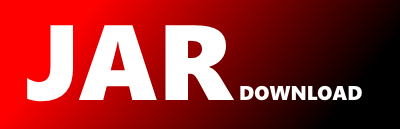
software.amazon.awssdk.services.transfer.model.ProtocolDetails Maven / Gradle / Ivy
Show all versions of transfer Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.transfer.model;
import java.beans.Transient;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The protocol settings that are configured for your server.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ProtocolDetails implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField PASSIVE_IP_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("PassiveIp").getter(getter(ProtocolDetails::passiveIp)).setter(setter(Builder::passiveIp))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PassiveIp").build()).build();
private static final SdkField TLS_SESSION_RESUMPTION_MODE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TlsSessionResumptionMode").getter(getter(ProtocolDetails::tlsSessionResumptionModeAsString))
.setter(setter(Builder::tlsSessionResumptionMode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TlsSessionResumptionMode").build())
.build();
private static final SdkField SET_STAT_OPTION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SetStatOption").getter(getter(ProtocolDetails::setStatOptionAsString))
.setter(setter(Builder::setStatOption))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SetStatOption").build()).build();
private static final SdkField> AS2_TRANSPORTS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("As2Transports")
.getter(getter(ProtocolDetails::as2TransportsAsStrings))
.setter(setter(Builder::as2TransportsWithStrings))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("As2Transports").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(PASSIVE_IP_FIELD,
TLS_SESSION_RESUMPTION_MODE_FIELD, SET_STAT_OPTION_FIELD, AS2_TRANSPORTS_FIELD));
private static final long serialVersionUID = 1L;
private final String passiveIp;
private final String tlsSessionResumptionMode;
private final String setStatOption;
private final List as2Transports;
private ProtocolDetails(BuilderImpl builder) {
this.passiveIp = builder.passiveIp;
this.tlsSessionResumptionMode = builder.tlsSessionResumptionMode;
this.setStatOption = builder.setStatOption;
this.as2Transports = builder.as2Transports;
}
/**
*
* Indicates passive mode, for FTP and FTPS protocols. Enter a single IPv4 address, such as the public IP address of
* a firewall, router, or load balancer. For example:
*
*
* aws transfer update-server --protocol-details PassiveIp=0.0.0.0
*
*
* Replace 0.0.0.0
in the example above with the actual IP address you want to use.
*
*
*
* If you change the PassiveIp
value, you must stop and then restart your Transfer Family server for
* the change to take effect. For details on using passive mode (PASV) in a NAT environment, see Configuring your FTPS server behind a firewall or NAT with Transfer Family.
*
*
*
* Special values
*
*
* The AUTO
and 0.0.0.0
are special values for the PassiveIp
parameter. The
* value PassiveIp=AUTO
is assigned by default to FTP and FTPS type servers. In this case, the server
* automatically responds with one of the endpoint IPs within the PASV response. PassiveIp=0.0.0.0
has
* a more unique application for its usage. For example, if you have a High Availability (HA) Network Load Balancer
* (NLB) environment, where you have 3 subnets, you can only specify a single IP address using the
* PassiveIp
parameter. This reduces the effectiveness of having High Availability. In this case, you
* can specify PassiveIp=0.0.0.0
. This tells the client to use the same IP address as the Control
* connection and utilize all AZs for their connections. Note, however, that not all FTP clients support the
* PassiveIp=0.0.0.0
response. FileZilla and WinSCP do support it. If you are using other clients,
* check to see if your client supports the PassiveIp=0.0.0.0
response.
*
*
* @return Indicates passive mode, for FTP and FTPS protocols. Enter a single IPv4 address, such as the public IP
* address of a firewall, router, or load balancer. For example:
*
* aws transfer update-server --protocol-details PassiveIp=0.0.0.0
*
*
* Replace 0.0.0.0
in the example above with the actual IP address you want to use.
*
*
*
* If you change the PassiveIp
value, you must stop and then restart your Transfer Family
* server for the change to take effect. For details on using passive mode (PASV) in a NAT environment, see
* Configuring your FTPS server behind a firewall or NAT with Transfer Family.
*
*
*
* Special values
*
*
* The AUTO
and 0.0.0.0
are special values for the PassiveIp
* parameter. The value PassiveIp=AUTO
is assigned by default to FTP and FTPS type servers. In
* this case, the server automatically responds with one of the endpoint IPs within the PASV response.
