
}
/**
*
* This is a variant of
* {@link #listMedicalVocabularies(software.amazon.awssdk.services.transcribe.model.ListMedicalVocabulariesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.transcribe.paginators.ListMedicalVocabulariesPublisher publisher = client.listMedicalVocabulariesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.transcribe.paginators.ListMedicalVocabulariesPublisher publisher = client.listMedicalVocabulariesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.transcribe.model.ListMedicalVocabulariesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listMedicalVocabularies(software.amazon.awssdk.services.transcribe.model.ListMedicalVocabulariesRequest)}
* operation.
*
*
* @param listMedicalVocabulariesRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.ListMedicalVocabularies
* @see AWS API Documentation
*/
default ListMedicalVocabulariesPublisher listMedicalVocabulariesPaginator(
ListMedicalVocabulariesRequest listMedicalVocabulariesRequest) {
return new ListMedicalVocabulariesPublisher(this, listMedicalVocabulariesRequest);
}
/**
*
* This is a variant of
* {@link #listMedicalVocabularies(software.amazon.awssdk.services.transcribe.model.ListMedicalVocabulariesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.transcribe.paginators.ListMedicalVocabulariesPublisher publisher = client.listMedicalVocabulariesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.transcribe.paginators.ListMedicalVocabulariesPublisher publisher = client.listMedicalVocabulariesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.transcribe.model.ListMedicalVocabulariesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listMedicalVocabularies(software.amazon.awssdk.services.transcribe.model.ListMedicalVocabulariesRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListMedicalVocabulariesRequest.Builder} avoiding
* the need to create one manually via {@link ListMedicalVocabulariesRequest#builder()}
*
*
* @param listMedicalVocabulariesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transcribe.model.ListMedicalVocabulariesRequest.Builder} to create
* a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.ListMedicalVocabularies
* @see AWS API Documentation
*/
default ListMedicalVocabulariesPublisher listMedicalVocabulariesPaginator(
Consumer listMedicalVocabulariesRequest) {
return listMedicalVocabulariesPaginator(ListMedicalVocabulariesRequest.builder()
.applyMutation(listMedicalVocabulariesRequest).build());
}
/**
*
* Lists all tags associated with the specified transcription job, vocabulary, model, or resource.
*
*
* To learn more about using tags with Amazon Transcribe, refer to Tagging resources.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - NotFoundException We can't find the requested resource. Check that the specified name is correct and
* try your request again.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.ListTagsForResource
* @see AWS API Documentation
*/
default CompletableFuture listTagsForResource(
ListTagsForResourceRequest listTagsForResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists all tags associated with the specified transcription job, vocabulary, model, or resource.
*
*
* To learn more about using tags with Amazon Transcribe, refer to Tagging resources.
*
*
*
* This is a convenience which creates an instance of the {@link ListTagsForResourceRequest.Builder} avoiding the
* need to create one manually via {@link ListTagsForResourceRequest#builder()}
*
*
* @param listTagsForResourceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transcribe.model.ListTagsForResourceRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - NotFoundException We can't find the requested resource. Check that the specified name is correct and
* try your request again.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.ListTagsForResource
* @see AWS API Documentation
*/
default CompletableFuture listTagsForResource(
Consumer listTagsForResourceRequest) {
return listTagsForResource(ListTagsForResourceRequest.builder().applyMutation(listTagsForResourceRequest).build());
}
/**
*
* Provides a list of transcription jobs that match the specified criteria. If no criteria are specified, all
* transcription jobs are returned.
*
*
* To get detailed information about a specific transcription job, use the operation.
*
*
* @param listTranscriptionJobsRequest
* @return A Java Future containing the result of the ListTranscriptionJobs operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.ListTranscriptionJobs
* @see AWS API Documentation
*/
default CompletableFuture listTranscriptionJobs(
ListTranscriptionJobsRequest listTranscriptionJobsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Provides a list of transcription jobs that match the specified criteria. If no criteria are specified, all
* transcription jobs are returned.
*
*
* To get detailed information about a specific transcription job, use the operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListTranscriptionJobsRequest.Builder} avoiding the
* need to create one manually via {@link ListTranscriptionJobsRequest#builder()}
*
*
* @param listTranscriptionJobsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transcribe.model.ListTranscriptionJobsRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListTranscriptionJobs operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.ListTranscriptionJobs
* @see AWS API Documentation
*/
default CompletableFuture listTranscriptionJobs(
Consumer listTranscriptionJobsRequest) {
return listTranscriptionJobs(ListTranscriptionJobsRequest.builder().applyMutation(listTranscriptionJobsRequest).build());
}
/**
*
* Provides a list of transcription jobs that match the specified criteria. If no criteria are specified, all
* transcription jobs are returned.
*
*
* To get detailed information about a specific transcription job, use the operation.
*
*
* @return A Java Future containing the result of the ListTranscriptionJobs operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.ListTranscriptionJobs
* @see AWS API Documentation
*/
default CompletableFuture listTranscriptionJobs() {
return listTranscriptionJobs(ListTranscriptionJobsRequest.builder().build());
}
/**
*
* This is a variant of
* {@link #listTranscriptionJobs(software.amazon.awssdk.services.transcribe.model.ListTranscriptionJobsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.transcribe.paginators.ListTranscriptionJobsPublisher publisher = client.listTranscriptionJobsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.transcribe.paginators.ListTranscriptionJobsPublisher publisher = client.listTranscriptionJobsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.transcribe.model.ListTranscriptionJobsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listTranscriptionJobs(software.amazon.awssdk.services.transcribe.model.ListTranscriptionJobsRequest)}
* operation.
