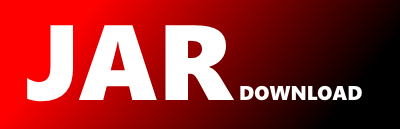
software.amazon.awssdk.services.transcribe.model.TranscriptionJobSummary Maven / Gradle / Ivy
Show all versions of transcribe Show documentation
/*
* Copyright 2014-2019 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.transcribe.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Provides a summary of information about a transcription job. .
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class TranscriptionJobSummary implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField TRANSCRIPTION_JOB_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(TranscriptionJobSummary::transcriptionJobName)).setter(setter(Builder::transcriptionJobName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TranscriptionJobName").build())
.build();
private static final SdkField CREATION_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.getter(getter(TranscriptionJobSummary::creationTime)).setter(setter(Builder::creationTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CreationTime").build()).build();
private static final SdkField COMPLETION_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.getter(getter(TranscriptionJobSummary::completionTime)).setter(setter(Builder::completionTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CompletionTime").build()).build();
private static final SdkField LANGUAGE_CODE_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(TranscriptionJobSummary::languageCodeAsString)).setter(setter(Builder::languageCode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LanguageCode").build()).build();
private static final SdkField TRANSCRIPTION_JOB_STATUS_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(TranscriptionJobSummary::transcriptionJobStatusAsString))
.setter(setter(Builder::transcriptionJobStatus))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TranscriptionJobStatus").build())
.build();
private static final SdkField FAILURE_REASON_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(TranscriptionJobSummary::failureReason)).setter(setter(Builder::failureReason))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("FailureReason").build()).build();
private static final SdkField OUTPUT_LOCATION_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(TranscriptionJobSummary::outputLocationTypeAsString)).setter(setter(Builder::outputLocationType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OutputLocationType").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(TRANSCRIPTION_JOB_NAME_FIELD,
CREATION_TIME_FIELD, COMPLETION_TIME_FIELD, LANGUAGE_CODE_FIELD, TRANSCRIPTION_JOB_STATUS_FIELD,
FAILURE_REASON_FIELD, OUTPUT_LOCATION_TYPE_FIELD));
private static final long serialVersionUID = 1L;
private final String transcriptionJobName;
private final Instant creationTime;
private final Instant completionTime;
private final String languageCode;
private final String transcriptionJobStatus;
private final String failureReason;
private final String outputLocationType;
private TranscriptionJobSummary(BuilderImpl builder) {
this.transcriptionJobName = builder.transcriptionJobName;
this.creationTime = builder.creationTime;
this.completionTime = builder.completionTime;
this.languageCode = builder.languageCode;
this.transcriptionJobStatus = builder.transcriptionJobStatus;
this.failureReason = builder.failureReason;
this.outputLocationType = builder.outputLocationType;
}
/**
*
* The name of the transcription job.
*
*
* @return The name of the transcription job.
*/
public String transcriptionJobName() {
return transcriptionJobName;
}
/**
*
* A timestamp that shows when the job was created.
*
*
* @return A timestamp that shows when the job was created.
*/
public Instant creationTime() {
return creationTime;
}
/**
*
* A timestamp that shows when the job was completed.
*
*
* @return A timestamp that shows when the job was completed.
*/
public Instant completionTime() {
return completionTime;
}
/**
*
* The language code for the input speech.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #languageCode} will
* return {@link LanguageCode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #languageCodeAsString}.
*
*
* @return The language code for the input speech.
* @see LanguageCode
*/
public LanguageCode languageCode() {
return LanguageCode.fromValue(languageCode);
}
/**
*
* The language code for the input speech.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #languageCode} will
* return {@link LanguageCode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #languageCodeAsString}.
*
*
* @return The language code for the input speech.
* @see LanguageCode
*/
public String languageCodeAsString() {
return languageCode;
}
/**
*
* The status of the transcription job. When the status is COMPLETED
, use the
* GetTranscriptionJob
operation to get the results of the transcription.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #transcriptionJobStatus} will return {@link TranscriptionJobStatus#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #transcriptionJobStatusAsString}.
*
*
* @return The status of the transcription job. When the status is COMPLETED
, use the
* GetTranscriptionJob
operation to get the results of the transcription.
* @see TranscriptionJobStatus
*/
public TranscriptionJobStatus transcriptionJobStatus() {
return TranscriptionJobStatus.fromValue(transcriptionJobStatus);
}
/**
*
* The status of the transcription job. When the status is COMPLETED
, use the
* GetTranscriptionJob
operation to get the results of the transcription.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #transcriptionJobStatus} will return {@link TranscriptionJobStatus#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #transcriptionJobStatusAsString}.
*
*
* @return The status of the transcription job. When the status is COMPLETED
, use the
* GetTranscriptionJob
operation to get the results of the transcription.
