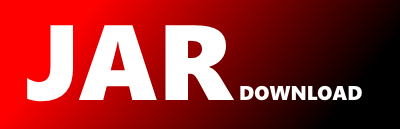
software.amazon.awssdk.services.transcribestreaming.model.StartMedicalStreamTranscriptionRequest Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.transcribestreaming.model;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class StartMedicalStreamTranscriptionRequest extends TranscribeStreamingRequest implements
ToCopyableBuilder {
private static final SdkField LANGUAGE_CODE_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("LanguageCode")
.getter(getter(StartMedicalStreamTranscriptionRequest::languageCodeAsString))
.setter(setter(Builder::languageCode))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("x-amzn-transcribe-language-code")
.build()).build();
private static final SdkField MEDIA_SAMPLE_RATE_HERTZ_FIELD = SdkField
. builder(MarshallingType.INTEGER)
.memberName("MediaSampleRateHertz")
.getter(getter(StartMedicalStreamTranscriptionRequest::mediaSampleRateHertz))
.setter(setter(Builder::mediaSampleRateHertz))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("x-amzn-transcribe-sample-rate")
.build()).build();
private static final SdkField MEDIA_ENCODING_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("MediaEncoding")
.getter(getter(StartMedicalStreamTranscriptionRequest::mediaEncodingAsString))
.setter(setter(Builder::mediaEncoding))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("x-amzn-transcribe-media-encoding")
.build()).build();
private static final SdkField VOCABULARY_NAME_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("VocabularyName")
.getter(getter(StartMedicalStreamTranscriptionRequest::vocabularyName))
.setter(setter(Builder::vocabularyName))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("x-amzn-transcribe-vocabulary-name")
.build()).build();
private static final SdkField SPECIALTY_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("Specialty")
.getter(getter(StartMedicalStreamTranscriptionRequest::specialtyAsString))
.setter(setter(Builder::specialty))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("x-amzn-transcribe-specialty").build())
.build();
private static final SdkField TYPE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Type")
.getter(getter(StartMedicalStreamTranscriptionRequest::typeAsString)).setter(setter(Builder::type))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("x-amzn-transcribe-type").build())
.build();
private static final SdkField SHOW_SPEAKER_LABEL_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("ShowSpeakerLabel")
.getter(getter(StartMedicalStreamTranscriptionRequest::showSpeakerLabel))
.setter(setter(Builder::showSpeakerLabel))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER)
.locationName("x-amzn-transcribe-show-speaker-label").build()).build();
private static final SdkField SESSION_ID_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("SessionId")
.getter(getter(StartMedicalStreamTranscriptionRequest::sessionId))
.setter(setter(Builder::sessionId))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("x-amzn-transcribe-session-id")
.build()).build();
private static final SdkField ENABLE_CHANNEL_IDENTIFICATION_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("EnableChannelIdentification")
.getter(getter(StartMedicalStreamTranscriptionRequest::enableChannelIdentification))
.setter(setter(Builder::enableChannelIdentification))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER)
.locationName("x-amzn-transcribe-enable-channel-identification").build()).build();
private static final SdkField NUMBER_OF_CHANNELS_FIELD = SdkField
. builder(MarshallingType.INTEGER)
.memberName("NumberOfChannels")
.getter(getter(StartMedicalStreamTranscriptionRequest::numberOfChannels))
.setter(setter(Builder::numberOfChannels))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER)
.locationName("x-amzn-transcribe-number-of-channels").build()).build();
private static final SdkField CONTENT_IDENTIFICATION_TYPE_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("ContentIdentificationType")
.getter(getter(StartMedicalStreamTranscriptionRequest::contentIdentificationTypeAsString))
.setter(setter(Builder::contentIdentificationType))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER)
.locationName("x-amzn-transcribe-content-identification-type").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(LANGUAGE_CODE_FIELD,
MEDIA_SAMPLE_RATE_HERTZ_FIELD, MEDIA_ENCODING_FIELD, VOCABULARY_NAME_FIELD, SPECIALTY_FIELD, TYPE_FIELD,
SHOW_SPEAKER_LABEL_FIELD, SESSION_ID_FIELD, ENABLE_CHANNEL_IDENTIFICATION_FIELD, NUMBER_OF_CHANNELS_FIELD,
CONTENT_IDENTIFICATION_TYPE_FIELD));
private static final Map> SDK_NAME_TO_FIELD = memberNameToFieldInitializer();
private final String languageCode;
private final Integer mediaSampleRateHertz;
private final String mediaEncoding;
private final String vocabularyName;
private final String specialty;
private final String type;
private final Boolean showSpeakerLabel;
private final String sessionId;
private final Boolean enableChannelIdentification;
private final Integer numberOfChannels;
private final String contentIdentificationType;
private StartMedicalStreamTranscriptionRequest(BuilderImpl builder) {
super(builder);
this.languageCode = builder.languageCode;
this.mediaSampleRateHertz = builder.mediaSampleRateHertz;
this.mediaEncoding = builder.mediaEncoding;
this.vocabularyName = builder.vocabularyName;
this.specialty = builder.specialty;
this.type = builder.type;
this.showSpeakerLabel = builder.showSpeakerLabel;
this.sessionId = builder.sessionId;
this.enableChannelIdentification = builder.enableChannelIdentification;
this.numberOfChannels = builder.numberOfChannels;
this.contentIdentificationType = builder.contentIdentificationType;
}
/**
*
* Specify the language code that represents the language spoken in your audio.
