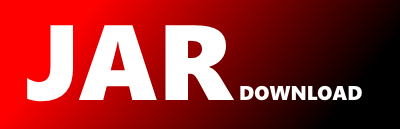
software.amazon.awssdk.services.transfer.DefaultTransferAsyncClient Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.transfer;
import java.util.Collections;
import java.util.List;
import java.util.concurrent.CompletableFuture;
import java.util.function.Consumer;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.awscore.client.handler.AwsAsyncClientHandler;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.core.ApiName;
import software.amazon.awssdk.core.RequestOverrideConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientOption;
import software.amazon.awssdk.core.client.handler.AsyncClientHandler;
import software.amazon.awssdk.core.client.handler.ClientExecutionParams;
import software.amazon.awssdk.core.http.HttpResponseHandler;
import software.amazon.awssdk.core.metrics.CoreMetric;
import software.amazon.awssdk.core.util.VersionInfo;
import software.amazon.awssdk.metrics.MetricCollector;
import software.amazon.awssdk.metrics.MetricPublisher;
import software.amazon.awssdk.metrics.NoOpMetricCollector;
import software.amazon.awssdk.protocols.core.ExceptionMetadata;
import software.amazon.awssdk.protocols.json.AwsJsonProtocol;
import software.amazon.awssdk.protocols.json.AwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.BaseAwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.JsonOperationMetadata;
import software.amazon.awssdk.services.transfer.model.AccessDeniedException;
import software.amazon.awssdk.services.transfer.model.ConflictException;
import software.amazon.awssdk.services.transfer.model.CreateServerRequest;
import software.amazon.awssdk.services.transfer.model.CreateServerResponse;
import software.amazon.awssdk.services.transfer.model.CreateUserRequest;
import software.amazon.awssdk.services.transfer.model.CreateUserResponse;
import software.amazon.awssdk.services.transfer.model.DeleteServerRequest;
import software.amazon.awssdk.services.transfer.model.DeleteServerResponse;
import software.amazon.awssdk.services.transfer.model.DeleteSshPublicKeyRequest;
import software.amazon.awssdk.services.transfer.model.DeleteSshPublicKeyResponse;
import software.amazon.awssdk.services.transfer.model.DeleteUserRequest;
import software.amazon.awssdk.services.transfer.model.DeleteUserResponse;
import software.amazon.awssdk.services.transfer.model.DescribeSecurityPolicyRequest;
import software.amazon.awssdk.services.transfer.model.DescribeSecurityPolicyResponse;
import software.amazon.awssdk.services.transfer.model.DescribeServerRequest;
import software.amazon.awssdk.services.transfer.model.DescribeServerResponse;
import software.amazon.awssdk.services.transfer.model.DescribeUserRequest;
import software.amazon.awssdk.services.transfer.model.DescribeUserResponse;
import software.amazon.awssdk.services.transfer.model.ImportSshPublicKeyRequest;
import software.amazon.awssdk.services.transfer.model.ImportSshPublicKeyResponse;
import software.amazon.awssdk.services.transfer.model.InternalServiceErrorException;
import software.amazon.awssdk.services.transfer.model.InvalidNextTokenException;
import software.amazon.awssdk.services.transfer.model.InvalidRequestException;
import software.amazon.awssdk.services.transfer.model.ListSecurityPoliciesRequest;
import software.amazon.awssdk.services.transfer.model.ListSecurityPoliciesResponse;
import software.amazon.awssdk.services.transfer.model.ListServersRequest;
import software.amazon.awssdk.services.transfer.model.ListServersResponse;
import software.amazon.awssdk.services.transfer.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.transfer.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.transfer.model.ListUsersRequest;
import software.amazon.awssdk.services.transfer.model.ListUsersResponse;
import software.amazon.awssdk.services.transfer.model.ResourceExistsException;
import software.amazon.awssdk.services.transfer.model.ResourceNotFoundException;
import software.amazon.awssdk.services.transfer.model.ServiceUnavailableException;
import software.amazon.awssdk.services.transfer.model.StartServerRequest;
import software.amazon.awssdk.services.transfer.model.StartServerResponse;
import software.amazon.awssdk.services.transfer.model.StopServerRequest;
import software.amazon.awssdk.services.transfer.model.StopServerResponse;
