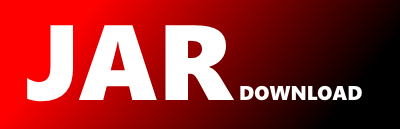
software.amazon.awssdk.services.transcribe.DefaultTranscribeAsyncClient Maven / Gradle / Ivy
Show all versions of transcribe Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.transcribe;
import java.util.Collections;
import java.util.List;
import java.util.concurrent.CompletableFuture;
import java.util.function.Consumer;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.awscore.client.handler.AwsAsyncClientHandler;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.awscore.internal.AwsProtocolMetadata;
import software.amazon.awssdk.awscore.internal.AwsServiceProtocol;
import software.amazon.awssdk.awscore.retry.AwsRetryStrategy;
import software.amazon.awssdk.core.RequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkPlugin;
import software.amazon.awssdk.core.SdkRequest;
import software.amazon.awssdk.core.client.config.ClientOverrideConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientOption;
import software.amazon.awssdk.core.client.handler.AsyncClientHandler;
import software.amazon.awssdk.core.client.handler.ClientExecutionParams;
import software.amazon.awssdk.core.http.HttpResponseHandler;
import software.amazon.awssdk.core.metrics.CoreMetric;
import software.amazon.awssdk.core.retry.RetryMode;
import software.amazon.awssdk.metrics.MetricCollector;
import software.amazon.awssdk.metrics.MetricPublisher;
import software.amazon.awssdk.metrics.NoOpMetricCollector;
import software.amazon.awssdk.protocols.core.ExceptionMetadata;
import software.amazon.awssdk.protocols.json.AwsJsonProtocol;
import software.amazon.awssdk.protocols.json.AwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.BaseAwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.JsonOperationMetadata;
import software.amazon.awssdk.retries.api.RetryStrategy;
import software.amazon.awssdk.services.transcribe.internal.TranscribeServiceClientConfigurationBuilder;
import software.amazon.awssdk.services.transcribe.model.BadRequestException;
import software.amazon.awssdk.services.transcribe.model.ConflictException;
import software.amazon.awssdk.services.transcribe.model.CreateCallAnalyticsCategoryRequest;
import software.amazon.awssdk.services.transcribe.model.CreateCallAnalyticsCategoryResponse;
import software.amazon.awssdk.services.transcribe.model.CreateLanguageModelRequest;
import software.amazon.awssdk.services.transcribe.model.CreateLanguageModelResponse;
import software.amazon.awssdk.services.transcribe.model.CreateMedicalVocabularyRequest;
import software.amazon.awssdk.services.transcribe.model.CreateMedicalVocabularyResponse;
import software.amazon.awssdk.services.transcribe.model.CreateVocabularyFilterRequest;
import software.amazon.awssdk.services.transcribe.model.CreateVocabularyFilterResponse;
import software.amazon.awssdk.services.transcribe.model.CreateVocabularyRequest;
import software.amazon.awssdk.services.transcribe.model.CreateVocabularyResponse;
import software.amazon.awssdk.services.transcribe.model.DeleteCallAnalyticsCategoryRequest;
import software.amazon.awssdk.services.transcribe.model.DeleteCallAnalyticsCategoryResponse;
import software.amazon.awssdk.services.transcribe.model.DeleteCallAnalyticsJobRequest;
import software.amazon.awssdk.services.transcribe.model.DeleteCallAnalyticsJobResponse;
import software.amazon.awssdk.services.transcribe.model.DeleteLanguageModelRequest;
import software.amazon.awssdk.services.transcribe.model.DeleteLanguageModelResponse;
import software.amazon.awssdk.services.transcribe.model.DeleteMedicalScribeJobRequest;
import software.amazon.awssdk.services.transcribe.model.DeleteMedicalScribeJobResponse;
import software.amazon.awssdk.services.transcribe.model.DeleteMedicalTranscriptionJobRequest;
import software.amazon.awssdk.services.transcribe.model.DeleteMedicalTranscriptionJobResponse;
import software.amazon.awssdk.services.transcribe.model.DeleteMedicalVocabularyRequest;
import software.amazon.awssdk.services.transcribe.model.DeleteMedicalVocabularyResponse;
import software.amazon.awssdk.services.transcribe.model.DeleteTranscriptionJobRequest;
import software.amazon.awssdk.services.transcribe.model.DeleteTranscriptionJobResponse;
import software.amazon.awssdk.services.transcribe.model.DeleteVocabularyFilterRequest;
import software.amazon.awssdk.services.transcribe.model.DeleteVocabularyFilterResponse;
import software.amazon.awssdk.services.transcribe.model.DeleteVocabularyRequest;
import software.amazon.awssdk.services.transcribe.model.DeleteVocabularyResponse;
import software.amazon.awssdk.services.transcribe.model.DescribeLanguageModelRequest;
import software.amazon.awssdk.services.transcribe.model.DescribeLanguageModelResponse;
import software.amazon.awssdk.services.transcribe.model.GetCallAnalyticsCategoryRequest;
import software.amazon.awssdk.services.transcribe.model.GetCallAnalyticsCategoryResponse;
import software.amazon.awssdk.services.transcribe.model.GetCallAnalyticsJobRequest;
import software.amazon.awssdk.services.transcribe.model.GetCallAnalyticsJobResponse;
import software.amazon.awssdk.services.transcribe.model.GetMedicalScribeJobRequest;
import software.amazon.awssdk.services.transcribe.model.GetMedicalScribeJobResponse;
import software.amazon.awssdk.services.transcribe.model.GetMedicalTranscriptionJobRequest;
import software.amazon.awssdk.services.transcribe.model.GetMedicalTranscriptionJobResponse;
import software.amazon.awssdk.services.transcribe.model.GetMedicalVocabularyRequest;
import software.amazon.awssdk.services.transcribe.model.GetMedicalVocabularyResponse;
import software.amazon.awssdk.services.transcribe.model.GetTranscriptionJobRequest;
import software.amazon.awssdk.services.transcribe.model.GetTranscriptionJobResponse;
import software.amazon.awssdk.services.transcribe.model.GetVocabularyFilterRequest;
import software.amazon.awssdk.services.transcribe.model.GetVocabularyFilterResponse;
import software.amazon.awssdk.services.transcribe.model.GetVocabularyRequest;
import software.amazon.awssdk.services.transcribe.model.GetVocabularyResponse;
import software.amazon.awssdk.services.transcribe.model.InternalFailureException;
import software.amazon.awssdk.services.transcribe.model.LimitExceededException;
import software.amazon.awssdk.services.transcribe.model.ListCallAnalyticsCategoriesRequest;
import software.amazon.awssdk.services.transcribe.model.ListCallAnalyticsCategoriesResponse;
import software.amazon.awssdk.services.transcribe.model.ListCallAnalyticsJobsRequest;
import software.amazon.awssdk.services.transcribe.model.ListCallAnalyticsJobsResponse;
import software.amazon.awssdk.services.transcribe.model.ListLanguageModelsRequest;
import software.amazon.awssdk.services.transcribe.model.ListLanguageModelsResponse;
import software.amazon.awssdk.services.transcribe.model.ListMedicalScribeJobsRequest;
import software.amazon.awssdk.services.transcribe.model.ListMedicalScribeJobsResponse;
import software.amazon.awssdk.services.transcribe.model.ListMedicalTranscriptionJobsRequest;