*
*
*
* Amazon Transcribe Medical only supports US English (en-US
).
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #languageCode} will
* return {@link LanguageCode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #languageCodeAsString}.
*
*
* @return Specify the language code that represents the language spoken in your audio.
*
* Amazon Transcribe Medical only supports US English (en-US
).
*
* @see LanguageCode
*/
public final LanguageCode languageCode() {
return LanguageCode.fromValue(languageCode);
}
/**
*
* Specify the language code that represents the language spoken in your audio.
*
*
*
* Amazon Transcribe Medical only supports US English (en-US
).
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #languageCode} will
* return {@link LanguageCode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #languageCodeAsString}.
*
*
* @return Specify the language code that represents the language spoken in your audio.
*
* Amazon Transcribe Medical only supports US English (en-US
).
*
* @see LanguageCode
*/
public final String languageCodeAsString() {
return languageCode;
}
/**
*
* The sample rate of the input audio (in hertz). Amazon Transcribe Medical supports a range from 16,000 Hz to
* 48,000 Hz. Note that the sample rate you specify must match that of your audio.
*
*
* @return The sample rate of the input audio (in hertz). Amazon Transcribe Medical supports a range from 16,000 Hz
* to 48,000 Hz. Note that the sample rate you specify must match that of your audio.
*/
public final Integer mediaSampleRateHertz() {
return mediaSampleRateHertz;
}
/**
*
* Specify the encoding used for the input audio. Supported formats are:
*
*
* -
*
* FLAC
*
*
* -
*
* OPUS-encoded audio in an Ogg container
*
*
* -
*
* PCM (only signed 16-bit little-endian audio formats, which does not include WAV)
*
*
*
*
* For more information, see Media formats.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #mediaEncoding}
* will return {@link MediaEncoding#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #mediaEncodingAsString}.
*
*
* @return Specify the encoding used for the input audio. Supported formats are:
*
* -
*
* FLAC
*
*
* -
*
* OPUS-encoded audio in an Ogg container
*
*
* -
*
* PCM (only signed 16-bit little-endian audio formats, which does not include WAV)
*
*
*
*
* For more information, see Media formats.
* @see MediaEncoding
*/
public final MediaEncoding mediaEncoding() {
return MediaEncoding.fromValue(mediaEncoding);
}
/**
*
* Specify the encoding used for the input audio. Supported formats are:
*
*
* -
*
* FLAC
*
*
* -
*
* OPUS-encoded audio in an Ogg container
*
*
* -
*
* PCM (only signed 16-bit little-endian audio formats, which does not include WAV)
*
*
*
*
* For more information, see Media formats.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #mediaEncoding}
* will return {@link MediaEncoding#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #mediaEncodingAsString}.
*
*
* @return Specify the encoding used for the input audio. Supported formats are:
*
* -
*
* FLAC
*
*
* -
*
* OPUS-encoded audio in an Ogg container
*
*
* -
*
* PCM (only signed 16-bit little-endian audio formats, which does not include WAV)
*
*
*
*
* For more information, see Media formats.