import software.amazon.awssdk.services.transfer.model.TagResourceRequest;
import software.amazon.awssdk.services.transfer.model.TagResourceResponse;
import software.amazon.awssdk.services.transfer.model.TestIdentityProviderRequest;
import software.amazon.awssdk.services.transfer.model.TestIdentityProviderResponse;
import software.amazon.awssdk.services.transfer.model.ThrottlingException;
import software.amazon.awssdk.services.transfer.model.TransferException;
import software.amazon.awssdk.services.transfer.model.TransferRequest;
import software.amazon.awssdk.services.transfer.model.UntagResourceRequest;
import software.amazon.awssdk.services.transfer.model.UntagResourceResponse;
import software.amazon.awssdk.services.transfer.model.UpdateServerRequest;
import software.amazon.awssdk.services.transfer.model.UpdateServerResponse;
import software.amazon.awssdk.services.transfer.model.UpdateUserRequest;
import software.amazon.awssdk.services.transfer.model.UpdateUserResponse;
import software.amazon.awssdk.services.transfer.paginators.ListSecurityPoliciesPublisher;
import software.amazon.awssdk.services.transfer.paginators.ListServersPublisher;
import software.amazon.awssdk.services.transfer.paginators.ListTagsForResourcePublisher;
import software.amazon.awssdk.services.transfer.paginators.ListUsersPublisher;
import software.amazon.awssdk.services.transfer.transform.CreateServerRequestMarshaller;
import software.amazon.awssdk.services.transfer.transform.CreateUserRequestMarshaller;
import software.amazon.awssdk.services.transfer.transform.DeleteServerRequestMarshaller;
import software.amazon.awssdk.services.transfer.transform.DeleteSshPublicKeyRequestMarshaller;
import software.amazon.awssdk.services.transfer.transform.DeleteUserRequestMarshaller;
import software.amazon.awssdk.services.transfer.transform.DescribeSecurityPolicyRequestMarshaller;
import software.amazon.awssdk.services.transfer.transform.DescribeServerRequestMarshaller;
import software.amazon.awssdk.services.transfer.transform.DescribeUserRequestMarshaller;
import software.amazon.awssdk.services.transfer.transform.ImportSshPublicKeyRequestMarshaller;
import software.amazon.awssdk.services.transfer.transform.ListSecurityPoliciesRequestMarshaller;
import software.amazon.awssdk.services.transfer.transform.ListServersRequestMarshaller;
import software.amazon.awssdk.services.transfer.transform.ListTagsForResourceRequestMarshaller;
import software.amazon.awssdk.services.transfer.transform.ListUsersRequestMarshaller;
import software.amazon.awssdk.services.transfer.transform.StartServerRequestMarshaller;
import software.amazon.awssdk.services.transfer.transform.StopServerRequestMarshaller;
import software.amazon.awssdk.services.transfer.transform.TagResourceRequestMarshaller;
import software.amazon.awssdk.services.transfer.transform.TestIdentityProviderRequestMarshaller;
import software.amazon.awssdk.services.transfer.transform.UntagResourceRequestMarshaller;
import software.amazon.awssdk.services.transfer.transform.UpdateServerRequestMarshaller;
import software.amazon.awssdk.services.transfer.transform.UpdateUserRequestMarshaller;
import software.amazon.awssdk.utils.CompletableFutureUtils;
/**
* Internal implementation of {@link TransferAsyncClient}.
*
* @see TransferAsyncClient#builder()
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
final class DefaultTransferAsyncClient implements TransferAsyncClient {
private static final Logger log = LoggerFactory.getLogger(DefaultTransferAsyncClient.class);
private final AsyncClientHandler clientHandler;
private final AwsJsonProtocolFactory protocolFactory;
private final SdkClientConfiguration clientConfiguration;
protected DefaultTransferAsyncClient(SdkClientConfiguration clientConfiguration) {
this.clientHandler = new AwsAsyncClientHandler(clientConfiguration);
this.clientConfiguration = clientConfiguration;
this.protocolFactory = init(AwsJsonProtocolFactory.builder()).build();
}
@Override
public final String serviceName() {
return SERVICE_NAME;
}
/**
*
* Instantiates an autoscaling virtual server based on the selected file transfer protocol in AWS. When you make
* updates to your file transfer protocol-enabled server or when you work with users, use the service-generated
* ServerId
property that is assigned to the newly created server.
