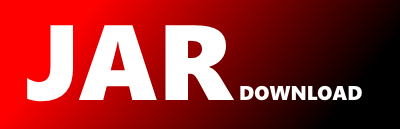
software.amazon.awssdk.services.transfer.model.As2ConnectorConfig Maven / Gradle / Ivy
Show all versions of transfer Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.transfer.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Contains the details for an AS2 connector object. The connector object is used for AS2 outbound processes, to connect
* the Transfer Family customer with the trading partner.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class As2ConnectorConfig implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField LOCAL_PROFILE_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("LocalProfileId").getter(getter(As2ConnectorConfig::localProfileId))
.setter(setter(Builder::localProfileId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LocalProfileId").build()).build();
private static final SdkField PARTNER_PROFILE_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("PartnerProfileId").getter(getter(As2ConnectorConfig::partnerProfileId))
.setter(setter(Builder::partnerProfileId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PartnerProfileId").build()).build();
private static final SdkField MESSAGE_SUBJECT_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("MessageSubject").getter(getter(As2ConnectorConfig::messageSubject))
.setter(setter(Builder::messageSubject))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MessageSubject").build()).build();
private static final SdkField COMPRESSION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Compression").getter(getter(As2ConnectorConfig::compressionAsString))
.setter(setter(Builder::compression))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Compression").build()).build();
private static final SdkField ENCRYPTION_ALGORITHM_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("EncryptionAlgorithm").getter(getter(As2ConnectorConfig::encryptionAlgorithmAsString))
.setter(setter(Builder::encryptionAlgorithm))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EncryptionAlgorithm").build())
.build();
private static final SdkField SIGNING_ALGORITHM_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SigningAlgorithm").getter(getter(As2ConnectorConfig::signingAlgorithmAsString))
.setter(setter(Builder::signingAlgorithm))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SigningAlgorithm").build()).build();
private static final SdkField MDN_SIGNING_ALGORITHM_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("MdnSigningAlgorithm").getter(getter(As2ConnectorConfig::mdnSigningAlgorithmAsString))
.setter(setter(Builder::mdnSigningAlgorithm))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MdnSigningAlgorithm").build())
.build();
private static final SdkField MDN_RESPONSE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("MdnResponse").getter(getter(As2ConnectorConfig::mdnResponseAsString))
.setter(setter(Builder::mdnResponse))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MdnResponse").build()).build();
private static final SdkField BASIC_AUTH_SECRET_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("BasicAuthSecretId").getter(getter(As2ConnectorConfig::basicAuthSecretId))
.setter(setter(Builder::basicAuthSecretId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("BasicAuthSecretId").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(LOCAL_PROFILE_ID_FIELD,
PARTNER_PROFILE_ID_FIELD, MESSAGE_SUBJECT_FIELD, COMPRESSION_FIELD, ENCRYPTION_ALGORITHM_FIELD,
SIGNING_ALGORITHM_FIELD, MDN_SIGNING_ALGORITHM_FIELD, MDN_RESPONSE_FIELD, BASIC_AUTH_SECRET_ID_FIELD));
private static final long serialVersionUID = 1L;
private final String localProfileId;
private final String partnerProfileId;
private final String messageSubject;
private final String compression;
private final String encryptionAlgorithm;
private final String signingAlgorithm;
private final String mdnSigningAlgorithm;
private final String mdnResponse;
private final String basicAuthSecretId;
private As2ConnectorConfig(BuilderImpl builder) {
this.localProfileId = builder.localProfileId;
this.partnerProfileId = builder.partnerProfileId;
this.messageSubject = builder.messageSubject;
this.compression = builder.compression;
this.encryptionAlgorithm = builder.encryptionAlgorithm;
this.signingAlgorithm = builder.signingAlgorithm;
this.mdnSigningAlgorithm = builder.mdnSigningAlgorithm;
this.mdnResponse = builder.mdnResponse;
this.basicAuthSecretId = builder.basicAuthSecretId;
}
/**
*
* A unique identifier for the AS2 local profile.
*
*
* @return A unique identifier for the AS2 local profile.
*/
public final String localProfileId() {
return localProfileId;
}
/**
*
* A unique identifier for the partner profile for the connector.
*
*
* @return A unique identifier for the partner profile for the connector.
