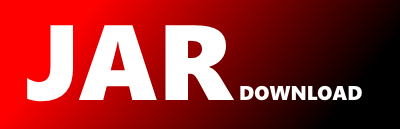
software.amazon.awssdk.services.transcribe.model.LanguageModel Maven / Gradle / Ivy
Show all versions of transcribe Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.transcribe.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Provides information about a custom language model, including:
*
*
* -
*
* The base model name
*
*
* -
*
* When the model was created
*
*
* -
*
* The location of the files used to train the model
*
*
* -
*
* When the model was last modified
*
*
* -
*
* The name you chose for the model
*
*
* -
*
* The model's language
*
*
* -
*
* The model's processing state
*
*
* -
*
* Any available upgrades for the base model
*
*
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class LanguageModel implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField MODEL_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ModelName").getter(getter(LanguageModel::modelName)).setter(setter(Builder::modelName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ModelName").build()).build();
private static final SdkField CREATE_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("CreateTime").getter(getter(LanguageModel::createTime)).setter(setter(Builder::createTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CreateTime").build()).build();
private static final SdkField LAST_MODIFIED_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("LastModifiedTime").getter(getter(LanguageModel::lastModifiedTime))
.setter(setter(Builder::lastModifiedTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LastModifiedTime").build()).build();
private static final SdkField LANGUAGE_CODE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("LanguageCode").getter(getter(LanguageModel::languageCodeAsString)).setter(setter(Builder::languageCode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LanguageCode").build()).build();
private static final SdkField BASE_MODEL_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("BaseModelName").getter(getter(LanguageModel::baseModelNameAsString))
.setter(setter(Builder::baseModelName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("BaseModelName").build()).build();
private static final SdkField MODEL_STATUS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ModelStatus").getter(getter(LanguageModel::modelStatusAsString)).setter(setter(Builder::modelStatus))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ModelStatus").build()).build();
private static final SdkField UPGRADE_AVAILABILITY_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("UpgradeAvailability").getter(getter(LanguageModel::upgradeAvailability))
.setter(setter(Builder::upgradeAvailability))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("UpgradeAvailability").build())
.build();
private static final SdkField FAILURE_REASON_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("FailureReason").getter(getter(LanguageModel::failureReason)).setter(setter(Builder::failureReason))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("FailureReason").build()).build();
private static final SdkField INPUT_DATA_CONFIG_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("InputDataConfig")
.getter(getter(LanguageModel::inputDataConfig)).setter(setter(Builder::inputDataConfig))
.constructor(InputDataConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InputDataConfig").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(MODEL_NAME_FIELD,
CREATE_TIME_FIELD, LAST_MODIFIED_TIME_FIELD, LANGUAGE_CODE_FIELD, BASE_MODEL_NAME_FIELD, MODEL_STATUS_FIELD,
UPGRADE_AVAILABILITY_FIELD, FAILURE_REASON_FIELD, INPUT_DATA_CONFIG_FIELD));
private static final long serialVersionUID = 1L;
private final String modelName;
private final Instant createTime;
private final Instant lastModifiedTime;
private final String languageCode;
private final String baseModelName;
private final String modelStatus;
private final Boolean upgradeAvailability;
private final String failureReason;
private final InputDataConfig inputDataConfig;
private LanguageModel(BuilderImpl builder) {
this.modelName = builder.modelName;
this.createTime = builder.createTime;
this.lastModifiedTime = builder.lastModifiedTime;
this.languageCode = builder.languageCode;
this.baseModelName = builder.baseModelName;
this.modelStatus = builder.modelStatus;
this.upgradeAvailability = builder.upgradeAvailability;
this.failureReason = builder.failureReason;
this.inputDataConfig = builder.inputDataConfig;
}
/**
*
* A unique name, chosen by you, for your custom language model.
*
*
* This name is case sensitive, cannot contain spaces, and must be unique within an Amazon Web Services account.
*
*
* @return A unique name, chosen by you, for your custom language model.
*
* This name is case sensitive, cannot contain spaces, and must be unique within an Amazon Web Services
* account.
*/
public final String modelName() {
return modelName;
}
/**
*
* The date and time the specified custom language model was created.
*
*
* Timestamps are in the format YYYY-MM-DD'T'HH:MM:SS.SSSSSS-UTC
. For example,
* 2022-05-04T12:32:58.761000-07:00
represents 12:32 PM UTC-7 on May 4, 2022.
*
*
* @return The date and time the specified custom language model was created.
*
* Timestamps are in the format YYYY-MM-DD'T'HH:MM:SS.SSSSSS-UTC
. For example,
* 2022-05-04T12:32:58.761000-07:00
represents 12:32 PM UTC-7 on May 4, 2022.
*/
public final Instant createTime() {
return createTime;
}
/**
*
* The date and time the specified custom language model was last modified.
*
*
* Timestamps are in the format YYYY-MM-DD'T'HH:MM:SS.SSSSSS-UTC
. For example,
* 2022-05-04T12:32:58.761000-07:00
represents 12:32 PM UTC-7 on May 4, 2022.
*
*
* @return The date and time the specified custom language model was last modified.
*
* Timestamps are in the format YYYY-MM-DD'T'HH:MM:SS.SSSSSS-UTC
. For example,
* 2022-05-04T12:32:58.761000-07:00
represents 12:32 PM UTC-7 on May 4, 2022.
*/
public final Instant lastModifiedTime() {
return lastModifiedTime;
}
/**
*
* The language code used to create your custom language model. Each custom language model must contain terms in
* only one language, and the language you select for your custom language model must match the language of your
* training and tuning data.
*
*
* For a list of supported languages and their associated language codes, refer to the Supported languages table.
* Note that US English (en-US
) is the only language supported with Amazon Transcribe Medical.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #languageCode} will
* return {@link CLMLanguageCode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #languageCodeAsString}.
*
*
* @return The language code used to create your custom language model. Each custom language model must contain
* terms in only one language, and the language you select for your custom language model must match the
* language of your training and tuning data.
*
* For a list of supported languages and their associated language codes, refer to the Supported languages
* table. Note that US English (en-US
) is the only language supported with Amazon Transcribe
* Medical.
* @see CLMLanguageCode
*/
public final CLMLanguageCode languageCode() {
return CLMLanguageCode.fromValue(languageCode);
}
/**
*
* The language code used to create your custom language model. Each custom language model must contain terms in
* only one language, and the language you select for your custom language model must match the language of your
* training and tuning data.
*
*
* For a list of supported languages and their associated language codes, refer to the Supported languages table.
* Note that US English (en-US
) is the only language supported with Amazon Transcribe Medical.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #languageCode} will
* return {@link CLMLanguageCode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #languageCodeAsString}.
*
*
* @return The language code used to create your custom language model. Each custom language model must contain
* terms in only one language, and the language you select for your custom language model must match the
* language of your training and tuning data.
*
* For a list of supported languages and their associated language codes, refer to the Supported languages
* table. Note that US English (en-US
) is the only language supported with Amazon Transcribe
* Medical.
* @see CLMLanguageCode
*/
public final String languageCodeAsString() {
return languageCode;
}
/**
*
* The Amazon Transcribe standard language model, or base model, used to create your custom language model.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #baseModelName}
* will return {@link BaseModelName#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #baseModelNameAsString}.
*
*
* @return The Amazon Transcribe standard language model, or base model, used to create your custom language model.
* @see BaseModelName
*/
public final BaseModelName baseModelName() {
return BaseModelName.fromValue(baseModelName);
}
/**
*
* The Amazon Transcribe standard language model, or base model, used to create your custom language model.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #baseModelName}
* will return {@link BaseModelName#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #baseModelNameAsString}.
*
*
* @return The Amazon Transcribe standard language model, or base model, used to create your custom language model.
* @see BaseModelName
*/
public final String baseModelNameAsString() {
return baseModelName;
}
/**
*
* The status of the specified custom language model. When the status displays as COMPLETED
the model
* is ready for use.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #modelStatus} will
* return {@link ModelStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #modelStatusAsString}.
*
*
* @return The status of the specified custom language model. When the status displays as COMPLETED
the
* model is ready for use.
* @see ModelStatus
*/
public final ModelStatus modelStatus() {
return ModelStatus.fromValue(modelStatus);
}
/**
*
* The status of the specified custom language model. When the status displays as COMPLETED
the model
* is ready for use.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #modelStatus} will
* return {@link ModelStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #modelStatusAsString}.
*
*
* @return The status of the specified custom language model. When the status displays as COMPLETED
the
* model is ready for use.