*
*
* @param createServerRequest
* @return A Java Future containing the result of the CreateServer operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ServiceUnavailableException The request has failed because the AWS Transfer Family service is not
* available.
* - InternalServiceErrorException This exception is thrown when an error occurs in the AWS Transfer
* Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ResourceExistsException The requested resource does not exist.
* - ThrottlingException The request was denied due to request throttling.
*
* HTTP Status Code: 400
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.CreateServer
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture createServer(CreateServerRequest createServerRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, createServerRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transfer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateServer");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateServerResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateServer").withMarshaller(new CreateServerRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(createServerRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = createServerRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a user and associates them with an existing file transfer protocol-enabled server. You can only create
* and associate users with servers that have the IdentityProviderType
set to
* SERVICE_MANAGED
. Using parameters for CreateUser
, you can specify the user name, set
* the home directory, store the user's public key, and assign the user's AWS Identity and Access Management (IAM)
* role. You can also optionally add a scope-down policy, and assign metadata with tags that can be used to group
* and search for users.
*
*
* @param createUserRequest
* @return A Java Future containing the result of the CreateUser operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceUnavailableException The request has failed because the AWS Transfer Family service is not
* available.
* - InternalServiceErrorException This exception is thrown when an error occurs in the AWS Transfer
* Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ResourceExistsException The requested resource does not exist.
* - ResourceNotFoundException This exception is thrown when a resource is not found by the AWS Transfer
* Family service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.CreateUser
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture createUser(CreateUserRequest createUserRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, createUserRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transfer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateUser");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateUserResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("CreateUser")
.withMarshaller(new CreateUserRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(createUserRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = createUserRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes the file transfer protocol-enabled server that you specify.
*
*
* No response returns from this operation.
*
*
* @param deleteServerRequest
* @return A Java Future containing the result of the DeleteServer operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ServiceUnavailableException The request has failed because the AWS Transfer Family service is not
* available.
* - InternalServiceErrorException This exception is thrown when an error occurs in the AWS Transfer
* Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ResourceNotFoundException This exception is thrown when a resource is not found by the AWS Transfer
* Family service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.DeleteServer
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deleteServer(DeleteServerRequest deleteServerRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteServerRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transfer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteServer");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteServerResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteServer").withMarshaller(new DeleteServerRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(deleteServerRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = deleteServerRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes a user's Secure Shell (SSH) public key.
*
*
* No response is returned from this operation.
*
*
* @param deleteSshPublicKeyRequest
* @return A Java Future containing the result of the DeleteSshPublicKey operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceUnavailableException The request has failed because the AWS Transfer Family service is not
* available.
* - InternalServiceErrorException This exception is thrown when an error occurs in the AWS Transfer
* Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ResourceNotFoundException This exception is thrown when a resource is not found by the AWS Transfer
* Family service.
* - ThrottlingException The request was denied due to request throttling.
*
* HTTP Status Code: 400
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.DeleteSshPublicKey
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture deleteSshPublicKey(DeleteSshPublicKeyRequest deleteSshPublicKeyRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteSshPublicKeyRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transfer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteSshPublicKey");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteSshPublicKeyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteSshPublicKey")
.withMarshaller(new DeleteSshPublicKeyRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(deleteSshPublicKeyRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = deleteSshPublicKeyRequest.overrideConfiguration()
.orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes the user belonging to a file transfer protocol-enabled server you specify.
*
*
* No response returns from this operation.
*
*
*
* When you delete a user from a server, the user's information is lost.
*
*
*
* @param deleteUserRequest
* @return A Java Future containing the result of the DeleteUser operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceUnavailableException The request has failed because the AWS Transfer Family service is not
* available.