* @see MediaEncoding
*/
public final String mediaEncodingAsString() {
return mediaEncoding;
}
/**
*
* Specify the name of the custom vocabulary that you want to use when processing your transcription. Note that
* vocabulary names are case sensitive.
*
*
* @return Specify the name of the custom vocabulary that you want to use when processing your transcription. Note
* that vocabulary names are case sensitive.
*/
public final String vocabularyName() {
return vocabularyName;
}
/**
*
* Specify the medical specialty contained in your audio.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #specialty} will
* return {@link Specialty#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #specialtyAsString}.
*
*
* @return Specify the medical specialty contained in your audio.
* @see Specialty
*/
public final Specialty specialty() {
return Specialty.fromValue(specialty);
}
/**
*
* Specify the medical specialty contained in your audio.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #specialty} will
* return {@link Specialty#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #specialtyAsString}.
*
*
* @return Specify the medical specialty contained in your audio.
* @see Specialty
*/
public final String specialtyAsString() {
return specialty;
}
/**
*
* Specify the type of input audio. For example, choose DICTATION
for a provider dictating patient
* notes and CONVERSATION
for a dialogue between a patient and a medical professional.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #type} will return
* {@link Type#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #typeAsString}.
*
*
* @return Specify the type of input audio. For example, choose DICTATION
for a provider dictating
* patient notes and CONVERSATION
for a dialogue between a patient and a medical professional.
* @see Type
*/
public final Type type() {
return Type.fromValue(type);
}
/**
*
* Specify the type of input audio. For example, choose DICTATION
for a provider dictating patient
* notes and CONVERSATION
for a dialogue between a patient and a medical professional.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #type} will return
* {@link Type#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #typeAsString}.
*
*
* @return Specify the type of input audio. For example, choose DICTATION
for a provider dictating
* patient notes and CONVERSATION
for a dialogue between a patient and a medical professional.
* @see Type
*/
public final String typeAsString() {
return type;
}
/**
*
* Enables speaker partitioning (diarization) in your transcription output. Speaker partitioning labels the speech
* from individual speakers in your media file.
*
*
* For more information, see Partitioning speakers (diarization).
*
*
* @return Enables speaker partitioning (diarization) in your transcription output. Speaker partitioning labels the
* speech from individual speakers in your media file.
*
* For more information, see Partitioning speakers
* (diarization).
*/
public final Boolean showSpeakerLabel() {
return showSpeakerLabel;
}
/**
*
* Specify a name for your transcription session. If you don't include this parameter in your request, Amazon
* Transcribe Medical generates an ID and returns it in the response.
*
*
* @return Specify a name for your transcription session. If you don't include this parameter in your request,
* Amazon Transcribe Medical generates an ID and returns it in the response.
*/
public final String sessionId() {
return sessionId;
}
/**
*
* Enables channel identification in multi-channel audio.
*
*
* Channel identification transcribes the audio on each channel independently, then appends the output for each
* channel into one transcript.
*
*
* If you have multi-channel audio and do not enable channel identification, your audio is transcribed in a
* continuous manner and your transcript is not separated by channel.
*
*
* If you include EnableChannelIdentification
in your request, you must also include
* NumberOfChannels
.
*
*
* For more information, see Transcribing
* multi-channel audio.
*
*
* @return Enables channel identification in multi-channel audio.
*
* Channel identification transcribes the audio on each channel independently, then appends the output for
* each channel into one transcript.
*
*
* If you have multi-channel audio and do not enable channel identification, your audio is transcribed in a
* continuous manner and your transcript is not separated by channel.
*
*
* If you include EnableChannelIdentification
in your request, you must also include
* NumberOfChannels
.
*
*
* For more information, see Transcribing multi-channel
* audio.
*/
public final Boolean enableChannelIdentification() {
return enableChannelIdentification;
}
/**
*
* Specify the number of channels in your audio stream. This value must be 2
, as only two channels are
* supported. If your audio doesn't contain multiple channels, do not include this parameter in your request.
*
*
* If you include NumberOfChannels
in your request, you must also include
* EnableChannelIdentification
.
*
*
* @return Specify the number of channels in your audio stream. This value must be 2
, as only two
* channels are supported. If your audio doesn't contain multiple channels, do not include this parameter in
* your request.
