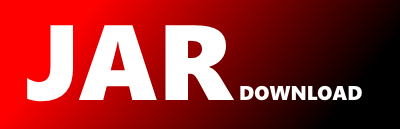
software.amazon.awssdk.services.transfer.model.UpdateServerRequest Maven / Gradle / Ivy
Show all versions of transfer Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.transfer.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class UpdateServerRequest extends TransferRequest implements
ToCopyableBuilder {
private static final SdkField CERTIFICATE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Certificate").getter(getter(UpdateServerRequest::certificate)).setter(setter(Builder::certificate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Certificate").build()).build();
private static final SdkField ENDPOINT_DETAILS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("EndpointDetails")
.getter(getter(UpdateServerRequest::endpointDetails)).setter(setter(Builder::endpointDetails))
.constructor(EndpointDetails::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EndpointDetails").build()).build();
private static final SdkField ENDPOINT_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("EndpointType").getter(getter(UpdateServerRequest::endpointTypeAsString))
.setter(setter(Builder::endpointType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EndpointType").build()).build();
private static final SdkField HOST_KEY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("HostKey").getter(getter(UpdateServerRequest::hostKey)).setter(setter(Builder::hostKey))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("HostKey").build()).build();
private static final SdkField IDENTITY_PROVIDER_DETAILS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("IdentityProviderDetails")
.getter(getter(UpdateServerRequest::identityProviderDetails)).setter(setter(Builder::identityProviderDetails))
.constructor(IdentityProviderDetails::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IdentityProviderDetails").build())
.build();
private static final SdkField LOGGING_ROLE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("LoggingRole").getter(getter(UpdateServerRequest::loggingRole)).setter(setter(Builder::loggingRole))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LoggingRole").build()).build();
private static final SdkField> PROTOCOLS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Protocols")
.getter(getter(UpdateServerRequest::protocolsAsStrings))
.setter(setter(Builder::protocolsWithStrings))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Protocols").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField SECURITY_POLICY_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SecurityPolicyName").getter(getter(UpdateServerRequest::securityPolicyName))
.setter(setter(Builder::securityPolicyName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SecurityPolicyName").build())
.build();
private static final SdkField SERVER_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ServerId").getter(getter(UpdateServerRequest::serverId)).setter(setter(Builder::serverId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ServerId").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(CERTIFICATE_FIELD,
ENDPOINT_DETAILS_FIELD, ENDPOINT_TYPE_FIELD, HOST_KEY_FIELD, IDENTITY_PROVIDER_DETAILS_FIELD, LOGGING_ROLE_FIELD,
PROTOCOLS_FIELD, SECURITY_POLICY_NAME_FIELD, SERVER_ID_FIELD));
private final String certificate;
private final EndpointDetails endpointDetails;
private final String endpointType;
private final String hostKey;
private final IdentityProviderDetails identityProviderDetails;
private final String loggingRole;
private final List protocols;
private final String securityPolicyName;
private final String serverId;
private UpdateServerRequest(BuilderImpl builder) {
super(builder);
this.certificate = builder.certificate;
this.endpointDetails = builder.endpointDetails;
this.endpointType = builder.endpointType;
this.hostKey = builder.hostKey;
this.identityProviderDetails = builder.identityProviderDetails;
this.loggingRole = builder.loggingRole;
this.protocols = builder.protocols;
this.securityPolicyName = builder.securityPolicyName;
this.serverId = builder.serverId;
}
/**
*
* The Amazon Resource Name (ARN) of the AWS Certificate Manager (ACM) certificate. Required when
* Protocols
is set to FTPS
.
*
*
* To request a new public certificate, see Request a public
* certificate in the AWS Certificate Manager User Guide.
*
*
* To import an existing certificate into ACM, see Importing certificates into
* ACM in the AWS Certificate Manager User Guide.
*
*
* To request a private certificate to use FTPS through private IP addresses, see Request a private
* certificate in the AWS Certificate Manager User Guide.
*
*
* Certificates with the following cryptographic algorithms and key sizes are supported:
*
*
* -
*
* 2048-bit RSA (RSA_2048)
*
*
* -
*
* 4096-bit RSA (RSA_4096)
*
*
* -
*
* Elliptic Prime Curve 256 bit (EC_prime256v1)
*
*
* -
*
* Elliptic Prime Curve 384 bit (EC_secp384r1)
*
*
* -
*
* Elliptic Prime Curve 521 bit (EC_secp521r1)
*
*
*
*
*
* The certificate must be a valid SSL/TLS X.509 version 3 certificate with FQDN or IP address specified and
* information about the issuer.