import software.amazon.awssdk.services.transcribe.model.ListMedicalTranscriptionJobsResponse;
import software.amazon.awssdk.services.transcribe.model.ListMedicalVocabulariesRequest;
import software.amazon.awssdk.services.transcribe.model.ListMedicalVocabulariesResponse;
import software.amazon.awssdk.services.transcribe.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.transcribe.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.transcribe.model.ListTranscriptionJobsRequest;
import software.amazon.awssdk.services.transcribe.model.ListTranscriptionJobsResponse;
import software.amazon.awssdk.services.transcribe.model.ListVocabulariesRequest;
import software.amazon.awssdk.services.transcribe.model.ListVocabulariesResponse;
import software.amazon.awssdk.services.transcribe.model.ListVocabularyFiltersRequest;
import software.amazon.awssdk.services.transcribe.model.ListVocabularyFiltersResponse;
import software.amazon.awssdk.services.transcribe.model.NotFoundException;
import software.amazon.awssdk.services.transcribe.model.StartCallAnalyticsJobRequest;
import software.amazon.awssdk.services.transcribe.model.StartCallAnalyticsJobResponse;
import software.amazon.awssdk.services.transcribe.model.StartMedicalScribeJobRequest;
import software.amazon.awssdk.services.transcribe.model.StartMedicalScribeJobResponse;
import software.amazon.awssdk.services.transcribe.model.StartMedicalTranscriptionJobRequest;
import software.amazon.awssdk.services.transcribe.model.StartMedicalTranscriptionJobResponse;
import software.amazon.awssdk.services.transcribe.model.StartTranscriptionJobRequest;
import software.amazon.awssdk.services.transcribe.model.StartTranscriptionJobResponse;
import software.amazon.awssdk.services.transcribe.model.TagResourceRequest;
import software.amazon.awssdk.services.transcribe.model.TagResourceResponse;
import software.amazon.awssdk.services.transcribe.model.TranscribeException;
import software.amazon.awssdk.services.transcribe.model.UntagResourceRequest;
import software.amazon.awssdk.services.transcribe.model.UntagResourceResponse;
import software.amazon.awssdk.services.transcribe.model.UpdateCallAnalyticsCategoryRequest;
import software.amazon.awssdk.services.transcribe.model.UpdateCallAnalyticsCategoryResponse;
import software.amazon.awssdk.services.transcribe.model.UpdateMedicalVocabularyRequest;
import software.amazon.awssdk.services.transcribe.model.UpdateMedicalVocabularyResponse;
import software.amazon.awssdk.services.transcribe.model.UpdateVocabularyFilterRequest;
import software.amazon.awssdk.services.transcribe.model.UpdateVocabularyFilterResponse;
import software.amazon.awssdk.services.transcribe.model.UpdateVocabularyRequest;
import software.amazon.awssdk.services.transcribe.model.UpdateVocabularyResponse;
import software.amazon.awssdk.services.transcribe.transform.CreateCallAnalyticsCategoryRequestMarshaller;
import software.amazon.awssdk.services.transcribe.transform.CreateLanguageModelRequestMarshaller;
import software.amazon.awssdk.services.transcribe.transform.CreateMedicalVocabularyRequestMarshaller;
import software.amazon.awssdk.services.transcribe.transform.CreateVocabularyFilterRequestMarshaller;
import software.amazon.awssdk.services.transcribe.transform.CreateVocabularyRequestMarshaller;
import software.amazon.awssdk.services.transcribe.transform.DeleteCallAnalyticsCategoryRequestMarshaller;
import software.amazon.awssdk.services.transcribe.transform.DeleteCallAnalyticsJobRequestMarshaller;
import software.amazon.awssdk.services.transcribe.transform.DeleteLanguageModelRequestMarshaller;
import software.amazon.awssdk.services.transcribe.transform.DeleteMedicalScribeJobRequestMarshaller;
import software.amazon.awssdk.services.transcribe.transform.DeleteMedicalTranscriptionJobRequestMarshaller;
import software.amazon.awssdk.services.transcribe.transform.DeleteMedicalVocabularyRequestMarshaller;
import software.amazon.awssdk.services.transcribe.transform.DeleteTranscriptionJobRequestMarshaller;
import software.amazon.awssdk.services.transcribe.transform.DeleteVocabularyFilterRequestMarshaller;
import software.amazon.awssdk.services.transcribe.transform.DeleteVocabularyRequestMarshaller;
import software.amazon.awssdk.services.transcribe.transform.DescribeLanguageModelRequestMarshaller;
import software.amazon.awssdk.services.transcribe.transform.GetCallAnalyticsCategoryRequestMarshaller;
import software.amazon.awssdk.services.transcribe.transform.GetCallAnalyticsJobRequestMarshaller;
import software.amazon.awssdk.services.transcribe.transform.GetMedicalScribeJobRequestMarshaller;
import software.amazon.awssdk.services.transcribe.transform.GetMedicalTranscriptionJobRequestMarshaller;
import software.amazon.awssdk.services.transcribe.transform.GetMedicalVocabularyRequestMarshaller;
import software.amazon.awssdk.services.transcribe.transform.GetTranscriptionJobRequestMarshaller;
import software.amazon.awssdk.services.transcribe.transform.GetVocabularyFilterRequestMarshaller;
import software.amazon.awssdk.services.transcribe.transform.GetVocabularyRequestMarshaller;
import software.amazon.awssdk.services.transcribe.transform.ListCallAnalyticsCategoriesRequestMarshaller;
import software.amazon.awssdk.services.transcribe.transform.ListCallAnalyticsJobsRequestMarshaller;
import software.amazon.awssdk.services.transcribe.transform.ListLanguageModelsRequestMarshaller;
import software.amazon.awssdk.services.transcribe.transform.ListMedicalScribeJobsRequestMarshaller;
import software.amazon.awssdk.services.transcribe.transform.ListMedicalTranscriptionJobsRequestMarshaller;
import software.amazon.awssdk.services.transcribe.transform.ListMedicalVocabulariesRequestMarshaller;
import software.amazon.awssdk.services.transcribe.transform.ListTagsForResourceRequestMarshaller;
import software.amazon.awssdk.services.transcribe.transform.ListTranscriptionJobsRequestMarshaller;
import software.amazon.awssdk.services.transcribe.transform.ListVocabulariesRequestMarshaller;
import software.amazon.awssdk.services.transcribe.transform.ListVocabularyFiltersRequestMarshaller;
import software.amazon.awssdk.services.transcribe.transform.StartCallAnalyticsJobRequestMarshaller;
import software.amazon.awssdk.services.transcribe.transform.StartMedicalScribeJobRequestMarshaller;
import software.amazon.awssdk.services.transcribe.transform.StartMedicalTranscriptionJobRequestMarshaller;
import software.amazon.awssdk.services.transcribe.transform.StartTranscriptionJobRequestMarshaller;
import software.amazon.awssdk.services.transcribe.transform.TagResourceRequestMarshaller;
import software.amazon.awssdk.services.transcribe.transform.UntagResourceRequestMarshaller;
import software.amazon.awssdk.services.transcribe.transform.UpdateCallAnalyticsCategoryRequestMarshaller;
import software.amazon.awssdk.services.transcribe.transform.UpdateMedicalVocabularyRequestMarshaller;
