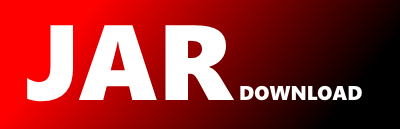
software.amazon.awssdk.services.wisdom.DefaultWisdomAsyncClient Maven / Gradle / Ivy
Show all versions of wisdom Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.wisdom;
import java.util.Collections;
import java.util.List;
import java.util.concurrent.CompletableFuture;
import java.util.function.Consumer;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.awscore.client.handler.AwsAsyncClientHandler;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.awscore.internal.AwsProtocolMetadata;
import software.amazon.awssdk.awscore.internal.AwsServiceProtocol;
import software.amazon.awssdk.awscore.retry.AwsRetryStrategy;
import software.amazon.awssdk.core.RequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkPlugin;
import software.amazon.awssdk.core.SdkRequest;
import software.amazon.awssdk.core.client.config.ClientOverrideConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientOption;
import software.amazon.awssdk.core.client.handler.AsyncClientHandler;
import software.amazon.awssdk.core.client.handler.ClientExecutionParams;
import software.amazon.awssdk.core.http.HttpResponseHandler;
import software.amazon.awssdk.core.metrics.CoreMetric;
import software.amazon.awssdk.core.retry.RetryMode;
import software.amazon.awssdk.metrics.MetricCollector;
import software.amazon.awssdk.metrics.MetricPublisher;
import software.amazon.awssdk.metrics.NoOpMetricCollector;
import software.amazon.awssdk.protocols.core.ExceptionMetadata;
import software.amazon.awssdk.protocols.json.AwsJsonProtocol;
import software.amazon.awssdk.protocols.json.AwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.BaseAwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.JsonOperationMetadata;
import software.amazon.awssdk.retries.api.RetryStrategy;
import software.amazon.awssdk.services.wisdom.internal.WisdomServiceClientConfigurationBuilder;
import software.amazon.awssdk.services.wisdom.model.AccessDeniedException;
import software.amazon.awssdk.services.wisdom.model.ConflictException;
import software.amazon.awssdk.services.wisdom.model.CreateAssistantAssociationRequest;
import software.amazon.awssdk.services.wisdom.model.CreateAssistantAssociationResponse;
import software.amazon.awssdk.services.wisdom.model.CreateAssistantRequest;
import software.amazon.awssdk.services.wisdom.model.CreateAssistantResponse;
import software.amazon.awssdk.services.wisdom.model.CreateContentRequest;
import software.amazon.awssdk.services.wisdom.model.CreateContentResponse;
import software.amazon.awssdk.services.wisdom.model.CreateKnowledgeBaseRequest;
import software.amazon.awssdk.services.wisdom.model.CreateKnowledgeBaseResponse;
import software.amazon.awssdk.services.wisdom.model.CreateQuickResponseRequest;
import software.amazon.awssdk.services.wisdom.model.CreateQuickResponseResponse;
import software.amazon.awssdk.services.wisdom.model.CreateSessionRequest;
import software.amazon.awssdk.services.wisdom.model.CreateSessionResponse;
import software.amazon.awssdk.services.wisdom.model.DeleteAssistantAssociationRequest;
import software.amazon.awssdk.services.wisdom.model.DeleteAssistantAssociationResponse;
import software.amazon.awssdk.services.wisdom.model.DeleteAssistantRequest;
import software.amazon.awssdk.services.wisdom.model.DeleteAssistantResponse;
import software.amazon.awssdk.services.wisdom.model.DeleteContentRequest;
import software.amazon.awssdk.services.wisdom.model.DeleteContentResponse;
import software.amazon.awssdk.services.wisdom.model.DeleteImportJobRequest;
import software.amazon.awssdk.services.wisdom.model.DeleteImportJobResponse;
import software.amazon.awssdk.services.wisdom.model.DeleteKnowledgeBaseRequest;
import software.amazon.awssdk.services.wisdom.model.DeleteKnowledgeBaseResponse;
import software.amazon.awssdk.services.wisdom.model.DeleteQuickResponseRequest;
import software.amazon.awssdk.services.wisdom.model.DeleteQuickResponseResponse;
import software.amazon.awssdk.services.wisdom.model.GetAssistantAssociationRequest;
import software.amazon.awssdk.services.wisdom.model.GetAssistantAssociationResponse;
import software.amazon.awssdk.services.wisdom.model.GetAssistantRequest;
import software.amazon.awssdk.services.wisdom.model.GetAssistantResponse;
import software.amazon.awssdk.services.wisdom.model.GetContentRequest;
import software.amazon.awssdk.services.wisdom.model.GetContentResponse;
import software.amazon.awssdk.services.wisdom.model.GetContentSummaryRequest;
import software.amazon.awssdk.services.wisdom.model.GetContentSummaryResponse;
import software.amazon.awssdk.services.wisdom.model.GetImportJobRequest;
import software.amazon.awssdk.services.wisdom.model.GetImportJobResponse;
import software.amazon.awssdk.services.wisdom.model.GetKnowledgeBaseRequest;
import software.amazon.awssdk.services.wisdom.model.GetKnowledgeBaseResponse;
import software.amazon.awssdk.services.wisdom.model.GetQuickResponseRequest;
import software.amazon.awssdk.services.wisdom.model.GetQuickResponseResponse;
import software.amazon.awssdk.services.wisdom.model.GetRecommendationsRequest;
import software.amazon.awssdk.services.wisdom.model.GetRecommendationsResponse;
import software.amazon.awssdk.services.wisdom.model.GetSessionRequest;
import software.amazon.awssdk.services.wisdom.model.GetSessionResponse;
import software.amazon.awssdk.services.wisdom.model.ListAssistantAssociationsRequest;
import software.amazon.awssdk.services.wisdom.model.ListAssistantAssociationsResponse;
import software.amazon.awssdk.services.wisdom.model.ListAssistantsRequest;
import software.amazon.awssdk.services.wisdom.model.ListAssistantsResponse;
import software.amazon.awssdk.services.wisdom.model.ListContentsRequest;
import software.amazon.awssdk.services.wisdom.model.ListContentsResponse;
import software.amazon.awssdk.services.wisdom.model.ListImportJobsRequest;
import software.amazon.awssdk.services.wisdom.model.ListImportJobsResponse;
import software.amazon.awssdk.services.wisdom.model.ListKnowledgeBasesRequest;
import software.amazon.awssdk.services.wisdom.model.ListKnowledgeBasesResponse;
import software.amazon.awssdk.services.wisdom.model.ListQuickResponsesRequest;
import software.amazon.awssdk.services.wisdom.model.ListQuickResponsesResponse;
import software.amazon.awssdk.services.wisdom.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.wisdom.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.wisdom.model.NotifyRecommendationsReceivedRequest;
import software.amazon.awssdk.services.wisdom.model.NotifyRecommendationsReceivedResponse;
import software.amazon.awssdk.services.wisdom.model.PreconditionFailedException;
import software.amazon.awssdk.services.wisdom.model.QueryAssistantRequest;
import software.amazon.awssdk.services.wisdom.model.QueryAssistantResponse;
import software.amazon.awssdk.services.wisdom.model.RemoveKnowledgeBaseTemplateUriRequest;
import software.amazon.awssdk.services.wisdom.model.RemoveKnowledgeBaseTemplateUriResponse;
import software.amazon.awssdk.services.wisdom.model.RequestTimeoutException;
import software.amazon.awssdk.services.wisdom.model.ResourceNotFoundException;
import software.amazon.awssdk.services.wisdom.model.SearchContentRequest;
import software.amazon.awssdk.services.wisdom.model.SearchContentResponse;
import software.amazon.awssdk.services.wisdom.model.SearchQuickResponsesRequest;
import software.amazon.awssdk.services.wisdom.model.SearchQuickResponsesResponse;
import software.amazon.awssdk.services.wisdom.model.SearchSessionsRequest;
import software.amazon.awssdk.services.wisdom.model.SearchSessionsResponse;
import software.amazon.awssdk.services.wisdom.model.ServiceQuotaExceededException;
import software.amazon.awssdk.services.wisdom.model.StartContentUploadRequest;
import software.amazon.awssdk.services.wisdom.model.StartContentUploadResponse;
import software.amazon.awssdk.services.wisdom.model.StartImportJobRequest;
import software.amazon.awssdk.services.wisdom.model.StartImportJobResponse;
import software.amazon.awssdk.services.wisdom.model.TagResourceRequest;
import software.amazon.awssdk.services.wisdom.model.TagResourceResponse;
import software.amazon.awssdk.services.wisdom.model.TooManyTagsException;
import software.amazon.awssdk.services.wisdom.model.UntagResourceRequest;
import software.amazon.awssdk.services.wisdom.model.UntagResourceResponse;
import software.amazon.awssdk.services.wisdom.model.UpdateContentRequest;
import software.amazon.awssdk.services.wisdom.model.UpdateContentResponse;
import software.amazon.awssdk.services.wisdom.model.UpdateKnowledgeBaseTemplateUriRequest;
import software.amazon.awssdk.services.wisdom.model.UpdateKnowledgeBaseTemplateUriResponse;
import software.amazon.awssdk.services.wisdom.model.UpdateQuickResponseRequest;
import software.amazon.awssdk.services.wisdom.model.UpdateQuickResponseResponse;
import software.amazon.awssdk.services.wisdom.model.ValidationException;
import software.amazon.awssdk.services.wisdom.model.WisdomException;
import software.amazon.awssdk.services.wisdom.transform.CreateAssistantAssociationRequestMarshaller;
import software.amazon.awssdk.services.wisdom.transform.CreateAssistantRequestMarshaller;
import software.amazon.awssdk.services.wisdom.transform.CreateContentRequestMarshaller;
import software.amazon.awssdk.services.wisdom.transform.CreateKnowledgeBaseRequestMarshaller;
import software.amazon.awssdk.services.wisdom.transform.CreateQuickResponseRequestMarshaller;
import software.amazon.awssdk.services.wisdom.transform.CreateSessionRequestMarshaller;
import software.amazon.awssdk.services.wisdom.transform.DeleteAssistantAssociationRequestMarshaller;
import software.amazon.awssdk.services.wisdom.transform.DeleteAssistantRequestMarshaller;
import software.amazon.awssdk.services.wisdom.transform.DeleteContentRequestMarshaller;
import software.amazon.awssdk.services.wisdom.transform.DeleteImportJobRequestMarshaller;
import software.amazon.awssdk.services.wisdom.transform.DeleteKnowledgeBaseRequestMarshaller;
import software.amazon.awssdk.services.wisdom.transform.DeleteQuickResponseRequestMarshaller;
import software.amazon.awssdk.services.wisdom.transform.GetAssistantAssociationRequestMarshaller;
import software.amazon.awssdk.services.wisdom.transform.GetAssistantRequestMarshaller;
import software.amazon.awssdk.services.wisdom.transform.GetContentRequestMarshaller;
import software.amazon.awssdk.services.wisdom.transform.GetContentSummaryRequestMarshaller;
import software.amazon.awssdk.services.wisdom.transform.GetImportJobRequestMarshaller;
import software.amazon.awssdk.services.wisdom.transform.GetKnowledgeBaseRequestMarshaller;
import software.amazon.awssdk.services.wisdom.transform.GetQuickResponseRequestMarshaller;
import software.amazon.awssdk.services.wisdom.transform.GetRecommendationsRequestMarshaller;
import software.amazon.awssdk.services.wisdom.transform.GetSessionRequestMarshaller;
import software.amazon.awssdk.services.wisdom.transform.ListAssistantAssociationsRequestMarshaller;
import software.amazon.awssdk.services.wisdom.transform.ListAssistantsRequestMarshaller;
import software.amazon.awssdk.services.wisdom.transform.ListContentsRequestMarshaller;
import software.amazon.awssdk.services.wisdom.transform.ListImportJobsRequestMarshaller;
import software.amazon.awssdk.services.wisdom.transform.ListKnowledgeBasesRequestMarshaller;
import software.amazon.awssdk.services.wisdom.transform.ListQuickResponsesRequestMarshaller;
import software.amazon.awssdk.services.wisdom.transform.ListTagsForResourceRequestMarshaller;
import software.amazon.awssdk.services.wisdom.transform.NotifyRecommendationsReceivedRequestMarshaller;
import software.amazon.awssdk.services.wisdom.transform.QueryAssistantRequestMarshaller;
import software.amazon.awssdk.services.wisdom.transform.RemoveKnowledgeBaseTemplateUriRequestMarshaller;
import software.amazon.awssdk.services.wisdom.transform.SearchContentRequestMarshaller;
import software.amazon.awssdk.services.wisdom.transform.SearchQuickResponsesRequestMarshaller;
import software.amazon.awssdk.services.wisdom.transform.SearchSessionsRequestMarshaller;
import software.amazon.awssdk.services.wisdom.transform.StartContentUploadRequestMarshaller;
import software.amazon.awssdk.services.wisdom.transform.StartImportJobRequestMarshaller;
import software.amazon.awssdk.services.wisdom.transform.TagResourceRequestMarshaller;
import software.amazon.awssdk.services.wisdom.transform.UntagResourceRequestMarshaller;
import software.amazon.awssdk.services.wisdom.transform.UpdateContentRequestMarshaller;
import software.amazon.awssdk.services.wisdom.transform.UpdateKnowledgeBaseTemplateUriRequestMarshaller;
import software.amazon.awssdk.services.wisdom.transform.UpdateQuickResponseRequestMarshaller;
import software.amazon.awssdk.utils.CompletableFutureUtils;
/**
* Internal implementation of {@link WisdomAsyncClient}.
*
* @see WisdomAsyncClient#builder()
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
final class DefaultWisdomAsyncClient implements WisdomAsyncClient {
private static final Logger log = LoggerFactory.getLogger(DefaultWisdomAsyncClient.class);
private static final AwsProtocolMetadata protocolMetadata = AwsProtocolMetadata.builder()
.serviceProtocol(AwsServiceProtocol.REST_JSON).build();
private final AsyncClientHandler clientHandler;
private final AwsJsonProtocolFactory protocolFactory;
private final SdkClientConfiguration clientConfiguration;
protected DefaultWisdomAsyncClient(SdkClientConfiguration clientConfiguration) {
this.clientHandler = new AwsAsyncClientHandler(clientConfiguration);
this.clientConfiguration = clientConfiguration.toBuilder().option(SdkClientOption.SDK_CLIENT, this).build();
this.protocolFactory = init(AwsJsonProtocolFactory.builder()).build();
}
/**
*
* Creates an Amazon Connect Wisdom assistant.