* @see ModelStatus
*/
public final String modelStatusAsString() {
return modelStatus;
}
/**
*
* Shows if a more current base model is available for use with the specified custom language model.
*
*
* If false
, your custom language model is using the most up-to-date base model.
*
*
* If true
, there is a newer base model available than the one your language model is using.
*
*
* Note that to update a base model, you must recreate the custom language model using the new base model. Base
* model upgrades for existing custom language models are not supported.
*
*
* @return Shows if a more current base model is available for use with the specified custom language model.
*
* If false
, your custom language model is using the most up-to-date base model.
*
*
* If true
, there is a newer base model available than the one your language model is using.
*
*
* Note that to update a base model, you must recreate the custom language model using the new base model.
* Base model upgrades for existing custom language models are not supported.
*/
public final Boolean upgradeAvailability() {
return upgradeAvailability;
}
/**
*
* If ModelStatus
is FAILED
, FailureReason
contains information about why the
* custom language model request failed. See also: Common Errors.
*
*
* @return If ModelStatus
is FAILED
, FailureReason
contains information about
* why the custom language model request failed. See also: Common Errors.
*/
public final String failureReason() {
return failureReason;
}
/**
*
* The Amazon S3 location of the input files used to train and tune your custom language model, in addition to the
* data access role ARN (Amazon Resource Name) that has permissions to access these data.
*
*
* @return The Amazon S3 location of the input files used to train and tune your custom language model, in addition
* to the data access role ARN (Amazon Resource Name) that has permissions to access these data.
*/
public final InputDataConfig inputDataConfig() {
return inputDataConfig;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(modelName());
hashCode = 31 * hashCode + Objects.hashCode(createTime());
hashCode = 31 * hashCode + Objects.hashCode(lastModifiedTime());
hashCode = 31 * hashCode + Objects.hashCode(languageCodeAsString());
hashCode = 31 * hashCode + Objects.hashCode(baseModelNameAsString());
hashCode = 31 * hashCode + Objects.hashCode(modelStatusAsString());
hashCode = 31 * hashCode + Objects.hashCode(upgradeAvailability());
hashCode = 31 * hashCode + Objects.hashCode(failureReason());
hashCode = 31 * hashCode + Objects.hashCode(inputDataConfig());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof LanguageModel)) {
return false;
}
LanguageModel other = (LanguageModel) obj;
return Objects.equals(modelName(), other.modelName()) && Objects.equals(createTime(), other.createTime())
&& Objects.equals(lastModifiedTime(), other.lastModifiedTime())
&& Objects.equals(languageCodeAsString(), other.languageCodeAsString())
&& Objects.equals(baseModelNameAsString(), other.baseModelNameAsString())
&& Objects.equals(modelStatusAsString(), other.modelStatusAsString())
&& Objects.equals(upgradeAvailability(), other.upgradeAvailability())
&& Objects.equals(failureReason(), other.failureReason())
&& Objects.equals(inputDataConfig(), other.inputDataConfig());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("LanguageModel").add("ModelName", modelName()).add("CreateTime", createTime())
.add("LastModifiedTime", lastModifiedTime()).add("LanguageCode", languageCodeAsString())
.add("BaseModelName", baseModelNameAsString()).add("ModelStatus", modelStatusAsString())
.add("UpgradeAvailability", upgradeAvailability()).add("FailureReason", failureReason())
.add("InputDataConfig", inputDataConfig()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ModelName":
return Optional.ofNullable(clazz.cast(modelName()));
case "CreateTime":
return Optional.ofNullable(clazz.cast(createTime()));
case "LastModifiedTime":
return Optional.ofNullable(clazz.cast(lastModifiedTime()));
case "LanguageCode":
return Optional.ofNullable(clazz.cast(languageCodeAsString()));
case "BaseModelName":
return Optional.ofNullable(clazz.cast(baseModelNameAsString()));
case "ModelStatus":
return Optional.ofNullable(clazz.cast(modelStatusAsString()));
case "UpgradeAvailability":
return Optional.ofNullable(clazz.cast(upgradeAvailability()));
case "FailureReason":
return Optional.ofNullable(clazz.cast(failureReason()));
case "InputDataConfig":
return Optional.ofNullable(clazz.cast(inputDataConfig()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function