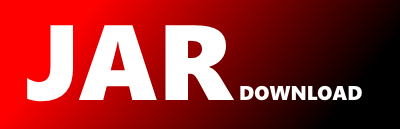
software.amazon.awssdk.services.transfer.model.DescribedUser Maven / Gradle / Ivy
Show all versions of transfer Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.transfer.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Describes the properties of a user that was specified.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class DescribedUser implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField ARN_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Arn")
.getter(getter(DescribedUser::arn)).setter(setter(Builder::arn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Arn").build()).build();
private static final SdkField HOME_DIRECTORY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("HomeDirectory").getter(getter(DescribedUser::homeDirectory)).setter(setter(Builder::homeDirectory))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("HomeDirectory").build()).build();
private static final SdkField> HOME_DIRECTORY_MAPPINGS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("HomeDirectoryMappings")
.getter(getter(DescribedUser::homeDirectoryMappings))
.setter(setter(Builder::homeDirectoryMappings))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("HomeDirectoryMappings").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(HomeDirectoryMapEntry::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField HOME_DIRECTORY_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("HomeDirectoryType").getter(getter(DescribedUser::homeDirectoryTypeAsString))
.setter(setter(Builder::homeDirectoryType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("HomeDirectoryType").build()).build();
private static final SdkField POLICY_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Policy")
.getter(getter(DescribedUser::policy)).setter(setter(Builder::policy))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Policy").build()).build();
private static final SdkField POSIX_PROFILE_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("PosixProfile").getter(getter(DescribedUser::posixProfile)).setter(setter(Builder::posixProfile))
.constructor(PosixProfile::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PosixProfile").build()).build();
private static final SdkField ROLE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Role")
.getter(getter(DescribedUser::role)).setter(setter(Builder::role))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Role").build()).build();
private static final SdkField> SSH_PUBLIC_KEYS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("SshPublicKeys")
.getter(getter(DescribedUser::sshPublicKeys))
.setter(setter(Builder::sshPublicKeys))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SshPublicKeys").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(SshPublicKey::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> TAGS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Tags")
.getter(getter(DescribedUser::tags))
.setter(setter(Builder::tags))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Tags").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Tag::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField USER_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("UserName").getter(getter(DescribedUser::userName)).setter(setter(Builder::userName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("UserName").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ARN_FIELD,
HOME_DIRECTORY_FIELD, HOME_DIRECTORY_MAPPINGS_FIELD, HOME_DIRECTORY_TYPE_FIELD, POLICY_FIELD, POSIX_PROFILE_FIELD,
ROLE_FIELD, SSH_PUBLIC_KEYS_FIELD, TAGS_FIELD, USER_NAME_FIELD));
private static final long serialVersionUID = 1L;
private final String arn;
private final String homeDirectory;
private final List homeDirectoryMappings;
private final String homeDirectoryType;
private final String policy;
private final PosixProfile posixProfile;
private final String role;
private final List sshPublicKeys;
private final List tags;
private final String userName;
private DescribedUser(BuilderImpl builder) {
this.arn = builder.arn;
this.homeDirectory = builder.homeDirectory;
this.homeDirectoryMappings = builder.homeDirectoryMappings;
this.homeDirectoryType = builder.homeDirectoryType;
this.policy = builder.policy;
this.posixProfile = builder.posixProfile;
this.role = builder.role;
this.sshPublicKeys = builder.sshPublicKeys;
this.tags = builder.tags;
this.userName = builder.userName;
}
/**
*
* Specifies the unique Amazon Resource Name (ARN) for the user that was requested to be described.
*
*
* @return Specifies the unique Amazon Resource Name (ARN) for the user that was requested to be described.
*/
public final String arn() {
return arn;
}
/**
*
* The landing directory (folder) for a user when they log in to the server using the client.
*
*
* A HomeDirectory
example is /bucket_name/home/mydirectory
.
*
*
*
* The HomeDirectory
parameter is only used if HomeDirectoryType
is set to
* PATH
.
*
*
*
* @return The landing directory (folder) for a user when they log in to the server using the client.
*
* A HomeDirectory
example is /bucket_name/home/mydirectory
.