* - InternalServiceErrorException This exception is thrown when an error occurs in the AWS Transfer
* Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ResourceNotFoundException This exception is thrown when a resource is not found by the AWS Transfer
* Family service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.DeleteUser
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deleteUser(DeleteUserRequest deleteUserRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteUserRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transfer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteUser");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteUserResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("DeleteUser")
.withMarshaller(new DeleteUserRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(deleteUserRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = deleteUserRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Describes the security policy that is attached to your file transfer protocol-enabled server. The response
* contains a description of the security policy's properties. For more information about security policies, see Working with security
* policies.
*
*
* @param describeSecurityPolicyRequest
* @return A Java Future containing the result of the DescribeSecurityPolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceUnavailableException The request has failed because the AWS Transfer Family service is not
* available.
* - InternalServiceErrorException This exception is thrown when an error occurs in the AWS Transfer
* Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ResourceNotFoundException This exception is thrown when a resource is not found by the AWS Transfer
* Family service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.DescribeSecurityPolicy
* @see AWS API Documentation
*/
@Override
public CompletableFuture describeSecurityPolicy(
DescribeSecurityPolicyRequest describeSecurityPolicyRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeSecurityPolicyRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transfer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeSecurityPolicy");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeSecurityPolicyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeSecurityPolicy")
.withMarshaller(new DescribeSecurityPolicyRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(describeSecurityPolicyRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = describeSecurityPolicyRequest.overrideConfiguration().orElse(
null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Describes a file transfer protocol-enabled server that you specify by passing the ServerId
* parameter.
*
*
* The response contains a description of a server's properties. When you set EndpointType
to VPC, the
* response will contain the EndpointDetails
.
*
*
* @param describeServerRequest
* @return A Java Future containing the result of the DescribeServer operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceUnavailableException The request has failed because the AWS Transfer Family service is not
* available.
* - InternalServiceErrorException This exception is thrown when an error occurs in the AWS Transfer
* Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ResourceNotFoundException This exception is thrown when a resource is not found by the AWS Transfer
* Family service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.DescribeServer
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture describeServer(DescribeServerRequest describeServerRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeServerRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transfer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeServer");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeServerResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeServer")
.withMarshaller(new DescribeServerRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(describeServerRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = describeServerRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Describes the user assigned to the specific file transfer protocol-enabled server, as identified by its
* ServerId
property.
*
*
* The response from this call returns the properties of the user associated with the ServerId
value
* that was specified.
*
*
* @param describeUserRequest
* @return A Java Future containing the result of the DescribeUser operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceUnavailableException The request has failed because the AWS Transfer Family service is not
* available.
* - InternalServiceErrorException This exception is thrown when an error occurs in the AWS Transfer
* Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ResourceNotFoundException This exception is thrown when a resource is not found by the AWS Transfer
* Family service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.DescribeUser
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture describeUser(DescribeUserRequest describeUserRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeUserRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transfer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeUser");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DescribeUserResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeUser").withMarshaller(new DescribeUserRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(describeUserRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = describeUserRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Adds a Secure Shell (SSH) public key to a user account identified by a UserName
value assigned to
* the specific file transfer protocol-enabled server, identified by ServerId
.
*
*
* The response returns the UserName
value, the ServerId
value, and the name of the
* SshPublicKeyId
.
*
*
* @param importSshPublicKeyRequest
* @return A Java Future containing the result of the ImportSshPublicKey operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceUnavailableException The request has failed because the AWS Transfer Family service is not
* available.
* - InternalServiceErrorException This exception is thrown when an error occurs in the AWS Transfer
* Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ResourceExistsException The requested resource does not exist.
* - ResourceNotFoundException This exception is thrown when a resource is not found by the AWS Transfer
* Family service.
* - ThrottlingException The request was denied due to request throttling.
*
* HTTP Status Code: 400
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ImportSshPublicKey
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture importSshPublicKey(ImportSshPublicKeyRequest importSshPublicKeyRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, importSshPublicKeyRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transfer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ImportSshPublicKey");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ImportSshPublicKeyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ImportSshPublicKey")
.withMarshaller(new ImportSshPublicKeyRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(importSshPublicKeyRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = importSshPublicKeyRequest.overrideConfiguration()
.orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Lists the security policies that are attached to your file transfer protocol-enabled servers.
*
*
* @param listSecurityPoliciesRequest
* @return A Java Future containing the result of the ListSecurityPolicies operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceUnavailableException The request has failed because the AWS Transfer Family service is not
* available.
