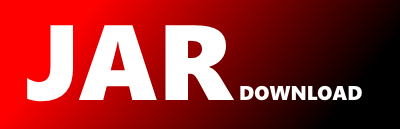
software.amazon.awssdk.services.transfer.model.S3InputFileLocation Maven / Gradle / Ivy
Show all versions of transfer Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.transfer.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Specifies the customer input Amazon S3 file location. If it is used inside
* copyStepDetails.DestinationFileLocation
, it should be the S3 copy destination.
*
*
* You need to provide the bucket and key. The key can represent either a path or a file. This is determined by whether
* or not you end the key value with the forward slash (/) character. If the final character is "/", then your file is
* copied to the folder, and its name does not change. If, rather, the final character is alphanumeric, your uploaded
* file is renamed to the path value. In this case, if a file with that name already exists, it is overwritten.
*
*
* For example, if your path is shared-files/bob/
, your uploaded files are copied to the
* shared-files/bob/
, folder. If your path is shared-files/today
, each uploaded file is copied
* to the shared-files
folder and named today
: each upload overwrites the previous version of
* the bob file.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class S3InputFileLocation implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField BUCKET_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Bucket")
.getter(getter(S3InputFileLocation::bucket)).setter(setter(Builder::bucket))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Bucket").build()).build();
private static final SdkField KEY_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Key")
.getter(getter(S3InputFileLocation::key)).setter(setter(Builder::key))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Key").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(BUCKET_FIELD, KEY_FIELD));
private static final long serialVersionUID = 1L;
private final String bucket;
private final String key;
private S3InputFileLocation(BuilderImpl builder) {
this.bucket = builder.bucket;
this.key = builder.key;
}
/**
*
* Specifies the S3 bucket for the customer input file.
*
*
* @return Specifies the S3 bucket for the customer input file.
*/
public final String bucket() {
return bucket;
}
/**
*
* The name assigned to the file when it was created in Amazon S3. You use the object key to retrieve the object.
*
*
* @return The name assigned to the file when it was created in Amazon S3. You use the object key to retrieve the
* object.
*/
public final String key() {
return key;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(bucket());
hashCode = 31 * hashCode + Objects.hashCode(key());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof S3InputFileLocation)) {
return false;
}
S3InputFileLocation other = (S3InputFileLocation) obj;
return Objects.equals(bucket(), other.bucket()) && Objects.equals(key(), other.key());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("S3InputFileLocation").add("Bucket", bucket()).add("Key", key()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Bucket":
return Optional.ofNullable(clazz.cast(bucket()));
case "Key":
return Optional.ofNullable(clazz.cast(key()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function