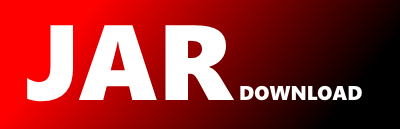
software.amazon.awssdk.services.wisdom.model.UpdateQuickResponseRequest Maven / Gradle / Ivy
Show all versions of wisdom Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.wisdom.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class UpdateQuickResponseRequest extends WisdomRequest implements
ToCopyableBuilder {
private static final SdkField> CHANNELS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("channels")
.getter(getter(UpdateQuickResponseRequest::channels))
.setter(setter(Builder::channels))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("channels").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField CONTENT_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("content")
.getter(getter(UpdateQuickResponseRequest::content)).setter(setter(Builder::content))
.constructor(QuickResponseDataProvider::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("content").build()).build();
private static final SdkField CONTENT_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("contentType").getter(getter(UpdateQuickResponseRequest::contentType))
.setter(setter(Builder::contentType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("contentType").build()).build();
private static final SdkField DESCRIPTION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("description").getter(getter(UpdateQuickResponseRequest::description))
.setter(setter(Builder::description))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("description").build()).build();
private static final SdkField GROUPING_CONFIGURATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("groupingConfiguration")
.getter(getter(UpdateQuickResponseRequest::groupingConfiguration)).setter(setter(Builder::groupingConfiguration))
.constructor(GroupingConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("groupingConfiguration").build())
.build();
private static final SdkField IS_ACTIVE_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("isActive").getter(getter(UpdateQuickResponseRequest::isActive)).setter(setter(Builder::isActive))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("isActive").build()).build();
private static final SdkField KNOWLEDGE_BASE_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("knowledgeBaseId").getter(getter(UpdateQuickResponseRequest::knowledgeBaseId))
.setter(setter(Builder::knowledgeBaseId))
.traits(LocationTrait.builder().location(MarshallLocation.PATH).locationName("knowledgeBaseId").build()).build();
private static final SdkField LANGUAGE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("language").getter(getter(UpdateQuickResponseRequest::language)).setter(setter(Builder::language))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("language").build()).build();
private static final SdkField NAME_FIELD = SdkField. builder(MarshallingType.STRING).memberName("name")
.getter(getter(UpdateQuickResponseRequest::name)).setter(setter(Builder::name))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("name").build()).build();
private static final SdkField QUICK_RESPONSE_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("quickResponseId").getter(getter(UpdateQuickResponseRequest::quickResponseId))
.setter(setter(Builder::quickResponseId))
.traits(LocationTrait.builder().location(MarshallLocation.PATH).locationName("quickResponseId").build()).build();
private static final SdkField REMOVE_DESCRIPTION_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("removeDescription").getter(getter(UpdateQuickResponseRequest::removeDescription))
.setter(setter(Builder::removeDescription))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("removeDescription").build()).build();
private static final SdkField REMOVE_GROUPING_CONFIGURATION_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("removeGroupingConfiguration")
.getter(getter(UpdateQuickResponseRequest::removeGroupingConfiguration))
.setter(setter(Builder::removeGroupingConfiguration))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("removeGroupingConfiguration")
.build()).build();
private static final SdkField REMOVE_SHORTCUT_KEY_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("removeShortcutKey").getter(getter(UpdateQuickResponseRequest::removeShortcutKey))
.setter(setter(Builder::removeShortcutKey))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("removeShortcutKey").build()).build();
private static final SdkField SHORTCUT_KEY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("shortcutKey").getter(getter(UpdateQuickResponseRequest::shortcutKey))
.setter(setter(Builder::shortcutKey))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("shortcutKey").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(CHANNELS_FIELD, CONTENT_FIELD,
CONTENT_TYPE_FIELD, DESCRIPTION_FIELD, GROUPING_CONFIGURATION_FIELD, IS_ACTIVE_FIELD, KNOWLEDGE_BASE_ID_FIELD,
LANGUAGE_FIELD, NAME_FIELD, QUICK_RESPONSE_ID_FIELD, REMOVE_DESCRIPTION_FIELD, REMOVE_GROUPING_CONFIGURATION_FIELD,
REMOVE_SHORTCUT_KEY_FIELD, SHORTCUT_KEY_FIELD));
private static final Map> SDK_NAME_TO_FIELD = memberNameToFieldInitializer();
private final List channels;
private final QuickResponseDataProvider content;
private final String contentType;
private final String description;
private final GroupingConfiguration groupingConfiguration;
private final Boolean isActive;
private final String knowledgeBaseId;
private final String language;
private final String name;
private final String quickResponseId;
private final Boolean removeDescription;
private final Boolean removeGroupingConfiguration;
private final Boolean removeShortcutKey;
private final String shortcutKey;
private UpdateQuickResponseRequest(BuilderImpl builder) {
super(builder);
this.channels = builder.channels;
this.content = builder.content;
this.contentType = builder.contentType;
this.description = builder.description;
this.groupingConfiguration = builder.groupingConfiguration;
this.isActive = builder.isActive;
this.knowledgeBaseId = builder.knowledgeBaseId;
this.language = builder.language;
this.name = builder.name;
this.quickResponseId = builder.quickResponseId;
this.removeDescription = builder.removeDescription;
this.removeGroupingConfiguration = builder.removeGroupingConfiguration;
this.removeShortcutKey = builder.removeShortcutKey;
this.shortcutKey = builder.shortcutKey;
}
/**
* For responses, this returns true if the service returned a value for the Channels property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasChannels() {
return channels != null && !(channels instanceof SdkAutoConstructList);
}
/**
*
* The Amazon Connect contact channels this quick response applies to. The supported contact channel types include
* Chat
.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasChannels} method.
*
*
* @return The Amazon Connect contact channels this quick response applies to. The supported contact channel types
* include Chat
.
*/
public final List channels() {
return channels;
}
/**
*
* The updated content of the quick response.
*
*
* @return The updated content of the quick response.
*/
public final QuickResponseDataProvider content() {
return content;
}
/**
*
* The media type of the quick response content.
*
*
* -
*
* Use application/x.quickresponse;format=plain
for quick response written in plain text.
*
*
* -
*
* Use application/x.quickresponse;format=markdown
for quick response written in richtext.
*
*
*
*
* @return The media type of the quick response content.
*
* -
*
* Use application/x.quickresponse;format=plain
for quick response written in plain text.
*
*
* -
*
* Use application/x.quickresponse;format=markdown
for quick response written in richtext.
*
*
*/
public final String contentType() {
return contentType;
}
/**
*
* The updated description of the quick response.
*
*
* @return The updated description of the quick response.
*/
public final String description() {
return description;
}
/**
*
* The updated grouping configuration of the quick response.
*
*
* @return The updated grouping configuration of the quick response.
*/
public final GroupingConfiguration groupingConfiguration() {
return groupingConfiguration;
}
/**
*
* Whether the quick response is active.
*
*
* @return Whether the quick response is active.
*/
public final Boolean isActive() {
return isActive;
}
/**
*
* The identifier of the knowledge base. This should not be a QUICK_RESPONSES type knowledge base if you're storing
* Wisdom Content resource to it. Can be either the ID or the ARN. URLs cannot contain the ARN.
*
*
* @return The identifier of the knowledge base. This should not be a QUICK_RESPONSES type knowledge base if you're
* storing Wisdom Content resource to it. Can be either the ID or the ARN. URLs cannot contain the ARN.
*/
public final String knowledgeBaseId() {
return knowledgeBaseId;
}
/**
*
* The language code value for the language in which the quick response is written. The supported language codes
* include de_DE
, en_US
, es_ES
, fr_FR
, id_ID
,
* it_IT
, ja_JP
, ko_KR
, pt_BR
, zh_CN
,
* zh_TW
*
*
* @return The language code value for the language in which the quick response is written. The supported language
* codes include de_DE
, en_US
, es_ES
, fr_FR
,
* id_ID
, it_IT
, ja_JP
, ko_KR
, pt_BR
,
* zh_CN
, zh_TW
*/
public final String language() {
return language;
}
/**
*
* The name of the quick response.
*
*
* @return The name of the quick response.
*/
public final String name() {
return name;
}
/**
*
* The identifier of the quick response.
*
*
* @return The identifier of the quick response.
*/
public final String quickResponseId() {
return quickResponseId;
}
/**
*
* Whether to remove the description from the quick response.
*
*
* @return Whether to remove the description from the quick response.
*/
public final Boolean removeDescription() {
return removeDescription;
}
/**
*
* Whether to remove the grouping configuration of the quick response.
*
*
* @return Whether to remove the grouping configuration of the quick response.
*/
public final Boolean removeGroupingConfiguration() {
return removeGroupingConfiguration;
}
/**
*
* Whether to remove the shortcut key of the quick response.
*
*
* @return Whether to remove the shortcut key of the quick response.
*/
public final Boolean removeShortcutKey() {
return removeShortcutKey;
}
/**
*
* The shortcut key of the quick response. The value should be unique across the knowledge base.
*
*
* @return The shortcut key of the quick response. The value should be unique across the knowledge base.
*/
public final String shortcutKey() {
return shortcutKey;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(hasChannels() ? channels() : null);
hashCode = 31 * hashCode + Objects.hashCode(content());
hashCode = 31 * hashCode + Objects.hashCode(contentType());
hashCode = 31 * hashCode + Objects.hashCode(description());
hashCode = 31 * hashCode + Objects.hashCode(groupingConfiguration());
hashCode = 31 * hashCode + Objects.hashCode(isActive());
hashCode = 31 * hashCode + Objects.hashCode(knowledgeBaseId());
hashCode = 31 * hashCode + Objects.hashCode(language());
hashCode = 31 * hashCode + Objects.hashCode(name());
hashCode = 31 * hashCode + Objects.hashCode(quickResponseId());
hashCode = 31 * hashCode + Objects.hashCode(removeDescription());
hashCode = 31 * hashCode + Objects.hashCode(removeGroupingConfiguration());
hashCode = 31 * hashCode + Objects.hashCode(removeShortcutKey());
hashCode = 31 * hashCode + Objects.hashCode(shortcutKey());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof UpdateQuickResponseRequest)) {
return false;
}
UpdateQuickResponseRequest other = (UpdateQuickResponseRequest) obj;
return hasChannels() == other.hasChannels() && Objects.equals(channels(), other.channels())
&& Objects.equals(content(), other.content()) && Objects.equals(contentType(), other.contentType())
&& Objects.equals(description(), other.description())
&& Objects.equals(groupingConfiguration(), other.groupingConfiguration())
&& Objects.equals(isActive(), other.isActive()) && Objects.equals(knowledgeBaseId(), other.knowledgeBaseId())
&& Objects.equals(language(), other.language()) && Objects.equals(name(), other.name())
&& Objects.equals(quickResponseId(), other.quickResponseId())
&& Objects.equals(removeDescription(), other.removeDescription())
&& Objects.equals(removeGroupingConfiguration(), other.removeGroupingConfiguration())
&& Objects.equals(removeShortcutKey(), other.removeShortcutKey())
&& Objects.equals(shortcutKey(), other.shortcutKey());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("UpdateQuickResponseRequest")
.add("Channels", channels() == null ? null : "*** Sensitive Data Redacted ***").add("Content", content())
.add("ContentType", contentType()).add("Description", description())
.add("GroupingConfiguration", groupingConfiguration()).add("IsActive", isActive())
.add("KnowledgeBaseId", knowledgeBaseId()).add("Language", language()).add("Name", name())
.add("QuickResponseId", quickResponseId()).add("RemoveDescription", removeDescription())
.add("RemoveGroupingConfiguration", removeGroupingConfiguration()).add("RemoveShortcutKey", removeShortcutKey())
.add("ShortcutKey", shortcutKey()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "channels":
return Optional.ofNullable(clazz.cast(channels()));
case "content":
return Optional.ofNullable(clazz.cast(content()));
case "contentType":
return Optional.ofNullable(clazz.cast(contentType()));
case "description":
return Optional.ofNullable(clazz.cast(description()));
case "groupingConfiguration":
return Optional.ofNullable(clazz.cast(groupingConfiguration()));
case "isActive":
return Optional.ofNullable(clazz.cast(isActive()));
case "knowledgeBaseId":
return Optional.ofNullable(clazz.cast(knowledgeBaseId()));
case "language":
return Optional.ofNullable(clazz.cast(language()));
case "name":
return Optional.ofNullable(clazz.cast(name()));
case "quickResponseId":
return Optional.ofNullable(clazz.cast(quickResponseId()));
case "removeDescription":
return Optional.ofNullable(clazz.cast(removeDescription()));
case "removeGroupingConfiguration":
return Optional.ofNullable(clazz.cast(removeGroupingConfiguration()));
case "removeShortcutKey":
return Optional.ofNullable(clazz.cast(removeShortcutKey()));
case "shortcutKey":
return Optional.ofNullable(clazz.cast(shortcutKey()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Map> memberNameToFieldInitializer() {
Map> map = new HashMap<>();
map.put("channels", CHANNELS_FIELD);
map.put("content", CONTENT_FIELD);
map.put("contentType", CONTENT_TYPE_FIELD);
map.put("description", DESCRIPTION_FIELD);
map.put("groupingConfiguration", GROUPING_CONFIGURATION_FIELD);
map.put("isActive", IS_ACTIVE_FIELD);
map.put("knowledgeBaseId", KNOWLEDGE_BASE_ID_FIELD);
map.put("language", LANGUAGE_FIELD);
map.put("name", NAME_FIELD);
map.put("quickResponseId", QUICK_RESPONSE_ID_FIELD);
map.put("removeDescription", REMOVE_DESCRIPTION_FIELD);
map.put("removeGroupingConfiguration", REMOVE_GROUPING_CONFIGURATION_FIELD);
map.put("removeShortcutKey", REMOVE_SHORTCUT_KEY_FIELD);
map.put("shortcutKey", SHORTCUT_KEY_FIELD);
return Collections.unmodifiableMap(map);
}
private static Function