* @see TranscriptionJobStatus
*/
public String transcriptionJobStatusAsString() {
return transcriptionJobStatus;
}
/**
*
* If the TranscriptionJobStatus
field is FAILED
, a description of the error.
*
*
* @return If the TranscriptionJobStatus
field is FAILED
, a description of the error.
*/
public String failureReason() {
return failureReason;
}
/**
*
* Indicates the location of the output of the transcription job.
*
*
* If the value is CUSTOMER_BUCKET
then the location is the S3 bucket specified in the
* outputBucketName
field when the transcription job was started with the
* StartTranscriptionJob
operation.
*
*
* If the value is SERVICE_BUCKET
then the output is stored by Amazon Transcribe and can be retrieved
* using the URI in the GetTranscriptionJob
response's TranscriptFileUri
field.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #outputLocationType} will return {@link OutputLocationType#UNKNOWN_TO_SDK_VERSION}. The raw value returned
* by the service is available from {@link #outputLocationTypeAsString}.
*
*
* @return Indicates the location of the output of the transcription job.
*
* If the value is CUSTOMER_BUCKET
then the location is the S3 bucket specified in the
* outputBucketName
field when the transcription job was started with the
* StartTranscriptionJob
operation.
*
*
* If the value is SERVICE_BUCKET
then the output is stored by Amazon Transcribe and can be
* retrieved using the URI in the GetTranscriptionJob
response's TranscriptFileUri
* field.
* @see OutputLocationType
*/
public OutputLocationType outputLocationType() {
return OutputLocationType.fromValue(outputLocationType);
}
/**
*
* Indicates the location of the output of the transcription job.
*
*
* If the value is CUSTOMER_BUCKET
then the location is the S3 bucket specified in the
* outputBucketName
field when the transcription job was started with the
* StartTranscriptionJob
operation.
*
*
* If the value is SERVICE_BUCKET
then the output is stored by Amazon Transcribe and can be retrieved
* using the URI in the GetTranscriptionJob
response's TranscriptFileUri
field.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #outputLocationType} will return {@link OutputLocationType#UNKNOWN_TO_SDK_VERSION}. The raw value returned
* by the service is available from {@link #outputLocationTypeAsString}.
*
*
* @return Indicates the location of the output of the transcription job.
*
* If the value is CUSTOMER_BUCKET
then the location is the S3 bucket specified in the
* outputBucketName
field when the transcription job was started with the
* StartTranscriptionJob
operation.
*
*
* If the value is SERVICE_BUCKET
then the output is stored by Amazon Transcribe and can be
* retrieved using the URI in the GetTranscriptionJob
response's TranscriptFileUri
* field.
* @see OutputLocationType
*/
public String outputLocationTypeAsString() {
return outputLocationType;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(transcriptionJobName());
hashCode = 31 * hashCode + Objects.hashCode(creationTime());
hashCode = 31 * hashCode + Objects.hashCode(completionTime());
hashCode = 31 * hashCode + Objects.hashCode(languageCodeAsString());
hashCode = 31 * hashCode + Objects.hashCode(transcriptionJobStatusAsString());
hashCode = 31 * hashCode + Objects.hashCode(failureReason());
hashCode = 31 * hashCode + Objects.hashCode(outputLocationTypeAsString());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof TranscriptionJobSummary)) {
return false;
}
TranscriptionJobSummary other = (TranscriptionJobSummary) obj;
return Objects.equals(transcriptionJobName(), other.transcriptionJobName())
&& Objects.equals(creationTime(), other.creationTime())
&& Objects.equals(completionTime(), other.completionTime())
&& Objects.equals(languageCodeAsString(), other.languageCodeAsString())
&& Objects.equals(transcriptionJobStatusAsString(), other.transcriptionJobStatusAsString())
&& Objects.equals(failureReason(), other.failureReason())
&& Objects.equals(outputLocationTypeAsString(), other.outputLocationTypeAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("TranscriptionJobSummary").add("TranscriptionJobName", transcriptionJobName())
.add("CreationTime", creationTime()).add("CompletionTime", completionTime())
.add("LanguageCode", languageCodeAsString()).add("TranscriptionJobStatus", transcriptionJobStatusAsString())
.add("FailureReason", failureReason()).add("OutputLocationType", outputLocationTypeAsString()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "TranscriptionJobName":
return Optional.ofNullable(clazz.cast(transcriptionJobName()));
case "CreationTime":
return Optional.ofNullable(clazz.cast(creationTime()));
case "CompletionTime":
return Optional.ofNullable(clazz.cast(completionTime()));
case "LanguageCode":
return Optional.ofNullable(clazz.cast(languageCodeAsString()));
case "TranscriptionJobStatus":
return Optional.ofNullable(clazz.cast(transcriptionJobStatusAsString()));
case "FailureReason":
return Optional.ofNullable(clazz.cast(failureReason()));
case "OutputLocationType":
return Optional.ofNullable(clazz.cast(outputLocationTypeAsString()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function
*
* If the value is CUSTOMER_BUCKET
then the location is the S3 bucket specified in the
* outputBucketName
field when the transcription job was started with the
* StartTranscriptionJob
operation.