* PassiveIp=0.0.0.0
has a more unique application for its usage. For example, if you have a
* High Availability (HA) Network Load Balancer (NLB) environment, where you have 3 subnets, you can only
* specify a single IP address using the PassiveIp
parameter. This reduces the effectiveness of
* having High Availability. In this case, you can specify PassiveIp=0.0.0.0
. This tells the
* client to use the same IP address as the Control connection and utilize all AZs for their connections.
* Note, however, that not all FTP clients support the PassiveIp=0.0.0.0
response. FileZilla
* and WinSCP do support it. If you are using other clients, check to see if your client supports the
* PassiveIp=0.0.0.0
response.
*/
public final String passiveIp() {
return passiveIp;
}
/**
*
* A property used with Transfer Family servers that use the FTPS protocol. TLS Session Resumption provides a
* mechanism to resume or share a negotiated secret key between the control and data connection for an FTPS session.
* TlsSessionResumptionMode
determines whether or not the server resumes recent, negotiated sessions
* through a unique session ID. This property is available during CreateServer
and
* UpdateServer
calls. If a TlsSessionResumptionMode
value is not specified during
* CreateServer
, it is set to ENFORCED
by default.
*
*
* -
*
* DISABLED
: the server does not process TLS session resumption client requests and creates a new TLS
* session for each request.
*
*
* -
*
* ENABLED
: the server processes and accepts clients that are performing TLS session resumption. The
* server doesn't reject client data connections that do not perform the TLS session resumption client processing.
*
*
* -
*
* ENFORCED
: the server processes and accepts clients that are performing TLS session resumption. The
* server rejects client data connections that do not perform the TLS session resumption client processing. Before
* you set the value to ENFORCED
, test your clients.
*
*
*
* Not all FTPS clients perform TLS session resumption. So, if you choose to enforce TLS session resumption, you
* prevent any connections from FTPS clients that don't perform the protocol negotiation. To determine whether or
* not you can use the ENFORCED
value, you need to test your clients.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #tlsSessionResumptionMode} will return {@link TlsSessionResumptionMode#UNKNOWN_TO_SDK_VERSION}. The raw
* value returned by the service is available from {@link #tlsSessionResumptionModeAsString}.
*
*
* @return A property used with Transfer Family servers that use the FTPS protocol. TLS Session Resumption provides
* a mechanism to resume or share a negotiated secret key between the control and data connection for an
* FTPS session. TlsSessionResumptionMode
determines whether or not the server resumes recent,
* negotiated sessions through a unique session ID. This property is available during
* CreateServer
and UpdateServer
calls. If a TlsSessionResumptionMode
* value is not specified during CreateServer
, it is set to ENFORCED
by
* default.
*
* -
*
* DISABLED
: the server does not process TLS session resumption client requests and creates a
* new TLS session for each request.
*
*
* -
*
* ENABLED
: the server processes and accepts clients that are performing TLS session
* resumption. The server doesn't reject client data connections that do not perform the TLS session
* resumption client processing.
*
*
* -
*
* ENFORCED
: the server processes and accepts clients that are performing TLS session
* resumption. The server rejects client data connections that do not perform the TLS session resumption
* client processing. Before you set the value to ENFORCED
, test your clients.
*
*
*
* Not all FTPS clients perform TLS session resumption. So, if you choose to enforce TLS session resumption,
* you prevent any connections from FTPS clients that don't perform the protocol negotiation. To determine
* whether or not you can use the ENFORCED
value, you need to test your clients.
*
*
* @see TlsSessionResumptionMode
*/
public final TlsSessionResumptionMode tlsSessionResumptionMode() {
return TlsSessionResumptionMode.fromValue(tlsSessionResumptionMode);
}
/**
*
* A property used with Transfer Family servers that use the FTPS protocol. TLS Session Resumption provides a
* mechanism to resume or share a negotiated secret key between the control and data connection for an FTPS session.
* TlsSessionResumptionMode
determines whether or not the server resumes recent, negotiated sessions
* through a unique session ID. This property is available during CreateServer
and
* UpdateServer
calls. If a TlsSessionResumptionMode
value is not specified during
* CreateServer
, it is set to ENFORCED
by default.
*
*
* -
*
* DISABLED
: the server does not process TLS session resumption client requests and creates a new TLS
* session for each request.
*
*
* -
*
* ENABLED
: the server processes and accepts clients that are performing TLS session resumption. The
* server doesn't reject client data connections that do not perform the TLS session resumption client processing.
*
*
* -
*
* ENFORCED
: the server processes and accepts clients that are performing TLS session resumption. The
* server rejects client data connections that do not perform the TLS session resumption client processing. Before
* you set the value to ENFORCED
, test your clients.
*
*
*
* Not all FTPS clients perform TLS session resumption. So, if you choose to enforce TLS session resumption, you
* prevent any connections from FTPS clients that don't perform the protocol negotiation. To determine whether or
* not you can use the ENFORCED
value, you need to test your clients.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #tlsSessionResumptionMode} will return {@link TlsSessionResumptionMode#UNKNOWN_TO_SDK_VERSION}. The raw
* value returned by the service is available from {@link #tlsSessionResumptionModeAsString}.
*
*
* @return A property used with Transfer Family servers that use the FTPS protocol. TLS Session Resumption provides
* a mechanism to resume or share a negotiated secret key between the control and data connection for an
* FTPS session. TlsSessionResumptionMode
determines whether or not the server resumes recent,
* negotiated sessions through a unique session ID. This property is available during
* CreateServer
and UpdateServer
calls. If a TlsSessionResumptionMode
* value is not specified during CreateServer
, it is set to ENFORCED
by
* default.
*
* -
*
* DISABLED
: the server does not process TLS session resumption client requests and creates a
* new TLS session for each request.
*
*
* -
*
* ENABLED
: the server processes and accepts clients that are performing TLS session
* resumption. The server doesn't reject client data connections that do not perform the TLS session
* resumption client processing.
*
*
* -
*
* ENFORCED
: the server processes and accepts clients that are performing TLS session
* resumption. The server rejects client data connections that do not perform the TLS session resumption
* client processing. Before you set the value to ENFORCED
, test your clients.
*
*
*
* Not all FTPS clients perform TLS session resumption. So, if you choose to enforce TLS session resumption,
* you prevent any connections from FTPS clients that don't perform the protocol negotiation. To determine
* whether or not you can use the ENFORCED
value, you need to test your clients.
*
*
* @see TlsSessionResumptionMode
*/
public final String tlsSessionResumptionModeAsString() {
return tlsSessionResumptionMode;
}
/**
*
* Use the SetStatOption
to ignore the error that is generated when the client attempts to use
* SETSTAT
on a file you are uploading to an S3 bucket.
*
*
* Some SFTP file transfer clients can attempt to change the attributes of remote files, including timestamp and
* permissions, using commands, such as SETSTAT
when uploading the file. However, these commands are
* not compatible with object storage systems, such as Amazon S3. Due to this incompatibility, file uploads from
* these clients can result in errors even when the file is otherwise successfully uploaded.
*
*
* Set the value to ENABLE_NO_OP
to have the Transfer Family server ignore the SETSTAT
* command, and upload files without needing to make any changes to your SFTP client. While the
* SetStatOption
ENABLE_NO_OP
setting ignores the error, it does generate a log entry in
* Amazon CloudWatch Logs, so you can determine when the client is making a SETSTAT
call.
*
*
*
* If you want to preserve the original timestamp for your file, and modify other file attributes using
* SETSTAT
, you can use Amazon EFS as backend storage with Transfer Family.
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #setStatOption}
* will return {@link SetStatOption#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #setStatOptionAsString}.
*
*
* @return Use the SetStatOption
to ignore the error that is generated when the client attempts to use
* SETSTAT
on a file you are uploading to an S3 bucket.
*
* Some SFTP file transfer clients can attempt to change the attributes of remote files, including timestamp
* and permissions, using commands, such as SETSTAT
when uploading the file. However, these
* commands are not compatible with object storage systems, such as Amazon S3. Due to this incompatibility,
* file uploads from these clients can result in errors even when the file is otherwise successfully
* uploaded.
*
*
* Set the value to ENABLE_NO_OP
to have the Transfer Family server ignore the
* SETSTAT
command, and upload files without needing to make any changes to your SFTP client.
* While the SetStatOption
ENABLE_NO_OP
setting ignores the error, it does
* generate a log entry in Amazon CloudWatch Logs, so you can determine when the client is making a
* SETSTAT
call.
*
*
*
* If you want to preserve the original timestamp for your file, and modify other file attributes using
* SETSTAT
, you can use Amazon EFS as backend storage with Transfer Family.
*
* @see SetStatOption
*/
public final SetStatOption setStatOption() {
return SetStatOption.fromValue(setStatOption);
}
/**
*
* Use the SetStatOption
to ignore the error that is generated when the client attempts to use
* SETSTAT
on a file you are uploading to an S3 bucket.