* - InternalServiceErrorException This exception is thrown when an error occurs in the AWS Transfer
* Family service.
* - InvalidNextTokenException The
NextToken
parameter that was passed is invalid.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ListSecurityPolicies
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture listSecurityPolicies(
ListSecurityPoliciesRequest listSecurityPoliciesRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, listSecurityPoliciesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transfer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListSecurityPolicies");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListSecurityPoliciesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListSecurityPolicies")
.withMarshaller(new ListSecurityPoliciesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(listSecurityPoliciesRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = listSecurityPoliciesRequest.overrideConfiguration().orElse(
null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Lists the security policies that are attached to your file transfer protocol-enabled servers.
*
*
*
* This is a variant of
* {@link #listSecurityPolicies(software.amazon.awssdk.services.transfer.model.ListSecurityPoliciesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.transfer.paginators.ListSecurityPoliciesPublisher publisher = client.listSecurityPoliciesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.transfer.paginators.ListSecurityPoliciesPublisher publisher = client.listSecurityPoliciesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.transfer.model.ListSecurityPoliciesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listSecurityPolicies(software.amazon.awssdk.services.transfer.model.ListSecurityPoliciesRequest)}
* operation.
*
*
* @param listSecurityPoliciesRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceUnavailableException The request has failed because the AWS Transfer Family service is not
* available.
* - InternalServiceErrorException This exception is thrown when an error occurs in the AWS Transfer
* Family service.
* - InvalidNextTokenException The
NextToken
parameter that was passed is invalid.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ListSecurityPolicies
* @see AWS
* API Documentation
*/
public ListSecurityPoliciesPublisher listSecurityPoliciesPaginator(ListSecurityPoliciesRequest listSecurityPoliciesRequest) {
return new ListSecurityPoliciesPublisher(this, applyPaginatorUserAgent(listSecurityPoliciesRequest));
}
/**
*
* Lists the file transfer protocol-enabled servers that are associated with your AWS account.
*
*
* @param listServersRequest
* @return A Java Future containing the result of the ListServers operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceUnavailableException The request has failed because the AWS Transfer Family service is not
* available.
* - InternalServiceErrorException This exception is thrown when an error occurs in the AWS Transfer
* Family service.
* - InvalidNextTokenException The
NextToken
parameter that was passed is invalid.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ListServers
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture listServers(ListServersRequest listServersRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, listServersRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transfer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListServers");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListServersResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListServers").withMarshaller(new ListServersRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(listServersRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = listServersRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Lists the file transfer protocol-enabled servers that are associated with your AWS account.
*
*
*
* This is a variant of {@link #listServers(software.amazon.awssdk.services.transfer.model.ListServersRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.transfer.paginators.ListServersPublisher publisher = client.listServersPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.transfer.paginators.ListServersPublisher publisher = client.listServersPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.transfer.model.ListServersResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listServers(software.amazon.awssdk.services.transfer.model.ListServersRequest)} operation.
*
*
* @param listServersRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceUnavailableException The request has failed because the AWS Transfer Family service is not
* available.
* - InternalServiceErrorException This exception is thrown when an error occurs in the AWS Transfer
* Family service.
* - InvalidNextTokenException The
NextToken
parameter that was passed is invalid.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ListServers
* @see AWS API
* Documentation
*/
public ListServersPublisher listServersPaginator(ListServersRequest listServersRequest) {
return new ListServersPublisher(this, applyPaginatorUserAgent(listServersRequest));
}
/**
*
* Lists all of the tags associated with the Amazon Resource Number (ARN) you specify. The resource can be a user,
* server, or role.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceUnavailableException The request has failed because the AWS Transfer Family service is not
* available.
* - InternalServiceErrorException This exception is thrown when an error occurs in the AWS Transfer
* Family service.
* - InvalidNextTokenException The
NextToken
parameter that was passed is invalid.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ListTagsForResource
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture listTagsForResource(
ListTagsForResourceRequest listTagsForResourceRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, listTagsForResourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transfer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListTagsForResource");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListTagsForResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListTagsForResource")
.withMarshaller(new ListTagsForResourceRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(listTagsForResourceRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = listTagsForResourceRequest.overrideConfiguration().orElse(
null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Lists all of the tags associated with the Amazon Resource Number (ARN) you specify. The resource can be a user,
* server, or role.
