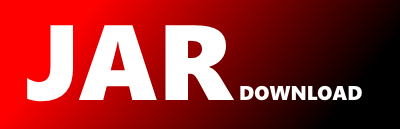
software.amazon.awssdk.services.trustedadvisor.model.Recommendation Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.trustedadvisor.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.traits.TimestampFormatTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* A Recommendation for an Account
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class Recommendation implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField ARN_FIELD = SdkField. builder(MarshallingType.STRING).memberName("arn")
.getter(getter(Recommendation::arn)).setter(setter(Builder::arn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("arn").build()).build();
private static final SdkField> AWS_SERVICES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("awsServices")
.getter(getter(Recommendation::awsServices))
.setter(setter(Builder::awsServices))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("awsServices").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField CHECK_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("checkArn").getter(getter(Recommendation::checkArn)).setter(setter(Builder::checkArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("checkArn").build()).build();
private static final SdkField CREATED_AT_FIELD = SdkField
. builder(MarshallingType.INSTANT)
.memberName("createdAt")
.getter(getter(Recommendation::createdAt))
.setter(setter(Builder::createdAt))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("createdAt").build(),
TimestampFormatTrait.create(TimestampFormatTrait.Format.ISO_8601)).build();
private static final SdkField CREATED_BY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("createdBy").getter(getter(Recommendation::createdBy)).setter(setter(Builder::createdBy))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("createdBy").build()).build();
private static final SdkField DESCRIPTION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("description").getter(getter(Recommendation::description)).setter(setter(Builder::description))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("description").build()).build();
private static final SdkField ID_FIELD = SdkField. builder(MarshallingType.STRING).memberName("id")
.getter(getter(Recommendation::id)).setter(setter(Builder::id))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("id").build()).build();
private static final SdkField LAST_UPDATED_AT_FIELD = SdkField
. builder(MarshallingType.INSTANT)
.memberName("lastUpdatedAt")
.getter(getter(Recommendation::lastUpdatedAt))
.setter(setter(Builder::lastUpdatedAt))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("lastUpdatedAt").build(),
TimestampFormatTrait.create(TimestampFormatTrait.Format.ISO_8601)).build();
private static final SdkField LIFECYCLE_STAGE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("lifecycleStage").getter(getter(Recommendation::lifecycleStageAsString))
.setter(setter(Builder::lifecycleStage))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("lifecycleStage").build()).build();
private static final SdkField NAME_FIELD = SdkField. builder(MarshallingType.STRING).memberName("name")
.getter(getter(Recommendation::name)).setter(setter(Builder::name))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("name").build()).build();
private static final SdkField PILLAR_SPECIFIC_AGGREGATES_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("pillarSpecificAggregates")
.getter(getter(Recommendation::pillarSpecificAggregates)).setter(setter(Builder::pillarSpecificAggregates))
.constructor(RecommendationPillarSpecificAggregates::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("pillarSpecificAggregates").build())
.build();
private static final SdkField> PILLARS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("pillars")
.getter(getter(Recommendation::pillarsAsStrings))
.setter(setter(Builder::pillarsWithStrings))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("pillars").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField RESOLVED_AT_FIELD = SdkField
. builder(MarshallingType.INSTANT)
.memberName("resolvedAt")
.getter(getter(Recommendation::resolvedAt))
.setter(setter(Builder::resolvedAt))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("resolvedAt").build(),
TimestampFormatTrait.create(TimestampFormatTrait.Format.ISO_8601)).build();
private static final SdkField RESOURCES_AGGREGATES_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("resourcesAggregates")
.getter(getter(Recommendation::resourcesAggregates)).setter(setter(Builder::resourcesAggregates))
.constructor(RecommendationResourcesAggregates::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("resourcesAggregates").build())
.build();
private static final SdkField SOURCE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("source")
.getter(getter(Recommendation::sourceAsString)).setter(setter(Builder::source))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("source").build()).build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("status")
.getter(getter(Recommendation::statusAsString)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("status").build()).build();
private static final SdkField TYPE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("type")
.getter(getter(Recommendation::typeAsString)).setter(setter(Builder::type))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("type").build()).build();
private static final SdkField UPDATE_REASON_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("updateReason").getter(getter(Recommendation::updateReason)).setter(setter(Builder::updateReason))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("updateReason").build()).build();
private static final SdkField UPDATE_REASON_CODE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("updateReasonCode").getter(getter(Recommendation::updateReasonCodeAsString))
.setter(setter(Builder::updateReasonCode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("updateReasonCode").build()).build();
private static final SdkField UPDATED_ON_BEHALF_OF_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("updatedOnBehalfOf").getter(getter(Recommendation::updatedOnBehalfOf))
.setter(setter(Builder::updatedOnBehalfOf))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("updatedOnBehalfOf").build()).build();
private static final SdkField UPDATED_ON_BEHALF_OF_JOB_TITLE_FIELD = SdkField
. builder(MarshallingType.STRING).memberName("updatedOnBehalfOfJobTitle")
.getter(getter(Recommendation::updatedOnBehalfOfJobTitle)).setter(setter(Builder::updatedOnBehalfOfJobTitle))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("updatedOnBehalfOfJobTitle").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ARN_FIELD, AWS_SERVICES_FIELD,
CHECK_ARN_FIELD, CREATED_AT_FIELD, CREATED_BY_FIELD, DESCRIPTION_FIELD, ID_FIELD, LAST_UPDATED_AT_FIELD,
LIFECYCLE_STAGE_FIELD, NAME_FIELD, PILLAR_SPECIFIC_AGGREGATES_FIELD, PILLARS_FIELD, RESOLVED_AT_FIELD,
RESOURCES_AGGREGATES_FIELD, SOURCE_FIELD, STATUS_FIELD, TYPE_FIELD, UPDATE_REASON_FIELD, UPDATE_REASON_CODE_FIELD,
UPDATED_ON_BEHALF_OF_FIELD, UPDATED_ON_BEHALF_OF_JOB_TITLE_FIELD));
private static final long serialVersionUID = 1L;
private final String arn;
private final List awsServices;
private final String checkArn;
private final Instant createdAt;
private final String createdBy;
private final String description;
private final String id;
private final Instant lastUpdatedAt;
private final String lifecycleStage;
private final String name;
private final RecommendationPillarSpecificAggregates pillarSpecificAggregates;
private final List pillars;
private final Instant resolvedAt;
private final RecommendationResourcesAggregates resourcesAggregates;
private final String source;
private final String status;
private final String type;
private final String updateReason;
private final String updateReasonCode;
private final String updatedOnBehalfOf;
private final String updatedOnBehalfOfJobTitle;
private Recommendation(BuilderImpl builder) {
this.arn = builder.arn;
this.awsServices = builder.awsServices;
this.checkArn = builder.checkArn;
this.createdAt = builder.createdAt;
this.createdBy = builder.createdBy;
this.description = builder.description;
this.id = builder.id;
this.lastUpdatedAt = builder.lastUpdatedAt;
this.lifecycleStage = builder.lifecycleStage;
this.name = builder.name;
this.pillarSpecificAggregates = builder.pillarSpecificAggregates;
this.pillars = builder.pillars;
this.resolvedAt = builder.resolvedAt;
this.resourcesAggregates = builder.resourcesAggregates;
this.source = builder.source;
this.status = builder.status;
this.type = builder.type;
this.updateReason = builder.updateReason;
this.updateReasonCode = builder.updateReasonCode;
this.updatedOnBehalfOf = builder.updatedOnBehalfOf;
this.updatedOnBehalfOfJobTitle = builder.updatedOnBehalfOfJobTitle;
}
/**
*
* The ARN of the Recommendation
*
*
* @return The ARN of the Recommendation
*/
public final String arn() {
return arn;
}
/**
* For responses, this returns true if the service returned a value for the AwsServices property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasAwsServices() {
return awsServices != null && !(awsServices instanceof SdkAutoConstructList);
}
/**
*
* The AWS Services that the Recommendation applies to
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasAwsServices} method.
*
*
* @return The AWS Services that the Recommendation applies to
*/
public final List awsServices() {
return awsServices;
}
/**
*
* The AWS Trusted Advisor Check ARN that relates to the Recommendation
*
*
* @return The AWS Trusted Advisor Check ARN that relates to the Recommendation
*/
public final String checkArn() {
return checkArn;
}
/**
*
* When the Recommendation was created, if created by AWS Trusted Advisor Priority
*
*
* @return When the Recommendation was created, if created by AWS Trusted Advisor Priority
*/
public final Instant createdAt() {
return createdAt;
}
/**
*
* The creator, if created by AWS Trusted Advisor Priority
*
*
* @return The creator, if created by AWS Trusted Advisor Priority
*/
public final String createdBy() {
return createdBy;
}
/**
*
* A description for AWS Trusted Advisor recommendations
*
*
* @return A description for AWS Trusted Advisor recommendations
*/
public final String description() {
return description;
}
/**
*
* The ID which identifies where the Recommendation was produced
*
*
* @return The ID which identifies where the Recommendation was produced
*/
public final String id() {
return id;
}
/**
*
* When the Recommendation was last updated
*
*
* @return When the Recommendation was last updated
*/
public final Instant lastUpdatedAt() {
return lastUpdatedAt;
}
/**
*
* The lifecycle stage from AWS Trusted Advisor Priority
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #lifecycleStage}
* will return {@link RecommendationLifecycleStage#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #lifecycleStageAsString}.
*
*
* @return The lifecycle stage from AWS Trusted Advisor Priority
* @see RecommendationLifecycleStage
*/
public final RecommendationLifecycleStage lifecycleStage() {
return RecommendationLifecycleStage.fromValue(lifecycleStage);
}
/**
*
* The lifecycle stage from AWS Trusted Advisor Priority
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #lifecycleStage}
* will return {@link RecommendationLifecycleStage#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #lifecycleStageAsString}.
*
*
* @return The lifecycle stage from AWS Trusted Advisor Priority
* @see RecommendationLifecycleStage
*/
public final String lifecycleStageAsString() {
return lifecycleStage;
}
/**
*
* The name of the AWS Trusted Advisor Recommendation
*
*
* @return The name of the AWS Trusted Advisor Recommendation
*/
public final String name() {
return name;
}
/**
*
* The pillar aggregations for cost savings
*
*
* @return The pillar aggregations for cost savings
*/
public final RecommendationPillarSpecificAggregates pillarSpecificAggregates() {
return pillarSpecificAggregates;
}
/**
*
* The Pillars that the Recommendation is optimizing
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasPillars} method.
*
*
* @return The Pillars that the Recommendation is optimizing
*/
public final List pillars() {
return RecommendationPillarListCopier.copyStringToEnum(pillars);
}
/**
* For responses, this returns true if the service returned a value for the Pillars property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasPillars() {
return pillars != null && !(pillars instanceof SdkAutoConstructList);
}
/**
*
* The Pillars that the Recommendation is optimizing
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasPillars} method.
*
*
* @return The Pillars that the Recommendation is optimizing
*/
public final List pillarsAsStrings() {
return pillars;
}
/**
*
* When the Recommendation was resolved
*
*
* @return When the Recommendation was resolved
*/
public final Instant resolvedAt() {
return resolvedAt;
}
/**
*
* An aggregation of all resources
*
*
* @return An aggregation of all resources
*/
public final RecommendationResourcesAggregates resourcesAggregates() {
return resourcesAggregates;
}
/**
*
* The source of the Recommendation
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #source} will
* return {@link RecommendationSource#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #sourceAsString}.
*
*
* @return The source of the Recommendation
* @see RecommendationSource
*/
public final RecommendationSource source() {
return RecommendationSource.fromValue(source);
}
/**
*
* The source of the Recommendation
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #source} will
* return {@link RecommendationSource#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #sourceAsString}.
*
*
* @return The source of the Recommendation
* @see RecommendationSource
*/
public final String sourceAsString() {
return source;
}
/**
*
* The status of the Recommendation
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link RecommendationStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #statusAsString}.
*
*
* @return The status of the Recommendation
* @see RecommendationStatus
*/
public final RecommendationStatus status() {
return RecommendationStatus.fromValue(status);
}
/**
*
* The status of the Recommendation
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link RecommendationStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #statusAsString}.
*
*
* @return The status of the Recommendation
* @see RecommendationStatus
*/
public final String statusAsString() {
return status;
}
/**
*
* Whether the Recommendation was automated or generated by AWS Trusted Advisor Priority
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #type} will return
* {@link RecommendationType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #typeAsString}.
*
*
* @return Whether the Recommendation was automated or generated by AWS Trusted Advisor Priority
* @see RecommendationType
*/
public final RecommendationType type() {
return RecommendationType.fromValue(type);
}
/**
*
* Whether the Recommendation was automated or generated by AWS Trusted Advisor Priority
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #type} will return
* {@link RecommendationType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #typeAsString}.
*
*
* @return Whether the Recommendation was automated or generated by AWS Trusted Advisor Priority
* @see RecommendationType
*/
public final String typeAsString() {
return type;
}
/**
*
* Reason for the lifecycle stage change
*
*
* @return Reason for the lifecycle stage change
*/
public final String updateReason() {
return updateReason;
}
/**
*
* Reason code for the lifecycle state change
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #updateReasonCode}
* will return {@link UpdateRecommendationLifecycleStageReasonCode#UNKNOWN_TO_SDK_VERSION}. The raw value returned
* by the service is available from {@link #updateReasonCodeAsString}.
*
*
* @return Reason code for the lifecycle state change
* @see UpdateRecommendationLifecycleStageReasonCode
*/
public final UpdateRecommendationLifecycleStageReasonCode updateReasonCode() {
return UpdateRecommendationLifecycleStageReasonCode.fromValue(updateReasonCode);
}
/**
*
* Reason code for the lifecycle state change
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #updateReasonCode}
* will return {@link UpdateRecommendationLifecycleStageReasonCode#UNKNOWN_TO_SDK_VERSION}. The raw value returned
* by the service is available from {@link #updateReasonCodeAsString}.
*
*
* @return Reason code for the lifecycle state change
* @see UpdateRecommendationLifecycleStageReasonCode
*/
public final String updateReasonCodeAsString() {
return updateReasonCode;
}
/**
*
* The person on whose behalf a Technical Account Manager (TAM) updated the recommendation. This information is only
* available when a Technical Account Manager takes an action on a recommendation managed by AWS Trusted Advisor
* Priority
*
*
* @return The person on whose behalf a Technical Account Manager (TAM) updated the recommendation. This information
* is only available when a Technical Account Manager takes an action on a recommendation managed by AWS
* Trusted Advisor Priority
*/
public final String updatedOnBehalfOf() {
return updatedOnBehalfOf;
}
/**
*
* The job title of the person on whose behalf a Technical Account Manager (TAM) updated the recommendation. This
* information is only available when a Technical Account Manager takes an action on a recommendation managed by AWS
* Trusted Advisor Priority
*
*
* @return The job title of the person on whose behalf a Technical Account Manager (TAM) updated the recommendation.
* This information is only available when a Technical Account Manager takes an action on a recommendation
* managed by AWS Trusted Advisor Priority
*/
public final String updatedOnBehalfOfJobTitle() {
return updatedOnBehalfOfJobTitle;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(arn());
hashCode = 31 * hashCode + Objects.hashCode(hasAwsServices() ? awsServices() : null);
hashCode = 31 * hashCode + Objects.hashCode(checkArn());
hashCode = 31 * hashCode + Objects.hashCode(createdAt());
hashCode = 31 * hashCode + Objects.hashCode(createdBy());
hashCode = 31 * hashCode + Objects.hashCode(description());
hashCode = 31 * hashCode + Objects.hashCode(id());
hashCode = 31 * hashCode + Objects.hashCode(lastUpdatedAt());
hashCode = 31 * hashCode + Objects.hashCode(lifecycleStageAsString());
hashCode = 31 * hashCode + Objects.hashCode(name());
hashCode = 31 * hashCode + Objects.hashCode(pillarSpecificAggregates());
hashCode = 31 * hashCode + Objects.hashCode(hasPillars() ? pillarsAsStrings() : null);
hashCode = 31 * hashCode + Objects.hashCode(resolvedAt());
hashCode = 31 * hashCode + Objects.hashCode(resourcesAggregates());
hashCode = 31 * hashCode + Objects.hashCode(sourceAsString());
hashCode = 31 * hashCode + Objects.hashCode(statusAsString());
hashCode = 31 * hashCode + Objects.hashCode(typeAsString());
hashCode = 31 * hashCode + Objects.hashCode(updateReason());
hashCode = 31 * hashCode + Objects.hashCode(updateReasonCodeAsString());
hashCode = 31 * hashCode + Objects.hashCode(updatedOnBehalfOf());
hashCode = 31 * hashCode + Objects.hashCode(updatedOnBehalfOfJobTitle());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof Recommendation)) {
return false;
}
Recommendation other = (Recommendation) obj;
return Objects.equals(arn(), other.arn()) && hasAwsServices() == other.hasAwsServices()
&& Objects.equals(awsServices(), other.awsServices()) && Objects.equals(checkArn(), other.checkArn())
&& Objects.equals(createdAt(), other.createdAt()) && Objects.equals(createdBy(), other.createdBy())
&& Objects.equals(description(), other.description()) && Objects.equals(id(), other.id())
&& Objects.equals(lastUpdatedAt(), other.lastUpdatedAt())
&& Objects.equals(lifecycleStageAsString(), other.lifecycleStageAsString())
&& Objects.equals(name(), other.name())
&& Objects.equals(pillarSpecificAggregates(), other.pillarSpecificAggregates())
&& hasPillars() == other.hasPillars() && Objects.equals(pillarsAsStrings(), other.pillarsAsStrings())
&& Objects.equals(resolvedAt(), other.resolvedAt())
&& Objects.equals(resourcesAggregates(), other.resourcesAggregates())
&& Objects.equals(sourceAsString(), other.sourceAsString())
&& Objects.equals(statusAsString(), other.statusAsString())
&& Objects.equals(typeAsString(), other.typeAsString()) && Objects.equals(updateReason(), other.updateReason())
&& Objects.equals(updateReasonCodeAsString(), other.updateReasonCodeAsString())
&& Objects.equals(updatedOnBehalfOf(), other.updatedOnBehalfOf())
&& Objects.equals(updatedOnBehalfOfJobTitle(), other.updatedOnBehalfOfJobTitle());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("Recommendation").add("Arn", arn()).add("AwsServices", hasAwsServices() ? awsServices() : null)
.add("CheckArn", checkArn()).add("CreatedAt", createdAt()).add("CreatedBy", createdBy())
.add("Description", description()).add("Id", id()).add("LastUpdatedAt", lastUpdatedAt())
.add("LifecycleStage", lifecycleStageAsString()).add("Name", name())
.add("PillarSpecificAggregates", pillarSpecificAggregates())
.add("Pillars", hasPillars() ? pillarsAsStrings() : null).add("ResolvedAt", resolvedAt())
.add("ResourcesAggregates", resourcesAggregates()).add("Source", sourceAsString())
.add("Status", statusAsString()).add("Type", typeAsString())
.add("UpdateReason", updateReason() == null ? null : "*** Sensitive Data Redacted ***")
.add("UpdateReasonCode", updateReasonCodeAsString()).add("UpdatedOnBehalfOf", updatedOnBehalfOf())
.add("UpdatedOnBehalfOfJobTitle", updatedOnBehalfOfJobTitle()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "arn":
return Optional.ofNullable(clazz.cast(arn()));
case "awsServices":
return Optional.ofNullable(clazz.cast(awsServices()));
case "checkArn":
return Optional.ofNullable(clazz.cast(checkArn()));
case "createdAt":
return Optional.ofNullable(clazz.cast(createdAt()));
case "createdBy":
return Optional.ofNullable(clazz.cast(createdBy()));
case "description":
return Optional.ofNullable(clazz.cast(description()));
case "id":
return Optional.ofNullable(clazz.cast(id()));
case "lastUpdatedAt":
return Optional.ofNullable(clazz.cast(lastUpdatedAt()));
case "lifecycleStage":
return Optional.ofNullable(clazz.cast(lifecycleStageAsString()));
case "name":
return Optional.ofNullable(clazz.cast(name()));
case "pillarSpecificAggregates":
return Optional.ofNullable(clazz.cast(pillarSpecificAggregates()));
case "pillars":
return Optional.ofNullable(clazz.cast(pillarsAsStrings()));
case "resolvedAt":
return Optional.ofNullable(clazz.cast(resolvedAt()));
case "resourcesAggregates":
return Optional.ofNullable(clazz.cast(resourcesAggregates()));
case "source":
return Optional.ofNullable(clazz.cast(sourceAsString()));
case "status":
return Optional.ofNullable(clazz.cast(statusAsString()));
case "type":
return Optional.ofNullable(clazz.cast(typeAsString()));
case "updateReason":
return Optional.ofNullable(clazz.cast(updateReason()));
case "updateReasonCode":
return Optional.ofNullable(clazz.cast(updateReasonCodeAsString()));
case "updatedOnBehalfOf":
return Optional.ofNullable(clazz.cast(updatedOnBehalfOf()));
case "updatedOnBehalfOfJobTitle":
return Optional.ofNullable(clazz.cast(updatedOnBehalfOfJobTitle()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function