*
*
*
* @return The Amazon Resource Name (ARN) of the AWS Certificate Manager (ACM) certificate. Required when
* Protocols
is set to FTPS
.
*
* To request a new public certificate, see Request a public
* certificate in the AWS Certificate Manager User Guide.
*
*
* To import an existing certificate into ACM, see Importing certificates
* into ACM in the AWS Certificate Manager User Guide.
*
*
* To request a private certificate to use FTPS through private IP addresses, see Request a private
* certificate in the AWS Certificate Manager User Guide.
*
*
* Certificates with the following cryptographic algorithms and key sizes are supported:
*
*
* -
*
* 2048-bit RSA (RSA_2048)
*
*
* -
*
* 4096-bit RSA (RSA_4096)
*
*
* -
*
* Elliptic Prime Curve 256 bit (EC_prime256v1)
*
*
* -
*
* Elliptic Prime Curve 384 bit (EC_secp384r1)
*
*
* -
*
* Elliptic Prime Curve 521 bit (EC_secp521r1)
*
*
*
*
*
* The certificate must be a valid SSL/TLS X.509 version 3 certificate with FQDN or IP address specified and
* information about the issuer.
*
*/
public final String certificate() {
return certificate;
}
/**
*
* The virtual private cloud (VPC) endpoint settings that are configured for your server. With a VPC endpoint, you
* can restrict access to your server to resources only within your VPC. To control incoming internet traffic, you
* will need to associate one or more Elastic IP addresses with your server's endpoint.
*
*
* @return The virtual private cloud (VPC) endpoint settings that are configured for your server. With a VPC
* endpoint, you can restrict access to your server to resources only within your VPC. To control incoming
* internet traffic, you will need to associate one or more Elastic IP addresses with your server's
* endpoint.
*/
public final EndpointDetails endpointDetails() {
return endpointDetails;
}
/**
*
* The type of endpoint that you want your server to connect to. You can choose to connect to the public internet or
* a VPC endpoint. With a VPC endpoint, you can restrict access to your server and resources only within your VPC.
*
*
*
* It is recommended that you use VPC
as the EndpointType
. With this endpoint type, you
* have the option to directly associate up to three Elastic IPv4 addresses (BYO IP included) with your server's
* endpoint and use VPC security groups to restrict traffic by the client's public IP address. This is not possible
* with EndpointType
set to VPC_ENDPOINT
.
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #endpointType} will
* return {@link EndpointType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #endpointTypeAsString}.
*
*
* @return The type of endpoint that you want your server to connect to. You can choose to connect to the public
* internet or a VPC endpoint. With a VPC endpoint, you can restrict access to your server and resources
* only within your VPC.
*
* It is recommended that you use VPC
as the EndpointType
. With this endpoint
* type, you have the option to directly associate up to three Elastic IPv4 addresses (BYO IP included) with
* your server's endpoint and use VPC security groups to restrict traffic by the client's public IP address.
* This is not possible with EndpointType
set to VPC_ENDPOINT
.
*
* @see EndpointType
*/
public final EndpointType endpointType() {
return EndpointType.fromValue(endpointType);
}
/**
*
* The type of endpoint that you want your server to connect to. You can choose to connect to the public internet or
* a VPC endpoint. With a VPC endpoint, you can restrict access to your server and resources only within your VPC.
*
*
*
* It is recommended that you use VPC
as the EndpointType
. With this endpoint type, you
* have the option to directly associate up to three Elastic IPv4 addresses (BYO IP included) with your server's
* endpoint and use VPC security groups to restrict traffic by the client's public IP address. This is not possible
* with EndpointType
set to VPC_ENDPOINT
.
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #endpointType} will
* return {@link EndpointType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #endpointTypeAsString}.
*
*
* @return The type of endpoint that you want your server to connect to. You can choose to connect to the public
* internet or a VPC endpoint. With a VPC endpoint, you can restrict access to your server and resources
* only within your VPC.
*
* It is recommended that you use VPC
as the EndpointType
. With this endpoint
* type, you have the option to directly associate up to three Elastic IPv4 addresses (BYO IP included) with
* your server's endpoint and use VPC security groups to restrict traffic by the client's public IP address.
* This is not possible with EndpointType
set to VPC_ENDPOINT
.
*
* @see EndpointType
*/
public final String endpointTypeAsString() {
return endpointType;
}
/**
*
* The RSA private key as generated by ssh-keygen -N "" -m PEM -f my-new-server-key
.
*
*
*
* If you aren't planning to migrate existing users from an existing server to a new server, don't update the host
* key. Accidentally changing a server's host key can be disruptive.
*
*
*
* For more information, see Change the host key for your SFTP-enabled server in the AWS Transfer Family User Guide.
*
*
* @return The RSA private key as generated by ssh-keygen -N "" -m PEM -f my-new-server-key
.
*
*
* If you aren't planning to migrate existing users from an existing server to a new server, don't update
* the host key. Accidentally changing a server's host key can be disruptive.
*
*
*
* For more information, see Change the host key for your SFTP-enabled server in the AWS Transfer Family User Guide.
*/
public final String hostKey() {
return hostKey;
}
/**
*
* An array containing all of the information required to call a customer's authentication API method.
*
*
* @return An array containing all of the information required to call a customer's authentication API method.
*/
public final IdentityProviderDetails identityProviderDetails() {
return identityProviderDetails;
}
/**
*
* Changes the AWS Identity and Access Management (IAM) role that allows Amazon S3 events to be logged in Amazon
* CloudWatch, turning logging on or off.
*
*
* @return Changes the AWS Identity and Access Management (IAM) role that allows Amazon S3 events to be logged in
* Amazon CloudWatch, turning logging on or off.
*/
public final String loggingRole() {
return loggingRole;
}
/**
*
* Specifies the file transfer protocol or protocols over which your file transfer protocol client can connect to
* your server's endpoint. The available protocols are:
*
*
* -
*
* Secure Shell (SSH) File Transfer Protocol (SFTP): File transfer over SSH
*
*
* -
*
* File Transfer Protocol Secure (FTPS): File transfer with TLS encryption
*
*
* -
*
* File Transfer Protocol (FTP): Unencrypted file transfer
*
*
*
*
*
* If you select FTPS
, you must choose a certificate stored in AWS Certificate Manager (ACM) which will
* be used to identify your server when clients connect to it over FTPS.
*
*
* If Protocol
includes either FTP
or FTPS
, then the
* EndpointType
must be VPC
and the IdentityProviderType
must be
* API_GATEWAY
.
*
*
* If Protocol
includes FTP
, then AddressAllocationIds
cannot be associated.
*
*
* If Protocol
is set only to SFTP
, the EndpointType
can be set to
* PUBLIC
and the IdentityProviderType
can be set to SERVICE_MANAGED
.
*
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasProtocols()} to see if a value was sent in this field.
*
*
* @return Specifies the file transfer protocol or protocols over which your file transfer protocol client can
* connect to your server's endpoint. The available protocols are:
*
* -
*
* Secure Shell (SSH) File Transfer Protocol (SFTP): File transfer over SSH
*
*
* -
*
* File Transfer Protocol Secure (FTPS): File transfer with TLS encryption
*
*
* -
*
* File Transfer Protocol (FTP): Unencrypted file transfer
*
*
*
*
*
* If you select FTPS
, you must choose a certificate stored in AWS Certificate Manager (ACM)
* which will be used to identify your server when clients connect to it over FTPS.
*
*
* If Protocol
includes either FTP
or FTPS
, then the
* EndpointType
must be VPC
and the IdentityProviderType
must be
* API_GATEWAY
.
*
*
* If Protocol
includes FTP
, then AddressAllocationIds
cannot be
* associated.
*
*
* If Protocol
is set only to SFTP
, the EndpointType
can be set to
* PUBLIC
and the IdentityProviderType
can be set to SERVICE_MANAGED
.
*
*/
public final List protocols() {
return ProtocolsCopier.copyStringToEnum(protocols);
}
/**
* Returns true if the Protocols property was specified by the sender (it may be empty), or false if the sender did
* not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS service.
*/
public final boolean hasProtocols() {
return protocols != null && !(protocols instanceof SdkAutoConstructList);
}
/**
*
* Specifies the file transfer protocol or protocols over which your file transfer protocol client can connect to
* your server's endpoint. The available protocols are:
*
*
* -
*
* Secure Shell (SSH) File Transfer Protocol (SFTP): File transfer over SSH
*
*
* -
*
* File Transfer Protocol Secure (FTPS): File transfer with TLS encryption
*
*
* -
*
* File Transfer Protocol (FTP): Unencrypted file transfer
*
*
*
*
*
* If you select FTPS
, you must choose a certificate stored in AWS Certificate Manager (ACM) which will
* be used to identify your server when clients connect to it over FTPS.
*
*
* If Protocol
includes either FTP
or FTPS
, then the
* EndpointType
must be VPC
and the IdentityProviderType
must be
* API_GATEWAY
.