import software.amazon.awssdk.services.transcribe.transform.UpdateVocabularyFilterRequestMarshaller;
import software.amazon.awssdk.services.transcribe.transform.UpdateVocabularyRequestMarshaller;
import software.amazon.awssdk.utils.CompletableFutureUtils;
/**
* Internal implementation of {@link TranscribeAsyncClient}.
*
* @see TranscribeAsyncClient#builder()
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
final class DefaultTranscribeAsyncClient implements TranscribeAsyncClient {
private static final Logger log = LoggerFactory.getLogger(DefaultTranscribeAsyncClient.class);
private static final AwsProtocolMetadata protocolMetadata = AwsProtocolMetadata.builder()
.serviceProtocol(AwsServiceProtocol.AWS_JSON).build();
private final AsyncClientHandler clientHandler;
private final AwsJsonProtocolFactory protocolFactory;
private final SdkClientConfiguration clientConfiguration;
protected DefaultTranscribeAsyncClient(SdkClientConfiguration clientConfiguration) {
this.clientHandler = new AwsAsyncClientHandler(clientConfiguration);
this.clientConfiguration = clientConfiguration.toBuilder().option(SdkClientOption.SDK_CLIENT, this).build();
this.protocolFactory = init(AwsJsonProtocolFactory.builder()).build();
}
/**
*
* Creates a new Call Analytics category.
*
*
* All categories are automatically applied to your Call Analytics transcriptions. Note that in order to apply
* categories to your transcriptions, you must create them before submitting your transcription request, as
* categories cannot be applied retroactively.
*
*
* When creating a new category, you can use the InputType
parameter to label the category as a
* POST_CALL
or a REAL_TIME
category. POST_CALL
categories can only be
* applied to post-call transcriptions and REAL_TIME
categories can only be applied to real-time
* transcriptions. If you do not include InputType
, your category is created as a
* POST_CALL
category by default.
*
*
* Call Analytics categories are composed of rules. For each category, you must create between 1 and 20 rules. Rules
* can include these parameters: , , , and .
*
*
* To update an existing category, see .
*
*
* To learn more about Call Analytics categories, see Creating categories for
* post-call transcriptions and Creating categories for
* real-time transcriptions.
*
*
* @param createCallAnalyticsCategoryRequest
* @return A Java Future containing the result of the CreateCallAnalyticsCategory operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - ConflictException A resource already exists with this name. Resource names must be unique within an
* Amazon Web Services account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.CreateCallAnalyticsCategory
* @see AWS API Documentation
*/
@Override
public CompletableFuture createCallAnalyticsCategory(
CreateCallAnalyticsCategoryRequest createCallAnalyticsCategoryRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createCallAnalyticsCategoryRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createCallAnalyticsCategoryRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transcribe");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateCallAnalyticsCategory");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateCallAnalyticsCategoryResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateCallAnalyticsCategory").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateCallAnalyticsCategoryRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createCallAnalyticsCategoryRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a new custom language model.
*
*
* When creating a new custom language model, you must specify:
*
*
* -
*
* If you want a Wideband (audio sample rates over 16,000 Hz) or Narrowband (audio sample rates under 16,000 Hz)
* base model
*
*
* -
*
* The location of your training and tuning files (this must be an Amazon S3 URI)
*
*
* -
*
* The language of your model
*
*
* -
*
* A unique name for your model
*
*
*
*
* @param createLanguageModelRequest
* @return A Java Future containing the result of the CreateLanguageModel operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - ConflictException A resource already exists with this name. Resource names must be unique within an
* Amazon Web Services account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.CreateLanguageModel
* @see AWS API Documentation
*/
@Override
public CompletableFuture createLanguageModel(
CreateLanguageModelRequest createLanguageModelRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createLanguageModelRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createLanguageModelRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transcribe");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateLanguageModel");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateLanguageModelResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateLanguageModel").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateLanguageModelRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createLanguageModelRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a new custom medical vocabulary.
*
*
* Before creating a new custom medical vocabulary, you must first upload a text file that contains your vocabulary
* table into an Amazon S3 bucket. Note that this differs from , where you can include a list of terms within your
* request using the Phrases
flag; CreateMedicalVocabulary
does not support the
* Phrases
flag and only accepts vocabularies in table format.
*
*
* Each language has a character set that contains all allowed characters for that specific language. If you use
* unsupported characters, your custom vocabulary request fails. Refer to Character Sets for Custom Vocabularies
* to get the character set for your language.
*
*
* For more information, see Custom vocabularies.
*
*
* @param createMedicalVocabularyRequest
* @return A Java Future containing the result of the CreateMedicalVocabulary operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - ConflictException A resource already exists with this name. Resource names must be unique within an
* Amazon Web Services account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.CreateMedicalVocabulary
* @see AWS API Documentation
*/
@Override
public CompletableFuture createMedicalVocabulary(
CreateMedicalVocabularyRequest createMedicalVocabularyRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createMedicalVocabularyRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createMedicalVocabularyRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transcribe");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateMedicalVocabulary");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateMedicalVocabularyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateMedicalVocabulary").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateMedicalVocabularyRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createMedicalVocabularyRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a new custom vocabulary.
