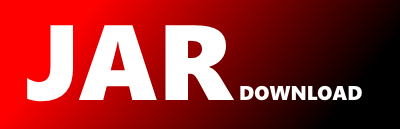
software.amazon.awssdk.services.transcribe.model.StartCallAnalyticsJobRequest Maven / Gradle / Ivy
Show all versions of transcribe Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.transcribe.model;
import java.beans.Transient;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class StartCallAnalyticsJobRequest extends TranscribeRequest implements
ToCopyableBuilder {
private static final SdkField CALL_ANALYTICS_JOB_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("CallAnalyticsJobName").getter(getter(StartCallAnalyticsJobRequest::callAnalyticsJobName))
.setter(setter(Builder::callAnalyticsJobName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CallAnalyticsJobName").build())
.build();
private static final SdkField MEDIA_FIELD = SdkField. builder(MarshallingType.SDK_POJO).memberName("Media")
.getter(getter(StartCallAnalyticsJobRequest::media)).setter(setter(Builder::media)).constructor(Media::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Media").build()).build();
private static final SdkField OUTPUT_LOCATION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("OutputLocation").getter(getter(StartCallAnalyticsJobRequest::outputLocation))
.setter(setter(Builder::outputLocation))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OutputLocation").build()).build();
private static final SdkField OUTPUT_ENCRYPTION_KMS_KEY_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("OutputEncryptionKMSKeyId").getter(getter(StartCallAnalyticsJobRequest::outputEncryptionKMSKeyId))
.setter(setter(Builder::outputEncryptionKMSKeyId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OutputEncryptionKMSKeyId").build())
.build();
private static final SdkField DATA_ACCESS_ROLE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("DataAccessRoleArn").getter(getter(StartCallAnalyticsJobRequest::dataAccessRoleArn))
.setter(setter(Builder::dataAccessRoleArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DataAccessRoleArn").build()).build();
private static final SdkField SETTINGS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("Settings")
.getter(getter(StartCallAnalyticsJobRequest::settings)).setter(setter(Builder::settings))
.constructor(CallAnalyticsJobSettings::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Settings").build()).build();
private static final SdkField> CHANNEL_DEFINITIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("ChannelDefinitions")
.getter(getter(StartCallAnalyticsJobRequest::channelDefinitions))
.setter(setter(Builder::channelDefinitions))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ChannelDefinitions").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(ChannelDefinition::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(CALL_ANALYTICS_JOB_NAME_FIELD,
MEDIA_FIELD, OUTPUT_LOCATION_FIELD, OUTPUT_ENCRYPTION_KMS_KEY_ID_FIELD, DATA_ACCESS_ROLE_ARN_FIELD, SETTINGS_FIELD,
CHANNEL_DEFINITIONS_FIELD));
private final String callAnalyticsJobName;
private final Media media;
private final String outputLocation;
private final String outputEncryptionKMSKeyId;
private final String dataAccessRoleArn;
private final CallAnalyticsJobSettings settings;
private final List channelDefinitions;
private StartCallAnalyticsJobRequest(BuilderImpl builder) {
super(builder);
this.callAnalyticsJobName = builder.callAnalyticsJobName;
this.media = builder.media;
this.outputLocation = builder.outputLocation;
this.outputEncryptionKMSKeyId = builder.outputEncryptionKMSKeyId;
this.dataAccessRoleArn = builder.dataAccessRoleArn;
this.settings = builder.settings;
this.channelDefinitions = builder.channelDefinitions;
}
/**
*
* A unique name, chosen by you, for your Call Analytics job.
*
*
* This name is case sensitive, cannot contain spaces, and must be unique within an Amazon Web Services account. If
* you try to create a new job with the same name as an existing job, you get a ConflictException
* error.
*
*
* @return A unique name, chosen by you, for your Call Analytics job.
*
* This name is case sensitive, cannot contain spaces, and must be unique within an Amazon Web Services
* account. If you try to create a new job with the same name as an existing job, you get a
* ConflictException
error.
*/
public final String callAnalyticsJobName() {
return callAnalyticsJobName;
}
/**
*
* Describes the Amazon S3 location of the media file you want to use in your Call Analytics request.
*
*
* @return Describes the Amazon S3 location of the media file you want to use in your Call Analytics request.
*/
public final Media media() {
return media;
}
/**
*
* The Amazon S3 location where you want your Call Analytics transcription output stored. You can use any of the
* following formats to specify the output location:
*
*
* -
*
* s3://DOC-EXAMPLE-BUCKET
*
*
* -
*
* s3://DOC-EXAMPLE-BUCKET/my-output-folder/
*
*
* -
*
* s3://DOC-EXAMPLE-BUCKET/my-output-folder/my-call-analytics-job.json
*
*
*
*
* Unless you specify a file name (option 3), the name of your output file has a default value that matches the name
* you specified for your transcription job using the CallAnalyticsJobName
parameter.
*
*
* You can specify a KMS key to encrypt your output using the OutputEncryptionKMSKeyId
parameter. If
* you do not specify a KMS key, Amazon Transcribe uses the default Amazon S3 key for server-side encryption.
*
*
* If you do not specify OutputLocation
, your transcript is placed in a service-managed Amazon S3
* bucket and you are provided with a URI to access your transcript.
*
*
* @return The Amazon S3 location where you want your Call Analytics transcription output stored. You can use any of
* the following formats to specify the output location:
*
* -
*
* s3://DOC-EXAMPLE-BUCKET
*
*
* -
*
* s3://DOC-EXAMPLE-BUCKET/my-output-folder/
*
*
* -
*
* s3://DOC-EXAMPLE-BUCKET/my-output-folder/my-call-analytics-job.json
*
*
*
*
* Unless you specify a file name (option 3), the name of your output file has a default value that matches
* the name you specified for your transcription job using the CallAnalyticsJobName
parameter.
*
*
* You can specify a KMS key to encrypt your output using the OutputEncryptionKMSKeyId
* parameter. If you do not specify a KMS key, Amazon Transcribe uses the default Amazon S3 key for
* server-side encryption.
*
*
* If you do not specify OutputLocation
, your transcript is placed in a service-managed Amazon
* S3 bucket and you are provided with a URI to access your transcript.
*/
public final String outputLocation() {
return outputLocation;
}
/**
*
* The KMS key you want to use to encrypt your Call Analytics output.
*
*
* If using a key located in the current Amazon Web Services account, you can specify your KMS key in one of
* four ways:
*
*
* -
*
* Use the KMS key ID itself. For example, 1234abcd-12ab-34cd-56ef-1234567890ab
.
*
*
* -
*
* Use an alias for the KMS key ID. For example, alias/ExampleAlias
.
*
*
* -
*
* Use the Amazon Resource Name (ARN) for the KMS key ID. For example,
* arn:aws:kms:region:account-ID:key/1234abcd-12ab-34cd-56ef-1234567890ab
.
*
*
* -
*
* Use the ARN for the KMS key alias. For example, arn:aws:kms:region:account-ID:alias/ExampleAlias
.
*
*
*
*
* If using a key located in a different Amazon Web Services account than the current Amazon Web Services
* account, you can specify your KMS key in one of two ways:
*
*
* -
*
* Use the ARN for the KMS key ID. For example,
* arn:aws:kms:region:account-ID:key/1234abcd-12ab-34cd-56ef-1234567890ab
.
*
*
* -
*
* Use the ARN for the KMS key alias. For example, arn:aws:kms:region:account-ID:alias/ExampleAlias
.
*
*
*
*
* If you do not specify an encryption key, your output is encrypted with the default Amazon S3 key (SSE-S3).
*
*
* If you specify a KMS key to encrypt your output, you must also specify an output location using the
* OutputLocation
parameter.
*
*
* Note that the role making the request must have permission to use the specified KMS key.
*
*
* @return The KMS key you want to use to encrypt your Call Analytics output.
*
* If using a key located in the current Amazon Web Services account, you can specify your KMS key in
* one of four ways:
*
*
* -
*
* Use the KMS key ID itself. For example, 1234abcd-12ab-34cd-56ef-1234567890ab
.
*
*
* -
*
* Use an alias for the KMS key ID. For example, alias/ExampleAlias
.
*
*
* -
*
* Use the Amazon Resource Name (ARN) for the KMS key ID. For example,
* arn:aws:kms:region:account-ID:key/1234abcd-12ab-34cd-56ef-1234567890ab
.
*
*
* -
*
* Use the ARN for the KMS key alias. For example,
* arn:aws:kms:region:account-ID:alias/ExampleAlias
.
*
*
*
*
* If using a key located in a different Amazon Web Services account than the current Amazon Web
* Services account, you can specify your KMS key in one of two ways:
*
*
* -
*
* Use the ARN for the KMS key ID. For example,
* arn:aws:kms:region:account-ID:key/1234abcd-12ab-34cd-56ef-1234567890ab
.
*
*
* -
*
* Use the ARN for the KMS key alias. For example,
* arn:aws:kms:region:account-ID:alias/ExampleAlias
.
*
*
*
*
* If you do not specify an encryption key, your output is encrypted with the default Amazon S3 key
* (SSE-S3).
*
*
* If you specify a KMS key to encrypt your output, you must also specify an output location using the
* OutputLocation
parameter.
*
*
* Note that the role making the request must have permission to use the specified KMS key.
*/
public final String outputEncryptionKMSKeyId() {
return outputEncryptionKMSKeyId;
}
/**
*
* The Amazon Resource Name (ARN) of an IAM role that has permissions to access the Amazon S3 bucket that contains
* your input files. If the role that you specify doesn’t have the appropriate permissions to access the specified
* Amazon S3 location, your request fails.
*
*
* IAM role ARNs have the format arn:partition:iam::account:role/role-name-with-path
. For example:
* arn:aws:iam::111122223333:role/Admin
.
*
*
* For more information, see IAM ARNs.
*
*
* @return The Amazon Resource Name (ARN) of an IAM role that has permissions to access the Amazon S3 bucket that
* contains your input files. If the role that you specify doesn’t have the appropriate permissions to
* access the specified Amazon S3 location, your request fails.
*
* IAM role ARNs have the format arn:partition:iam::account:role/role-name-with-path
. For
* example: arn:aws:iam::111122223333:role/Admin
.
*
*
* For more information, see IAM
* ARNs.
*/
public final String dataAccessRoleArn() {
return dataAccessRoleArn;
}
/**
*
* Specify additional optional settings in your request, including content redaction; allows you to apply custom
* language models, vocabulary filters, and custom vocabularies to your Call Analytics job.
*
*
* @return Specify additional optional settings in your request, including content redaction; allows you to apply
* custom language models, vocabulary filters, and custom vocabularies to your Call Analytics job.
*/
public final CallAnalyticsJobSettings settings() {
return settings;
}
/**
* For responses, this returns true if the service returned a value for the ChannelDefinitions property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasChannelDefinitions() {
return channelDefinitions != null && !(channelDefinitions instanceof SdkAutoConstructList);
}
/**
*
* Makes it possible to specify which speaker is on which channel. For example, if your agent is the first
* participant to speak, you would set ChannelId
to 0
(to indicate the first channel) and
* ParticipantRole
to AGENT
(to indicate that it's the agent speaking).
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasChannelDefinitions} method.
*
*
* @return Makes it possible to specify which speaker is on which channel. For example, if your agent is the first
* participant to speak, you would set ChannelId
to 0
(to indicate the first
* channel) and ParticipantRole
to AGENT
(to indicate that it's the agent
* speaking).
*/
public final List channelDefinitions() {
return channelDefinitions;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(callAnalyticsJobName());
hashCode = 31 * hashCode + Objects.hashCode(media());
hashCode = 31 * hashCode + Objects.hashCode(outputLocation());
hashCode = 31 * hashCode + Objects.hashCode(outputEncryptionKMSKeyId());
hashCode = 31 * hashCode + Objects.hashCode(dataAccessRoleArn());
hashCode = 31 * hashCode + Objects.hashCode(settings());
hashCode = 31 * hashCode + Objects.hashCode(hasChannelDefinitions() ? channelDefinitions() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof StartCallAnalyticsJobRequest)) {
return false;
}
StartCallAnalyticsJobRequest other = (StartCallAnalyticsJobRequest) obj;
return Objects.equals(callAnalyticsJobName(), other.callAnalyticsJobName()) && Objects.equals(media(), other.media())
&& Objects.equals(outputLocation(), other.outputLocation())
&& Objects.equals(outputEncryptionKMSKeyId(), other.outputEncryptionKMSKeyId())
&& Objects.equals(dataAccessRoleArn(), other.dataAccessRoleArn()) && Objects.equals(settings(), other.settings())
&& hasChannelDefinitions() == other.hasChannelDefinitions()
&& Objects.equals(channelDefinitions(), other.channelDefinitions());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("StartCallAnalyticsJobRequest").add("CallAnalyticsJobName", callAnalyticsJobName())
.add("Media", media()).add("OutputLocation", outputLocation())
.add("OutputEncryptionKMSKeyId", outputEncryptionKMSKeyId()).add("DataAccessRoleArn", dataAccessRoleArn())
.add("Settings", settings()).add("ChannelDefinitions", hasChannelDefinitions() ? channelDefinitions() : null)
.build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "CallAnalyticsJobName":
return Optional.ofNullable(clazz.cast(callAnalyticsJobName()));
case "Media":
return Optional.ofNullable(clazz.cast(media()));
case "OutputLocation":
return Optional.ofNullable(clazz.cast(outputLocation()));
case "OutputEncryptionKMSKeyId":
return Optional.ofNullable(clazz.cast(outputEncryptionKMSKeyId()));
case "DataAccessRoleArn":
return Optional.ofNullable(clazz.cast(dataAccessRoleArn()));
case "Settings":
return Optional.ofNullable(clazz.cast(settings()));
case "ChannelDefinitions":
return Optional.ofNullable(clazz.cast(channelDefinitions()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function