*
*
* @param createAssistantRequest
* @return A Java Future containing the result of the CreateAssistant operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ConflictException The request could not be processed because of conflict in the current state of the
* resource. For example, if you're using a
Create
API (such as CreateAssistant
)
* that accepts name, a conflicting resource (usually with the same name) is being created or mutated.
* - ValidationException The input fails to satisfy the constraints specified by a service.
* - ServiceQuotaExceededException You've exceeded your service quota. To perform the requested action,
* remove some of the relevant resources, or use service quotas to request a service quota increase.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WisdomException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WisdomAsyncClient.CreateAssistant
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture createAssistant(CreateAssistantRequest createAssistantRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createAssistantRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createAssistantRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Wisdom");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateAssistant");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateAssistantResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateAssistant").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateAssistantRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createAssistantRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates an association between an Amazon Connect Wisdom assistant and another resource. Currently, the only
* supported association is with a knowledge base. An assistant can have only a single association.
*
*
* @param createAssistantAssociationRequest
* @return A Java Future containing the result of the CreateAssistantAssociation operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ConflictException The request could not be processed because of conflict in the current state of the
* resource. For example, if you're using a
Create
API (such as CreateAssistant
)
* that accepts name, a conflicting resource (usually with the same name) is being created or mutated.
* - ValidationException The input fails to satisfy the constraints specified by a service.
* - ServiceQuotaExceededException You've exceeded your service quota. To perform the requested action,
* remove some of the relevant resources, or use service quotas to request a service quota increase.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WisdomException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WisdomAsyncClient.CreateAssistantAssociation
* @see AWS API Documentation
*/
@Override
public CompletableFuture createAssistantAssociation(
CreateAssistantAssociationRequest createAssistantAssociationRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createAssistantAssociationRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createAssistantAssociationRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Wisdom");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateAssistantAssociation");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateAssistantAssociationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateAssistantAssociation").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateAssistantAssociationRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createAssistantAssociationRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates Wisdom content. Before to calling this API, use StartContentUpload
* to upload an asset.
*
*
* @param createContentRequest
* @return A Java Future containing the result of the CreateContent operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ConflictException The request could not be processed because of conflict in the current state of the
* resource. For example, if you're using a
Create
API (such as CreateAssistant
)
* that accepts name, a conflicting resource (usually with the same name) is being created or mutated.
* - ValidationException The input fails to satisfy the constraints specified by a service.
* - ServiceQuotaExceededException You've exceeded your service quota. To perform the requested action,
* remove some of the relevant resources, or use service quotas to request a service quota increase.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WisdomException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WisdomAsyncClient.CreateContent
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture createContent(CreateContentRequest createContentRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createContentRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createContentRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Wisdom");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateContent");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateContentResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateContent").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateContentRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createContentRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a knowledge base.
*
*
*
* When using this API, you cannot reuse Amazon AppIntegrations
* DataIntegrations with external knowledge bases such as Salesforce and ServiceNow. If you do, you'll get an
* InvalidRequestException
error.
*
*
* For example, you're programmatically managing your external knowledge base, and you want to add or remove one of
* the fields that is being ingested from Salesforce. Do the following:
*
*
* -
*
* Call DeleteKnowledgeBase
* .
*
*
* -
*
* Call
* DeleteDataIntegration.
*
*
* -
*
* Call
* CreateDataIntegration to recreate the DataIntegration or a create different one.
*
*
* -
*
* Call CreateKnowledgeBase.
*
*
*
*
*
* @param createKnowledgeBaseRequest
* @return A Java Future containing the result of the CreateKnowledgeBase operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ConflictException The request could not be processed because of conflict in the current state of the
* resource. For example, if you're using a
Create
API (such as CreateAssistant
)
* that accepts name, a conflicting resource (usually with the same name) is being created or mutated.
* - ValidationException The input fails to satisfy the constraints specified by a service.
* - ServiceQuotaExceededException You've exceeded your service quota. To perform the requested action,
* remove some of the relevant resources, or use service quotas to request a service quota increase.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WisdomException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WisdomAsyncClient.CreateKnowledgeBase
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture createKnowledgeBase(
CreateKnowledgeBaseRequest createKnowledgeBaseRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createKnowledgeBaseRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createKnowledgeBaseRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Wisdom");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateKnowledgeBase");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateKnowledgeBaseResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateKnowledgeBase").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateKnowledgeBaseRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createKnowledgeBaseRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a Wisdom quick response.
*
*
* @param createQuickResponseRequest
* @return A Java Future containing the result of the CreateQuickResponse operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ConflictException The request could not be processed because of conflict in the current state of the
* resource. For example, if you're using a
Create
API (such as CreateAssistant
)
* that accepts name, a conflicting resource (usually with the same name) is being created or mutated.