*
*
* When creating a new custom vocabulary, you can either upload a text file that contains your new entries, phrases,
* and terms into an Amazon S3 bucket and include the URI in your request. Or you can include a list of terms
* directly in your request using the Phrases
flag.
*
*
* Each language has a character set that contains all allowed characters for that specific language. If you use
* unsupported characters, your custom vocabulary request fails. Refer to Character Sets for Custom Vocabularies
* to get the character set for your language.
*
*
* For more information, see Custom vocabularies.
*
*
* @param createVocabularyRequest
* @return A Java Future containing the result of the CreateVocabulary operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - ConflictException A resource already exists with this name. Resource names must be unique within an
* Amazon Web Services account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.CreateVocabulary
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture createVocabulary(CreateVocabularyRequest createVocabularyRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createVocabularyRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createVocabularyRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transcribe");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateVocabulary");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateVocabularyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateVocabulary").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateVocabularyRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createVocabularyRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a new custom vocabulary filter.
*
*
* You can use custom vocabulary filters to mask, delete, or flag specific words from your transcript. Custom
* vocabulary filters are commonly used to mask profanity in transcripts.
*
*
* Each language has a character set that contains all allowed characters for that specific language. If you use
* unsupported characters, your custom vocabulary filter request fails. Refer to Character Sets for Custom Vocabularies
* to get the character set for your language.
*
*
* For more information, see Vocabulary filtering.
*
*
* @param createVocabularyFilterRequest
* @return A Java Future containing the result of the CreateVocabularyFilter operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - ConflictException A resource already exists with this name. Resource names must be unique within an
* Amazon Web Services account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.CreateVocabularyFilter
* @see AWS API Documentation
*/
@Override
public CompletableFuture createVocabularyFilter(
CreateVocabularyFilterRequest createVocabularyFilterRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createVocabularyFilterRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createVocabularyFilterRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transcribe");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateVocabularyFilter");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateVocabularyFilterResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateVocabularyFilter").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateVocabularyFilterRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createVocabularyFilterRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes a Call Analytics category. To use this operation, specify the name of the category you want to delete
* using CategoryName
. Category names are case sensitive.
*
*
* @param deleteCallAnalyticsCategoryRequest
* @return A Java Future containing the result of the DeleteCallAnalyticsCategory operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - NotFoundException We can't find the requested resource. Check that the specified name is correct and
* try your request again.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.DeleteCallAnalyticsCategory
* @see AWS API Documentation
*/
@Override
public CompletableFuture deleteCallAnalyticsCategory(
DeleteCallAnalyticsCategoryRequest deleteCallAnalyticsCategoryRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteCallAnalyticsCategoryRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteCallAnalyticsCategoryRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transcribe");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteCallAnalyticsCategory");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteCallAnalyticsCategoryResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteCallAnalyticsCategory").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteCallAnalyticsCategoryRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteCallAnalyticsCategoryRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes a Call Analytics job. To use this operation, specify the name of the job you want to delete using
* CallAnalyticsJobName
. Job names are case sensitive.
*
*
* @param deleteCallAnalyticsJobRequest
* @return A Java Future containing the result of the DeleteCallAnalyticsJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.DeleteCallAnalyticsJob
* @see AWS API Documentation
*/
@Override
public CompletableFuture deleteCallAnalyticsJob(
DeleteCallAnalyticsJobRequest deleteCallAnalyticsJobRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteCallAnalyticsJobRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteCallAnalyticsJobRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transcribe");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteCallAnalyticsJob");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteCallAnalyticsJobResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteCallAnalyticsJob").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteCallAnalyticsJobRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteCallAnalyticsJobRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes a custom language model. To use this operation, specify the name of the language model you want to delete
* using ModelName
. custom language model names are case sensitive.
*
*
* @param deleteLanguageModelRequest
* @return A Java Future containing the result of the DeleteLanguageModel operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.DeleteLanguageModel
* @see AWS API Documentation
*/
@Override
public CompletableFuture deleteLanguageModel(
DeleteLanguageModelRequest deleteLanguageModelRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteLanguageModelRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteLanguageModelRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transcribe");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteLanguageModel");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteLanguageModelResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteLanguageModel").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteLanguageModelRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteLanguageModelRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes a Medical Scribe job. To use this operation, specify the name of the job you want to delete using
* MedicalScribeJobName
. Job names are case sensitive.
*
*
* @param deleteMedicalScribeJobRequest
* @return A Java Future containing the result of the DeleteMedicalScribeJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.DeleteMedicalScribeJob
* @see AWS API Documentation
*/
@Override
public CompletableFuture deleteMedicalScribeJob(
DeleteMedicalScribeJobRequest deleteMedicalScribeJobRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteMedicalScribeJobRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteMedicalScribeJobRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transcribe");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteMedicalScribeJob");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteMedicalScribeJobResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteMedicalScribeJob").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteMedicalScribeJobRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteMedicalScribeJobRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes a medical transcription job. To use this operation, specify the name of the job you want to delete using
* MedicalTranscriptionJobName
. Job names are case sensitive.
*
*
* @param deleteMedicalTranscriptionJobRequest
* @return A Java Future containing the result of the DeleteMedicalTranscriptionJob operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.DeleteMedicalTranscriptionJob
* @see AWS API Documentation
*/
@Override
public CompletableFuture deleteMedicalTranscriptionJob(
DeleteMedicalTranscriptionJobRequest deleteMedicalTranscriptionJobRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteMedicalTranscriptionJobRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
deleteMedicalTranscriptionJobRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transcribe");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteMedicalTranscriptionJob");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteMedicalTranscriptionJobResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteMedicalTranscriptionJob").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteMedicalTranscriptionJobRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteMedicalTranscriptionJobRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes a custom medical vocabulary. To use this operation, specify the name of the custom vocabulary you want to
* delete using VocabularyName
. Custom vocabulary names are case sensitive.