*
*
*
* This is a variant of
* {@link #listTagsForResource(software.amazon.awssdk.services.transfer.model.ListTagsForResourceRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.transfer.paginators.ListTagsForResourcePublisher publisher = client.listTagsForResourcePaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.transfer.paginators.ListTagsForResourcePublisher publisher = client.listTagsForResourcePaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.transfer.model.ListTagsForResourceResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listTagsForResource(software.amazon.awssdk.services.transfer.model.ListTagsForResourceRequest)}
* operation.
*
*
* @param listTagsForResourceRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceUnavailableException The request has failed because the AWS Transfer Family service is not
* available.
* - InternalServiceErrorException This exception is thrown when an error occurs in the AWS Transfer
* Family service.
* - InvalidNextTokenException The
NextToken
parameter that was passed is invalid.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ListTagsForResource
* @see AWS
* API Documentation
*/
public ListTagsForResourcePublisher listTagsForResourcePaginator(ListTagsForResourceRequest listTagsForResourceRequest) {
return new ListTagsForResourcePublisher(this, applyPaginatorUserAgent(listTagsForResourceRequest));
}
/**
*
* Lists the users for a file transfer protocol-enabled server that you specify by passing the ServerId
* parameter.
*
*
* @param listUsersRequest
* @return A Java Future containing the result of the ListUsers operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceUnavailableException The request has failed because the AWS Transfer Family service is not
* available.
* - InternalServiceErrorException This exception is thrown when an error occurs in the AWS Transfer
* Family service.
* - InvalidNextTokenException The
NextToken
parameter that was passed is invalid.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ResourceNotFoundException This exception is thrown when a resource is not found by the AWS Transfer
* Family service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ListUsers
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture listUsers(ListUsersRequest listUsersRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, listUsersRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transfer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListUsers");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListUsersResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("ListUsers")
.withMarshaller(new ListUsersRequestMarshaller(protocolFactory)).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withMetricCollector(apiCallMetricCollector)
.withInput(listUsersRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = listUsersRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Lists the users for a file transfer protocol-enabled server that you specify by passing the ServerId
* parameter.
*
*
*
* This is a variant of {@link #listUsers(software.amazon.awssdk.services.transfer.model.ListUsersRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.transfer.paginators.ListUsersPublisher publisher = client.listUsersPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.transfer.paginators.ListUsersPublisher publisher = client.listUsersPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.transfer.model.ListUsersResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listUsers(software.amazon.awssdk.services.transfer.model.ListUsersRequest)} operation.
*
*
* @param listUsersRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceUnavailableException The request has failed because the AWS Transfer Family service is not
* available.
* - InternalServiceErrorException This exception is thrown when an error occurs in the AWS Transfer
* Family service.
* - InvalidNextTokenException The
NextToken
parameter that was passed is invalid.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ResourceNotFoundException This exception is thrown when a resource is not found by the AWS Transfer
* Family service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ListUsers
* @see AWS API
* Documentation
*/
public ListUsersPublisher listUsersPaginator(ListUsersRequest listUsersRequest) {
return new ListUsersPublisher(this, applyPaginatorUserAgent(listUsersRequest));
}
/**
*
* Changes the state of a file transfer protocol-enabled server from OFFLINE
to ONLINE
. It
* has no impact on a server that is already ONLINE
. An ONLINE
server can accept and
* process file transfer jobs.
*
*
* The state of STARTING
indicates that the server is in an intermediate state, either not fully able
* to respond, or not fully online. The values of START_FAILED
can indicate an error condition.
*
*
* No response is returned from this call.
*
*
* @param startServerRequest
* @return A Java Future containing the result of the StartServer operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceUnavailableException The request has failed because the AWS Transfer Family service is not
* available.
* - InternalServiceErrorException This exception is thrown when an error occurs in the AWS Transfer
* Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ResourceNotFoundException This exception is thrown when a resource is not found by the AWS Transfer
* Family service.
* - ThrottlingException The request was denied due to request throttling.