* - ValidationException The input fails to satisfy the constraints specified by a service.
* - ServiceQuotaExceededException You've exceeded your service quota. To perform the requested action,
* remove some of the relevant resources, or use service quotas to request a service quota increase.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WisdomException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WisdomAsyncClient.CreateQuickResponse
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture createQuickResponse(
CreateQuickResponseRequest createQuickResponseRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createQuickResponseRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createQuickResponseRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Wisdom");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateQuickResponse");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateQuickResponseResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateQuickResponse").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateQuickResponseRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createQuickResponseRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a session. A session is a contextual container used for generating recommendations. Amazon Connect
* creates a new Wisdom session for each contact on which Wisdom is enabled.
*
*
* @param createSessionRequest
* @return A Java Future containing the result of the CreateSession operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ConflictException The request could not be processed because of conflict in the current state of the
* resource. For example, if you're using a
Create
API (such as CreateAssistant
)
* that accepts name, a conflicting resource (usually with the same name) is being created or mutated.
* - ValidationException The input fails to satisfy the constraints specified by a service.
* - ResourceNotFoundException The specified resource does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WisdomException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WisdomAsyncClient.CreateSession
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture createSession(CreateSessionRequest createSessionRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createSessionRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createSessionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Wisdom");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateSession");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateSessionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateSession").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateSessionRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createSessionRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes an assistant.
*
*
* @param deleteAssistantRequest
* @return A Java Future containing the result of the DeleteAssistant operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the constraints specified by a service.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WisdomException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WisdomAsyncClient.DeleteAssistant
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deleteAssistant(DeleteAssistantRequest deleteAssistantRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteAssistantRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteAssistantRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Wisdom");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteAssistant");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteAssistantResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteAssistant").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteAssistantRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteAssistantRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes an assistant association.
*
*
* @param deleteAssistantAssociationRequest
* @return A Java Future containing the result of the DeleteAssistantAssociation operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the constraints specified by a service.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WisdomException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WisdomAsyncClient.DeleteAssistantAssociation
* @see AWS API Documentation
*/
@Override
public CompletableFuture deleteAssistantAssociation(
DeleteAssistantAssociationRequest deleteAssistantAssociationRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteAssistantAssociationRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteAssistantAssociationRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Wisdom");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteAssistantAssociation");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteAssistantAssociationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteAssistantAssociation").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteAssistantAssociationRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteAssistantAssociationRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes the content.
*
*
* @param deleteContentRequest
* @return A Java Future containing the result of the DeleteContent operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the constraints specified by a service.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WisdomException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WisdomAsyncClient.DeleteContent
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deleteContent(DeleteContentRequest deleteContentRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteContentRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteContentRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Wisdom");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteContent");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteContentResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteContent").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteContentRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteContentRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes the quick response import job.
*
*
* @param deleteImportJobRequest
* @return A Java Future containing the result of the DeleteImportJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ConflictException The request could not be processed because of conflict in the current state of the
* resource. For example, if you're using a
Create
API (such as CreateAssistant
)
* that accepts name, a conflicting resource (usually with the same name) is being created or mutated.
* - ValidationException The input fails to satisfy the constraints specified by a service.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WisdomException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WisdomAsyncClient.DeleteImportJob
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deleteImportJob(DeleteImportJobRequest deleteImportJobRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteImportJobRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteImportJobRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Wisdom");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteImportJob");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteImportJobResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteImportJob").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteImportJobRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteImportJobRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes the knowledge base.
*
*
*
* When you use this API to delete an external knowledge base such as Salesforce or ServiceNow, you must also delete
* the Amazon
* AppIntegrations DataIntegration. This is because you can't reuse the DataIntegration after it's been
* associated with an external knowledge base. However, you can delete and recreate it. See DeleteDataIntegration and CreateDataIntegration in the Amazon AppIntegrations API Reference.
*
*
*
* @param deleteKnowledgeBaseRequest
* @return A Java Future containing the result of the DeleteKnowledgeBase operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ConflictException The request could not be processed because of conflict in the current state of the
* resource. For example, if you're using a
Create
API (such as CreateAssistant
)
* that accepts name, a conflicting resource (usually with the same name) is being created or mutated.
* - ValidationException The input fails to satisfy the constraints specified by a service.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WisdomException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WisdomAsyncClient.DeleteKnowledgeBase
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture deleteKnowledgeBase(
DeleteKnowledgeBaseRequest deleteKnowledgeBaseRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteKnowledgeBaseRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteKnowledgeBaseRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Wisdom");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteKnowledgeBase");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteKnowledgeBaseResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteKnowledgeBase").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteKnowledgeBaseRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteKnowledgeBaseRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes a quick response.
*
*
* @param deleteQuickResponseRequest
* @return A Java Future containing the result of the DeleteQuickResponse operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the constraints specified by a service.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WisdomException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WisdomAsyncClient.DeleteQuickResponse
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture deleteQuickResponse(
DeleteQuickResponseRequest deleteQuickResponseRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteQuickResponseRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteQuickResponseRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Wisdom");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteQuickResponse");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteQuickResponseResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteQuickResponse").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteQuickResponseRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteQuickResponseRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Retrieves information about an assistant.
*
*
* @param getAssistantRequest
* @return A Java Future containing the result of the GetAssistant operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the constraints specified by a service.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WisdomException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WisdomAsyncClient.GetAssistant
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture getAssistant(GetAssistantRequest getAssistantRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getAssistantRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getAssistantRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Wisdom");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetAssistant");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
GetAssistantResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetAssistant").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetAssistantRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getAssistantRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Retrieves information about an assistant association.
*
*
* @param getAssistantAssociationRequest
* @return A Java Future containing the result of the GetAssistantAssociation operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the constraints specified by a service.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WisdomException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WisdomAsyncClient.GetAssistantAssociation
* @see AWS API Documentation
*/
@Override
public CompletableFuture getAssistantAssociation(
GetAssistantAssociationRequest getAssistantAssociationRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getAssistantAssociationRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getAssistantAssociationRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Wisdom");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetAssistantAssociation");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetAssistantAssociationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetAssistantAssociation").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetAssistantAssociationRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getAssistantAssociationRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Retrieves content, including a pre-signed URL to download the content.
*
*
* @param getContentRequest
* @return A Java Future containing the result of the GetContent operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the constraints specified by a service.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WisdomException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WisdomAsyncClient.GetContent
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture getContent(GetContentRequest getContentRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getContentRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getContentRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Wisdom");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetContent");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
GetContentResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("GetContent")
.withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetContentRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getContentRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Retrieves summary information about the content.
*
*
* @param getContentSummaryRequest
* @return A Java Future containing the result of the GetContentSummary operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the constraints specified by a service.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WisdomException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WisdomAsyncClient.GetContentSummary
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture getContentSummary(GetContentSummaryRequest getContentSummaryRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getContentSummaryRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getContentSummaryRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Wisdom");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetContentSummary");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetContentSummaryResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetContentSummary").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetContentSummaryRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getContentSummaryRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Retrieves the started import job.
*
*
* @param getImportJobRequest
* @return A Java Future containing the result of the GetImportJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the constraints specified by a service.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WisdomException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WisdomAsyncClient.GetImportJob
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture getImportJob(GetImportJobRequest getImportJobRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getImportJobRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getImportJobRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Wisdom");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetImportJob");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
GetImportJobResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetImportJob").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetImportJobRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getImportJobRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Retrieves information about the knowledge base.
*
*
* @param getKnowledgeBaseRequest
* @return A Java Future containing the result of the GetKnowledgeBase operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the constraints specified by a service.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WisdomException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WisdomAsyncClient.GetKnowledgeBase
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture getKnowledgeBase(GetKnowledgeBaseRequest getKnowledgeBaseRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getKnowledgeBaseRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getKnowledgeBaseRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Wisdom");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetKnowledgeBase");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetKnowledgeBaseResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetKnowledgeBase").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetKnowledgeBaseRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getKnowledgeBaseRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Retrieves the quick response.
*
*
* @param getQuickResponseRequest
* @return A Java Future containing the result of the GetQuickResponse operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the constraints specified by a service.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WisdomException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WisdomAsyncClient.GetQuickResponse
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture getQuickResponse(GetQuickResponseRequest getQuickResponseRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getQuickResponseRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getQuickResponseRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Wisdom");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetQuickResponse");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetQuickResponseResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetQuickResponse").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetQuickResponseRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getQuickResponseRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Retrieves recommendations for the specified session. To avoid retrieving the same recommendations in subsequent
* calls, use NotifyRecommendationsReceived. This API supports long-polling behavior with the waitTimeSeconds
* parameter. Short poll is the default behavior and only returns recommendations already available. To perform a
* manual query against an assistant, use QueryAssistant.
*
*
* @param getRecommendationsRequest
* @return A Java Future containing the result of the GetRecommendations operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the constraints specified by a service.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WisdomException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WisdomAsyncClient.GetRecommendations
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture getRecommendations(GetRecommendationsRequest getRecommendationsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getRecommendationsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getRecommendationsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Wisdom");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetRecommendations");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetRecommendationsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetRecommendations").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetRecommendationsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getRecommendationsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Retrieves information for a specified session.
*
*
* @param getSessionRequest
* @return A Java Future containing the result of the GetSession operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the constraints specified by a service.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WisdomException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WisdomAsyncClient.GetSession
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture getSession(GetSessionRequest getSessionRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getSessionRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getSessionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Wisdom");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetSession");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
GetSessionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("GetSession")
.withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetSessionRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getSessionRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Lists information about assistant associations.
*
*
* @param listAssistantAssociationsRequest
* @return A Java Future containing the result of the ListAssistantAssociations operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the constraints specified by a service.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WisdomException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WisdomAsyncClient.ListAssistantAssociations
* @see AWS API Documentation
*/
@Override
public CompletableFuture listAssistantAssociations(
ListAssistantAssociationsRequest listAssistantAssociationsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listAssistantAssociationsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listAssistantAssociationsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Wisdom");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListAssistantAssociations");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListAssistantAssociationsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListAssistantAssociations").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListAssistantAssociationsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listAssistantAssociationsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Lists information about assistants.
*
*
* @param listAssistantsRequest
* @return A Java Future containing the result of the ListAssistants operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the constraints specified by a service.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WisdomException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WisdomAsyncClient.ListAssistants
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture listAssistants(ListAssistantsRequest listAssistantsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listAssistantsRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listAssistantsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Wisdom");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListAssistants");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListAssistantsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListAssistants").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListAssistantsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listAssistantsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Lists the content.
*
*
* @param listContentsRequest
* @return A Java Future containing the result of the ListContents operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the constraints specified by a service.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WisdomException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WisdomAsyncClient.ListContents
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture listContents(ListContentsRequest listContentsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listContentsRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listContentsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Wisdom");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListContents");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListContentsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListContents").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListContentsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listContentsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Lists information about import jobs.
*
*
* @param listImportJobsRequest
* @return A Java Future containing the result of the ListImportJobs operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the constraints specified by a service.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WisdomException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WisdomAsyncClient.ListImportJobs
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture listImportJobs(ListImportJobsRequest listImportJobsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listImportJobsRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listImportJobsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Wisdom");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListImportJobs");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListImportJobsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListImportJobs").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListImportJobsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listImportJobsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Lists the knowledge bases.
*
*
* @param listKnowledgeBasesRequest
* @return A Java Future containing the result of the ListKnowledgeBases operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the constraints specified by a service.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WisdomException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WisdomAsyncClient.ListKnowledgeBases
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture listKnowledgeBases(ListKnowledgeBasesRequest listKnowledgeBasesRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listKnowledgeBasesRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listKnowledgeBasesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Wisdom");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListKnowledgeBases");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListKnowledgeBasesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListKnowledgeBases").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListKnowledgeBasesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listKnowledgeBasesRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Lists information about quick response.
*
*
* @param listQuickResponsesRequest
* @return A Java Future containing the result of the ListQuickResponses operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the constraints specified by a service.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WisdomException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WisdomAsyncClient.ListQuickResponses
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture listQuickResponses(ListQuickResponsesRequest listQuickResponsesRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listQuickResponsesRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listQuickResponsesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Wisdom");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListQuickResponses");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListQuickResponsesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListQuickResponses").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListQuickResponsesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listQuickResponsesRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Lists the tags for the specified resource.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException The specified resource does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WisdomException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WisdomAsyncClient.ListTagsForResource
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture listTagsForResource(
ListTagsForResourceRequest listTagsForResourceRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listTagsForResourceRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listTagsForResourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Wisdom");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListTagsForResource");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListTagsForResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListTagsForResource").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListTagsForResourceRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listTagsForResourceRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Removes the specified recommendations from the specified assistant's queue of newly available recommendations.
* You can use this API in conjunction with GetRecommendations
* and a waitTimeSeconds
input for long-polling behavior and avoiding duplicate recommendations.
*
*
* @param notifyRecommendationsReceivedRequest
* @return A Java Future containing the result of the NotifyRecommendationsReceived operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the constraints specified by a service.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WisdomException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WisdomAsyncClient.NotifyRecommendationsReceived
* @see AWS API Documentation
*/
@Override
public CompletableFuture notifyRecommendationsReceived(
NotifyRecommendationsReceivedRequest notifyRecommendationsReceivedRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(notifyRecommendationsReceivedRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
notifyRecommendationsReceivedRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Wisdom");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "NotifyRecommendationsReceived");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, NotifyRecommendationsReceivedResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("NotifyRecommendationsReceived").withProtocolMetadata(protocolMetadata)
.withMarshaller(new NotifyRecommendationsReceivedRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(notifyRecommendationsReceivedRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Performs a manual search against the specified assistant. To retrieve recommendations for an assistant, use GetRecommendations.
*
*
* @param queryAssistantRequest
* @return A Java Future containing the result of the QueryAssistant operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - RequestTimeoutException The request reached the service more than 15 minutes after the date stamp on
* the request or more than 15 minutes after the request expiration date (such as for pre-signed URLs), or
* the date stamp on the request is more than 15 minutes in the future.
* - ValidationException The input fails to satisfy the constraints specified by a service.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WisdomException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WisdomAsyncClient.QueryAssistant
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture queryAssistant(QueryAssistantRequest queryAssistantRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(queryAssistantRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, queryAssistantRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Wisdom");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "QueryAssistant");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, QueryAssistantResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("QueryAssistant").withProtocolMetadata(protocolMetadata)
.withMarshaller(new QueryAssistantRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(queryAssistantRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Removes a URI template from a knowledge base.