*
*
* @param deleteMedicalVocabularyRequest
* @return A Java Future containing the result of the DeleteMedicalVocabulary operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - NotFoundException We can't find the requested resource. Check that the specified name is correct and
* try your request again.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.DeleteMedicalVocabulary
* @see AWS API Documentation
*/
@Override
public CompletableFuture deleteMedicalVocabulary(
DeleteMedicalVocabularyRequest deleteMedicalVocabularyRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteMedicalVocabularyRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteMedicalVocabularyRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transcribe");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteMedicalVocabulary");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteMedicalVocabularyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteMedicalVocabulary").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteMedicalVocabularyRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteMedicalVocabularyRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes a transcription job. To use this operation, specify the name of the job you want to delete using
* TranscriptionJobName
. Job names are case sensitive.
*
*
* @param deleteTranscriptionJobRequest
* @return A Java Future containing the result of the DeleteTranscriptionJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.DeleteTranscriptionJob
* @see AWS API Documentation
*/
@Override
public CompletableFuture deleteTranscriptionJob(
DeleteTranscriptionJobRequest deleteTranscriptionJobRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteTranscriptionJobRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteTranscriptionJobRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transcribe");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteTranscriptionJob");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteTranscriptionJobResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteTranscriptionJob").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteTranscriptionJobRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteTranscriptionJobRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes a custom vocabulary. To use this operation, specify the name of the custom vocabulary you want to delete
* using VocabularyName
. Custom vocabulary names are case sensitive.
*
*
* @param deleteVocabularyRequest
* @return A Java Future containing the result of the DeleteVocabulary operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - NotFoundException We can't find the requested resource. Check that the specified name is correct and
* try your request again.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.DeleteVocabulary
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture deleteVocabulary(DeleteVocabularyRequest deleteVocabularyRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteVocabularyRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteVocabularyRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transcribe");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteVocabulary");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteVocabularyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteVocabulary").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteVocabularyRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteVocabularyRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes a custom vocabulary filter. To use this operation, specify the name of the custom vocabulary filter you
* want to delete using VocabularyFilterName
. Custom vocabulary filter names are case sensitive.
*
*
* @param deleteVocabularyFilterRequest
* @return A Java Future containing the result of the DeleteVocabularyFilter operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - NotFoundException We can't find the requested resource. Check that the specified name is correct and
* try your request again.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.DeleteVocabularyFilter
* @see AWS API Documentation
*/
@Override
public CompletableFuture deleteVocabularyFilter(
DeleteVocabularyFilterRequest deleteVocabularyFilterRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteVocabularyFilterRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteVocabularyFilterRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transcribe");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteVocabularyFilter");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteVocabularyFilterResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteVocabularyFilter").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteVocabularyFilterRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteVocabularyFilterRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Provides information about the specified custom language model.
*
*
* This operation also shows if the base language model that you used to create your custom language model has been
* updated. If Amazon Transcribe has updated the base model, you can create a new custom language model using the
* updated base model.
*
*
* If you tried to create a new custom language model and the request wasn't successful, you can use
* DescribeLanguageModel
to help identify the reason for this failure.
*
*
* @param describeLanguageModelRequest
* @return A Java Future containing the result of the DescribeLanguageModel operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - NotFoundException We can't find the requested resource. Check that the specified name is correct and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.DescribeLanguageModel
* @see AWS API Documentation
*/
@Override
public CompletableFuture describeLanguageModel(
DescribeLanguageModelRequest describeLanguageModelRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeLanguageModelRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeLanguageModelRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transcribe");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeLanguageModel");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeLanguageModelResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeLanguageModel").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeLanguageModelRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeLanguageModelRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Provides information about the specified Call Analytics category.
*
*
* To get a list of your Call Analytics categories, use the operation.
*
*
* @param getCallAnalyticsCategoryRequest
* @return A Java Future containing the result of the GetCallAnalyticsCategory operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - NotFoundException We can't find the requested resource. Check that the specified name is correct and
* try your request again.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.GetCallAnalyticsCategory
* @see AWS API Documentation
*/
@Override
public CompletableFuture getCallAnalyticsCategory(
GetCallAnalyticsCategoryRequest getCallAnalyticsCategoryRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getCallAnalyticsCategoryRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getCallAnalyticsCategoryRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transcribe");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetCallAnalyticsCategory");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetCallAnalyticsCategoryResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetCallAnalyticsCategory").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetCallAnalyticsCategoryRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getCallAnalyticsCategoryRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Provides information about the specified Call Analytics job.
*
*
* To view the job's status, refer to CallAnalyticsJobStatus
. If the status is COMPLETED
,
* the job is finished. You can find your completed transcript at the URI specified in
* TranscriptFileUri
. If the status is FAILED
, FailureReason
provides details
* on why your transcription job failed.
*
*
* If you enabled personally identifiable information (PII) redaction, the redacted transcript appears at the
* location specified in RedactedTranscriptFileUri
.
*
*
* If you chose to redact the audio in your media file, you can find your redacted media file at the location
* specified in RedactedMediaFileUri
.
*
*
* To get a list of your Call Analytics jobs, use the operation.
*
*
* @param getCallAnalyticsJobRequest
* @return A Java Future containing the result of the GetCallAnalyticsJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - NotFoundException We can't find the requested resource. Check that the specified name is correct and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.GetCallAnalyticsJob
* @see AWS API Documentation
*/
@Override
public CompletableFuture getCallAnalyticsJob(
GetCallAnalyticsJobRequest getCallAnalyticsJobRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getCallAnalyticsJobRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getCallAnalyticsJobRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transcribe");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetCallAnalyticsJob");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetCallAnalyticsJobResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetCallAnalyticsJob").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetCallAnalyticsJobRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getCallAnalyticsJobRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Provides information about the specified Medical Scribe job.
*
*
* To view the status of the specified medical transcription job, check the MedicalScribeJobStatus
* field. If the status is COMPLETED
, the job is finished. You can find the results at the location
* specified in MedicalScribeOutput
. If the status is FAILED
, FailureReason
* provides details on why your Medical Scribe job failed.
*
*
* To get a list of your Medical Scribe jobs, use the operation.
*
*
* @param getMedicalScribeJobRequest
* @return A Java Future containing the result of the GetMedicalScribeJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - NotFoundException We can't find the requested resource. Check that the specified name is correct and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.GetMedicalScribeJob
* @see AWS API Documentation
*/
@Override
public CompletableFuture getMedicalScribeJob(
GetMedicalScribeJobRequest getMedicalScribeJobRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getMedicalScribeJobRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getMedicalScribeJobRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transcribe");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetMedicalScribeJob");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetMedicalScribeJobResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetMedicalScribeJob").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetMedicalScribeJobRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getMedicalScribeJobRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Provides information about the specified medical transcription job.
