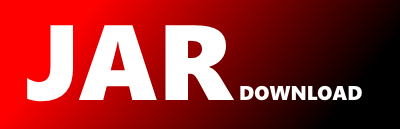
software.amazon.awssdk.services.translate.TranslateAsyncClient Maven / Gradle / Ivy
Show all versions of translate Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.translate;
import java.util.concurrent.CompletableFuture;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkPublicApi;
import software.amazon.awssdk.annotations.ThreadSafe;
import software.amazon.awssdk.awscore.AwsClient;
import software.amazon.awssdk.services.translate.model.CreateParallelDataRequest;
import software.amazon.awssdk.services.translate.model.CreateParallelDataResponse;
import software.amazon.awssdk.services.translate.model.DeleteParallelDataRequest;
import software.amazon.awssdk.services.translate.model.DeleteParallelDataResponse;
import software.amazon.awssdk.services.translate.model.DeleteTerminologyRequest;
import software.amazon.awssdk.services.translate.model.DeleteTerminologyResponse;
import software.amazon.awssdk.services.translate.model.DescribeTextTranslationJobRequest;
import software.amazon.awssdk.services.translate.model.DescribeTextTranslationJobResponse;
import software.amazon.awssdk.services.translate.model.GetParallelDataRequest;
import software.amazon.awssdk.services.translate.model.GetParallelDataResponse;
import software.amazon.awssdk.services.translate.model.GetTerminologyRequest;
import software.amazon.awssdk.services.translate.model.GetTerminologyResponse;
import software.amazon.awssdk.services.translate.model.ImportTerminologyRequest;
import software.amazon.awssdk.services.translate.model.ImportTerminologyResponse;
import software.amazon.awssdk.services.translate.model.ListLanguagesRequest;
import software.amazon.awssdk.services.translate.model.ListLanguagesResponse;
import software.amazon.awssdk.services.translate.model.ListParallelDataRequest;
import software.amazon.awssdk.services.translate.model.ListParallelDataResponse;
import software.amazon.awssdk.services.translate.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.translate.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.translate.model.ListTerminologiesRequest;
import software.amazon.awssdk.services.translate.model.ListTerminologiesResponse;
import software.amazon.awssdk.services.translate.model.ListTextTranslationJobsRequest;
import software.amazon.awssdk.services.translate.model.ListTextTranslationJobsResponse;
import software.amazon.awssdk.services.translate.model.StartTextTranslationJobRequest;
import software.amazon.awssdk.services.translate.model.StartTextTranslationJobResponse;
import software.amazon.awssdk.services.translate.model.StopTextTranslationJobRequest;
import software.amazon.awssdk.services.translate.model.StopTextTranslationJobResponse;
import software.amazon.awssdk.services.translate.model.TagResourceRequest;
import software.amazon.awssdk.services.translate.model.TagResourceResponse;
import software.amazon.awssdk.services.translate.model.TranslateDocumentRequest;
import software.amazon.awssdk.services.translate.model.TranslateDocumentResponse;
import software.amazon.awssdk.services.translate.model.TranslateTextRequest;
import software.amazon.awssdk.services.translate.model.TranslateTextResponse;
import software.amazon.awssdk.services.translate.model.UntagResourceRequest;
import software.amazon.awssdk.services.translate.model.UntagResourceResponse;
import software.amazon.awssdk.services.translate.model.UpdateParallelDataRequest;
import software.amazon.awssdk.services.translate.model.UpdateParallelDataResponse;
import software.amazon.awssdk.services.translate.paginators.ListLanguagesPublisher;
import software.amazon.awssdk.services.translate.paginators.ListParallelDataPublisher;
import software.amazon.awssdk.services.translate.paginators.ListTerminologiesPublisher;
import software.amazon.awssdk.services.translate.paginators.ListTextTranslationJobsPublisher;
/**
* Service client for accessing Amazon Translate asynchronously. This can be created using the static {@link #builder()}
* method.The asynchronous client performs non-blocking I/O when configured with any {@code SdkAsyncHttpClient}
* supported in the SDK. However, full non-blocking is not guaranteed as the async client may perform blocking calls in
* some cases such as credentials retrieval and endpoint discovery as part of the async API call.
*
*
* Provides translation of the input content from the source language to the target language.
*
*/
@Generated("software.amazon.awssdk:codegen")
@SdkPublicApi
@ThreadSafe
public interface TranslateAsyncClient extends AwsClient {
String SERVICE_NAME = "translate";
/**
* Value for looking up the service's metadata from the
* {@link software.amazon.awssdk.regions.ServiceMetadataProvider}.
*/
String SERVICE_METADATA_ID = "translate";
/**
*
* Creates a parallel data resource in Amazon Translate by importing an input file from Amazon S3. Parallel data
* files contain examples that show how you want segments of text to be translated. By adding parallel data, you can
* influence the style, tone, and word choice in your translation output.
*
*
* @param createParallelDataRequest
* @return A Java Future containing the result of the CreateParallelData operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidParameterValueException The value of the parameter is not valid. Review the value of the
* parameter you are using to correct it, and then retry your operation.
* - InvalidRequestException The request that you made is not valid. Check your request to determine why
* it's not valid and then retry the request.
* - LimitExceededException The specified limit has been exceeded. Review your request and retry it with a
* quantity below the stated limit.
* - TooManyRequestsException You have made too many requests within a short period of time. Wait for a
* short time and then try your request again.
* - TooManyTagsException You have added too many tags to this resource. The maximum is 50 tags.
* - ConflictException There was a conflict processing the request. Try your request again.
* - ConcurrentModificationException Another modification is being made. That modification must complete
* before you can make your change.
* - InternalServerException An internal server error occurred. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranslateException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranslateAsyncClient.CreateParallelData
* @see AWS
* API Documentation
*/
default CompletableFuture createParallelData(CreateParallelDataRequest createParallelDataRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a parallel data resource in Amazon Translate by importing an input file from Amazon S3. Parallel data
* files contain examples that show how you want segments of text to be translated. By adding parallel data, you can
* influence the style, tone, and word choice in your translation output.
*
*
*
* This is a convenience which creates an instance of the {@link CreateParallelDataRequest.Builder} avoiding the
* need to create one manually via {@link CreateParallelDataRequest#builder()}
*
*
* @param createParallelDataRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.translate.model.CreateParallelDataRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the CreateParallelData operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidParameterValueException The value of the parameter is not valid. Review the value of the
* parameter you are using to correct it, and then retry your operation.
* - InvalidRequestException The request that you made is not valid. Check your request to determine why
* it's not valid and then retry the request.
* - LimitExceededException The specified limit has been exceeded. Review your request and retry it with a
* quantity below the stated limit.
* - TooManyRequestsException You have made too many requests within a short period of time. Wait for a
* short time and then try your request again.
* - TooManyTagsException You have added too many tags to this resource. The maximum is 50 tags.
* - ConflictException There was a conflict processing the request. Try your request again.
* - ConcurrentModificationException Another modification is being made. That modification must complete
* before you can make your change.
* - InternalServerException An internal server error occurred. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranslateException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranslateAsyncClient.CreateParallelData
* @see AWS
* API Documentation
*/
default CompletableFuture createParallelData(
Consumer createParallelDataRequest) {
return createParallelData(CreateParallelDataRequest.builder().applyMutation(createParallelDataRequest).build());
}
/**
*
* Deletes a parallel data resource in Amazon Translate.
*
*
* @param deleteParallelDataRequest
* @return A Java Future containing the result of the DeleteParallelData operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ConcurrentModificationException Another modification is being made. That modification must complete
* before you can make your change.
* - ResourceNotFoundException The resource you are looking for has not been found. Review the resource
* you're looking for and see if a different resource will accomplish your needs before retrying the revised
* request.
* - TooManyRequestsException You have made too many requests within a short period of time. Wait for a
* short time and then try your request again.
* - InternalServerException An internal server error occurred. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranslateException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranslateAsyncClient.DeleteParallelData
* @see AWS
* API Documentation
*/
default CompletableFuture deleteParallelData(DeleteParallelDataRequest deleteParallelDataRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a parallel data resource in Amazon Translate.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteParallelDataRequest.Builder} avoiding the
* need to create one manually via {@link DeleteParallelDataRequest#builder()}
*
*
* @param deleteParallelDataRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.translate.model.DeleteParallelDataRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DeleteParallelData operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ConcurrentModificationException Another modification is being made. That modification must complete
* before you can make your change.
* - ResourceNotFoundException The resource you are looking for has not been found. Review the resource
* you're looking for and see if a different resource will accomplish your needs before retrying the revised
* request.
* - TooManyRequestsException You have made too many requests within a short period of time. Wait for a
* short time and then try your request again.
* - InternalServerException An internal server error occurred. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranslateException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranslateAsyncClient.DeleteParallelData
* @see AWS
* API Documentation
*/
default CompletableFuture deleteParallelData(
Consumer deleteParallelDataRequest) {
return deleteParallelData(DeleteParallelDataRequest.builder().applyMutation(deleteParallelDataRequest).build());
}
/**
*
* A synchronous action that deletes a custom terminology.
*
*
* @param deleteTerminologyRequest
* @return A Java Future containing the result of the DeleteTerminology operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException The resource you are looking for has not been found. Review the resource
* you're looking for and see if a different resource will accomplish your needs before retrying the revised
* request.
* - TooManyRequestsException You have made too many requests within a short period of time. Wait for a
* short time and then try your request again.
* - InvalidParameterValueException The value of the parameter is not valid. Review the value of the
* parameter you are using to correct it, and then retry your operation.
* - InternalServerException An internal server error occurred. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranslateException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranslateAsyncClient.DeleteTerminology
* @see AWS
* API Documentation
*/
default CompletableFuture deleteTerminology(DeleteTerminologyRequest deleteTerminologyRequest) {
throw new UnsupportedOperationException();
}
/**
*
* A synchronous action that deletes a custom terminology.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteTerminologyRequest.Builder} avoiding the need
* to create one manually via {@link DeleteTerminologyRequest#builder()}
*
*
* @param deleteTerminologyRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.translate.model.DeleteTerminologyRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DeleteTerminology operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException The resource you are looking for has not been found. Review the resource
* you're looking for and see if a different resource will accomplish your needs before retrying the revised
* request.
* - TooManyRequestsException You have made too many requests within a short period of time. Wait for a
* short time and then try your request again.
* - InvalidParameterValueException The value of the parameter is not valid. Review the value of the
* parameter you are using to correct it, and then retry your operation.
* - InternalServerException An internal server error occurred. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranslateException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranslateAsyncClient.DeleteTerminology
* @see AWS
* API Documentation
*/
default CompletableFuture deleteTerminology(
Consumer deleteTerminologyRequest) {
return deleteTerminology(DeleteTerminologyRequest.builder().applyMutation(deleteTerminologyRequest).build());
}
/**
*
* Gets the properties associated with an asynchronous batch translation job including name, ID, status, source and
* target languages, input/output S3 buckets, and so on.
*
*
* @param describeTextTranslationJobRequest
* @return A Java Future containing the result of the DescribeTextTranslationJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException The resource you are looking for has not been found. Review the resource
* you're looking for and see if a different resource will accomplish your needs before retrying the revised
* request.
* - TooManyRequestsException You have made too many requests within a short period of time. Wait for a
* short time and then try your request again.
* - InternalServerException An internal server error occurred. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranslateException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranslateAsyncClient.DescribeTextTranslationJob
* @see AWS API Documentation
*/
default CompletableFuture describeTextTranslationJob(
DescribeTextTranslationJobRequest describeTextTranslationJobRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets the properties associated with an asynchronous batch translation job including name, ID, status, source and
* target languages, input/output S3 buckets, and so on.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeTextTranslationJobRequest.Builder} avoiding
* the need to create one manually via {@link DescribeTextTranslationJobRequest#builder()}
*
*
* @param describeTextTranslationJobRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.translate.model.DescribeTextTranslationJobRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the DescribeTextTranslationJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException The resource you are looking for has not been found. Review the resource
* you're looking for and see if a different resource will accomplish your needs before retrying the revised
* request.
* - TooManyRequestsException You have made too many requests within a short period of time. Wait for a
* short time and then try your request again.
* - InternalServerException An internal server error occurred. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranslateException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranslateAsyncClient.DescribeTextTranslationJob
* @see AWS API Documentation
*/
default CompletableFuture describeTextTranslationJob(
Consumer describeTextTranslationJobRequest) {
return describeTextTranslationJob(DescribeTextTranslationJobRequest.builder()
.applyMutation(describeTextTranslationJobRequest).build());
}
/**
*
* Provides information about a parallel data resource.
*
*
* @param getParallelDataRequest
* @return A Java Future containing the result of the GetParallelData operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException The resource you are looking for has not been found. Review the resource
* you're looking for and see if a different resource will accomplish your needs before retrying the revised
* request.
* - InvalidParameterValueException The value of the parameter is not valid. Review the value of the
* parameter you are using to correct it, and then retry your operation.
* - TooManyRequestsException You have made too many requests within a short period of time. Wait for a
* short time and then try your request again.
* - InternalServerException An internal server error occurred. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranslateException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranslateAsyncClient.GetParallelData
* @see AWS API
* Documentation
*/
default CompletableFuture getParallelData(GetParallelDataRequest getParallelDataRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Provides information about a parallel data resource.
*
*
*
* This is a convenience which creates an instance of the {@link GetParallelDataRequest.Builder} avoiding the need
* to create one manually via {@link GetParallelDataRequest#builder()}
*
*
* @param getParallelDataRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.translate.model.GetParallelDataRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetParallelData operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException The resource you are looking for has not been found. Review the resource
* you're looking for and see if a different resource will accomplish your needs before retrying the revised
* request.
* - InvalidParameterValueException The value of the parameter is not valid. Review the value of the
* parameter you are using to correct it, and then retry your operation.
* - TooManyRequestsException You have made too many requests within a short period of time. Wait for a
* short time and then try your request again.
* - InternalServerException An internal server error occurred. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranslateException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranslateAsyncClient.GetParallelData
* @see AWS API
* Documentation
*/
default CompletableFuture getParallelData(
Consumer getParallelDataRequest) {
return getParallelData(GetParallelDataRequest.builder().applyMutation(getParallelDataRequest).build());
}
/**
*
* Retrieves a custom terminology.
*
*
* @param getTerminologyRequest
* @return A Java Future containing the result of the GetTerminology operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException The resource you are looking for has not been found. Review the resource
* you're looking for and see if a different resource will accomplish your needs before retrying the revised
* request.
* - InvalidParameterValueException The value of the parameter is not valid. Review the value of the
* parameter you are using to correct it, and then retry your operation.
* - TooManyRequestsException You have made too many requests within a short period of time. Wait for a
* short time and then try your request again.
* - InternalServerException An internal server error occurred. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranslateException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranslateAsyncClient.GetTerminology
* @see AWS API
* Documentation
*/
default CompletableFuture getTerminology(GetTerminologyRequest getTerminologyRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves a custom terminology.
*
*
*
* This is a convenience which creates an instance of the {@link GetTerminologyRequest.Builder} avoiding the need to
* create one manually via {@link GetTerminologyRequest#builder()}
*
*
* @param getTerminologyRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.translate.model.GetTerminologyRequest.Builder} to create a request.
* @return A Java Future containing the result of the GetTerminology operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException The resource you are looking for has not been found. Review the resource
* you're looking for and see if a different resource will accomplish your needs before retrying the revised
* request.
* - InvalidParameterValueException The value of the parameter is not valid. Review the value of the
* parameter you are using to correct it, and then retry your operation.
* - TooManyRequestsException You have made too many requests within a short period of time. Wait for a
* short time and then try your request again.
* - InternalServerException An internal server error occurred. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranslateException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranslateAsyncClient.GetTerminology
* @see AWS API
* Documentation
*/
default CompletableFuture getTerminology(Consumer getTerminologyRequest) {
return getTerminology(GetTerminologyRequest.builder().applyMutation(getTerminologyRequest).build());
}
/**
*
* Creates or updates a custom terminology, depending on whether one already exists for the given terminology name.
* Importing a terminology with the same name as an existing one will merge the terminologies based on the chosen
* merge strategy. The only supported merge strategy is OVERWRITE, where the imported terminology overwrites the
* existing terminology of the same name.
*
*
* If you import a terminology that overwrites an existing one, the new terminology takes up to 10 minutes to fully
* propagate. After that, translations have access to the new terminology.
*
*
* @param importTerminologyRequest
* @return A Java Future containing the result of the ImportTerminology operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidParameterValueException The value of the parameter is not valid. Review the value of the
* parameter you are using to correct it, and then retry your operation.
* - LimitExceededException The specified limit has been exceeded. Review your request and retry it with a
* quantity below the stated limit.
* - TooManyRequestsException You have made too many requests within a short period of time. Wait for a
* short time and then try your request again.
* - TooManyTagsException You have added too many tags to this resource. The maximum is 50 tags.
* - ConcurrentModificationException Another modification is being made. That modification must complete
* before you can make your change.
* - InternalServerException An internal server error occurred. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranslateException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranslateAsyncClient.ImportTerminology
* @see AWS
* API Documentation
*/
default CompletableFuture importTerminology(ImportTerminologyRequest importTerminologyRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates or updates a custom terminology, depending on whether one already exists for the given terminology name.
* Importing a terminology with the same name as an existing one will merge the terminologies based on the chosen
* merge strategy. The only supported merge strategy is OVERWRITE, where the imported terminology overwrites the
* existing terminology of the same name.
*
*
* If you import a terminology that overwrites an existing one, the new terminology takes up to 10 minutes to fully
* propagate. After that, translations have access to the new terminology.
*
*
*
* This is a convenience which creates an instance of the {@link ImportTerminologyRequest.Builder} avoiding the need
* to create one manually via {@link ImportTerminologyRequest#builder()}
*
*
* @param importTerminologyRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.translate.model.ImportTerminologyRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ImportTerminology operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidParameterValueException The value of the parameter is not valid. Review the value of the
* parameter you are using to correct it, and then retry your operation.
* - LimitExceededException The specified limit has been exceeded. Review your request and retry it with a
* quantity below the stated limit.
* - TooManyRequestsException You have made too many requests within a short period of time. Wait for a
* short time and then try your request again.
* - TooManyTagsException You have added too many tags to this resource. The maximum is 50 tags.
* - ConcurrentModificationException Another modification is being made. That modification must complete
* before you can make your change.
* - InternalServerException An internal server error occurred. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranslateException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranslateAsyncClient.ImportTerminology
* @see AWS
* API Documentation
*/
default CompletableFuture importTerminology(
Consumer importTerminologyRequest) {
return importTerminology(ImportTerminologyRequest.builder().applyMutation(importTerminologyRequest).build());
}
/**
*
* Provides a list of languages (RFC-5646 codes and names) that Amazon Translate supports.
*
*
* @param listLanguagesRequest
* @return A Java Future containing the result of the ListLanguages operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidParameterValueException The value of the parameter is not valid. Review the value of the
* parameter you are using to correct it, and then retry your operation.
* - TooManyRequestsException You have made too many requests within a short period of time. Wait for a
* short time and then try your request again.
* - UnsupportedDisplayLanguageCodeException Requested display language code is not supported.
* - InternalServerException An internal server error occurred. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranslateException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranslateAsyncClient.ListLanguages
* @see AWS API
* Documentation
*/
default CompletableFuture listLanguages(ListLanguagesRequest listLanguagesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Provides a list of languages (RFC-5646 codes and names) that Amazon Translate supports.
*
*
*
* This is a convenience which creates an instance of the {@link ListLanguagesRequest.Builder} avoiding the need to
* create one manually via {@link ListLanguagesRequest#builder()}
*
*
* @param listLanguagesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.translate.model.ListLanguagesRequest.Builder} to create a request.
* @return A Java Future containing the result of the ListLanguages operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidParameterValueException The value of the parameter is not valid. Review the value of the
* parameter you are using to correct it, and then retry your operation.
* - TooManyRequestsException You have made too many requests within a short period of time. Wait for a
* short time and then try your request again.
* - UnsupportedDisplayLanguageCodeException Requested display language code is not supported.
* - InternalServerException An internal server error occurred. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranslateException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranslateAsyncClient.ListLanguages
* @see AWS API
* Documentation
*/
default CompletableFuture listLanguages(Consumer listLanguagesRequest) {
return listLanguages(ListLanguagesRequest.builder().applyMutation(listLanguagesRequest).build());
}
/**
*
* This is a variant of {@link #listLanguages(software.amazon.awssdk.services.translate.model.ListLanguagesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.translate.paginators.ListLanguagesPublisher publisher = client.listLanguagesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.translate.paginators.ListLanguagesPublisher publisher = client.listLanguagesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.translate.model.ListLanguagesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listLanguages(software.amazon.awssdk.services.translate.model.ListLanguagesRequest)} operation.
*
*
* @param listLanguagesRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidParameterValueException The value of the parameter is not valid. Review the value of the
* parameter you are using to correct it, and then retry your operation.
* - TooManyRequestsException You have made too many requests within a short period of time. Wait for a
* short time and then try your request again.
* - UnsupportedDisplayLanguageCodeException Requested display language code is not supported.
* - InternalServerException An internal server error occurred. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranslateException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranslateAsyncClient.ListLanguages
* @see AWS API
* Documentation
*/
default ListLanguagesPublisher listLanguagesPaginator(ListLanguagesRequest listLanguagesRequest) {
return new ListLanguagesPublisher(this, listLanguagesRequest);
}
/**
*
* This is a variant of {@link #listLanguages(software.amazon.awssdk.services.translate.model.ListLanguagesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.translate.paginators.ListLanguagesPublisher publisher = client.listLanguagesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.translate.paginators.ListLanguagesPublisher publisher = client.listLanguagesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.translate.model.ListLanguagesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listLanguages(software.amazon.awssdk.services.translate.model.ListLanguagesRequest)} operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListLanguagesRequest.Builder} avoiding the need to
* create one manually via {@link ListLanguagesRequest#builder()}
*
*
* @param listLanguagesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.translate.model.ListLanguagesRequest.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidParameterValueException The value of the parameter is not valid. Review the value of the
* parameter you are using to correct it, and then retry your operation.
* - TooManyRequestsException You have made too many requests within a short period of time. Wait for a
* short time and then try your request again.
* - UnsupportedDisplayLanguageCodeException Requested display language code is not supported.
* - InternalServerException An internal server error occurred. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranslateException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranslateAsyncClient.ListLanguages
* @see AWS API
* Documentation
*/
default ListLanguagesPublisher listLanguagesPaginator(Consumer listLanguagesRequest) {
return listLanguagesPaginator(ListLanguagesRequest.builder().applyMutation(listLanguagesRequest).build());
}
/**
*
* Provides a list of your parallel data resources in Amazon Translate.
*
*
* @param listParallelDataRequest
* @return A Java Future containing the result of the ListParallelData operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidParameterValueException The value of the parameter is not valid. Review the value of the
* parameter you are using to correct it, and then retry your operation.
* - TooManyRequestsException You have made too many requests within a short period of time. Wait for a
* short time and then try your request again.
* - InternalServerException An internal server error occurred. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranslateException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranslateAsyncClient.ListParallelData
* @see AWS
* API Documentation
*/
default CompletableFuture listParallelData(ListParallelDataRequest listParallelDataRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Provides a list of your parallel data resources in Amazon Translate.
*
*
*
* This is a convenience which creates an instance of the {@link ListParallelDataRequest.Builder} avoiding the need
* to create one manually via {@link ListParallelDataRequest#builder()}
*
*
* @param listParallelDataRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.translate.model.ListParallelDataRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListParallelData operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidParameterValueException The value of the parameter is not valid. Review the value of the
* parameter you are using to correct it, and then retry your operation.
* - TooManyRequestsException You have made too many requests within a short period of time. Wait for a
* short time and then try your request again.
* - InternalServerException An internal server error occurred. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranslateException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranslateAsyncClient.ListParallelData
* @see AWS
* API Documentation
*/
default CompletableFuture listParallelData(
Consumer listParallelDataRequest) {
return listParallelData(ListParallelDataRequest.builder().applyMutation(listParallelDataRequest).build());
}
/**
*
* This is a variant of
* {@link #listParallelData(software.amazon.awssdk.services.translate.model.ListParallelDataRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.translate.paginators.ListParallelDataPublisher publisher = client.listParallelDataPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.translate.paginators.ListParallelDataPublisher publisher = client.listParallelDataPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.translate.model.ListParallelDataResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listParallelData(software.amazon.awssdk.services.translate.model.ListParallelDataRequest)} operation.
*
*
* @param listParallelDataRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidParameterValueException The value of the parameter is not valid. Review the value of the
* parameter you are using to correct it, and then retry your operation.
* - TooManyRequestsException You have made too many requests within a short period of time. Wait for a
* short time and then try your request again.
* - InternalServerException An internal server error occurred. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranslateException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranslateAsyncClient.ListParallelData
* @see AWS
* API Documentation
*/
default ListParallelDataPublisher listParallelDataPaginator(ListParallelDataRequest listParallelDataRequest) {
return new ListParallelDataPublisher(this, listParallelDataRequest);
}
/**
*
* This is a variant of
* {@link #listParallelData(software.amazon.awssdk.services.translate.model.ListParallelDataRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.translate.paginators.ListParallelDataPublisher publisher = client.listParallelDataPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.translate.paginators.ListParallelDataPublisher publisher = client.listParallelDataPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.translate.model.ListParallelDataResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listParallelData(software.amazon.awssdk.services.translate.model.ListParallelDataRequest)} operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListParallelDataRequest.Builder} avoiding the need
* to create one manually via {@link ListParallelDataRequest#builder()}
*
*
* @param listParallelDataRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.translate.model.ListParallelDataRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidParameterValueException The value of the parameter is not valid. Review the value of the
* parameter you are using to correct it, and then retry your operation.
* - TooManyRequestsException You have made too many requests within a short period of time. Wait for a
* short time and then try your request again.
* - InternalServerException An internal server error occurred. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranslateException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranslateAsyncClient.ListParallelData
* @see AWS
* API Documentation
*/
default ListParallelDataPublisher listParallelDataPaginator(Consumer listParallelDataRequest) {
return listParallelDataPaginator(ListParallelDataRequest.builder().applyMutation(listParallelDataRequest).build());
}
/**
*
* Lists all tags associated with a given Amazon Translate resource. For more information, see Tagging your resources.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidParameterValueException The value of the parameter is not valid. Review the value of the
* parameter you are using to correct it, and then retry your operation.
* - ResourceNotFoundException The resource you are looking for has not been found. Review the resource
* you're looking for and see if a different resource will accomplish your needs before retrying the revised
* request.
* - InternalServerException An internal server error occurred. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranslateException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranslateAsyncClient.ListTagsForResource
* @see AWS
* API Documentation
*/
default CompletableFuture listTagsForResource(
ListTagsForResourceRequest listTagsForResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists all tags associated with a given Amazon Translate resource. For more information, see Tagging your resources.
*
*
*
* This is a convenience which creates an instance of the {@link ListTagsForResourceRequest.Builder} avoiding the
* need to create one manually via {@link ListTagsForResourceRequest#builder()}
*
*
* @param listTagsForResourceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.translate.model.ListTagsForResourceRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidParameterValueException The value of the parameter is not valid. Review the value of the
* parameter you are using to correct it, and then retry your operation.
* - ResourceNotFoundException The resource you are looking for has not been found. Review the resource
* you're looking for and see if a different resource will accomplish your needs before retrying the revised
* request.
* - InternalServerException An internal server error occurred. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranslateException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranslateAsyncClient.ListTagsForResource
* @see AWS
* API Documentation
*/
default CompletableFuture listTagsForResource(
Consumer listTagsForResourceRequest) {
return listTagsForResource(ListTagsForResourceRequest.builder().applyMutation(listTagsForResourceRequest).build());
}
/**
*
* Provides a list of custom terminologies associated with your account.
*
*
* @param listTerminologiesRequest
* @return A Java Future containing the result of the ListTerminologies operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidParameterValueException The value of the parameter is not valid. Review the value of the
* parameter you are using to correct it, and then retry your operation.
* - TooManyRequestsException You have made too many requests within a short period of time. Wait for a
* short time and then try your request again.
* - InternalServerException An internal server error occurred. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranslateException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranslateAsyncClient.ListTerminologies
* @see AWS
* API Documentation
*/
default CompletableFuture listTerminologies(ListTerminologiesRequest listTerminologiesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Provides a list of custom terminologies associated with your account.
*
*
*
* This is a convenience which creates an instance of the {@link ListTerminologiesRequest.Builder} avoiding the need
* to create one manually via {@link ListTerminologiesRequest#builder()}
*
*
* @param listTerminologiesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.translate.model.ListTerminologiesRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListTerminologies operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidParameterValueException The value of the parameter is not valid. Review the value of the
* parameter you are using to correct it, and then retry your operation.
* - TooManyRequestsException You have made too many requests within a short period of time. Wait for a
* short time and then try your request again.
* - InternalServerException An internal server error occurred. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranslateException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranslateAsyncClient.ListTerminologies
* @see AWS
* API Documentation
*/
default CompletableFuture listTerminologies(
Consumer listTerminologiesRequest) {
return listTerminologies(ListTerminologiesRequest.builder().applyMutation(listTerminologiesRequest).build());
}
/**
*
* Provides a list of custom terminologies associated with your account.
*
*
* @return A Java Future containing the result of the ListTerminologies operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidParameterValueException The value of the parameter is not valid. Review the value of the
* parameter you are using to correct it, and then retry your operation.
* - TooManyRequestsException You have made too many requests within a short period of time. Wait for a
* short time and then try your request again.
* - InternalServerException An internal server error occurred. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranslateException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranslateAsyncClient.ListTerminologies
* @see AWS
* API Documentation
*/
default CompletableFuture listTerminologies() {
return listTerminologies(ListTerminologiesRequest.builder().build());
}
/**
*
* This is a variant of
* {@link #listTerminologies(software.amazon.awssdk.services.translate.model.ListTerminologiesRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.translate.paginators.ListTerminologiesPublisher publisher = client.listTerminologiesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.translate.paginators.ListTerminologiesPublisher publisher = client.listTerminologiesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.translate.model.ListTerminologiesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listTerminologies(software.amazon.awssdk.services.translate.model.ListTerminologiesRequest)}
* operation.
*
*
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidParameterValueException The value of the parameter is not valid. Review the value of the
* parameter you are using to correct it, and then retry your operation.
* - TooManyRequestsException You have made too many requests within a short period of time. Wait for a
* short time and then try your request again.
* - InternalServerException An internal server error occurred. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranslateException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranslateAsyncClient.ListTerminologies
* @see AWS
* API Documentation
*/
default ListTerminologiesPublisher listTerminologiesPaginator() {
return listTerminologiesPaginator(ListTerminologiesRequest.builder().build());
}
/**
*
* This is a variant of
* {@link #listTerminologies(software.amazon.awssdk.services.translate.model.ListTerminologiesRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.translate.paginators.ListTerminologiesPublisher publisher = client.listTerminologiesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.translate.paginators.ListTerminologiesPublisher publisher = client.listTerminologiesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.translate.model.ListTerminologiesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listTerminologies(software.amazon.awssdk.services.translate.model.ListTerminologiesRequest)}
* operation.
*
*
* @param listTerminologiesRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidParameterValueException The value of the parameter is not valid. Review the value of the
* parameter you are using to correct it, and then retry your operation.
* - TooManyRequestsException You have made too many requests within a short period of time. Wait for a
* short time and then try your request again.
* - InternalServerException An internal server error occurred. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranslateException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranslateAsyncClient.ListTerminologies
* @see AWS
* API Documentation
*/
default ListTerminologiesPublisher listTerminologiesPaginator(ListTerminologiesRequest listTerminologiesRequest) {
return new ListTerminologiesPublisher(this, listTerminologiesRequest);
}
/**
*
* This is a variant of
* {@link #listTerminologies(software.amazon.awssdk.services.translate.model.ListTerminologiesRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.translate.paginators.ListTerminologiesPublisher publisher = client.listTerminologiesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.translate.paginators.ListTerminologiesPublisher publisher = client.listTerminologiesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.translate.model.ListTerminologiesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listTerminologies(software.amazon.awssdk.services.translate.model.ListTerminologiesRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListTerminologiesRequest.Builder} avoiding the need
* to create one manually via {@link ListTerminologiesRequest#builder()}
*
*
* @param listTerminologiesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.translate.model.ListTerminologiesRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidParameterValueException The value of the parameter is not valid. Review the value of the
* parameter you are using to correct it, and then retry your operation.
* - TooManyRequestsException You have made too many requests within a short period of time. Wait for a
* short time and then try your request again.
* - InternalServerException An internal server error occurred. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranslateException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranslateAsyncClient.ListTerminologies
* @see AWS
* API Documentation
*/
default ListTerminologiesPublisher listTerminologiesPaginator(
Consumer listTerminologiesRequest) {
return listTerminologiesPaginator(ListTerminologiesRequest.builder().applyMutation(listTerminologiesRequest).build());
}
/**
*
* Gets a list of the batch translation jobs that you have submitted.
*
*
* @param listTextTranslationJobsRequest
* @return A Java Future containing the result of the ListTextTranslationJobs operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidRequestException The request that you made is not valid. Check your request to determine why
* it's not valid and then retry the request.
* - TooManyRequestsException You have made too many requests within a short period of time. Wait for a
* short time and then try your request again.
* - InvalidFilterException The filter specified for the operation is not valid. Specify a different
* filter.
* - InternalServerException An internal server error occurred. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranslateException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranslateAsyncClient.ListTextTranslationJobs
* @see AWS API Documentation
*/
default CompletableFuture listTextTranslationJobs(
ListTextTranslationJobsRequest listTextTranslationJobsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets a list of the batch translation jobs that you have submitted.
*
*
*
* This is a convenience which creates an instance of the {@link ListTextTranslationJobsRequest.Builder} avoiding
* the need to create one manually via {@link ListTextTranslationJobsRequest#builder()}
*
*
* @param listTextTranslationJobsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.translate.model.ListTextTranslationJobsRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListTextTranslationJobs operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidRequestException The request that you made is not valid. Check your request to determine why
* it's not valid and then retry the request.
* - TooManyRequestsException You have made too many requests within a short period of time. Wait for a
* short time and then try your request again.
* - InvalidFilterException The filter specified for the operation is not valid. Specify a different
* filter.
* - InternalServerException An internal server error occurred. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranslateException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranslateAsyncClient.ListTextTranslationJobs
* @see AWS API Documentation
*/
default CompletableFuture listTextTranslationJobs(
Consumer listTextTranslationJobsRequest) {
return listTextTranslationJobs(ListTextTranslationJobsRequest.builder().applyMutation(listTextTranslationJobsRequest)
.build());
}
/**
*
* This is a variant of
* {@link #listTextTranslationJobs(software.amazon.awssdk.services.translate.model.ListTextTranslationJobsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.translate.paginators.ListTextTranslationJobsPublisher publisher = client.listTextTranslationJobsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.translate.paginators.ListTextTranslationJobsPublisher publisher = client.listTextTranslationJobsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.translate.model.ListTextTranslationJobsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listTextTranslationJobs(software.amazon.awssdk.services.translate.model.ListTextTranslationJobsRequest)}
* operation.
*
*
* @param listTextTranslationJobsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidRequestException The request that you made is not valid. Check your request to determine why
* it's not valid and then retry the request.
* - TooManyRequestsException You have made too many requests within a short period of time. Wait for a
* short time and then try your request again.
* - InvalidFilterException The filter specified for the operation is not valid. Specify a different
* filter.
* - InternalServerException An internal server error occurred. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranslateException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranslateAsyncClient.ListTextTranslationJobs
* @see AWS API Documentation
*/
default ListTextTranslationJobsPublisher listTextTranslationJobsPaginator(
ListTextTranslationJobsRequest listTextTranslationJobsRequest) {
return new ListTextTranslationJobsPublisher(this, listTextTranslationJobsRequest);
}
/**
*
* This is a variant of
* {@link #listTextTranslationJobs(software.amazon.awssdk.services.translate.model.ListTextTranslationJobsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.translate.paginators.ListTextTranslationJobsPublisher publisher = client.listTextTranslationJobsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.translate.paginators.ListTextTranslationJobsPublisher publisher = client.listTextTranslationJobsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.translate.model.ListTextTranslationJobsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listTextTranslationJobs(software.amazon.awssdk.services.translate.model.ListTextTranslationJobsRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListTextTranslationJobsRequest.Builder} avoiding
* the need to create one manually via {@link ListTextTranslationJobsRequest#builder()}
*
*
* @param listTextTranslationJobsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.translate.model.ListTextTranslationJobsRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidRequestException The request that you made is not valid. Check your request to determine why
* it's not valid and then retry the request.
* - TooManyRequestsException You have made too many requests within a short period of time. Wait for a
* short time and then try your request again.
* - InvalidFilterException The filter specified for the operation is not valid. Specify a different
* filter.
* - InternalServerException An internal server error occurred. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranslateException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranslateAsyncClient.ListTextTranslationJobs
* @see AWS API Documentation
*/
default ListTextTranslationJobsPublisher listTextTranslationJobsPaginator(
Consumer listTextTranslationJobsRequest) {
return listTextTranslationJobsPaginator(ListTextTranslationJobsRequest.builder()
.applyMutation(listTextTranslationJobsRequest).build());
}
/**
*
* Starts an asynchronous batch translation job. Use batch translation jobs to translate large volumes of text
* across multiple documents at once. For batch translation, you can input documents with different source languages
* (specify auto
as the source language). You can specify one or more target languages. Batch
* translation translates each input document into each of the target languages. For more information, see Asynchronous batch processing.
*
*
* Batch translation jobs can be described with the DescribeTextTranslationJob operation, listed with the
* ListTextTranslationJobs operation, and stopped with the StopTextTranslationJob operation.
*
*
* @param startTextTranslationJobRequest
* @return A Java Future containing the result of the StartTextTranslationJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - TooManyRequestsException You have made too many requests within a short period of time. Wait for a
* short time and then try your request again.
* - UnsupportedLanguagePairException Amazon Translate does not support translation from the language of
* the source text into the requested target language. For more information, see Supported languages.
* - InvalidRequestException The request that you made is not valid. Check your request to determine why
* it's not valid and then retry the request.
* - ResourceNotFoundException The resource you are looking for has not been found. Review the resource
* you're looking for and see if a different resource will accomplish your needs before retrying the revised
* request.
* - InvalidParameterValueException The value of the parameter is not valid. Review the value of the
* parameter you are using to correct it, and then retry your operation.
* - InternalServerException An internal server error occurred. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranslateException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranslateAsyncClient.StartTextTranslationJob
* @see AWS API Documentation
*/
default CompletableFuture startTextTranslationJob(
StartTextTranslationJobRequest startTextTranslationJobRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Starts an asynchronous batch translation job. Use batch translation jobs to translate large volumes of text
* across multiple documents at once. For batch translation, you can input documents with different source languages
* (specify auto
as the source language). You can specify one or more target languages. Batch
* translation translates each input document into each of the target languages. For more information, see Asynchronous batch processing.
*
*
* Batch translation jobs can be described with the DescribeTextTranslationJob operation, listed with the
* ListTextTranslationJobs operation, and stopped with the StopTextTranslationJob operation.
*
*
*
* This is a convenience which creates an instance of the {@link StartTextTranslationJobRequest.Builder} avoiding
* the need to create one manually via {@link StartTextTranslationJobRequest#builder()}
*
*
* @param startTextTranslationJobRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.translate.model.StartTextTranslationJobRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the StartTextTranslationJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - TooManyRequestsException You have made too many requests within a short period of time. Wait for a
* short time and then try your request again.
* - UnsupportedLanguagePairException Amazon Translate does not support translation from the language of
* the source text into the requested target language. For more information, see Supported languages.
* - InvalidRequestException The request that you made is not valid. Check your request to determine why
* it's not valid and then retry the request.
* - ResourceNotFoundException The resource you are looking for has not been found. Review the resource
* you're looking for and see if a different resource will accomplish your needs before retrying the revised
* request.
* - InvalidParameterValueException The value of the parameter is not valid. Review the value of the
* parameter you are using to correct it, and then retry your operation.
* - InternalServerException An internal server error occurred. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranslateException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranslateAsyncClient.StartTextTranslationJob
* @see AWS API Documentation
*/
default CompletableFuture startTextTranslationJob(
Consumer startTextTranslationJobRequest) {
return startTextTranslationJob(StartTextTranslationJobRequest.builder().applyMutation(startTextTranslationJobRequest)
.build());
}
/**
*
* Stops an asynchronous batch translation job that is in progress.
*
*
* If the job's state is IN_PROGRESS
, the job will be marked for termination and put into the
* STOP_REQUESTED
state. If the job completes before it can be stopped, it is put into the
* COMPLETED
state. Otherwise, the job is put into the STOPPED
state.
*
*
* Asynchronous batch translation jobs are started with the StartTextTranslationJob operation. You can use
* the DescribeTextTranslationJob or ListTextTranslationJobs operations to get a batch translation
* job's JobId
.
*
*
* @param stopTextTranslationJobRequest
* @return A Java Future containing the result of the StopTextTranslationJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException The resource you are looking for has not been found. Review the resource
* you're looking for and see if a different resource will accomplish your needs before retrying the revised
* request.
* - TooManyRequestsException You have made too many requests within a short period of time. Wait for a
* short time and then try your request again.
* - InternalServerException An internal server error occurred. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranslateException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranslateAsyncClient.StopTextTranslationJob
* @see AWS API Documentation
*/
default CompletableFuture stopTextTranslationJob(
StopTextTranslationJobRequest stopTextTranslationJobRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Stops an asynchronous batch translation job that is in progress.
*
*
* If the job's state is IN_PROGRESS
, the job will be marked for termination and put into the
* STOP_REQUESTED
state. If the job completes before it can be stopped, it is put into the
* COMPLETED
state. Otherwise, the job is put into the STOPPED
state.
*
*
* Asynchronous batch translation jobs are started with the StartTextTranslationJob operation. You can use
* the DescribeTextTranslationJob or ListTextTranslationJobs operations to get a batch translation
* job's JobId
.
*
*
*
* This is a convenience which creates an instance of the {@link StopTextTranslationJobRequest.Builder} avoiding the
* need to create one manually via {@link StopTextTranslationJobRequest#builder()}
*
*
* @param stopTextTranslationJobRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.translate.model.StopTextTranslationJobRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the StopTextTranslationJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException The resource you are looking for has not been found. Review the resource
* you're looking for and see if a different resource will accomplish your needs before retrying the revised
* request.
* - TooManyRequestsException You have made too many requests within a short period of time. Wait for a
* short time and then try your request again.
* - InternalServerException An internal server error occurred. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranslateException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranslateAsyncClient.StopTextTranslationJob
* @see AWS API Documentation
*/
default CompletableFuture stopTextTranslationJob(
Consumer stopTextTranslationJobRequest) {
return stopTextTranslationJob(StopTextTranslationJobRequest.builder().applyMutation(stopTextTranslationJobRequest)
.build());
}
/**
*
* Associates a specific tag with a resource. A tag is a key-value pair that adds as a metadata to a resource. For
* more information, see Tagging your
* resources.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidParameterValueException The value of the parameter is not valid. Review the value of the
* parameter you are using to correct it, and then retry your operation.
* - ConcurrentModificationException Another modification is being made. That modification must complete
* before you can make your change.
* - ResourceNotFoundException The resource you are looking for has not been found. Review the resource
* you're looking for and see if a different resource will accomplish your needs before retrying the revised
* request.
* - TooManyTagsException You have added too many tags to this resource. The maximum is 50 tags.
* - InternalServerException An internal server error occurred. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranslateException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranslateAsyncClient.TagResource
* @see AWS API
* Documentation
*/
default CompletableFuture tagResource(TagResourceRequest tagResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Associates a specific tag with a resource. A tag is a key-value pair that adds as a metadata to a resource. For
* more information, see Tagging your
* resources.
*
*
*
* This is a convenience which creates an instance of the {@link TagResourceRequest.Builder} avoiding the need to
* create one manually via {@link TagResourceRequest#builder()}
*
*
* @param tagResourceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.translate.model.TagResourceRequest.Builder} to create a request.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidParameterValueException The value of the parameter is not valid. Review the value of the
* parameter you are using to correct it, and then retry your operation.
* - ConcurrentModificationException Another modification is being made. That modification must complete
* before you can make your change.
* - ResourceNotFoundException The resource you are looking for has not been found. Review the resource
* you're looking for and see if a different resource will accomplish your needs before retrying the revised
* request.
* - TooManyTagsException You have added too many tags to this resource. The maximum is 50 tags.
* - InternalServerException An internal server error occurred. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranslateException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranslateAsyncClient.TagResource
* @see AWS API
* Documentation
*/
default CompletableFuture tagResource(Consumer tagResourceRequest) {
return tagResource(TagResourceRequest.builder().applyMutation(tagResourceRequest).build());
}
/**
*
* Translates the input document from the source language to the target language. This synchronous operation
* supports text, HTML, or Word documents as the input document. TranslateDocument
supports
* translations from English to any supported language, and from any supported language to English. Therefore,
* specify either the source language code or the target language code as “en” (English).
*
*
* If you set the Formality
parameter, the request will fail if the target language does not support
* formality. For a list of target languages that support formality, see Setting
* formality.
*
*
* @param translateDocumentRequest
* @return A Java Future containing the result of the TranslateDocument operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidRequestException The request that you made is not valid. Check your request to determine why
* it's not valid and then retry the request.
* - LimitExceededException The specified limit has been exceeded. Review your request and retry it with a
* quantity below the stated limit.
* - TooManyRequestsException You have made too many requests within a short period of time. Wait for a
* short time and then try your request again.
* - ResourceNotFoundException The resource you are looking for has not been found. Review the resource
* you're looking for and see if a different resource will accomplish your needs before retrying the revised
* request.
* - UnsupportedLanguagePairException Amazon Translate does not support translation from the language of
* the source text into the requested target language. For more information, see Supported languages.
* - InternalServerException An internal server error occurred. Retry your request.
* - ServiceUnavailableException The Amazon Translate service is temporarily unavailable. Wait a bit and
* then retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranslateException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranslateAsyncClient.TranslateDocument
* @see AWS
* API Documentation
*/
default CompletableFuture translateDocument(TranslateDocumentRequest translateDocumentRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Translates the input document from the source language to the target language. This synchronous operation
* supports text, HTML, or Word documents as the input document. TranslateDocument
supports
* translations from English to any supported language, and from any supported language to English. Therefore,
* specify either the source language code or the target language code as “en” (English).
*
*
* If you set the Formality
parameter, the request will fail if the target language does not support
* formality. For a list of target languages that support formality, see Setting
* formality.
*
*
*
* This is a convenience which creates an instance of the {@link TranslateDocumentRequest.Builder} avoiding the need
* to create one manually via {@link TranslateDocumentRequest#builder()}
*
*
* @param translateDocumentRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.translate.model.TranslateDocumentRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the TranslateDocument operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidRequestException The request that you made is not valid. Check your request to determine why
* it's not valid and then retry the request.
* - LimitExceededException The specified limit has been exceeded. Review your request and retry it with a
* quantity below the stated limit.
* - TooManyRequestsException You have made too many requests within a short period of time. Wait for a
* short time and then try your request again.
* - ResourceNotFoundException The resource you are looking for has not been found. Review the resource
* you're looking for and see if a different resource will accomplish your needs before retrying the revised
* request.
* - UnsupportedLanguagePairException Amazon Translate does not support translation from the language of
* the source text into the requested target language. For more information, see Supported languages.
* - InternalServerException An internal server error occurred. Retry your request.
* - ServiceUnavailableException The Amazon Translate service is temporarily unavailable. Wait a bit and
* then retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranslateException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranslateAsyncClient.TranslateDocument
* @see AWS
* API Documentation
*/
default CompletableFuture translateDocument(
Consumer translateDocumentRequest) {
return translateDocument(TranslateDocumentRequest.builder().applyMutation(translateDocumentRequest).build());
}
/**
*
* Translates input text from the source language to the target language. For a list of available languages and
* language codes, see Supported
* languages.
*
*
* @param translateTextRequest
* @return A Java Future containing the result of the TranslateText operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidRequestException The request that you made is not valid. Check your request to determine why
* it's not valid and then retry the request.
* - TextSizeLimitExceededException The size of the text you submitted exceeds the size limit. Reduce the
* size of the text or use a smaller document and then retry your request.
* - TooManyRequestsException You have made too many requests within a short period of time. Wait for a
* short time and then try your request again.
* - UnsupportedLanguagePairException Amazon Translate does not support translation from the language of
* the source text into the requested target language. For more information, see Supported languages.
* - DetectedLanguageLowConfidenceException The confidence that Amazon Comprehend accurately detected the
* source language is low. If a low confidence level is acceptable for your application, you can use the
* language in the exception to call Amazon Translate again. For more information, see the DetectDominantLanguage operation in the Amazon Comprehend Developer Guide.
* - ResourceNotFoundException The resource you are looking for has not been found. Review the resource
* you're looking for and see if a different resource will accomplish your needs before retrying the revised
* request.
* - InternalServerException An internal server error occurred. Retry your request.
* - ServiceUnavailableException The Amazon Translate service is temporarily unavailable. Wait a bit and
* then retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranslateException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranslateAsyncClient.TranslateText
* @see AWS API
* Documentation
*/
default CompletableFuture translateText(TranslateTextRequest translateTextRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Translates input text from the source language to the target language. For a list of available languages and
* language codes, see Supported
* languages.
*
*
*
* This is a convenience which creates an instance of the {@link TranslateTextRequest.Builder} avoiding the need to
* create one manually via {@link TranslateTextRequest#builder()}
*
*
* @param translateTextRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.translate.model.TranslateTextRequest.Builder} to create a request.
* @return A Java Future containing the result of the TranslateText operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidRequestException The request that you made is not valid. Check your request to determine why
* it's not valid and then retry the request.
* - TextSizeLimitExceededException The size of the text you submitted exceeds the size limit. Reduce the
* size of the text or use a smaller document and then retry your request.
* - TooManyRequestsException You have made too many requests within a short period of time. Wait for a
* short time and then try your request again.
* - UnsupportedLanguagePairException Amazon Translate does not support translation from the language of
* the source text into the requested target language. For more information, see Supported languages.
* - DetectedLanguageLowConfidenceException The confidence that Amazon Comprehend accurately detected the
* source language is low. If a low confidence level is acceptable for your application, you can use the
* language in the exception to call Amazon Translate again. For more information, see the DetectDominantLanguage operation in the Amazon Comprehend Developer Guide.
* - ResourceNotFoundException The resource you are looking for has not been found. Review the resource
* you're looking for and see if a different resource will accomplish your needs before retrying the revised
* request.
* - InternalServerException An internal server error occurred. Retry your request.
* - ServiceUnavailableException The Amazon Translate service is temporarily unavailable. Wait a bit and
* then retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranslateException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranslateAsyncClient.TranslateText
* @see AWS API
* Documentation
*/
default CompletableFuture translateText(Consumer translateTextRequest) {
return translateText(TranslateTextRequest.builder().applyMutation(translateTextRequest).build());
}
/**
*
* Removes a specific tag associated with an Amazon Translate resource. For more information, see Tagging your resources.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidParameterValueException The value of the parameter is not valid. Review the value of the
* parameter you are using to correct it, and then retry your operation.
* - ConcurrentModificationException Another modification is being made. That modification must complete
* before you can make your change.
* - ResourceNotFoundException The resource you are looking for has not been found. Review the resource
* you're looking for and see if a different resource will accomplish your needs before retrying the revised
* request.
* - InternalServerException An internal server error occurred. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranslateException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranslateAsyncClient.UntagResource
* @see AWS API
* Documentation
*/
default CompletableFuture untagResource(UntagResourceRequest untagResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Removes a specific tag associated with an Amazon Translate resource. For more information, see Tagging your resources.
*
*
*
* This is a convenience which creates an instance of the {@link UntagResourceRequest.Builder} avoiding the need to
* create one manually via {@link UntagResourceRequest#builder()}
*
*
* @param untagResourceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.translate.model.UntagResourceRequest.Builder} to create a request.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidParameterValueException The value of the parameter is not valid. Review the value of the
* parameter you are using to correct it, and then retry your operation.
* - ConcurrentModificationException Another modification is being made. That modification must complete
* before you can make your change.
* - ResourceNotFoundException The resource you are looking for has not been found. Review the resource
* you're looking for and see if a different resource will accomplish your needs before retrying the revised
* request.
* - InternalServerException An internal server error occurred. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranslateException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranslateAsyncClient.UntagResource
* @see AWS API
* Documentation
*/
default CompletableFuture untagResource(Consumer untagResourceRequest) {
return untagResource(UntagResourceRequest.builder().applyMutation(untagResourceRequest).build());
}
/**
*
* Updates a previously created parallel data resource by importing a new input file from Amazon S3.
*
*
* @param updateParallelDataRequest
* @return A Java Future containing the result of the UpdateParallelData operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ConcurrentModificationException Another modification is being made. That modification must complete
* before you can make your change.
* - InvalidParameterValueException The value of the parameter is not valid. Review the value of the
* parameter you are using to correct it, and then retry your operation.
* - InvalidRequestException The request that you made is not valid. Check your request to determine why
* it's not valid and then retry the request.
* - LimitExceededException The specified limit has been exceeded. Review your request and retry it with a
* quantity below the stated limit.
* - TooManyRequestsException You have made too many requests within a short period of time. Wait for a
* short time and then try your request again.
* - ConflictException There was a conflict processing the request. Try your request again.
* - ResourceNotFoundException The resource you are looking for has not been found. Review the resource
* you're looking for and see if a different resource will accomplish your needs before retrying the revised
* request.
* - InternalServerException An internal server error occurred. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranslateException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranslateAsyncClient.UpdateParallelData
* @see AWS
* API Documentation
*/
default CompletableFuture updateParallelData(UpdateParallelDataRequest updateParallelDataRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates a previously created parallel data resource by importing a new input file from Amazon S3.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateParallelDataRequest.Builder} avoiding the
* need to create one manually via {@link UpdateParallelDataRequest#builder()}
*
*
* @param updateParallelDataRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.translate.model.UpdateParallelDataRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the UpdateParallelData operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ConcurrentModificationException Another modification is being made. That modification must complete
* before you can make your change.
* - InvalidParameterValueException The value of the parameter is not valid. Review the value of the
* parameter you are using to correct it, and then retry your operation.
* - InvalidRequestException The request that you made is not valid. Check your request to determine why
* it's not valid and then retry the request.
* - LimitExceededException The specified limit has been exceeded. Review your request and retry it with a
* quantity below the stated limit.
* - TooManyRequestsException You have made too many requests within a short period of time. Wait for a
* short time and then try your request again.
* - ConflictException There was a conflict processing the request. Try your request again.
* - ResourceNotFoundException The resource you are looking for has not been found. Review the resource
* you're looking for and see if a different resource will accomplish your needs before retrying the revised
* request.
* - InternalServerException An internal server error occurred. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - TranslateException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample TranslateAsyncClient.UpdateParallelData
* @see AWS
* API Documentation
*/
default CompletableFuture updateParallelData(
Consumer updateParallelDataRequest) {
return updateParallelData(UpdateParallelDataRequest.builder().applyMutation(updateParallelDataRequest).build());
}
@Override
default TranslateServiceClientConfiguration serviceClientConfiguration() {
throw new UnsupportedOperationException();
}
/**
* Create a {@link TranslateAsyncClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static TranslateAsyncClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link TranslateAsyncClient}.
*/
static TranslateAsyncClientBuilder builder() {
return new DefaultTranslateAsyncClientBuilder();
}
}