*/
public final String partnerProfileId() {
return partnerProfileId;
}
/**
*
* Used as the Subject
HTTP header attribute in AS2 messages that are being sent with the connector.
*
*
* @return Used as the Subject
HTTP header attribute in AS2 messages that are being sent with the
* connector.
*/
public final String messageSubject() {
return messageSubject;
}
/**
*
* Specifies whether the AS2 file is compressed.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #compression} will
* return {@link CompressionEnum#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #compressionAsString}.
*
*
* @return Specifies whether the AS2 file is compressed.
* @see CompressionEnum
*/
public final CompressionEnum compression() {
return CompressionEnum.fromValue(compression);
}
/**
*
* Specifies whether the AS2 file is compressed.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #compression} will
* return {@link CompressionEnum#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #compressionAsString}.
*
*
* @return Specifies whether the AS2 file is compressed.
* @see CompressionEnum
*/
public final String compressionAsString() {
return compression;
}
/**
*
* The algorithm that is used to encrypt the file.
*
*
* Note the following:
*
*
* -
*
* Do not use the DES_EDE3_CBC
algorithm unless you must support a legacy client that requires it, as
* it is a weak encryption algorithm.
*
*
* -
*
* You can only specify NONE
if the URL for your connector uses HTTPS. Using HTTPS ensures that no
* traffic is sent in clear text.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #encryptionAlgorithm} will return {@link EncryptionAlg#UNKNOWN_TO_SDK_VERSION}. The raw value returned by
* the service is available from {@link #encryptionAlgorithmAsString}.
*
*
* @return The algorithm that is used to encrypt the file.
*
* Note the following:
*
*
* -
*
* Do not use the DES_EDE3_CBC
algorithm unless you must support a legacy client that requires
* it, as it is a weak encryption algorithm.
*
*
* -
*
* You can only specify NONE
if the URL for your connector uses HTTPS. Using HTTPS ensures that
* no traffic is sent in clear text.
*
*
* @see EncryptionAlg
*/
public final EncryptionAlg encryptionAlgorithm() {
return EncryptionAlg.fromValue(encryptionAlgorithm);
}
/**
*
* The algorithm that is used to encrypt the file.
*
*
* Note the following:
*
*
* -
*
* Do not use the DES_EDE3_CBC
algorithm unless you must support a legacy client that requires it, as
* it is a weak encryption algorithm.
*
*
* -
*
* You can only specify NONE
if the URL for your connector uses HTTPS. Using HTTPS ensures that no
* traffic is sent in clear text.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #encryptionAlgorithm} will return {@link EncryptionAlg#UNKNOWN_TO_SDK_VERSION}. The raw value returned by
* the service is available from {@link #encryptionAlgorithmAsString}.
*
*
* @return The algorithm that is used to encrypt the file.
*
* Note the following:
*
*
* -
*
* Do not use the DES_EDE3_CBC
algorithm unless you must support a legacy client that requires
* it, as it is a weak encryption algorithm.
*
*
* -
*
* You can only specify NONE
if the URL for your connector uses HTTPS. Using HTTPS ensures that
* no traffic is sent in clear text.
*
*
* @see EncryptionAlg
*/
public final String encryptionAlgorithmAsString() {
return encryptionAlgorithm;
}
/**
*
* The algorithm that is used to sign the AS2 messages sent with the connector.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #signingAlgorithm}
* will return {@link SigningAlg#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #signingAlgorithmAsString}.
*
*
* @return The algorithm that is used to sign the AS2 messages sent with the connector.
* @see SigningAlg
*/
public final SigningAlg signingAlgorithm() {
return SigningAlg.fromValue(signingAlgorithm);
}
/**
*
* The algorithm that is used to sign the AS2 messages sent with the connector.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #signingAlgorithm}
* will return {@link SigningAlg#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #signingAlgorithmAsString}.
*
*
* @return The algorithm that is used to sign the AS2 messages sent with the connector.
* @see SigningAlg
*/
public final String signingAlgorithmAsString() {
return signingAlgorithm;
}
/**
*
* The signing algorithm for the MDN response.
*
*
*
* If set to DEFAULT (or not set at all), the value for SigningAlgorithm
is used.