*
*
* To view the status of the specified medical transcription job, check the TranscriptionJobStatus
* field. If the status is COMPLETED
, the job is finished. You can find the results at the location
* specified in TranscriptFileUri
. If the status is FAILED
, FailureReason
* provides details on why your transcription job failed.
*
*
* To get a list of your medical transcription jobs, use the operation.
*
*
* @param getMedicalTranscriptionJobRequest
* @return A Java Future containing the result of the GetMedicalTranscriptionJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - NotFoundException We can't find the requested resource. Check that the specified name is correct and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.GetMedicalTranscriptionJob
* @see AWS API Documentation
*/
@Override
public CompletableFuture getMedicalTranscriptionJob(
GetMedicalTranscriptionJobRequest getMedicalTranscriptionJobRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getMedicalTranscriptionJobRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getMedicalTranscriptionJobRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transcribe");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetMedicalTranscriptionJob");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetMedicalTranscriptionJobResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetMedicalTranscriptionJob").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetMedicalTranscriptionJobRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getMedicalTranscriptionJobRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Provides information about the specified custom medical vocabulary.
*
*
* To view the status of the specified custom medical vocabulary, check the VocabularyState
field. If
* the status is READY
, your custom vocabulary is available to use. If the status is
* FAILED
, FailureReason
provides details on why your vocabulary failed.
*
*
* To get a list of your custom medical vocabularies, use the operation.
*
*
* @param getMedicalVocabularyRequest
* @return A Java Future containing the result of the GetMedicalVocabulary operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - NotFoundException We can't find the requested resource. Check that the specified name is correct and
* try your request again.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.GetMedicalVocabulary
* @see AWS API Documentation
*/
@Override
public CompletableFuture getMedicalVocabulary(
GetMedicalVocabularyRequest getMedicalVocabularyRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getMedicalVocabularyRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getMedicalVocabularyRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transcribe");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetMedicalVocabulary");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetMedicalVocabularyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetMedicalVocabulary").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetMedicalVocabularyRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getMedicalVocabularyRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Provides information about the specified transcription job.
*
*
* To view the status of the specified transcription job, check the TranscriptionJobStatus
field. If
* the status is COMPLETED
, the job is finished. You can find the results at the location specified in
* TranscriptFileUri
. If the status is FAILED
, FailureReason
provides details
* on why your transcription job failed.
*
*
* If you enabled content redaction, the redacted transcript can be found at the location specified in
* RedactedTranscriptFileUri
.
*
*
* To get a list of your transcription jobs, use the operation.
*
*
* @param getTranscriptionJobRequest
* @return A Java Future containing the result of the GetTranscriptionJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - NotFoundException We can't find the requested resource. Check that the specified name is correct and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.GetTranscriptionJob
* @see AWS API Documentation
*/
@Override
public CompletableFuture getTranscriptionJob(
GetTranscriptionJobRequest getTranscriptionJobRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getTranscriptionJobRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getTranscriptionJobRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transcribe");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetTranscriptionJob");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetTranscriptionJobResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetTranscriptionJob").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetTranscriptionJobRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getTranscriptionJobRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Provides information about the specified custom vocabulary.
*
*
* To view the status of the specified custom vocabulary, check the VocabularyState
field. If the
* status is READY
, your custom vocabulary is available to use. If the status is FAILED
,
* FailureReason
provides details on why your custom vocabulary failed.
*
*
* To get a list of your custom vocabularies, use the operation.
*
*
* @param getVocabularyRequest
* @return A Java Future containing the result of the GetVocabulary operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - NotFoundException We can't find the requested resource. Check that the specified name is correct and
* try your request again.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.GetVocabulary
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture getVocabulary(GetVocabularyRequest getVocabularyRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getVocabularyRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getVocabularyRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transcribe");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetVocabulary");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
GetVocabularyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetVocabulary").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetVocabularyRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getVocabularyRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Provides information about the specified custom vocabulary filter.
*
*
* To get a list of your custom vocabulary filters, use the operation.
*
*
* @param getVocabularyFilterRequest
* @return A Java Future containing the result of the GetVocabularyFilter operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - NotFoundException We can't find the requested resource. Check that the specified name is correct and
* try your request again.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.GetVocabularyFilter
* @see AWS API Documentation
*/
@Override
public CompletableFuture getVocabularyFilter(
GetVocabularyFilterRequest getVocabularyFilterRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getVocabularyFilterRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getVocabularyFilterRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transcribe");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetVocabularyFilter");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetVocabularyFilterResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetVocabularyFilter").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetVocabularyFilterRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getVocabularyFilterRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Provides a list of Call Analytics categories, including all rules that make up each category.
*
*
* To get detailed information about a specific Call Analytics category, use the operation.
*
*
* @param listCallAnalyticsCategoriesRequest
* @return A Java Future containing the result of the ListCallAnalyticsCategories operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.ListCallAnalyticsCategories
* @see AWS API Documentation
*/
@Override
public CompletableFuture listCallAnalyticsCategories(
ListCallAnalyticsCategoriesRequest listCallAnalyticsCategoriesRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listCallAnalyticsCategoriesRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listCallAnalyticsCategoriesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transcribe");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListCallAnalyticsCategories");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListCallAnalyticsCategoriesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListCallAnalyticsCategories").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListCallAnalyticsCategoriesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listCallAnalyticsCategoriesRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Provides a list of Call Analytics jobs that match the specified criteria. If no criteria are specified, all Call
* Analytics jobs are returned.
*
*
* To get detailed information about a specific Call Analytics job, use the operation.
*
*
* @param listCallAnalyticsJobsRequest
* @return A Java Future containing the result of the ListCallAnalyticsJobs operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.ListCallAnalyticsJobs
* @see AWS API Documentation
*/
@Override
public CompletableFuture listCallAnalyticsJobs(
ListCallAnalyticsJobsRequest listCallAnalyticsJobsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listCallAnalyticsJobsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listCallAnalyticsJobsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transcribe");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListCallAnalyticsJobs");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListCallAnalyticsJobsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListCallAnalyticsJobs").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListCallAnalyticsJobsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listCallAnalyticsJobsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Provides a list of custom language models that match the specified criteria. If no criteria are specified, all
* custom language models are returned.
*
*
* To get detailed information about a specific custom language model, use the operation.
*
*
* @param listLanguageModelsRequest
* @return A Java Future containing the result of the ListLanguageModels operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.ListLanguageModels
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture listLanguageModels(ListLanguageModelsRequest listLanguageModelsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listLanguageModelsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listLanguageModelsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transcribe");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListLanguageModels");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListLanguageModelsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListLanguageModels").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListLanguageModelsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listLanguageModelsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Provides a list of Medical Scribe jobs that match the specified criteria. If no criteria are specified, all
* Medical Scribe jobs are returned.
*
*
* To get detailed information about a specific Medical Scribe job, use the operation.
*
*
* @param listMedicalScribeJobsRequest
* @return A Java Future containing the result of the ListMedicalScribeJobs operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.ListMedicalScribeJobs
* @see AWS API Documentation
*/
@Override
public CompletableFuture listMedicalScribeJobs(
ListMedicalScribeJobsRequest listMedicalScribeJobsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listMedicalScribeJobsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listMedicalScribeJobsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transcribe");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListMedicalScribeJobs");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListMedicalScribeJobsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListMedicalScribeJobs").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListMedicalScribeJobsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listMedicalScribeJobsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Provides a list of medical transcription jobs that match the specified criteria. If no criteria are specified,
* all medical transcription jobs are returned.
*
*
* To get detailed information about a specific medical transcription job, use the operation.
*
*
* @param listMedicalTranscriptionJobsRequest
* @return A Java Future containing the result of the ListMedicalTranscriptionJobs operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.ListMedicalTranscriptionJobs
* @see AWS API Documentation
*/
@Override
public CompletableFuture listMedicalTranscriptionJobs(
ListMedicalTranscriptionJobsRequest listMedicalTranscriptionJobsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listMedicalTranscriptionJobsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listMedicalTranscriptionJobsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transcribe");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListMedicalTranscriptionJobs");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListMedicalTranscriptionJobsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListMedicalTranscriptionJobs").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListMedicalTranscriptionJobsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listMedicalTranscriptionJobsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Provides a list of custom medical vocabularies that match the specified criteria. If no criteria are specified,
* all custom medical vocabularies are returned.
*
*
* To get detailed information about a specific custom medical vocabulary, use the operation.
*
*
* @param listMedicalVocabulariesRequest
* @return A Java Future containing the result of the ListMedicalVocabularies operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.ListMedicalVocabularies
* @see AWS API Documentation
*/
@Override
public CompletableFuture listMedicalVocabularies(
ListMedicalVocabulariesRequest listMedicalVocabulariesRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listMedicalVocabulariesRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listMedicalVocabulariesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transcribe");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListMedicalVocabularies");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListMedicalVocabulariesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListMedicalVocabularies").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListMedicalVocabulariesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listMedicalVocabulariesRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Lists all tags associated with the specified transcription job, vocabulary, model, or resource.
*
*
* To learn more about using tags with Amazon Transcribe, refer to Tagging resources.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - NotFoundException We can't find the requested resource. Check that the specified name is correct and
* try your request again.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.ListTagsForResource
* @see AWS API Documentation
*/
@Override
public CompletableFuture listTagsForResource(
ListTagsForResourceRequest listTagsForResourceRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listTagsForResourceRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listTagsForResourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transcribe");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListTagsForResource");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListTagsForResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListTagsForResource").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListTagsForResourceRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listTagsForResourceRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Provides a list of transcription jobs that match the specified criteria. If no criteria are specified, all
* transcription jobs are returned.
*
*
* To get detailed information about a specific transcription job, use the operation.
*
*
* @param listTranscriptionJobsRequest
* @return A Java Future containing the result of the ListTranscriptionJobs operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.ListTranscriptionJobs
* @see AWS API Documentation
*/
@Override
public CompletableFuture listTranscriptionJobs(
ListTranscriptionJobsRequest listTranscriptionJobsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listTranscriptionJobsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listTranscriptionJobsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transcribe");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListTranscriptionJobs");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListTranscriptionJobsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListTranscriptionJobs").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListTranscriptionJobsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listTranscriptionJobsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Provides a list of custom vocabularies that match the specified criteria. If no criteria are specified, all
* custom vocabularies are returned.
*
*
* To get detailed information about a specific custom vocabulary, use the operation.
*
*
* @param listVocabulariesRequest
* @return A Java Future containing the result of the ListVocabularies operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.ListVocabularies
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture listVocabularies(ListVocabulariesRequest listVocabulariesRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listVocabulariesRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listVocabulariesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transcribe");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListVocabularies");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListVocabulariesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListVocabularies").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListVocabulariesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listVocabulariesRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Provides a list of custom vocabulary filters that match the specified criteria. If no criteria are specified, all
* custom vocabularies are returned.
*
*
* To get detailed information about a specific custom vocabulary filter, use the operation.
*
*
* @param listVocabularyFiltersRequest
* @return A Java Future containing the result of the ListVocabularyFilters operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.ListVocabularyFilters
* @see AWS API Documentation
*/
@Override
public CompletableFuture listVocabularyFilters(
ListVocabularyFiltersRequest listVocabularyFiltersRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listVocabularyFiltersRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listVocabularyFiltersRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transcribe");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListVocabularyFilters");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListVocabularyFiltersResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListVocabularyFilters").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListVocabularyFiltersRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listVocabularyFiltersRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Transcribes the audio from a customer service call and applies any additional Request Parameters you choose to
* include in your request.
*
*
* In addition to many standard transcription features, Call Analytics provides you with call characteristics, call
* summarization, speaker sentiment, and optional redaction of your text transcript and your audio file. You can
* also apply custom categories to flag specified conditions. To learn more about these features and insights, refer
* to Analyzing call center audio
* with Call Analytics.
*
*
* If you want to apply categories to your Call Analytics job, you must create them before submitting your job
* request. Categories cannot be retroactively applied to a job. To create a new category, use the operation. To
* learn more about Call Analytics categories, see Creating categories for
* post-call transcriptions and Creating categories for
* real-time transcriptions.
*
*
* To make a StartCallAnalyticsJob
request, you must first upload your media file into an Amazon S3
* bucket; you can then specify the Amazon S3 location of the file using the Media
parameter.
*
*
* Note that job queuing is enabled by default for Call Analytics jobs.
*
*
* You must include the following parameters in your StartCallAnalyticsJob
request:
*
*
* -
*
* region
: The Amazon Web Services Region where you are making your request. For a list of Amazon Web
* Services Regions supported with Amazon Transcribe, refer to Amazon Transcribe endpoints and quotas.
*
*
* -
*
* CallAnalyticsJobName
: A custom name that you create for your transcription job that's unique within
* your Amazon Web Services account.
*
*
* -
*
* DataAccessRoleArn
: The Amazon Resource Name (ARN) of an IAM role that has permissions to access the
* Amazon S3 bucket that contains your input files.
*
*
* -
*
* Media
(MediaFileUri
or RedactedMediaFileUri
): The Amazon S3 location of
* your media file.
*
*
*
*
*
* With Call Analytics, you can redact the audio contained in your media file by including
* RedactedMediaFileUri
, instead of MediaFileUri
, to specify the location of your input
* audio. If you choose to redact your audio, you can find your redacted media at the location specified in the
* RedactedMediaFileUri
field of your response.
*
*
*
* @param startCallAnalyticsJobRequest
* @return A Java Future containing the result of the StartCallAnalyticsJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - ConflictException A resource already exists with this name. Resource names must be unique within an
* Amazon Web Services account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.StartCallAnalyticsJob
* @see AWS API Documentation
*/
@Override
public CompletableFuture startCallAnalyticsJob(
StartCallAnalyticsJobRequest startCallAnalyticsJobRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(startCallAnalyticsJobRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, startCallAnalyticsJobRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transcribe");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "StartCallAnalyticsJob");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, StartCallAnalyticsJobResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("StartCallAnalyticsJob").withProtocolMetadata(protocolMetadata)
.withMarshaller(new StartCallAnalyticsJobRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(startCallAnalyticsJobRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Transcribes patient-clinician conversations and generates clinical notes.
*
*
* Amazon Web Services HealthScribe automatically provides rich conversation transcripts, identifies speaker roles,
* classifies dialogues, extracts medical terms, and generates preliminary clinical notes. To learn more about these
* features, refer to Amazon Web
* Services HealthScribe.
*
*
* To make a StartMedicalScribeJob
request, you must first upload your media file into an Amazon S3
* bucket; you can then specify the Amazon S3 location of the file using the Media
parameter.
*
*
* You must include the following parameters in your StartMedicalTranscriptionJob
request:
*
*
* -
*
* DataAccessRoleArn
: The ARN of an IAM role with the these minimum permissions: read permission on
* input file Amazon S3 bucket specified in Media
, write permission on the Amazon S3 bucket specified
* in OutputBucketName
, and full permissions on the KMS key specified in
* OutputEncryptionKMSKeyId
(if set). The role should also allow transcribe.amazonaws.com
* to assume it.
*
*
* -
*
* Media
(MediaFileUri
): The Amazon S3 location of your media file.
*
*
* -
*
* MedicalScribeJobName
: A custom name you create for your MedicalScribe job that is unique within your
* Amazon Web Services account.
*
*
* -
*
* OutputBucketName
: The Amazon S3 bucket where you want your output files stored.
*
*
* -
*
* Settings
: A MedicalScribeSettings
obect that must set exactly one of
* ShowSpeakerLabels
or ChannelIdentification
to true. If ShowSpeakerLabels
* is true, MaxSpeakerLabels
must also be set.
*
*
* -
*
* ChannelDefinitions
: A MedicalScribeChannelDefinitions
array should be set if and only
* if the ChannelIdentification
value of Settings
is set to true.
*
*
*
*
* @param startMedicalScribeJobRequest
* @return A Java Future containing the result of the StartMedicalScribeJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - ConflictException A resource already exists with this name. Resource names must be unique within an
* Amazon Web Services account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.StartMedicalScribeJob
* @see AWS API Documentation
*/
@Override
public CompletableFuture startMedicalScribeJob(
StartMedicalScribeJobRequest startMedicalScribeJobRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(startMedicalScribeJobRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, startMedicalScribeJobRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transcribe");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "StartMedicalScribeJob");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, StartMedicalScribeJobResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("StartMedicalScribeJob").withProtocolMetadata(protocolMetadata)
.withMarshaller(new StartMedicalScribeJobRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(startMedicalScribeJobRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Transcribes the audio from a medical dictation or conversation and applies any additional Request Parameters you
* choose to include in your request.
*
*
* In addition to many standard transcription features, Amazon Transcribe Medical provides you with a robust medical
* vocabulary and, optionally, content identification, which adds flags to personal health information (PHI). To
* learn more about these features, refer to How Amazon Transcribe Medical
* works.
*
*
* To make a StartMedicalTranscriptionJob
request, you must first upload your media file into an Amazon
* S3 bucket; you can then specify the Amazon S3 location of the file using the Media
parameter.
*
*
* You must include the following parameters in your StartMedicalTranscriptionJob
request:
*
*
* -
*
* region
: The Amazon Web Services Region where you are making your request. For a list of Amazon Web
* Services Regions supported with Amazon Transcribe, refer to Amazon Transcribe endpoints and quotas.
*
*
* -
*
* MedicalTranscriptionJobName
: A custom name you create for your transcription job that is unique
* within your Amazon Web Services account.
*
*
* -
*
* Media
(MediaFileUri
): The Amazon S3 location of your media file.
*
*
* -
*
* LanguageCode
: This must be en-US
.
*
*
* -
*
* OutputBucketName
: The Amazon S3 bucket where you want your transcript stored. If you want your
* output stored in a sub-folder of this bucket, you must also include OutputKey
.
*
*
* -
*
* Specialty
: This must be PRIMARYCARE
.
*
*
* -
*
* Type
: Choose whether your audio is a conversation or a dictation.
*
*
*
*
* @param startMedicalTranscriptionJobRequest
* @return A Java Future containing the result of the StartMedicalTranscriptionJob operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BadRequestException Your request didn't pass one or more validation tests. This can occur when the
* entity you're trying to delete doesn't exist or if it's in a non-terminal state (such as
*
IN PROGRESS
). See the exception message field for more information.
* - LimitExceededException You've either sent too many requests or your input file is too long. Wait
* before retrying your request, or use a smaller file and try your request again.
* - InternalFailureException There was an internal error. Check the error message, correct the issue, and
* try your request again.
* - ConflictException A resource already exists with this name. Resource names must be unique within an
* Amazon Web Services account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranscribeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranscribeAsyncClient.StartMedicalTranscriptionJob
* @see AWS API Documentation
*/
@Override
public CompletableFuture startMedicalTranscriptionJob(
StartMedicalTranscriptionJobRequest startMedicalTranscriptionJobRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(startMedicalTranscriptionJobRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, startMedicalTranscriptionJobRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transcribe");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "StartMedicalTranscriptionJob");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, StartMedicalTranscriptionJobResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams