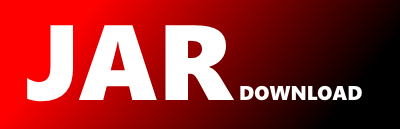
software.amazon.awssdk.services.transcribe.model.CreateVocabularyResponse Maven / Gradle / Ivy
Show all versions of transcribe Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.transcribe.model;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class CreateVocabularyResponse extends TranscribeResponse implements
ToCopyableBuilder {
private static final SdkField VOCABULARY_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("VocabularyName").getter(getter(CreateVocabularyResponse::vocabularyName))
.setter(setter(Builder::vocabularyName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("VocabularyName").build()).build();
private static final SdkField LANGUAGE_CODE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("LanguageCode").getter(getter(CreateVocabularyResponse::languageCodeAsString))
.setter(setter(Builder::languageCode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LanguageCode").build()).build();
private static final SdkField VOCABULARY_STATE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("VocabularyState").getter(getter(CreateVocabularyResponse::vocabularyStateAsString))
.setter(setter(Builder::vocabularyState))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("VocabularyState").build()).build();
private static final SdkField LAST_MODIFIED_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("LastModifiedTime").getter(getter(CreateVocabularyResponse::lastModifiedTime))
.setter(setter(Builder::lastModifiedTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LastModifiedTime").build()).build();
private static final SdkField FAILURE_REASON_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("FailureReason").getter(getter(CreateVocabularyResponse::failureReason))
.setter(setter(Builder::failureReason))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("FailureReason").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(VOCABULARY_NAME_FIELD,
LANGUAGE_CODE_FIELD, VOCABULARY_STATE_FIELD, LAST_MODIFIED_TIME_FIELD, FAILURE_REASON_FIELD));
private final String vocabularyName;
private final String languageCode;
private final String vocabularyState;
private final Instant lastModifiedTime;
private final String failureReason;
private CreateVocabularyResponse(BuilderImpl builder) {
super(builder);
this.vocabularyName = builder.vocabularyName;
this.languageCode = builder.languageCode;
this.vocabularyState = builder.vocabularyState;
this.lastModifiedTime = builder.lastModifiedTime;
this.failureReason = builder.failureReason;
}
/**
*
* The name you chose for your custom vocabulary.
*
*
* @return The name you chose for your custom vocabulary.
*/
public final String vocabularyName() {
return vocabularyName;
}
/**
*
* The language code you selected for your custom vocabulary.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #languageCode} will
* return {@link LanguageCode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #languageCodeAsString}.
*
*
* @return The language code you selected for your custom vocabulary.
* @see LanguageCode
*/
public final LanguageCode languageCode() {
return LanguageCode.fromValue(languageCode);
}
/**
*
* The language code you selected for your custom vocabulary.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #languageCode} will
* return {@link LanguageCode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #languageCodeAsString}.
*
*
* @return The language code you selected for your custom vocabulary.
* @see LanguageCode
*/
public final String languageCodeAsString() {
return languageCode;
}
/**
*
* The processing state of your custom vocabulary. If the state is READY
, you can use the custom
* vocabulary in a StartTranscriptionJob
request.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #vocabularyState}
* will return {@link VocabularyState#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #vocabularyStateAsString}.
*
*
* @return The processing state of your custom vocabulary. If the state is READY
, you can use the
* custom vocabulary in a StartTranscriptionJob
request.
* @see VocabularyState
*/
public final VocabularyState vocabularyState() {
return VocabularyState.fromValue(vocabularyState);
}
/**
*
* The processing state of your custom vocabulary. If the state is READY
, you can use the custom
* vocabulary in a StartTranscriptionJob
request.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #vocabularyState}
* will return {@link VocabularyState#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #vocabularyStateAsString}.
*
*
* @return The processing state of your custom vocabulary. If the state is READY
, you can use the
* custom vocabulary in a StartTranscriptionJob
request.
* @see VocabularyState
*/
public final String vocabularyStateAsString() {
return vocabularyState;
}
/**
*
* The date and time you created your custom vocabulary.
*
*
* Timestamps are in the format YYYY-MM-DD'T'HH:MM:SS.SSSSSS-UTC
. For example,
* 2022-05-04T12:32:58.761000-07:00
represents 12:32 PM UTC-7 on May 4, 2022.
*
*
* @return The date and time you created your custom vocabulary.
*
* Timestamps are in the format YYYY-MM-DD'T'HH:MM:SS.SSSSSS-UTC
. For example,
* 2022-05-04T12:32:58.761000-07:00
represents 12:32 PM UTC-7 on May 4, 2022.
*/
public final Instant lastModifiedTime() {
return lastModifiedTime;
}
/**
*
* If VocabularyState
is FAILED
, FailureReason
contains information about why
* the custom vocabulary request failed. See also: Common Errors.
*
*
* @return If VocabularyState
is FAILED
, FailureReason
contains information
* about why the custom vocabulary request failed. See also: Common Errors.
*/
public final String failureReason() {
return failureReason;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(vocabularyName());
hashCode = 31 * hashCode + Objects.hashCode(languageCodeAsString());
hashCode = 31 * hashCode + Objects.hashCode(vocabularyStateAsString());
hashCode = 31 * hashCode + Objects.hashCode(lastModifiedTime());
hashCode = 31 * hashCode + Objects.hashCode(failureReason());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CreateVocabularyResponse)) {
return false;
}
CreateVocabularyResponse other = (CreateVocabularyResponse) obj;
return Objects.equals(vocabularyName(), other.vocabularyName())
&& Objects.equals(languageCodeAsString(), other.languageCodeAsString())
&& Objects.equals(vocabularyStateAsString(), other.vocabularyStateAsString())
&& Objects.equals(lastModifiedTime(), other.lastModifiedTime())
&& Objects.equals(failureReason(), other.failureReason());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("CreateVocabularyResponse").add("VocabularyName", vocabularyName())
.add("LanguageCode", languageCodeAsString()).add("VocabularyState", vocabularyStateAsString())
.add("LastModifiedTime", lastModifiedTime()).add("FailureReason", failureReason()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "VocabularyName":
return Optional.ofNullable(clazz.cast(vocabularyName()));
case "LanguageCode":
return Optional.ofNullable(clazz.cast(languageCodeAsString()));
case "VocabularyState":
return Optional.ofNullable(clazz.cast(vocabularyStateAsString()));
case "LastModifiedTime":
return Optional.ofNullable(clazz.cast(lastModifiedTime()));
case "FailureReason":
return Optional.ofNullable(clazz.cast(failureReason()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function