*
*
* If Protocol
includes FTP
, then AddressAllocationIds
cannot be associated.
*
*
* If Protocol
is set only to SFTP
, the EndpointType
can be set to
* PUBLIC
and the IdentityProviderType
can be set to SERVICE_MANAGED
.
*
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasProtocols()} to see if a value was sent in this field.
*
*
* @return Specifies the file transfer protocol or protocols over which your file transfer protocol client can
* connect to your server's endpoint. The available protocols are:
*
* -
*
* Secure Shell (SSH) File Transfer Protocol (SFTP): File transfer over SSH
*
*
* -
*
* File Transfer Protocol Secure (FTPS): File transfer with TLS encryption
*
*
* -
*
* File Transfer Protocol (FTP): Unencrypted file transfer
*
*
*
*
*
* If you select FTPS
, you must choose a certificate stored in AWS Certificate Manager (ACM)
* which will be used to identify your server when clients connect to it over FTPS.
*
*
* If Protocol
includes either FTP
or FTPS
, then the
* EndpointType
must be VPC
and the IdentityProviderType
must be
* API_GATEWAY
.
*
*
* If Protocol
includes FTP
, then AddressAllocationIds
cannot be
* associated.
*
*
* If Protocol
is set only to SFTP
, the EndpointType
can be set to
* PUBLIC
and the IdentityProviderType
can be set to SERVICE_MANAGED
.
*
*/
public final List protocolsAsStrings() {
return protocols;
}
/**
*
* Specifies the name of the security policy that is attached to the server.
*
*
* @return Specifies the name of the security policy that is attached to the server.
*/
public final String securityPolicyName() {
return securityPolicyName;
}
/**
*
* A system-assigned unique identifier for a server instance that the user account is assigned to.
*
*
* @return A system-assigned unique identifier for a server instance that the user account is assigned to.
*/
public final String serverId() {
return serverId;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(certificate());
hashCode = 31 * hashCode + Objects.hashCode(endpointDetails());
hashCode = 31 * hashCode + Objects.hashCode(endpointTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(hostKey());
hashCode = 31 * hashCode + Objects.hashCode(identityProviderDetails());
hashCode = 31 * hashCode + Objects.hashCode(loggingRole());
hashCode = 31 * hashCode + Objects.hashCode(hasProtocols() ? protocolsAsStrings() : null);
hashCode = 31 * hashCode + Objects.hashCode(securityPolicyName());
hashCode = 31 * hashCode + Objects.hashCode(serverId());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof UpdateServerRequest)) {
return false;
}
UpdateServerRequest other = (UpdateServerRequest) obj;
return Objects.equals(certificate(), other.certificate()) && Objects.equals(endpointDetails(), other.endpointDetails())
&& Objects.equals(endpointTypeAsString(), other.endpointTypeAsString())
&& Objects.equals(hostKey(), other.hostKey())
&& Objects.equals(identityProviderDetails(), other.identityProviderDetails())
&& Objects.equals(loggingRole(), other.loggingRole()) && hasProtocols() == other.hasProtocols()
&& Objects.equals(protocolsAsStrings(), other.protocolsAsStrings())
&& Objects.equals(securityPolicyName(), other.securityPolicyName())
&& Objects.equals(serverId(), other.serverId());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("UpdateServerRequest").add("Certificate", certificate())
.add("EndpointDetails", endpointDetails()).add("EndpointType", endpointTypeAsString())
.add("HostKey", hostKey() == null ? null : "*** Sensitive Data Redacted ***")
.add("IdentityProviderDetails", identityProviderDetails()).add("LoggingRole", loggingRole())
.add("Protocols", hasProtocols() ? protocolsAsStrings() : null).add("SecurityPolicyName", securityPolicyName())
.add("ServerId", serverId()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Certificate":
return Optional.ofNullable(clazz.cast(certificate()));
case "EndpointDetails":
return Optional.ofNullable(clazz.cast(endpointDetails()));
case "EndpointType":
return Optional.ofNullable(clazz.cast(endpointTypeAsString()));
case "HostKey":
return Optional.ofNullable(clazz.cast(hostKey()));
case "IdentityProviderDetails":
return Optional.ofNullable(clazz.cast(identityProviderDetails()));
case "LoggingRole":
return Optional.ofNullable(clazz.cast(loggingRole()));
case "Protocols":
return Optional.ofNullable(clazz.cast(protocolsAsStrings()));
case "SecurityPolicyName":
return Optional.ofNullable(clazz.cast(securityPolicyName()));
case "ServerId":
return Optional.ofNullable(clazz.cast(serverId()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function