*
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #mdnSigningAlgorithm} will return {@link MdnSigningAlg#UNKNOWN_TO_SDK_VERSION}. The raw value returned by
* the service is available from {@link #mdnSigningAlgorithmAsString}.
*
*
* @return The signing algorithm for the MDN response.
*
* If set to DEFAULT (or not set at all), the value for SigningAlgorithm
is used.
*
* @see MdnSigningAlg
*/
public final MdnSigningAlg mdnSigningAlgorithm() {
return MdnSigningAlg.fromValue(mdnSigningAlgorithm);
}
/**
*
* The signing algorithm for the MDN response.
*
*
*
* If set to DEFAULT (or not set at all), the value for SigningAlgorithm
is used.
*
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #mdnSigningAlgorithm} will return {@link MdnSigningAlg#UNKNOWN_TO_SDK_VERSION}. The raw value returned by
* the service is available from {@link #mdnSigningAlgorithmAsString}.
*
*
* @return The signing algorithm for the MDN response.
*
* If set to DEFAULT (or not set at all), the value for SigningAlgorithm
is used.
*
* @see MdnSigningAlg
*/
public final String mdnSigningAlgorithmAsString() {
return mdnSigningAlgorithm;
}
/**
*
* Used for outbound requests (from an Transfer Family server to a partner AS2 server) to determine whether the
* partner response for transfers is synchronous or asynchronous. Specify either of the following values:
*
*
* -
*
* SYNC
: The system expects a synchronous MDN response, confirming that the file was transferred
* successfully (or not).
*
*
* -
*
* NONE
: Specifies that no MDN response is required.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #mdnResponse} will
* return {@link MdnResponse#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #mdnResponseAsString}.
*
*
* @return Used for outbound requests (from an Transfer Family server to a partner AS2 server) to determine whether
* the partner response for transfers is synchronous or asynchronous. Specify either of the following
* values:
*
* -
*
* SYNC
: The system expects a synchronous MDN response, confirming that the file was
* transferred successfully (or not).
*
*
* -
*
* NONE
: Specifies that no MDN response is required.
*
*
* @see MdnResponse
*/
public final MdnResponse mdnResponse() {
return MdnResponse.fromValue(mdnResponse);
}
/**
*
* Used for outbound requests (from an Transfer Family server to a partner AS2 server) to determine whether the
* partner response for transfers is synchronous or asynchronous. Specify either of the following values:
*
*
* -
*
* SYNC
: The system expects a synchronous MDN response, confirming that the file was transferred
* successfully (or not).
*
*
* -
*
* NONE
: Specifies that no MDN response is required.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #mdnResponse} will
* return {@link MdnResponse#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #mdnResponseAsString}.
*
*
* @return Used for outbound requests (from an Transfer Family server to a partner AS2 server) to determine whether
* the partner response for transfers is synchronous or asynchronous. Specify either of the following
* values:
*
* -
*
* SYNC
: The system expects a synchronous MDN response, confirming that the file was
* transferred successfully (or not).
*
*
* -
*
* NONE
: Specifies that no MDN response is required.
*
*
* @see MdnResponse
*/
public final String mdnResponseAsString() {
return mdnResponse;
}
/**
*
* Provides Basic authentication support to the AS2 Connectors API. To use Basic authentication, you must provide
* the name or Amazon Resource Name (ARN) of a secret in Secrets Manager.
*
*
* The default value for this parameter is null
, which indicates that Basic authentication is not
* enabled for the connector.
*
*
* If the connector should use Basic authentication, the secret needs to be in the following format:
*
*
* { "Username": "user-name", "Password": "user-password" }
*
*
* Replace user-name
and user-password
with the credentials for the actual user that is
* being authenticated.
*
*
* Note the following:
*
*
* -
*
* You are storing these credentials in Secrets Manager, not passing them directly into this API.
*
*
* -
*
* If you are using the API, SDKs, or CloudFormation to configure your connector, then you must create the secret
* before you can enable Basic authentication. However, if you are using the Amazon Web Services management console,
* you can have the system create the secret for you.
*
*
*
*
* If you have previously enabled Basic authentication for a connector, you can disable it by using the
* UpdateConnector
API call. For example, if you are using the CLI, you can run the following command
* to remove Basic authentication:
*
*
* update-connector --connector-id my-connector-id --as2-config 'BasicAuthSecretId=""'
*
*
* @return Provides Basic authentication support to the AS2 Connectors API. To use Basic authentication, you must
* provide the name or Amazon Resource Name (ARN) of a secret in Secrets Manager.
*
* The default value for this parameter is null
, which indicates that Basic authentication is
* not enabled for the connector.
*
*
* If the connector should use Basic authentication, the secret needs to be in the following format:
*
*
* { "Username": "user-name", "Password": "user-password" }
*
*
* Replace user-name
and user-password
with the credentials for the actual user
* that is being authenticated.
*
*
* Note the following:
*
*
* -
*
* You are storing these credentials in Secrets Manager, not passing them directly into this API.
*
*
* -
*
* If you are using the API, SDKs, or CloudFormation to configure your connector, then you must create the
* secret before you can enable Basic authentication. However, if you are using the Amazon Web Services
* management console, you can have the system create the secret for you.
*
*
*
*
* If you have previously enabled Basic authentication for a connector, you can disable it by using the
* UpdateConnector
API call. For example, if you are using the CLI, you can run the following
* command to remove Basic authentication:
*
*
* update-connector --connector-id my-connector-id --as2-config 'BasicAuthSecretId=""'
*/
public final String basicAuthSecretId() {
return basicAuthSecretId;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(localProfileId());
hashCode = 31 * hashCode + Objects.hashCode(partnerProfileId());
hashCode = 31 * hashCode + Objects.hashCode(messageSubject());
hashCode = 31 * hashCode + Objects.hashCode(compressionAsString());
hashCode = 31 * hashCode + Objects.hashCode(encryptionAlgorithmAsString());
hashCode = 31 * hashCode + Objects.hashCode(signingAlgorithmAsString());
hashCode = 31 * hashCode + Objects.hashCode(mdnSigningAlgorithmAsString());
hashCode = 31 * hashCode + Objects.hashCode(mdnResponseAsString());
hashCode = 31 * hashCode + Objects.hashCode(basicAuthSecretId());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof As2ConnectorConfig)) {
return false;
}
As2ConnectorConfig other = (As2ConnectorConfig) obj;
return Objects.equals(localProfileId(), other.localProfileId())
&& Objects.equals(partnerProfileId(), other.partnerProfileId())
&& Objects.equals(messageSubject(), other.messageSubject())
&& Objects.equals(compressionAsString(), other.compressionAsString())
&& Objects.equals(encryptionAlgorithmAsString(), other.encryptionAlgorithmAsString())
&& Objects.equals(signingAlgorithmAsString(), other.signingAlgorithmAsString())
&& Objects.equals(mdnSigningAlgorithmAsString(), other.mdnSigningAlgorithmAsString())
&& Objects.equals(mdnResponseAsString(), other.mdnResponseAsString())
&& Objects.equals(basicAuthSecretId(), other.basicAuthSecretId());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("As2ConnectorConfig").add("LocalProfileId", localProfileId())
.add("PartnerProfileId", partnerProfileId()).add("MessageSubject", messageSubject())
.add("Compression", compressionAsString()).add("EncryptionAlgorithm", encryptionAlgorithmAsString())
.add("SigningAlgorithm", signingAlgorithmAsString()).add("MdnSigningAlgorithm", mdnSigningAlgorithmAsString())
.add("MdnResponse", mdnResponseAsString()).add("BasicAuthSecretId", basicAuthSecretId()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "LocalProfileId":
return Optional.ofNullable(clazz.cast(localProfileId()));
case "PartnerProfileId":
return Optional.ofNullable(clazz.cast(partnerProfileId()));
case "MessageSubject":
return Optional.ofNullable(clazz.cast(messageSubject()));
case "Compression":
return Optional.ofNullable(clazz.cast(compressionAsString()));
case "EncryptionAlgorithm":
return Optional.ofNullable(clazz.cast(encryptionAlgorithmAsString()));
case "SigningAlgorithm":
return Optional.ofNullable(clazz.cast(signingAlgorithmAsString()));
case "MdnSigningAlgorithm":
return Optional.ofNullable(clazz.cast(mdnSigningAlgorithmAsString()));
case "MdnResponse":
return Optional.ofNullable(clazz.cast(mdnResponseAsString()));
case "BasicAuthSecretId":
return Optional.ofNullable(clazz.cast(basicAuthSecretId()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
*
* Note the following:
*
*
* -
*
* Do not use the DES_EDE3_CBC
algorithm unless you must support a legacy client that
* requires it, as it is a weak encryption algorithm.
*
*
* -
*
* You can only specify NONE
if the URL for your connector uses HTTPS. Using HTTPS ensures
* that no traffic is sent in clear text.
*
*
* @see EncryptionAlg
* @return Returns a reference to this object so that method calls can be chained together.
* @see EncryptionAlg
*/
Builder encryptionAlgorithm(String encryptionAlgorithm);
/**
*
* The algorithm that is used to encrypt the file.
*
*
* Note the following:
*
*
* -
*
* Do not use the DES_EDE3_CBC
algorithm unless you must support a legacy client that requires it,
* as it is a weak encryption algorithm.
*
*
* -
*
* You can only specify NONE
if the URL for your connector uses HTTPS. Using HTTPS ensures that no
* traffic is sent in clear text.
*
*
*
*
* @param encryptionAlgorithm
* The algorithm that is used to encrypt the file.
*
* Note the following:
*
*
* -
*
* Do not use the DES_EDE3_CBC
algorithm unless you must support a legacy client that
* requires it, as it is a weak encryption algorithm.
*
*
* -
*
* You can only specify NONE
if the URL for your connector uses HTTPS. Using HTTPS ensures
* that no traffic is sent in clear text.
*
*
* @see EncryptionAlg
* @return Returns a reference to this object so that method calls can be chained together.
* @see EncryptionAlg
*/
Builder encryptionAlgorithm(EncryptionAlg encryptionAlgorithm);
/**
*
* The algorithm that is used to sign the AS2 messages sent with the connector.
*
*
* @param signingAlgorithm
* The algorithm that is used to sign the AS2 messages sent with the connector.
* @see SigningAlg
* @return Returns a reference to this object so that method calls can be chained together.
* @see SigningAlg
*/
Builder signingAlgorithm(String signingAlgorithm);
/**
*
* The algorithm that is used to sign the AS2 messages sent with the connector.
*
*
* @param signingAlgorithm
* The algorithm that is used to sign the AS2 messages sent with the connector.
* @see SigningAlg
* @return Returns a reference to this object so that method calls can be chained together.
* @see SigningAlg
*/
Builder signingAlgorithm(SigningAlg signingAlgorithm);
/**
*
* The signing algorithm for the MDN response.
*
*
*
* If set to DEFAULT (or not set at all), the value for SigningAlgorithm
is used.
*
*
*
* @param mdnSigningAlgorithm
* The signing algorithm for the MDN response.
*
* If set to DEFAULT (or not set at all), the value for SigningAlgorithm
is used.
*
* @see MdnSigningAlg
* @return Returns a reference to this object so that method calls can be chained together.
* @see MdnSigningAlg
*/
Builder mdnSigningAlgorithm(String mdnSigningAlgorithm);
/**
*
* The signing algorithm for the MDN response.
*
*
*
* If set to DEFAULT (or not set at all), the value for SigningAlgorithm
is used.
*
*
*
* @param mdnSigningAlgorithm
* The signing algorithm for the MDN response.
*
* If set to DEFAULT (or not set at all), the value for SigningAlgorithm
is used.
*
* @see MdnSigningAlg
* @return Returns a reference to this object so that method calls can be chained together.
* @see MdnSigningAlg
*/
Builder mdnSigningAlgorithm(MdnSigningAlg mdnSigningAlgorithm);
/**
*
* Used for outbound requests (from an Transfer Family server to a partner AS2 server) to determine whether the
* partner response for transfers is synchronous or asynchronous. Specify either of the following values:
*
*
* -
*
* SYNC
: The system expects a synchronous MDN response, confirming that the file was transferred
* successfully (or not).
*
*
* -
*
* NONE
: Specifies that no MDN response is required.
*
*
*
*
* @param mdnResponse
* Used for outbound requests (from an Transfer Family server to a partner AS2 server) to determine
* whether the partner response for transfers is synchronous or asynchronous. Specify either of the
* following values:
*
* -
*
* SYNC
: The system expects a synchronous MDN response, confirming that the file was
* transferred successfully (or not).