*
*
*
* The HomeDirectory
parameter is only used if HomeDirectoryType
is set to
* PATH
.
*
*/
public final String homeDirectory() {
return homeDirectory;
}
/**
* For responses, this returns true if the service returned a value for the HomeDirectoryMappings property. This
* DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasHomeDirectoryMappings() {
return homeDirectoryMappings != null && !(homeDirectoryMappings instanceof SdkAutoConstructList);
}
/**
*
* Logical directory mappings that specify what Amazon S3 or Amazon EFS paths and keys should be visible to your
* user and how you want to make them visible. You must specify the Entry
and Target
pair,
* where Entry
shows how the path is made visible and Target
is the actual Amazon S3 or
* Amazon EFS path. If you only specify a target, it is displayed as is. You also must ensure that your Identity and
* Access Management (IAM) role provides access to paths in Target
. This value can be set only when
* HomeDirectoryType
is set to LOGICAL.
*
*
* In most cases, you can use this value instead of the session policy to lock your user down to the designated home
* directory ("chroot
"). To do this, you can set Entry
to '/' and set Target
* to the HomeDirectory parameter value.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasHomeDirectoryMappings} method.
*
*
* @return Logical directory mappings that specify what Amazon S3 or Amazon EFS paths and keys should be visible to
* your user and how you want to make them visible. You must specify the Entry
and
* Target
pair, where Entry
shows how the path is made visible and
* Target
is the actual Amazon S3 or Amazon EFS path. If you only specify a target, it is
* displayed as is. You also must ensure that your Identity and Access Management (IAM) role provides access
* to paths in Target
. This value can be set only when HomeDirectoryType
is set to
* LOGICAL.
*
* In most cases, you can use this value instead of the session policy to lock your user down to the
* designated home directory ("chroot
"). To do this, you can set Entry
to '/' and
* set Target
to the HomeDirectory parameter value.
*/
public final List homeDirectoryMappings() {
return homeDirectoryMappings;
}
/**
*
* The type of landing directory (folder) that you want your users' home directory to be when they log in to the
* server. If you set it to PATH
, the user will see the absolute Amazon S3 bucket or Amazon EFS path as
* is in their file transfer protocol clients. If you set it to LOGICAL
, you need to provide mappings
* in the HomeDirectoryMappings
for how you want to make Amazon S3 or Amazon EFS paths visible to your
* users.
*
*
*
* If HomeDirectoryType
is LOGICAL
, you must provide mappings, using the
* HomeDirectoryMappings
parameter. If, on the other hand, HomeDirectoryType
is
* PATH
, you provide an absolute path using the HomeDirectory
parameter. You cannot have
* both HomeDirectory
and HomeDirectoryMappings
in your template.
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #homeDirectoryType}
* will return {@link HomeDirectoryType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #homeDirectoryTypeAsString}.
*
*
* @return The type of landing directory (folder) that you want your users' home directory to be when they log in to
* the server. If you set it to PATH
, the user will see the absolute Amazon S3 bucket or Amazon
* EFS path as is in their file transfer protocol clients. If you set it to LOGICAL
, you need
* to provide mappings in the HomeDirectoryMappings
for how you want to make Amazon S3 or
* Amazon EFS paths visible to your users.
*
* If HomeDirectoryType
is LOGICAL
, you must provide mappings, using the
* HomeDirectoryMappings
parameter. If, on the other hand, HomeDirectoryType
is
* PATH
, you provide an absolute path using the HomeDirectory
parameter. You
* cannot have both HomeDirectory
and HomeDirectoryMappings
in your template.
*
* @see HomeDirectoryType
*/
public final HomeDirectoryType homeDirectoryType() {
return HomeDirectoryType.fromValue(homeDirectoryType);
}
/**
*
* The type of landing directory (folder) that you want your users' home directory to be when they log in to the
* server. If you set it to PATH
, the user will see the absolute Amazon S3 bucket or Amazon EFS path as
* is in their file transfer protocol clients. If you set it to LOGICAL
, you need to provide mappings
* in the HomeDirectoryMappings
for how you want to make Amazon S3 or Amazon EFS paths visible to your
* users.