*
*
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.ListTranscriptionJobs
* @see AWS API Documentation
*/
default ListTranscriptionJobsPublisher listTranscriptionJobsPaginator() {
return listTranscriptionJobsPaginator(ListTranscriptionJobsRequest.builder().build());
}
/**
*
* This is a variant of
* {@link #listTranscriptionJobs(software.amazon.awssdk.services.transcribe.model.ListTranscriptionJobsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.transcribe.paginators.ListTranscriptionJobsPublisher publisher = client.listTranscriptionJobsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.transcribe.paginators.ListTranscriptionJobsPublisher publisher = client.listTranscriptionJobsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.transcribe.model.ListTranscriptionJobsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listTranscriptionJobs(software.amazon.awssdk.services.transcribe.model.ListTranscriptionJobsRequest)}
* operation.
*
*
* @param listTranscriptionJobsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.ListTranscriptionJobs
* @see AWS API Documentation
*/
default ListTranscriptionJobsPublisher listTranscriptionJobsPaginator(
ListTranscriptionJobsRequest listTranscriptionJobsRequest) {
return new ListTranscriptionJobsPublisher(this, listTranscriptionJobsRequest);
}
/**
*
* This is a variant of
* {@link #listTranscriptionJobs(software.amazon.awssdk.services.transcribe.model.ListTranscriptionJobsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.transcribe.paginators.ListTranscriptionJobsPublisher publisher = client.listTranscriptionJobsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.transcribe.paginators.ListTranscriptionJobsPublisher publisher = client.listTranscriptionJobsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.transcribe.model.ListTranscriptionJobsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listTranscriptionJobs(software.amazon.awssdk.services.transcribe.model.ListTranscriptionJobsRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListTranscriptionJobsRequest.Builder} avoiding the
* need to create one manually via {@link ListTranscriptionJobsRequest#builder()}
*
*
* @param listTranscriptionJobsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transcribe.model.ListTranscriptionJobsRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.ListTranscriptionJobs
* @see AWS API Documentation
*/
default ListTranscriptionJobsPublisher listTranscriptionJobsPaginator(
Consumer listTranscriptionJobsRequest) {
return listTranscriptionJobsPaginator(ListTranscriptionJobsRequest.builder().applyMutation(listTranscriptionJobsRequest)
.build());
}
/**
*
* Provides a list of custom vocabularies that match the specified criteria. If no criteria are specified, all
* custom vocabularies are returned.
*
*
* To get detailed information about a specific custom vocabulary, use the operation.
*
*
* @param listVocabulariesRequest
* @return A Java Future containing the result of the ListVocabularies operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.ListVocabularies
* @see AWS
* API Documentation
*/
default CompletableFuture listVocabularies(ListVocabulariesRequest listVocabulariesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Provides a list of custom vocabularies that match the specified criteria. If no criteria are specified, all
* custom vocabularies are returned.
*
*
* To get detailed information about a specific custom vocabulary, use the operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListVocabulariesRequest.Builder} avoiding the need
* to create one manually via {@link ListVocabulariesRequest#builder()}
*
*
* @param listVocabulariesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transcribe.model.ListVocabulariesRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListVocabularies operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.ListVocabularies
* @see AWS
* API Documentation
*/
default CompletableFuture listVocabularies(
Consumer listVocabulariesRequest) {
return listVocabularies(ListVocabulariesRequest.builder().applyMutation(listVocabulariesRequest).build());
}
/**
*
* Provides a list of custom vocabularies that match the specified criteria. If no criteria are specified, all
* custom vocabularies are returned.
*
*
* To get detailed information about a specific custom vocabulary, use the operation.
*
*
* @return A Java Future containing the result of the ListVocabularies operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.ListVocabularies
* @see AWS
* API Documentation
*/
default CompletableFuture listVocabularies() {
return listVocabularies(ListVocabulariesRequest.builder().build());
}
/**
*
* This is a variant of
* {@link #listVocabularies(software.amazon.awssdk.services.transcribe.model.ListVocabulariesRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.transcribe.paginators.ListVocabulariesPublisher publisher = client.listVocabulariesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.transcribe.paginators.ListVocabulariesPublisher publisher = client.listVocabulariesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.transcribe.model.ListVocabulariesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listVocabularies(software.amazon.awssdk.services.transcribe.model.ListVocabulariesRequest)}
* operation.
*
*
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.ListVocabularies
* @see AWS
* API Documentation
*/
default ListVocabulariesPublisher listVocabulariesPaginator() {
return listVocabulariesPaginator(ListVocabulariesRequest.builder().build());
}
/**
*
* This is a variant of
* {@link #listVocabularies(software.amazon.awssdk.services.transcribe.model.ListVocabulariesRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.transcribe.paginators.ListVocabulariesPublisher publisher = client.listVocabulariesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.transcribe.paginators.ListVocabulariesPublisher publisher = client.listVocabulariesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.transcribe.model.ListVocabulariesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listVocabularies(software.amazon.awssdk.services.transcribe.model.ListVocabulariesRequest)}
* operation.
*
*
* @param listVocabulariesRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.ListVocabularies
* @see AWS
* API Documentation
*/
default ListVocabulariesPublisher listVocabulariesPaginator(ListVocabulariesRequest listVocabulariesRequest) {
return new ListVocabulariesPublisher(this, listVocabulariesRequest);
}
/**
*
* This is a variant of
* {@link #listVocabularies(software.amazon.awssdk.services.transcribe.model.ListVocabulariesRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.transcribe.paginators.ListVocabulariesPublisher publisher = client.listVocabulariesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.transcribe.paginators.ListVocabulariesPublisher publisher = client.listVocabulariesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.transcribe.model.ListVocabulariesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listVocabularies(software.amazon.awssdk.services.transcribe.model.ListVocabulariesRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListVocabulariesRequest.Builder} avoiding the need
* to create one manually via {@link ListVocabulariesRequest#builder()}
*
*
* @param listVocabulariesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transcribe.model.ListVocabulariesRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.ListVocabularies
* @see AWS
* API Documentation
*/
default ListVocabulariesPublisher listVocabulariesPaginator(Consumer listVocabulariesRequest) {
return listVocabulariesPaginator(ListVocabulariesRequest.builder().applyMutation(listVocabulariesRequest).build());
}
/**
*
* Provides a list of custom vocabulary filters that match the specified criteria. If no criteria are specified, all
* custom vocabularies are returned.
*
*
* To get detailed information about a specific custom vocabulary filter, use the operation.
*
*
* @param listVocabularyFiltersRequest
* @return A Java Future containing the result of the ListVocabularyFilters operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.ListVocabularyFilters
* @see AWS API Documentation
*/
default CompletableFuture listVocabularyFilters(
ListVocabularyFiltersRequest listVocabularyFiltersRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Provides a list of custom vocabulary filters that match the specified criteria. If no criteria are specified, all
* custom vocabularies are returned.
*
*
* To get detailed information about a specific custom vocabulary filter, use the operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListVocabularyFiltersRequest.Builder} avoiding the
* need to create one manually via {@link ListVocabularyFiltersRequest#builder()}
*
*
* @param listVocabularyFiltersRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transcribe.model.ListVocabularyFiltersRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListVocabularyFilters operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.ListVocabularyFilters
* @see AWS API Documentation
*/
default CompletableFuture listVocabularyFilters(
Consumer listVocabularyFiltersRequest) {
return listVocabularyFilters(ListVocabularyFiltersRequest.builder().applyMutation(listVocabularyFiltersRequest).build());
}
/**
*
* This is a variant of
* {@link #listVocabularyFilters(software.amazon.awssdk.services.transcribe.model.ListVocabularyFiltersRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.transcribe.paginators.ListVocabularyFiltersPublisher publisher = client.listVocabularyFiltersPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.transcribe.paginators.ListVocabularyFiltersPublisher publisher = client.listVocabularyFiltersPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.transcribe.model.ListVocabularyFiltersResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listVocabularyFilters(software.amazon.awssdk.services.transcribe.model.ListVocabularyFiltersRequest)}
* operation.
*
*
* @param listVocabularyFiltersRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.ListVocabularyFilters
* @see AWS API Documentation
*/
default ListVocabularyFiltersPublisher listVocabularyFiltersPaginator(
ListVocabularyFiltersRequest listVocabularyFiltersRequest) {
return new ListVocabularyFiltersPublisher(this, listVocabularyFiltersRequest);
}
/**
*
* This is a variant of
* {@link #listVocabularyFilters(software.amazon.awssdk.services.transcribe.model.ListVocabularyFiltersRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.transcribe.paginators.ListVocabularyFiltersPublisher publisher = client.listVocabularyFiltersPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.transcribe.paginators.ListVocabularyFiltersPublisher publisher = client.listVocabularyFiltersPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.transcribe.model.ListVocabularyFiltersResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listVocabularyFilters(software.amazon.awssdk.services.transcribe.model.ListVocabularyFiltersRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListVocabularyFiltersRequest.Builder} avoiding the
* need to create one manually via {@link ListVocabularyFiltersRequest#builder()}
*
*
* @param listVocabularyFiltersRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transcribe.model.ListVocabularyFiltersRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.ListVocabularyFilters
* @see AWS API Documentation
*/
default ListVocabularyFiltersPublisher listVocabularyFiltersPaginator(
Consumer listVocabularyFiltersRequest) {
return listVocabularyFiltersPaginator(ListVocabularyFiltersRequest.builder().applyMutation(listVocabularyFiltersRequest)
.build());
}
/**
*
* Transcribes the audio from a customer service call and applies any additional Request Parameters you choose to
* include in your request.
*
*
* In addition to many standard transcription features, Call Analytics provides you with call characteristics, call
* summarization, speaker sentiment, and optional redaction of your text transcript and your audio file. You can
* also apply custom categories to flag specified conditions. To learn more about these features and insights, refer
* to Analyzing call center audio
* with Call Analytics.
*
*
* If you want to apply categories to your Call Analytics job, you must create them before submitting your job
* request. Categories cannot be retroactively applied to a job. To create a new category, use the operation. To
* learn more about Call Analytics categories, see Creating categories for
* post-call transcriptions and Creating categories for
* real-time transcriptions.
*
*
* To make a StartCallAnalyticsJob
request, you must first upload your media file into an Amazon S3
* bucket; you can then specify the Amazon S3 location of the file using the Media
parameter.
*
*
* Note that job queuing is enabled by default for Call Analytics jobs.
*
*
* You must include the following parameters in your StartCallAnalyticsJob
request:
*
*
* -
*
* region
: The Amazon Web Services Region where you are making your request. For a list of Amazon Web
* Services Regions supported with Amazon Transcribe, refer to Amazon Transcribe endpoints and quotas.
*
*
* -
*
* CallAnalyticsJobName
: A custom name that you create for your transcription job that's unique within
* your Amazon Web Services account.
*
*
* -
*
* DataAccessRoleArn
: The Amazon Resource Name (ARN) of an IAM role that has permissions to access the
* Amazon S3 bucket that contains your input files.
*
*
* -
*
* Media
(MediaFileUri
or RedactedMediaFileUri
): The Amazon S3 location of
* your media file.
*
*
*
*
*
* With Call Analytics, you can redact the audio contained in your media file by including
* RedactedMediaFileUri
, instead of MediaFileUri
, to specify the location of your input
* audio. If you choose to redact your audio, you can find your redacted media at the location specified in the
* RedactedMediaFileUri
field of your response.
*
*
*
* @param startCallAnalyticsJobRequest
* @return A Java Future containing the result of the StartCallAnalyticsJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - ConflictException A resource already exists with this name. Resource names must be unique within an
* Amazon Web Services account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.StartCallAnalyticsJob
* @see AWS API Documentation
*/
default CompletableFuture startCallAnalyticsJob(
StartCallAnalyticsJobRequest startCallAnalyticsJobRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Transcribes the audio from a customer service call and applies any additional Request Parameters you choose to
* include in your request.
*
*
* In addition to many standard transcription features, Call Analytics provides you with call characteristics, call
* summarization, speaker sentiment, and optional redaction of your text transcript and your audio file. You can
* also apply custom categories to flag specified conditions. To learn more about these features and insights, refer
* to Analyzing call center audio
* with Call Analytics.
*
*
* If you want to apply categories to your Call Analytics job, you must create them before submitting your job
* request. Categories cannot be retroactively applied to a job. To create a new category, use the operation. To
* learn more about Call Analytics categories, see Creating categories for
* post-call transcriptions and Creating categories for
* real-time transcriptions.
*
*
* To make a StartCallAnalyticsJob
request, you must first upload your media file into an Amazon S3
* bucket; you can then specify the Amazon S3 location of the file using the Media
parameter.
*
*
* Note that job queuing is enabled by default for Call Analytics jobs.
*
*
* You must include the following parameters in your StartCallAnalyticsJob
request:
*
*
* -
*
* region
: The Amazon Web Services Region where you are making your request. For a list of Amazon Web
* Services Regions supported with Amazon Transcribe, refer to Amazon Transcribe endpoints and quotas.
*
*
* -
*
* CallAnalyticsJobName
: A custom name that you create for your transcription job that's unique within
* your Amazon Web Services account.
*
*
* -
*
* DataAccessRoleArn
: The Amazon Resource Name (ARN) of an IAM role that has permissions to access the
* Amazon S3 bucket that contains your input files.
*
*
* -
*
* Media
(MediaFileUri
or RedactedMediaFileUri
): The Amazon S3 location of
* your media file.
*
*
*
*
*
* With Call Analytics, you can redact the audio contained in your media file by including
* RedactedMediaFileUri
, instead of MediaFileUri
, to specify the location of your input
* audio. If you choose to redact your audio, you can find your redacted media at the location specified in the
* RedactedMediaFileUri
field of your response.
*
*
*
* This is a convenience which creates an instance of the {@link StartCallAnalyticsJobRequest.Builder} avoiding the
* need to create one manually via {@link StartCallAnalyticsJobRequest#builder()}
*
*
* @param startCallAnalyticsJobRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transcribe.model.StartCallAnalyticsJobRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the StartCallAnalyticsJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - ConflictException A resource already exists with this name. Resource names must be unique within an
* Amazon Web Services account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.StartCallAnalyticsJob
* @see AWS API Documentation
*/
default CompletableFuture startCallAnalyticsJob(
Consumer startCallAnalyticsJobRequest) {
return startCallAnalyticsJob(StartCallAnalyticsJobRequest.builder().applyMutation(startCallAnalyticsJobRequest).build());
}
/**
*
* Transcribes patient-clinician conversations and generates clinical notes.
*
*
* Amazon Web Services HealthScribe automatically provides rich conversation transcripts, identifies speaker roles,
* classifies dialogues, extracts medical terms, and generates preliminary clinical notes. To learn more about these
* features, refer to Amazon Web
* Services HealthScribe.
*
*
* To make a StartMedicalScribeJob
request, you must first upload your media file into an Amazon S3
* bucket; you can then specify the Amazon S3 location of the file using the Media
parameter.
*
*
* You must include the following parameters in your StartMedicalTranscriptionJob
request:
*
*
* -
*
* DataAccessRoleArn
: The ARN of an IAM role with the these minimum permissions: read permission on
* input file Amazon S3 bucket specified in Media
, write permission on the Amazon S3 bucket specified
* in OutputBucketName
, and full permissions on the KMS key specified in
* OutputEncryptionKMSKeyId
(if set). The role should also allow transcribe.amazonaws.com
* to assume it.
*
*
* -
*
* Media
(MediaFileUri
): The Amazon S3 location of your media file.
*
*
* -
*
* MedicalScribeJobName
: A custom name you create for your MedicalScribe job that is unique within your
* Amazon Web Services account.
*
*
* -
*
* OutputBucketName
: The Amazon S3 bucket where you want your output files stored.
*
*
* -
*
* Settings
: A MedicalScribeSettings
obect that must set exactly one of
* ShowSpeakerLabels
or ChannelIdentification
to true. If ShowSpeakerLabels
* is true, MaxSpeakerLabels
must also be set.
*
*
* -
*
* ChannelDefinitions
: A MedicalScribeChannelDefinitions
array should be set if and only
* if the ChannelIdentification
value of Settings
is set to true.
*
*
*
*
* @param startMedicalScribeJobRequest
* @return A Java Future containing the result of the StartMedicalScribeJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - ConflictException A resource already exists with this name. Resource names must be unique within an
* Amazon Web Services account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.StartMedicalScribeJob
* @see AWS API Documentation
*/
default CompletableFuture startMedicalScribeJob(
StartMedicalScribeJobRequest startMedicalScribeJobRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Transcribes patient-clinician conversations and generates clinical notes.
*
*
* Amazon Web Services HealthScribe automatically provides rich conversation transcripts, identifies speaker roles,
* classifies dialogues, extracts medical terms, and generates preliminary clinical notes. To learn more about these
* features, refer to Amazon Web
* Services HealthScribe.
*
*
* To make a StartMedicalScribeJob
request, you must first upload your media file into an Amazon S3
* bucket; you can then specify the Amazon S3 location of the file using the Media
parameter.
*
*
* You must include the following parameters in your StartMedicalTranscriptionJob
request:
*
*
* -
*
* DataAccessRoleArn
: The ARN of an IAM role with the these minimum permissions: read permission on
* input file Amazon S3 bucket specified in Media
, write permission on the Amazon S3 bucket specified
* in OutputBucketName
, and full permissions on the KMS key specified in
* OutputEncryptionKMSKeyId
(if set). The role should also allow transcribe.amazonaws.com
* to assume it.
*
*
* -
*
* Media
(MediaFileUri
): The Amazon S3 location of your media file.
*
*
* -
*
* MedicalScribeJobName
: A custom name you create for your MedicalScribe job that is unique within your
* Amazon Web Services account.
*
*
* -
*
* OutputBucketName
: The Amazon S3 bucket where you want your output files stored.
*
*
* -
*
* Settings
: A MedicalScribeSettings
obect that must set exactly one of
* ShowSpeakerLabels
or ChannelIdentification
to true. If ShowSpeakerLabels
* is true, MaxSpeakerLabels
must also be set.
*
*
* -
*
* ChannelDefinitions
: A MedicalScribeChannelDefinitions
array should be set if and only
* if the ChannelIdentification
value of Settings
is set to true.
*
*
*
*
*
* This is a convenience which creates an instance of the {@link StartMedicalScribeJobRequest.Builder} avoiding the
* need to create one manually via {@link StartMedicalScribeJobRequest#builder()}
*
*
* @param startMedicalScribeJobRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transcribe.model.StartMedicalScribeJobRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the StartMedicalScribeJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - ConflictException A resource already exists with this name. Resource names must be unique within an
* Amazon Web Services account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.StartMedicalScribeJob
* @see AWS API Documentation
*/
default CompletableFuture startMedicalScribeJob(
Consumer startMedicalScribeJobRequest) {
return startMedicalScribeJob(StartMedicalScribeJobRequest.builder().applyMutation(startMedicalScribeJobRequest).build());
}
/**
*
* Transcribes the audio from a medical dictation or conversation and applies any additional Request Parameters you
* choose to include in your request.
*
*
* In addition to many standard transcription features, Amazon Transcribe Medical provides you with a robust medical
* vocabulary and, optionally, content identification, which adds flags to personal health information (PHI). To
* learn more about these features, refer to How Amazon Transcribe Medical
* works.
*
*
* To make a StartMedicalTranscriptionJob
request, you must first upload your media file into an Amazon
* S3 bucket; you can then specify the Amazon S3 location of the file using the Media
parameter.
*
*
* You must include the following parameters in your StartMedicalTranscriptionJob
request:
*
*
* -
*
* region
: The Amazon Web Services Region where you are making your request. For a list of Amazon Web
* Services Regions supported with Amazon Transcribe, refer to Amazon Transcribe endpoints and quotas.
*
*
* -
*
* MedicalTranscriptionJobName
: A custom name you create for your transcription job that is unique
* within your Amazon Web Services account.
*
*
* -
*
* Media
(MediaFileUri
): The Amazon S3 location of your media file.
*
*
* -
*
* LanguageCode
: This must be en-US
.
*
*
* -
*
* OutputBucketName
: The Amazon S3 bucket where you want your transcript stored. If you want your
* output stored in a sub-folder of this bucket, you must also include OutputKey
.
*
*
* -
*
* Specialty
: This must be PRIMARYCARE
.
*
*
* -
*
* Type
: Choose whether your audio is a conversation or a dictation.
*
*
*
*
* @param startMedicalTranscriptionJobRequest
* @return A Java Future containing the result of the StartMedicalTranscriptionJob operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - ConflictException A resource already exists with this name. Resource names must be unique within an
* Amazon Web Services account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.StartMedicalTranscriptionJob
* @see AWS API Documentation
*/
default CompletableFuture startMedicalTranscriptionJob(
StartMedicalTranscriptionJobRequest startMedicalTranscriptionJobRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Transcribes the audio from a medical dictation or conversation and applies any additional Request Parameters you
* choose to include in your request.
*
*
* In addition to many standard transcription features, Amazon Transcribe Medical provides you with a robust medical
* vocabulary and, optionally, content identification, which adds flags to personal health information (PHI). To
* learn more about these features, refer to How Amazon Transcribe Medical
* works.
*
*
* To make a StartMedicalTranscriptionJob
request, you must first upload your media file into an Amazon
* S3 bucket; you can then specify the Amazon S3 location of the file using the Media
parameter.
*
*
* You must include the following parameters in your StartMedicalTranscriptionJob
request:
*
*
* -
*
* region
: The Amazon Web Services Region where you are making your request. For a list of Amazon Web
* Services Regions supported with Amazon Transcribe, refer to Amazon Transcribe endpoints and quotas.
*
*
* -
*
* MedicalTranscriptionJobName
: A custom name you create for your transcription job that is unique
* within your Amazon Web Services account.
*
*
* -
*
* Media
(MediaFileUri
): The Amazon S3 location of your media file.
*
*
* -
*
* LanguageCode
: This must be en-US
.
*
*
* -
*
* OutputBucketName
: The Amazon S3 bucket where you want your transcript stored. If you want your
* output stored in a sub-folder of this bucket, you must also include OutputKey
.
*
*
* -
*
* Specialty
: This must be PRIMARYCARE
.
*
*
* -
*
* Type
: Choose whether your audio is a conversation or a dictation.
*
*
*
*
*
* This is a convenience which creates an instance of the {@link StartMedicalTranscriptionJobRequest.Builder}
* avoiding the need to create one manually via {@link StartMedicalTranscriptionJobRequest#builder()}
*
*
* @param startMedicalTranscriptionJobRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transcribe.model.StartMedicalTranscriptionJobRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the StartMedicalTranscriptionJob operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - ConflictException A resource already exists with this name. Resource names must be unique within an
* Amazon Web Services account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.StartMedicalTranscriptionJob
* @see AWS API Documentation
*/
default CompletableFuture startMedicalTranscriptionJob(
Consumer startMedicalTranscriptionJobRequest) {
return startMedicalTranscriptionJob(StartMedicalTranscriptionJobRequest.builder()
.applyMutation(startMedicalTranscriptionJobRequest).build());
}
/**
*
* Transcribes the audio from a media file and applies any additional Request Parameters you choose to include in
* your request.
*
*
* To make a StartTranscriptionJob
request, you must first upload your media file into an Amazon S3
* bucket; you can then specify the Amazon S3 location of the file using the Media
parameter.
*
*
* You must include the following parameters in your StartTranscriptionJob
request:
*
*
* -
*
* region
: The Amazon Web Services Region where you are making your request. For a list of Amazon Web
* Services Regions supported with Amazon Transcribe, refer to Amazon Transcribe endpoints and quotas.
*
*
* -
*
* TranscriptionJobName
: A custom name you create for your transcription job that is unique within your
* Amazon Web Services account.
*
*
* -
*
* Media
(MediaFileUri
): The Amazon S3 location of your media file.
*
*
* -
*
* One of LanguageCode
, IdentifyLanguage
, or IdentifyMultipleLanguages
: If
* you know the language of your media file, specify it using the LanguageCode
parameter; you can find
* all valid language codes in the Supported languages table.
* If you do not know the languages spoken in your media, use either IdentifyLanguage
or
* IdentifyMultipleLanguages
and let Amazon Transcribe identify the languages for you.
*
*
*
*
* @param startTranscriptionJobRequest
* @return A Java Future containing the result of the StartTranscriptionJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - ConflictException A resource already exists with this name. Resource names must be unique within an
* Amazon Web Services account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.StartTranscriptionJob
* @see AWS API Documentation
*/
default CompletableFuture startTranscriptionJob(
StartTranscriptionJobRequest startTranscriptionJobRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Transcribes the audio from a media file and applies any additional Request Parameters you choose to include in
* your request.
*
*
* To make a StartTranscriptionJob
request, you must first upload your media file into an Amazon S3
* bucket; you can then specify the Amazon S3 location of the file using the Media
parameter.
*
*
* You must include the following parameters in your StartTranscriptionJob
request:
*
*
* -
*
* region
: The Amazon Web Services Region where you are making your request. For a list of Amazon Web
* Services Regions supported with Amazon Transcribe, refer to Amazon Transcribe endpoints and quotas.
*
*
* -
*
* TranscriptionJobName
: A custom name you create for your transcription job that is unique within your
* Amazon Web Services account.
*
*
* -
*
* Media
(MediaFileUri
): The Amazon S3 location of your media file.
*
*
* -
*
* One of LanguageCode
, IdentifyLanguage
, or IdentifyMultipleLanguages
: If
* you know the language of your media file, specify it using the LanguageCode
parameter; you can find
* all valid language codes in the Supported languages table.
* If you do not know the languages spoken in your media, use either IdentifyLanguage
or
* IdentifyMultipleLanguages
and let Amazon Transcribe identify the languages for you.
*
*
*
*
*
* This is a convenience which creates an instance of the {@link StartTranscriptionJobRequest.Builder} avoiding the
* need to create one manually via {@link StartTranscriptionJobRequest#builder()}
*
*
* @param startTranscriptionJobRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transcribe.model.StartTranscriptionJobRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the StartTranscriptionJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - ConflictException A resource already exists with this name. Resource names must be unique within an
* Amazon Web Services account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.StartTranscriptionJob
* @see AWS API Documentation
*/
default CompletableFuture startTranscriptionJob(
Consumer startTranscriptionJobRequest) {
return startTranscriptionJob(StartTranscriptionJobRequest.builder().applyMutation(startTranscriptionJobRequest).build());
}
/**
*
* Adds one or more custom tags, each in the form of a key:value pair, to the specified resource.
*
*
* To learn more about using tags with Amazon Transcribe, refer to Tagging resources.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - ConflictException A resource already exists with this name. Resource names must be unique within an
* Amazon Web Services account.
* - NotFoundException We can't find the requested resource. Check that the specified name is correct and
* try your request again.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.TagResource
* @see AWS API
* Documentation
*/
default CompletableFuture tagResource(TagResourceRequest tagResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Adds one or more custom tags, each in the form of a key:value pair, to the specified resource.
*
*
* To learn more about using tags with Amazon Transcribe, refer to Tagging resources.
*
*
*
* This is a convenience which creates an instance of the {@link TagResourceRequest.Builder} avoiding the need to
* create one manually via {@link TagResourceRequest#builder()}
*
*
* @param tagResourceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transcribe.model.TagResourceRequest.Builder} to create a request.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - ConflictException A resource already exists with this name. Resource names must be unique within an
* Amazon Web Services account.
* - NotFoundException We can't find the requested resource. Check that the specified name is correct and
* try your request again.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.TagResource
* @see AWS API
* Documentation
*/
default CompletableFuture tagResource(Consumer tagResourceRequest) {
return tagResource(TagResourceRequest.builder().applyMutation(tagResourceRequest).build());
}
/**
*
* Removes the specified tags from the specified Amazon Transcribe resource.
*
*
* If you include UntagResource
in your request, you must also include ResourceArn
and
* TagKeys
.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - ConflictException A resource already exists with this name. Resource names must be unique within an
* Amazon Web Services account.
* - NotFoundException We can't find the requested resource. Check that the specified name is correct and
* try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.UntagResource
* @see AWS API
* Documentation
*/
default CompletableFuture untagResource(UntagResourceRequest untagResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Removes the specified tags from the specified Amazon Transcribe resource.
*
*
* If you include UntagResource
in your request, you must also include ResourceArn
and
* TagKeys
.
*
*
*
* This is a convenience which creates an instance of the {@link UntagResourceRequest.Builder} avoiding the need to
* create one manually via {@link UntagResourceRequest#builder()}
*
*
* @param untagResourceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transcribe.model.UntagResourceRequest.Builder} to create a request.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - ConflictException A resource already exists with this name. Resource names must be unique within an
* Amazon Web Services account.
* - NotFoundException We can't find the requested resource. Check that the specified name is correct and
* try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.UntagResource
* @see AWS API
* Documentation
*/
default CompletableFuture untagResource(Consumer untagResourceRequest) {
return untagResource(UntagResourceRequest.builder().applyMutation(untagResourceRequest).build());
}
/**
*
* Updates the specified Call Analytics category with new rules. Note that the
* UpdateCallAnalyticsCategory
operation overwrites all existing rules contained in the specified
* category. You cannot append additional rules onto an existing category.
*
*
* To create a new category, see .
*
*
* @param updateCallAnalyticsCategoryRequest
* @return A Java Future containing the result of the UpdateCallAnalyticsCategory operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - NotFoundException We can't find the requested resource. Check that the specified name is correct and
* try your request again.
* - ConflictException A resource already exists with this name. Resource names must be unique within an
* Amazon Web Services account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.UpdateCallAnalyticsCategory
* @see AWS API Documentation
*/
default CompletableFuture updateCallAnalyticsCategory(
UpdateCallAnalyticsCategoryRequest updateCallAnalyticsCategoryRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates the specified Call Analytics category with new rules. Note that the
* UpdateCallAnalyticsCategory
operation overwrites all existing rules contained in the specified
* category. You cannot append additional rules onto an existing category.
*
*
* To create a new category, see .
*
*
*
* This is a convenience which creates an instance of the {@link UpdateCallAnalyticsCategoryRequest.Builder}
* avoiding the need to create one manually via {@link UpdateCallAnalyticsCategoryRequest#builder()}
*
*
* @param updateCallAnalyticsCategoryRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transcribe.model.UpdateCallAnalyticsCategoryRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the UpdateCallAnalyticsCategory operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - NotFoundException We can't find the requested resource. Check that the specified name is correct and
* try your request again.
* - ConflictException A resource already exists with this name. Resource names must be unique within an
* Amazon Web Services account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.UpdateCallAnalyticsCategory
* @see AWS API Documentation
*/
default CompletableFuture updateCallAnalyticsCategory(
Consumer updateCallAnalyticsCategoryRequest) {
return updateCallAnalyticsCategory(UpdateCallAnalyticsCategoryRequest.builder()
.applyMutation(updateCallAnalyticsCategoryRequest).build());
}
/**
*
* Updates an existing custom medical vocabulary with new values. This operation overwrites all existing information
* with your new values; you cannot append new terms onto an existing custom vocabulary.
*
*
* @param updateMedicalVocabularyRequest
* @return A Java Future containing the result of the UpdateMedicalVocabulary operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - NotFoundException We can't find the requested resource. Check that the specified name is correct and
* try your request again.
* - ConflictException A resource already exists with this name. Resource names must be unique within an
* Amazon Web Services account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.UpdateMedicalVocabulary
* @see AWS API Documentation
*/
default CompletableFuture updateMedicalVocabulary(
UpdateMedicalVocabularyRequest updateMedicalVocabularyRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates an existing custom medical vocabulary with new values. This operation overwrites all existing information
* with your new values; you cannot append new terms onto an existing custom vocabulary.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateMedicalVocabularyRequest.Builder} avoiding
* the need to create one manually via {@link UpdateMedicalVocabularyRequest#builder()}
*
*
* @param updateMedicalVocabularyRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transcribe.model.UpdateMedicalVocabularyRequest.Builder} to create
* a request.
* @return A Java Future containing the result of the UpdateMedicalVocabulary operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - NotFoundException We can't find the requested resource. Check that the specified name is correct and
* try your request again.
* - ConflictException A resource already exists with this name. Resource names must be unique within an
* Amazon Web Services account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.UpdateMedicalVocabulary
* @see AWS API Documentation
*/
default CompletableFuture updateMedicalVocabulary(
Consumer updateMedicalVocabularyRequest) {
return updateMedicalVocabulary(UpdateMedicalVocabularyRequest.builder().applyMutation(updateMedicalVocabularyRequest)
.build());
}
/**
*
* Updates an existing custom vocabulary with new values. This operation overwrites all existing information with
* your new values; you cannot append new terms onto an existing custom vocabulary.
*
*
* @param updateVocabularyRequest
* @return A Java Future containing the result of the UpdateVocabulary operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - NotFoundException We can't find the requested resource. Check that the specified name is correct and
* try your request again.
* - ConflictException A resource already exists with this name. Resource names must be unique within an
* Amazon Web Services account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.UpdateVocabulary
* @see AWS
* API Documentation
*/
default CompletableFuture updateVocabulary(UpdateVocabularyRequest updateVocabularyRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates an existing custom vocabulary with new values. This operation overwrites all existing information with
* your new values; you cannot append new terms onto an existing custom vocabulary.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateVocabularyRequest.Builder} avoiding the need
* to create one manually via {@link UpdateVocabularyRequest#builder()}
*
*
* @param updateVocabularyRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transcribe.model.UpdateVocabularyRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the UpdateVocabulary operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - NotFoundException We can't find the requested resource. Check that the specified name is correct and
* try your request again.
* - ConflictException A resource already exists with this name. Resource names must be unique within an
* Amazon Web Services account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.UpdateVocabulary
* @see AWS
* API Documentation
*/
default CompletableFuture updateVocabulary(
Consumer updateVocabularyRequest) {
return updateVocabulary(UpdateVocabularyRequest.builder().applyMutation(updateVocabularyRequest).build());
}
/**
*
* Updates an existing custom vocabulary filter with a new list of words. The new list you provide overwrites all
* previous entries; you cannot append new terms onto an existing custom vocabulary filter.
*
*
* @param updateVocabularyFilterRequest
* @return A Java Future containing the result of the UpdateVocabularyFilter operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - NotFoundException We can't find the requested resource. Check that the specified name is correct and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.UpdateVocabularyFilter
* @see AWS API Documentation
*/
default CompletableFuture updateVocabularyFilter(
UpdateVocabularyFilterRequest updateVocabularyFilterRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates an existing custom vocabulary filter with a new list of words. The new list you provide overwrites all
* previous entries; you cannot append new terms onto an existing custom vocabulary filter.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateVocabularyFilterRequest.Builder} avoiding the
* need to create one manually via {@link UpdateVocabularyFilterRequest#builder()}
*
*
* @param updateVocabularyFilterRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transcribe.model.UpdateVocabularyFilterRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the UpdateVocabularyFilter operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - NotFoundException We can't find the requested resource. Check that the specified name is correct and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.UpdateVocabularyFilter
* @see AWS API Documentation
*/
default CompletableFuture updateVocabularyFilter(
Consumer updateVocabularyFilterRequest) {
return updateVocabularyFilter(UpdateVocabularyFilterRequest.builder().applyMutation(updateVocabularyFilterRequest)
.build());
}
@Override
default TranscribeServiceClientConfiguration serviceClientConfiguration() {
throw new UnsupportedOperationException();
}
/**
* Create a {@link TranscribeAsyncClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static TranscribeAsyncClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link TranscribeAsyncClient}.
*/
static TranscribeAsyncClientBuilder builder() {
return new DefaultTranscribeAsyncClientBuilder();
}
}