*
*
* -
*
* NONE
: Specifies that no MDN response is required.
*
*
* @see MdnResponse
* @return Returns a reference to this object so that method calls can be chained together.
* @see MdnResponse
*/
Builder mdnResponse(String mdnResponse);
/**
*
* Used for outbound requests (from an Transfer Family server to a partner AS2 server) to determine whether the
* partner response for transfers is synchronous or asynchronous. Specify either of the following values:
*
*
* -
*
* SYNC
: The system expects a synchronous MDN response, confirming that the file was transferred
* successfully (or not).
*
*
* -
*
* NONE
: Specifies that no MDN response is required.
*
*
*
*
* @param mdnResponse
* Used for outbound requests (from an Transfer Family server to a partner AS2 server) to determine
* whether the partner response for transfers is synchronous or asynchronous. Specify either of the
* following values:
*
* -
*
* SYNC
: The system expects a synchronous MDN response, confirming that the file was
* transferred successfully (or not).
*
*
* -
*
* NONE
: Specifies that no MDN response is required.
*
*
* @see MdnResponse
* @return Returns a reference to this object so that method calls can be chained together.
* @see MdnResponse
*/
Builder mdnResponse(MdnResponse mdnResponse);
/**
*
* Provides Basic authentication support to the AS2 Connectors API. To use Basic authentication, you must
* provide the name or Amazon Resource Name (ARN) of a secret in Secrets Manager.
*
*
* The default value for this parameter is null
, which indicates that Basic authentication is not
* enabled for the connector.
*
*
* If the connector should use Basic authentication, the secret needs to be in the following format:
*
*
* { "Username": "user-name", "Password": "user-password" }
*
*
* Replace user-name
and user-password
with the credentials for the actual user that
* is being authenticated.
*
*
* Note the following:
*
*
* -
*
* You are storing these credentials in Secrets Manager, not passing them directly into this API.
*
*
* -
*
* If you are using the API, SDKs, or CloudFormation to configure your connector, then you must create the
* secret before you can enable Basic authentication. However, if you are using the Amazon Web Services
* management console, you can have the system create the secret for you.
*
*
*
*
* If you have previously enabled Basic authentication for a connector, you can disable it by using the
* UpdateConnector
API call. For example, if you are using the CLI, you can run the following
* command to remove Basic authentication:
*
*
* update-connector --connector-id my-connector-id --as2-config 'BasicAuthSecretId=""'
*
*
* @param basicAuthSecretId
* Provides Basic authentication support to the AS2 Connectors API. To use Basic authentication, you must
* provide the name or Amazon Resource Name (ARN) of a secret in Secrets Manager.
*
* The default value for this parameter is null
, which indicates that Basic authentication
* is not enabled for the connector.
*
*
* If the connector should use Basic authentication, the secret needs to be in the following format:
*
*
* { "Username": "user-name", "Password": "user-password" }
*
*
* Replace user-name
and user-password
with the credentials for the actual user
* that is being authenticated.
*
*
* Note the following:
*
*
* -
*
* You are storing these credentials in Secrets Manager, not passing them directly into this API.
*
*
* -
*
* If you are using the API, SDKs, or CloudFormation to configure your connector, then you must create
* the secret before you can enable Basic authentication. However, if you are using the Amazon Web
* Services management console, you can have the system create the secret for you.
*
*
*
*
* If you have previously enabled Basic authentication for a connector, you can disable it by using the
* UpdateConnector
API call. For example, if you are using the CLI, you can run the
* following command to remove Basic authentication:
*
*
* update-connector --connector-id my-connector-id --as2-config 'BasicAuthSecretId=""'
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder basicAuthSecretId(String basicAuthSecretId);
}
static final class BuilderImpl implements Builder {
private String localProfileId;
private String partnerProfileId;
private String messageSubject;
private String compression;
private String encryptionAlgorithm;
private String signingAlgorithm;
private String mdnSigningAlgorithm;
private String mdnResponse;
private String basicAuthSecretId;
private BuilderImpl() {
}
private BuilderImpl(As2ConnectorConfig model) {
localProfileId(model.localProfileId);
partnerProfileId(model.partnerProfileId);
messageSubject(model.messageSubject);
compression(model.compression);
encryptionAlgorithm(model.encryptionAlgorithm);
signingAlgorithm(model.signingAlgorithm);
mdnSigningAlgorithm(model.mdnSigningAlgorithm);
mdnResponse(model.mdnResponse);
basicAuthSecretId(model.basicAuthSecretId);
}
public final String getLocalProfileId() {
return localProfileId;
}
public final void setLocalProfileId(String localProfileId) {
this.localProfileId = localProfileId;
}
@Override
public final Builder localProfileId(String localProfileId) {
this.localProfileId = localProfileId;
return this;
}
public final String getPartnerProfileId() {
return partnerProfileId;
}
public final void setPartnerProfileId(String partnerProfileId) {
this.partnerProfileId = partnerProfileId;
}
@Override
public final Builder partnerProfileId(String partnerProfileId) {
this.partnerProfileId = partnerProfileId;
return this;
}
public final String getMessageSubject() {
return messageSubject;
}
public final void setMessageSubject(String messageSubject) {
this.messageSubject = messageSubject;
}
@Override
public final Builder messageSubject(String messageSubject) {
this.messageSubject = messageSubject;
return this;
}
public final String getCompression() {
return compression;
}
public final void setCompression(String compression) {
this.compression = compression;
}
@Override
public final Builder compression(String compression) {
this.compression = compression;
return this;
}
@Override
public final Builder compression(CompressionEnum compression) {
this.compression(compression == null ? null : compression.toString());
return this;
}
public final String getEncryptionAlgorithm() {
return encryptionAlgorithm;
}
public final void setEncryptionAlgorithm(String encryptionAlgorithm) {
this.encryptionAlgorithm = encryptionAlgorithm;
}
@Override
public final Builder encryptionAlgorithm(String encryptionAlgorithm) {
this.encryptionAlgorithm = encryptionAlgorithm;
return this;
}
@Override
public final Builder encryptionAlgorithm(EncryptionAlg encryptionAlgorithm) {
this.encryptionAlgorithm(encryptionAlgorithm == null ? null : encryptionAlgorithm.toString());
return this;
}
public final String getSigningAlgorithm() {
return signingAlgorithm;
}
public final void setSigningAlgorithm(String signingAlgorithm) {
this.signingAlgorithm = signingAlgorithm;
}
@Override
public final Builder signingAlgorithm(String signingAlgorithm) {
this.signingAlgorithm = signingAlgorithm;
return this;
}
@Override
public final Builder signingAlgorithm(SigningAlg signingAlgorithm) {
this.signingAlgorithm(signingAlgorithm == null ? null : signingAlgorithm.toString());
return this;
}
public final String getMdnSigningAlgorithm() {
return mdnSigningAlgorithm;
}
public final void setMdnSigningAlgorithm(String mdnSigningAlgorithm) {
this.mdnSigningAlgorithm = mdnSigningAlgorithm;
}
@Override
public final Builder mdnSigningAlgorithm(String mdnSigningAlgorithm) {
this.mdnSigningAlgorithm = mdnSigningAlgorithm;
return this;
}
@Override
public final Builder mdnSigningAlgorithm(MdnSigningAlg mdnSigningAlgorithm) {
this.mdnSigningAlgorithm(mdnSigningAlgorithm == null ? null : mdnSigningAlgorithm.toString());
return this;
}
public final String getMdnResponse() {
return mdnResponse;
}
public final void setMdnResponse(String mdnResponse) {
this.mdnResponse = mdnResponse;
}
@Override
public final Builder mdnResponse(String mdnResponse) {
this.mdnResponse = mdnResponse;
return this;
}
@Override
public final Builder mdnResponse(MdnResponse mdnResponse) {
this.mdnResponse(mdnResponse == null ? null : mdnResponse.toString());
return this;
}
public final String getBasicAuthSecretId() {
return basicAuthSecretId;
}
public final void setBasicAuthSecretId(String basicAuthSecretId) {
this.basicAuthSecretId = basicAuthSecretId;
}
@Override
public final Builder basicAuthSecretId(String basicAuthSecretId) {
this.basicAuthSecretId = basicAuthSecretId;
return this;
}
@Override
public As2ConnectorConfig build() {
return new As2ConnectorConfig(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
}
}