*
*
* Some SFTP file transfer clients can attempt to change the attributes of remote files, including timestamp and
* permissions, using commands, such as SETSTAT
when uploading the file. However, these commands are
* not compatible with object storage systems, such as Amazon S3. Due to this incompatibility, file uploads from
* these clients can result in errors even when the file is otherwise successfully uploaded.
*
*
* Set the value to ENABLE_NO_OP
to have the Transfer Family server ignore the SETSTAT
* command, and upload files without needing to make any changes to your SFTP client. While the
* SetStatOption
ENABLE_NO_OP
setting ignores the error, it does generate a log entry in
* Amazon CloudWatch Logs, so you can determine when the client is making a SETSTAT
call.
*
*
*
* If you want to preserve the original timestamp for your file, and modify other file attributes using
* SETSTAT
, you can use Amazon EFS as backend storage with Transfer Family.
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #setStatOption}
* will return {@link SetStatOption#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #setStatOptionAsString}.
*
*
* @return Use the SetStatOption
to ignore the error that is generated when the client attempts to use
* SETSTAT
on a file you are uploading to an S3 bucket.
*
* Some SFTP file transfer clients can attempt to change the attributes of remote files, including timestamp
* and permissions, using commands, such as SETSTAT
when uploading the file. However, these
* commands are not compatible with object storage systems, such as Amazon S3. Due to this incompatibility,
* file uploads from these clients can result in errors even when the file is otherwise successfully
* uploaded.
*
*
* Set the value to ENABLE_NO_OP
to have the Transfer Family server ignore the
* SETSTAT
command, and upload files without needing to make any changes to your SFTP client.
* While the SetStatOption
ENABLE_NO_OP
setting ignores the error, it does
* generate a log entry in Amazon CloudWatch Logs, so you can determine when the client is making a
* SETSTAT
call.
*
*
*
* If you want to preserve the original timestamp for your file, and modify other file attributes using
* SETSTAT
, you can use Amazon EFS as backend storage with Transfer Family.
*
* @see SetStatOption
*/
public final String setStatOptionAsString() {
return setStatOption;
}
/**
*
* Indicates the transport method for the AS2 messages. Currently, only HTTP is supported.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasAs2Transports} method.
*
*
* @return Indicates the transport method for the AS2 messages. Currently, only HTTP is supported.
*/
public final List as2Transports() {
return As2TransportsCopier.copyStringToEnum(as2Transports);
}
/**
* For responses, this returns true if the service returned a value for the As2Transports property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasAs2Transports() {
return as2Transports != null && !(as2Transports instanceof SdkAutoConstructList);
}
/**
*
* Indicates the transport method for the AS2 messages. Currently, only HTTP is supported.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasAs2Transports} method.
*
*
* @return Indicates the transport method for the AS2 messages. Currently, only HTTP is supported.
*/
public final List as2TransportsAsStrings() {
return as2Transports;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(passiveIp());
hashCode = 31 * hashCode + Objects.hashCode(tlsSessionResumptionModeAsString());
hashCode = 31 * hashCode + Objects.hashCode(setStatOptionAsString());
hashCode = 31 * hashCode + Objects.hashCode(hasAs2Transports() ? as2TransportsAsStrings() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ProtocolDetails)) {
return false;
}
ProtocolDetails other = (ProtocolDetails) obj;
return Objects.equals(passiveIp(), other.passiveIp())
&& Objects.equals(tlsSessionResumptionModeAsString(), other.tlsSessionResumptionModeAsString())
&& Objects.equals(setStatOptionAsString(), other.setStatOptionAsString())
&& hasAs2Transports() == other.hasAs2Transports()
&& Objects.equals(as2TransportsAsStrings(), other.as2TransportsAsStrings());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ProtocolDetails").add("PassiveIp", passiveIp())
.add("TlsSessionResumptionMode", tlsSessionResumptionModeAsString())
.add("SetStatOption", setStatOptionAsString())
.add("As2Transports", hasAs2Transports() ? as2TransportsAsStrings() : null).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "PassiveIp":
return Optional.ofNullable(clazz.cast(passiveIp()));
case "TlsSessionResumptionMode":
return Optional.ofNullable(clazz.cast(tlsSessionResumptionModeAsString()));
case "SetStatOption":
return Optional.ofNullable(clazz.cast(setStatOptionAsString()));
case "As2Transports":
return Optional.ofNullable(clazz.cast(as2TransportsAsStrings()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function