*
* If you include NumberOfChannels
in your request, you must also include
* EnableChannelIdentification
.
*/
public final Integer numberOfChannels() {
return numberOfChannels;
}
/**
*
* Labels all personal health information (PHI) identified in your transcript.
*
*
* Content identification is performed at the segment level; PHI is flagged upon complete transcription of an audio
* segment.
*
*
* For more information, see Identifying
* personal health information (PHI) in a transcription.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #contentIdentificationType} will return {@link MedicalContentIdentificationType#UNKNOWN_TO_SDK_VERSION}.
* The raw value returned by the service is available from {@link #contentIdentificationTypeAsString}.
*
*
* @return Labels all personal health information (PHI) identified in your transcript.
*
* Content identification is performed at the segment level; PHI is flagged upon complete transcription of
* an audio segment.
*
*
* For more information, see Identifying personal health
* information (PHI) in a transcription.
* @see MedicalContentIdentificationType
*/
public final MedicalContentIdentificationType contentIdentificationType() {
return MedicalContentIdentificationType.fromValue(contentIdentificationType);
}
/**
*
* Labels all personal health information (PHI) identified in your transcript.
*
*
* Content identification is performed at the segment level; PHI is flagged upon complete transcription of an audio
* segment.
*
*
* For more information, see Identifying
* personal health information (PHI) in a transcription.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #contentIdentificationType} will return {@link MedicalContentIdentificationType#UNKNOWN_TO_SDK_VERSION}.
* The raw value returned by the service is available from {@link #contentIdentificationTypeAsString}.
*
*
* @return Labels all personal health information (PHI) identified in your transcript.
*
* Content identification is performed at the segment level; PHI is flagged upon complete transcription of
* an audio segment.
*
*
* For more information, see Identifying personal health
* information (PHI) in a transcription.
* @see MedicalContentIdentificationType
*/
public final String contentIdentificationTypeAsString() {
return contentIdentificationType;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(languageCodeAsString());
hashCode = 31 * hashCode + Objects.hashCode(mediaSampleRateHertz());
hashCode = 31 * hashCode + Objects.hashCode(mediaEncodingAsString());
hashCode = 31 * hashCode + Objects.hashCode(vocabularyName());
hashCode = 31 * hashCode + Objects.hashCode(specialtyAsString());
hashCode = 31 * hashCode + Objects.hashCode(typeAsString());
hashCode = 31 * hashCode + Objects.hashCode(showSpeakerLabel());
hashCode = 31 * hashCode + Objects.hashCode(sessionId());
hashCode = 31 * hashCode + Objects.hashCode(enableChannelIdentification());
hashCode = 31 * hashCode + Objects.hashCode(numberOfChannels());
hashCode = 31 * hashCode + Objects.hashCode(contentIdentificationTypeAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof StartMedicalStreamTranscriptionRequest)) {
return false;
}
StartMedicalStreamTranscriptionRequest other = (StartMedicalStreamTranscriptionRequest) obj;
return Objects.equals(languageCodeAsString(), other.languageCodeAsString())
&& Objects.equals(mediaSampleRateHertz(), other.mediaSampleRateHertz())
&& Objects.equals(mediaEncodingAsString(), other.mediaEncodingAsString())
&& Objects.equals(vocabularyName(), other.vocabularyName())
&& Objects.equals(specialtyAsString(), other.specialtyAsString())
&& Objects.equals(typeAsString(), other.typeAsString())
&& Objects.equals(showSpeakerLabel(), other.showSpeakerLabel()) && Objects.equals(sessionId(), other.sessionId())
&& Objects.equals(enableChannelIdentification(), other.enableChannelIdentification())
&& Objects.equals(numberOfChannels(), other.numberOfChannels())
&& Objects.equals(contentIdentificationTypeAsString(), other.contentIdentificationTypeAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("StartMedicalStreamTranscriptionRequest").add("LanguageCode", languageCodeAsString())
.add("MediaSampleRateHertz", mediaSampleRateHertz()).add("MediaEncoding", mediaEncodingAsString())
.add("VocabularyName", vocabularyName()).add("Specialty", specialtyAsString()).add("Type", typeAsString())
.add("ShowSpeakerLabel", showSpeakerLabel()).add("SessionId", sessionId())
.add("EnableChannelIdentification", enableChannelIdentification()).add("NumberOfChannels", numberOfChannels())
.add("ContentIdentificationType", contentIdentificationTypeAsString()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "LanguageCode":
return Optional.ofNullable(clazz.cast(languageCodeAsString()));
case "MediaSampleRateHertz":
return Optional.ofNullable(clazz.cast(mediaSampleRateHertz()));
case "MediaEncoding":
return Optional.ofNullable(clazz.cast(mediaEncodingAsString()));
case "VocabularyName":
return Optional.ofNullable(clazz.cast(vocabularyName()));
case "Specialty":
return Optional.ofNullable(clazz.cast(specialtyAsString()));
case "Type":
return Optional.ofNullable(clazz.cast(typeAsString()));
case "ShowSpeakerLabel":
return Optional.ofNullable(clazz.cast(showSpeakerLabel()));
case "SessionId":
return Optional.ofNullable(clazz.cast(sessionId()));
case "EnableChannelIdentification":
return Optional.ofNullable(clazz.cast(enableChannelIdentification()));
case "NumberOfChannels":
return Optional.ofNullable(clazz.cast(numberOfChannels()));
case "ContentIdentificationType":
return Optional.ofNullable(clazz.cast(contentIdentificationTypeAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Map> memberNameToFieldInitializer() {
Map> map = new HashMap<>();
map.put("x-amzn-transcribe-language-code", LANGUAGE_CODE_FIELD);
map.put("x-amzn-transcribe-sample-rate", MEDIA_SAMPLE_RATE_HERTZ_FIELD);
map.put("x-amzn-transcribe-media-encoding", MEDIA_ENCODING_FIELD);
map.put("x-amzn-transcribe-vocabulary-name", VOCABULARY_NAME_FIELD);
map.put("x-amzn-transcribe-specialty", SPECIALTY_FIELD);
map.put("x-amzn-transcribe-type", TYPE_FIELD);
map.put("x-amzn-transcribe-show-speaker-label", SHOW_SPEAKER_LABEL_FIELD);
map.put("x-amzn-transcribe-session-id", SESSION_ID_FIELD);
map.put("x-amzn-transcribe-enable-channel-identification", ENABLE_CHANNEL_IDENTIFICATION_FIELD);
map.put("x-amzn-transcribe-number-of-channels", NUMBER_OF_CHANNELS_FIELD);
map.put("x-amzn-transcribe-content-identification-type", CONTENT_IDENTIFICATION_TYPE_FIELD);
return Collections.unmodifiableMap(map);
}
private static Function
*
* Amazon Transcribe Medical only supports US English (en-US
).
*
* @see LanguageCode
* @return Returns a reference to this object so that method calls can be chained together.
* @see LanguageCode
*/
Builder languageCode(String languageCode);
/**
*
* Specify the language code that represents the language spoken in your audio.
*
*
*
* Amazon Transcribe Medical only supports US English (en-US
).
*
*
*
* @param languageCode
* Specify the language code that represents the language spoken in your audio.
*
* Amazon Transcribe Medical only supports US English (en-US
).
*
* @see LanguageCode
* @return Returns a reference to this object so that method calls can be chained together.
* @see LanguageCode
*/
Builder languageCode(LanguageCode languageCode);
/**
*
* The sample rate of the input audio (in hertz). Amazon Transcribe Medical supports a range from 16,000 Hz to
* 48,000 Hz. Note that the sample rate you specify must match that of your audio.
*
*
* @param mediaSampleRateHertz
* The sample rate of the input audio (in hertz). Amazon Transcribe Medical supports a range from 16,000
* Hz to 48,000 Hz. Note that the sample rate you specify must match that of your audio.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder mediaSampleRateHertz(Integer mediaSampleRateHertz);
/**
*
* Specify the encoding used for the input audio. Supported formats are:
*
*
* -
*
* FLAC
*
*
* -
*
* OPUS-encoded audio in an Ogg container
*
*
* -
*
* PCM (only signed 16-bit little-endian audio formats, which does not include WAV)
*
*
*
*
* For more information, see Media formats.
*
*
* @param mediaEncoding
* Specify the encoding used for the input audio. Supported formats are:
*
* -
*
* FLAC
*
*
* -
*
* OPUS-encoded audio in an Ogg container
*
*
* -
*
* PCM (only signed 16-bit little-endian audio formats, which does not include WAV)
*
*
*
*
* For more information, see Media
* formats.
* @see MediaEncoding
* @return Returns a reference to this object so that method calls can be chained together.
* @see MediaEncoding
*/
Builder mediaEncoding(String mediaEncoding);
/**
*
* Specify the encoding used for the input audio. Supported formats are:
*
*
* -
*
* FLAC
*
*
* -
*
* OPUS-encoded audio in an Ogg container
*
*
* -
*
* PCM (only signed 16-bit little-endian audio formats, which does not include WAV)
*
*
*
*
* For more information, see Media formats.
*
*
* @param mediaEncoding
* Specify the encoding used for the input audio. Supported formats are:
*
* -
*
* FLAC
*
*
* -
*
* OPUS-encoded audio in an Ogg container
*
*
* -
*
* PCM (only signed 16-bit little-endian audio formats, which does not include WAV)
*
*
*
*
* For more information, see Media
* formats.
* @see MediaEncoding
* @return Returns a reference to this object so that method calls can be chained together.
* @see MediaEncoding
*/
Builder mediaEncoding(MediaEncoding mediaEncoding);
/**
*
* Specify the name of the custom vocabulary that you want to use when processing your transcription. Note that
* vocabulary names are case sensitive.
*
*
* @param vocabularyName
* Specify the name of the custom vocabulary that you want to use when processing your transcription.
* Note that vocabulary names are case sensitive.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder vocabularyName(String vocabularyName);
/**
*
* Specify the medical specialty contained in your audio.
*
*
* @param specialty
* Specify the medical specialty contained in your audio.
* @see Specialty
* @return Returns a reference to this object so that method calls can be chained together.
* @see Specialty
*/
Builder specialty(String specialty);
/**
*
* Specify the medical specialty contained in your audio.
*
*
* @param specialty
* Specify the medical specialty contained in your audio.
* @see Specialty
* @return Returns a reference to this object so that method calls can be chained together.
* @see Specialty
*/
Builder specialty(Specialty specialty);
/**
*
* Specify the type of input audio. For example, choose DICTATION
for a provider dictating patient
* notes and CONVERSATION
for a dialogue between a patient and a medical professional.
*
*
* @param type
* Specify the type of input audio. For example, choose DICTATION
for a provider dictating
* patient notes and CONVERSATION
for a dialogue between a patient and a medical
* professional.
* @see Type
* @return Returns a reference to this object so that method calls can be chained together.
* @see Type
*/
Builder type(String type);
/**
*
* Specify the type of input audio. For example, choose DICTATION
for a provider dictating patient
* notes and CONVERSATION
for a dialogue between a patient and a medical professional.
*
*
* @param type
* Specify the type of input audio. For example, choose DICTATION
for a provider dictating
* patient notes and CONVERSATION
for a dialogue between a patient and a medical
* professional.
* @see Type
* @return Returns a reference to this object so that method calls can be chained together.
* @see Type
*/
Builder type(Type type);
/**
*
* Enables speaker partitioning (diarization) in your transcription output. Speaker partitioning labels the
* speech from individual speakers in your media file.
*
*
* For more information, see Partitioning speakers
* (diarization).
*
*
* @param showSpeakerLabel
* Enables speaker partitioning (diarization) in your transcription output. Speaker partitioning labels
* the speech from individual speakers in your media file.
*
* For more information, see Partitioning speakers
* (diarization).
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder showSpeakerLabel(Boolean showSpeakerLabel);
/**
*
* Specify a name for your transcription session. If you don't include this parameter in your request, Amazon
* Transcribe Medical generates an ID and returns it in the response.
*
*
* @param sessionId
* Specify a name for your transcription session. If you don't include this parameter in your request,
* Amazon Transcribe Medical generates an ID and returns it in the response.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder sessionId(String sessionId);
/**
*
* Enables channel identification in multi-channel audio.
*
*
* Channel identification transcribes the audio on each channel independently, then appends the output for each
* channel into one transcript.
*
*
* If you have multi-channel audio and do not enable channel identification, your audio is transcribed in a
* continuous manner and your transcript is not separated by channel.
*
*
* If you include EnableChannelIdentification
in your request, you must also include
* NumberOfChannels
.
*
*
* For more information, see Transcribing multi-channel audio.
*
*
* @param enableChannelIdentification
* Enables channel identification in multi-channel audio.
*
* Channel identification transcribes the audio on each channel independently, then appends the output
* for each channel into one transcript.
*
*
* If you have multi-channel audio and do not enable channel identification, your audio is transcribed in
* a continuous manner and your transcript is not separated by channel.
*
*
* If you include EnableChannelIdentification
in your request, you must also include
* NumberOfChannels
.
*
*
* For more information, see Transcribing multi-channel
* audio.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder enableChannelIdentification(Boolean enableChannelIdentification);
/**
*
* Specify the number of channels in your audio stream. This value must be 2
, as only two channels
* are supported. If your audio doesn't contain multiple channels, do not include this parameter in your
* request.
*
*
* If you include NumberOfChannels
in your request, you must also include
* EnableChannelIdentification
.
*
*
* @param numberOfChannels
* Specify the number of channels in your audio stream. This value must be 2
, as only two
* channels are supported. If your audio doesn't contain multiple channels, do not include this parameter
* in your request.
*
* If you include NumberOfChannels
in your request, you must also include
* EnableChannelIdentification
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder numberOfChannels(Integer numberOfChannels);
/**
*
* Labels all personal health information (PHI) identified in your transcript.
*
*
* Content identification is performed at the segment level; PHI is flagged upon complete transcription of an
* audio segment.
*
*
* For more information, see Identifying
* personal health information (PHI) in a transcription.
*
*
* @param contentIdentificationType
* Labels all personal health information (PHI) identified in your transcript.
*
* Content identification is performed at the segment level; PHI is flagged upon complete transcription
* of an audio segment.
*
*
* For more information, see Identifying personal health
* information (PHI) in a transcription.
* @see MedicalContentIdentificationType
* @return Returns a reference to this object so that method calls can be chained together.
* @see MedicalContentIdentificationType
*/
Builder contentIdentificationType(String contentIdentificationType);
/**
*
* Labels all personal health information (PHI) identified in your transcript.
*
*
* Content identification is performed at the segment level; PHI is flagged upon complete transcription of an
* audio segment.
*
*
* For more information, see Identifying
* personal health information (PHI) in a transcription.
*
*
* @param contentIdentificationType
* Labels all personal health information (PHI) identified in your transcript.
*
* Content identification is performed at the segment level; PHI is flagged upon complete transcription
* of an audio segment.
*
*
* For more information, see Identifying personal health
* information (PHI) in a transcription.
* @see MedicalContentIdentificationType
* @return Returns a reference to this object so that method calls can be chained together.
* @see MedicalContentIdentificationType
*/
Builder contentIdentificationType(MedicalContentIdentificationType contentIdentificationType);
@Override
Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration);
@Override
Builder overrideConfiguration(Consumer builderConsumer);
}
static final class BuilderImpl extends TranscribeStreamingRequest.BuilderImpl implements Builder {
private String languageCode;
private Integer mediaSampleRateHertz;
private String mediaEncoding;
private String vocabularyName;
private String specialty;
private String type;
private Boolean showSpeakerLabel;
private String sessionId;
private Boolean enableChannelIdentification;
private Integer numberOfChannels;
private String contentIdentificationType;
private BuilderImpl() {
}
private BuilderImpl(StartMedicalStreamTranscriptionRequest model) {
super(model);
languageCode(model.languageCode);
mediaSampleRateHertz(model.mediaSampleRateHertz);
mediaEncoding(model.mediaEncoding);
vocabularyName(model.vocabularyName);
specialty(model.specialty);
type(model.type);
showSpeakerLabel(model.showSpeakerLabel);
sessionId(model.sessionId);
enableChannelIdentification(model.enableChannelIdentification);
numberOfChannels(model.numberOfChannels);
contentIdentificationType(model.contentIdentificationType);
}
public final String getLanguageCode() {
return languageCode;
}
public final void setLanguageCode(String languageCode) {
this.languageCode = languageCode;
}
@Override
public final Builder languageCode(String languageCode) {
this.languageCode = languageCode;
return this;
}
@Override
public final Builder languageCode(LanguageCode languageCode) {
this.languageCode(languageCode == null ? null : languageCode.toString());
return this;
}
public final Integer getMediaSampleRateHertz() {
return mediaSampleRateHertz;
}
public final void setMediaSampleRateHertz(Integer mediaSampleRateHertz) {
this.mediaSampleRateHertz = mediaSampleRateHertz;
}
@Override
public final Builder mediaSampleRateHertz(Integer mediaSampleRateHertz) {
this.mediaSampleRateHertz = mediaSampleRateHertz;
return this;
}
public final String getMediaEncoding() {
return mediaEncoding;
}
public final void setMediaEncoding(String mediaEncoding) {
this.mediaEncoding = mediaEncoding;
}
@Override
public final Builder mediaEncoding(String mediaEncoding) {
this.mediaEncoding = mediaEncoding;
return this;
}
@Override
public final Builder mediaEncoding(MediaEncoding mediaEncoding) {
this.mediaEncoding(mediaEncoding == null ? null : mediaEncoding.toString());
return this;
}
public final String getVocabularyName() {
return vocabularyName;
}
public final void setVocabularyName(String vocabularyName) {
this.vocabularyName = vocabularyName;
}
@Override
public final Builder vocabularyName(String vocabularyName) {
this.vocabularyName = vocabularyName;
return this;
}
public final String getSpecialty() {
return specialty;
}
public final void setSpecialty(String specialty) {
this.specialty = specialty;
}
@Override
public final Builder specialty(String specialty) {
this.specialty = specialty;
return this;
}
@Override
public final Builder specialty(Specialty specialty) {
this.specialty(specialty == null ? null : specialty.toString());
return this;
}
public final String getType() {
return type;
}
public final void setType(String type) {
this.type = type;
}
@Override
public final Builder type(String type) {
this.type = type;
return this;
}
@Override
public final Builder type(Type type) {
this.type(type == null ? null : type.toString());
return this;
}
public final Boolean getShowSpeakerLabel() {
return showSpeakerLabel;
}
public final void setShowSpeakerLabel(Boolean showSpeakerLabel) {
this.showSpeakerLabel = showSpeakerLabel;
}
@Override
public final Builder showSpeakerLabel(Boolean showSpeakerLabel) {
this.showSpeakerLabel = showSpeakerLabel;
return this;
}
public final String getSessionId() {
return sessionId;
}
public final void setSessionId(String sessionId) {
this.sessionId = sessionId;
}
@Override
public final Builder sessionId(String sessionId) {
this.sessionId = sessionId;
return this;
}
public final Boolean getEnableChannelIdentification() {
return enableChannelIdentification;
}
public final void setEnableChannelIdentification(Boolean enableChannelIdentification) {
this.enableChannelIdentification = enableChannelIdentification;
}
@Override
public final Builder enableChannelIdentification(Boolean enableChannelIdentification) {
this.enableChannelIdentification = enableChannelIdentification;
return this;
}
public final Integer getNumberOfChannels() {
return numberOfChannels;
}
public final void setNumberOfChannels(Integer numberOfChannels) {
this.numberOfChannels = numberOfChannels;
}
@Override
public final Builder numberOfChannels(Integer numberOfChannels) {
this.numberOfChannels = numberOfChannels;
return this;
}
public final String getContentIdentificationType() {
return contentIdentificationType;
}
public final void setContentIdentificationType(String contentIdentificationType) {
this.contentIdentificationType = contentIdentificationType;
}
@Override
public final Builder contentIdentificationType(String contentIdentificationType) {
this.contentIdentificationType = contentIdentificationType;
return this;
}
@Override
public final Builder contentIdentificationType(MedicalContentIdentificationType contentIdentificationType) {
this.contentIdentificationType(contentIdentificationType == null ? null : contentIdentificationType.toString());
return this;
}
@Override
public Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration) {
super.overrideConfiguration(overrideConfiguration);
return this;
}
@Override
public Builder overrideConfiguration(Consumer builderConsumer) {
super.overrideConfiguration(builderConsumer);
return this;
}
@Override
public StartMedicalStreamTranscriptionRequest build() {
return new StartMedicalStreamTranscriptionRequest(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
@Override
public Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
}
}