*
*
*
* If HomeDirectoryType
is LOGICAL
, you must provide mappings, using the
* HomeDirectoryMappings
parameter. If, on the other hand, HomeDirectoryType
is
* PATH
, you provide an absolute path using the HomeDirectory
parameter. You cannot have
* both HomeDirectory
and HomeDirectoryMappings
in your template.
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #homeDirectoryType}
* will return {@link HomeDirectoryType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #homeDirectoryTypeAsString}.
*
*
* @return The type of landing directory (folder) that you want your users' home directory to be when they log in to
* the server. If you set it to PATH
, the user will see the absolute Amazon S3 bucket or Amazon
* EFS path as is in their file transfer protocol clients. If you set it to LOGICAL
, you need
* to provide mappings in the HomeDirectoryMappings
for how you want to make Amazon S3 or
* Amazon EFS paths visible to your users.
*
* If HomeDirectoryType
is LOGICAL
, you must provide mappings, using the
* HomeDirectoryMappings
parameter. If, on the other hand, HomeDirectoryType
is
* PATH
, you provide an absolute path using the HomeDirectory
parameter. You
* cannot have both HomeDirectory
and HomeDirectoryMappings
in your template.
*
* @see HomeDirectoryType
*/
public final String homeDirectoryTypeAsString() {
return homeDirectoryType;
}
/**
*
* A session policy for your user so that you can use the same Identity and Access Management (IAM) role across
* multiple users. This policy scopes down a user's access to portions of their Amazon S3 bucket. Variables that you
* can use inside this policy include ${Transfer:UserName}
, ${Transfer:HomeDirectory}
, and
* ${Transfer:HomeBucket}
.
*
*
* @return A session policy for your user so that you can use the same Identity and Access Management (IAM) role
* across multiple users. This policy scopes down a user's access to portions of their Amazon S3 bucket.
* Variables that you can use inside this policy include ${Transfer:UserName}
,
* ${Transfer:HomeDirectory}
, and ${Transfer:HomeBucket}
.
*/
public final String policy() {
return policy;
}
/**
*
* Specifies the full POSIX identity, including user ID (Uid
), group ID (Gid
), and any
* secondary groups IDs (SecondaryGids
), that controls your users' access to your Amazon Elastic File
* System (Amazon EFS) file systems. The POSIX permissions that are set on files and directories in your file system
* determine the level of access your users get when transferring files into and out of your Amazon EFS file
* systems.
*
*
* @return Specifies the full POSIX identity, including user ID (Uid
), group ID (Gid
), and
* any secondary groups IDs (SecondaryGids
), that controls your users' access to your Amazon
* Elastic File System (Amazon EFS) file systems. The POSIX permissions that are set on files and
* directories in your file system determine the level of access your users get when transferring files into
* and out of your Amazon EFS file systems.
*/
public final PosixProfile posixProfile() {
return posixProfile;
}
/**
*
* The Amazon Resource Name (ARN) of the Identity and Access Management (IAM) role that controls your users' access
* to your Amazon S3 bucket or Amazon EFS file system. The policies attached to this role determine the level of
* access that you want to provide your users when transferring files into and out of your Amazon S3 bucket or
* Amazon EFS file system. The IAM role should also contain a trust relationship that allows the server to access
* your resources when servicing your users' transfer requests.
*
*
* @return The Amazon Resource Name (ARN) of the Identity and Access Management (IAM) role that controls your users'
* access to your Amazon S3 bucket or Amazon EFS file system. The policies attached to this role determine
* the level of access that you want to provide your users when transferring files into and out of your
* Amazon S3 bucket or Amazon EFS file system. The IAM role should also contain a trust relationship that
* allows the server to access your resources when servicing your users' transfer requests.
*/
public final String role() {
return role;
}
/**
* For responses, this returns true if the service returned a value for the SshPublicKeys property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasSshPublicKeys() {
return sshPublicKeys != null && !(sshPublicKeys instanceof SdkAutoConstructList);
}
/**
*
* Specifies the public key portion of the Secure Shell (SSH) keys stored for the described user.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasSshPublicKeys} method.
*
*
* @return Specifies the public key portion of the Secure Shell (SSH) keys stored for the described user.
*/
public final List sshPublicKeys() {
return sshPublicKeys;
}
/**
* For responses, this returns true if the service returned a value for the Tags property. This DOES NOT check that
* the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is useful
* because the SDK will never return a null collection or map, but you may need to differentiate between the service
* returning nothing (or null) and the service returning an empty collection or map. For requests, this returns true
* if a value for the property was specified in the request builder, and false if a value was not specified.
*/
public final boolean hasTags() {
return tags != null && !(tags instanceof SdkAutoConstructList);
}
/**
*
* Specifies the key-value pairs for the user requested. Tag can be used to search for and group users for a variety
* of purposes.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasTags} method.
*
*
* @return Specifies the key-value pairs for the user requested. Tag can be used to search for and group users for a
* variety of purposes.
*/
public final List tags() {
return tags;
}
/**
*
* Specifies the name of the user that was requested to be described. User names are used for authentication
* purposes. This is the string that will be used by your user when they log in to your server.
*
*
* @return Specifies the name of the user that was requested to be described. User names are used for authentication
* purposes. This is the string that will be used by your user when they log in to your server.
*/
public final String userName() {
return userName;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(arn());
hashCode = 31 * hashCode + Objects.hashCode(homeDirectory());
hashCode = 31 * hashCode + Objects.hashCode(hasHomeDirectoryMappings() ? homeDirectoryMappings() : null);
hashCode = 31 * hashCode + Objects.hashCode(homeDirectoryTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(policy());
hashCode = 31 * hashCode + Objects.hashCode(posixProfile());
hashCode = 31 * hashCode + Objects.hashCode(role());
hashCode = 31 * hashCode + Objects.hashCode(hasSshPublicKeys() ? sshPublicKeys() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasTags() ? tags() : null);
hashCode = 31 * hashCode + Objects.hashCode(userName());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof DescribedUser)) {
return false;
}
DescribedUser other = (DescribedUser) obj;
return Objects.equals(arn(), other.arn()) && Objects.equals(homeDirectory(), other.homeDirectory())
&& hasHomeDirectoryMappings() == other.hasHomeDirectoryMappings()
&& Objects.equals(homeDirectoryMappings(), other.homeDirectoryMappings())
&& Objects.equals(homeDirectoryTypeAsString(), other.homeDirectoryTypeAsString())
&& Objects.equals(policy(), other.policy()) && Objects.equals(posixProfile(), other.posixProfile())
&& Objects.equals(role(), other.role()) && hasSshPublicKeys() == other.hasSshPublicKeys()
&& Objects.equals(sshPublicKeys(), other.sshPublicKeys()) && hasTags() == other.hasTags()
&& Objects.equals(tags(), other.tags()) && Objects.equals(userName(), other.userName());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("DescribedUser").add("Arn", arn()).add("HomeDirectory", homeDirectory())
.add("HomeDirectoryMappings", hasHomeDirectoryMappings() ? homeDirectoryMappings() : null)
.add("HomeDirectoryType", homeDirectoryTypeAsString()).add("Policy", policy())
.add("PosixProfile", posixProfile()).add("Role", role())
.add("SshPublicKeys", hasSshPublicKeys() ? sshPublicKeys() : null).add("Tags", hasTags() ? tags() : null)
.add("UserName", userName()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Arn":
return Optional.ofNullable(clazz.cast(arn()));
case "HomeDirectory":
return Optional.ofNullable(clazz.cast(homeDirectory()));
case "HomeDirectoryMappings":
return Optional.ofNullable(clazz.cast(homeDirectoryMappings()));
case "HomeDirectoryType":
return Optional.ofNullable(clazz.cast(homeDirectoryTypeAsString()));
case "Policy":
return Optional.ofNullable(clazz.cast(policy()));
case "PosixProfile":
return Optional.ofNullable(clazz.cast(posixProfile()));
case "Role":
return Optional.ofNullable(clazz.cast(role()));
case "SshPublicKeys":
return Optional.ofNullable(clazz.cast(sshPublicKeys()));
case "Tags":
return Optional.ofNullable(clazz.cast(tags()));
case "UserName":
return Optional.ofNullable(clazz.cast(userName()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function