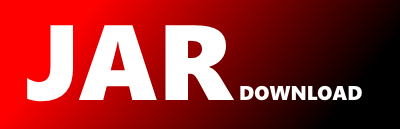
software.amazon.awssdk.services.wellarchitected.model.CheckDetail Maven / Gradle / Ivy
Show all versions of wellarchitected Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.wellarchitected.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Account details for a Well-Architected best practice in relation to Trusted Advisor checks.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class CheckDetail implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField ID_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Id")
.getter(getter(CheckDetail::id)).setter(setter(Builder::id))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Id").build()).build();
private static final SdkField NAME_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Name")
.getter(getter(CheckDetail::name)).setter(setter(Builder::name))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Name").build()).build();
private static final SdkField DESCRIPTION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Description").getter(getter(CheckDetail::description)).setter(setter(Builder::description))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Description").build()).build();
private static final SdkField PROVIDER_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Provider").getter(getter(CheckDetail::providerAsString)).setter(setter(Builder::provider))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Provider").build()).build();
private static final SdkField LENS_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("LensArn").getter(getter(CheckDetail::lensArn)).setter(setter(Builder::lensArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LensArn").build()).build();
private static final SdkField PILLAR_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("PillarId").getter(getter(CheckDetail::pillarId)).setter(setter(Builder::pillarId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PillarId").build()).build();
private static final SdkField QUESTION_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("QuestionId").getter(getter(CheckDetail::questionId)).setter(setter(Builder::questionId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("QuestionId").build()).build();
private static final SdkField CHOICE_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ChoiceId").getter(getter(CheckDetail::choiceId)).setter(setter(Builder::choiceId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ChoiceId").build()).build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Status")
.getter(getter(CheckDetail::statusAsString)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Status").build()).build();
private static final SdkField ACCOUNT_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AccountId").getter(getter(CheckDetail::accountId)).setter(setter(Builder::accountId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AccountId").build()).build();
private static final SdkField FLAGGED_RESOURCES_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("FlaggedResources").getter(getter(CheckDetail::flaggedResources))
.setter(setter(Builder::flaggedResources))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("FlaggedResources").build()).build();
private static final SdkField REASON_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Reason")
.getter(getter(CheckDetail::reasonAsString)).setter(setter(Builder::reason))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Reason").build()).build();
private static final SdkField UPDATED_AT_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("UpdatedAt").getter(getter(CheckDetail::updatedAt)).setter(setter(Builder::updatedAt))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("UpdatedAt").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ID_FIELD, NAME_FIELD,
DESCRIPTION_FIELD, PROVIDER_FIELD, LENS_ARN_FIELD, PILLAR_ID_FIELD, QUESTION_ID_FIELD, CHOICE_ID_FIELD, STATUS_FIELD,
ACCOUNT_ID_FIELD, FLAGGED_RESOURCES_FIELD, REASON_FIELD, UPDATED_AT_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("Id", ID_FIELD);
put("Name", NAME_FIELD);
put("Description", DESCRIPTION_FIELD);
put("Provider", PROVIDER_FIELD);
put("LensArn", LENS_ARN_FIELD);
put("PillarId", PILLAR_ID_FIELD);
put("QuestionId", QUESTION_ID_FIELD);
put("ChoiceId", CHOICE_ID_FIELD);
put("Status", STATUS_FIELD);
put("AccountId", ACCOUNT_ID_FIELD);
put("FlaggedResources", FLAGGED_RESOURCES_FIELD);
put("Reason", REASON_FIELD);
put("UpdatedAt", UPDATED_AT_FIELD);
}
});
private static final long serialVersionUID = 1L;
private final String id;
private final String name;
private final String description;
private final String provider;
private final String lensArn;
private final String pillarId;
private final String questionId;
private final String choiceId;
private final String status;
private final String accountId;
private final Integer flaggedResources;
private final String reason;
private final Instant updatedAt;
private CheckDetail(BuilderImpl builder) {
this.id = builder.id;
this.name = builder.name;
this.description = builder.description;
this.provider = builder.provider;
this.lensArn = builder.lensArn;
this.pillarId = builder.pillarId;
this.questionId = builder.questionId;
this.choiceId = builder.choiceId;
this.status = builder.status;
this.accountId = builder.accountId;
this.flaggedResources = builder.flaggedResources;
this.reason = builder.reason;
this.updatedAt = builder.updatedAt;
}
/**
*
* Trusted Advisor check ID.
*
*
* @return Trusted Advisor check ID.
*/
public final String id() {
return id;
}
/**
*
* Trusted Advisor check name.
*
*
* @return Trusted Advisor check name.
*/
public final String name() {
return name;
}
/**
*
* Trusted Advisor check description.
*
*
* @return Trusted Advisor check description.
*/
public final String description() {
return description;
}
/**
*
* Provider of the check related to the best practice.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #provider} will
* return {@link CheckProvider#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #providerAsString}.
*
*
* @return Provider of the check related to the best practice.
* @see CheckProvider
*/
public final CheckProvider provider() {
return CheckProvider.fromValue(provider);
}
/**
*
* Provider of the check related to the best practice.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #provider} will
* return {@link CheckProvider#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #providerAsString}.
*
*
* @return Provider of the check related to the best practice.
* @see CheckProvider
*/
public final String providerAsString() {
return provider;
}
/**
*
* Well-Architected Lens ARN associated to the check.
*
*
* @return Well-Architected Lens ARN associated to the check.
*/
public final String lensArn() {
return lensArn;
}
/**
* Returns the value of the PillarId property for this object.
*
* @return The value of the PillarId property for this object.
*/
public final String pillarId() {
return pillarId;
}
/**
* Returns the value of the QuestionId property for this object.
*
* @return The value of the QuestionId property for this object.
*/
public final String questionId() {
return questionId;
}
/**
* Returns the value of the ChoiceId property for this object.
*
* @return The value of the ChoiceId property for this object.
*/
public final String choiceId() {
return choiceId;
}
/**
*
* Status associated to the check.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link CheckStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statusAsString}.
*
*
* @return Status associated to the check.
* @see CheckStatus
*/
public final CheckStatus status() {
return CheckStatus.fromValue(status);
}
/**
*
* Status associated to the check.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link CheckStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statusAsString}.
*
*
* @return Status associated to the check.
* @see CheckStatus
*/
public final String statusAsString() {
return status;
}
/**
* Returns the value of the AccountId property for this object.
*
* @return The value of the AccountId property for this object.
*/
public final String accountId() {
return accountId;
}
/**
*
* Count of flagged resources associated to the check.
*
*
* @return Count of flagged resources associated to the check.
*/
public final Integer flaggedResources() {
return flaggedResources;
}
/**
*
* Reason associated to the check.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #reason} will
* return {@link CheckFailureReason#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #reasonAsString}.
*
*
* @return Reason associated to the check.
* @see CheckFailureReason
*/
public final CheckFailureReason reason() {
return CheckFailureReason.fromValue(reason);
}
/**
*
* Reason associated to the check.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #reason} will
* return {@link CheckFailureReason#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #reasonAsString}.
*
*
* @return Reason associated to the check.
* @see CheckFailureReason
*/
public final String reasonAsString() {
return reason;
}
/**
* Returns the value of the UpdatedAt property for this object.
*
* @return The value of the UpdatedAt property for this object.
*/
public final Instant updatedAt() {
return updatedAt;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(id());
hashCode = 31 * hashCode + Objects.hashCode(name());
hashCode = 31 * hashCode + Objects.hashCode(description());
hashCode = 31 * hashCode + Objects.hashCode(providerAsString());
hashCode = 31 * hashCode + Objects.hashCode(lensArn());
hashCode = 31 * hashCode + Objects.hashCode(pillarId());
hashCode = 31 * hashCode + Objects.hashCode(questionId());
hashCode = 31 * hashCode + Objects.hashCode(choiceId());
hashCode = 31 * hashCode + Objects.hashCode(statusAsString());
hashCode = 31 * hashCode + Objects.hashCode(accountId());
hashCode = 31 * hashCode + Objects.hashCode(flaggedResources());
hashCode = 31 * hashCode + Objects.hashCode(reasonAsString());
hashCode = 31 * hashCode + Objects.hashCode(updatedAt());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CheckDetail)) {
return false;
}
CheckDetail other = (CheckDetail) obj;
return Objects.equals(id(), other.id()) && Objects.equals(name(), other.name())
&& Objects.equals(description(), other.description())
&& Objects.equals(providerAsString(), other.providerAsString()) && Objects.equals(lensArn(), other.lensArn())
&& Objects.equals(pillarId(), other.pillarId()) && Objects.equals(questionId(), other.questionId())
&& Objects.equals(choiceId(), other.choiceId()) && Objects.equals(statusAsString(), other.statusAsString())
&& Objects.equals(accountId(), other.accountId()) && Objects.equals(flaggedResources(), other.flaggedResources())
&& Objects.equals(reasonAsString(), other.reasonAsString()) && Objects.equals(updatedAt(), other.updatedAt());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("CheckDetail").add("Id", id()).add("Name", name()).add("Description", description())
.add("Provider", providerAsString()).add("LensArn", lensArn()).add("PillarId", pillarId())
.add("QuestionId", questionId()).add("ChoiceId", choiceId()).add("Status", statusAsString())
.add("AccountId", accountId()).add("FlaggedResources", flaggedResources()).add("Reason", reasonAsString())
.add("UpdatedAt", updatedAt()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Id":
return Optional.ofNullable(clazz.cast(id()));
case "Name":
return Optional.ofNullable(clazz.cast(name()));
case "Description":
return Optional.ofNullable(clazz.cast(description()));
case "Provider":
return Optional.ofNullable(clazz.cast(providerAsString()));
case "LensArn":
return Optional.ofNullable(clazz.cast(lensArn()));
case "PillarId":
return Optional.ofNullable(clazz.cast(pillarId()));
case "QuestionId":
return Optional.ofNullable(clazz.cast(questionId()));
case "ChoiceId":
return Optional.ofNullable(clazz.cast(choiceId()));
case "Status":
return Optional.ofNullable(clazz.cast(statusAsString()));
case "AccountId":
return Optional.ofNullable(clazz.cast(accountId()));
case "FlaggedResources":
return Optional.ofNullable(clazz.cast(flaggedResources()));
case "Reason":
return Optional.ofNullable(clazz.cast(reasonAsString()));
case "UpdatedAt":
return Optional.ofNullable(clazz.cast(updatedAt()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function