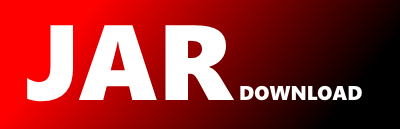
software.amazon.awssdk.services.transfer.DefaultTransferAsyncClient Maven / Gradle / Ivy
Show all versions of transfer Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.transfer;
import java.util.Collections;
import java.util.List;
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.ScheduledExecutorService;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.awscore.client.handler.AwsAsyncClientHandler;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.awscore.internal.AwsProtocolMetadata;
import software.amazon.awssdk.awscore.internal.AwsServiceProtocol;
import software.amazon.awssdk.core.RequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkPlugin;
import software.amazon.awssdk.core.SdkRequest;
import software.amazon.awssdk.core.client.config.SdkClientConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientOption;
import software.amazon.awssdk.core.client.handler.AsyncClientHandler;
import software.amazon.awssdk.core.client.handler.ClientExecutionParams;
import software.amazon.awssdk.core.http.HttpResponseHandler;
import software.amazon.awssdk.core.metrics.CoreMetric;
import software.amazon.awssdk.metrics.MetricCollector;
import software.amazon.awssdk.metrics.MetricPublisher;
import software.amazon.awssdk.metrics.NoOpMetricCollector;
import software.amazon.awssdk.protocols.core.ExceptionMetadata;
import software.amazon.awssdk.protocols.json.AwsJsonProtocol;
import software.amazon.awssdk.protocols.json.AwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.BaseAwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.JsonOperationMetadata;
import software.amazon.awssdk.services.transfer.internal.TransferServiceClientConfigurationBuilder;
import software.amazon.awssdk.services.transfer.model.AccessDeniedException;
import software.amazon.awssdk.services.transfer.model.ConflictException;
import software.amazon.awssdk.services.transfer.model.CreateAccessRequest;
import software.amazon.awssdk.services.transfer.model.CreateAccessResponse;
import software.amazon.awssdk.services.transfer.model.CreateAgreementRequest;
import software.amazon.awssdk.services.transfer.model.CreateAgreementResponse;
import software.amazon.awssdk.services.transfer.model.CreateConnectorRequest;
import software.amazon.awssdk.services.transfer.model.CreateConnectorResponse;
import software.amazon.awssdk.services.transfer.model.CreateProfileRequest;
import software.amazon.awssdk.services.transfer.model.CreateProfileResponse;
import software.amazon.awssdk.services.transfer.model.CreateServerRequest;
import software.amazon.awssdk.services.transfer.model.CreateServerResponse;
import software.amazon.awssdk.services.transfer.model.CreateUserRequest;
import software.amazon.awssdk.services.transfer.model.CreateUserResponse;
import software.amazon.awssdk.services.transfer.model.CreateWorkflowRequest;
import software.amazon.awssdk.services.transfer.model.CreateWorkflowResponse;
import software.amazon.awssdk.services.transfer.model.DeleteAccessRequest;
import software.amazon.awssdk.services.transfer.model.DeleteAccessResponse;
import software.amazon.awssdk.services.transfer.model.DeleteAgreementRequest;
import software.amazon.awssdk.services.transfer.model.DeleteAgreementResponse;
import software.amazon.awssdk.services.transfer.model.DeleteCertificateRequest;
import software.amazon.awssdk.services.transfer.model.DeleteCertificateResponse;
import software.amazon.awssdk.services.transfer.model.DeleteConnectorRequest;
import software.amazon.awssdk.services.transfer.model.DeleteConnectorResponse;
import software.amazon.awssdk.services.transfer.model.DeleteHostKeyRequest;
import software.amazon.awssdk.services.transfer.model.DeleteHostKeyResponse;
import software.amazon.awssdk.services.transfer.model.DeleteProfileRequest;
import software.amazon.awssdk.services.transfer.model.DeleteProfileResponse;
import software.amazon.awssdk.services.transfer.model.DeleteServerRequest;
import software.amazon.awssdk.services.transfer.model.DeleteServerResponse;
import software.amazon.awssdk.services.transfer.model.DeleteSshPublicKeyRequest;
import software.amazon.awssdk.services.transfer.model.DeleteSshPublicKeyResponse;
import software.amazon.awssdk.services.transfer.model.DeleteUserRequest;
import software.amazon.awssdk.services.transfer.model.DeleteUserResponse;
import software.amazon.awssdk.services.transfer.model.DeleteWorkflowRequest;
import software.amazon.awssdk.services.transfer.model.DeleteWorkflowResponse;
import software.amazon.awssdk.services.transfer.model.DescribeAccessRequest;
import software.amazon.awssdk.services.transfer.model.DescribeAccessResponse;
import software.amazon.awssdk.services.transfer.model.DescribeAgreementRequest;
import software.amazon.awssdk.services.transfer.model.DescribeAgreementResponse;
import software.amazon.awssdk.services.transfer.model.DescribeCertificateRequest;
import software.amazon.awssdk.services.transfer.model.DescribeCertificateResponse;
import software.amazon.awssdk.services.transfer.model.DescribeConnectorRequest;
import software.amazon.awssdk.services.transfer.model.DescribeConnectorResponse;
import software.amazon.awssdk.services.transfer.model.DescribeExecutionRequest;
import software.amazon.awssdk.services.transfer.model.DescribeExecutionResponse;
import software.amazon.awssdk.services.transfer.model.DescribeHostKeyRequest;
import software.amazon.awssdk.services.transfer.model.DescribeHostKeyResponse;
import software.amazon.awssdk.services.transfer.model.DescribeProfileRequest;
import software.amazon.awssdk.services.transfer.model.DescribeProfileResponse;
import software.amazon.awssdk.services.transfer.model.DescribeSecurityPolicyRequest;
import software.amazon.awssdk.services.transfer.model.DescribeSecurityPolicyResponse;
import software.amazon.awssdk.services.transfer.model.DescribeServerRequest;
import software.amazon.awssdk.services.transfer.model.DescribeServerResponse;
import software.amazon.awssdk.services.transfer.model.DescribeUserRequest;
import software.amazon.awssdk.services.transfer.model.DescribeUserResponse;
import software.amazon.awssdk.services.transfer.model.DescribeWorkflowRequest;
import software.amazon.awssdk.services.transfer.model.DescribeWorkflowResponse;
import software.amazon.awssdk.services.transfer.model.ImportCertificateRequest;
import software.amazon.awssdk.services.transfer.model.ImportCertificateResponse;
import software.amazon.awssdk.services.transfer.model.ImportHostKeyRequest;
import software.amazon.awssdk.services.transfer.model.ImportHostKeyResponse;
import software.amazon.awssdk.services.transfer.model.ImportSshPublicKeyRequest;
import software.amazon.awssdk.services.transfer.model.ImportSshPublicKeyResponse;
import software.amazon.awssdk.services.transfer.model.InternalServiceErrorException;
import software.amazon.awssdk.services.transfer.model.InvalidNextTokenException;
import software.amazon.awssdk.services.transfer.model.InvalidRequestException;
import software.amazon.awssdk.services.transfer.model.ListAccessesRequest;
import software.amazon.awssdk.services.transfer.model.ListAccessesResponse;
import software.amazon.awssdk.services.transfer.model.ListAgreementsRequest;
import software.amazon.awssdk.services.transfer.model.ListAgreementsResponse;
import software.amazon.awssdk.services.transfer.model.ListCertificatesRequest;
import software.amazon.awssdk.services.transfer.model.ListCertificatesResponse;
import software.amazon.awssdk.services.transfer.model.ListConnectorsRequest;
import software.amazon.awssdk.services.transfer.model.ListConnectorsResponse;
import software.amazon.awssdk.services.transfer.model.ListExecutionsRequest;
import software.amazon.awssdk.services.transfer.model.ListExecutionsResponse;
import software.amazon.awssdk.services.transfer.model.ListHostKeysRequest;
import software.amazon.awssdk.services.transfer.model.ListHostKeysResponse;
import software.amazon.awssdk.services.transfer.model.ListProfilesRequest;
import software.amazon.awssdk.services.transfer.model.ListProfilesResponse;
import software.amazon.awssdk.services.transfer.model.ListSecurityPoliciesRequest;
import software.amazon.awssdk.services.transfer.model.ListSecurityPoliciesResponse;
import software.amazon.awssdk.services.transfer.model.ListServersRequest;
import software.amazon.awssdk.services.transfer.model.ListServersResponse;
import software.amazon.awssdk.services.transfer.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.transfer.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.transfer.model.ListUsersRequest;
import software.amazon.awssdk.services.transfer.model.ListUsersResponse;
import software.amazon.awssdk.services.transfer.model.ListWorkflowsRequest;
import software.amazon.awssdk.services.transfer.model.ListWorkflowsResponse;
import software.amazon.awssdk.services.transfer.model.ResourceExistsException;
import software.amazon.awssdk.services.transfer.model.ResourceNotFoundException;
import software.amazon.awssdk.services.transfer.model.SendWorkflowStepStateRequest;
import software.amazon.awssdk.services.transfer.model.SendWorkflowStepStateResponse;
import software.amazon.awssdk.services.transfer.model.ServiceUnavailableException;
import software.amazon.awssdk.services.transfer.model.StartDirectoryListingRequest;
import software.amazon.awssdk.services.transfer.model.StartDirectoryListingResponse;
import software.amazon.awssdk.services.transfer.model.StartFileTransferRequest;
import software.amazon.awssdk.services.transfer.model.StartFileTransferResponse;
import software.amazon.awssdk.services.transfer.model.StartServerRequest;
import software.amazon.awssdk.services.transfer.model.StartServerResponse;
import software.amazon.awssdk.services.transfer.model.StopServerRequest;
import software.amazon.awssdk.services.transfer.model.StopServerResponse;
import software.amazon.awssdk.services.transfer.model.TagResourceRequest;
import software.amazon.awssdk.services.transfer.model.TagResourceResponse;
import software.amazon.awssdk.services.transfer.model.TestConnectionRequest;
import software.amazon.awssdk.services.transfer.model.TestConnectionResponse;
import software.amazon.awssdk.services.transfer.model.TestIdentityProviderRequest;
import software.amazon.awssdk.services.transfer.model.TestIdentityProviderResponse;
import software.amazon.awssdk.services.transfer.model.ThrottlingException;
import software.amazon.awssdk.services.transfer.model.TransferException;
import software.amazon.awssdk.services.transfer.model.UntagResourceRequest;
import software.amazon.awssdk.services.transfer.model.UntagResourceResponse;
import software.amazon.awssdk.services.transfer.model.UpdateAccessRequest;
import software.amazon.awssdk.services.transfer.model.UpdateAccessResponse;
import software.amazon.awssdk.services.transfer.model.UpdateAgreementRequest;
import software.amazon.awssdk.services.transfer.model.UpdateAgreementResponse;
import software.amazon.awssdk.services.transfer.model.UpdateCertificateRequest;
import software.amazon.awssdk.services.transfer.model.UpdateCertificateResponse;
import software.amazon.awssdk.services.transfer.model.UpdateConnectorRequest;
import software.amazon.awssdk.services.transfer.model.UpdateConnectorResponse;
import software.amazon.awssdk.services.transfer.model.UpdateHostKeyRequest;
import software.amazon.awssdk.services.transfer.model.UpdateHostKeyResponse;
import software.amazon.awssdk.services.transfer.model.UpdateProfileRequest;
import software.amazon.awssdk.services.transfer.model.UpdateProfileResponse;
import software.amazon.awssdk.services.transfer.model.UpdateServerRequest;
import software.amazon.awssdk.services.transfer.model.UpdateServerResponse;
import software.amazon.awssdk.services.transfer.model.UpdateUserRequest;
import software.amazon.awssdk.services.transfer.model.UpdateUserResponse;
import software.amazon.awssdk.services.transfer.transform.CreateAccessRequestMarshaller;
import software.amazon.awssdk.services.transfer.transform.CreateAgreementRequestMarshaller;
import software.amazon.awssdk.services.transfer.transform.CreateConnectorRequestMarshaller;
import software.amazon.awssdk.services.transfer.transform.CreateProfileRequestMarshaller;
import software.amazon.awssdk.services.transfer.transform.CreateServerRequestMarshaller;
import software.amazon.awssdk.services.transfer.transform.CreateUserRequestMarshaller;
import software.amazon.awssdk.services.transfer.transform.CreateWorkflowRequestMarshaller;
import software.amazon.awssdk.services.transfer.transform.DeleteAccessRequestMarshaller;
import software.amazon.awssdk.services.transfer.transform.DeleteAgreementRequestMarshaller;
import software.amazon.awssdk.services.transfer.transform.DeleteCertificateRequestMarshaller;
import software.amazon.awssdk.services.transfer.transform.DeleteConnectorRequestMarshaller;
import software.amazon.awssdk.services.transfer.transform.DeleteHostKeyRequestMarshaller;
import software.amazon.awssdk.services.transfer.transform.DeleteProfileRequestMarshaller;
import software.amazon.awssdk.services.transfer.transform.DeleteServerRequestMarshaller;
import software.amazon.awssdk.services.transfer.transform.DeleteSshPublicKeyRequestMarshaller;
import software.amazon.awssdk.services.transfer.transform.DeleteUserRequestMarshaller;
import software.amazon.awssdk.services.transfer.transform.DeleteWorkflowRequestMarshaller;
import software.amazon.awssdk.services.transfer.transform.DescribeAccessRequestMarshaller;
import software.amazon.awssdk.services.transfer.transform.DescribeAgreementRequestMarshaller;
import software.amazon.awssdk.services.transfer.transform.DescribeCertificateRequestMarshaller;
import software.amazon.awssdk.services.transfer.transform.DescribeConnectorRequestMarshaller;
import software.amazon.awssdk.services.transfer.transform.DescribeExecutionRequestMarshaller;
import software.amazon.awssdk.services.transfer.transform.DescribeHostKeyRequestMarshaller;
import software.amazon.awssdk.services.transfer.transform.DescribeProfileRequestMarshaller;
import software.amazon.awssdk.services.transfer.transform.DescribeSecurityPolicyRequestMarshaller;
import software.amazon.awssdk.services.transfer.transform.DescribeServerRequestMarshaller;
