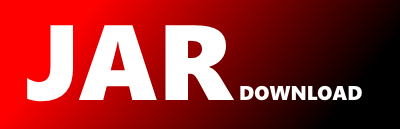
software.amazon.awssdk.services.transfer.TransferAsyncClient Maven / Gradle / Ivy
Show all versions of transfer Show documentation
/*
* Copyright 2014-2019 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.transfer;
import java.util.concurrent.CompletableFuture;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkClient;
import software.amazon.awssdk.services.transfer.model.CreateServerRequest;
import software.amazon.awssdk.services.transfer.model.CreateServerResponse;
import software.amazon.awssdk.services.transfer.model.CreateUserRequest;
import software.amazon.awssdk.services.transfer.model.CreateUserResponse;
import software.amazon.awssdk.services.transfer.model.DeleteServerRequest;
import software.amazon.awssdk.services.transfer.model.DeleteServerResponse;
import software.amazon.awssdk.services.transfer.model.DeleteSshPublicKeyRequest;
import software.amazon.awssdk.services.transfer.model.DeleteSshPublicKeyResponse;
import software.amazon.awssdk.services.transfer.model.DeleteUserRequest;
import software.amazon.awssdk.services.transfer.model.DeleteUserResponse;
import software.amazon.awssdk.services.transfer.model.DescribeServerRequest;
import software.amazon.awssdk.services.transfer.model.DescribeServerResponse;
import software.amazon.awssdk.services.transfer.model.DescribeUserRequest;
import software.amazon.awssdk.services.transfer.model.DescribeUserResponse;
import software.amazon.awssdk.services.transfer.model.ImportSshPublicKeyRequest;
import software.amazon.awssdk.services.transfer.model.ImportSshPublicKeyResponse;
import software.amazon.awssdk.services.transfer.model.ListServersRequest;
import software.amazon.awssdk.services.transfer.model.ListServersResponse;
import software.amazon.awssdk.services.transfer.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.transfer.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.transfer.model.ListUsersRequest;
import software.amazon.awssdk.services.transfer.model.ListUsersResponse;
import software.amazon.awssdk.services.transfer.model.StartServerRequest;
import software.amazon.awssdk.services.transfer.model.StartServerResponse;
import software.amazon.awssdk.services.transfer.model.StopServerRequest;
import software.amazon.awssdk.services.transfer.model.StopServerResponse;
import software.amazon.awssdk.services.transfer.model.TagResourceRequest;
import software.amazon.awssdk.services.transfer.model.TagResourceResponse;
import software.amazon.awssdk.services.transfer.model.TestIdentityProviderRequest;
import software.amazon.awssdk.services.transfer.model.TestIdentityProviderResponse;
import software.amazon.awssdk.services.transfer.model.UntagResourceRequest;
import software.amazon.awssdk.services.transfer.model.UntagResourceResponse;
import software.amazon.awssdk.services.transfer.model.UpdateServerRequest;
import software.amazon.awssdk.services.transfer.model.UpdateServerResponse;
import software.amazon.awssdk.services.transfer.model.UpdateUserRequest;
import software.amazon.awssdk.services.transfer.model.UpdateUserResponse;
import software.amazon.awssdk.services.transfer.paginators.ListServersPublisher;
import software.amazon.awssdk.services.transfer.paginators.ListTagsForResourcePublisher;
import software.amazon.awssdk.services.transfer.paginators.ListUsersPublisher;
/**
* Service client for accessing AWS Transfer asynchronously. This can be created using the static {@link #builder()}
* method.
*
*
* AWS Transfer for SFTP is a fully managed service that enables the transfer of files directly into and out of Amazon
* S3 using the Secure File Transfer Protocol (SFTP)—also known as Secure Shell (SSH) File Transfer Protocol. AWS helps
* you seamlessly migrate your file transfer workflows to AWS Transfer for SFTP—by integrating with existing
* authentication systems, and providing DNS routing with Amazon Route 53—so nothing changes for your customers and
* partners, or their applications. With your data in S3, you can use it with AWS services for processing, analytics,
* machine learning, and archiving. Getting started with AWS Transfer for SFTP (AWS SFTP) is easy; there is no
* infrastructure to buy and set up.
*
*/
@Generated("software.amazon.awssdk:codegen")
public interface TransferAsyncClient extends SdkClient {
String SERVICE_NAME = "transfer";
/**
* Create a {@link TransferAsyncClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static TransferAsyncClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link TransferAsyncClient}.
*/
static TransferAsyncClientBuilder builder() {
return new DefaultTransferAsyncClientBuilder();
}
/**
*
* Instantiates an autoscaling virtual server based on Secure File Transfer Protocol (SFTP) in AWS. When you make
* updates to your server or when you work with users, use the service-generated ServerId
property that
* is assigned to the newly created server.
*
*
* @param createServerRequest
* @return A Java Future containing the result of the CreateServer operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceUnavailableException The request has failed because the AWS Transfer for SFTP service is not
* available.
* - InternalServiceErrorException This exception is thrown when an error occurs in the AWS Transfer for
* SFTP service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ResourceExistsException The requested resource does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.CreateServer
* @see AWS API
* Documentation
*/
default CompletableFuture createServer(CreateServerRequest createServerRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Instantiates an autoscaling virtual server based on Secure File Transfer Protocol (SFTP) in AWS. When you make
* updates to your server or when you work with users, use the service-generated ServerId
property that
* is assigned to the newly created server.
*
*
*
* This is a convenience which creates an instance of the {@link CreateServerRequest.Builder} avoiding the need to
* create one manually via {@link CreateServerRequest#builder()}
*
*
* @param createServerRequest
* A {@link Consumer} that will call methods on {@link CreateServerRequest.Builder} to create a request.
* @return A Java Future containing the result of the CreateServer operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceUnavailableException The request has failed because the AWS Transfer for SFTP service is not
* available.
* - InternalServiceErrorException This exception is thrown when an error occurs in the AWS Transfer for
* SFTP service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ResourceExistsException The requested resource does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.CreateServer
* @see AWS API
* Documentation
*/
default CompletableFuture createServer(Consumer createServerRequest) {
return createServer(CreateServerRequest.builder().applyMutation(createServerRequest).build());
}
/**
*
* Creates a user and associates them with an existing Secure File Transfer Protocol (SFTP) server. You can only
* create and associate users with SFTP servers that have the IdentityProviderType
set to
* SERVICE_MANAGED
. Using parameters for CreateUser
, you can specify the user name, set
* the home directory, store the user's public key, and assign the user's AWS Identity and Access Management (IAM)
* role. You can also optionally add a scope-down policy, and assign metadata with tags that can be used to group
* and search for users.
*
*
* @param createUserRequest
* @return A Java Future containing the result of the CreateUser operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceUnavailableException The request has failed because the AWS Transfer for SFTP service is not
* available.
* - InternalServiceErrorException This exception is thrown when an error occurs in the AWS Transfer for
* SFTP service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ResourceExistsException The requested resource does not exist.
* - ResourceNotFoundException This exception is thrown when a resource is not found by the AWS Transfer
* for SFTP service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.CreateUser
* @see AWS API
* Documentation
*/
default CompletableFuture createUser(CreateUserRequest createUserRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a user and associates them with an existing Secure File Transfer Protocol (SFTP) server. You can only
* create and associate users with SFTP servers that have the IdentityProviderType
set to
* SERVICE_MANAGED
. Using parameters for CreateUser
, you can specify the user name, set
* the home directory, store the user's public key, and assign the user's AWS Identity and Access Management (IAM)
* role. You can also optionally add a scope-down policy, and assign metadata with tags that can be used to group
* and search for users.
*
*
*
* This is a convenience which creates an instance of the {@link CreateUserRequest.Builder} avoiding the need to
* create one manually via {@link CreateUserRequest#builder()}
*
*
* @param createUserRequest
* A {@link Consumer} that will call methods on {@link CreateUserRequest.Builder} to create a request.
* @return A Java Future containing the result of the CreateUser operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceUnavailableException The request has failed because the AWS Transfer for SFTP service is not
* available.
* - InternalServiceErrorException This exception is thrown when an error occurs in the AWS Transfer for
* SFTP service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ResourceExistsException The requested resource does not exist.
* - ResourceNotFoundException This exception is thrown when a resource is not found by the AWS Transfer
* for SFTP service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.CreateUser
* @see AWS API
* Documentation
*/
default CompletableFuture createUser(Consumer createUserRequest) {
return createUser(CreateUserRequest.builder().applyMutation(createUserRequest).build());
}
/**
*
* Deletes the Secure File Transfer Protocol (SFTP) server that you specify.
*
*
* No response returns from this operation.
*
*
* @param deleteServerRequest
* @return A Java Future containing the result of the DeleteServer operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceUnavailableException The request has failed because the AWS Transfer for SFTP service is not
* available.
* - InternalServiceErrorException This exception is thrown when an error occurs in the AWS Transfer for
* SFTP service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ResourceNotFoundException This exception is thrown when a resource is not found by the AWS Transfer
* for SFTP service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.DeleteServer
* @see AWS API
* Documentation
*/
default CompletableFuture deleteServer(DeleteServerRequest deleteServerRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the Secure File Transfer Protocol (SFTP) server that you specify.
*
*
* No response returns from this operation.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteServerRequest.Builder} avoiding the need to
* create one manually via {@link DeleteServerRequest#builder()}
*
*
* @param deleteServerRequest
* A {@link Consumer} that will call methods on {@link DeleteServerRequest.Builder} to create a request.
* @return A Java Future containing the result of the DeleteServer operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceUnavailableException The request has failed because the AWS Transfer for SFTP service is not
* available.
* - InternalServiceErrorException This exception is thrown when an error occurs in the AWS Transfer for
* SFTP service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ResourceNotFoundException This exception is thrown when a resource is not found by the AWS Transfer
* for SFTP service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.DeleteServer
* @see AWS API
* Documentation
*/
default CompletableFuture deleteServer(Consumer deleteServerRequest) {
return deleteServer(DeleteServerRequest.builder().applyMutation(deleteServerRequest).build());
}
/**
*
* Deletes a user's Secure Shell (SSH) public key.
*
*
* No response is returned from this operation.
*
*
* @param deleteSshPublicKeyRequest
* @return A Java Future containing the result of the DeleteSshPublicKey operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceUnavailableException The request has failed because the AWS Transfer for SFTP service is not
* available.
* - InternalServiceErrorException This exception is thrown when an error occurs in the AWS Transfer for
* SFTP service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ResourceNotFoundException This exception is thrown when a resource is not found by the AWS Transfer
* for SFTP service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.DeleteSshPublicKey
* @see AWS
* API Documentation
*/
default CompletableFuture deleteSshPublicKey(DeleteSshPublicKeyRequest deleteSshPublicKeyRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a user's Secure Shell (SSH) public key.
*
*
* No response is returned from this operation.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteSshPublicKeyRequest.Builder} avoiding the
* need to create one manually via {@link DeleteSshPublicKeyRequest#builder()}
*
*
* @param deleteSshPublicKeyRequest
* A {@link Consumer} that will call methods on {@link DeleteSshPublicKeyRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DeleteSshPublicKey operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceUnavailableException The request has failed because the AWS Transfer for SFTP service is not
* available.
* - InternalServiceErrorException This exception is thrown when an error occurs in the AWS Transfer for
* SFTP service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ResourceNotFoundException This exception is thrown when a resource is not found by the AWS Transfer
* for SFTP service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.DeleteSshPublicKey
* @see AWS
* API Documentation
*/
default CompletableFuture deleteSshPublicKey(
Consumer deleteSshPublicKeyRequest) {
return deleteSshPublicKey(DeleteSshPublicKeyRequest.builder().applyMutation(deleteSshPublicKeyRequest).build());
}
/**
*
* Deletes the user belonging to the server you specify.
*
*
* No response returns from this operation.
*
*
*
* When you delete a user from a server, the user's information is lost.
*
*
*
* @param deleteUserRequest
* @return A Java Future containing the result of the DeleteUser operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceUnavailableException The request has failed because the AWS Transfer for SFTP service is not
* available.
* - InternalServiceErrorException This exception is thrown when an error occurs in the AWS Transfer for
* SFTP service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ResourceNotFoundException This exception is thrown when a resource is not found by the AWS Transfer
* for SFTP service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.DeleteUser
* @see AWS API
* Documentation
*/
default CompletableFuture deleteUser(DeleteUserRequest deleteUserRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the user belonging to the server you specify.
*
*
* No response returns from this operation.
*
*
*
* When you delete a user from a server, the user's information is lost.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteUserRequest.Builder} avoiding the need to
* create one manually via {@link DeleteUserRequest#builder()}
*
*
* @param deleteUserRequest
* A {@link Consumer} that will call methods on {@link DeleteUserRequest.Builder} to create a request.
* @return A Java Future containing the result of the DeleteUser operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceUnavailableException The request has failed because the AWS Transfer for SFTP service is not
* available.
* - InternalServiceErrorException This exception is thrown when an error occurs in the AWS Transfer for
* SFTP service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ResourceNotFoundException This exception is thrown when a resource is not found by the AWS Transfer
* for SFTP service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.DeleteUser
* @see AWS API
* Documentation
*/
default CompletableFuture deleteUser(Consumer deleteUserRequest) {
return deleteUser(DeleteUserRequest.builder().applyMutation(deleteUserRequest).build());
}
/**
*
* Describes the server that you specify by passing the ServerId
parameter.
*
*
* The response contains a description of the server's properties.
*
*
* @param describeServerRequest
* @return A Java Future containing the result of the DescribeServer operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceUnavailableException The request has failed because the AWS Transfer for SFTP service is not
* available.
* - InternalServiceErrorException This exception is thrown when an error occurs in the AWS Transfer for
* SFTP service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ResourceNotFoundException This exception is thrown when a resource is not found by the AWS Transfer
* for SFTP service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.DescribeServer
* @see AWS API
* Documentation
*/
default CompletableFuture describeServer(DescribeServerRequest describeServerRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Describes the server that you specify by passing the ServerId
parameter.
*
*
* The response contains a description of the server's properties.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeServerRequest.Builder} avoiding the need to
* create one manually via {@link DescribeServerRequest#builder()}
*
*
* @param describeServerRequest
* A {@link Consumer} that will call methods on {@link DescribeServerRequest.Builder} to create a request.
* @return A Java Future containing the result of the DescribeServer operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceUnavailableException The request has failed because the AWS Transfer for SFTP service is not
* available.
* - InternalServiceErrorException This exception is thrown when an error occurs in the AWS Transfer for
* SFTP service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ResourceNotFoundException This exception is thrown when a resource is not found by the AWS Transfer
* for SFTP service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.DescribeServer
* @see AWS API
* Documentation
*/
default CompletableFuture describeServer(Consumer describeServerRequest) {
return describeServer(DescribeServerRequest.builder().applyMutation(describeServerRequest).build());
}
/**
*
* Describes the user assigned to a specific server, as identified by its ServerId
property.
*
*
* The response from this call returns the properties of the user associated with the ServerId
value
* that was specified.
*
*
* @param describeUserRequest
* @return A Java Future containing the result of the DescribeUser operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceUnavailableException The request has failed because the AWS Transfer for SFTP service is not
* available.
* - InternalServiceErrorException This exception is thrown when an error occurs in the AWS Transfer for
* SFTP service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ResourceNotFoundException This exception is thrown when a resource is not found by the AWS Transfer
* for SFTP service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.DescribeUser
* @see AWS API
* Documentation
*/
default CompletableFuture describeUser(DescribeUserRequest describeUserRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Describes the user assigned to a specific server, as identified by its ServerId
property.
*
*
* The response from this call returns the properties of the user associated with the ServerId
value
* that was specified.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeUserRequest.Builder} avoiding the need to
* create one manually via {@link DescribeUserRequest#builder()}
*
*
* @param describeUserRequest
* A {@link Consumer} that will call methods on {@link DescribeUserRequest.Builder} to create a request.
* @return A Java Future containing the result of the DescribeUser operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceUnavailableException The request has failed because the AWS Transfer for SFTP service is not
* available.
* - InternalServiceErrorException This exception is thrown when an error occurs in the AWS Transfer for
* SFTP service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ResourceNotFoundException This exception is thrown when a resource is not found by the AWS Transfer
* for SFTP service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.DescribeUser
* @see AWS API
* Documentation
*/
default CompletableFuture describeUser(Consumer describeUserRequest) {
return describeUser(DescribeUserRequest.builder().applyMutation(describeUserRequest).build());
}
/**
*
* Adds a Secure Shell (SSH) public key to a user account identified by a UserName
value assigned to a
* specific server, identified by ServerId
.
*
*
* The response returns the UserName
value, the ServerId
value, and the name of the
* SshPublicKeyId
.
*
*
* @param importSshPublicKeyRequest
* @return A Java Future containing the result of the ImportSshPublicKey operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceUnavailableException The request has failed because the AWS Transfer for SFTP service is not
* available.
* - InternalServiceErrorException This exception is thrown when an error occurs in the AWS Transfer for
* SFTP service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ResourceExistsException The requested resource does not exist.
* - ResourceNotFoundException This exception is thrown when a resource is not found by the AWS Transfer
* for SFTP service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ImportSshPublicKey
* @see AWS
* API Documentation
*/
default CompletableFuture importSshPublicKey(ImportSshPublicKeyRequest importSshPublicKeyRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Adds a Secure Shell (SSH) public key to a user account identified by a UserName
value assigned to a
* specific server, identified by ServerId
.
*
*
* The response returns the UserName
value, the ServerId
value, and the name of the
* SshPublicKeyId
.
*
*
*
* This is a convenience which creates an instance of the {@link ImportSshPublicKeyRequest.Builder} avoiding the
* need to create one manually via {@link ImportSshPublicKeyRequest#builder()}
*
*
* @param importSshPublicKeyRequest
* A {@link Consumer} that will call methods on {@link ImportSshPublicKeyRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ImportSshPublicKey operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceUnavailableException The request has failed because the AWS Transfer for SFTP service is not
* available.
* - InternalServiceErrorException This exception is thrown when an error occurs in the AWS Transfer for
* SFTP service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ResourceExistsException The requested resource does not exist.
* - ResourceNotFoundException This exception is thrown when a resource is not found by the AWS Transfer
* for SFTP service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ImportSshPublicKey
* @see AWS
* API Documentation
*/
default CompletableFuture importSshPublicKey(
Consumer importSshPublicKeyRequest) {
return importSshPublicKey(ImportSshPublicKeyRequest.builder().applyMutation(importSshPublicKeyRequest).build());
}
/**
*
* Lists the Secure File Transfer Protocol (SFTP) servers that are associated with your AWS account.
*
*
* @param listServersRequest
* @return A Java Future containing the result of the ListServers operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceUnavailableException The request has failed because the AWS Transfer for SFTP service is not
* available.
* - InternalServiceErrorException This exception is thrown when an error occurs in the AWS Transfer for
* SFTP service.
* - InvalidNextTokenException The
NextToken
parameter that was passed is invalid.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ListServers
* @see AWS API
* Documentation
*/
default CompletableFuture listServers(ListServersRequest listServersRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists the Secure File Transfer Protocol (SFTP) servers that are associated with your AWS account.
*
*
*
* This is a convenience which creates an instance of the {@link ListServersRequest.Builder} avoiding the need to
* create one manually via {@link ListServersRequest#builder()}
*
*
* @param listServersRequest
* A {@link Consumer} that will call methods on {@link ListServersRequest.Builder} to create a request.
* @return A Java Future containing the result of the ListServers operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceUnavailableException The request has failed because the AWS Transfer for SFTP service is not
* available.
* - InternalServiceErrorException This exception is thrown when an error occurs in the AWS Transfer for
* SFTP service.
* - InvalidNextTokenException The
NextToken
parameter that was passed is invalid.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ListServers
* @see AWS API
* Documentation
*/
default CompletableFuture listServers(Consumer listServersRequest) {
return listServers(ListServersRequest.builder().applyMutation(listServersRequest).build());
}
/**
*
* Lists the Secure File Transfer Protocol (SFTP) servers that are associated with your AWS account.
*
*
* @return A Java Future containing the result of the ListServers operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceUnavailableException The request has failed because the AWS Transfer for SFTP service is not
* available.
* - InternalServiceErrorException This exception is thrown when an error occurs in the AWS Transfer for
* SFTP service.
* - InvalidNextTokenException The
NextToken
parameter that was passed is invalid.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ListServers
* @see AWS API
* Documentation
*/
default CompletableFuture listServers() {
return listServers(ListServersRequest.builder().build());
}
/**
*
* Lists the Secure File Transfer Protocol (SFTP) servers that are associated with your AWS account.
*
*
*
* This is a variant of {@link #listServers(software.amazon.awssdk.services.transfer.model.ListServersRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.transfer.paginators.ListServersPublisher publisher = client.listServersPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.transfer.paginators.ListServersPublisher publisher = client.listServersPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.transfer.model.ListServersResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Note: If you prefer to have control on service calls, use the
* {@link #listServers(software.amazon.awssdk.services.transfer.model.ListServersRequest)} operation.
*
*
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceUnavailableException The request has failed because the AWS Transfer for SFTP service is not
* available.
* - InternalServiceErrorException This exception is thrown when an error occurs in the AWS Transfer for
* SFTP service.
* - InvalidNextTokenException The
NextToken
parameter that was passed is invalid.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ListServers
* @see AWS API
* Documentation
*/
default ListServersPublisher listServersPaginator() {
return listServersPaginator(ListServersRequest.builder().build());
}
/**
*
* Lists the Secure File Transfer Protocol (SFTP) servers that are associated with your AWS account.
*
*
*
* This is a variant of {@link #listServers(software.amazon.awssdk.services.transfer.model.ListServersRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.transfer.paginators.ListServersPublisher publisher = client.listServersPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.transfer.paginators.ListServersPublisher publisher = client.listServersPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.transfer.model.ListServersResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Note: If you prefer to have control on service calls, use the
* {@link #listServers(software.amazon.awssdk.services.transfer.model.ListServersRequest)} operation.
*
*
* @param listServersRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceUnavailableException The request has failed because the AWS Transfer for SFTP service is not
* available.
* - InternalServiceErrorException This exception is thrown when an error occurs in the AWS Transfer for
* SFTP service.
* - InvalidNextTokenException The
NextToken
parameter that was passed is invalid.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ListServers
* @see AWS API
* Documentation
*/
default ListServersPublisher listServersPaginator(ListServersRequest listServersRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists the Secure File Transfer Protocol (SFTP) servers that are associated with your AWS account.
*
*
*
* This is a variant of {@link #listServers(software.amazon.awssdk.services.transfer.model.ListServersRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.transfer.paginators.ListServersPublisher publisher = client.listServersPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.transfer.paginators.ListServersPublisher publisher = client.listServersPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.transfer.model.ListServersResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Note: If you prefer to have control on service calls, use the
* {@link #listServers(software.amazon.awssdk.services.transfer.model.ListServersRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListServersRequest.Builder} avoiding the need to
* create one manually via {@link ListServersRequest#builder()}
*
*
* @param listServersRequest
* A {@link Consumer} that will call methods on {@link ListServersRequest.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceUnavailableException The request has failed because the AWS Transfer for SFTP service is not
* available.
* - InternalServiceErrorException This exception is thrown when an error occurs in the AWS Transfer for
* SFTP service.
* - InvalidNextTokenException The
NextToken
parameter that was passed is invalid.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ListServers
* @see AWS API
* Documentation
*/
default ListServersPublisher listServersPaginator(Consumer listServersRequest) {
return listServersPaginator(ListServersRequest.builder().applyMutation(listServersRequest).build());
}
/**
*
* Lists all of the tags associated with the Amazon Resource Number (ARN) you specify. The resource can be a user,
* server, or role.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceUnavailableException The request has failed because the AWS Transfer for SFTP service is not
* available.
* - InternalServiceErrorException This exception is thrown when an error occurs in the AWS Transfer for
* SFTP service.
* - InvalidNextTokenException The
NextToken
parameter that was passed is invalid.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ListTagsForResource
* @see AWS
* API Documentation
*/
default CompletableFuture listTagsForResource(
ListTagsForResourceRequest listTagsForResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists all of the tags associated with the Amazon Resource Number (ARN) you specify. The resource can be a user,
* server, or role.
*
*
*
* This is a convenience which creates an instance of the {@link ListTagsForResourceRequest.Builder} avoiding the
* need to create one manually via {@link ListTagsForResourceRequest#builder()}
*
*
* @param listTagsForResourceRequest
* A {@link Consumer} that will call methods on {@link ListTagsForResourceRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceUnavailableException The request has failed because the AWS Transfer for SFTP service is not
* available.
* - InternalServiceErrorException This exception is thrown when an error occurs in the AWS Transfer for
* SFTP service.
* - InvalidNextTokenException The
NextToken
parameter that was passed is invalid.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ListTagsForResource
* @see AWS
* API Documentation
*/
default CompletableFuture listTagsForResource(
Consumer listTagsForResourceRequest) {
return listTagsForResource(ListTagsForResourceRequest.builder().applyMutation(listTagsForResourceRequest).build());
}
/**
*
* Lists all of the tags associated with the Amazon Resource Number (ARN) you specify. The resource can be a user,
* server, or role.
*
*
*
* This is a variant of
* {@link #listTagsForResource(software.amazon.awssdk.services.transfer.model.ListTagsForResourceRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.transfer.paginators.ListTagsForResourcePublisher publisher = client.listTagsForResourcePaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.transfer.paginators.ListTagsForResourcePublisher publisher = client.listTagsForResourcePaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.transfer.model.ListTagsForResourceResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Note: If you prefer to have control on service calls, use the
* {@link #listTagsForResource(software.amazon.awssdk.services.transfer.model.ListTagsForResourceRequest)}
* operation.
*
*
* @param listTagsForResourceRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceUnavailableException The request has failed because the AWS Transfer for SFTP service is not
* available.
* - InternalServiceErrorException This exception is thrown when an error occurs in the AWS Transfer for
* SFTP service.
* - InvalidNextTokenException The
NextToken
parameter that was passed is invalid.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ListTagsForResource
* @see AWS
* API Documentation
*/
default ListTagsForResourcePublisher listTagsForResourcePaginator(ListTagsForResourceRequest listTagsForResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists all of the tags associated with the Amazon Resource Number (ARN) you specify. The resource can be a user,
* server, or role.
*
*
*
* This is a variant of
* {@link #listTagsForResource(software.amazon.awssdk.services.transfer.model.ListTagsForResourceRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.transfer.paginators.ListTagsForResourcePublisher publisher = client.listTagsForResourcePaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.transfer.paginators.ListTagsForResourcePublisher publisher = client.listTagsForResourcePaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.transfer.model.ListTagsForResourceResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Note: If you prefer to have control on service calls, use the
* {@link #listTagsForResource(software.amazon.awssdk.services.transfer.model.ListTagsForResourceRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListTagsForResourceRequest.Builder} avoiding the
* need to create one manually via {@link ListTagsForResourceRequest#builder()}
*
*
* @param listTagsForResourceRequest
* A {@link Consumer} that will call methods on {@link ListTagsForResourceRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceUnavailableException The request has failed because the AWS Transfer for SFTP service is not
* available.
* - InternalServiceErrorException This exception is thrown when an error occurs in the AWS Transfer for
* SFTP service.
* - InvalidNextTokenException The
NextToken
parameter that was passed is invalid.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ListTagsForResource
* @see AWS
* API Documentation
*/
default ListTagsForResourcePublisher listTagsForResourcePaginator(
Consumer listTagsForResourceRequest) {
return listTagsForResourcePaginator(ListTagsForResourceRequest.builder().applyMutation(listTagsForResourceRequest)
.build());
}
/**
*
* Lists the users for the server that you specify by passing the ServerId
parameter.
*
*
* @param listUsersRequest
* @return A Java Future containing the result of the ListUsers operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceUnavailableException The request has failed because the AWS Transfer for SFTP service is not
* available.
* - InternalServiceErrorException This exception is thrown when an error occurs in the AWS Transfer for
* SFTP service.
* - InvalidNextTokenException The
NextToken
parameter that was passed is invalid.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ResourceNotFoundException This exception is thrown when a resource is not found by the AWS Transfer
* for SFTP service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ListUsers
* @see AWS API
* Documentation
*/
default CompletableFuture listUsers(ListUsersRequest listUsersRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists the users for the server that you specify by passing the ServerId
parameter.
*
*
*
* This is a convenience which creates an instance of the {@link ListUsersRequest.Builder} avoiding the need to
* create one manually via {@link ListUsersRequest#builder()}
*
*
* @param listUsersRequest
* A {@link Consumer} that will call methods on {@link ListUsersRequest.Builder} to create a request.
* @return A Java Future containing the result of the ListUsers operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceUnavailableException The request has failed because the AWS Transfer for SFTP service is not
* available.
* - InternalServiceErrorException This exception is thrown when an error occurs in the AWS Transfer for
* SFTP service.
* - InvalidNextTokenException The
NextToken
parameter that was passed is invalid.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ResourceNotFoundException This exception is thrown when a resource is not found by the AWS Transfer
* for SFTP service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ListUsers
* @see AWS API
* Documentation
*/
default CompletableFuture listUsers(Consumer listUsersRequest) {
return listUsers(ListUsersRequest.builder().applyMutation(listUsersRequest).build());
}
/**
*
* Lists the users for the server that you specify by passing the ServerId
parameter.
*
*
*
* This is a variant of {@link #listUsers(software.amazon.awssdk.services.transfer.model.ListUsersRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.transfer.paginators.ListUsersPublisher publisher = client.listUsersPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.transfer.paginators.ListUsersPublisher publisher = client.listUsersPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.transfer.model.ListUsersResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Note: If you prefer to have control on service calls, use the
* {@link #listUsers(software.amazon.awssdk.services.transfer.model.ListUsersRequest)} operation.
*
*
* @param listUsersRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceUnavailableException The request has failed because the AWS Transfer for SFTP service is not
* available.
* - InternalServiceErrorException This exception is thrown when an error occurs in the AWS Transfer for
* SFTP service.
* - InvalidNextTokenException The
NextToken
parameter that was passed is invalid.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ResourceNotFoundException This exception is thrown when a resource is not found by the AWS Transfer
* for SFTP service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ListUsers
* @see AWS API
* Documentation
*/
default ListUsersPublisher listUsersPaginator(ListUsersRequest listUsersRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists the users for the server that you specify by passing the ServerId
parameter.
*
*
*
* This is a variant of {@link #listUsers(software.amazon.awssdk.services.transfer.model.ListUsersRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.transfer.paginators.ListUsersPublisher publisher = client.listUsersPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.transfer.paginators.ListUsersPublisher publisher = client.listUsersPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.transfer.model.ListUsersResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Note: If you prefer to have control on service calls, use the
* {@link #listUsers(software.amazon.awssdk.services.transfer.model.ListUsersRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListUsersRequest.Builder} avoiding the need to
* create one manually via {@link ListUsersRequest#builder()}
*
*
* @param listUsersRequest
* A {@link Consumer} that will call methods on {@link ListUsersRequest.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceUnavailableException The request has failed because the AWS Transfer for SFTP service is not
* available.
* - InternalServiceErrorException This exception is thrown when an error occurs in the AWS Transfer for
* SFTP service.
* - InvalidNextTokenException The
NextToken
parameter that was passed is invalid.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ResourceNotFoundException This exception is thrown when a resource is not found by the AWS Transfer
* for SFTP service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.ListUsers
* @see AWS API
* Documentation
*/
default ListUsersPublisher listUsersPaginator(Consumer listUsersRequest) {
return listUsersPaginator(ListUsersRequest.builder().applyMutation(listUsersRequest).build());
}
/**
*
* Changes the state of a Secure File Transfer Protocol (SFTP) server from OFFLINE
to
* ONLINE
. It has no impact on an SFTP server that is already ONLINE
. An
* ONLINE
server can accept and process file transfer jobs.
*
*
* The state of STARTING
indicates that the server is in an intermediate state, either not fully able
* to respond, or not fully online. The values of START_FAILED
can indicate an error condition.
*
*
* No response is returned from this call.
*
*
* @param startServerRequest
* @return A Java Future containing the result of the StartServer operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceUnavailableException The request has failed because the AWS Transfer for SFTP service is not
* available.
* - InternalServiceErrorException This exception is thrown when an error occurs in the AWS Transfer for
* SFTP service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ResourceNotFoundException This exception is thrown when a resource is not found by the AWS Transfer
* for SFTP service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.StartServer
* @see AWS API
* Documentation
*/
default CompletableFuture startServer(StartServerRequest startServerRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Changes the state of a Secure File Transfer Protocol (SFTP) server from OFFLINE
to
* ONLINE
. It has no impact on an SFTP server that is already ONLINE
. An
* ONLINE
server can accept and process file transfer jobs.
*
*
* The state of STARTING
indicates that the server is in an intermediate state, either not fully able
* to respond, or not fully online. The values of START_FAILED
can indicate an error condition.
*
*
* No response is returned from this call.
*
*
*
* This is a convenience which creates an instance of the {@link StartServerRequest.Builder} avoiding the need to
* create one manually via {@link StartServerRequest#builder()}
*
*
* @param startServerRequest
* A {@link Consumer} that will call methods on {@link StartServerRequest.Builder} to create a request.
* @return A Java Future containing the result of the StartServer operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceUnavailableException The request has failed because the AWS Transfer for SFTP service is not
* available.
* - InternalServiceErrorException This exception is thrown when an error occurs in the AWS Transfer for
* SFTP service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ResourceNotFoundException This exception is thrown when a resource is not found by the AWS Transfer
* for SFTP service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.StartServer
* @see AWS API
* Documentation
*/
default CompletableFuture startServer(Consumer startServerRequest) {
return startServer(StartServerRequest.builder().applyMutation(startServerRequest).build());
}
/**
*
* Changes the state of an SFTP server from ONLINE
to OFFLINE
. An OFFLINE
* server cannot accept and process file transfer jobs. Information tied to your server such as server and user
* properties are not affected by stopping your server. Stopping a server will not reduce or impact your Secure File
* Transfer Protocol (SFTP) endpoint billing.
*
*
* The state of STOPPING
indicates that the server is in an intermediate state, either not fully able
* to respond, or not fully offline. The values of STOP_FAILED
can indicate an error condition.
*
*
* No response is returned from this call.
*
*
* @param stopServerRequest
* @return A Java Future containing the result of the StopServer operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceUnavailableException The request has failed because the AWS Transfer for SFTP service is not
* available.
* - InternalServiceErrorException This exception is thrown when an error occurs in the AWS Transfer for
* SFTP service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ResourceNotFoundException This exception is thrown when a resource is not found by the AWS Transfer
* for SFTP service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.StopServer
* @see AWS API
* Documentation
*/
default CompletableFuture stopServer(StopServerRequest stopServerRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Changes the state of an SFTP server from ONLINE
to OFFLINE
. An OFFLINE
* server cannot accept and process file transfer jobs. Information tied to your server such as server and user
* properties are not affected by stopping your server. Stopping a server will not reduce or impact your Secure File
* Transfer Protocol (SFTP) endpoint billing.
*
*
* The state of STOPPING
indicates that the server is in an intermediate state, either not fully able
* to respond, or not fully offline. The values of STOP_FAILED
can indicate an error condition.
*
*
* No response is returned from this call.
*
*
*
* This is a convenience which creates an instance of the {@link StopServerRequest.Builder} avoiding the need to
* create one manually via {@link StopServerRequest#builder()}
*
*
* @param stopServerRequest
* A {@link Consumer} that will call methods on {@link StopServerRequest.Builder} to create a request.
* @return A Java Future containing the result of the StopServer operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceUnavailableException The request has failed because the AWS Transfer for SFTP service is not
* available.
* - InternalServiceErrorException This exception is thrown when an error occurs in the AWS Transfer for
* SFTP service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ResourceNotFoundException This exception is thrown when a resource is not found by the AWS Transfer
* for SFTP service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.StopServer
* @see AWS API
* Documentation
*/
default CompletableFuture stopServer(Consumer stopServerRequest) {
return stopServer(StopServerRequest.builder().applyMutation(stopServerRequest).build());
}
/**
*
* Attaches a key-value pair to a resource, as identified by its Amazon Resource Name (ARN). Resources are users,
* servers, roles, and other entities.
*
*
* There is no response returned from this call.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceUnavailableException The request has failed because the AWS Transfer for SFTP service is not
* available.
* - InternalServiceErrorException This exception is thrown when an error occurs in the AWS Transfer for
* SFTP service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.TagResource
* @see AWS API
* Documentation
*/
default CompletableFuture tagResource(TagResourceRequest tagResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Attaches a key-value pair to a resource, as identified by its Amazon Resource Name (ARN). Resources are users,
* servers, roles, and other entities.
*
*
* There is no response returned from this call.
*
*
*
* This is a convenience which creates an instance of the {@link TagResourceRequest.Builder} avoiding the need to
* create one manually via {@link TagResourceRequest#builder()}
*
*
* @param tagResourceRequest
* A {@link Consumer} that will call methods on {@link TagResourceRequest.Builder} to create a request.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceUnavailableException The request has failed because the AWS Transfer for SFTP service is not
* available.
* - InternalServiceErrorException This exception is thrown when an error occurs in the AWS Transfer for
* SFTP service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.TagResource
* @see AWS API
* Documentation
*/
default CompletableFuture tagResource(Consumer tagResourceRequest) {
return tagResource(TagResourceRequest.builder().applyMutation(tagResourceRequest).build());
}
/**
*
* If the IdentityProviderType
of the server is API_Gateway
, tests whether your API
* Gateway is set up successfully. We highly recommend that you call this operation to test your authentication
* method as soon as you create your server. By doing so, you can troubleshoot issues with the API Gateway
* integration to ensure that your users can successfully use the service.
*
*
* @param testIdentityProviderRequest
* @return A Java Future containing the result of the TestIdentityProvider operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceUnavailableException The request has failed because the AWS Transfer for SFTP service is not
* available.
* - InternalServiceErrorException This exception is thrown when an error occurs in the AWS Transfer for
* SFTP service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ResourceNotFoundException This exception is thrown when a resource is not found by the AWS Transfer
* for SFTP service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.TestIdentityProvider
* @see AWS
* API Documentation
*/
default CompletableFuture testIdentityProvider(
TestIdentityProviderRequest testIdentityProviderRequest) {
throw new UnsupportedOperationException();
}
/**
*
* If the IdentityProviderType
of the server is API_Gateway
, tests whether your API
* Gateway is set up successfully. We highly recommend that you call this operation to test your authentication
* method as soon as you create your server. By doing so, you can troubleshoot issues with the API Gateway
* integration to ensure that your users can successfully use the service.
*
*
*
* This is a convenience which creates an instance of the {@link TestIdentityProviderRequest.Builder} avoiding the
* need to create one manually via {@link TestIdentityProviderRequest#builder()}
*
*
* @param testIdentityProviderRequest
* A {@link Consumer} that will call methods on {@link TestIdentityProviderRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the TestIdentityProvider operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceUnavailableException The request has failed because the AWS Transfer for SFTP service is not
* available.
* - InternalServiceErrorException This exception is thrown when an error occurs in the AWS Transfer for
* SFTP service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ResourceNotFoundException This exception is thrown when a resource is not found by the AWS Transfer
* for SFTP service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.TestIdentityProvider
* @see AWS
* API Documentation
*/
default CompletableFuture testIdentityProvider(
Consumer testIdentityProviderRequest) {
return testIdentityProvider(TestIdentityProviderRequest.builder().applyMutation(testIdentityProviderRequest).build());
}
/**
*
* Detaches a key-value pair from a resource, as identified by its Amazon Resource Name (ARN). Resources are users,
* servers, roles, and other entities.
*
*
* No response is returned from this call.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceUnavailableException The request has failed because the AWS Transfer for SFTP service is not
* available.
* - InternalServiceErrorException This exception is thrown when an error occurs in the AWS Transfer for
* SFTP service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.UntagResource
* @see AWS API
* Documentation
*/
default CompletableFuture untagResource(UntagResourceRequest untagResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Detaches a key-value pair from a resource, as identified by its Amazon Resource Name (ARN). Resources are users,
* servers, roles, and other entities.
*
*
* No response is returned from this call.
*
*
*
* This is a convenience which creates an instance of the {@link UntagResourceRequest.Builder} avoiding the need to
* create one manually via {@link UntagResourceRequest#builder()}
*
*
* @param untagResourceRequest
* A {@link Consumer} that will call methods on {@link UntagResourceRequest.Builder} to create a request.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceUnavailableException The request has failed because the AWS Transfer for SFTP service is not
* available.
* - InternalServiceErrorException This exception is thrown when an error occurs in the AWS Transfer for
* SFTP service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.UntagResource
* @see AWS API
* Documentation
*/
default CompletableFuture untagResource(Consumer untagResourceRequest) {
return untagResource(UntagResourceRequest.builder().applyMutation(untagResourceRequest).build());
}
/**
*
* Updates the server properties after that server has been created.
*
*
* The UpdateServer
call returns the ServerId
of the Secure File Transfer Protocol (SFTP)
* server you updated.
*
*
* @param updateServerRequest
* @return A Java Future containing the result of the UpdateServer operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceUnavailableException The request has failed because the AWS Transfer for SFTP service is not
* available.
* - InternalServiceErrorException This exception is thrown when an error occurs in the AWS Transfer for
* SFTP service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ResourceNotFoundException This exception is thrown when a resource is not found by the AWS Transfer
* for SFTP service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.UpdateServer
* @see AWS API
* Documentation
*/
default CompletableFuture updateServer(UpdateServerRequest updateServerRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates the server properties after that server has been created.
*
*
* The UpdateServer
call returns the ServerId
of the Secure File Transfer Protocol (SFTP)
* server you updated.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateServerRequest.Builder} avoiding the need to
* create one manually via {@link UpdateServerRequest#builder()}
*
*
* @param updateServerRequest
* A {@link Consumer} that will call methods on {@link UpdateServerRequest.Builder} to create a request.
* @return A Java Future containing the result of the UpdateServer operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceUnavailableException The request has failed because the AWS Transfer for SFTP service is not
* available.
* - InternalServiceErrorException This exception is thrown when an error occurs in the AWS Transfer for
* SFTP service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ResourceNotFoundException This exception is thrown when a resource is not found by the AWS Transfer
* for SFTP service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.UpdateServer
* @see AWS API
* Documentation
*/
default CompletableFuture updateServer(Consumer updateServerRequest) {
return updateServer(UpdateServerRequest.builder().applyMutation(updateServerRequest).build());
}
/**
*
* Assigns new properties to a user. Parameters you pass modify any or all of the following: the home directory,
* role, and policy for the UserName
and ServerId
you specify.
*
*
* The response returns the ServerId
and the UserName
for the updated user.
*
*
* @param updateUserRequest
* @return A Java Future containing the result of the UpdateUser operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceUnavailableException The request has failed because the AWS Transfer for SFTP service is not
* available.
* - InternalServiceErrorException This exception is thrown when an error occurs in the AWS Transfer for
* SFTP service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ResourceNotFoundException This exception is thrown when a resource is not found by the AWS Transfer
* for SFTP service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.UpdateUser
* @see AWS API
* Documentation
*/
default CompletableFuture updateUser(UpdateUserRequest updateUserRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Assigns new properties to a user. Parameters you pass modify any or all of the following: the home directory,
* role, and policy for the UserName
and ServerId
you specify.
*
*
* The response returns the ServerId
and the UserName
for the updated user.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateUserRequest.Builder} avoiding the need to
* create one manually via {@link UpdateUserRequest#builder()}
*
*
* @param updateUserRequest
* A {@link Consumer} that will call methods on {@link UpdateUserRequest.Builder} to create a request.
* @return A Java Future containing the result of the UpdateUser operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceUnavailableException The request has failed because the AWS Transfer for SFTP service is not
* available.
* - InternalServiceErrorException This exception is thrown when an error occurs in the AWS Transfer for
* SFTP service.
* - InvalidRequestException This exception is thrown when the client submits a malformed request.
* - ResourceNotFoundException This exception is thrown when a resource is not found by the AWS Transfer
* for SFTP service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TransferException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TransferAsyncClient.UpdateUser
* @see AWS API
* Documentation
*/
default CompletableFuture updateUser(Consumer updateUserRequest) {
return updateUser(UpdateUserRequest.builder().applyMutation(updateUserRequest).build());
}
}