*
*
* @param removeKnowledgeBaseTemplateUriRequest
* @return A Java Future containing the result of the RemoveKnowledgeBaseTemplateUri operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the constraints specified by a service.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WisdomException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WisdomAsyncClient.RemoveKnowledgeBaseTemplateUri
* @see AWS API Documentation
*/
@Override
public CompletableFuture removeKnowledgeBaseTemplateUri(
RemoveKnowledgeBaseTemplateUriRequest removeKnowledgeBaseTemplateUriRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(removeKnowledgeBaseTemplateUriRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
removeKnowledgeBaseTemplateUriRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Wisdom");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "RemoveKnowledgeBaseTemplateUri");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, RemoveKnowledgeBaseTemplateUriResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("RemoveKnowledgeBaseTemplateUri").withProtocolMetadata(protocolMetadata)
.withMarshaller(new RemoveKnowledgeBaseTemplateUriRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(removeKnowledgeBaseTemplateUriRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Searches for content in a specified knowledge base. Can be used to get a specific content resource by its name.
*
*
* @param searchContentRequest
* @return A Java Future containing the result of the SearchContent operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the constraints specified by a service.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WisdomException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WisdomAsyncClient.SearchContent
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture searchContent(SearchContentRequest searchContentRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(searchContentRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, searchContentRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Wisdom");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "SearchContent");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
SearchContentResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("SearchContent").withProtocolMetadata(protocolMetadata)
.withMarshaller(new SearchContentRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(searchContentRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Searches existing Wisdom quick responses in a Wisdom knowledge base.
*
*
* @param searchQuickResponsesRequest
* @return A Java Future containing the result of the SearchQuickResponses operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - RequestTimeoutException The request reached the service more than 15 minutes after the date stamp on
* the request or more than 15 minutes after the request expiration date (such as for pre-signed URLs), or
* the date stamp on the request is more than 15 minutes in the future.
* - ValidationException The input fails to satisfy the constraints specified by a service.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WisdomException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WisdomAsyncClient.SearchQuickResponses
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture searchQuickResponses(
SearchQuickResponsesRequest searchQuickResponsesRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(searchQuickResponsesRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, searchQuickResponsesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Wisdom");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "SearchQuickResponses");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, SearchQuickResponsesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("SearchQuickResponses").withProtocolMetadata(protocolMetadata)
.withMarshaller(new SearchQuickResponsesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(searchQuickResponsesRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Searches for sessions.
*
*
* @param searchSessionsRequest
* @return A Java Future containing the result of the SearchSessions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the constraints specified by a service.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WisdomException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WisdomAsyncClient.SearchSessions
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture searchSessions(SearchSessionsRequest searchSessionsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(searchSessionsRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, searchSessionsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Wisdom");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "SearchSessions");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, SearchSessionsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("SearchSessions").withProtocolMetadata(protocolMetadata)
.withMarshaller(new SearchSessionsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(searchSessionsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Get a URL to upload content to a knowledge base. To upload content, first make a PUT request to the returned URL
* with your file, making sure to include the required headers. Then use CreateContent to
* finalize the content creation process or UpdateContent to modify
* an existing resource. You can only upload content to a knowledge base of type CUSTOM.
*
*
* @param startContentUploadRequest
* @return A Java Future containing the result of the StartContentUpload operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the constraints specified by a service.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WisdomException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WisdomAsyncClient.StartContentUpload
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture startContentUpload(StartContentUploadRequest startContentUploadRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(startContentUploadRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, startContentUploadRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Wisdom");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "StartContentUpload");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, StartContentUploadResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("StartContentUpload").withProtocolMetadata(protocolMetadata)
.withMarshaller(new StartContentUploadRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(startContentUploadRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Start an asynchronous job to import Wisdom resources from an uploaded source file. Before calling this API, use
* StartContentUpload
* to upload an asset that contains the resource data.
*
*
* -
*
* For importing Wisdom quick responses, you need to upload a csv file including the quick responses. For
* information about how to format the csv file for importing quick responses, see Import quick responses.
*
*
*
*
* @param startImportJobRequest
* @return A Java Future containing the result of the StartImportJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ConflictException The request could not be processed because of conflict in the current state of the
* resource. For example, if you're using a
Create
API (such as CreateAssistant
)
* that accepts name, a conflicting resource (usually with the same name) is being created or mutated.
* - ValidationException The input fails to satisfy the constraints specified by a service.
* - ServiceQuotaExceededException You've exceeded your service quota. To perform the requested action,
* remove some of the relevant resources, or use service quotas to request a service quota increase.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WisdomException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WisdomAsyncClient.StartImportJob
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture startImportJob(StartImportJobRequest startImportJobRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(startImportJobRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, startImportJobRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Wisdom");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "StartImportJob");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, StartImportJobResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams