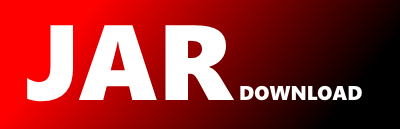
software.amazon.awssdk.services.transfer.TransferAsyncClient Maven / Gradle / Ivy
Show all versions of transfer Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.transfer;
import java.util.concurrent.CompletableFuture;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkPublicApi;
import software.amazon.awssdk.annotations.ThreadSafe;
import software.amazon.awssdk.awscore.AwsClient;
import software.amazon.awssdk.services.transfer.model.CreateAccessRequest;
import software.amazon.awssdk.services.transfer.model.CreateAccessResponse;
import software.amazon.awssdk.services.transfer.model.CreateAgreementRequest;
import software.amazon.awssdk.services.transfer.model.CreateAgreementResponse;
import software.amazon.awssdk.services.transfer.model.CreateConnectorRequest;
import software.amazon.awssdk.services.transfer.model.CreateConnectorResponse;
import software.amazon.awssdk.services.transfer.model.CreateProfileRequest;
import software.amazon.awssdk.services.transfer.model.CreateProfileResponse;
import software.amazon.awssdk.services.transfer.model.CreateServerRequest;
import software.amazon.awssdk.services.transfer.model.CreateServerResponse;
import software.amazon.awssdk.services.transfer.model.CreateUserRequest;
import software.amazon.awssdk.services.transfer.model.CreateUserResponse;
import software.amazon.awssdk.services.transfer.model.CreateWorkflowRequest;
import software.amazon.awssdk.services.transfer.model.CreateWorkflowResponse;
import software.amazon.awssdk.services.transfer.model.DeleteAccessRequest;
import software.amazon.awssdk.services.transfer.model.DeleteAccessResponse;
import software.amazon.awssdk.services.transfer.model.DeleteAgreementRequest;
import software.amazon.awssdk.services.transfer.model.DeleteAgreementResponse;
import software.amazon.awssdk.services.transfer.model.DeleteCertificateRequest;
import software.amazon.awssdk.services.transfer.model.DeleteCertificateResponse;
import software.amazon.awssdk.services.transfer.model.DeleteConnectorRequest;
import software.amazon.awssdk.services.transfer.model.DeleteConnectorResponse;
import software.amazon.awssdk.services.transfer.model.DeleteHostKeyRequest;
import software.amazon.awssdk.services.transfer.model.DeleteHostKeyResponse;
import software.amazon.awssdk.services.transfer.model.DeleteProfileRequest;
import software.amazon.awssdk.services.transfer.model.DeleteProfileResponse;
import software.amazon.awssdk.services.transfer.model.DeleteServerRequest;
import software.amazon.awssdk.services.transfer.model.DeleteServerResponse;
import software.amazon.awssdk.services.transfer.model.DeleteSshPublicKeyRequest;
import software.amazon.awssdk.services.transfer.model.DeleteSshPublicKeyResponse;
import software.amazon.awssdk.services.transfer.model.DeleteUserRequest;
import software.amazon.awssdk.services.transfer.model.DeleteUserResponse;
import software.amazon.awssdk.services.transfer.model.DeleteWorkflowRequest;
import software.amazon.awssdk.services.transfer.model.DeleteWorkflowResponse;
import software.amazon.awssdk.services.transfer.model.DescribeAccessRequest;
import software.amazon.awssdk.services.transfer.model.DescribeAccessResponse;
import software.amazon.awssdk.services.transfer.model.DescribeAgreementRequest;
import software.amazon.awssdk.services.transfer.model.DescribeAgreementResponse;
import software.amazon.awssdk.services.transfer.model.DescribeCertificateRequest;
import software.amazon.awssdk.services.transfer.model.DescribeCertificateResponse;
import software.amazon.awssdk.services.transfer.model.DescribeConnectorRequest;
import software.amazon.awssdk.services.transfer.model.DescribeConnectorResponse;
import software.amazon.awssdk.services.transfer.model.DescribeExecutionRequest;
import software.amazon.awssdk.services.transfer.model.DescribeExecutionResponse;
import software.amazon.awssdk.services.transfer.model.DescribeHostKeyRequest;
import software.amazon.awssdk.services.transfer.model.DescribeHostKeyResponse;
import software.amazon.awssdk.services.transfer.model.DescribeProfileRequest;
import software.amazon.awssdk.services.transfer.model.DescribeProfileResponse;
import software.amazon.awssdk.services.transfer.model.DescribeSecurityPolicyRequest;
import software.amazon.awssdk.services.transfer.model.DescribeSecurityPolicyResponse;
import software.amazon.awssdk.services.transfer.model.DescribeServerRequest;
import software.amazon.awssdk.services.transfer.model.DescribeServerResponse;
import software.amazon.awssdk.services.transfer.model.DescribeUserRequest;
import software.amazon.awssdk.services.transfer.model.DescribeUserResponse;
import software.amazon.awssdk.services.transfer.model.DescribeWorkflowRequest;
import software.amazon.awssdk.services.transfer.model.DescribeWorkflowResponse;
import software.amazon.awssdk.services.transfer.model.ImportCertificateRequest;
import software.amazon.awssdk.services.transfer.model.ImportCertificateResponse;
import software.amazon.awssdk.services.transfer.model.ImportHostKeyRequest;
import software.amazon.awssdk.services.transfer.model.ImportHostKeyResponse;
import software.amazon.awssdk.services.transfer.model.ImportSshPublicKeyRequest;
import software.amazon.awssdk.services.transfer.model.ImportSshPublicKeyResponse;
import software.amazon.awssdk.services.transfer.model.ListAccessesRequest;
import software.amazon.awssdk.services.transfer.model.ListAccessesResponse;
import software.amazon.awssdk.services.transfer.model.ListAgreementsRequest;
import software.amazon.awssdk.services.transfer.model.ListAgreementsResponse;
import software.amazon.awssdk.services.transfer.model.ListCertificatesRequest;
import software.amazon.awssdk.services.transfer.model.ListCertificatesResponse;
import software.amazon.awssdk.services.transfer.model.ListConnectorsRequest;
import software.amazon.awssdk.services.transfer.model.ListConnectorsResponse;
import software.amazon.awssdk.services.transfer.model.ListExecutionsRequest;
import software.amazon.awssdk.services.transfer.model.ListExecutionsResponse;
import software.amazon.awssdk.services.transfer.model.ListHostKeysRequest;
import software.amazon.awssdk.services.transfer.model.ListHostKeysResponse;
import software.amazon.awssdk.services.transfer.model.ListProfilesRequest;
import software.amazon.awssdk.services.transfer.model.ListProfilesResponse;
import software.amazon.awssdk.services.transfer.model.ListSecurityPoliciesRequest;
import software.amazon.awssdk.services.transfer.model.ListSecurityPoliciesResponse;
import software.amazon.awssdk.services.transfer.model.ListServersRequest;
import software.amazon.awssdk.services.transfer.model.ListServersResponse;
import software.amazon.awssdk.services.transfer.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.transfer.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.transfer.model.ListUsersRequest;
import software.amazon.awssdk.services.transfer.model.ListUsersResponse;
import software.amazon.awssdk.services.transfer.model.ListWorkflowsRequest;
import software.amazon.awssdk.services.transfer.model.ListWorkflowsResponse;
import software.amazon.awssdk.services.transfer.model.SendWorkflowStepStateRequest;
import software.amazon.awssdk.services.transfer.model.SendWorkflowStepStateResponse;
import software.amazon.awssdk.services.transfer.model.StartDirectoryListingRequest;
import software.amazon.awssdk.services.transfer.model.StartDirectoryListingResponse;
import software.amazon.awssdk.services.transfer.model.StartFileTransferRequest;
import software.amazon.awssdk.services.transfer.model.StartFileTransferResponse;
import software.amazon.awssdk.services.transfer.model.StartServerRequest;
import software.amazon.awssdk.services.transfer.model.StartServerResponse;
import software.amazon.awssdk.services.transfer.model.StopServerRequest;
import software.amazon.awssdk.services.transfer.model.StopServerResponse;
import software.amazon.awssdk.services.transfer.model.TagResourceRequest;
import software.amazon.awssdk.services.transfer.model.TagResourceResponse;
import software.amazon.awssdk.services.transfer.model.TestConnectionRequest;
import software.amazon.awssdk.services.transfer.model.TestConnectionResponse;
import software.amazon.awssdk.services.transfer.model.TestIdentityProviderRequest;
import software.amazon.awssdk.services.transfer.model.TestIdentityProviderResponse;
import software.amazon.awssdk.services.transfer.model.UntagResourceRequest;
import software.amazon.awssdk.services.transfer.model.UntagResourceResponse;
import software.amazon.awssdk.services.transfer.model.UpdateAccessRequest;
import software.amazon.awssdk.services.transfer.model.UpdateAccessResponse;
import software.amazon.awssdk.services.transfer.model.UpdateAgreementRequest;
import software.amazon.awssdk.services.transfer.model.UpdateAgreementResponse;
import software.amazon.awssdk.services.transfer.model.UpdateCertificateRequest;
import software.amazon.awssdk.services.transfer.model.UpdateCertificateResponse;
import software.amazon.awssdk.services.transfer.model.UpdateConnectorRequest;
import software.amazon.awssdk.services.transfer.model.UpdateConnectorResponse;
import software.amazon.awssdk.services.transfer.model.UpdateHostKeyRequest;
import software.amazon.awssdk.services.transfer.model.UpdateHostKeyResponse;
import software.amazon.awssdk.services.transfer.model.UpdateProfileRequest;
import software.amazon.awssdk.services.transfer.model.UpdateProfileResponse;
import software.amazon.awssdk.services.transfer.model.UpdateServerRequest;
import software.amazon.awssdk.services.transfer.model.UpdateServerResponse;
import software.amazon.awssdk.services.transfer.model.UpdateUserRequest;
import software.amazon.awssdk.services.transfer.model.UpdateUserResponse;
import software.amazon.awssdk.services.transfer.paginators.ListAccessesPublisher;
import software.amazon.awssdk.services.transfer.paginators.ListAgreementsPublisher;
import software.amazon.awssdk.services.transfer.paginators.ListCertificatesPublisher;
import software.amazon.awssdk.services.transfer.paginators.ListConnectorsPublisher;
import software.amazon.awssdk.services.transfer.paginators.ListExecutionsPublisher;
import software.amazon.awssdk.services.transfer.paginators.ListProfilesPublisher;
import software.amazon.awssdk.services.transfer.paginators.ListSecurityPoliciesPublisher;
import software.amazon.awssdk.services.transfer.paginators.ListServersPublisher;
import software.amazon.awssdk.services.transfer.paginators.ListTagsForResourcePublisher;
import software.amazon.awssdk.services.transfer.paginators.ListUsersPublisher;
import software.amazon.awssdk.services.transfer.paginators.ListWorkflowsPublisher;
import software.amazon.awssdk.services.transfer.waiters.TransferAsyncWaiter;
/**
* Service client for accessing AWS Transfer asynchronously. This can be created using the static {@link #builder()}
* method.The asynchronous client performs non-blocking I/O when configured with any {@code SdkAsyncHttpClient}
* supported in the SDK. However, full non-blocking is not guaranteed as the async client may perform blocking calls in
* some cases such as credentials retrieval and endpoint discovery as part of the async API call.
*
*
* Transfer Family is a fully managed service that enables the transfer of files over the File Transfer Protocol (FTP),
* File Transfer Protocol over SSL (FTPS), or Secure Shell (SSH) File Transfer Protocol (SFTP) directly into and out of
* Amazon Simple Storage Service (Amazon S3) or Amazon EFS. Additionally, you can use Applicability Statement 2 (AS2) to
* transfer files into and out of Amazon S3. Amazon Web Services helps you seamlessly migrate your file transfer
* workflows to Transfer Family by integrating with existing authentication systems, and providing DNS routing with
* Amazon Route 53 so nothing changes for your customers and partners, or their applications. With your data in Amazon
* S3, you can use it with Amazon Web Services for processing, analytics, machine learning, and archiving. Getting
* started with Transfer Family is easy since there is no infrastructure to buy and set up.
*
*/
@Generated("software.amazon.awssdk:codegen")
@SdkPublicApi
@ThreadSafe
public interface TransferAsyncClient extends AwsClient {
String SERVICE_NAME = "transfer";
/**
* Value for looking up the service's metadata from the
* {@link software.amazon.awssdk.regions.ServiceMetadataProvider}.
*/
String SERVICE_METADATA_ID = "transfer";
/**
*
* Used by administrators to choose which groups in the directory should have access to upload and download files
* over the enabled protocols using Transfer Family. For example, a Microsoft Active Directory might contain 50,000
* users, but only a small fraction might need the ability to transfer files to the server. An administrator can use
* CreateAccess
to limit the access to the correct set of users who need this ability.
*
*
* @param createAccessRequest
* @return A Java Future containing the result of the CreateAccess operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - ResourceExistsException The requested resource does not exist, or exists in a region other than the
* one specified for the command.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.CreateAccess
* @see AWS API
* Documentation
*/
default CompletableFuture createAccess(CreateAccessRequest createAccessRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Used by administrators to choose which groups in the directory should have access to upload and download files
* over the enabled protocols using Transfer Family. For example, a Microsoft Active Directory might contain 50,000
* users, but only a small fraction might need the ability to transfer files to the server. An administrator can use
* CreateAccess
to limit the access to the correct set of users who need this ability.
*
*
*
* This is a convenience which creates an instance of the {@link CreateAccessRequest.Builder} avoiding the need to
* create one manually via {@link CreateAccessRequest#builder()}
*
*
* @param createAccessRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transfer.model.CreateAccessRequest.Builder} to create a request.
* @return A Java Future containing the result of the CreateAccess operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - ResourceExistsException The requested resource does not exist, or exists in a region other than the
* one specified for the command.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.CreateAccess
* @see AWS API
* Documentation
*/
default CompletableFuture createAccess(Consumer createAccessRequest) {
return createAccess(CreateAccessRequest.builder().applyMutation(createAccessRequest).build());
}
/**
*
* Creates an agreement. An agreement is a bilateral trading partner agreement, or partnership, between an Transfer
* Family server and an AS2 process. The agreement defines the file and message transfer relationship between the
* server and the AS2 process. To define an agreement, Transfer Family combines a server, local profile, partner
* profile, certificate, and other attributes.
*
*
* The partner is identified with the PartnerProfileId
, and the AS2 process is identified with the
* LocalProfileId
.
*
*
* @param createAgreementRequest
* @return A Java Future containing the result of the CreateAgreement operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - ResourceExistsException The requested resource does not exist, or exists in a region other than the
* one specified for the command.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.CreateAgreement
* @see AWS API
* Documentation
*/
default CompletableFuture createAgreement(CreateAgreementRequest createAgreementRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates an agreement. An agreement is a bilateral trading partner agreement, or partnership, between an Transfer
* Family server and an AS2 process. The agreement defines the file and message transfer relationship between the
* server and the AS2 process. To define an agreement, Transfer Family combines a server, local profile, partner
* profile, certificate, and other attributes.
*
*
* The partner is identified with the PartnerProfileId
, and the AS2 process is identified with the
* LocalProfileId
.
*
*
*
* This is a convenience which creates an instance of the {@link CreateAgreementRequest.Builder} avoiding the need
* to create one manually via {@link CreateAgreementRequest#builder()}
*
*
* @param createAgreementRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transfer.model.CreateAgreementRequest.Builder} to create a request.
* @return A Java Future containing the result of the CreateAgreement operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - ResourceExistsException The requested resource does not exist, or exists in a region other than the
* one specified for the command.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.CreateAgreement
* @see AWS API
* Documentation
*/
default CompletableFuture createAgreement(
Consumer createAgreementRequest) {
return createAgreement(CreateAgreementRequest.builder().applyMutation(createAgreementRequest).build());
}
/**
*
* Creates the connector, which captures the parameters for a connection for the AS2 or SFTP protocol. For AS2, the
* connector is required for sending files to an externally hosted AS2 server. For SFTP, the connector is required
* when sending files to an SFTP server or receiving files from an SFTP server. For more details about connectors,
* see Configure AS2
* connectors and Create SFTP
* connectors.
*
*
*
* You must specify exactly one configuration object: either for AS2 (As2Config
) or SFTP (
* SftpConfig
).
*
*
*
* @param createConnectorRequest
* @return A Java Future containing the result of the CreateConnector operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - ResourceExistsException The requested resource does not exist, or exists in a region other than the
* one specified for the command.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.CreateConnector
* @see AWS API
* Documentation
*/
default CompletableFuture createConnector(CreateConnectorRequest createConnectorRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates the connector, which captures the parameters for a connection for the AS2 or SFTP protocol. For AS2, the
* connector is required for sending files to an externally hosted AS2 server. For SFTP, the connector is required
* when sending files to an SFTP server or receiving files from an SFTP server. For more details about connectors,
* see Configure AS2
* connectors and Create SFTP
* connectors.
*
*
*
* You must specify exactly one configuration object: either for AS2 (As2Config
) or SFTP (
* SftpConfig
).
*
*
*
* This is a convenience which creates an instance of the {@link CreateConnectorRequest.Builder} avoiding the need
* to create one manually via {@link CreateConnectorRequest#builder()}
*
*
* @param createConnectorRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transfer.model.CreateConnectorRequest.Builder} to create a request.
* @return A Java Future containing the result of the CreateConnector operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - ResourceExistsException The requested resource does not exist, or exists in a region other than the
* one specified for the command.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.CreateConnector
* @see AWS API
* Documentation
*/
default CompletableFuture createConnector(
Consumer createConnectorRequest) {
return createConnector(CreateConnectorRequest.builder().applyMutation(createConnectorRequest).build());
}
/**
*
* Creates the local or partner profile to use for AS2 transfers.
*
*
* @param createProfileRequest
* @return A Java Future containing the result of the CreateProfile operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.CreateProfile
* @see AWS API
* Documentation
*/
default CompletableFuture createProfile(CreateProfileRequest createProfileRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates the local or partner profile to use for AS2 transfers.
*
*
*
* This is a convenience which creates an instance of the {@link CreateProfileRequest.Builder} avoiding the need to
* create one manually via {@link CreateProfileRequest#builder()}
*
*
* @param createProfileRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transfer.model.CreateProfileRequest.Builder} to create a request.
* @return A Java Future containing the result of the CreateProfile operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.CreateProfile
* @see AWS API
* Documentation
*/
default CompletableFuture createProfile(Consumer createProfileRequest) {
return createProfile(CreateProfileRequest.builder().applyMutation(createProfileRequest).build());
}
/**
*
* Instantiates an auto-scaling virtual server based on the selected file transfer protocol in Amazon Web Services.
* When you make updates to your file transfer protocol-enabled server or when you work with users, use the
* service-generated ServerId
property that is assigned to the newly created server.
*
*
* @param createServerRequest
* @return A Java Future containing the result of the CreateServer operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - ResourceExistsException The requested resource does not exist, or exists in a region other than the
* one specified for the command.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.CreateServer
* @see AWS API
* Documentation
*/
default CompletableFuture createServer(CreateServerRequest createServerRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Instantiates an auto-scaling virtual server based on the selected file transfer protocol in Amazon Web Services.
* When you make updates to your file transfer protocol-enabled server or when you work with users, use the
* service-generated ServerId
property that is assigned to the newly created server.
*
*
*
* This is a convenience which creates an instance of the {@link CreateServerRequest.Builder} avoiding the need to
* create one manually via {@link CreateServerRequest#builder()}
*
*
* @param createServerRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transfer.model.CreateServerRequest.Builder} to create a request.
* @return A Java Future containing the result of the CreateServer operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - ResourceExistsException The requested resource does not exist, or exists in a region other than the
* one specified for the command.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.CreateServer
* @see AWS API
* Documentation
*/
default CompletableFuture createServer(Consumer createServerRequest) {
return createServer(CreateServerRequest.builder().applyMutation(createServerRequest).build());
}
/**
*
* Creates a user and associates them with an existing file transfer protocol-enabled server. You can only create
* and associate users with servers that have the IdentityProviderType
set to
* SERVICE_MANAGED
. Using parameters for CreateUser
, you can specify the user name, set
* the home directory, store the user's public key, and assign the user's Identity and Access Management (IAM) role.
* You can also optionally add a session policy, and assign metadata with tags that can be used to group and search
* for users.
*
*
* @param createUserRequest
* @return A Java Future containing the result of the CreateUser operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - ResourceExistsException The requested resource does not exist, or exists in a region other than the
* one specified for the command.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.CreateUser
* @see AWS API
* Documentation
*/
default CompletableFuture createUser(CreateUserRequest createUserRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a user and associates them with an existing file transfer protocol-enabled server. You can only create
* and associate users with servers that have the IdentityProviderType
set to
* SERVICE_MANAGED
. Using parameters for CreateUser
, you can specify the user name, set
* the home directory, store the user's public key, and assign the user's Identity and Access Management (IAM) role.
* You can also optionally add a session policy, and assign metadata with tags that can be used to group and search
* for users.
*
*
*
* This is a convenience which creates an instance of the {@link CreateUserRequest.Builder} avoiding the need to
* create one manually via {@link CreateUserRequest#builder()}
*
*
* @param createUserRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transfer.model.CreateUserRequest.Builder} to create a request.
* @return A Java Future containing the result of the CreateUser operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - ResourceExistsException The requested resource does not exist, or exists in a region other than the
* one specified for the command.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.CreateUser
* @see AWS API
* Documentation
*/
default CompletableFuture createUser(Consumer createUserRequest) {
return createUser(CreateUserRequest.builder().applyMutation(createUserRequest).build());
}
/**
*
* Allows you to create a workflow with specified steps and step details the workflow invokes after file transfer
* completes. After creating a workflow, you can associate the workflow created with any transfer servers by
* specifying the workflow-details
field in CreateServer
and UpdateServer
* operations.
*
*
* @param createWorkflowRequest
* @return A Java Future containing the result of the CreateWorkflow operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - ResourceExistsException The requested resource does not exist, or exists in a region other than the
* one specified for the command.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.CreateWorkflow
* @see AWS API
* Documentation
*/
default CompletableFuture createWorkflow(CreateWorkflowRequest createWorkflowRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Allows you to create a workflow with specified steps and step details the workflow invokes after file transfer
* completes. After creating a workflow, you can associate the workflow created with any transfer servers by
* specifying the workflow-details
field in CreateServer
and UpdateServer
* operations.
*
*
*
* This is a convenience which creates an instance of the {@link CreateWorkflowRequest.Builder} avoiding the need to
* create one manually via {@link CreateWorkflowRequest#builder()}
*
*
* @param createWorkflowRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transfer.model.CreateWorkflowRequest.Builder} to create a request.
* @return A Java Future containing the result of the CreateWorkflow operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - ResourceExistsException The requested resource does not exist, or exists in a region other than the
* one specified for the command.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.CreateWorkflow
* @see AWS API
* Documentation
*/
default CompletableFuture createWorkflow(Consumer createWorkflowRequest) {
return createWorkflow(CreateWorkflowRequest.builder().applyMutation(createWorkflowRequest).build());
}
/**
*
* Allows you to delete the access specified in the ServerID
and ExternalID
parameters.
*
*
* @param deleteAccessRequest
* @return A Java Future containing the result of the DeleteAccess operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.DeleteAccess
* @see AWS API
* Documentation
*/
default CompletableFuture deleteAccess(DeleteAccessRequest deleteAccessRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Allows you to delete the access specified in the ServerID
and ExternalID
parameters.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteAccessRequest.Builder} avoiding the need to
* create one manually via {@link DeleteAccessRequest#builder()}
*
*
* @param deleteAccessRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transfer.model.DeleteAccessRequest.Builder} to create a request.
* @return A Java Future containing the result of the DeleteAccess operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.DeleteAccess
* @see AWS API
* Documentation
*/
default CompletableFuture deleteAccess(Consumer deleteAccessRequest) {
return deleteAccess(DeleteAccessRequest.builder().applyMutation(deleteAccessRequest).build());
}
/**
*
* Delete the agreement that's specified in the provided AgreementId
.
*
*
* @param deleteAgreementRequest
* @return A Java Future containing the result of the DeleteAgreement operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.DeleteAgreement
* @see AWS API
* Documentation
*/
default CompletableFuture deleteAgreement(DeleteAgreementRequest deleteAgreementRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Delete the agreement that's specified in the provided AgreementId
.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteAgreementRequest.Builder} avoiding the need
* to create one manually via {@link DeleteAgreementRequest#builder()}
*
*
* @param deleteAgreementRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transfer.model.DeleteAgreementRequest.Builder} to create a request.
* @return A Java Future containing the result of the DeleteAgreement operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.DeleteAgreement
* @see AWS API
* Documentation
*/
default CompletableFuture deleteAgreement(
Consumer deleteAgreementRequest) {
return deleteAgreement(DeleteAgreementRequest.builder().applyMutation(deleteAgreementRequest).build());
}
/**
*
* Deletes the certificate that's specified in the CertificateId
parameter.
*
*
* @param deleteCertificateRequest
* @return A Java Future containing the result of the DeleteCertificate operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.DeleteCertificate
* @see AWS
* API Documentation
*/
default CompletableFuture deleteCertificate(DeleteCertificateRequest deleteCertificateRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the certificate that's specified in the CertificateId
parameter.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteCertificateRequest.Builder} avoiding the need
* to create one manually via {@link DeleteCertificateRequest#builder()}
*
*
* @param deleteCertificateRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transfer.model.DeleteCertificateRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DeleteCertificate operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.DeleteCertificate
* @see AWS
* API Documentation
*/
default CompletableFuture deleteCertificate(
Consumer deleteCertificateRequest) {
return deleteCertificate(DeleteCertificateRequest.builder().applyMutation(deleteCertificateRequest).build());
}
/**
*
* Deletes the connector that's specified in the provided ConnectorId
.
*
*
* @param deleteConnectorRequest
* @return A Java Future containing the result of the DeleteConnector operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.DeleteConnector
* @see AWS API
* Documentation
*/
default CompletableFuture deleteConnector(DeleteConnectorRequest deleteConnectorRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the connector that's specified in the provided ConnectorId
.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteConnectorRequest.Builder} avoiding the need
* to create one manually via {@link DeleteConnectorRequest#builder()}
*
*
* @param deleteConnectorRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transfer.model.DeleteConnectorRequest.Builder} to create a request.
* @return A Java Future containing the result of the DeleteConnector operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.DeleteConnector
* @see AWS API
* Documentation
*/
default CompletableFuture deleteConnector(
Consumer deleteConnectorRequest) {
return deleteConnector(DeleteConnectorRequest.builder().applyMutation(deleteConnectorRequest).build());
}
/**
*
* Deletes the host key that's specified in the HostKeyId
parameter.
*
*
* @param deleteHostKeyRequest
* @return A Java Future containing the result of the DeleteHostKey operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.DeleteHostKey
* @see AWS API
* Documentation
*/
default CompletableFuture deleteHostKey(DeleteHostKeyRequest deleteHostKeyRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the host key that's specified in the HostKeyId
parameter.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteHostKeyRequest.Builder} avoiding the need to
* create one manually via {@link DeleteHostKeyRequest#builder()}
*
*
* @param deleteHostKeyRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transfer.model.DeleteHostKeyRequest.Builder} to create a request.
* @return A Java Future containing the result of the DeleteHostKey operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.DeleteHostKey
* @see AWS API
* Documentation
*/
default CompletableFuture deleteHostKey(Consumer deleteHostKeyRequest) {
return deleteHostKey(DeleteHostKeyRequest.builder().applyMutation(deleteHostKeyRequest).build());
}
/**
*
* Deletes the profile that's specified in the ProfileId
parameter.
*
*
* @param deleteProfileRequest
* @return A Java Future containing the result of the DeleteProfile operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.DeleteProfile
* @see AWS API
* Documentation
*/
default CompletableFuture deleteProfile(DeleteProfileRequest deleteProfileRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the profile that's specified in the ProfileId
parameter.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteProfileRequest.Builder} avoiding the need to
* create one manually via {@link DeleteProfileRequest#builder()}
*
*
* @param deleteProfileRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transfer.model.DeleteProfileRequest.Builder} to create a request.
* @return A Java Future containing the result of the DeleteProfile operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.DeleteProfile
* @see AWS API
* Documentation
*/
default CompletableFuture deleteProfile(Consumer deleteProfileRequest) {
return deleteProfile(DeleteProfileRequest.builder().applyMutation(deleteProfileRequest).build());
}
/**
*
* Deletes the file transfer protocol-enabled server that you specify.
*
*
* No response returns from this operation.
*
*
* @param deleteServerRequest
* @return A Java Future containing the result of the DeleteServer operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.DeleteServer
* @see AWS API
* Documentation
*/
default CompletableFuture deleteServer(DeleteServerRequest deleteServerRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the file transfer protocol-enabled server that you specify.
*
*
* No response returns from this operation.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteServerRequest.Builder} avoiding the need to
* create one manually via {@link DeleteServerRequest#builder()}
*
*
* @param deleteServerRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transfer.model.DeleteServerRequest.Builder} to create a request.
* @return A Java Future containing the result of the DeleteServer operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.DeleteServer
* @see AWS API
* Documentation
*/
default CompletableFuture deleteServer(Consumer deleteServerRequest) {
return deleteServer(DeleteServerRequest.builder().applyMutation(deleteServerRequest).build());
}
/**
*
* Deletes a user's Secure Shell (SSH) public key.
*
*
* @param deleteSshPublicKeyRequest
* @return A Java Future containing the result of the DeleteSshPublicKey operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.DeleteSshPublicKey
* @see AWS
* API Documentation
*/
default CompletableFuture deleteSshPublicKey(DeleteSshPublicKeyRequest deleteSshPublicKeyRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a user's Secure Shell (SSH) public key.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteSshPublicKeyRequest.Builder} avoiding the
* need to create one manually via {@link DeleteSshPublicKeyRequest#builder()}
*
*
* @param deleteSshPublicKeyRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transfer.model.DeleteSshPublicKeyRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DeleteSshPublicKey operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.DeleteSshPublicKey
* @see AWS
* API Documentation
*/
default CompletableFuture deleteSshPublicKey(
Consumer deleteSshPublicKeyRequest) {
return deleteSshPublicKey(DeleteSshPublicKeyRequest.builder().applyMutation(deleteSshPublicKeyRequest).build());
}
/**
*
* Deletes the user belonging to a file transfer protocol-enabled server you specify.
*
*
* No response returns from this operation.
*
*
*
* When you delete a user from a server, the user's information is lost.
*
*
*
* @param deleteUserRequest
* @return A Java Future containing the result of the DeleteUser operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.DeleteUser
* @see AWS API
* Documentation
*/
default CompletableFuture deleteUser(DeleteUserRequest deleteUserRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the user belonging to a file transfer protocol-enabled server you specify.
*
*
* No response returns from this operation.
*
*
*
* When you delete a user from a server, the user's information is lost.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteUserRequest.Builder} avoiding the need to
* create one manually via {@link DeleteUserRequest#builder()}
*
*
* @param deleteUserRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transfer.model.DeleteUserRequest.Builder} to create a request.
* @return A Java Future containing the result of the DeleteUser operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.DeleteUser
* @see AWS API
* Documentation
*/
default CompletableFuture deleteUser(Consumer deleteUserRequest) {
return deleteUser(DeleteUserRequest.builder().applyMutation(deleteUserRequest).build());
}
/**
*
* Deletes the specified workflow.
*
*
* @param deleteWorkflowRequest
* @return A Java Future containing the result of the DeleteWorkflow operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.DeleteWorkflow
* @see AWS API
* Documentation
*/
default CompletableFuture deleteWorkflow(DeleteWorkflowRequest deleteWorkflowRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the specified workflow.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteWorkflowRequest.Builder} avoiding the need to
* create one manually via {@link DeleteWorkflowRequest#builder()}
*
*
* @param deleteWorkflowRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transfer.model.DeleteWorkflowRequest.Builder} to create a request.
* @return A Java Future containing the result of the DeleteWorkflow operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.DeleteWorkflow
* @see AWS API
* Documentation
*/
default CompletableFuture deleteWorkflow(Consumer deleteWorkflowRequest) {
return deleteWorkflow(DeleteWorkflowRequest.builder().applyMutation(deleteWorkflowRequest).build());
}
/**
*
* Describes the access that is assigned to the specific file transfer protocol-enabled server, as identified by its
* ServerId
property and its ExternalId
.
*
*
* The response from this call returns the properties of the access that is associated with the
* ServerId
value that was specified.
*
*
* @param describeAccessRequest
* @return A Java Future containing the result of the DescribeAccess operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.DescribeAccess
* @see AWS API
* Documentation
*/
default CompletableFuture describeAccess(DescribeAccessRequest describeAccessRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Describes the access that is assigned to the specific file transfer protocol-enabled server, as identified by its
* ServerId
property and its ExternalId
.
*
*
* The response from this call returns the properties of the access that is associated with the
* ServerId
value that was specified.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeAccessRequest.Builder} avoiding the need to
* create one manually via {@link DescribeAccessRequest#builder()}
*
*
* @param describeAccessRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transfer.model.DescribeAccessRequest.Builder} to create a request.
* @return A Java Future containing the result of the DescribeAccess operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.DescribeAccess
* @see AWS API
* Documentation
*/
default CompletableFuture describeAccess(Consumer describeAccessRequest) {
return describeAccess(DescribeAccessRequest.builder().applyMutation(describeAccessRequest).build());
}
/**
*
* Describes the agreement that's identified by the AgreementId
.
*
*
* @param describeAgreementRequest
* @return A Java Future containing the result of the DescribeAgreement operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.DescribeAgreement
* @see AWS
* API Documentation
*/
default CompletableFuture describeAgreement(DescribeAgreementRequest describeAgreementRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Describes the agreement that's identified by the AgreementId
.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeAgreementRequest.Builder} avoiding the need
* to create one manually via {@link DescribeAgreementRequest#builder()}
*
*
* @param describeAgreementRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transfer.model.DescribeAgreementRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DescribeAgreement operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.DescribeAgreement
* @see AWS
* API Documentation
*/
default CompletableFuture describeAgreement(
Consumer describeAgreementRequest) {
return describeAgreement(DescribeAgreementRequest.builder().applyMutation(describeAgreementRequest).build());
}
/**
*
* Describes the certificate that's identified by the CertificateId
.
*
*
* @param describeCertificateRequest
* @return A Java Future containing the result of the DescribeCertificate operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.DescribeCertificate
* @see AWS
* API Documentation
*/
default CompletableFuture describeCertificate(
DescribeCertificateRequest describeCertificateRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Describes the certificate that's identified by the CertificateId
.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeCertificateRequest.Builder} avoiding the
* need to create one manually via {@link DescribeCertificateRequest#builder()}
*
*
* @param describeCertificateRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transfer.model.DescribeCertificateRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DescribeCertificate operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.DescribeCertificate
* @see AWS
* API Documentation
*/
default CompletableFuture describeCertificate(
Consumer describeCertificateRequest) {
return describeCertificate(DescribeCertificateRequest.builder().applyMutation(describeCertificateRequest).build());
}
/**
*
* Describes the connector that's identified by the ConnectorId.
*
*
* @param describeConnectorRequest
* @return A Java Future containing the result of the DescribeConnector operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.DescribeConnector
* @see AWS
* API Documentation
*/
default CompletableFuture describeConnector(DescribeConnectorRequest describeConnectorRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Describes the connector that's identified by the ConnectorId.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeConnectorRequest.Builder} avoiding the need
* to create one manually via {@link DescribeConnectorRequest#builder()}
*
*
* @param describeConnectorRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transfer.model.DescribeConnectorRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DescribeConnector operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.DescribeConnector
* @see AWS
* API Documentation
*/
default CompletableFuture describeConnector(
Consumer describeConnectorRequest) {
return describeConnector(DescribeConnectorRequest.builder().applyMutation(describeConnectorRequest).build());
}
/**
*
* You can use DescribeExecution
to check the details of the execution of the specified workflow.
*
*
*
* This API call only returns details for in-progress workflows.
*
*
* If you provide an ID for an execution that is not in progress, or if the execution doesn't match the specified
* workflow ID, you receive a ResourceNotFound
exception.
*
*
*
* @param describeExecutionRequest
* @return A Java Future containing the result of the DescribeExecution operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.DescribeExecution
* @see AWS
* API Documentation
*/
default CompletableFuture describeExecution(DescribeExecutionRequest describeExecutionRequest) {
throw new UnsupportedOperationException();
}
/**
*
* You can use DescribeExecution
to check the details of the execution of the specified workflow.
*
*
*
* This API call only returns details for in-progress workflows.
*
*
* If you provide an ID for an execution that is not in progress, or if the execution doesn't match the specified
* workflow ID, you receive a ResourceNotFound
exception.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeExecutionRequest.Builder} avoiding the need
* to create one manually via {@link DescribeExecutionRequest#builder()}
*
*
* @param describeExecutionRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transfer.model.DescribeExecutionRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DescribeExecution operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.DescribeExecution
* @see AWS
* API Documentation
*/
default CompletableFuture describeExecution(
Consumer describeExecutionRequest) {
return describeExecution(DescribeExecutionRequest.builder().applyMutation(describeExecutionRequest).build());
}
/**
*
* Returns the details of the host key that's specified by the HostKeyId
and ServerId
.
*
*
* @param describeHostKeyRequest
* @return A Java Future containing the result of the DescribeHostKey operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.DescribeHostKey
* @see AWS API
* Documentation
*/
default CompletableFuture describeHostKey(DescribeHostKeyRequest describeHostKeyRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns the details of the host key that's specified by the HostKeyId
and ServerId
.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeHostKeyRequest.Builder} avoiding the need
* to create one manually via {@link DescribeHostKeyRequest#builder()}
*
*
* @param describeHostKeyRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transfer.model.DescribeHostKeyRequest.Builder} to create a request.
* @return A Java Future containing the result of the DescribeHostKey operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.DescribeHostKey
* @see AWS API
* Documentation
*/
default CompletableFuture describeHostKey(
Consumer describeHostKeyRequest) {
return describeHostKey(DescribeHostKeyRequest.builder().applyMutation(describeHostKeyRequest).build());
}
/**
*
* Returns the details of the profile that's specified by the ProfileId
.
*
*
* @param describeProfileRequest
* @return A Java Future containing the result of the DescribeProfile operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.DescribeProfile
* @see AWS API
* Documentation
*/
default CompletableFuture describeProfile(DescribeProfileRequest describeProfileRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns the details of the profile that's specified by the ProfileId
.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeProfileRequest.Builder} avoiding the need
* to create one manually via {@link DescribeProfileRequest#builder()}
*
*
* @param describeProfileRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transfer.model.DescribeProfileRequest.Builder} to create a request.
* @return A Java Future containing the result of the DescribeProfile operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.DescribeProfile
* @see AWS API
* Documentation
*/
default CompletableFuture describeProfile(
Consumer describeProfileRequest) {
return describeProfile(DescribeProfileRequest.builder().applyMutation(describeProfileRequest).build());
}
/**
*
* Describes the security policy that is attached to your server or SFTP connector. The response contains a
* description of the security policy's properties. For more information about security policies, see Working with security
* policies for servers or Working with
* security policies for SFTP connectors.
*
*
* @param describeSecurityPolicyRequest
* @return A Java Future containing the result of the DescribeSecurityPolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.DescribeSecurityPolicy
* @see AWS API Documentation
*/
default CompletableFuture describeSecurityPolicy(
DescribeSecurityPolicyRequest describeSecurityPolicyRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Describes the security policy that is attached to your server or SFTP connector. The response contains a
* description of the security policy's properties. For more information about security policies, see Working with security
* policies for servers or Working with
* security policies for SFTP connectors.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeSecurityPolicyRequest.Builder} avoiding the
* need to create one manually via {@link DescribeSecurityPolicyRequest#builder()}
*
*
* @param describeSecurityPolicyRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transfer.model.DescribeSecurityPolicyRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DescribeSecurityPolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.DescribeSecurityPolicy
* @see AWS API Documentation
*/
default CompletableFuture describeSecurityPolicy(
Consumer describeSecurityPolicyRequest) {
return describeSecurityPolicy(DescribeSecurityPolicyRequest.builder().applyMutation(describeSecurityPolicyRequest)
.build());
}
/**
*
* Describes a file transfer protocol-enabled server that you specify by passing the ServerId
* parameter.
*
*
* The response contains a description of a server's properties. When you set EndpointType
to VPC, the
* response will contain the EndpointDetails
.
*
*
* @param describeServerRequest
* @return A Java Future containing the result of the DescribeServer operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.DescribeServer
* @see AWS API
* Documentation
*/
default CompletableFuture describeServer(DescribeServerRequest describeServerRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Describes a file transfer protocol-enabled server that you specify by passing the ServerId
* parameter.
*
*
* The response contains a description of a server's properties. When you set EndpointType
to VPC, the
* response will contain the EndpointDetails
.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeServerRequest.Builder} avoiding the need to
* create one manually via {@link DescribeServerRequest#builder()}
*
*
* @param describeServerRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transfer.model.DescribeServerRequest.Builder} to create a request.
* @return A Java Future containing the result of the DescribeServer operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.DescribeServer
* @see AWS API
* Documentation
*/
default CompletableFuture describeServer(Consumer describeServerRequest) {
return describeServer(DescribeServerRequest.builder().applyMutation(describeServerRequest).build());
}
/**
*
* Describes the user assigned to the specific file transfer protocol-enabled server, as identified by its
* ServerId
property.
*
*
* The response from this call returns the properties of the user associated with the ServerId
value
* that was specified.
*
*
* @param describeUserRequest
* @return A Java Future containing the result of the DescribeUser operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.DescribeUser
* @see AWS API
* Documentation
*/
default CompletableFuture describeUser(DescribeUserRequest describeUserRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Describes the user assigned to the specific file transfer protocol-enabled server, as identified by its
* ServerId
property.
*
*
* The response from this call returns the properties of the user associated with the ServerId
value
* that was specified.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeUserRequest.Builder} avoiding the need to
* create one manually via {@link DescribeUserRequest#builder()}
*
*
* @param describeUserRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transfer.model.DescribeUserRequest.Builder} to create a request.
* @return A Java Future containing the result of the DescribeUser operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.DescribeUser
* @see AWS API
* Documentation
*/
default CompletableFuture describeUser(Consumer describeUserRequest) {
return describeUser(DescribeUserRequest.builder().applyMutation(describeUserRequest).build());
}
/**
*
* Describes the specified workflow.
*
*
* @param describeWorkflowRequest
* @return A Java Future containing the result of the DescribeWorkflow operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.DescribeWorkflow
* @see AWS API
* Documentation
*/
default CompletableFuture describeWorkflow(DescribeWorkflowRequest describeWorkflowRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Describes the specified workflow.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeWorkflowRequest.Builder} avoiding the need
* to create one manually via {@link DescribeWorkflowRequest#builder()}
*
*
* @param describeWorkflowRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transfer.model.DescribeWorkflowRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DescribeWorkflow operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.DescribeWorkflow
* @see AWS API
* Documentation
*/
default CompletableFuture describeWorkflow(
Consumer describeWorkflowRequest) {
return describeWorkflow(DescribeWorkflowRequest.builder().applyMutation(describeWorkflowRequest).build());
}
/**
*
* Imports the signing and encryption certificates that you need to create local (AS2) profiles and partner
* profiles.
*
*
* @param importCertificateRequest
* @return A Java Future containing the result of the ImportCertificate operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ImportCertificate
* @see AWS
* API Documentation
*/
default CompletableFuture importCertificate(ImportCertificateRequest importCertificateRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Imports the signing and encryption certificates that you need to create local (AS2) profiles and partner
* profiles.
*
*
*
* This is a convenience which creates an instance of the {@link ImportCertificateRequest.Builder} avoiding the need
* to create one manually via {@link ImportCertificateRequest#builder()}
*
*
* @param importCertificateRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transfer.model.ImportCertificateRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ImportCertificate operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ImportCertificate
* @see AWS
* API Documentation
*/
default CompletableFuture importCertificate(
Consumer importCertificateRequest) {
return importCertificate(ImportCertificateRequest.builder().applyMutation(importCertificateRequest).build());
}
/**
*
* Adds a host key to the server that's specified by the ServerId
parameter.
*
*
* @param importHostKeyRequest
* @return A Java Future containing the result of the ImportHostKey operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - ResourceExistsException The requested resource does not exist, or exists in a region other than the
* one specified for the command.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ImportHostKey
* @see AWS API
* Documentation
*/
default CompletableFuture importHostKey(ImportHostKeyRequest importHostKeyRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Adds a host key to the server that's specified by the ServerId
parameter.
*
*
*
* This is a convenience which creates an instance of the {@link ImportHostKeyRequest.Builder} avoiding the need to
* create one manually via {@link ImportHostKeyRequest#builder()}
*
*
* @param importHostKeyRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transfer.model.ImportHostKeyRequest.Builder} to create a request.
* @return A Java Future containing the result of the ImportHostKey operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - ResourceExistsException The requested resource does not exist, or exists in a region other than the
* one specified for the command.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ImportHostKey
* @see AWS API
* Documentation
*/
default CompletableFuture importHostKey(Consumer importHostKeyRequest) {
return importHostKey(ImportHostKeyRequest.builder().applyMutation(importHostKeyRequest).build());
}
/**
*
* Adds a Secure Shell (SSH) public key to a Transfer Family user identified by a UserName
value
* assigned to the specific file transfer protocol-enabled server, identified by ServerId
.
*
*
* The response returns the UserName
value, the ServerId
value, and the name of the
* SshPublicKeyId
.
*
*
* @param importSshPublicKeyRequest
* @return A Java Future containing the result of the ImportSshPublicKey operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - ResourceExistsException The requested resource does not exist, or exists in a region other than the
* one specified for the command.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ImportSshPublicKey
* @see AWS
* API Documentation
*/
default CompletableFuture importSshPublicKey(ImportSshPublicKeyRequest importSshPublicKeyRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Adds a Secure Shell (SSH) public key to a Transfer Family user identified by a UserName
value
* assigned to the specific file transfer protocol-enabled server, identified by ServerId
.
*
*
* The response returns the UserName
value, the ServerId
value, and the name of the
* SshPublicKeyId
.
*
*
*
* This is a convenience which creates an instance of the {@link ImportSshPublicKeyRequest.Builder} avoiding the
* need to create one manually via {@link ImportSshPublicKeyRequest#builder()}
*
*
* @param importSshPublicKeyRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transfer.model.ImportSshPublicKeyRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ImportSshPublicKey operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - ResourceExistsException The requested resource does not exist, or exists in a region other than the
* one specified for the command.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ImportSshPublicKey
* @see AWS
* API Documentation
*/
default CompletableFuture importSshPublicKey(
Consumer importSshPublicKeyRequest) {
return importSshPublicKey(ImportSshPublicKeyRequest.builder().applyMutation(importSshPublicKeyRequest).build());
}
/**
*
* Lists the details for all the accesses you have on your server.
*
*
* @param listAccessesRequest
* @return A Java Future containing the result of the ListAccesses operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - InvalidNextTokenException The
NextToken
parameter that was passed is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ListAccesses
* @see AWS API
* Documentation
*/
default CompletableFuture listAccesses(ListAccessesRequest listAccessesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists the details for all the accesses you have on your server.
*
*
*
* This is a convenience which creates an instance of the {@link ListAccessesRequest.Builder} avoiding the need to
* create one manually via {@link ListAccessesRequest#builder()}
*
*
* @param listAccessesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transfer.model.ListAccessesRequest.Builder} to create a request.
* @return A Java Future containing the result of the ListAccesses operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - InvalidNextTokenException The
NextToken
parameter that was passed is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ListAccesses
* @see AWS API
* Documentation
*/
default CompletableFuture listAccesses(Consumer listAccessesRequest) {
return listAccesses(ListAccessesRequest.builder().applyMutation(listAccessesRequest).build());
}
/**
*
* This is a variant of {@link #listAccesses(software.amazon.awssdk.services.transfer.model.ListAccessesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.transfer.paginators.ListAccessesPublisher publisher = client.listAccessesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.transfer.paginators.ListAccessesPublisher publisher = client.listAccessesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.transfer.model.ListAccessesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listAccesses(software.amazon.awssdk.services.transfer.model.ListAccessesRequest)} operation.
*
*
* @param listAccessesRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - InvalidNextTokenException The
NextToken
parameter that was passed is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ListAccesses
* @see AWS API
* Documentation
*/
default ListAccessesPublisher listAccessesPaginator(ListAccessesRequest listAccessesRequest) {
return new ListAccessesPublisher(this, listAccessesRequest);
}
/**
*
* This is a variant of {@link #listAccesses(software.amazon.awssdk.services.transfer.model.ListAccessesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.transfer.paginators.ListAccessesPublisher publisher = client.listAccessesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.transfer.paginators.ListAccessesPublisher publisher = client.listAccessesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.transfer.model.ListAccessesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listAccesses(software.amazon.awssdk.services.transfer.model.ListAccessesRequest)} operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListAccessesRequest.Builder} avoiding the need to
* create one manually via {@link ListAccessesRequest#builder()}
*
*
* @param listAccessesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transfer.model.ListAccessesRequest.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - InvalidNextTokenException The
NextToken
parameter that was passed is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ListAccesses
* @see AWS API
* Documentation
*/
default ListAccessesPublisher listAccessesPaginator(Consumer listAccessesRequest) {
return listAccessesPaginator(ListAccessesRequest.builder().applyMutation(listAccessesRequest).build());
}
/**
*
* Returns a list of the agreements for the server that's identified by the ServerId
that you supply.
* If you want to limit the results to a certain number, supply a value for the MaxResults
parameter.
* If you ran the command previously and received a value for NextToken
, you can supply that value to
* continue listing agreements from where you left off.
*
*
* @param listAgreementsRequest
* @return A Java Future containing the result of the ListAgreements operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - InvalidNextTokenException The
NextToken
parameter that was passed is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ListAgreements
* @see AWS API
* Documentation
*/
default CompletableFuture listAgreements(ListAgreementsRequest listAgreementsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of the agreements for the server that's identified by the ServerId
that you supply.
* If you want to limit the results to a certain number, supply a value for the MaxResults
parameter.
* If you ran the command previously and received a value for NextToken
, you can supply that value to
* continue listing agreements from where you left off.
*
*
*
* This is a convenience which creates an instance of the {@link ListAgreementsRequest.Builder} avoiding the need to
* create one manually via {@link ListAgreementsRequest#builder()}
*
*
* @param listAgreementsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transfer.model.ListAgreementsRequest.Builder} to create a request.
* @return A Java Future containing the result of the ListAgreements operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - InvalidNextTokenException The
NextToken
parameter that was passed is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ListAgreements
* @see AWS API
* Documentation
*/
default CompletableFuture listAgreements(Consumer listAgreementsRequest) {
return listAgreements(ListAgreementsRequest.builder().applyMutation(listAgreementsRequest).build());
}
/**
*
* This is a variant of
* {@link #listAgreements(software.amazon.awssdk.services.transfer.model.ListAgreementsRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.transfer.paginators.ListAgreementsPublisher publisher = client.listAgreementsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.transfer.paginators.ListAgreementsPublisher publisher = client.listAgreementsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.transfer.model.ListAgreementsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listAgreements(software.amazon.awssdk.services.transfer.model.ListAgreementsRequest)} operation.
*
*
* @param listAgreementsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - InvalidNextTokenException The
NextToken
parameter that was passed is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ListAgreements
* @see AWS API
* Documentation
*/
default ListAgreementsPublisher listAgreementsPaginator(ListAgreementsRequest listAgreementsRequest) {
return new ListAgreementsPublisher(this, listAgreementsRequest);
}
/**
*
* This is a variant of
* {@link #listAgreements(software.amazon.awssdk.services.transfer.model.ListAgreementsRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.transfer.paginators.ListAgreementsPublisher publisher = client.listAgreementsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.transfer.paginators.ListAgreementsPublisher publisher = client.listAgreementsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.transfer.model.ListAgreementsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listAgreements(software.amazon.awssdk.services.transfer.model.ListAgreementsRequest)} operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListAgreementsRequest.Builder} avoiding the need to
* create one manually via {@link ListAgreementsRequest#builder()}
*
*
* @param listAgreementsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transfer.model.ListAgreementsRequest.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - InvalidNextTokenException The
NextToken
parameter that was passed is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ListAgreements
* @see AWS API
* Documentation
*/
default ListAgreementsPublisher listAgreementsPaginator(Consumer listAgreementsRequest) {
return listAgreementsPaginator(ListAgreementsRequest.builder().applyMutation(listAgreementsRequest).build());
}
/**
*
* Returns a list of the current certificates that have been imported into Transfer Family. If you want to limit the
* results to a certain number, supply a value for the MaxResults
parameter. If you ran the command
* previously and received a value for the NextToken
parameter, you can supply that value to continue
* listing certificates from where you left off.
*
*
* @param listCertificatesRequest
* @return A Java Future containing the result of the ListCertificates operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - InvalidNextTokenException The
NextToken
parameter that was passed is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ListCertificates
* @see AWS API
* Documentation
*/
default CompletableFuture listCertificates(ListCertificatesRequest listCertificatesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of the current certificates that have been imported into Transfer Family. If you want to limit the
* results to a certain number, supply a value for the MaxResults
parameter. If you ran the command
* previously and received a value for the NextToken
parameter, you can supply that value to continue
* listing certificates from where you left off.
*
*
*
* This is a convenience which creates an instance of the {@link ListCertificatesRequest.Builder} avoiding the need
* to create one manually via {@link ListCertificatesRequest#builder()}
*
*
* @param listCertificatesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transfer.model.ListCertificatesRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListCertificates operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - InvalidNextTokenException The
NextToken
parameter that was passed is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ListCertificates
* @see AWS API
* Documentation
*/
default CompletableFuture listCertificates(
Consumer listCertificatesRequest) {
return listCertificates(ListCertificatesRequest.builder().applyMutation(listCertificatesRequest).build());
}
/**
*
* This is a variant of
* {@link #listCertificates(software.amazon.awssdk.services.transfer.model.ListCertificatesRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.transfer.paginators.ListCertificatesPublisher publisher = client.listCertificatesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.transfer.paginators.ListCertificatesPublisher publisher = client.listCertificatesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.transfer.model.ListCertificatesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listCertificates(software.amazon.awssdk.services.transfer.model.ListCertificatesRequest)} operation.
*
*
* @param listCertificatesRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - InvalidNextTokenException The
NextToken
parameter that was passed is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ListCertificates
* @see AWS API
* Documentation
*/
default ListCertificatesPublisher listCertificatesPaginator(ListCertificatesRequest listCertificatesRequest) {
return new ListCertificatesPublisher(this, listCertificatesRequest);
}
/**
*
* This is a variant of
* {@link #listCertificates(software.amazon.awssdk.services.transfer.model.ListCertificatesRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.transfer.paginators.ListCertificatesPublisher publisher = client.listCertificatesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.transfer.paginators.ListCertificatesPublisher publisher = client.listCertificatesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.transfer.model.ListCertificatesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listCertificates(software.amazon.awssdk.services.transfer.model.ListCertificatesRequest)} operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListCertificatesRequest.Builder} avoiding the need
* to create one manually via {@link ListCertificatesRequest#builder()}
*
*
* @param listCertificatesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transfer.model.ListCertificatesRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - InvalidNextTokenException The
NextToken
parameter that was passed is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ListCertificates
* @see AWS API
* Documentation
*/
default ListCertificatesPublisher listCertificatesPaginator(Consumer listCertificatesRequest) {
return listCertificatesPaginator(ListCertificatesRequest.builder().applyMutation(listCertificatesRequest).build());
}
/**
*
* Lists the connectors for the specified Region.
*
*
* @param listConnectorsRequest
* @return A Java Future containing the result of the ListConnectors operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - InvalidNextTokenException The
NextToken
parameter that was passed is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ListConnectors
* @see AWS API
* Documentation
*/
default CompletableFuture listConnectors(ListConnectorsRequest listConnectorsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists the connectors for the specified Region.
*
*
*
* This is a convenience which creates an instance of the {@link ListConnectorsRequest.Builder} avoiding the need to
* create one manually via {@link ListConnectorsRequest#builder()}
*
*
* @param listConnectorsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transfer.model.ListConnectorsRequest.Builder} to create a request.
* @return A Java Future containing the result of the ListConnectors operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - InvalidNextTokenException The
NextToken
parameter that was passed is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ListConnectors
* @see AWS API
* Documentation
*/
default CompletableFuture listConnectors(Consumer listConnectorsRequest) {
return listConnectors(ListConnectorsRequest.builder().applyMutation(listConnectorsRequest).build());
}
/**
*
* This is a variant of
* {@link #listConnectors(software.amazon.awssdk.services.transfer.model.ListConnectorsRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.transfer.paginators.ListConnectorsPublisher publisher = client.listConnectorsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.transfer.paginators.ListConnectorsPublisher publisher = client.listConnectorsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.transfer.model.ListConnectorsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listConnectors(software.amazon.awssdk.services.transfer.model.ListConnectorsRequest)} operation.
*
*
* @param listConnectorsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - InvalidNextTokenException The
NextToken
parameter that was passed is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ListConnectors
* @see AWS API
* Documentation
*/
default ListConnectorsPublisher listConnectorsPaginator(ListConnectorsRequest listConnectorsRequest) {
return new ListConnectorsPublisher(this, listConnectorsRequest);
}
/**
*
* This is a variant of
* {@link #listConnectors(software.amazon.awssdk.services.transfer.model.ListConnectorsRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.transfer.paginators.ListConnectorsPublisher publisher = client.listConnectorsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.transfer.paginators.ListConnectorsPublisher publisher = client.listConnectorsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.transfer.model.ListConnectorsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listConnectors(software.amazon.awssdk.services.transfer.model.ListConnectorsRequest)} operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListConnectorsRequest.Builder} avoiding the need to
* create one manually via {@link ListConnectorsRequest#builder()}
*
*
* @param listConnectorsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transfer.model.ListConnectorsRequest.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - InvalidNextTokenException The
NextToken
parameter that was passed is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ListConnectors
* @see AWS API
* Documentation
*/
default ListConnectorsPublisher listConnectorsPaginator(Consumer listConnectorsRequest) {
return listConnectorsPaginator(ListConnectorsRequest.builder().applyMutation(listConnectorsRequest).build());
}
/**
*
* Lists all in-progress executions for the specified workflow.
*
*
*
* If the specified workflow ID cannot be found, ListExecutions
returns a ResourceNotFound
* exception.
*
*
*
* @param listExecutionsRequest
* @return A Java Future containing the result of the ListExecutions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - InvalidNextTokenException The
NextToken
parameter that was passed is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ListExecutions
* @see AWS API
* Documentation
*/
default CompletableFuture listExecutions(ListExecutionsRequest listExecutionsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists all in-progress executions for the specified workflow.
*
*
*
* If the specified workflow ID cannot be found, ListExecutions
returns a ResourceNotFound
* exception.
*
*
*
* This is a convenience which creates an instance of the {@link ListExecutionsRequest.Builder} avoiding the need to
* create one manually via {@link ListExecutionsRequest#builder()}
*
*
* @param listExecutionsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transfer.model.ListExecutionsRequest.Builder} to create a request.
* @return A Java Future containing the result of the ListExecutions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - InvalidNextTokenException The
NextToken
parameter that was passed is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ListExecutions
* @see AWS API
* Documentation
*/
default CompletableFuture listExecutions(Consumer listExecutionsRequest) {
return listExecutions(ListExecutionsRequest.builder().applyMutation(listExecutionsRequest).build());
}
/**
*
* This is a variant of
* {@link #listExecutions(software.amazon.awssdk.services.transfer.model.ListExecutionsRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.transfer.paginators.ListExecutionsPublisher publisher = client.listExecutionsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.transfer.paginators.ListExecutionsPublisher publisher = client.listExecutionsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.transfer.model.ListExecutionsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listExecutions(software.amazon.awssdk.services.transfer.model.ListExecutionsRequest)} operation.
*
*
* @param listExecutionsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - InvalidNextTokenException The
NextToken
parameter that was passed is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ListExecutions
* @see AWS API
* Documentation
*/
default ListExecutionsPublisher listExecutionsPaginator(ListExecutionsRequest listExecutionsRequest) {
return new ListExecutionsPublisher(this, listExecutionsRequest);
}
/**
*
* This is a variant of
* {@link #listExecutions(software.amazon.awssdk.services.transfer.model.ListExecutionsRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.transfer.paginators.ListExecutionsPublisher publisher = client.listExecutionsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.transfer.paginators.ListExecutionsPublisher publisher = client.listExecutionsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.transfer.model.ListExecutionsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listExecutions(software.amazon.awssdk.services.transfer.model.ListExecutionsRequest)} operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListExecutionsRequest.Builder} avoiding the need to
* create one manually via {@link ListExecutionsRequest#builder()}
*
*
* @param listExecutionsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transfer.model.ListExecutionsRequest.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - InvalidNextTokenException The
NextToken
parameter that was passed is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ListExecutions
* @see AWS API
* Documentation
*/
default ListExecutionsPublisher listExecutionsPaginator(Consumer listExecutionsRequest) {
return listExecutionsPaginator(ListExecutionsRequest.builder().applyMutation(listExecutionsRequest).build());
}
/**
*
* Returns a list of host keys for the server that's specified by the ServerId
parameter.
*
*
* @param listHostKeysRequest
* @return A Java Future containing the result of the ListHostKeys operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - InvalidNextTokenException The
NextToken
parameter that was passed is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ListHostKeys
* @see AWS API
* Documentation
*/
default CompletableFuture listHostKeys(ListHostKeysRequest listHostKeysRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of host keys for the server that's specified by the ServerId
parameter.
*
*
*
* This is a convenience which creates an instance of the {@link ListHostKeysRequest.Builder} avoiding the need to
* create one manually via {@link ListHostKeysRequest#builder()}
*
*
* @param listHostKeysRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transfer.model.ListHostKeysRequest.Builder} to create a request.
* @return A Java Future containing the result of the ListHostKeys operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - InvalidNextTokenException The
NextToken
parameter that was passed is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ListHostKeys
* @see AWS API
* Documentation
*/
default CompletableFuture listHostKeys(Consumer listHostKeysRequest) {
return listHostKeys(ListHostKeysRequest.builder().applyMutation(listHostKeysRequest).build());
}
/**
*
* Returns a list of the profiles for your system. If you want to limit the results to a certain number, supply a
* value for the MaxResults
parameter. If you ran the command previously and received a value for
* NextToken
, you can supply that value to continue listing profiles from where you left off.
*
*
* @param listProfilesRequest
* @return A Java Future containing the result of the ListProfiles operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - InvalidNextTokenException The
NextToken
parameter that was passed is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ListProfiles
* @see AWS API
* Documentation
*/
default CompletableFuture listProfiles(ListProfilesRequest listProfilesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of the profiles for your system. If you want to limit the results to a certain number, supply a
* value for the MaxResults
parameter. If you ran the command previously and received a value for
* NextToken
, you can supply that value to continue listing profiles from where you left off.
*
*
*
* This is a convenience which creates an instance of the {@link ListProfilesRequest.Builder} avoiding the need to
* create one manually via {@link ListProfilesRequest#builder()}
*
*
* @param listProfilesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transfer.model.ListProfilesRequest.Builder} to create a request.
* @return A Java Future containing the result of the ListProfiles operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - InvalidNextTokenException The
NextToken
parameter that was passed is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ListProfiles
* @see AWS API
* Documentation
*/
default CompletableFuture listProfiles(Consumer listProfilesRequest) {
return listProfiles(ListProfilesRequest.builder().applyMutation(listProfilesRequest).build());
}
/**
*
* This is a variant of {@link #listProfiles(software.amazon.awssdk.services.transfer.model.ListProfilesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.transfer.paginators.ListProfilesPublisher publisher = client.listProfilesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.transfer.paginators.ListProfilesPublisher publisher = client.listProfilesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.transfer.model.ListProfilesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listProfiles(software.amazon.awssdk.services.transfer.model.ListProfilesRequest)} operation.
*
*
* @param listProfilesRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - InvalidNextTokenException The
NextToken
parameter that was passed is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ListProfiles
* @see AWS API
* Documentation
*/
default ListProfilesPublisher listProfilesPaginator(ListProfilesRequest listProfilesRequest) {
return new ListProfilesPublisher(this, listProfilesRequest);
}
/**
*
* This is a variant of {@link #listProfiles(software.amazon.awssdk.services.transfer.model.ListProfilesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.transfer.paginators.ListProfilesPublisher publisher = client.listProfilesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.transfer.paginators.ListProfilesPublisher publisher = client.listProfilesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.transfer.model.ListProfilesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listProfiles(software.amazon.awssdk.services.transfer.model.ListProfilesRequest)} operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListProfilesRequest.Builder} avoiding the need to
* create one manually via {@link ListProfilesRequest#builder()}
*
*
* @param listProfilesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transfer.model.ListProfilesRequest.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - InvalidNextTokenException The
NextToken
parameter that was passed is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ListProfiles
* @see AWS API
* Documentation
*/
default ListProfilesPublisher listProfilesPaginator(Consumer listProfilesRequest) {
return listProfilesPaginator(ListProfilesRequest.builder().applyMutation(listProfilesRequest).build());
}
/**
*
* Lists the security policies that are attached to your servers and SFTP connectors. For more information about
* security policies, see Working with security
* policies for servers or Working with
* security policies for SFTP connectors.
*
*
* @param listSecurityPoliciesRequest
* @return A Java Future containing the result of the ListSecurityPolicies operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - InvalidNextTokenException The
NextToken
parameter that was passed is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ListSecurityPolicies
* @see AWS
* API Documentation
*/
default CompletableFuture listSecurityPolicies(
ListSecurityPoliciesRequest listSecurityPoliciesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists the security policies that are attached to your servers and SFTP connectors. For more information about
* security policies, see Working with security
* policies for servers or Working with
* security policies for SFTP connectors.
*
*
*
* This is a convenience which creates an instance of the {@link ListSecurityPoliciesRequest.Builder} avoiding the
* need to create one manually via {@link ListSecurityPoliciesRequest#builder()}
*
*
* @param listSecurityPoliciesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transfer.model.ListSecurityPoliciesRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListSecurityPolicies operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - InvalidNextTokenException The
NextToken
parameter that was passed is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ListSecurityPolicies
* @see AWS
* API Documentation
*/
default CompletableFuture listSecurityPolicies(
Consumer listSecurityPoliciesRequest) {
return listSecurityPolicies(ListSecurityPoliciesRequest.builder().applyMutation(listSecurityPoliciesRequest).build());
}
/**
*
* This is a variant of
* {@link #listSecurityPolicies(software.amazon.awssdk.services.transfer.model.ListSecurityPoliciesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.transfer.paginators.ListSecurityPoliciesPublisher publisher = client.listSecurityPoliciesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.transfer.paginators.ListSecurityPoliciesPublisher publisher = client.listSecurityPoliciesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.transfer.model.ListSecurityPoliciesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listSecurityPolicies(software.amazon.awssdk.services.transfer.model.ListSecurityPoliciesRequest)}
* operation.
*
*
* @param listSecurityPoliciesRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - InvalidNextTokenException The
NextToken
parameter that was passed is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ListSecurityPolicies
* @see AWS
* API Documentation
*/
default ListSecurityPoliciesPublisher listSecurityPoliciesPaginator(ListSecurityPoliciesRequest listSecurityPoliciesRequest) {
return new ListSecurityPoliciesPublisher(this, listSecurityPoliciesRequest);
}
/**
*
* This is a variant of
* {@link #listSecurityPolicies(software.amazon.awssdk.services.transfer.model.ListSecurityPoliciesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.transfer.paginators.ListSecurityPoliciesPublisher publisher = client.listSecurityPoliciesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.transfer.paginators.ListSecurityPoliciesPublisher publisher = client.listSecurityPoliciesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.transfer.model.ListSecurityPoliciesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listSecurityPolicies(software.amazon.awssdk.services.transfer.model.ListSecurityPoliciesRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListSecurityPoliciesRequest.Builder} avoiding the
* need to create one manually via {@link ListSecurityPoliciesRequest#builder()}
*
*
* @param listSecurityPoliciesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transfer.model.ListSecurityPoliciesRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - InvalidNextTokenException The
NextToken
parameter that was passed is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ListSecurityPolicies
* @see AWS
* API Documentation
*/
default ListSecurityPoliciesPublisher listSecurityPoliciesPaginator(
Consumer listSecurityPoliciesRequest) {
return listSecurityPoliciesPaginator(ListSecurityPoliciesRequest.builder().applyMutation(listSecurityPoliciesRequest)
.build());
}
/**
*
* Lists the file transfer protocol-enabled servers that are associated with your Amazon Web Services account.
*
*
* @param listServersRequest
* @return A Java Future containing the result of the ListServers operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - InvalidNextTokenException The
NextToken
parameter that was passed is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ListServers
* @see AWS API
* Documentation
*/
default CompletableFuture listServers(ListServersRequest listServersRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists the file transfer protocol-enabled servers that are associated with your Amazon Web Services account.
*
*
*
* This is a convenience which creates an instance of the {@link ListServersRequest.Builder} avoiding the need to
* create one manually via {@link ListServersRequest#builder()}
*
*
* @param listServersRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transfer.model.ListServersRequest.Builder} to create a request.
* @return A Java Future containing the result of the ListServers operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - InvalidNextTokenException The
NextToken
parameter that was passed is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ListServers
* @see AWS API
* Documentation
*/
default CompletableFuture listServers(Consumer listServersRequest) {
return listServers(ListServersRequest.builder().applyMutation(listServersRequest).build());
}
/**
*
* Lists the file transfer protocol-enabled servers that are associated with your Amazon Web Services account.
*
*
* @return A Java Future containing the result of the ListServers operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - InvalidNextTokenException The
NextToken
parameter that was passed is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ListServers
* @see AWS API
* Documentation
*/
default CompletableFuture listServers() {
return listServers(ListServersRequest.builder().build());
}
/**
*
* This is a variant of {@link #listServers(software.amazon.awssdk.services.transfer.model.ListServersRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.transfer.paginators.ListServersPublisher publisher = client.listServersPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.transfer.paginators.ListServersPublisher publisher = client.listServersPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.transfer.model.ListServersResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listServers(software.amazon.awssdk.services.transfer.model.ListServersRequest)} operation.
*
*
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - InvalidNextTokenException The
NextToken
parameter that was passed is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ListServers
* @see AWS API
* Documentation
*/
default ListServersPublisher listServersPaginator() {
return listServersPaginator(ListServersRequest.builder().build());
}
/**
*
* This is a variant of {@link #listServers(software.amazon.awssdk.services.transfer.model.ListServersRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.transfer.paginators.ListServersPublisher publisher = client.listServersPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.transfer.paginators.ListServersPublisher publisher = client.listServersPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.transfer.model.ListServersResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listServers(software.amazon.awssdk.services.transfer.model.ListServersRequest)} operation.
*
*
* @param listServersRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - InvalidNextTokenException The
NextToken
parameter that was passed is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ListServers
* @see AWS API
* Documentation
*/
default ListServersPublisher listServersPaginator(ListServersRequest listServersRequest) {
return new ListServersPublisher(this, listServersRequest);
}
/**
*
* This is a variant of {@link #listServers(software.amazon.awssdk.services.transfer.model.ListServersRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.transfer.paginators.ListServersPublisher publisher = client.listServersPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.transfer.paginators.ListServersPublisher publisher = client.listServersPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.transfer.model.ListServersResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listServers(software.amazon.awssdk.services.transfer.model.ListServersRequest)} operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListServersRequest.Builder} avoiding the need to
* create one manually via {@link ListServersRequest#builder()}
*
*
* @param listServersRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transfer.model.ListServersRequest.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - InvalidNextTokenException The
NextToken
parameter that was passed is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ListServers
* @see AWS API
* Documentation
*/
default ListServersPublisher listServersPaginator(Consumer listServersRequest) {
return listServersPaginator(ListServersRequest.builder().applyMutation(listServersRequest).build());
}
/**
*
* Lists all of the tags associated with the Amazon Resource Name (ARN) that you specify. The resource can be a
* user, server, or role.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - InvalidNextTokenException The
NextToken
parameter that was passed is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ListTagsForResource
* @see AWS
* API Documentation
*/
default CompletableFuture listTagsForResource(
ListTagsForResourceRequest listTagsForResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists all of the tags associated with the Amazon Resource Name (ARN) that you specify. The resource can be a
* user, server, or role.
*
*
*
* This is a convenience which creates an instance of the {@link ListTagsForResourceRequest.Builder} avoiding the
* need to create one manually via {@link ListTagsForResourceRequest#builder()}
*
*
* @param listTagsForResourceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transfer.model.ListTagsForResourceRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - InvalidNextTokenException The
NextToken
parameter that was passed is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ListTagsForResource
* @see AWS
* API Documentation
*/
default CompletableFuture listTagsForResource(
Consumer listTagsForResourceRequest) {
return listTagsForResource(ListTagsForResourceRequest.builder().applyMutation(listTagsForResourceRequest).build());
}
/**
*
* This is a variant of
* {@link #listTagsForResource(software.amazon.awssdk.services.transfer.model.ListTagsForResourceRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.transfer.paginators.ListTagsForResourcePublisher publisher = client.listTagsForResourcePaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.transfer.paginators.ListTagsForResourcePublisher publisher = client.listTagsForResourcePaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.transfer.model.ListTagsForResourceResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listTagsForResource(software.amazon.awssdk.services.transfer.model.ListTagsForResourceRequest)}
* operation.
*
*
* @param listTagsForResourceRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - InvalidNextTokenException The
NextToken
parameter that was passed is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ListTagsForResource
* @see AWS
* API Documentation
*/
default ListTagsForResourcePublisher listTagsForResourcePaginator(ListTagsForResourceRequest listTagsForResourceRequest) {
return new ListTagsForResourcePublisher(this, listTagsForResourceRequest);
}
/**
*
* This is a variant of
* {@link #listTagsForResource(software.amazon.awssdk.services.transfer.model.ListTagsForResourceRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.transfer.paginators.ListTagsForResourcePublisher publisher = client.listTagsForResourcePaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.transfer.paginators.ListTagsForResourcePublisher publisher = client.listTagsForResourcePaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.transfer.model.ListTagsForResourceResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listTagsForResource(software.amazon.awssdk.services.transfer.model.ListTagsForResourceRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListTagsForResourceRequest.Builder} avoiding the
* need to create one manually via {@link ListTagsForResourceRequest#builder()}
*
*
* @param listTagsForResourceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transfer.model.ListTagsForResourceRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - InvalidNextTokenException The
NextToken
parameter that was passed is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ListTagsForResource
* @see AWS
* API Documentation
*/
default ListTagsForResourcePublisher listTagsForResourcePaginator(
Consumer listTagsForResourceRequest) {
return listTagsForResourcePaginator(ListTagsForResourceRequest.builder().applyMutation(listTagsForResourceRequest)
.build());
}
/**
*
* Lists the users for a file transfer protocol-enabled server that you specify by passing the ServerId
* parameter.
*
*
* @param listUsersRequest
* @return A Java Future containing the result of the ListUsers operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - InvalidNextTokenException The
NextToken
parameter that was passed is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ListUsers
* @see AWS API
* Documentation
*/
default CompletableFuture listUsers(ListUsersRequest listUsersRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists the users for a file transfer protocol-enabled server that you specify by passing the ServerId
* parameter.
*
*
*
* This is a convenience which creates an instance of the {@link ListUsersRequest.Builder} avoiding the need to
* create one manually via {@link ListUsersRequest#builder()}
*
*
* @param listUsersRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transfer.model.ListUsersRequest.Builder} to create a request.
* @return A Java Future containing the result of the ListUsers operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - InvalidNextTokenException The
NextToken
parameter that was passed is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ListUsers
* @see AWS API
* Documentation
*/
default CompletableFuture listUsers(Consumer listUsersRequest) {
return listUsers(ListUsersRequest.builder().applyMutation(listUsersRequest).build());
}
/**
*
* This is a variant of {@link #listUsers(software.amazon.awssdk.services.transfer.model.ListUsersRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.transfer.paginators.ListUsersPublisher publisher = client.listUsersPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.transfer.paginators.ListUsersPublisher publisher = client.listUsersPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.transfer.model.ListUsersResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listUsers(software.amazon.awssdk.services.transfer.model.ListUsersRequest)} operation.
*
*
* @param listUsersRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - InvalidNextTokenException The
NextToken
parameter that was passed is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ListUsers
* @see AWS API
* Documentation
*/
default ListUsersPublisher listUsersPaginator(ListUsersRequest listUsersRequest) {
return new ListUsersPublisher(this, listUsersRequest);
}
/**
*
* This is a variant of {@link #listUsers(software.amazon.awssdk.services.transfer.model.ListUsersRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.transfer.paginators.ListUsersPublisher publisher = client.listUsersPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.transfer.paginators.ListUsersPublisher publisher = client.listUsersPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.transfer.model.ListUsersResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listUsers(software.amazon.awssdk.services.transfer.model.ListUsersRequest)} operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListUsersRequest.Builder} avoiding the need to
* create one manually via {@link ListUsersRequest#builder()}
*
*
* @param listUsersRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transfer.model.ListUsersRequest.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - InvalidNextTokenException The
NextToken
parameter that was passed is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ListUsers
* @see AWS API
* Documentation
*/
default ListUsersPublisher listUsersPaginator(Consumer listUsersRequest) {
return listUsersPaginator(ListUsersRequest.builder().applyMutation(listUsersRequest).build());
}
/**
*
* Lists all workflows associated with your Amazon Web Services account for your current region.
*
*
* @param listWorkflowsRequest
* @return A Java Future containing the result of the ListWorkflows operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - InvalidNextTokenException The
NextToken
parameter that was passed is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ListWorkflows
* @see AWS API
* Documentation
*/
default CompletableFuture listWorkflows(ListWorkflowsRequest listWorkflowsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists all workflows associated with your Amazon Web Services account for your current region.
*
*
*
* This is a convenience which creates an instance of the {@link ListWorkflowsRequest.Builder} avoiding the need to
* create one manually via {@link ListWorkflowsRequest#builder()}
*
*
* @param listWorkflowsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transfer.model.ListWorkflowsRequest.Builder} to create a request.
* @return A Java Future containing the result of the ListWorkflows operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - InvalidNextTokenException The
NextToken
parameter that was passed is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ListWorkflows
* @see AWS API
* Documentation
*/
default CompletableFuture listWorkflows(Consumer listWorkflowsRequest) {
return listWorkflows(ListWorkflowsRequest.builder().applyMutation(listWorkflowsRequest).build());
}
/**
*
* This is a variant of {@link #listWorkflows(software.amazon.awssdk.services.transfer.model.ListWorkflowsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.transfer.paginators.ListWorkflowsPublisher publisher = client.listWorkflowsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.transfer.paginators.ListWorkflowsPublisher publisher = client.listWorkflowsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.transfer.model.ListWorkflowsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listWorkflows(software.amazon.awssdk.services.transfer.model.ListWorkflowsRequest)} operation.
*
*
* @param listWorkflowsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - InvalidNextTokenException The
NextToken
parameter that was passed is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ListWorkflows
* @see AWS API
* Documentation
*/
default ListWorkflowsPublisher listWorkflowsPaginator(ListWorkflowsRequest listWorkflowsRequest) {
return new ListWorkflowsPublisher(this, listWorkflowsRequest);
}
/**
*
* This is a variant of {@link #listWorkflows(software.amazon.awssdk.services.transfer.model.ListWorkflowsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.transfer.paginators.ListWorkflowsPublisher publisher = client.listWorkflowsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.transfer.paginators.ListWorkflowsPublisher publisher = client.listWorkflowsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.transfer.model.ListWorkflowsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listWorkflows(software.amazon.awssdk.services.transfer.model.ListWorkflowsRequest)} operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListWorkflowsRequest.Builder} avoiding the need to
* create one manually via {@link ListWorkflowsRequest#builder()}
*
*
* @param listWorkflowsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transfer.model.ListWorkflowsRequest.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - InvalidNextTokenException The
NextToken
parameter that was passed is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ListWorkflows
* @see AWS API
* Documentation
*/
default ListWorkflowsPublisher listWorkflowsPaginator(Consumer listWorkflowsRequest) {
return listWorkflowsPaginator(ListWorkflowsRequest.builder().applyMutation(listWorkflowsRequest).build());
}
/**
*
* Sends a callback for asynchronous custom steps.
*
*
* The ExecutionId
, WorkflowId
, and Token
are passed to the target resource
* during execution of a custom step of a workflow. You must include those with their callback as well as providing
* a status.
*
*
* @param sendWorkflowStepStateRequest
* @return A Java Future containing the result of the SendWorkflowStepState operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.SendWorkflowStepState
* @see AWS API Documentation
*/
default CompletableFuture sendWorkflowStepState(
SendWorkflowStepStateRequest sendWorkflowStepStateRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Sends a callback for asynchronous custom steps.
*
*
* The ExecutionId
, WorkflowId
, and Token
are passed to the target resource
* during execution of a custom step of a workflow. You must include those with their callback as well as providing
* a status.
*
*
*
* This is a convenience which creates an instance of the {@link SendWorkflowStepStateRequest.Builder} avoiding the
* need to create one manually via {@link SendWorkflowStepStateRequest#builder()}
*
*
* @param sendWorkflowStepStateRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transfer.model.SendWorkflowStepStateRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the SendWorkflowStepState operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.SendWorkflowStepState
* @see AWS API Documentation
*/
default CompletableFuture sendWorkflowStepState(
Consumer sendWorkflowStepStateRequest) {
return sendWorkflowStepState(SendWorkflowStepStateRequest.builder().applyMutation(sendWorkflowStepStateRequest).build());
}
/**
*
* Retrieves a list of the contents of a directory from a remote SFTP server. You specify the connector ID, the
* output path, and the remote directory path. You can also specify the optional MaxItems
value to
* control the maximum number of items that are listed from the remote directory. This API returns a list of all
* files and directories in the remote directory (up to the maximum value), but does not return files or folders in
* sub-directories. That is, it only returns a list of files and directories one-level deep.
*
*
* After you receive the listing file, you can provide the files that you want to transfer to the
* RetrieveFilePaths
parameter of the StartFileTransfer
API call.