import software.amazon.awssdk.services.transfer.transform.DescribeUserRequestMarshaller;
import software.amazon.awssdk.services.transfer.transform.DescribeWorkflowRequestMarshaller;
import software.amazon.awssdk.services.transfer.transform.ImportCertificateRequestMarshaller;
import software.amazon.awssdk.services.transfer.transform.ImportHostKeyRequestMarshaller;
import software.amazon.awssdk.services.transfer.transform.ImportSshPublicKeyRequestMarshaller;
import software.amazon.awssdk.services.transfer.transform.ListAccessesRequestMarshaller;
import software.amazon.awssdk.services.transfer.transform.ListAgreementsRequestMarshaller;
import software.amazon.awssdk.services.transfer.transform.ListCertificatesRequestMarshaller;
import software.amazon.awssdk.services.transfer.transform.ListConnectorsRequestMarshaller;
import software.amazon.awssdk.services.transfer.transform.ListExecutionsRequestMarshaller;
import software.amazon.awssdk.services.transfer.transform.ListHostKeysRequestMarshaller;
import software.amazon.awssdk.services.transfer.transform.ListProfilesRequestMarshaller;
import software.amazon.awssdk.services.transfer.transform.ListSecurityPoliciesRequestMarshaller;
import software.amazon.awssdk.services.transfer.transform.ListServersRequestMarshaller;
import software.amazon.awssdk.services.transfer.transform.ListTagsForResourceRequestMarshaller;
import software.amazon.awssdk.services.transfer.transform.ListUsersRequestMarshaller;
import software.amazon.awssdk.services.transfer.transform.ListWorkflowsRequestMarshaller;
import software.amazon.awssdk.services.transfer.transform.SendWorkflowStepStateRequestMarshaller;
import software.amazon.awssdk.services.transfer.transform.StartDirectoryListingRequestMarshaller;
import software.amazon.awssdk.services.transfer.transform.StartFileTransferRequestMarshaller;
import software.amazon.awssdk.services.transfer.transform.StartServerRequestMarshaller;
import software.amazon.awssdk.services.transfer.transform.StopServerRequestMarshaller;
import software.amazon.awssdk.services.transfer.transform.TagResourceRequestMarshaller;
import software.amazon.awssdk.services.transfer.transform.TestConnectionRequestMarshaller;
import software.amazon.awssdk.services.transfer.transform.TestIdentityProviderRequestMarshaller;
import software.amazon.awssdk.services.transfer.transform.UntagResourceRequestMarshaller;
import software.amazon.awssdk.services.transfer.transform.UpdateAccessRequestMarshaller;
import software.amazon.awssdk.services.transfer.transform.UpdateAgreementRequestMarshaller;
import software.amazon.awssdk.services.transfer.transform.UpdateCertificateRequestMarshaller;
import software.amazon.awssdk.services.transfer.transform.UpdateConnectorRequestMarshaller;
import software.amazon.awssdk.services.transfer.transform.UpdateHostKeyRequestMarshaller;
import software.amazon.awssdk.services.transfer.transform.UpdateProfileRequestMarshaller;
import software.amazon.awssdk.services.transfer.transform.UpdateServerRequestMarshaller;
import software.amazon.awssdk.services.transfer.transform.UpdateUserRequestMarshaller;
import software.amazon.awssdk.services.transfer.waiters.TransferAsyncWaiter;
import software.amazon.awssdk.utils.CompletableFutureUtils;
/**
* Internal implementation of {@link TransferAsyncClient}.
*
* @see TransferAsyncClient#builder()
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
final class DefaultTransferAsyncClient implements TransferAsyncClient {
private static final Logger log = LoggerFactory.getLogger(DefaultTransferAsyncClient.class);
private static final AwsProtocolMetadata protocolMetadata = AwsProtocolMetadata.builder()
.serviceProtocol(AwsServiceProtocol.AWS_JSON).build();
private final AsyncClientHandler clientHandler;
private final AwsJsonProtocolFactory protocolFactory;
private final SdkClientConfiguration clientConfiguration;
private final ScheduledExecutorService executorService;
protected DefaultTransferAsyncClient(SdkClientConfiguration clientConfiguration) {
this.clientHandler = new AwsAsyncClientHandler(clientConfiguration);
this.clientConfiguration = clientConfiguration.toBuilder().option(SdkClientOption.SDK_CLIENT, this).build();
this.protocolFactory = init(AwsJsonProtocolFactory.builder()).build();
this.executorService = clientConfiguration.option(SdkClientOption.SCHEDULED_EXECUTOR_SERVICE);
}
/**
*
* Used by administrators to choose which groups in the directory should have access to upload and download files
* over the enabled protocols using Transfer Family. For example, a Microsoft Active Directory might contain 50,000
* users, but only a small fraction might need the ability to transfer files to the server. An administrator can use
* CreateAccess
to limit the access to the correct set of users who need this ability.
*
*
* @param createAccessRequest
* @return A Java Future containing the result of the CreateAccess operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - ResourceExistsException The requested resource does not exist, or exists in a region other than the
* one specified for the command.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.CreateAccess
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture createAccess(CreateAccessRequest createAccessRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createAccessRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createAccessRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transfer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateAccess");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateAccessResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateAccess").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateAccessRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createAccessRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates an agreement. An agreement is a bilateral trading partner agreement, or partnership, between an Transfer
* Family server and an AS2 process. The agreement defines the file and message transfer relationship between the
* server and the AS2 process. To define an agreement, Transfer Family combines a server, local profile, partner
* profile, certificate, and other attributes.
*
*
* The partner is identified with the PartnerProfileId
, and the AS2 process is identified with the
* LocalProfileId
.
*
*
* @param createAgreementRequest
* @return A Java Future containing the result of the CreateAgreement operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - ResourceExistsException The requested resource does not exist, or exists in a region other than the
* one specified for the command.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.CreateAgreement
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture createAgreement(CreateAgreementRequest createAgreementRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createAgreementRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createAgreementRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transfer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateAgreement");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateAgreementResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateAgreement").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateAgreementRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createAgreementRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates the connector, which captures the parameters for a connection for the AS2 or SFTP protocol. For AS2, the
* connector is required for sending files to an externally hosted AS2 server. For SFTP, the connector is required
* when sending files to an SFTP server or receiving files from an SFTP server. For more details about connectors,
* see Configure AS2
* connectors and Create SFTP
* connectors.
*
*
*
* You must specify exactly one configuration object: either for AS2 (As2Config
) or SFTP (
* SftpConfig
).
*
*
*
* @param createConnectorRequest
* @return A Java Future containing the result of the CreateConnector operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - ResourceExistsException The requested resource does not exist, or exists in a region other than the
* one specified for the command.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.CreateConnector
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture createConnector(CreateConnectorRequest createConnectorRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createConnectorRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createConnectorRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transfer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateConnector");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateConnectorResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateConnector").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateConnectorRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createConnectorRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates the local or partner profile to use for AS2 transfers.
*
*
* @param createProfileRequest
* @return A Java Future containing the result of the CreateProfile operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.CreateProfile
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture createProfile(CreateProfileRequest createProfileRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createProfileRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createProfileRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transfer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateProfile");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateProfileResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateProfile").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateProfileRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createProfileRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Instantiates an auto-scaling virtual server based on the selected file transfer protocol in Amazon Web Services.
* When you make updates to your file transfer protocol-enabled server or when you work with users, use the
* service-generated ServerId
property that is assigned to the newly created server.
*
*
* @param createServerRequest
* @return A Java Future containing the result of the CreateServer operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - ResourceExistsException The requested resource does not exist, or exists in a region other than the
* one specified for the command.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.CreateServer
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture createServer(CreateServerRequest createServerRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createServerRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createServerRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transfer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateServer");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateServerResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateServer").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateServerRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createServerRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a user and associates them with an existing file transfer protocol-enabled server. You can only create
* and associate users with servers that have the IdentityProviderType
set to
* SERVICE_MANAGED
. Using parameters for CreateUser
, you can specify the user name, set
* the home directory, store the user's public key, and assign the user's Identity and Access Management (IAM) role.
* You can also optionally add a session policy, and assign metadata with tags that can be used to group and search
* for users.
*
*
* @param createUserRequest
* @return A Java Future containing the result of the CreateUser operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - ResourceExistsException The requested resource does not exist, or exists in a region other than the
* one specified for the command.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.CreateUser
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture createUser(CreateUserRequest createUserRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createUserRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createUserRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transfer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateUser");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateUserResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("CreateUser")
.withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateUserRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createUserRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Allows you to create a workflow with specified steps and step details the workflow invokes after file transfer
* completes. After creating a workflow, you can associate the workflow created with any transfer servers by
* specifying the workflow-details
field in CreateServer
and UpdateServer
* operations.
*
*
* @param createWorkflowRequest
* @return A Java Future containing the result of the CreateWorkflow operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - ResourceExistsException The requested resource does not exist, or exists in a region other than the
* one specified for the command.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.CreateWorkflow
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture createWorkflow(CreateWorkflowRequest createWorkflowRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createWorkflowRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createWorkflowRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transfer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateWorkflow");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateWorkflowResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateWorkflow").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateWorkflowRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createWorkflowRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Allows you to delete the access specified in the ServerID
and ExternalID
parameters.
*
*
* @param deleteAccessRequest
* @return A Java Future containing the result of the DeleteAccess operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.DeleteAccess
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deleteAccess(DeleteAccessRequest deleteAccessRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteAccessRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteAccessRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transfer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteAccess");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteAccessResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteAccess").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteAccessRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteAccessRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Delete the agreement that's specified in the provided AgreementId
.
*
*
* @param deleteAgreementRequest
* @return A Java Future containing the result of the DeleteAgreement operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.DeleteAgreement
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deleteAgreement(DeleteAgreementRequest deleteAgreementRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteAgreementRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteAgreementRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transfer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteAgreement");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteAgreementResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteAgreement").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteAgreementRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteAgreementRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes the certificate that's specified in the CertificateId
parameter.
*
*
* @param deleteCertificateRequest
* @return A Java Future containing the result of the DeleteCertificate operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.DeleteCertificate
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture deleteCertificate(DeleteCertificateRequest deleteCertificateRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteCertificateRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteCertificateRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transfer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteCertificate");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteCertificateResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteCertificate").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteCertificateRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteCertificateRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes the connector that's specified in the provided ConnectorId
.
*
*
* @param deleteConnectorRequest
* @return A Java Future containing the result of the DeleteConnector operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.DeleteConnector
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deleteConnector(DeleteConnectorRequest deleteConnectorRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteConnectorRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteConnectorRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transfer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteConnector");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteConnectorResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteConnector").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteConnectorRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteConnectorRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes the host key that's specified in the HostKeyId
parameter.
*
*
* @param deleteHostKeyRequest
* @return A Java Future containing the result of the DeleteHostKey operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.DeleteHostKey
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deleteHostKey(DeleteHostKeyRequest deleteHostKeyRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteHostKeyRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteHostKeyRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transfer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteHostKey");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteHostKeyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteHostKey").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteHostKeyRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteHostKeyRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes the profile that's specified in the ProfileId
parameter.
*
*
* @param deleteProfileRequest
* @return A Java Future containing the result of the DeleteProfile operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.DeleteProfile
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deleteProfile(DeleteProfileRequest deleteProfileRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteProfileRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteProfileRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transfer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteProfile");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteProfileResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteProfile").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteProfileRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteProfileRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes the file transfer protocol-enabled server that you specify.
*
*
* No response returns from this operation.
*
*
* @param deleteServerRequest
* @return A Java Future containing the result of the DeleteServer operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.DeleteServer
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deleteServer(DeleteServerRequest deleteServerRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteServerRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteServerRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transfer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteServer");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteServerResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteServer").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteServerRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteServerRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes a user's Secure Shell (SSH) public key.
*
*
* @param deleteSshPublicKeyRequest
* @return A Java Future containing the result of the DeleteSshPublicKey operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.DeleteSshPublicKey
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture deleteSshPublicKey(DeleteSshPublicKeyRequest deleteSshPublicKeyRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteSshPublicKeyRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteSshPublicKeyRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transfer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteSshPublicKey");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteSshPublicKeyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteSshPublicKey").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteSshPublicKeyRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteSshPublicKeyRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes the user belonging to a file transfer protocol-enabled server you specify.
*
*
* No response returns from this operation.
*
*
*
* When you delete a user from a server, the user's information is lost.
*
*
*
* @param deleteUserRequest
* @return A Java Future containing the result of the DeleteUser operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.DeleteUser
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deleteUser(DeleteUserRequest deleteUserRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteUserRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteUserRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transfer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteUser");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteUserResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("DeleteUser")
.withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteUserRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteUserRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes the specified workflow.
*
*
* @param deleteWorkflowRequest
* @return A Java Future containing the result of the DeleteWorkflow operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.DeleteWorkflow
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deleteWorkflow(DeleteWorkflowRequest deleteWorkflowRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteWorkflowRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteWorkflowRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transfer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteWorkflow");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteWorkflowResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteWorkflow").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteWorkflowRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteWorkflowRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Describes the access that is assigned to the specific file transfer protocol-enabled server, as identified by its
* ServerId
property and its ExternalId
.
*
*
* The response from this call returns the properties of the access that is associated with the
* ServerId
value that was specified.
*
*
* @param describeAccessRequest
* @return A Java Future containing the result of the DescribeAccess operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.DescribeAccess
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture describeAccess(DescribeAccessRequest describeAccessRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeAccessRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeAccessRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transfer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeAccess");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeAccessResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeAccess").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeAccessRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeAccessRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Describes the agreement that's identified by the AgreementId
.
*
*
* @param describeAgreementRequest
* @return A Java Future containing the result of the DescribeAgreement operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.DescribeAgreement
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture describeAgreement(DescribeAgreementRequest describeAgreementRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeAgreementRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeAgreementRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transfer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeAgreement");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeAgreementResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeAgreement").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeAgreementRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeAgreementRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Describes the certificate that's identified by the CertificateId
.
*
*
* @param describeCertificateRequest
* @return A Java Future containing the result of the DescribeCertificate operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.DescribeCertificate
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture describeCertificate(
DescribeCertificateRequest describeCertificateRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeCertificateRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeCertificateRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transfer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeCertificate");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeCertificateResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeCertificate").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeCertificateRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeCertificateRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Describes the connector that's identified by the ConnectorId.
*
*
* @param describeConnectorRequest
* @return A Java Future containing the result of the DescribeConnector operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.DescribeConnector
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture describeConnector(DescribeConnectorRequest describeConnectorRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeConnectorRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeConnectorRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transfer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeConnector");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeConnectorResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeConnector").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeConnectorRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeConnectorRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* You can use DescribeExecution
to check the details of the execution of the specified workflow.
*
*
*
* This API call only returns details for in-progress workflows.
*
*
* If you provide an ID for an execution that is not in progress, or if the execution doesn't match the specified
* workflow ID, you receive a ResourceNotFound
exception.
*
*
*
* @param describeExecutionRequest
* @return A Java Future containing the result of the DescribeExecution operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.DescribeExecution
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture describeExecution(DescribeExecutionRequest describeExecutionRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeExecutionRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeExecutionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transfer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeExecution");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeExecutionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeExecution").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeExecutionRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeExecutionRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns the details of the host key that's specified by the HostKeyId
and ServerId
.
*
*
* @param describeHostKeyRequest
* @return A Java Future containing the result of the DescribeHostKey operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.DescribeHostKey
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture describeHostKey(DescribeHostKeyRequest describeHostKeyRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeHostKeyRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeHostKeyRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transfer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeHostKey");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeHostKeyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeHostKey").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeHostKeyRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeHostKeyRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns the details of the profile that's specified by the ProfileId
.
*
*
* @param describeProfileRequest
* @return A Java Future containing the result of the DescribeProfile operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.DescribeProfile
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture describeProfile(DescribeProfileRequest describeProfileRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeProfileRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeProfileRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transfer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeProfile");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeProfileResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeProfile").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeProfileRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeProfileRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Describes the security policy that is attached to your server or SFTP connector. The response contains a
* description of the security policy's properties. For more information about security policies, see Working with security
* policies for servers or Working with
* security policies for SFTP connectors.
*
*
* @param describeSecurityPolicyRequest
* @return A Java Future containing the result of the DescribeSecurityPolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.DescribeSecurityPolicy
* @see AWS API Documentation
*/
@Override
public CompletableFuture describeSecurityPolicy(
DescribeSecurityPolicyRequest describeSecurityPolicyRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeSecurityPolicyRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeSecurityPolicyRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transfer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeSecurityPolicy");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeSecurityPolicyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeSecurityPolicy").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeSecurityPolicyRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeSecurityPolicyRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Describes a file transfer protocol-enabled server that you specify by passing the ServerId
* parameter.
*
*
* The response contains a description of a server's properties. When you set EndpointType
to VPC, the
* response will contain the EndpointDetails
.
*
*
* @param describeServerRequest
* @return A Java Future containing the result of the DescribeServer operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.DescribeServer
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture describeServer(DescribeServerRequest describeServerRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeServerRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeServerRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transfer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeServer");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeServerResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeServer").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeServerRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeServerRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Describes the user assigned to the specific file transfer protocol-enabled server, as identified by its
* ServerId
property.
*
*
* The response from this call returns the properties of the user associated with the ServerId
value
* that was specified.
*
*
* @param describeUserRequest
* @return A Java Future containing the result of the DescribeUser operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.DescribeUser
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture describeUser(DescribeUserRequest describeUserRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeUserRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeUserRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transfer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeUser");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DescribeUserResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeUser").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeUserRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeUserRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Describes the specified workflow.
*
*
* @param describeWorkflowRequest
* @return A Java Future containing the result of the DescribeWorkflow operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.DescribeWorkflow
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture describeWorkflow(DescribeWorkflowRequest describeWorkflowRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeWorkflowRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeWorkflowRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transfer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeWorkflow");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeWorkflowResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeWorkflow").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeWorkflowRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeWorkflowRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Imports the signing and encryption certificates that you need to create local (AS2) profiles and partner
* profiles.
*
*
* @param importCertificateRequest
* @return A Java Future containing the result of the ImportCertificate operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ImportCertificate
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture importCertificate(ImportCertificateRequest importCertificateRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(importCertificateRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, importCertificateRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transfer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ImportCertificate");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ImportCertificateResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ImportCertificate").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ImportCertificateRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(importCertificateRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Adds a host key to the server that's specified by the ServerId
parameter.
*
*
* @param importHostKeyRequest
* @return A Java Future containing the result of the ImportHostKey operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - ResourceExistsException The requested resource does not exist, or exists in a region other than the
* one specified for the command.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ImportHostKey
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture importHostKey(ImportHostKeyRequest importHostKeyRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(importHostKeyRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, importHostKeyRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transfer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ImportHostKey");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ImportHostKeyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ImportHostKey").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ImportHostKeyRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(importHostKeyRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Adds a Secure Shell (SSH) public key to a Transfer Family user identified by a UserName
value
* assigned to the specific file transfer protocol-enabled server, identified by ServerId
.
*
*
* The response returns the UserName
value, the ServerId
value, and the name of the
* SshPublicKeyId
.
*
*
* @param importSshPublicKeyRequest
* @return A Java Future containing the result of the ImportSshPublicKey operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - ResourceExistsException The requested resource does not exist, or exists in a region other than the
* one specified for the command.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ImportSshPublicKey
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture importSshPublicKey(ImportSshPublicKeyRequest importSshPublicKeyRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(importSshPublicKeyRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, importSshPublicKeyRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transfer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ImportSshPublicKey");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ImportSshPublicKeyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ImportSshPublicKey").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ImportSshPublicKeyRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(importSshPublicKeyRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Lists the details for all the accesses you have on your server.
*
*
* @param listAccessesRequest
* @return A Java Future containing the result of the ListAccesses operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - InvalidNextTokenException The
NextToken
parameter that was passed is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ListAccesses
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture listAccesses(ListAccessesRequest listAccessesRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listAccessesRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listAccessesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transfer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListAccesses");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListAccessesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListAccesses").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListAccessesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listAccessesRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns a list of the agreements for the server that's identified by the ServerId
that you supply.
* If you want to limit the results to a certain number, supply a value for the MaxResults
parameter.
* If you ran the command previously and received a value for NextToken
, you can supply that value to
* continue listing agreements from where you left off.
*
*
* @param listAgreementsRequest
* @return A Java Future containing the result of the ListAgreements operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - InvalidNextTokenException The
NextToken
parameter that was passed is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ListAgreements
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture listAgreements(ListAgreementsRequest listAgreementsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listAgreementsRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listAgreementsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transfer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListAgreements");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListAgreementsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListAgreements").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListAgreementsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listAgreementsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns a list of the current certificates that have been imported into Transfer Family. If you want to limit the
* results to a certain number, supply a value for the MaxResults
parameter. If you ran the command
* previously and received a value for the NextToken
parameter, you can supply that value to continue
* listing certificates from where you left off.
*
*
* @param listCertificatesRequest
* @return A Java Future containing the result of the ListCertificates operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - InvalidNextTokenException The
NextToken
parameter that was passed is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ListCertificates
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture listCertificates(ListCertificatesRequest listCertificatesRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listCertificatesRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listCertificatesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transfer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListCertificates");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListCertificatesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListCertificates").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListCertificatesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listCertificatesRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Lists the connectors for the specified Region.
*
*
* @param listConnectorsRequest
* @return A Java Future containing the result of the ListConnectors operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - InvalidNextTokenException The
NextToken
parameter that was passed is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ListConnectors
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture listConnectors(ListConnectorsRequest listConnectorsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listConnectorsRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listConnectorsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transfer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListConnectors");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListConnectorsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListConnectors").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListConnectorsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listConnectorsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Lists all in-progress executions for the specified workflow.
*
*
*
* If the specified workflow ID cannot be found, ListExecutions
returns a ResourceNotFound
* exception.
*
*
*
* @param listExecutionsRequest
* @return A Java Future containing the result of the ListExecutions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - InvalidNextTokenException The
NextToken
parameter that was passed is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ListExecutions
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture listExecutions(ListExecutionsRequest listExecutionsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listExecutionsRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listExecutionsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Transfer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListExecutions");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListExecutionsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams