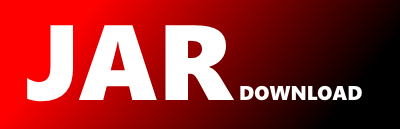
software.amazon.awssdk.services.translate.TranslateClient Maven / Gradle / Ivy
Show all versions of translate Show documentation
/*
* Copyright 2014-2019 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.translate;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.core.SdkClient;
import software.amazon.awssdk.core.exception.SdkClientException;
import software.amazon.awssdk.regions.ServiceMetadata;
import software.amazon.awssdk.services.translate.model.DeleteTerminologyRequest;
import software.amazon.awssdk.services.translate.model.DeleteTerminologyResponse;
import software.amazon.awssdk.services.translate.model.DetectedLanguageLowConfidenceException;
import software.amazon.awssdk.services.translate.model.GetTerminologyRequest;
import software.amazon.awssdk.services.translate.model.GetTerminologyResponse;
import software.amazon.awssdk.services.translate.model.ImportTerminologyRequest;
import software.amazon.awssdk.services.translate.model.ImportTerminologyResponse;
import software.amazon.awssdk.services.translate.model.InternalServerException;
import software.amazon.awssdk.services.translate.model.InvalidParameterValueException;
import software.amazon.awssdk.services.translate.model.InvalidRequestException;
import software.amazon.awssdk.services.translate.model.LimitExceededException;
import software.amazon.awssdk.services.translate.model.ListTerminologiesRequest;
import software.amazon.awssdk.services.translate.model.ListTerminologiesResponse;
import software.amazon.awssdk.services.translate.model.ResourceNotFoundException;
import software.amazon.awssdk.services.translate.model.ServiceUnavailableException;
import software.amazon.awssdk.services.translate.model.TextSizeLimitExceededException;
import software.amazon.awssdk.services.translate.model.TooManyRequestsException;
import software.amazon.awssdk.services.translate.model.TranslateException;
import software.amazon.awssdk.services.translate.model.TranslateTextRequest;
import software.amazon.awssdk.services.translate.model.TranslateTextResponse;
import software.amazon.awssdk.services.translate.model.UnsupportedLanguagePairException;
/**
* Service client for accessing Amazon Translate. This can be created using the static {@link #builder()} method.
*
*
* Provides translation between one source language and another of the same set of languages.
*
*/
@Generated("software.amazon.awssdk:codegen")
public interface TranslateClient extends SdkClient {
String SERVICE_NAME = "translate";
/**
* Create a {@link TranslateClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static TranslateClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link TranslateClient}.
*/
static TranslateClientBuilder builder() {
return new DefaultTranslateClientBuilder();
}
/**
*
* A synchronous action that deletes a custom terminology.
*
*
* @param deleteTerminologyRequest
* @return Result of the DeleteTerminology operation returned by the service.
* @throws ResourceNotFoundException
* The resource you are looking for has not been found. Review the resource you're looking for and see if a
* different resource will accomplish your needs before retrying the revised request. .
* @throws TooManyRequestsException
* You have made too many requests within a short period of time. Wait for a short time and then try your
* request again.
* @throws InternalServerException
* An internal server error occurred. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranslateException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranslateClient.DeleteTerminology
* @see AWS
* API Documentation
*/
default DeleteTerminologyResponse deleteTerminology(DeleteTerminologyRequest deleteTerminologyRequest)
throws ResourceNotFoundException, TooManyRequestsException, InternalServerException, AwsServiceException,
SdkClientException, TranslateException {
throw new UnsupportedOperationException();
}
/**
*
* A synchronous action that deletes a custom terminology.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteTerminologyRequest.Builder} avoiding the need
* to create one manually via {@link DeleteTerminologyRequest#builder()}
*
*
* @param deleteTerminologyRequest
* A {@link Consumer} that will call methods on {@link DeleteTerminologyRequest.Builder} to create a request.
* @return Result of the DeleteTerminology operation returned by the service.
* @throws ResourceNotFoundException
* The resource you are looking for has not been found. Review the resource you're looking for and see if a
* different resource will accomplish your needs before retrying the revised request. .
* @throws TooManyRequestsException
* You have made too many requests within a short period of time. Wait for a short time and then try your
* request again.
* @throws InternalServerException
* An internal server error occurred. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranslateException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranslateClient.DeleteTerminology
* @see AWS
* API Documentation
*/
default DeleteTerminologyResponse deleteTerminology(Consumer deleteTerminologyRequest)
throws ResourceNotFoundException, TooManyRequestsException, InternalServerException, AwsServiceException,
SdkClientException, TranslateException {
return deleteTerminology(DeleteTerminologyRequest.builder().applyMutation(deleteTerminologyRequest).build());
}
/**
*
* Retrieves a custom terminology.
*
*
* @param getTerminologyRequest
* @return Result of the GetTerminology operation returned by the service.
* @throws ResourceNotFoundException
* The resource you are looking for has not been found. Review the resource you're looking for and see if a
* different resource will accomplish your needs before retrying the revised request. .
* @throws InvalidParameterValueException
* The value of the parameter is invalid. Review the value of the parameter you are using to correct it, and
* then retry your operation.
* @throws TooManyRequestsException
* You have made too many requests within a short period of time. Wait for a short time and then try your
* request again.
* @throws InternalServerException
* An internal server error occurred. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranslateException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranslateClient.GetTerminology
* @see AWS API
* Documentation
*/
default GetTerminologyResponse getTerminology(GetTerminologyRequest getTerminologyRequest) throws ResourceNotFoundException,
InvalidParameterValueException, TooManyRequestsException, InternalServerException, AwsServiceException,
SdkClientException, TranslateException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves a custom terminology.
*
*
*
* This is a convenience which creates an instance of the {@link GetTerminologyRequest.Builder} avoiding the need to
* create one manually via {@link GetTerminologyRequest#builder()}
*
*
* @param getTerminologyRequest
* A {@link Consumer} that will call methods on {@link GetTerminologyRequest.Builder} to create a request.
* @return Result of the GetTerminology operation returned by the service.
* @throws ResourceNotFoundException
* The resource you are looking for has not been found. Review the resource you're looking for and see if a
* different resource will accomplish your needs before retrying the revised request. .
* @throws InvalidParameterValueException
* The value of the parameter is invalid. Review the value of the parameter you are using to correct it, and
* then retry your operation.
* @throws TooManyRequestsException
* You have made too many requests within a short period of time. Wait for a short time and then try your
* request again.
* @throws InternalServerException
* An internal server error occurred. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranslateException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranslateClient.GetTerminology
* @see AWS API
* Documentation
*/
default GetTerminologyResponse getTerminology(Consumer getTerminologyRequest)
throws ResourceNotFoundException, InvalidParameterValueException, TooManyRequestsException, InternalServerException,
AwsServiceException, SdkClientException, TranslateException {
return getTerminology(GetTerminologyRequest.builder().applyMutation(getTerminologyRequest).build());
}
/**
*
* Creates or updates a custom terminology, depending on whether or not one already exists for the given terminology
* name. Importing a terminology with the same name as an existing one will merge the terminologies based on the
* chosen merge strategy. Currently, the only supported merge strategy is OVERWRITE, and so the imported terminology
* will overwrite an existing terminology of the same name.
*
*
* If you import a terminology that overwrites an existing one, the new terminology take up to 10 minutes to fully
* propagate and be available for use in a translation due to cache policies with the DataPlane service that
* performs the translations.
*
*
* @param importTerminologyRequest
* @return Result of the ImportTerminology operation returned by the service.
* @throws InvalidParameterValueException
* The value of the parameter is invalid. Review the value of the parameter you are using to correct it, and
* then retry your operation.
* @throws LimitExceededException
* The specified limit has been exceeded. Review your request and retry it with a quantity below the stated
* limit.
* @throws TooManyRequestsException
* You have made too many requests within a short period of time. Wait for a short time and then try your
* request again.
* @throws InternalServerException
* An internal server error occurred. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranslateException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranslateClient.ImportTerminology
* @see AWS
* API Documentation
*/
default ImportTerminologyResponse importTerminology(ImportTerminologyRequest importTerminologyRequest)
throws InvalidParameterValueException, LimitExceededException, TooManyRequestsException, InternalServerException,
AwsServiceException, SdkClientException, TranslateException {
throw new UnsupportedOperationException();
}
/**
*
* Creates or updates a custom terminology, depending on whether or not one already exists for the given terminology
* name. Importing a terminology with the same name as an existing one will merge the terminologies based on the
* chosen merge strategy. Currently, the only supported merge strategy is OVERWRITE, and so the imported terminology
* will overwrite an existing terminology of the same name.
*
*
* If you import a terminology that overwrites an existing one, the new terminology take up to 10 minutes to fully
* propagate and be available for use in a translation due to cache policies with the DataPlane service that
* performs the translations.
*
*
*
* This is a convenience which creates an instance of the {@link ImportTerminologyRequest.Builder} avoiding the need
* to create one manually via {@link ImportTerminologyRequest#builder()}
*
*
* @param importTerminologyRequest
* A {@link Consumer} that will call methods on {@link ImportTerminologyRequest.Builder} to create a request.
* @return Result of the ImportTerminology operation returned by the service.
* @throws InvalidParameterValueException
* The value of the parameter is invalid. Review the value of the parameter you are using to correct it, and
* then retry your operation.
* @throws LimitExceededException
* The specified limit has been exceeded. Review your request and retry it with a quantity below the stated
* limit.
* @throws TooManyRequestsException
* You have made too many requests within a short period of time. Wait for a short time and then try your
* request again.
* @throws InternalServerException
* An internal server error occurred. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranslateException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranslateClient.ImportTerminology
* @see AWS
* API Documentation
*/
default ImportTerminologyResponse importTerminology(Consumer importTerminologyRequest)
throws InvalidParameterValueException, LimitExceededException, TooManyRequestsException, InternalServerException,
AwsServiceException, SdkClientException, TranslateException {
return importTerminology(ImportTerminologyRequest.builder().applyMutation(importTerminologyRequest).build());
}
/**
*
* Provides a list of custom terminologies associated with your account.
*
*
* @return Result of the ListTerminologies operation returned by the service.
* @throws InvalidParameterValueException
* The value of the parameter is invalid. Review the value of the parameter you are using to correct it, and
* then retry your operation.
* @throws TooManyRequestsException
* You have made too many requests within a short period of time. Wait for a short time and then try your
* request again.
* @throws InternalServerException
* An internal server error occurred. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranslateException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranslateClient.ListTerminologies
* @see #listTerminologies(ListTerminologiesRequest)
* @see AWS
* API Documentation
*/
default ListTerminologiesResponse listTerminologies() throws InvalidParameterValueException, TooManyRequestsException,
InternalServerException, AwsServiceException, SdkClientException, TranslateException {
return listTerminologies(ListTerminologiesRequest.builder().build());
}
/**
*
* Provides a list of custom terminologies associated with your account.
*
*
* @param listTerminologiesRequest
* @return Result of the ListTerminologies operation returned by the service.
* @throws InvalidParameterValueException
* The value of the parameter is invalid. Review the value of the parameter you are using to correct it, and
* then retry your operation.
* @throws TooManyRequestsException
* You have made too many requests within a short period of time. Wait for a short time and then try your
* request again.
* @throws InternalServerException
* An internal server error occurred. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranslateException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranslateClient.ListTerminologies
* @see AWS
* API Documentation
*/
default ListTerminologiesResponse listTerminologies(ListTerminologiesRequest listTerminologiesRequest)
throws InvalidParameterValueException, TooManyRequestsException, InternalServerException, AwsServiceException,
SdkClientException, TranslateException {
throw new UnsupportedOperationException();
}
/**
*
* Provides a list of custom terminologies associated with your account.
*
*
*
* This is a convenience which creates an instance of the {@link ListTerminologiesRequest.Builder} avoiding the need
* to create one manually via {@link ListTerminologiesRequest#builder()}
*
*
* @param listTerminologiesRequest
* A {@link Consumer} that will call methods on {@link ListTerminologiesRequest.Builder} to create a request.
* @return Result of the ListTerminologies operation returned by the service.
* @throws InvalidParameterValueException
* The value of the parameter is invalid. Review the value of the parameter you are using to correct it, and
* then retry your operation.
* @throws TooManyRequestsException
* You have made too many requests within a short period of time. Wait for a short time and then try your
* request again.
* @throws InternalServerException
* An internal server error occurred. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranslateException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranslateClient.ListTerminologies
* @see AWS
* API Documentation
*/
default ListTerminologiesResponse listTerminologies(Consumer listTerminologiesRequest)
throws InvalidParameterValueException, TooManyRequestsException, InternalServerException, AwsServiceException,
SdkClientException, TranslateException {
return listTerminologies(ListTerminologiesRequest.builder().applyMutation(listTerminologiesRequest).build());
}
/**
*
* Translates input text from the source language to the target language. It is not necessary to use English (en) as
* either the source or the target language but not all language combinations are supported by Amazon Translate. For
* more information, see Supported Language
* Pairs.
*
*
* -
*
* Arabic (ar)
*
*
* -
*
* Chinese (Simplified) (zh)
*
*
* -
*
* Chinese (Traditional) (zh-TW)
*
*
* -
*
* Czech (cs)
*
*
* -
*
* Danish (da)
*
*
* -
*
* Dutch (nl)
*
*
* -
*
* English (en)
*
*
* -
*
* Finnish (fi)
*
*
* -
*
* French (fr)
*
*
* -
*
* German (de)
*
*
* -
*
* Hebrew (he)
*
*
* -
*
* Indonesian (id)
*
*
* -
*
* Italian (it)
*
*
* -
*
* Japanese (ja)
*
*
* -
*
* Korean (ko)
*
*
* -
*
* Polish (pl)
*
*
* -
*
* Portuguese (pt)
*
*
* -
*
* Russian (ru)
*
*
* -
*
* Spanish (es)
*
*
* -
*
* Swedish (sv)
*
*
* -
*
* Turkish (tr)
*
*
*
*
* To have Amazon Translate determine the source language of your text, you can specify auto
in the
* SourceLanguageCode
field. If you specify auto
, Amazon Translate will call Amazon
* Comprehend to determine the source language.
*
*
* @param translateTextRequest
* @return Result of the TranslateText operation returned by the service.
* @throws InvalidRequestException
* The request that you made is invalid. Check your request to determine why it's invalid and then retry the
* request.
* @throws TextSizeLimitExceededException
* The size of the text you submitted exceeds the size limit. Reduce the size of the text or use a smaller
* document and then retry your request.
* @throws TooManyRequestsException
* You have made too many requests within a short period of time. Wait for a short time and then try your
* request again.
* @throws UnsupportedLanguagePairException
* Amazon Translate does not support translation from the language of the source text into the requested
* target language. For more information, see how-to-error-msg.
* @throws DetectedLanguageLowConfidenceException
* The confidence that Amazon Comprehend accurately detected the source language is low. If a low confidence
* level is acceptable for your application, you can use the language in the exception to call Amazon
* Translate again. For more information, see the DetectDominantLanguage operation in the Amazon Comprehend Developer Guide.
* @throws ResourceNotFoundException
* The resource you are looking for has not been found. Review the resource you're looking for and see if a
* different resource will accomplish your needs before retrying the revised request. .
* @throws InternalServerException
* An internal server error occurred. Retry your request.
* @throws ServiceUnavailableException
* The Amazon Translate service is temporarily unavailable. Please wait a bit and then retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranslateException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranslateClient.TranslateText
* @see AWS API
* Documentation
*/
default TranslateTextResponse translateText(TranslateTextRequest translateTextRequest) throws InvalidRequestException,
TextSizeLimitExceededException, TooManyRequestsException, UnsupportedLanguagePairException,
DetectedLanguageLowConfidenceException, ResourceNotFoundException, InternalServerException,
ServiceUnavailableException, AwsServiceException, SdkClientException, TranslateException {
throw new UnsupportedOperationException();
}
/**
*
* Translates input text from the source language to the target language. It is not necessary to use English (en) as
* either the source or the target language but not all language combinations are supported by Amazon Translate. For
* more information, see Supported Language
* Pairs.
*
*
* -
*
* Arabic (ar)
*
*
* -
*
* Chinese (Simplified) (zh)
*
*
* -
*
* Chinese (Traditional) (zh-TW)
*
*
* -
*
* Czech (cs)
*
*
* -
*
* Danish (da)
*
*
* -
*
* Dutch (nl)
*
*
* -
*
* English (en)
*
*
* -
*
* Finnish (fi)
*
*
* -
*
* French (fr)
*
*
* -
*
* German (de)
*
*
* -
*
* Hebrew (he)
*
*
* -
*
* Indonesian (id)
*
*
* -
*
* Italian (it)
*
*
* -
*
* Japanese (ja)
*
*
* -
*
* Korean (ko)
*
*
* -
*
* Polish (pl)
*
*
* -
*
* Portuguese (pt)
*
*
* -
*
* Russian (ru)
*
*
* -
*
* Spanish (es)
*
*
* -
*
* Swedish (sv)
*
*
* -
*
* Turkish (tr)
*
*
*
*
* To have Amazon Translate determine the source language of your text, you can specify auto
in the
* SourceLanguageCode
field. If you specify auto
, Amazon Translate will call Amazon
* Comprehend to determine the source language.
*
*
*
* This is a convenience which creates an instance of the {@link TranslateTextRequest.Builder} avoiding the need to
* create one manually via {@link TranslateTextRequest#builder()}
*
*
* @param translateTextRequest
* A {@link Consumer} that will call methods on {@link TranslateTextRequest.Builder} to create a request.
* @return Result of the TranslateText operation returned by the service.
* @throws InvalidRequestException
* The request that you made is invalid. Check your request to determine why it's invalid and then retry the
* request.
* @throws TextSizeLimitExceededException
* The size of the text you submitted exceeds the size limit. Reduce the size of the text or use a smaller
* document and then retry your request.
* @throws TooManyRequestsException
* You have made too many requests within a short period of time. Wait for a short time and then try your
* request again.
* @throws UnsupportedLanguagePairException
* Amazon Translate does not support translation from the language of the source text into the requested
* target language. For more information, see how-to-error-msg.
* @throws DetectedLanguageLowConfidenceException
* The confidence that Amazon Comprehend accurately detected the source language is low. If a low confidence
* level is acceptable for your application, you can use the language in the exception to call Amazon
* Translate again. For more information, see the DetectDominantLanguage operation in the Amazon Comprehend Developer Guide.
* @throws ResourceNotFoundException
* The resource you are looking for has not been found. Review the resource you're looking for and see if a
* different resource will accomplish your needs before retrying the revised request. .
* @throws InternalServerException
* An internal server error occurred. Retry your request.
* @throws ServiceUnavailableException
* The Amazon Translate service is temporarily unavailable. Please wait a bit and then retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws TranslateException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample TranslateClient.TranslateText
* @see AWS API
* Documentation
*/
default TranslateTextResponse translateText(Consumer translateTextRequest)
throws InvalidRequestException, TextSizeLimitExceededException, TooManyRequestsException,
UnsupportedLanguagePairException, DetectedLanguageLowConfidenceException, ResourceNotFoundException,
InternalServerException, ServiceUnavailableException, AwsServiceException, SdkClientException, TranslateException {
return translateText(TranslateTextRequest.builder().applyMutation(translateTextRequest).build());
}
static ServiceMetadata serviceMetadata() {
return ServiceMetadata.of("translate");
}
}