*
*
* The naming convention for the output file is connector-ID-listing-ID.json
. The output
* file contains the following information:
*
*
* -
*
* filePath
: the complete path of a remote file, relative to the directory of the listing request for
* your SFTP connector on the remote server.
*
*
* -
*
* modifiedTimestamp
: the last time the file was modified, in UTC time format. This field is optional.
* If the remote file attributes don't contain a timestamp, it is omitted from the file listing.
*
*
* -
*
* size
: the size of the file, in bytes. This field is optional. If the remote file attributes don't
* contain a file size, it is omitted from the file listing.
*
*
* -
*
* path
: the complete path of a remote directory, relative to the directory of the listing request for
* your SFTP connector on the remote server.
*
*
* -
*
* truncated
: a flag indicating whether the list output contains all of the items contained in the
* remote directory or not. If your Truncated
output value is true, you can increase the value provided
* in the optional max-items
input attribute to be able to list more items (up to the maximum allowed
* list size of 10,000 items).
*
*
*
*
* @param startDirectoryListingRequest
* @return A Java Future containing the result of the StartDirectoryListing operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.StartDirectoryListing
* @see AWS API Documentation
*/
default CompletableFuture startDirectoryListing(
StartDirectoryListingRequest startDirectoryListingRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves a list of the contents of a directory from a remote SFTP server. You specify the connector ID, the
* output path, and the remote directory path. You can also specify the optional MaxItems
value to
* control the maximum number of items that are listed from the remote directory. This API returns a list of all
* files and directories in the remote directory (up to the maximum value), but does not return files or folders in
* sub-directories. That is, it only returns a list of files and directories one-level deep.
*
*
* After you receive the listing file, you can provide the files that you want to transfer to the
* RetrieveFilePaths
parameter of the StartFileTransfer
API call.
*
*
* The naming convention for the output file is connector-ID-listing-ID.json
. The output
* file contains the following information:
*
*
* -
*
* filePath
: the complete path of a remote file, relative to the directory of the listing request for
* your SFTP connector on the remote server.
*
*
* -
*
* modifiedTimestamp
: the last time the file was modified, in UTC time format. This field is optional.
* If the remote file attributes don't contain a timestamp, it is omitted from the file listing.
*
*
* -
*
* size
: the size of the file, in bytes. This field is optional. If the remote file attributes don't
* contain a file size, it is omitted from the file listing.
*
*
* -
*
* path
: the complete path of a remote directory, relative to the directory of the listing request for
* your SFTP connector on the remote server.
*
*
* -
*
* truncated
: a flag indicating whether the list output contains all of the items contained in the
* remote directory or not. If your Truncated
output value is true, you can increase the value provided
* in the optional max-items
input attribute to be able to list more items (up to the maximum allowed
* list size of 10,000 items).
*
*
*
*
*
* This is a convenience which creates an instance of the {@link StartDirectoryListingRequest.Builder} avoiding the
* need to create one manually via {@link StartDirectoryListingRequest#builder()}
*
*
* @param startDirectoryListingRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transfer.model.StartDirectoryListingRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the StartDirectoryListing operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.StartDirectoryListing
* @see AWS API Documentation
*/
default CompletableFuture startDirectoryListing(
Consumer startDirectoryListingRequest) {
return startDirectoryListing(StartDirectoryListingRequest.builder().applyMutation(startDirectoryListingRequest).build());
}
/**
*
* Begins a file transfer between local Amazon Web Services storage and a remote AS2 or SFTP server.
*
*
* -
*
* For an AS2 connector, you specify the ConnectorId
and one or more SendFilePaths
to
* identify the files you want to transfer.
*
*
* -
*
* For an SFTP connector, the file transfer can be either outbound or inbound. In both cases, you specify the
* ConnectorId
. Depending on the direction of the transfer, you also specify the following items:
*
*
* -
*
* If you are transferring file from a partner's SFTP server to Amazon Web Services storage, you specify one or more
* RetrieveFilePaths
to identify the files you want to transfer, and a LocalDirectoryPath
* to specify the destination folder.
*
*
* -
*
* If you are transferring file to a partner's SFTP server from Amazon Web Services storage, you specify one or more
* SendFilePaths
to identify the files you want to transfer, and a RemoteDirectoryPath
to
* specify the destination folder.
*
*
*
*
*
*
* @param startFileTransferRequest
* @return A Java Future containing the result of the StartFileTransfer operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.StartFileTransfer
* @see AWS
* API Documentation
*/
default CompletableFuture startFileTransfer(StartFileTransferRequest startFileTransferRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Begins a file transfer between local Amazon Web Services storage and a remote AS2 or SFTP server.
*
*
* -
*
* For an AS2 connector, you specify the ConnectorId
and one or more SendFilePaths
to
* identify the files you want to transfer.
*
*
* -
*
* For an SFTP connector, the file transfer can be either outbound or inbound. In both cases, you specify the
* ConnectorId
. Depending on the direction of the transfer, you also specify the following items:
*
*
* -
*
* If you are transferring file from a partner's SFTP server to Amazon Web Services storage, you specify one or more
* RetrieveFilePaths
to identify the files you want to transfer, and a LocalDirectoryPath
* to specify the destination folder.
*
*
* -
*
* If you are transferring file to a partner's SFTP server from Amazon Web Services storage, you specify one or more
* SendFilePaths
to identify the files you want to transfer, and a RemoteDirectoryPath
to
* specify the destination folder.
*
*
*
*
*
*
*
* This is a convenience which creates an instance of the {@link StartFileTransferRequest.Builder} avoiding the need
* to create one manually via {@link StartFileTransferRequest#builder()}
*
*
* @param startFileTransferRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transfer.model.StartFileTransferRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the StartFileTransfer operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.StartFileTransfer
* @see AWS
* API Documentation
*/
default CompletableFuture startFileTransfer(
Consumer startFileTransferRequest) {
return startFileTransfer(StartFileTransferRequest.builder().applyMutation(startFileTransferRequest).build());
}
/**
*
* Changes the state of a file transfer protocol-enabled server from OFFLINE
to ONLINE
. It
* has no impact on a server that is already ONLINE
. An ONLINE
server can accept and
* process file transfer jobs.
*
*
* The state of STARTING
indicates that the server is in an intermediate state, either not fully able
* to respond, or not fully online. The values of START_FAILED
can indicate an error condition.
*
*
* No response is returned from this call.
*
*
* @param startServerRequest
* @return A Java Future containing the result of the StartServer operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.StartServer
* @see AWS API
* Documentation
*/
default CompletableFuture startServer(StartServerRequest startServerRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Changes the state of a file transfer protocol-enabled server from OFFLINE
to ONLINE
. It
* has no impact on a server that is already ONLINE
. An ONLINE
server can accept and
* process file transfer jobs.
*
*
* The state of STARTING
indicates that the server is in an intermediate state, either not fully able
* to respond, or not fully online. The values of START_FAILED
can indicate an error condition.
*
*
* No response is returned from this call.
*
*
*
* This is a convenience which creates an instance of the {@link StartServerRequest.Builder} avoiding the need to
* create one manually via {@link StartServerRequest#builder()}
*
*
* @param startServerRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transfer.model.StartServerRequest.Builder} to create a request.
* @return A Java Future containing the result of the StartServer operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.StartServer
* @see AWS API
* Documentation
*/
default CompletableFuture startServer(Consumer startServerRequest) {
return startServer(StartServerRequest.builder().applyMutation(startServerRequest).build());
}
/**
*
* Changes the state of a file transfer protocol-enabled server from ONLINE
to OFFLINE
. An
* OFFLINE
server cannot accept and process file transfer jobs. Information tied to your server, such
* as server and user properties, are not affected by stopping your server.
*
*
*
* Stopping the server does not reduce or impact your file transfer protocol endpoint billing; you must delete the
* server to stop being billed.
*
*
*
* The state of STOPPING
indicates that the server is in an intermediate state, either not fully able
* to respond, or not fully offline. The values of STOP_FAILED
can indicate an error condition.
*
*
* No response is returned from this call.
*
*
* @param stopServerRequest
* @return A Java Future containing the result of the StopServer operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.StopServer
* @see AWS API
* Documentation
*/
default CompletableFuture stopServer(StopServerRequest stopServerRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Changes the state of a file transfer protocol-enabled server from ONLINE
to OFFLINE
. An
* OFFLINE
server cannot accept and process file transfer jobs. Information tied to your server, such
* as server and user properties, are not affected by stopping your server.
*
*
*
* Stopping the server does not reduce or impact your file transfer protocol endpoint billing; you must delete the
* server to stop being billed.
*
*
*
* The state of STOPPING
indicates that the server is in an intermediate state, either not fully able
* to respond, or not fully offline. The values of STOP_FAILED
can indicate an error condition.
*
*
* No response is returned from this call.
*
*
*
* This is a convenience which creates an instance of the {@link StopServerRequest.Builder} avoiding the need to
* create one manually via {@link StopServerRequest#builder()}
*
*
* @param stopServerRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transfer.model.StopServerRequest.Builder} to create a request.
* @return A Java Future containing the result of the StopServer operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.StopServer
* @see AWS API
* Documentation
*/
default CompletableFuture stopServer(Consumer stopServerRequest) {
return stopServer(StopServerRequest.builder().applyMutation(stopServerRequest).build());
}
/**
*
* Attaches a key-value pair to a resource, as identified by its Amazon Resource Name (ARN). Resources are users,
* servers, roles, and other entities.
*
*
* There is no response returned from this call.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.TagResource
* @see AWS API
* Documentation
*/
default CompletableFuture tagResource(TagResourceRequest tagResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Attaches a key-value pair to a resource, as identified by its Amazon Resource Name (ARN). Resources are users,
* servers, roles, and other entities.
*
*
* There is no response returned from this call.
*
*
*
* This is a convenience which creates an instance of the {@link TagResourceRequest.Builder} avoiding the need to
* create one manually via {@link TagResourceRequest#builder()}
*
*
* @param tagResourceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transfer.model.TagResourceRequest.Builder} to create a request.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.TagResource
* @see AWS API
* Documentation
*/
default CompletableFuture tagResource(Consumer tagResourceRequest) {
return tagResource(TagResourceRequest.builder().applyMutation(tagResourceRequest).build());
}
/**
*
* Tests whether your SFTP connector is set up successfully. We highly recommend that you call this operation to
* test your ability to transfer files between local Amazon Web Services storage and a trading partner's SFTP
* server.
*
*
* @param testConnectionRequest
* @return A Java Future containing the result of the TestConnection operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.TestConnection
* @see AWS API
* Documentation
*/
default CompletableFuture testConnection(TestConnectionRequest testConnectionRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Tests whether your SFTP connector is set up successfully. We highly recommend that you call this operation to
* test your ability to transfer files between local Amazon Web Services storage and a trading partner's SFTP
* server.
*
*
*
* This is a convenience which creates an instance of the {@link TestConnectionRequest.Builder} avoiding the need to
* create one manually via {@link TestConnectionRequest#builder()}
*
*
* @param testConnectionRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transfer.model.TestConnectionRequest.Builder} to create a request.
* @return A Java Future containing the result of the TestConnection operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.TestConnection
* @see AWS API
* Documentation
*/
default CompletableFuture testConnection(Consumer testConnectionRequest) {
return testConnection(TestConnectionRequest.builder().applyMutation(testConnectionRequest).build());
}
/**
*
* If the IdentityProviderType
of a file transfer protocol-enabled server is
* AWS_DIRECTORY_SERVICE
or API_Gateway
, tests whether your identity provider is set up
* successfully. We highly recommend that you call this operation to test your authentication method as soon as you
* create your server. By doing so, you can troubleshoot issues with the identity provider integration to ensure
* that your users can successfully use the service.
*
*
* The ServerId
and UserName
parameters are required. The ServerProtocol
,
* SourceIp
, and UserPassword
are all optional.
*
*
* Note the following:
*
*
* -
*
* You cannot use TestIdentityProvider
if the IdentityProviderType
of your server is
* SERVICE_MANAGED
.
*
*
* -
*
* TestIdentityProvider
does not work with keys: it only accepts passwords.
*
*
* -
*
* TestIdentityProvider
can test the password operation for a custom Identity Provider that handles
* keys and passwords.
*
*
* -
*
* If you provide any incorrect values for any parameters, the Response
field is empty.
*
*
* -
*
* If you provide a server ID for a server that uses service-managed users, you get an error:
*
*
* An error occurred (InvalidRequestException) when calling the TestIdentityProvider operation: s-server-ID not configured for external auth
*
*
* -
*
* If you enter a Server ID for the --server-id
parameter that does not identify an actual Transfer
* server, you receive the following error:
*
*
* An error occurred (ResourceNotFoundException) when calling the TestIdentityProvider operation: Unknown server
* .
*
*
* It is possible your sever is in a different region. You can specify a region by adding the following:
* --region region-code
, such as --region us-east-2
to specify a server in US East
* (Ohio).
*
*
*
*
* @param testIdentityProviderRequest
* @return A Java Future containing the result of the TestIdentityProvider operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.TestIdentityProvider
* @see AWS
* API Documentation
*/
default CompletableFuture testIdentityProvider(
TestIdentityProviderRequest testIdentityProviderRequest) {
throw new UnsupportedOperationException();
}
/**
*
* If the IdentityProviderType
of a file transfer protocol-enabled server is
* AWS_DIRECTORY_SERVICE
or API_Gateway
, tests whether your identity provider is set up
* successfully. We highly recommend that you call this operation to test your authentication method as soon as you
* create your server. By doing so, you can troubleshoot issues with the identity provider integration to ensure
* that your users can successfully use the service.
*
*
* The ServerId
and UserName
parameters are required. The ServerProtocol
,
* SourceIp
, and UserPassword
are all optional.
*
*
* Note the following:
*
*
* -
*
* You cannot use TestIdentityProvider
if the IdentityProviderType
of your server is
* SERVICE_MANAGED
.
*
*
* -
*
* TestIdentityProvider
does not work with keys: it only accepts passwords.
*
*
* -
*
* TestIdentityProvider
can test the password operation for a custom Identity Provider that handles
* keys and passwords.
*
*
* -
*
* If you provide any incorrect values for any parameters, the Response
field is empty.
*
*
* -
*
* If you provide a server ID for a server that uses service-managed users, you get an error:
*
*
* An error occurred (InvalidRequestException) when calling the TestIdentityProvider operation: s-server-ID not configured for external auth
*
*
* -
*
* If you enter a Server ID for the --server-id
parameter that does not identify an actual Transfer
* server, you receive the following error:
*
*
* An error occurred (ResourceNotFoundException) when calling the TestIdentityProvider operation: Unknown server
* .
*
*
* It is possible your sever is in a different region. You can specify a region by adding the following:
* --region region-code
, such as --region us-east-2
to specify a server in US East
* (Ohio).
*
*
*
*
*
* This is a convenience which creates an instance of the {@link TestIdentityProviderRequest.Builder} avoiding the
* need to create one manually via {@link TestIdentityProviderRequest#builder()}
*
*
* @param testIdentityProviderRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transfer.model.TestIdentityProviderRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the TestIdentityProvider operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.TestIdentityProvider
* @see AWS
* API Documentation
*/
default CompletableFuture testIdentityProvider(
Consumer testIdentityProviderRequest) {
return testIdentityProvider(TestIdentityProviderRequest.builder().applyMutation(testIdentityProviderRequest).build());
}
/**
*
* Detaches a key-value pair from a resource, as identified by its Amazon Resource Name (ARN). Resources are users,
* servers, roles, and other entities.
*
*
* No response is returned from this call.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.UntagResource
* @see AWS API
* Documentation
*/
default CompletableFuture untagResource(UntagResourceRequest untagResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Detaches a key-value pair from a resource, as identified by its Amazon Resource Name (ARN). Resources are users,
* servers, roles, and other entities.
*
*
* No response is returned from this call.
*
*
*
* This is a convenience which creates an instance of the {@link UntagResourceRequest.Builder} avoiding the need to
* create one manually via {@link UntagResourceRequest#builder()}
*
*
* @param untagResourceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transfer.model.UntagResourceRequest.Builder} to create a request.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.UntagResource
* @see AWS API
* Documentation
*/
default CompletableFuture untagResource(Consumer untagResourceRequest) {
return untagResource(UntagResourceRequest.builder().applyMutation(untagResourceRequest).build());
}
/**
*
* Allows you to update parameters for the access specified in the ServerID
and ExternalID
* parameters.
*
*
* @param updateAccessRequest
* @return A Java Future containing the result of the UpdateAccess operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - ResourceExistsException The requested resource does not exist, or exists in a region other than the
* one specified for the command.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.UpdateAccess
* @see AWS API
* Documentation
*/
default CompletableFuture updateAccess(UpdateAccessRequest updateAccessRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Allows you to update parameters for the access specified in the ServerID
and ExternalID
* parameters.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateAccessRequest.Builder} avoiding the need to
* create one manually via {@link UpdateAccessRequest#builder()}
*
*
* @param updateAccessRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transfer.model.UpdateAccessRequest.Builder} to create a request.
* @return A Java Future containing the result of the UpdateAccess operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - ResourceExistsException The requested resource does not exist, or exists in a region other than the
* one specified for the command.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.UpdateAccess
* @see AWS API
* Documentation
*/
default CompletableFuture updateAccess(Consumer updateAccessRequest) {
return updateAccess(UpdateAccessRequest.builder().applyMutation(updateAccessRequest).build());
}
/**
*
* Updates some of the parameters for an existing agreement. Provide the AgreementId
and the
* ServerId
for the agreement that you want to update, along with the new values for the parameters to
* update.
*
*
* @param updateAgreementRequest
* @return A Java Future containing the result of the UpdateAgreement operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - ResourceExistsException The requested resource does not exist, or exists in a region other than the
* one specified for the command.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.UpdateAgreement
* @see AWS API
* Documentation
*/
default CompletableFuture updateAgreement(UpdateAgreementRequest updateAgreementRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates some of the parameters for an existing agreement. Provide the AgreementId
and the
* ServerId
for the agreement that you want to update, along with the new values for the parameters to
* update.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateAgreementRequest.Builder} avoiding the need
* to create one manually via {@link UpdateAgreementRequest#builder()}
*
*
* @param updateAgreementRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transfer.model.UpdateAgreementRequest.Builder} to create a request.
* @return A Java Future containing the result of the UpdateAgreement operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - ResourceExistsException The requested resource does not exist, or exists in a region other than the
* one specified for the command.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.UpdateAgreement
* @see AWS API
* Documentation
*/
default CompletableFuture updateAgreement(
Consumer updateAgreementRequest) {
return updateAgreement(UpdateAgreementRequest.builder().applyMutation(updateAgreementRequest).build());
}
/**
*
* Updates the active and inactive dates for a certificate.
*
*
* @param updateCertificateRequest
* @return A Java Future containing the result of the UpdateCertificate operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.UpdateCertificate
* @see AWS
* API Documentation
*/
default CompletableFuture updateCertificate(UpdateCertificateRequest updateCertificateRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates the active and inactive dates for a certificate.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateCertificateRequest.Builder} avoiding the need
* to create one manually via {@link UpdateCertificateRequest#builder()}
*
*
* @param updateCertificateRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transfer.model.UpdateCertificateRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the UpdateCertificate operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.UpdateCertificate
* @see AWS
* API Documentation
*/
default CompletableFuture updateCertificate(
Consumer updateCertificateRequest) {
return updateCertificate(UpdateCertificateRequest.builder().applyMutation(updateCertificateRequest).build());
}
/**
*
* Updates some of the parameters for an existing connector. Provide the ConnectorId
for the connector
* that you want to update, along with the new values for the parameters to update.
*
*
* @param updateConnectorRequest
* @return A Java Future containing the result of the UpdateConnector operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - ResourceExistsException The requested resource does not exist, or exists in a region other than the
* one specified for the command.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.UpdateConnector
* @see AWS API
* Documentation
*/
default CompletableFuture updateConnector(UpdateConnectorRequest updateConnectorRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates some of the parameters for an existing connector. Provide the ConnectorId
for the connector
* that you want to update, along with the new values for the parameters to update.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateConnectorRequest.Builder} avoiding the need
* to create one manually via {@link UpdateConnectorRequest#builder()}
*
*
* @param updateConnectorRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transfer.model.UpdateConnectorRequest.Builder} to create a request.
* @return A Java Future containing the result of the UpdateConnector operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - ResourceExistsException The requested resource does not exist, or exists in a region other than the
* one specified for the command.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.UpdateConnector
* @see AWS API
* Documentation
*/
default CompletableFuture updateConnector(
Consumer updateConnectorRequest) {
return updateConnector(UpdateConnectorRequest.builder().applyMutation(updateConnectorRequest).build());
}
/**
*
* Updates the description for the host key that's specified by the ServerId
and HostKeyId
* parameters.
*
*
* @param updateHostKeyRequest
* @return A Java Future containing the result of the UpdateHostKey operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.UpdateHostKey
* @see AWS API
* Documentation
*/
default CompletableFuture updateHostKey(UpdateHostKeyRequest updateHostKeyRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates the description for the host key that's specified by the ServerId
and HostKeyId
* parameters.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateHostKeyRequest.Builder} avoiding the need to
* create one manually via {@link UpdateHostKeyRequest#builder()}
*
*
* @param updateHostKeyRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transfer.model.UpdateHostKeyRequest.Builder} to create a request.
* @return A Java Future containing the result of the UpdateHostKey operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.UpdateHostKey
* @see AWS API
* Documentation
*/
default CompletableFuture updateHostKey(Consumer updateHostKeyRequest) {
return updateHostKey(UpdateHostKeyRequest.builder().applyMutation(updateHostKeyRequest).build());
}
/**
*
* Updates some of the parameters for an existing profile. Provide the ProfileId
for the profile that
* you want to update, along with the new values for the parameters to update.
*
*
* @param updateProfileRequest
* @return A Java Future containing the result of the UpdateProfile operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.UpdateProfile
* @see AWS API
* Documentation
*/
default CompletableFuture updateProfile(UpdateProfileRequest updateProfileRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates some of the parameters for an existing profile. Provide the ProfileId
for the profile that
* you want to update, along with the new values for the parameters to update.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateProfileRequest.Builder} avoiding the need to
* create one manually via {@link UpdateProfileRequest#builder()}
*
*
* @param updateProfileRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transfer.model.UpdateProfileRequest.Builder} to create a request.
* @return A Java Future containing the result of the UpdateProfile operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.UpdateProfile
* @see AWS API
* Documentation
*/
default CompletableFuture updateProfile(Consumer updateProfileRequest) {
return updateProfile(UpdateProfileRequest.builder().applyMutation(updateProfileRequest).build());
}
/**
*
* Updates the file transfer protocol-enabled server's properties after that server has been created.
*
*
* The UpdateServer
call returns the ServerId
of the server you updated.
*
*
* @param updateServerRequest
* @return A Java Future containing the result of the UpdateServer operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ConflictException This exception is thrown when the
UpdateServer
is called for a file
* transfer protocol-enabled server that has VPC as the endpoint type and the server's
* VpcEndpointID
is not in the available state.
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - ResourceExistsException The requested resource does not exist, or exists in a region other than the
* one specified for the command.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.UpdateServer
* @see AWS API
* Documentation
*/
default CompletableFuture updateServer(UpdateServerRequest updateServerRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates the file transfer protocol-enabled server's properties after that server has been created.
*
*
* The UpdateServer
call returns the ServerId
of the server you updated.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateServerRequest.Builder} avoiding the need to
* create one manually via {@link UpdateServerRequest#builder()}
*
*
* @param updateServerRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transfer.model.UpdateServerRequest.Builder} to create a request.
* @return A Java Future containing the result of the UpdateServer operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ConflictException This exception is thrown when the
UpdateServer
is called for a file
* transfer protocol-enabled server that has VPC as the endpoint type and the server's
* VpcEndpointID
is not in the available state.
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - ResourceExistsException The requested resource does not exist, or exists in a region other than the
* one specified for the command.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.UpdateServer
* @see AWS API
* Documentation
*/
default CompletableFuture updateServer(Consumer updateServerRequest) {
return updateServer(UpdateServerRequest.builder().applyMutation(updateServerRequest).build());
}
/**
*
* Assigns new properties to a user. Parameters you pass modify any or all of the following: the home directory,
* role, and policy for the UserName
and ServerId
you specify.
*
*
* The response returns the ServerId
and the UserName
for the updated user.
*
*
* In the console, you can select Restricted when you create or update a user. This ensures that the user
* can't access anything outside of their home directory. The programmatic way to configure this behavior is to
* update the user. Set their HomeDirectoryType
to LOGICAL
, and specify
* HomeDirectoryMappings
with Entry
as root (/
) and Target
as
* their home directory.
*
*
* For example, if the user's home directory is /test/admin-user
, the following command updates the
* user so that their configuration in the console shows the Restricted flag as selected.
*
*
* aws transfer update-user --server-id <server-id> --user-name admin-user --home-directory-type LOGICAL --home-directory-mappings "[{\"Entry\":\"/\", \"Target\":\"/test/admin-user\"}]"
*
*
* @param updateUserRequest
* @return A Java Future containing the result of the UpdateUser operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.UpdateUser
* @see AWS API
* Documentation
*/
default CompletableFuture updateUser(UpdateUserRequest updateUserRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Assigns new properties to a user. Parameters you pass modify any or all of the following: the home directory,
* role, and policy for the UserName
and ServerId
you specify.
*
*
* The response returns the ServerId
and the UserName
for the updated user.
*
*
* In the console, you can select Restricted when you create or update a user. This ensures that the user
* can't access anything outside of their home directory. The programmatic way to configure this behavior is to
* update the user. Set their HomeDirectoryType
to LOGICAL
, and specify
* HomeDirectoryMappings
with Entry
as root (/
) and Target
as
* their home directory.
*
*
* For example, if the user's home directory is /test/admin-user
, the following command updates the
* user so that their configuration in the console shows the Restricted flag as selected.
*
*
* aws transfer update-user --server-id <server-id> --user-name admin-user --home-directory-type LOGICAL --home-directory-mappings "[{\"Entry\":\"/\", \"Target\":\"/test/admin-user\"}]"
*
*
*
* This is a convenience which creates an instance of the {@link UpdateUserRequest.Builder} avoiding the need to
* create one manually via {@link UpdateUserRequest#builder()}
*
*
* @param updateUserRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.transfer.model.UpdateUserRequest.Builder} to create a request.
* @return A Java Future containing the result of the UpdateUser operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException This exception is thrown when a resource is not found by the Amazon Web
* ServicesTransfer Family service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServiceErrorException This exception is thrown when an error occurs in the Transfer Family
* service.
* - ServiceUnavailableException The request has failed because the Amazon Web ServicesTransfer Family
* service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.UpdateUser
* @see AWS API
* Documentation
*/
default CompletableFuture updateUser(Consumer updateUserRequest) {
return updateUser(UpdateUserRequest.builder().applyMutation(updateUserRequest).build());
}
/**
* Create an instance of {@link TransferAsyncWaiter} using this client.
*
* Waiters created via this method are managed by the SDK and resources will be released when the service client is
* closed.
*
* @return an instance of {@link TransferAsyncWaiter}
*/
default TransferAsyncWaiter waiter() {
throw new UnsupportedOperationException();
}
@Override
default TransferServiceClientConfiguration serviceClientConfiguration() {
throw new UnsupportedOperationException();
}
/**
* Create a {@link TransferAsyncClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static TransferAsyncClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link TransferAsyncClient}.
*/
static TransferAsyncClientBuilder builder() {
return new DefaultTransferAsyncClientBuilder();
}
}