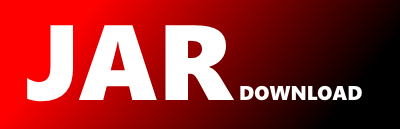
software.amazon.awssdk.services.waf.model.ActivatedRule Maven / Gradle / Ivy
Show all versions of waf Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.waf.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
*
* This is AWS WAF Classic documentation. For more information, see AWS WAF Classic in the
* developer guide.
*
*
* For the latest version of AWS WAF, use the AWS WAFV2 API and see the AWS WAF Developer Guide. With the
* latest version, AWS WAF has a single set of endpoints for regional and global use.
*
*
*
* The ActivatedRule
object in an UpdateWebACL request specifies a Rule
that you want
* to insert or delete, the priority of the Rule
in the WebACL
, and the action that you want
* AWS WAF to take when a web request matches the Rule
(ALLOW
, BLOCK
, or
* COUNT
).
*
*
* To specify whether to insert or delete a Rule
, use the Action
parameter in the
* WebACLUpdate data type.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ActivatedRule implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField PRIORITY_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("Priority").getter(getter(ActivatedRule::priority)).setter(setter(Builder::priority))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Priority").build()).build();
private static final SdkField RULE_ID_FIELD = SdkField. builder(MarshallingType.STRING).memberName("RuleId")
.getter(getter(ActivatedRule::ruleId)).setter(setter(Builder::ruleId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RuleId").build()).build();
private static final SdkField ACTION_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("Action").getter(getter(ActivatedRule::action)).setter(setter(Builder::action))
.constructor(WafAction::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Action").build()).build();
private static final SdkField OVERRIDE_ACTION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("OverrideAction")
.getter(getter(ActivatedRule::overrideAction)).setter(setter(Builder::overrideAction))
.constructor(WafOverrideAction::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OverrideAction").build()).build();
private static final SdkField TYPE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Type")
.getter(getter(ActivatedRule::typeAsString)).setter(setter(Builder::type))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Type").build()).build();
private static final SdkField> EXCLUDED_RULES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("ExcludedRules")
.getter(getter(ActivatedRule::excludedRules))
.setter(setter(Builder::excludedRules))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ExcludedRules").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(ExcludedRule::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(PRIORITY_FIELD, RULE_ID_FIELD,
ACTION_FIELD, OVERRIDE_ACTION_FIELD, TYPE_FIELD, EXCLUDED_RULES_FIELD));
private static final long serialVersionUID = 1L;
private final Integer priority;
private final String ruleId;
private final WafAction action;
private final WafOverrideAction overrideAction;
private final String type;
private final List excludedRules;
private ActivatedRule(BuilderImpl builder) {
this.priority = builder.priority;
this.ruleId = builder.ruleId;
this.action = builder.action;
this.overrideAction = builder.overrideAction;
this.type = builder.type;
this.excludedRules = builder.excludedRules;
}
/**
*
* Specifies the order in which the Rules
in a WebACL
are evaluated. Rules with a lower
* value for Priority
are evaluated before Rules
with a higher value. The value must be a
* unique integer. If you add multiple Rules
to a WebACL
, the values don't need to be
* consecutive.
*
*
* @return Specifies the order in which the Rules
in a WebACL
are evaluated. Rules with a
* lower value for Priority
are evaluated before Rules
with a higher value. The
* value must be a unique integer. If you add multiple Rules
to a WebACL
, the
* values don't need to be consecutive.
*/
public final Integer priority() {
return priority;
}
/**
*
* The RuleId
for a Rule
. You use RuleId
to get more information about a
* Rule
(see GetRule), update a Rule
(see UpdateRule), insert a
* Rule
into a WebACL
or delete a one from a WebACL
(see
* UpdateWebACL), or delete a Rule
from AWS WAF (see DeleteRule).
*
*
* RuleId
is returned by CreateRule and by ListRules.
*
*
* @return The RuleId
for a Rule
. You use RuleId
to get more information
* about a Rule
(see GetRule), update a Rule
(see UpdateRule),
* insert a Rule
into a WebACL
or delete a one from a WebACL
(see
* UpdateWebACL), or delete a Rule
from AWS WAF (see DeleteRule).
*
* RuleId
is returned by CreateRule and by ListRules.
*/
public final String ruleId() {
return ruleId;
}
/**
*
* Specifies the action that CloudFront or AWS WAF takes when a web request matches the conditions in the
* Rule
. Valid values for Action
include the following:
*
*
* -
*
* ALLOW
: CloudFront responds with the requested object.
*
*
* -
*
* BLOCK
: CloudFront responds with an HTTP 403 (Forbidden) status code.
*
*
* -
*
* COUNT
: AWS WAF increments a counter of requests that match the conditions in the rule and then
* continues to inspect the web request based on the remaining rules in the web ACL.
*
*
*
*
* ActivatedRule|OverrideAction
applies only when updating or adding a RuleGroup
to a
* WebACL
. In this case, you do not use ActivatedRule|Action
. For all other update
* requests, ActivatedRule|Action
is used instead of ActivatedRule|OverrideAction
.
*
*
* @return Specifies the action that CloudFront or AWS WAF takes when a web request matches the conditions in the
* Rule
. Valid values for Action
include the following:
*
* -
*
* ALLOW
: CloudFront responds with the requested object.
*
*
* -
*
* BLOCK
: CloudFront responds with an HTTP 403 (Forbidden) status code.
*
*
* -
*
* COUNT
: AWS WAF increments a counter of requests that match the conditions in the rule and
* then continues to inspect the web request based on the remaining rules in the web ACL.
*
*
*
*
* ActivatedRule|OverrideAction
applies only when updating or adding a RuleGroup
* to a WebACL
. In this case, you do not use ActivatedRule|Action
. For all other
* update requests, ActivatedRule|Action
is used instead of
* ActivatedRule|OverrideAction
.
*/
public final WafAction action() {
return action;
}
/**
*
* Use the OverrideAction
to test your RuleGroup
.
*
*
* Any rule in a RuleGroup
can potentially block a request. If you set the OverrideAction
* to None
, the RuleGroup
will block a request if any individual rule in the
* RuleGroup
matches the request and is configured to block that request. However if you first want to
* test the RuleGroup
, set the OverrideAction
to Count
. The
* RuleGroup
will then override any block action specified by individual rules contained within the
* group. Instead of blocking matching requests, those requests will be counted. You can view a record of counted
* requests using GetSampledRequests.
*
*
* ActivatedRule|OverrideAction
applies only when updating or adding a RuleGroup
to a
* WebACL
. In this case you do not use ActivatedRule|Action
. For all other update
* requests, ActivatedRule|Action
is used instead of ActivatedRule|OverrideAction
.
*
*
* @return Use the OverrideAction
to test your RuleGroup
.
*
* Any rule in a RuleGroup
can potentially block a request. If you set the
* OverrideAction
to None
, the RuleGroup
will block a request if any
* individual rule in the RuleGroup
matches the request and is configured to block that
* request. However if you first want to test the RuleGroup
, set the
* OverrideAction
to Count
. The RuleGroup
will then override any
* block action specified by individual rules contained within the group. Instead of blocking matching
* requests, those requests will be counted. You can view a record of counted requests using
* GetSampledRequests.
*
*
* ActivatedRule|OverrideAction
applies only when updating or adding a RuleGroup
* to a WebACL
. In this case you do not use ActivatedRule|Action
. For all other
* update requests, ActivatedRule|Action
is used instead of
* ActivatedRule|OverrideAction
.
*/
public final WafOverrideAction overrideAction() {
return overrideAction;
}
/**
*
* The rule type, either REGULAR
, as defined by Rule, RATE_BASED
, as defined by
* RateBasedRule, or GROUP
, as defined by RuleGroup. The default is REGULAR. Although
* this field is optional, be aware that if you try to add a RATE_BASED rule to a web ACL without setting the type,
* the UpdateWebACL request will fail because the request tries to add a REGULAR rule with the specified ID,
* which does not exist.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #type} will return
* {@link WafRuleType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #typeAsString}.
*
*
* @return The rule type, either REGULAR
, as defined by Rule, RATE_BASED
, as
* defined by RateBasedRule, or GROUP
, as defined by RuleGroup. The default is
* REGULAR. Although this field is optional, be aware that if you try to add a RATE_BASED rule to a web ACL
* without setting the type, the UpdateWebACL request will fail because the request tries to add a
* REGULAR rule with the specified ID, which does not exist.
* @see WafRuleType
*/
public final WafRuleType type() {
return WafRuleType.fromValue(type);
}
/**
*
* The rule type, either REGULAR
, as defined by Rule, RATE_BASED
, as defined by
* RateBasedRule, or GROUP
, as defined by RuleGroup. The default is REGULAR. Although
* this field is optional, be aware that if you try to add a RATE_BASED rule to a web ACL without setting the type,
* the UpdateWebACL request will fail because the request tries to add a REGULAR rule with the specified ID,
* which does not exist.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #type} will return
* {@link WafRuleType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #typeAsString}.
*
*
* @return The rule type, either REGULAR
, as defined by Rule, RATE_BASED
, as
* defined by RateBasedRule, or GROUP
, as defined by RuleGroup. The default is
* REGULAR. Although this field is optional, be aware that if you try to add a RATE_BASED rule to a web ACL
* without setting the type, the UpdateWebACL request will fail because the request tries to add a
* REGULAR rule with the specified ID, which does not exist.
* @see WafRuleType
*/
public final String typeAsString() {
return type;
}
/**
* For responses, this returns true if the service returned a value for the ExcludedRules property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasExcludedRules() {
return excludedRules != null && !(excludedRules instanceof SdkAutoConstructList);
}
/**
*
* An array of rules to exclude from a rule group. This is applicable only when the ActivatedRule
* refers to a RuleGroup
.
*
*
* Sometimes it is necessary to troubleshoot rule groups that are blocking traffic unexpectedly (false positives).
* One troubleshooting technique is to identify the specific rule within the rule group that is blocking the
* legitimate traffic and then disable (exclude) that particular rule. You can exclude rules from both your own rule
* groups and AWS Marketplace rule groups that have been associated with a web ACL.
*
*
* Specifying ExcludedRules
does not remove those rules from the rule group. Rather, it changes the
* action for the rules to COUNT
. Therefore, requests that match an ExcludedRule
are
* counted but not blocked. The RuleGroup
owner will receive COUNT metrics for each
* ExcludedRule
.
*
*
* If you want to exclude rules from a rule group that is already associated with a web ACL, perform the following
* steps:
*
*
* -
*
* Use the AWS WAF logs to identify the IDs of the rules that you want to exclude. For more information about the
* logs, see Logging Web ACL Traffic
* Information.
*
*
* -
*
* Submit an UpdateWebACL request that has two actions:
*
*
* -
*
* The first action deletes the existing rule group from the web ACL. That is, in the UpdateWebACL request,
* the first Updates:Action
should be DELETE
and Updates:ActivatedRule:RuleId
* should be the rule group that contains the rules that you want to exclude.
*
*
* -
*
* The second action inserts the same rule group back in, but specifying the rules to exclude. That is, the second
* Updates:Action
should be INSERT
, Updates:ActivatedRule:RuleId
should be
* the rule group that you just removed, and ExcludedRules
should contain the rules that you want to
* exclude.
*
*
*
*
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasExcludedRules} method.
*
*
* @return An array of rules to exclude from a rule group. This is applicable only when the
* ActivatedRule
refers to a RuleGroup
.
*
* Sometimes it is necessary to troubleshoot rule groups that are blocking traffic unexpectedly (false
* positives). One troubleshooting technique is to identify the specific rule within the rule group that is
* blocking the legitimate traffic and then disable (exclude) that particular rule. You can exclude rules
* from both your own rule groups and AWS Marketplace rule groups that have been associated with a web ACL.
*
*
* Specifying ExcludedRules
does not remove those rules from the rule group. Rather, it changes
* the action for the rules to COUNT
. Therefore, requests that match an
* ExcludedRule
are counted but not blocked. The RuleGroup
owner will receive
* COUNT metrics for each ExcludedRule
.
*
*
* If you want to exclude rules from a rule group that is already associated with a web ACL, perform the
* following steps:
*
*
* -
*
* Use the AWS WAF logs to identify the IDs of the rules that you want to exclude. For more information
* about the logs, see Logging
* Web ACL Traffic Information.
*
*
* -
*
* Submit an UpdateWebACL request that has two actions:
*
*
* -
*
* The first action deletes the existing rule group from the web ACL. That is, in the UpdateWebACL
* request, the first Updates:Action
should be DELETE
and
* Updates:ActivatedRule:RuleId
should be the rule group that contains the rules that you want
* to exclude.
*
*
* -
*
* The second action inserts the same rule group back in, but specifying the rules to exclude. That is, the
* second Updates:Action
should be INSERT
,
* Updates:ActivatedRule:RuleId
should be the rule group that you just removed, and
* ExcludedRules
should contain the rules that you want to exclude.
*
*
*
*
*/
public final List excludedRules() {
return excludedRules;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(priority());
hashCode = 31 * hashCode + Objects.hashCode(ruleId());
hashCode = 31 * hashCode + Objects.hashCode(action());
hashCode = 31 * hashCode + Objects.hashCode(overrideAction());
hashCode = 31 * hashCode + Objects.hashCode(typeAsString());
hashCode = 31 * hashCode + Objects.hashCode(hasExcludedRules() ? excludedRules() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ActivatedRule)) {
return false;
}
ActivatedRule other = (ActivatedRule) obj;
return Objects.equals(priority(), other.priority()) && Objects.equals(ruleId(), other.ruleId())
&& Objects.equals(action(), other.action()) && Objects.equals(overrideAction(), other.overrideAction())
&& Objects.equals(typeAsString(), other.typeAsString()) && hasExcludedRules() == other.hasExcludedRules()
&& Objects.equals(excludedRules(), other.excludedRules());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ActivatedRule").add("Priority", priority()).add("RuleId", ruleId()).add("Action", action())
.add("OverrideAction", overrideAction()).add("Type", typeAsString())
.add("ExcludedRules", hasExcludedRules() ? excludedRules() : null).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Priority":
return Optional.ofNullable(clazz.cast(priority()));
case "RuleId":
return Optional.ofNullable(clazz.cast(ruleId()));
case "Action":
return Optional.ofNullable(clazz.cast(action()));
case "OverrideAction":
return Optional.ofNullable(clazz.cast(overrideAction()));
case "Type":
return Optional.ofNullable(clazz.cast(typeAsString()));
case "ExcludedRules":
return Optional.ofNullable(clazz.cast(excludedRules()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function