*
* HTTP Status Code: 400
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.StartServer
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture startServer(StartServerRequest startServerRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, startServerRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transfer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "StartServer");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
StartServerResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("StartServer").withMarshaller(new StartServerRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(startServerRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = startServerRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Changes the state of a file transfer protocol-enabled server from ONLINE
to OFFLINE
. An
* OFFLINE
server cannot accept and process file transfer jobs. Information tied to your server, such
* as server and user properties, are not affected by stopping your server.
*
*
*
* Stopping the server will not reduce or impact your file transfer protocol endpoint billing; you must delete the
* server to stop being billed.
*
*
*
* The state of STOPPING
indicates that the server is in an intermediate state, either not fully able
* to respond, or not fully offline. The values of STOP_FAILED
can indicate an error condition.
*
*
* No response is returned from this call.
*
*
* @param stopServerRequest
* @return A Java Future containing the result of the StopServer operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceUnavailableException The request has failed because the AWS Transfer Family service is not
* available.
* - InternalServiceErrorException This exception is thrown when an error occurs in the AWS Transfer
* Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ResourceNotFoundException This exception is thrown when a resource is not found by the AWS Transfer
* Family service.
* - ThrottlingException The request was denied due to request throttling.
*
* HTTP Status Code: 400
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.StopServer
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture stopServer(StopServerRequest stopServerRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, stopServerRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transfer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "StopServer");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
StopServerResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("StopServer")
.withMarshaller(new StopServerRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(stopServerRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = stopServerRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Attaches a key-value pair to a resource, as identified by its Amazon Resource Name (ARN). Resources are users,
* servers, roles, and other entities.
*
*
* There is no response returned from this call.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceUnavailableException The request has failed because the AWS Transfer Family service is not
* available.
* - InternalServiceErrorException This exception is thrown when an error occurs in the AWS Transfer
* Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ResourceNotFoundException This exception is thrown when a resource is not found by the AWS Transfer
* Family service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.TagResource
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture tagResource(TagResourceRequest tagResourceRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, tagResourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transfer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "TagResource");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
TagResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("TagResource").withMarshaller(new TagResourceRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(tagResourceRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = tagResourceRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* If the IdentityProviderType
of a file transfer protocol-enabled server is API_Gateway
,
* tests whether your API Gateway is set up successfully. We highly recommend that you call this operation to test
* your authentication method as soon as you create your server. By doing so, you can troubleshoot issues with the
* API Gateway integration to ensure that your users can successfully use the service.
*
*
* @param testIdentityProviderRequest
* @return A Java Future containing the result of the TestIdentityProvider operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceUnavailableException The request has failed because the AWS Transfer Family service is not
* available.
* - InternalServiceErrorException This exception is thrown when an error occurs in the AWS Transfer
* Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ResourceNotFoundException This exception is thrown when a resource is not found by the AWS Transfer
* Family service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.TestIdentityProvider
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture testIdentityProvider(
TestIdentityProviderRequest testIdentityProviderRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, testIdentityProviderRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transfer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "TestIdentityProvider");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, TestIdentityProviderResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("TestIdentityProvider")
.withMarshaller(new TestIdentityProviderRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(testIdentityProviderRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = testIdentityProviderRequest.overrideConfiguration().orElse(
null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Detaches a key-value pair from a resource, as identified by its Amazon Resource Name (ARN). Resources are users,
* servers, roles, and other entities.
*
*
* No response is returned from this call.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceUnavailableException The request has failed because the AWS Transfer Family service is not
* available.
* - InternalServiceErrorException This exception is thrown when an error occurs in the AWS Transfer
* Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ResourceNotFoundException This exception is thrown when a resource is not found by the AWS Transfer
* Family service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.UntagResource
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture untagResource(UntagResourceRequest untagResourceRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, untagResourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transfer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UntagResource");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
UntagResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("UntagResource")
.withMarshaller(new UntagResourceRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(untagResourceRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = untagResourceRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Updates the file transfer protocol-enabled server's properties after that server has been created.
*
*
* The UpdateServer
call returns the ServerId
of the server you updated.
*
*
* @param updateServerRequest
* @return A Java Future containing the result of the UpdateServer operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ServiceUnavailableException The request has failed because the AWS Transfer Family service is not
* available.
* - ConflictException This exception is thrown when the
UpdatServer
is called for a file
* transfer protocol-enabled server that has VPC as the endpoint type and the server's
* VpcEndpointID
is not in the available state.
* - InternalServiceErrorException This exception is thrown when an error occurs in the AWS Transfer
* Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ResourceExistsException The requested resource does not exist.
* - ResourceNotFoundException This exception is thrown when a resource is not found by the AWS Transfer
* Family service.
* - ThrottlingException The request was denied due to request throttling.
*
* HTTP Status Code: 400
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.UpdateServer
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture updateServer(UpdateServerRequest updateServerRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, updateServerRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transfer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UpdateServer");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
UpdateServerResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("UpdateServer").withMarshaller(new UpdateServerRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(updateServerRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = updateServerRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Assigns new properties to a user. Parameters you pass modify any or all of the following: the home directory,
* role, and policy for the UserName
and ServerId
you specify.
*
*
* The response returns the ServerId
and the UserName
for the updated user.
*
*
* @param updateUserRequest
* @return A Java Future containing the result of the UpdateUser operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceUnavailableException The request has failed because the AWS Transfer Family service is not
* available.
* - InternalServiceErrorException This exception is thrown when an error occurs in the AWS Transfer
* Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ResourceNotFoundException This exception is thrown when a resource is not found by the AWS Transfer
* Family service.
* - ThrottlingException The request was denied due to request throttling.
*
* HTTP Status Code: 400
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.UpdateUser
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture updateUser(UpdateUserRequest updateUserRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, updateUserRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transfer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UpdateUser");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
UpdateUserResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("UpdateUser")
.withMarshaller(new UpdateUserRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(updateUserRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = updateUserRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
@Override
public void close() {
clientHandler.close();
}
private > T init(T builder) {
return builder
.clientConfiguration(clientConfiguration)
.defaultServiceExceptionSupplier(TransferException::builder)
.protocol(AwsJsonProtocol.AWS_JSON)
.protocolVersion("1.1")
.registerModeledException(
ExceptionMetadata.builder().errorCode("AccessDeniedException")
.exceptionBuilderSupplier(AccessDeniedException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ConflictException")
.exceptionBuilderSupplier(ConflictException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidRequestException")
.exceptionBuilderSupplier(InvalidRequestException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ResourceNotFoundException")
.exceptionBuilderSupplier(ResourceNotFoundException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ThrottlingException")
.exceptionBuilderSupplier(ThrottlingException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ServiceUnavailableException")
.exceptionBuilderSupplier(ServiceUnavailableException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InternalServiceError")
.exceptionBuilderSupplier(InternalServiceErrorException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ResourceExistsException")
.exceptionBuilderSupplier(ResourceExistsException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidNextTokenException")
.exceptionBuilderSupplier(InvalidNextTokenException::builder).build());
}
private static List resolveMetricPublishers(SdkClientConfiguration clientConfiguration,
RequestOverrideConfiguration requestOverrideConfiguration) {
List publishers = null;
if (requestOverrideConfiguration != null) {
publishers = requestOverrideConfiguration.metricPublishers();
}
if (publishers == null || publishers.isEmpty()) {
publishers = clientConfiguration.option(SdkClientOption.METRIC_PUBLISHERS);
}
if (publishers == null) {
publishers = Collections.emptyList();
}
return publishers;
}
private T applyPaginatorUserAgent(T request) {
Consumer userAgentApplier = b -> b.addApiName(ApiName.builder()
.version(VersionInfo.SDK_VERSION).name("PAGINATED").build());
AwsRequestOverrideConfiguration overrideConfiguration = request.overrideConfiguration()
.map(c -> c.toBuilder().applyMutation(userAgentApplier).build())
.orElse((AwsRequestOverrideConfiguration.builder().applyMutation(userAgentApplier).build()));
return (T) request.toBuilder().overrideConfiguration(overrideConfiguration).build();
}
private HttpResponseHandler createErrorResponseHandler(BaseAwsJsonProtocolFactory protocolFactory,
JsonOperationMetadata operationMetadata) {
return protocolFactory.createErrorResponseHandler(operationMetadata);
}
}