*
*
* If the value is SERVICE_BUCKET
then the output is stored by Amazon Transcribe and can be
* retrieved using the URI in the GetTranscriptionJob
response's
* TranscriptFileUri
field.
* @see OutputLocationType
* @return Returns a reference to this object so that method calls can be chained together.
* @see OutputLocationType
*/
Builder outputLocationType(String outputLocationType);
/**
*
* Indicates the location of the output of the transcription job.
*
*
* If the value is CUSTOMER_BUCKET
then the location is the S3 bucket specified in the
* outputBucketName
field when the transcription job was started with the
* StartTranscriptionJob
operation.
*
*
* If the value is SERVICE_BUCKET
then the output is stored by Amazon Transcribe and can be
* retrieved using the URI in the GetTranscriptionJob
response's TranscriptFileUri
* field.
*
*
* @param outputLocationType
* Indicates the location of the output of the transcription job.
*
* If the value is CUSTOMER_BUCKET
then the location is the S3 bucket specified in the
* outputBucketName
field when the transcription job was started with the
* StartTranscriptionJob
operation.
*
*
* If the value is SERVICE_BUCKET
then the output is stored by Amazon Transcribe and can be
* retrieved using the URI in the GetTranscriptionJob
response's
* TranscriptFileUri
field.
* @see OutputLocationType
* @return Returns a reference to this object so that method calls can be chained together.
* @see OutputLocationType
*/
Builder outputLocationType(OutputLocationType outputLocationType);
}
static final class BuilderImpl implements Builder {
private String transcriptionJobName;
private Instant creationTime;
private Instant completionTime;
private String languageCode;
private String transcriptionJobStatus;
private String failureReason;
private String outputLocationType;
private BuilderImpl() {
}
private BuilderImpl(TranscriptionJobSummary model) {
transcriptionJobName(model.transcriptionJobName);
creationTime(model.creationTime);
completionTime(model.completionTime);
languageCode(model.languageCode);
transcriptionJobStatus(model.transcriptionJobStatus);
failureReason(model.failureReason);
outputLocationType(model.outputLocationType);
}
public final String getTranscriptionJobName() {
return transcriptionJobName;
}
@Override
public final Builder transcriptionJobName(String transcriptionJobName) {
this.transcriptionJobName = transcriptionJobName;
return this;
}
public final void setTranscriptionJobName(String transcriptionJobName) {
this.transcriptionJobName = transcriptionJobName;
}
public final Instant getCreationTime() {
return creationTime;
}
@Override
public final Builder creationTime(Instant creationTime) {
this.creationTime = creationTime;
return this;
}
public final void setCreationTime(Instant creationTime) {
this.creationTime = creationTime;
}
public final Instant getCompletionTime() {
return completionTime;
}
@Override
public final Builder completionTime(Instant completionTime) {
this.completionTime = completionTime;
return this;
}
public final void setCompletionTime(Instant completionTime) {
this.completionTime = completionTime;
}
public final String getLanguageCodeAsString() {
return languageCode;
}
@Override
public final Builder languageCode(String languageCode) {
this.languageCode = languageCode;
return this;
}
@Override
public final Builder languageCode(LanguageCode languageCode) {
this.languageCode(languageCode.toString());
return this;
}
public final void setLanguageCode(String languageCode) {
this.languageCode = languageCode;
}
public final String getTranscriptionJobStatusAsString() {
return transcriptionJobStatus;
}
@Override
public final Builder transcriptionJobStatus(String transcriptionJobStatus) {
this.transcriptionJobStatus = transcriptionJobStatus;
return this;
}
@Override
public final Builder transcriptionJobStatus(TranscriptionJobStatus transcriptionJobStatus) {
this.transcriptionJobStatus(transcriptionJobStatus.toString());
return this;
}
public final void setTranscriptionJobStatus(String transcriptionJobStatus) {
this.transcriptionJobStatus = transcriptionJobStatus;
}
public final String getFailureReason() {
return failureReason;
}
@Override
public final Builder failureReason(String failureReason) {
this.failureReason = failureReason;
return this;
}
public final void setFailureReason(String failureReason) {
this.failureReason = failureReason;
}
public final String getOutputLocationTypeAsString() {
return outputLocationType;
}
@Override
public final Builder outputLocationType(String outputLocationType) {
this.outputLocationType = outputLocationType;
return this;
}
@Override
public final Builder outputLocationType(OutputLocationType outputLocationType) {
this.outputLocationType(outputLocationType.toString());
return this;
}
public final void setOutputLocationType(String outputLocationType) {
this.outputLocationType = outputLocationType;
}
@Override
public TranscriptionJobSummary build() {
return new TranscriptionJobSummary(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
}
}