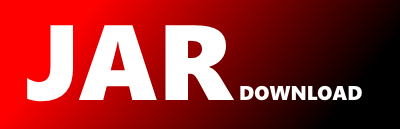
software.amazon.awssdk.services.workdocs.WorkDocsAsyncClient Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.workdocs;
import java.util.concurrent.CompletableFuture;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkClient;
import software.amazon.awssdk.services.workdocs.model.AbortDocumentVersionUploadRequest;
import software.amazon.awssdk.services.workdocs.model.AbortDocumentVersionUploadResponse;
import software.amazon.awssdk.services.workdocs.model.ActivateUserRequest;
import software.amazon.awssdk.services.workdocs.model.ActivateUserResponse;
import software.amazon.awssdk.services.workdocs.model.AddResourcePermissionsRequest;
import software.amazon.awssdk.services.workdocs.model.AddResourcePermissionsResponse;
import software.amazon.awssdk.services.workdocs.model.CreateCommentRequest;
import software.amazon.awssdk.services.workdocs.model.CreateCommentResponse;
import software.amazon.awssdk.services.workdocs.model.CreateCustomMetadataRequest;
import software.amazon.awssdk.services.workdocs.model.CreateCustomMetadataResponse;
import software.amazon.awssdk.services.workdocs.model.CreateFolderRequest;
import software.amazon.awssdk.services.workdocs.model.CreateFolderResponse;
import software.amazon.awssdk.services.workdocs.model.CreateLabelsRequest;
import software.amazon.awssdk.services.workdocs.model.CreateLabelsResponse;
import software.amazon.awssdk.services.workdocs.model.CreateNotificationSubscriptionRequest;
import software.amazon.awssdk.services.workdocs.model.CreateNotificationSubscriptionResponse;
import software.amazon.awssdk.services.workdocs.model.CreateUserRequest;
import software.amazon.awssdk.services.workdocs.model.CreateUserResponse;
import software.amazon.awssdk.services.workdocs.model.DeactivateUserRequest;
import software.amazon.awssdk.services.workdocs.model.DeactivateUserResponse;
import software.amazon.awssdk.services.workdocs.model.DeleteCommentRequest;
import software.amazon.awssdk.services.workdocs.model.DeleteCommentResponse;
import software.amazon.awssdk.services.workdocs.model.DeleteCustomMetadataRequest;
import software.amazon.awssdk.services.workdocs.model.DeleteCustomMetadataResponse;
import software.amazon.awssdk.services.workdocs.model.DeleteDocumentRequest;
import software.amazon.awssdk.services.workdocs.model.DeleteDocumentResponse;
import software.amazon.awssdk.services.workdocs.model.DeleteFolderContentsRequest;
import software.amazon.awssdk.services.workdocs.model.DeleteFolderContentsResponse;
import software.amazon.awssdk.services.workdocs.model.DeleteFolderRequest;
import software.amazon.awssdk.services.workdocs.model.DeleteFolderResponse;
import software.amazon.awssdk.services.workdocs.model.DeleteLabelsRequest;
import software.amazon.awssdk.services.workdocs.model.DeleteLabelsResponse;
import software.amazon.awssdk.services.workdocs.model.DeleteNotificationSubscriptionRequest;
import software.amazon.awssdk.services.workdocs.model.DeleteNotificationSubscriptionResponse;
import software.amazon.awssdk.services.workdocs.model.DeleteUserRequest;
import software.amazon.awssdk.services.workdocs.model.DeleteUserResponse;
import software.amazon.awssdk.services.workdocs.model.DescribeActivitiesRequest;
import software.amazon.awssdk.services.workdocs.model.DescribeActivitiesResponse;
import software.amazon.awssdk.services.workdocs.model.DescribeCommentsRequest;
import software.amazon.awssdk.services.workdocs.model.DescribeCommentsResponse;
import software.amazon.awssdk.services.workdocs.model.DescribeDocumentVersionsRequest;
import software.amazon.awssdk.services.workdocs.model.DescribeDocumentVersionsResponse;
import software.amazon.awssdk.services.workdocs.model.DescribeFolderContentsRequest;
import software.amazon.awssdk.services.workdocs.model.DescribeFolderContentsResponse;
import software.amazon.awssdk.services.workdocs.model.DescribeGroupsRequest;
import software.amazon.awssdk.services.workdocs.model.DescribeGroupsResponse;
import software.amazon.awssdk.services.workdocs.model.DescribeNotificationSubscriptionsRequest;
import software.amazon.awssdk.services.workdocs.model.DescribeNotificationSubscriptionsResponse;
import software.amazon.awssdk.services.workdocs.model.DescribeResourcePermissionsRequest;
import software.amazon.awssdk.services.workdocs.model.DescribeResourcePermissionsResponse;
import software.amazon.awssdk.services.workdocs.model.DescribeRootFoldersRequest;
import software.amazon.awssdk.services.workdocs.model.DescribeRootFoldersResponse;
import software.amazon.awssdk.services.workdocs.model.DescribeUsersRequest;
import software.amazon.awssdk.services.workdocs.model.DescribeUsersResponse;
import software.amazon.awssdk.services.workdocs.model.GetCurrentUserRequest;
import software.amazon.awssdk.services.workdocs.model.GetCurrentUserResponse;
import software.amazon.awssdk.services.workdocs.model.GetDocumentPathRequest;
import software.amazon.awssdk.services.workdocs.model.GetDocumentPathResponse;
import software.amazon.awssdk.services.workdocs.model.GetDocumentRequest;
import software.amazon.awssdk.services.workdocs.model.GetDocumentResponse;
import software.amazon.awssdk.services.workdocs.model.GetDocumentVersionRequest;
import software.amazon.awssdk.services.workdocs.model.GetDocumentVersionResponse;
import software.amazon.awssdk.services.workdocs.model.GetFolderPathRequest;
import software.amazon.awssdk.services.workdocs.model.GetFolderPathResponse;
import software.amazon.awssdk.services.workdocs.model.GetFolderRequest;
import software.amazon.awssdk.services.workdocs.model.GetFolderResponse;
import software.amazon.awssdk.services.workdocs.model.GetResourcesRequest;
import software.amazon.awssdk.services.workdocs.model.GetResourcesResponse;
import software.amazon.awssdk.services.workdocs.model.InitiateDocumentVersionUploadRequest;
import software.amazon.awssdk.services.workdocs.model.InitiateDocumentVersionUploadResponse;
import software.amazon.awssdk.services.workdocs.model.RemoveAllResourcePermissionsRequest;
import software.amazon.awssdk.services.workdocs.model.RemoveAllResourcePermissionsResponse;
import software.amazon.awssdk.services.workdocs.model.RemoveResourcePermissionRequest;
import software.amazon.awssdk.services.workdocs.model.RemoveResourcePermissionResponse;
import software.amazon.awssdk.services.workdocs.model.UpdateDocumentRequest;
import software.amazon.awssdk.services.workdocs.model.UpdateDocumentResponse;
import software.amazon.awssdk.services.workdocs.model.UpdateDocumentVersionRequest;
import software.amazon.awssdk.services.workdocs.model.UpdateDocumentVersionResponse;
import software.amazon.awssdk.services.workdocs.model.UpdateFolderRequest;
import software.amazon.awssdk.services.workdocs.model.UpdateFolderResponse;
import software.amazon.awssdk.services.workdocs.model.UpdateUserRequest;
import software.amazon.awssdk.services.workdocs.model.UpdateUserResponse;
import software.amazon.awssdk.services.workdocs.paginators.DescribeDocumentVersionsPublisher;
import software.amazon.awssdk.services.workdocs.paginators.DescribeFolderContentsPublisher;
import software.amazon.awssdk.services.workdocs.paginators.DescribeUsersPublisher;
/**
* Service client for accessing Amazon WorkDocs asynchronously. This can be created using the static {@link #builder()}
* method.
*
*
* The WorkDocs API is designed for the following use cases:
*
*
* -
*
* File Migration: File migration applications are supported for users who want to migrate their files from an
* on-premises or off-premises file system or service. Users can insert files into a user directory structure, as well
* as allow for basic metadata changes, such as modifications to the permissions of files.
*
*
* -
*
* Security: Support security applications are supported for users who have additional security needs, such as antivirus
* or data loss prevention. The API actions, along with AWS CloudTrail, allow these applications to detect when changes
* occur in Amazon WorkDocs. Then, the application can take the necessary actions and replace the target file. If the
* target file violates the policy, the application can also choose to email the user.
*
*
* -
*
* eDiscovery/Analytics: General administrative applications are supported, such as eDiscovery and analytics. These
* applications can choose to mimic or record the actions in an Amazon WorkDocs site, along with AWS CloudTrail, to
* replicate data for eDiscovery, backup, or analytical applications.
*
*
*
*
* All Amazon WorkDocs API actions are Amazon authenticated and certificate-signed. They not only require the use of the
* AWS SDK, but also allow for the exclusive use of IAM users and roles to help facilitate access, trust, and permission
* policies. By creating a role and allowing an IAM user to access the Amazon WorkDocs site, the IAM user gains full
* administrative visibility into the entire Amazon WorkDocs site (or as set in the IAM policy). This includes, but is
* not limited to, the ability to modify file permissions and upload any file to any user. This allows developers to
* perform the three use cases above, as well as give users the ability to grant access on a selective basis using the
* IAM model.
*
*/
@Generated("software.amazon.awssdk:codegen")
public interface WorkDocsAsyncClient extends SdkClient {
String SERVICE_NAME = "workdocs";
/**
* Create a {@link WorkDocsAsyncClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static WorkDocsAsyncClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link WorkDocsAsyncClient}.
*/
static WorkDocsAsyncClientBuilder builder() {
return new DefaultWorkDocsAsyncClientBuilder();
}
/**
*
* Aborts the upload of the specified document version that was previously initiated by
* InitiateDocumentVersionUpload. The client should make this call only when it no longer intends to upload
* the document version, or fails to do so.
*
*
* @param abortDocumentVersionUploadRequest
* @return A Java Future containing the result of the AbortDocumentVersionUpload operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - EntityNotExistsException The resource does not exist.
* - ProhibitedStateException The specified document version is not in the INITIALIZED state.
* - UnauthorizedOperationException The operation is not permitted.
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.AbortDocumentVersionUpload
* @see AWS API Documentation
*/
default CompletableFuture abortDocumentVersionUpload(
AbortDocumentVersionUploadRequest abortDocumentVersionUploadRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Aborts the upload of the specified document version that was previously initiated by
* InitiateDocumentVersionUpload. The client should make this call only when it no longer intends to upload
* the document version, or fails to do so.
*
*
*
* This is a convenience which creates an instance of the {@link AbortDocumentVersionUploadRequest.Builder} avoiding
* the need to create one manually via {@link AbortDocumentVersionUploadRequest#builder()}
*
*
* @param abortDocumentVersionUploadRequest
* A {@link Consumer} that will call methods on {@link AbortDocumentVersionUploadRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the AbortDocumentVersionUpload operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - EntityNotExistsException The resource does not exist.
* - ProhibitedStateException The specified document version is not in the INITIALIZED state.
* - UnauthorizedOperationException The operation is not permitted.
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.AbortDocumentVersionUpload
* @see AWS API Documentation
*/
default CompletableFuture abortDocumentVersionUpload(
Consumer abortDocumentVersionUploadRequest) {
return abortDocumentVersionUpload(AbortDocumentVersionUploadRequest.builder()
.applyMutation(abortDocumentVersionUploadRequest).build());
}
/**
*
* Activates the specified user. Only active users can access Amazon WorkDocs.
*
*
* @param activateUserRequest
* @return A Java Future containing the result of the ActivateUser operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - EntityNotExistsException The resource does not exist.
* - UnauthorizedOperationException The operation is not permitted.
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.ActivateUser
* @see AWS API
* Documentation
*/
default CompletableFuture activateUser(ActivateUserRequest activateUserRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Activates the specified user. Only active users can access Amazon WorkDocs.
*
*
*
* This is a convenience which creates an instance of the {@link ActivateUserRequest.Builder} avoiding the need to
* create one manually via {@link ActivateUserRequest#builder()}
*
*
* @param activateUserRequest
* A {@link Consumer} that will call methods on {@link ActivateUserRequest.Builder} to create a request.
* @return A Java Future containing the result of the ActivateUser operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - EntityNotExistsException The resource does not exist.
* - UnauthorizedOperationException The operation is not permitted.
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.ActivateUser
* @see AWS API
* Documentation
*/
default CompletableFuture activateUser(Consumer activateUserRequest) {
return activateUser(ActivateUserRequest.builder().applyMutation(activateUserRequest).build());
}
/**
*
* Creates a set of permissions for the specified folder or document. The resource permissions are overwritten if
* the principals already have different permissions.
*
*
* @param addResourcePermissionsRequest
* @return A Java Future containing the result of the AddResourcePermissions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - UnauthorizedOperationException The operation is not permitted.
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.AddResourcePermissions
* @see AWS API Documentation
*/
default CompletableFuture addResourcePermissions(
AddResourcePermissionsRequest addResourcePermissionsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a set of permissions for the specified folder or document. The resource permissions are overwritten if
* the principals already have different permissions.
*
*
*
* This is a convenience which creates an instance of the {@link AddResourcePermissionsRequest.Builder} avoiding the
* need to create one manually via {@link AddResourcePermissionsRequest#builder()}
*
*
* @param addResourcePermissionsRequest
* A {@link Consumer} that will call methods on {@link AddResourcePermissionsRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the AddResourcePermissions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - UnauthorizedOperationException The operation is not permitted.
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.AddResourcePermissions
* @see AWS API Documentation
*/
default CompletableFuture addResourcePermissions(
Consumer addResourcePermissionsRequest) {
return addResourcePermissions(AddResourcePermissionsRequest.builder().applyMutation(addResourcePermissionsRequest)
.build());
}
/**
*
* Adds a new comment to the specified document version.
*
*
* @param createCommentRequest
* @return A Java Future containing the result of the CreateComment operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - EntityNotExistsException The resource does not exist.
* - ProhibitedStateException The specified document version is not in the INITIALIZED state.
* - UnauthorizedOperationException The operation is not permitted.
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - DocumentLockedForCommentsException This exception is thrown when the document is locked for comments
* and user tries to create or delete a comment on that document.
* - InvalidCommentOperationException The requested operation is not allowed on the specified comment
* object.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.CreateComment
* @see AWS API
* Documentation
*/
default CompletableFuture createComment(CreateCommentRequest createCommentRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Adds a new comment to the specified document version.
*
*
*
* This is a convenience which creates an instance of the {@link CreateCommentRequest.Builder} avoiding the need to
* create one manually via {@link CreateCommentRequest#builder()}
*
*
* @param createCommentRequest
* A {@link Consumer} that will call methods on {@link CreateCommentRequest.Builder} to create a request.
* @return A Java Future containing the result of the CreateComment operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - EntityNotExistsException The resource does not exist.
* - ProhibitedStateException The specified document version is not in the INITIALIZED state.
* - UnauthorizedOperationException The operation is not permitted.
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - DocumentLockedForCommentsException This exception is thrown when the document is locked for comments
* and user tries to create or delete a comment on that document.
* - InvalidCommentOperationException The requested operation is not allowed on the specified comment
* object.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.CreateComment
* @see AWS API
* Documentation
*/
default CompletableFuture createComment(Consumer createCommentRequest) {
return createComment(CreateCommentRequest.builder().applyMutation(createCommentRequest).build());
}
/**
*
* Adds one or more custom properties to the specified resource (a folder, document, or version).
*
*
* @param createCustomMetadataRequest
* @return A Java Future containing the result of the CreateCustomMetadata operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - EntityNotExistsException The resource does not exist.
* - UnauthorizedOperationException The operation is not permitted.
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - ProhibitedStateException The specified document version is not in the INITIALIZED state.
* - CustomMetadataLimitExceededException The limit has been reached on the number of custom properties
* for the specified resource.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.CreateCustomMetadata
* @see AWS
* API Documentation
*/
default CompletableFuture createCustomMetadata(
CreateCustomMetadataRequest createCustomMetadataRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Adds one or more custom properties to the specified resource (a folder, document, or version).
*
*
*
* This is a convenience which creates an instance of the {@link CreateCustomMetadataRequest.Builder} avoiding the
* need to create one manually via {@link CreateCustomMetadataRequest#builder()}
*
*
* @param createCustomMetadataRequest
* A {@link Consumer} that will call methods on {@link CreateCustomMetadataRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the CreateCustomMetadata operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - EntityNotExistsException The resource does not exist.
* - UnauthorizedOperationException The operation is not permitted.
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - ProhibitedStateException The specified document version is not in the INITIALIZED state.
* - CustomMetadataLimitExceededException The limit has been reached on the number of custom properties
* for the specified resource.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.CreateCustomMetadata
* @see AWS
* API Documentation
*/
default CompletableFuture createCustomMetadata(
Consumer createCustomMetadataRequest) {
return createCustomMetadata(CreateCustomMetadataRequest.builder().applyMutation(createCustomMetadataRequest).build());
}
/**
*
* Creates a folder with the specified name and parent folder.
*
*
* @param createFolderRequest
* @return A Java Future containing the result of the CreateFolder operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - EntityNotExistsException The resource does not exist.
* - EntityAlreadyExistsException The resource already exists.
* - ProhibitedStateException The specified document version is not in the INITIALIZED state.
* - ConflictingOperationException Another operation is in progress on the resource that conflicts with
* the current operation.
* - LimitExceededException The maximum of 100,000 folders under the parent folder has been exceeded.
* - UnauthorizedOperationException The operation is not permitted.
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.CreateFolder
* @see AWS API
* Documentation
*/
default CompletableFuture createFolder(CreateFolderRequest createFolderRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a folder with the specified name and parent folder.
*
*
*
* This is a convenience which creates an instance of the {@link CreateFolderRequest.Builder} avoiding the need to
* create one manually via {@link CreateFolderRequest#builder()}
*
*
* @param createFolderRequest
* A {@link Consumer} that will call methods on {@link CreateFolderRequest.Builder} to create a request.
* @return A Java Future containing the result of the CreateFolder operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - EntityNotExistsException The resource does not exist.
* - EntityAlreadyExistsException The resource already exists.
* - ProhibitedStateException The specified document version is not in the INITIALIZED state.
* - ConflictingOperationException Another operation is in progress on the resource that conflicts with
* the current operation.
* - LimitExceededException The maximum of 100,000 folders under the parent folder has been exceeded.
* - UnauthorizedOperationException The operation is not permitted.
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.CreateFolder
* @see AWS API
* Documentation
*/
default CompletableFuture createFolder(Consumer createFolderRequest) {
return createFolder(CreateFolderRequest.builder().applyMutation(createFolderRequest).build());
}
/**
*
* Adds the specified list of labels to the given resource (a document or folder)
*
*
* @param createLabelsRequest
* @return A Java Future containing the result of the CreateLabels operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - EntityNotExistsException The resource does not exist.
* - UnauthorizedOperationException The operation is not permitted.
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - TooManyLabelsException The limit has been reached on the number of labels for the specified resource.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.CreateLabels
* @see AWS API
* Documentation
*/
default CompletableFuture createLabels(CreateLabelsRequest createLabelsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Adds the specified list of labels to the given resource (a document or folder)
*
*
*
* This is a convenience which creates an instance of the {@link CreateLabelsRequest.Builder} avoiding the need to
* create one manually via {@link CreateLabelsRequest#builder()}
*
*
* @param createLabelsRequest
* A {@link Consumer} that will call methods on {@link CreateLabelsRequest.Builder} to create a request.
* @return A Java Future containing the result of the CreateLabels operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - EntityNotExistsException The resource does not exist.
* - UnauthorizedOperationException The operation is not permitted.
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - TooManyLabelsException The limit has been reached on the number of labels for the specified resource.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.CreateLabels
* @see AWS API
* Documentation
*/
default CompletableFuture createLabels(Consumer createLabelsRequest) {
return createLabels(CreateLabelsRequest.builder().applyMutation(createLabelsRequest).build());
}
/**
*
* Configure Amazon WorkDocs to use Amazon SNS notifications. The endpoint receives a confirmation message, and must
* confirm the subscription.
*
*
* For more information, see Subscribe to
* Notifications in the Amazon WorkDocs Developer Guide.
*
*
* @param createNotificationSubscriptionRequest
* @return A Java Future containing the result of the CreateNotificationSubscription operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - TooManySubscriptionsException You've reached the limit on the number of subscriptions for the
* WorkDocs instance.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.CreateNotificationSubscription
* @see AWS API Documentation
*/
default CompletableFuture createNotificationSubscription(
CreateNotificationSubscriptionRequest createNotificationSubscriptionRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Configure Amazon WorkDocs to use Amazon SNS notifications. The endpoint receives a confirmation message, and must
* confirm the subscription.
*
*
* For more information, see Subscribe to
* Notifications in the Amazon WorkDocs Developer Guide.
*
*
*
* This is a convenience which creates an instance of the {@link CreateNotificationSubscriptionRequest.Builder}
* avoiding the need to create one manually via {@link CreateNotificationSubscriptionRequest#builder()}
*
*
* @param createNotificationSubscriptionRequest
* A {@link Consumer} that will call methods on {@link CreateNotificationSubscriptionRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the CreateNotificationSubscription operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - TooManySubscriptionsException You've reached the limit on the number of subscriptions for the
* WorkDocs instance.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.CreateNotificationSubscription
* @see AWS API Documentation
*/
default CompletableFuture createNotificationSubscription(
Consumer createNotificationSubscriptionRequest) {
return createNotificationSubscription(CreateNotificationSubscriptionRequest.builder()
.applyMutation(createNotificationSubscriptionRequest).build());
}
/**
*
* Creates a user in a Simple AD or Microsoft AD directory. The status of a newly created user is "ACTIVE". New
* users can access Amazon WorkDocs.
*
*
* @param createUserRequest
* @return A Java Future containing the result of the CreateUser operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - EntityAlreadyExistsException The resource already exists.
* - UnauthorizedOperationException The operation is not permitted.
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.CreateUser
* @see AWS API
* Documentation
*/
default CompletableFuture createUser(CreateUserRequest createUserRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a user in a Simple AD or Microsoft AD directory. The status of a newly created user is "ACTIVE". New
* users can access Amazon WorkDocs.
*
*
*
* This is a convenience which creates an instance of the {@link CreateUserRequest.Builder} avoiding the need to
* create one manually via {@link CreateUserRequest#builder()}
*
*
* @param createUserRequest
* A {@link Consumer} that will call methods on {@link CreateUserRequest.Builder} to create a request.
* @return A Java Future containing the result of the CreateUser operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - EntityAlreadyExistsException The resource already exists.
* - UnauthorizedOperationException The operation is not permitted.
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.CreateUser
* @see AWS API
* Documentation
*/
default CompletableFuture createUser(Consumer createUserRequest) {
return createUser(CreateUserRequest.builder().applyMutation(createUserRequest).build());
}
/**
*
* Deactivates the specified user, which revokes the user's access to Amazon WorkDocs.
*
*
* @param deactivateUserRequest
* @return A Java Future containing the result of the DeactivateUser operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - EntityNotExistsException The resource does not exist.
* - UnauthorizedOperationException The operation is not permitted.
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.DeactivateUser
* @see AWS API
* Documentation
*/
default CompletableFuture deactivateUser(DeactivateUserRequest deactivateUserRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deactivates the specified user, which revokes the user's access to Amazon WorkDocs.
*
*
*
* This is a convenience which creates an instance of the {@link DeactivateUserRequest.Builder} avoiding the need to
* create one manually via {@link DeactivateUserRequest#builder()}
*
*
* @param deactivateUserRequest
* A {@link Consumer} that will call methods on {@link DeactivateUserRequest.Builder} to create a request.
* @return A Java Future containing the result of the DeactivateUser operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - EntityNotExistsException The resource does not exist.
* - UnauthorizedOperationException The operation is not permitted.
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.DeactivateUser
* @see AWS API
* Documentation
*/
default CompletableFuture deactivateUser(Consumer deactivateUserRequest) {
return deactivateUser(DeactivateUserRequest.builder().applyMutation(deactivateUserRequest).build());
}
/**
*
* Deletes the specified comment from the document version.
*
*
* @param deleteCommentRequest
* @return A Java Future containing the result of the DeleteComment operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - EntityNotExistsException The resource does not exist.
* - ProhibitedStateException The specified document version is not in the INITIALIZED state.
* - UnauthorizedOperationException The operation is not permitted.
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - DocumentLockedForCommentsException This exception is thrown when the document is locked for comments
* and user tries to create or delete a comment on that document.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.DeleteComment
* @see AWS API
* Documentation
*/
default CompletableFuture deleteComment(DeleteCommentRequest deleteCommentRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the specified comment from the document version.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteCommentRequest.Builder} avoiding the need to
* create one manually via {@link DeleteCommentRequest#builder()}
*
*
* @param deleteCommentRequest
* A {@link Consumer} that will call methods on {@link DeleteCommentRequest.Builder} to create a request.
* @return A Java Future containing the result of the DeleteComment operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - EntityNotExistsException The resource does not exist.
* - ProhibitedStateException The specified document version is not in the INITIALIZED state.
* - UnauthorizedOperationException The operation is not permitted.
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - DocumentLockedForCommentsException This exception is thrown when the document is locked for comments
* and user tries to create or delete a comment on that document.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.DeleteComment
* @see AWS API
* Documentation
*/
default CompletableFuture deleteComment(Consumer deleteCommentRequest) {
return deleteComment(DeleteCommentRequest.builder().applyMutation(deleteCommentRequest).build());
}
/**
*
* Deletes custom metadata from the specified resource.
*
*
* @param deleteCustomMetadataRequest
* @return A Java Future containing the result of the DeleteCustomMetadata operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - EntityNotExistsException The resource does not exist.
* - UnauthorizedOperationException The operation is not permitted.
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - ProhibitedStateException The specified document version is not in the INITIALIZED state.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.DeleteCustomMetadata
* @see AWS
* API Documentation
*/
default CompletableFuture deleteCustomMetadata(
DeleteCustomMetadataRequest deleteCustomMetadataRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes custom metadata from the specified resource.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteCustomMetadataRequest.Builder} avoiding the
* need to create one manually via {@link DeleteCustomMetadataRequest#builder()}
*
*
* @param deleteCustomMetadataRequest
* A {@link Consumer} that will call methods on {@link DeleteCustomMetadataRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DeleteCustomMetadata operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - EntityNotExistsException The resource does not exist.
* - UnauthorizedOperationException The operation is not permitted.
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - ProhibitedStateException The specified document version is not in the INITIALIZED state.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.DeleteCustomMetadata
* @see AWS
* API Documentation
*/
default CompletableFuture deleteCustomMetadata(
Consumer deleteCustomMetadataRequest) {
return deleteCustomMetadata(DeleteCustomMetadataRequest.builder().applyMutation(deleteCustomMetadataRequest).build());
}
/**
*
* Permanently deletes the specified document and its associated metadata.
*
*
* @param deleteDocumentRequest
* @return A Java Future containing the result of the DeleteDocument operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - EntityNotExistsException The resource does not exist.
* - ProhibitedStateException The specified document version is not in the INITIALIZED state.
* - ConflictingOperationException Another operation is in progress on the resource that conflicts with
* the current operation.
* - ConcurrentModificationException The resource hierarchy is changing.
* - UnauthorizedOperationException The operation is not permitted.
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.DeleteDocument
* @see AWS API
* Documentation
*/
default CompletableFuture deleteDocument(DeleteDocumentRequest deleteDocumentRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Permanently deletes the specified document and its associated metadata.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteDocumentRequest.Builder} avoiding the need to
* create one manually via {@link DeleteDocumentRequest#builder()}
*
*
* @param deleteDocumentRequest
* A {@link Consumer} that will call methods on {@link DeleteDocumentRequest.Builder} to create a request.
* @return A Java Future containing the result of the DeleteDocument operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - EntityNotExistsException The resource does not exist.
* - ProhibitedStateException The specified document version is not in the INITIALIZED state.
* - ConflictingOperationException Another operation is in progress on the resource that conflicts with
* the current operation.
* - ConcurrentModificationException The resource hierarchy is changing.
* - UnauthorizedOperationException The operation is not permitted.
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.DeleteDocument
* @see AWS API
* Documentation
*/
default CompletableFuture deleteDocument(Consumer deleteDocumentRequest) {
return deleteDocument(DeleteDocumentRequest.builder().applyMutation(deleteDocumentRequest).build());
}
/**
*
* Permanently deletes the specified folder and its contents.
*
*
* @param deleteFolderRequest
* @return A Java Future containing the result of the DeleteFolder operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - EntityNotExistsException The resource does not exist.
* - ProhibitedStateException The specified document version is not in the INITIALIZED state.
* - ConflictingOperationException Another operation is in progress on the resource that conflicts with
* the current operation.
* - ConcurrentModificationException The resource hierarchy is changing.
* - UnauthorizedOperationException The operation is not permitted.
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.DeleteFolder
* @see AWS API
* Documentation
*/
default CompletableFuture deleteFolder(DeleteFolderRequest deleteFolderRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Permanently deletes the specified folder and its contents.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteFolderRequest.Builder} avoiding the need to
* create one manually via {@link DeleteFolderRequest#builder()}
*
*
* @param deleteFolderRequest
* A {@link Consumer} that will call methods on {@link DeleteFolderRequest.Builder} to create a request.
* @return A Java Future containing the result of the DeleteFolder operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - EntityNotExistsException The resource does not exist.
* - ProhibitedStateException The specified document version is not in the INITIALIZED state.
* - ConflictingOperationException Another operation is in progress on the resource that conflicts with
* the current operation.
* - ConcurrentModificationException The resource hierarchy is changing.
* - UnauthorizedOperationException The operation is not permitted.
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.DeleteFolder
* @see AWS API
* Documentation
*/
default CompletableFuture deleteFolder(Consumer deleteFolderRequest) {
return deleteFolder(DeleteFolderRequest.builder().applyMutation(deleteFolderRequest).build());
}
/**
*
* Deletes the contents of the specified folder.
*
*
* @param deleteFolderContentsRequest
* @return A Java Future containing the result of the DeleteFolderContents operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - EntityNotExistsException The resource does not exist.
* - ProhibitedStateException The specified document version is not in the INITIALIZED state.
* - ConflictingOperationException Another operation is in progress on the resource that conflicts with
* the current operation.
* - UnauthorizedOperationException The operation is not permitted.
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.DeleteFolderContents
* @see AWS
* API Documentation
*/
default CompletableFuture deleteFolderContents(
DeleteFolderContentsRequest deleteFolderContentsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the contents of the specified folder.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteFolderContentsRequest.Builder} avoiding the
* need to create one manually via {@link DeleteFolderContentsRequest#builder()}
*
*
* @param deleteFolderContentsRequest
* A {@link Consumer} that will call methods on {@link DeleteFolderContentsRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DeleteFolderContents operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - EntityNotExistsException The resource does not exist.
* - ProhibitedStateException The specified document version is not in the INITIALIZED state.
* - ConflictingOperationException Another operation is in progress on the resource that conflicts with
* the current operation.
* - UnauthorizedOperationException The operation is not permitted.
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.DeleteFolderContents
* @see AWS
* API Documentation
*/
default CompletableFuture deleteFolderContents(
Consumer deleteFolderContentsRequest) {
return deleteFolderContents(DeleteFolderContentsRequest.builder().applyMutation(deleteFolderContentsRequest).build());
}
/**
*
* Deletes the specified list of labels from a resource.
*
*
* @param deleteLabelsRequest
* @return A Java Future containing the result of the DeleteLabels operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - EntityNotExistsException The resource does not exist.
* - UnauthorizedOperationException The operation is not permitted.
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.DeleteLabels
* @see AWS API
* Documentation
*/
default CompletableFuture deleteLabels(DeleteLabelsRequest deleteLabelsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the specified list of labels from a resource.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteLabelsRequest.Builder} avoiding the need to
* create one manually via {@link DeleteLabelsRequest#builder()}
*
*
* @param deleteLabelsRequest
* A {@link Consumer} that will call methods on {@link DeleteLabelsRequest.Builder} to create a request.
* @return A Java Future containing the result of the DeleteLabels operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - EntityNotExistsException The resource does not exist.
* - UnauthorizedOperationException The operation is not permitted.
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.DeleteLabels
* @see AWS API
* Documentation
*/
default CompletableFuture deleteLabels(Consumer deleteLabelsRequest) {
return deleteLabels(DeleteLabelsRequest.builder().applyMutation(deleteLabelsRequest).build());
}
/**
*
* Deletes the specified subscription from the specified organization.
*
*
* @param deleteNotificationSubscriptionRequest
* @return A Java Future containing the result of the DeleteNotificationSubscription operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - EntityNotExistsException The resource does not exist.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - ProhibitedStateException The specified document version is not in the INITIALIZED state.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.DeleteNotificationSubscription
* @see AWS API Documentation
*/
default CompletableFuture deleteNotificationSubscription(
DeleteNotificationSubscriptionRequest deleteNotificationSubscriptionRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the specified subscription from the specified organization.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteNotificationSubscriptionRequest.Builder}
* avoiding the need to create one manually via {@link DeleteNotificationSubscriptionRequest#builder()}
*
*
* @param deleteNotificationSubscriptionRequest
* A {@link Consumer} that will call methods on {@link DeleteNotificationSubscriptionRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the DeleteNotificationSubscription operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - EntityNotExistsException The resource does not exist.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - ProhibitedStateException The specified document version is not in the INITIALIZED state.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.DeleteNotificationSubscription
* @see AWS API Documentation
*/
default CompletableFuture deleteNotificationSubscription(
Consumer deleteNotificationSubscriptionRequest) {
return deleteNotificationSubscription(DeleteNotificationSubscriptionRequest.builder()
.applyMutation(deleteNotificationSubscriptionRequest).build());
}
/**
*
* Deletes the specified user from a Simple AD or Microsoft AD directory.
*
*
* @param deleteUserRequest
* @return A Java Future containing the result of the DeleteUser operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - EntityNotExistsException The resource does not exist.
* - UnauthorizedOperationException The operation is not permitted.
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.DeleteUser
* @see AWS API
* Documentation
*/
default CompletableFuture deleteUser(DeleteUserRequest deleteUserRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the specified user from a Simple AD or Microsoft AD directory.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteUserRequest.Builder} avoiding the need to
* create one manually via {@link DeleteUserRequest#builder()}
*
*
* @param deleteUserRequest
* A {@link Consumer} that will call methods on {@link DeleteUserRequest.Builder} to create a request.
* @return A Java Future containing the result of the DeleteUser operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - EntityNotExistsException The resource does not exist.
* - UnauthorizedOperationException The operation is not permitted.
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.DeleteUser
* @see AWS API
* Documentation
*/
default CompletableFuture deleteUser(Consumer deleteUserRequest) {
return deleteUser(DeleteUserRequest.builder().applyMutation(deleteUserRequest).build());
}
/**
*
* Describes the user activities in a specified time period.
*
*
* @param describeActivitiesRequest
* @return A Java Future containing the result of the DescribeActivities operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - UnauthorizedOperationException The operation is not permitted.
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - InvalidArgumentException The pagination marker or limit fields are not valid.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.DescribeActivities
* @see AWS
* API Documentation
*/
default CompletableFuture describeActivities(DescribeActivitiesRequest describeActivitiesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Describes the user activities in a specified time period.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeActivitiesRequest.Builder} avoiding the
* need to create one manually via {@link DescribeActivitiesRequest#builder()}
*
*
* @param describeActivitiesRequest
* A {@link Consumer} that will call methods on {@link DescribeActivitiesRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DescribeActivities operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - UnauthorizedOperationException The operation is not permitted.
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - InvalidArgumentException The pagination marker or limit fields are not valid.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.DescribeActivities
* @see AWS
* API Documentation
*/
default CompletableFuture describeActivities(
Consumer describeActivitiesRequest) {
return describeActivities(DescribeActivitiesRequest.builder().applyMutation(describeActivitiesRequest).build());
}
/**
*
* List all the comments for the specified document version.
*
*
* @param describeCommentsRequest
* @return A Java Future containing the result of the DescribeComments operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - EntityNotExistsException The resource does not exist.
* - ProhibitedStateException The specified document version is not in the INITIALIZED state.
* - UnauthorizedOperationException The operation is not permitted.
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.DescribeComments
* @see AWS API
* Documentation
*/
default CompletableFuture describeComments(DescribeCommentsRequest describeCommentsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* List all the comments for the specified document version.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeCommentsRequest.Builder} avoiding the need
* to create one manually via {@link DescribeCommentsRequest#builder()}
*
*
* @param describeCommentsRequest
* A {@link Consumer} that will call methods on {@link DescribeCommentsRequest.Builder} to create a request.
* @return A Java Future containing the result of the DescribeComments operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - EntityNotExistsException The resource does not exist.
* - ProhibitedStateException The specified document version is not in the INITIALIZED state.
* - UnauthorizedOperationException The operation is not permitted.
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.DescribeComments
* @see AWS API
* Documentation
*/
default CompletableFuture describeComments(
Consumer describeCommentsRequest) {
return describeComments(DescribeCommentsRequest.builder().applyMutation(describeCommentsRequest).build());
}
/**
*
* Retrieves the document versions for the specified document.
*
*
* By default, only active versions are returned.
*
*
* @param describeDocumentVersionsRequest
* @return A Java Future containing the result of the DescribeDocumentVersions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - EntityNotExistsException The resource does not exist.
* - UnauthorizedOperationException The operation is not permitted.
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - InvalidArgumentException The pagination marker or limit fields are not valid.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - ProhibitedStateException The specified document version is not in the INITIALIZED state.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.DescribeDocumentVersions
* @see AWS API Documentation
*/
default CompletableFuture describeDocumentVersions(
DescribeDocumentVersionsRequest describeDocumentVersionsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the document versions for the specified document.
*
*
* By default, only active versions are returned.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeDocumentVersionsRequest.Builder} avoiding
* the need to create one manually via {@link DescribeDocumentVersionsRequest#builder()}
*
*
* @param describeDocumentVersionsRequest
* A {@link Consumer} that will call methods on {@link DescribeDocumentVersionsRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DescribeDocumentVersions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - EntityNotExistsException The resource does not exist.
* - UnauthorizedOperationException The operation is not permitted.
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - InvalidArgumentException The pagination marker or limit fields are not valid.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - ProhibitedStateException The specified document version is not in the INITIALIZED state.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.DescribeDocumentVersions
* @see AWS API Documentation
*/
default CompletableFuture describeDocumentVersions(
Consumer describeDocumentVersionsRequest) {
return describeDocumentVersions(DescribeDocumentVersionsRequest.builder().applyMutation(describeDocumentVersionsRequest)
.build());
}
/**
*
* Retrieves the document versions for the specified document.
*
*
* By default, only active versions are returned.
*
*
*
* This is a variant of
* {@link #describeDocumentVersions(software.amazon.awssdk.services.workdocs.model.DescribeDocumentVersionsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.workdocs.paginators.DescribeDocumentVersionsPublisher publisher = client.describeDocumentVersionsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.workdocs.paginators.DescribeDocumentVersionsPublisher publisher = client.describeDocumentVersionsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.workdocs.model.DescribeDocumentVersionsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of Limit won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeDocumentVersions(software.amazon.awssdk.services.workdocs.model.DescribeDocumentVersionsRequest)}
* operation.
*
*
* @param describeDocumentVersionsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - EntityNotExistsException The resource does not exist.
* - UnauthorizedOperationException The operation is not permitted.
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - InvalidArgumentException The pagination marker or limit fields are not valid.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - ProhibitedStateException The specified document version is not in the INITIALIZED state.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.DescribeDocumentVersions
* @see AWS API Documentation
*/
default DescribeDocumentVersionsPublisher describeDocumentVersionsPaginator(
DescribeDocumentVersionsRequest describeDocumentVersionsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the document versions for the specified document.
*
*
* By default, only active versions are returned.
*
*
*
* This is a variant of
* {@link #describeDocumentVersions(software.amazon.awssdk.services.workdocs.model.DescribeDocumentVersionsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.workdocs.paginators.DescribeDocumentVersionsPublisher publisher = client.describeDocumentVersionsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.workdocs.paginators.DescribeDocumentVersionsPublisher publisher = client.describeDocumentVersionsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.workdocs.model.DescribeDocumentVersionsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of Limit won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeDocumentVersions(software.amazon.awssdk.services.workdocs.model.DescribeDocumentVersionsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link DescribeDocumentVersionsRequest.Builder} avoiding
* the need to create one manually via {@link DescribeDocumentVersionsRequest#builder()}
*
*
* @param describeDocumentVersionsRequest
* A {@link Consumer} that will call methods on {@link DescribeDocumentVersionsRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - EntityNotExistsException The resource does not exist.
* - UnauthorizedOperationException The operation is not permitted.
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - InvalidArgumentException The pagination marker or limit fields are not valid.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - ProhibitedStateException The specified document version is not in the INITIALIZED state.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.DescribeDocumentVersions
* @see AWS API Documentation
*/
default DescribeDocumentVersionsPublisher describeDocumentVersionsPaginator(
Consumer describeDocumentVersionsRequest) {
return describeDocumentVersionsPaginator(DescribeDocumentVersionsRequest.builder()
.applyMutation(describeDocumentVersionsRequest).build());
}
/**
*
* Describes the contents of the specified folder, including its documents and subfolders.
*
*
* By default, Amazon WorkDocs returns the first 100 active document and folder metadata items. If there are more
* results, the response includes a marker that you can use to request the next set of results. You can also request
* initialized documents.
*
*
* @param describeFolderContentsRequest
* @return A Java Future containing the result of the DescribeFolderContents operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - EntityNotExistsException The resource does not exist.
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - InvalidArgumentException The pagination marker or limit fields are not valid.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - ProhibitedStateException The specified document version is not in the INITIALIZED state.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.DescribeFolderContents
* @see AWS API Documentation
*/
default CompletableFuture describeFolderContents(
DescribeFolderContentsRequest describeFolderContentsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Describes the contents of the specified folder, including its documents and subfolders.
*
*
* By default, Amazon WorkDocs returns the first 100 active document and folder metadata items. If there are more
* results, the response includes a marker that you can use to request the next set of results. You can also request
* initialized documents.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeFolderContentsRequest.Builder} avoiding the
* need to create one manually via {@link DescribeFolderContentsRequest#builder()}
*
*
* @param describeFolderContentsRequest
* A {@link Consumer} that will call methods on {@link DescribeFolderContentsRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DescribeFolderContents operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - EntityNotExistsException The resource does not exist.
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - InvalidArgumentException The pagination marker or limit fields are not valid.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - ProhibitedStateException The specified document version is not in the INITIALIZED state.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.DescribeFolderContents
* @see AWS API Documentation
*/
default CompletableFuture describeFolderContents(
Consumer describeFolderContentsRequest) {
return describeFolderContents(DescribeFolderContentsRequest.builder().applyMutation(describeFolderContentsRequest)
.build());
}
/**
*
* Describes the contents of the specified folder, including its documents and subfolders.
*
*
* By default, Amazon WorkDocs returns the first 100 active document and folder metadata items. If there are more
* results, the response includes a marker that you can use to request the next set of results. You can also request
* initialized documents.
*
*
*
* This is a variant of
* {@link #describeFolderContents(software.amazon.awssdk.services.workdocs.model.DescribeFolderContentsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.workdocs.paginators.DescribeFolderContentsPublisher publisher = client.describeFolderContentsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.workdocs.paginators.DescribeFolderContentsPublisher publisher = client.describeFolderContentsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.workdocs.model.DescribeFolderContentsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of Limit won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeFolderContents(software.amazon.awssdk.services.workdocs.model.DescribeFolderContentsRequest)}
* operation.
*
*
* @param describeFolderContentsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - EntityNotExistsException The resource does not exist.
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - InvalidArgumentException The pagination marker or limit fields are not valid.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - ProhibitedStateException The specified document version is not in the INITIALIZED state.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.DescribeFolderContents
* @see AWS API Documentation
*/
default DescribeFolderContentsPublisher describeFolderContentsPaginator(
DescribeFolderContentsRequest describeFolderContentsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Describes the contents of the specified folder, including its documents and subfolders.
*
*
* By default, Amazon WorkDocs returns the first 100 active document and folder metadata items. If there are more
* results, the response includes a marker that you can use to request the next set of results. You can also request
* initialized documents.
*
*
*
* This is a variant of
* {@link #describeFolderContents(software.amazon.awssdk.services.workdocs.model.DescribeFolderContentsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.workdocs.paginators.DescribeFolderContentsPublisher publisher = client.describeFolderContentsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.workdocs.paginators.DescribeFolderContentsPublisher publisher = client.describeFolderContentsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.workdocs.model.DescribeFolderContentsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of Limit won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeFolderContents(software.amazon.awssdk.services.workdocs.model.DescribeFolderContentsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link DescribeFolderContentsRequest.Builder} avoiding the
* need to create one manually via {@link DescribeFolderContentsRequest#builder()}
*
*
* @param describeFolderContentsRequest
* A {@link Consumer} that will call methods on {@link DescribeFolderContentsRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - EntityNotExistsException The resource does not exist.
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - InvalidArgumentException The pagination marker or limit fields are not valid.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - ProhibitedStateException The specified document version is not in the INITIALIZED state.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.DescribeFolderContents
* @see AWS API Documentation
*/
default DescribeFolderContentsPublisher describeFolderContentsPaginator(
Consumer describeFolderContentsRequest) {
return describeFolderContentsPaginator(DescribeFolderContentsRequest.builder()
.applyMutation(describeFolderContentsRequest).build());
}
/**
*
* Describes the groups specified by the query. Groups are defined by the underlying Active Directory.
*
*
* @param describeGroupsRequest
* @return A Java Future containing the result of the DescribeGroups operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - UnauthorizedOperationException The operation is not permitted.
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.DescribeGroups
* @see AWS API
* Documentation
*/
default CompletableFuture describeGroups(DescribeGroupsRequest describeGroupsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Describes the groups specified by the query. Groups are defined by the underlying Active Directory.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeGroupsRequest.Builder} avoiding the need to
* create one manually via {@link DescribeGroupsRequest#builder()}
*
*
* @param describeGroupsRequest
* A {@link Consumer} that will call methods on {@link DescribeGroupsRequest.Builder} to create a request.
* @return A Java Future containing the result of the DescribeGroups operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - UnauthorizedOperationException The operation is not permitted.
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.DescribeGroups
* @see AWS API
* Documentation
*/
default CompletableFuture describeGroups(Consumer describeGroupsRequest) {
return describeGroups(DescribeGroupsRequest.builder().applyMutation(describeGroupsRequest).build());
}
/**
*
* Lists the specified notification subscriptions.
*
*
* @param describeNotificationSubscriptionsRequest
* @return A Java Future containing the result of the DescribeNotificationSubscriptions operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - EntityNotExistsException The resource does not exist.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.DescribeNotificationSubscriptions
* @see AWS API Documentation
*/
default CompletableFuture describeNotificationSubscriptions(
DescribeNotificationSubscriptionsRequest describeNotificationSubscriptionsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists the specified notification subscriptions.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeNotificationSubscriptionsRequest.Builder}
* avoiding the need to create one manually via {@link DescribeNotificationSubscriptionsRequest#builder()}
*
*
* @param describeNotificationSubscriptionsRequest
* A {@link Consumer} that will call methods on {@link DescribeNotificationSubscriptionsRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the DescribeNotificationSubscriptions operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - EntityNotExistsException The resource does not exist.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.DescribeNotificationSubscriptions
* @see AWS API Documentation
*/
default CompletableFuture describeNotificationSubscriptions(
Consumer describeNotificationSubscriptionsRequest) {
return describeNotificationSubscriptions(DescribeNotificationSubscriptionsRequest.builder()
.applyMutation(describeNotificationSubscriptionsRequest).build());
}
/**
*
* Describes the permissions of a specified resource.
*
*
* @param describeResourcePermissionsRequest
* @return A Java Future containing the result of the DescribeResourcePermissions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - UnauthorizedOperationException The operation is not permitted.
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.DescribeResourcePermissions
* @see AWS API Documentation
*/
default CompletableFuture describeResourcePermissions(
DescribeResourcePermissionsRequest describeResourcePermissionsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Describes the permissions of a specified resource.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeResourcePermissionsRequest.Builder}
* avoiding the need to create one manually via {@link DescribeResourcePermissionsRequest#builder()}
*
*
* @param describeResourcePermissionsRequest
* A {@link Consumer} that will call methods on {@link DescribeResourcePermissionsRequest.Builder} to create
* a request.
* @return A Java Future containing the result of the DescribeResourcePermissions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - UnauthorizedOperationException The operation is not permitted.
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.DescribeResourcePermissions
* @see AWS API Documentation
*/
default CompletableFuture describeResourcePermissions(
Consumer describeResourcePermissionsRequest) {
return describeResourcePermissions(DescribeResourcePermissionsRequest.builder()
.applyMutation(describeResourcePermissionsRequest).build());
}
/**
*
* Describes the current user's special folders; the RootFolder
and the RecycleBin
.
* RootFolder
is the root of user's files and folders and RecycleBin
is the root of
* recycled items. This is not a valid action for SigV4 (administrative API) clients.
*
*
* This action requires an authentication token. To get an authentication token, register an application with Amazon
* WorkDocs. For more information, see Authentication and Access
* Control for User Applications in the Amazon WorkDocs Developer Guide.
*
*
* @param describeRootFoldersRequest
* @return A Java Future containing the result of the DescribeRootFolders operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - UnauthorizedOperationException The operation is not permitted.
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - InvalidArgumentException The pagination marker or limit fields are not valid.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.DescribeRootFolders
* @see AWS
* API Documentation
*/
default CompletableFuture describeRootFolders(
DescribeRootFoldersRequest describeRootFoldersRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Describes the current user's special folders; the RootFolder
and the RecycleBin
.
* RootFolder
is the root of user's files and folders and RecycleBin
is the root of
* recycled items. This is not a valid action for SigV4 (administrative API) clients.
*
*
* This action requires an authentication token. To get an authentication token, register an application with Amazon
* WorkDocs. For more information, see Authentication and Access
* Control for User Applications in the Amazon WorkDocs Developer Guide.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeRootFoldersRequest.Builder} avoiding the
* need to create one manually via {@link DescribeRootFoldersRequest#builder()}
*
*
* @param describeRootFoldersRequest
* A {@link Consumer} that will call methods on {@link DescribeRootFoldersRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DescribeRootFolders operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - UnauthorizedOperationException The operation is not permitted.
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - InvalidArgumentException The pagination marker or limit fields are not valid.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.DescribeRootFolders
* @see AWS
* API Documentation
*/
default CompletableFuture describeRootFolders(
Consumer describeRootFoldersRequest) {
return describeRootFolders(DescribeRootFoldersRequest.builder().applyMutation(describeRootFoldersRequest).build());
}
/**
*
* Describes the specified users. You can describe all users or filter the results (for example, by status or
* organization).
*
*
* By default, Amazon WorkDocs returns the first 24 active or pending users. If there are more results, the response
* includes a marker that you can use to request the next set of results.
*
*
* @param describeUsersRequest
* @return A Java Future containing the result of the DescribeUsers operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - EntityNotExistsException The resource does not exist.
* - UnauthorizedOperationException The operation is not permitted.
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - InvalidArgumentException The pagination marker or limit fields are not valid.
* - RequestedEntityTooLargeException The response is too large to return. The request must include a
* filter to reduce the size of the response.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.DescribeUsers
* @see AWS API
* Documentation
*/
default CompletableFuture describeUsers(DescribeUsersRequest describeUsersRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Describes the specified users. You can describe all users or filter the results (for example, by status or
* organization).
*
*
* By default, Amazon WorkDocs returns the first 24 active or pending users. If there are more results, the response
* includes a marker that you can use to request the next set of results.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeUsersRequest.Builder} avoiding the need to
* create one manually via {@link DescribeUsersRequest#builder()}
*
*
* @param describeUsersRequest
* A {@link Consumer} that will call methods on {@link DescribeUsersRequest.Builder} to create a request.
* @return A Java Future containing the result of the DescribeUsers operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - EntityNotExistsException The resource does not exist.
* - UnauthorizedOperationException The operation is not permitted.
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - InvalidArgumentException The pagination marker or limit fields are not valid.
* - RequestedEntityTooLargeException The response is too large to return. The request must include a
* filter to reduce the size of the response.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.DescribeUsers
* @see AWS API
* Documentation
*/
default CompletableFuture describeUsers(Consumer describeUsersRequest) {
return describeUsers(DescribeUsersRequest.builder().applyMutation(describeUsersRequest).build());
}
/**
*
* Describes the specified users. You can describe all users or filter the results (for example, by status or
* organization).
*
*
* By default, Amazon WorkDocs returns the first 24 active or pending users. If there are more results, the response
* includes a marker that you can use to request the next set of results.
*
*
*
* This is a variant of {@link #describeUsers(software.amazon.awssdk.services.workdocs.model.DescribeUsersRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.workdocs.paginators.DescribeUsersPublisher publisher = client.describeUsersPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.workdocs.paginators.DescribeUsersPublisher publisher = client.describeUsersPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.workdocs.model.DescribeUsersResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of Limit won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeUsers(software.amazon.awssdk.services.workdocs.model.DescribeUsersRequest)} operation.
*
*
* @param describeUsersRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - EntityNotExistsException The resource does not exist.
* - UnauthorizedOperationException The operation is not permitted.
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - InvalidArgumentException The pagination marker or limit fields are not valid.
* - RequestedEntityTooLargeException The response is too large to return. The request must include a
* filter to reduce the size of the response.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.DescribeUsers
* @see AWS API
* Documentation
*/
default DescribeUsersPublisher describeUsersPaginator(DescribeUsersRequest describeUsersRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Describes the specified users. You can describe all users or filter the results (for example, by status or
* organization).
*
*
* By default, Amazon WorkDocs returns the first 24 active or pending users. If there are more results, the response
* includes a marker that you can use to request the next set of results.
*
*
*
* This is a variant of {@link #describeUsers(software.amazon.awssdk.services.workdocs.model.DescribeUsersRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.workdocs.paginators.DescribeUsersPublisher publisher = client.describeUsersPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.workdocs.paginators.DescribeUsersPublisher publisher = client.describeUsersPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.workdocs.model.DescribeUsersResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of Limit won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeUsers(software.amazon.awssdk.services.workdocs.model.DescribeUsersRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link DescribeUsersRequest.Builder} avoiding the need to
* create one manually via {@link DescribeUsersRequest#builder()}
*
*
* @param describeUsersRequest
* A {@link Consumer} that will call methods on {@link DescribeUsersRequest.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - EntityNotExistsException The resource does not exist.
* - UnauthorizedOperationException The operation is not permitted.
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - InvalidArgumentException The pagination marker or limit fields are not valid.
* - RequestedEntityTooLargeException The response is too large to return. The request must include a
* filter to reduce the size of the response.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.DescribeUsers
* @see AWS API
* Documentation
*/
default DescribeUsersPublisher describeUsersPaginator(Consumer describeUsersRequest) {
return describeUsersPaginator(DescribeUsersRequest.builder().applyMutation(describeUsersRequest).build());
}
/**
*
* Retrieves details of the current user for whom the authentication token was generated. This is not a valid action
* for SigV4 (administrative API) clients.
*
*
* This action requires an authentication token. To get an authentication token, register an application with Amazon
* WorkDocs. For more information, see Authentication and Access
* Control for User Applications in the Amazon WorkDocs Developer Guide.
*
*
* @param getCurrentUserRequest
* @return A Java Future containing the result of the GetCurrentUser operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - EntityNotExistsException The resource does not exist.
* - UnauthorizedOperationException The operation is not permitted.
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.GetCurrentUser
* @see AWS API
* Documentation
*/
default CompletableFuture getCurrentUser(GetCurrentUserRequest getCurrentUserRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves details of the current user for whom the authentication token was generated. This is not a valid action
* for SigV4 (administrative API) clients.
*
*
* This action requires an authentication token. To get an authentication token, register an application with Amazon
* WorkDocs. For more information, see Authentication and Access
* Control for User Applications in the Amazon WorkDocs Developer Guide.
*
*
*
* This is a convenience which creates an instance of the {@link GetCurrentUserRequest.Builder} avoiding the need to
* create one manually via {@link GetCurrentUserRequest#builder()}
*
*
* @param getCurrentUserRequest
* A {@link Consumer} that will call methods on {@link GetCurrentUserRequest.Builder} to create a request.
* @return A Java Future containing the result of the GetCurrentUser operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - EntityNotExistsException The resource does not exist.
* - UnauthorizedOperationException The operation is not permitted.
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.GetCurrentUser
* @see AWS API
* Documentation
*/
default CompletableFuture getCurrentUser(Consumer getCurrentUserRequest) {
return getCurrentUser(GetCurrentUserRequest.builder().applyMutation(getCurrentUserRequest).build());
}
/**
*
* Retrieves details of a document.
*
*
* @param getDocumentRequest
* @return A Java Future containing the result of the GetDocument operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - EntityNotExistsException The resource does not exist.
* - UnauthorizedOperationException The operation is not permitted.
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - InvalidArgumentException The pagination marker or limit fields are not valid.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - InvalidPasswordException The password is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.GetDocument
* @see AWS API
* Documentation
*/
default CompletableFuture getDocument(GetDocumentRequest getDocumentRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves details of a document.
*
*
*
* This is a convenience which creates an instance of the {@link GetDocumentRequest.Builder} avoiding the need to
* create one manually via {@link GetDocumentRequest#builder()}
*
*
* @param getDocumentRequest
* A {@link Consumer} that will call methods on {@link GetDocumentRequest.Builder} to create a request.
* @return A Java Future containing the result of the GetDocument operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - EntityNotExistsException The resource does not exist.
* - UnauthorizedOperationException The operation is not permitted.
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - InvalidArgumentException The pagination marker or limit fields are not valid.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - InvalidPasswordException The password is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.GetDocument
* @see AWS API
* Documentation
*/
default CompletableFuture getDocument(Consumer getDocumentRequest) {
return getDocument(GetDocumentRequest.builder().applyMutation(getDocumentRequest).build());
}
/**
*
* Retrieves the path information (the hierarchy from the root folder) for the requested document.
*
*
* By default, Amazon WorkDocs returns a maximum of 100 levels upwards from the requested document and only includes
* the IDs of the parent folders in the path. You can limit the maximum number of levels. You can also request the
* names of the parent folders.
*
*
* @param getDocumentPathRequest
* @return A Java Future containing the result of the GetDocumentPath operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - EntityNotExistsException The resource does not exist.
* - UnauthorizedOperationException The operation is not permitted.
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.GetDocumentPath
* @see AWS API
* Documentation
*/
default CompletableFuture getDocumentPath(GetDocumentPathRequest getDocumentPathRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the path information (the hierarchy from the root folder) for the requested document.
*
*
* By default, Amazon WorkDocs returns a maximum of 100 levels upwards from the requested document and only includes
* the IDs of the parent folders in the path. You can limit the maximum number of levels. You can also request the
* names of the parent folders.
*
*
*
* This is a convenience which creates an instance of the {@link GetDocumentPathRequest.Builder} avoiding the need
* to create one manually via {@link GetDocumentPathRequest#builder()}
*
*
* @param getDocumentPathRequest
* A {@link Consumer} that will call methods on {@link GetDocumentPathRequest.Builder} to create a request.
* @return A Java Future containing the result of the GetDocumentPath operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - EntityNotExistsException The resource does not exist.
* - UnauthorizedOperationException The operation is not permitted.
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.GetDocumentPath
* @see AWS API
* Documentation
*/
default CompletableFuture getDocumentPath(
Consumer getDocumentPathRequest) {
return getDocumentPath(GetDocumentPathRequest.builder().applyMutation(getDocumentPathRequest).build());
}
/**
*
* Retrieves version metadata for the specified document.
*
*
* @param getDocumentVersionRequest
* @return A Java Future containing the result of the GetDocumentVersion operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - EntityNotExistsException The resource does not exist.
* - UnauthorizedOperationException The operation is not permitted.
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - ProhibitedStateException The specified document version is not in the INITIALIZED state.
* - InvalidPasswordException The password is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.GetDocumentVersion
* @see AWS
* API Documentation
*/
default CompletableFuture getDocumentVersion(GetDocumentVersionRequest getDocumentVersionRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves version metadata for the specified document.
*
*
*
* This is a convenience which creates an instance of the {@link GetDocumentVersionRequest.Builder} avoiding the
* need to create one manually via {@link GetDocumentVersionRequest#builder()}
*
*
* @param getDocumentVersionRequest
* A {@link Consumer} that will call methods on {@link GetDocumentVersionRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetDocumentVersion operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - EntityNotExistsException The resource does not exist.
* - UnauthorizedOperationException The operation is not permitted.
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - ProhibitedStateException The specified document version is not in the INITIALIZED state.
* - InvalidPasswordException The password is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.GetDocumentVersion
* @see AWS
* API Documentation
*/
default CompletableFuture getDocumentVersion(
Consumer getDocumentVersionRequest) {
return getDocumentVersion(GetDocumentVersionRequest.builder().applyMutation(getDocumentVersionRequest).build());
}
/**
*
* Retrieves the metadata of the specified folder.
*
*
* @param getFolderRequest
* @return A Java Future containing the result of the GetFolder operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - EntityNotExistsException The resource does not exist.
* - UnauthorizedOperationException The operation is not permitted.
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - InvalidArgumentException The pagination marker or limit fields are not valid.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - ProhibitedStateException The specified document version is not in the INITIALIZED state.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.GetFolder
* @see AWS API
* Documentation
*/
default CompletableFuture getFolder(GetFolderRequest getFolderRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the metadata of the specified folder.
*
*
*
* This is a convenience which creates an instance of the {@link GetFolderRequest.Builder} avoiding the need to
* create one manually via {@link GetFolderRequest#builder()}
*
*
* @param getFolderRequest
* A {@link Consumer} that will call methods on {@link GetFolderRequest.Builder} to create a request.
* @return A Java Future containing the result of the GetFolder operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - EntityNotExistsException The resource does not exist.
* - UnauthorizedOperationException The operation is not permitted.
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - InvalidArgumentException The pagination marker or limit fields are not valid.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - ProhibitedStateException The specified document version is not in the INITIALIZED state.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.GetFolder
* @see AWS API
* Documentation
*/
default CompletableFuture getFolder(Consumer getFolderRequest) {
return getFolder(GetFolderRequest.builder().applyMutation(getFolderRequest).build());
}
/**
*
* Retrieves the path information (the hierarchy from the root folder) for the specified folder.
*
*
* By default, Amazon WorkDocs returns a maximum of 100 levels upwards from the requested folder and only includes
* the IDs of the parent folders in the path. You can limit the maximum number of levels. You can also request the
* parent folder names.
*
*
* @param getFolderPathRequest
* @return A Java Future containing the result of the GetFolderPath operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - EntityNotExistsException The resource does not exist.
* - UnauthorizedOperationException The operation is not permitted.
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.GetFolderPath
* @see AWS API
* Documentation
*/
default CompletableFuture getFolderPath(GetFolderPathRequest getFolderPathRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the path information (the hierarchy from the root folder) for the specified folder.
*
*
* By default, Amazon WorkDocs returns a maximum of 100 levels upwards from the requested folder and only includes
* the IDs of the parent folders in the path. You can limit the maximum number of levels. You can also request the
* parent folder names.
*
*
*
* This is a convenience which creates an instance of the {@link GetFolderPathRequest.Builder} avoiding the need to
* create one manually via {@link GetFolderPathRequest#builder()}
*
*
* @param getFolderPathRequest
* A {@link Consumer} that will call methods on {@link GetFolderPathRequest.Builder} to create a request.
* @return A Java Future containing the result of the GetFolderPath operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - EntityNotExistsException The resource does not exist.
* - UnauthorizedOperationException The operation is not permitted.
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.GetFolderPath
* @see AWS API
* Documentation
*/
default CompletableFuture getFolderPath(Consumer getFolderPathRequest) {
return getFolderPath(GetFolderPathRequest.builder().applyMutation(getFolderPathRequest).build());
}
/**
*
* Retrieves a collection of resources, including folders and documents. The only CollectionType
* supported is SHARED_WITH_ME
.
*
*
* @param getResourcesRequest
* @return A Java Future containing the result of the GetResources operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - UnauthorizedOperationException The operation is not permitted.
* - InvalidArgumentException The pagination marker or limit fields are not valid.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.GetResources
* @see AWS API
* Documentation
*/
default CompletableFuture getResources(GetResourcesRequest getResourcesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves a collection of resources, including folders and documents. The only CollectionType
* supported is SHARED_WITH_ME
.
*
*
*
* This is a convenience which creates an instance of the {@link GetResourcesRequest.Builder} avoiding the need to
* create one manually via {@link GetResourcesRequest#builder()}
*
*
* @param getResourcesRequest
* A {@link Consumer} that will call methods on {@link GetResourcesRequest.Builder} to create a request.
* @return A Java Future containing the result of the GetResources operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - UnauthorizedOperationException The operation is not permitted.
* - InvalidArgumentException The pagination marker or limit fields are not valid.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.GetResources
* @see AWS API
* Documentation
*/
default CompletableFuture getResources(Consumer getResourcesRequest) {
return getResources(GetResourcesRequest.builder().applyMutation(getResourcesRequest).build());
}
/**
*
* Creates a new document object and version object.
*
*
* The client specifies the parent folder ID and name of the document to upload. The ID is optionally specified when
* creating a new version of an existing document. This is the first step to upload a document. Next, upload the
* document to the URL returned from the call, and then call UpdateDocumentVersion.
*
*
* To cancel the document upload, call AbortDocumentVersionUpload.
*
*
* @param initiateDocumentVersionUploadRequest
* @return A Java Future containing the result of the InitiateDocumentVersionUpload operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - EntityNotExistsException The resource does not exist.
* - EntityAlreadyExistsException The resource already exists.
* - StorageLimitExceededException The storage limit has been exceeded.
* - StorageLimitWillExceedException The storage limit will be exceeded.
* - ProhibitedStateException The specified document version is not in the INITIALIZED state.
* - UnauthorizedOperationException The operation is not permitted.
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - DraftUploadOutOfSyncException This exception is thrown when a valid checkout ID is not presented on
* document version upload calls for a document that has been checked out from Web client.
* - ResourceAlreadyCheckedOutException The resource is already checked out.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.InitiateDocumentVersionUpload
* @see AWS API Documentation
*/
default CompletableFuture initiateDocumentVersionUpload(
InitiateDocumentVersionUploadRequest initiateDocumentVersionUploadRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a new document object and version object.
*
*
* The client specifies the parent folder ID and name of the document to upload. The ID is optionally specified when
* creating a new version of an existing document. This is the first step to upload a document. Next, upload the
* document to the URL returned from the call, and then call UpdateDocumentVersion.
*
*
* To cancel the document upload, call AbortDocumentVersionUpload.
*
*
*
* This is a convenience which creates an instance of the {@link InitiateDocumentVersionUploadRequest.Builder}
* avoiding the need to create one manually via {@link InitiateDocumentVersionUploadRequest#builder()}
*
*
* @param initiateDocumentVersionUploadRequest
* A {@link Consumer} that will call methods on {@link InitiateDocumentVersionUploadRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the InitiateDocumentVersionUpload operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - EntityNotExistsException The resource does not exist.
* - EntityAlreadyExistsException The resource already exists.
* - StorageLimitExceededException The storage limit has been exceeded.
* - StorageLimitWillExceedException The storage limit will be exceeded.
* - ProhibitedStateException The specified document version is not in the INITIALIZED state.
* - UnauthorizedOperationException The operation is not permitted.
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - DraftUploadOutOfSyncException This exception is thrown when a valid checkout ID is not presented on
* document version upload calls for a document that has been checked out from Web client.
* - ResourceAlreadyCheckedOutException The resource is already checked out.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.InitiateDocumentVersionUpload
* @see AWS API Documentation
*/
default CompletableFuture initiateDocumentVersionUpload(
Consumer initiateDocumentVersionUploadRequest) {
return initiateDocumentVersionUpload(InitiateDocumentVersionUploadRequest.builder()
.applyMutation(initiateDocumentVersionUploadRequest).build());
}
/**
*
* Removes all the permissions from the specified resource.
*
*
* @param removeAllResourcePermissionsRequest
* @return A Java Future containing the result of the RemoveAllResourcePermissions operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - UnauthorizedOperationException The operation is not permitted.
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.RemoveAllResourcePermissions
* @see AWS API Documentation
*/
default CompletableFuture removeAllResourcePermissions(
RemoveAllResourcePermissionsRequest removeAllResourcePermissionsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Removes all the permissions from the specified resource.
*
*
*
* This is a convenience which creates an instance of the {@link RemoveAllResourcePermissionsRequest.Builder}
* avoiding the need to create one manually via {@link RemoveAllResourcePermissionsRequest#builder()}
*
*
* @param removeAllResourcePermissionsRequest
* A {@link Consumer} that will call methods on {@link RemoveAllResourcePermissionsRequest.Builder} to create
* a request.
* @return A Java Future containing the result of the RemoveAllResourcePermissions operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - UnauthorizedOperationException The operation is not permitted.
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.RemoveAllResourcePermissions
* @see AWS API Documentation
*/
default CompletableFuture removeAllResourcePermissions(
Consumer removeAllResourcePermissionsRequest) {
return removeAllResourcePermissions(RemoveAllResourcePermissionsRequest.builder()
.applyMutation(removeAllResourcePermissionsRequest).build());
}
/**
*
* Removes the permission for the specified principal from the specified resource.
*
*
* @param removeResourcePermissionRequest
* @return A Java Future containing the result of the RemoveResourcePermission operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - UnauthorizedOperationException The operation is not permitted.
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.RemoveResourcePermission
* @see AWS API Documentation
*/
default CompletableFuture removeResourcePermission(
RemoveResourcePermissionRequest removeResourcePermissionRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Removes the permission for the specified principal from the specified resource.
*
*
*
* This is a convenience which creates an instance of the {@link RemoveResourcePermissionRequest.Builder} avoiding
* the need to create one manually via {@link RemoveResourcePermissionRequest#builder()}
*
*
* @param removeResourcePermissionRequest
* A {@link Consumer} that will call methods on {@link RemoveResourcePermissionRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the RemoveResourcePermission operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - UnauthorizedOperationException The operation is not permitted.
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.RemoveResourcePermission
* @see AWS API Documentation
*/
default CompletableFuture removeResourcePermission(
Consumer removeResourcePermissionRequest) {
return removeResourcePermission(RemoveResourcePermissionRequest.builder().applyMutation(removeResourcePermissionRequest)
.build());
}
/**
*
* Updates the specified attributes of a document. The user must have access to both the document and its parent
* folder, if applicable.
*
*
* @param updateDocumentRequest
* @return A Java Future containing the result of the UpdateDocument operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - EntityNotExistsException The resource does not exist.
* - EntityAlreadyExistsException The resource already exists.
* - LimitExceededException The maximum of 100,000 folders under the parent folder has been exceeded.
* - ProhibitedStateException The specified document version is not in the INITIALIZED state.
* - ConflictingOperationException Another operation is in progress on the resource that conflicts with
* the current operation.
* - ConcurrentModificationException The resource hierarchy is changing.
* - UnauthorizedOperationException The operation is not permitted.
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.UpdateDocument
* @see AWS API
* Documentation
*/
default CompletableFuture updateDocument(UpdateDocumentRequest updateDocumentRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates the specified attributes of a document. The user must have access to both the document and its parent
* folder, if applicable.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateDocumentRequest.Builder} avoiding the need to
* create one manually via {@link UpdateDocumentRequest#builder()}
*
*
* @param updateDocumentRequest
* A {@link Consumer} that will call methods on {@link UpdateDocumentRequest.Builder} to create a request.
* @return A Java Future containing the result of the UpdateDocument operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - EntityNotExistsException The resource does not exist.
* - EntityAlreadyExistsException The resource already exists.
* - LimitExceededException The maximum of 100,000 folders under the parent folder has been exceeded.
* - ProhibitedStateException The specified document version is not in the INITIALIZED state.
* - ConflictingOperationException Another operation is in progress on the resource that conflicts with
* the current operation.
* - ConcurrentModificationException The resource hierarchy is changing.
* - UnauthorizedOperationException The operation is not permitted.
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.UpdateDocument
* @see AWS API
* Documentation
*/
default CompletableFuture updateDocument(Consumer updateDocumentRequest) {
return updateDocument(UpdateDocumentRequest.builder().applyMutation(updateDocumentRequest).build());
}
/**
*
* Changes the status of the document version to ACTIVE.
*
*
* Amazon WorkDocs also sets its document container to ACTIVE. This is the last step in a document upload, after the
* client uploads the document to an S3-presigned URL returned by InitiateDocumentVersionUpload.
*
*
* @param updateDocumentVersionRequest
* @return A Java Future containing the result of the UpdateDocumentVersion operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - EntityNotExistsException The resource does not exist.
* - ProhibitedStateException The specified document version is not in the INITIALIZED state.
* - ConcurrentModificationException The resource hierarchy is changing.
* - InvalidOperationException The operation is invalid.
* - UnauthorizedOperationException The operation is not permitted.
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.UpdateDocumentVersion
* @see AWS
* API Documentation
*/
default CompletableFuture updateDocumentVersion(
UpdateDocumentVersionRequest updateDocumentVersionRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Changes the status of the document version to ACTIVE.
*
*
* Amazon WorkDocs also sets its document container to ACTIVE. This is the last step in a document upload, after the
* client uploads the document to an S3-presigned URL returned by InitiateDocumentVersionUpload.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateDocumentVersionRequest.Builder} avoiding the
* need to create one manually via {@link UpdateDocumentVersionRequest#builder()}
*
*
* @param updateDocumentVersionRequest
* A {@link Consumer} that will call methods on {@link UpdateDocumentVersionRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the UpdateDocumentVersion operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - EntityNotExistsException The resource does not exist.
* - ProhibitedStateException The specified document version is not in the INITIALIZED state.
* - ConcurrentModificationException The resource hierarchy is changing.
* - InvalidOperationException The operation is invalid.
* - UnauthorizedOperationException The operation is not permitted.
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.UpdateDocumentVersion
* @see AWS
* API Documentation
*/
default CompletableFuture updateDocumentVersion(
Consumer updateDocumentVersionRequest) {
return updateDocumentVersion(UpdateDocumentVersionRequest.builder().applyMutation(updateDocumentVersionRequest).build());
}
/**
*
* Updates the specified attributes of the specified folder. The user must have access to both the folder and its
* parent folder, if applicable.
*
*
* @param updateFolderRequest
* @return A Java Future containing the result of the UpdateFolder operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - EntityNotExistsException The resource does not exist.
* - EntityAlreadyExistsException The resource already exists.
* - ProhibitedStateException The specified document version is not in the INITIALIZED state.
* - ConflictingOperationException Another operation is in progress on the resource that conflicts with
* the current operation.
* - ConcurrentModificationException The resource hierarchy is changing.
* - LimitExceededException The maximum of 100,000 folders under the parent folder has been exceeded.
* - UnauthorizedOperationException The operation is not permitted.
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.UpdateFolder
* @see AWS API
* Documentation
*/
default CompletableFuture updateFolder(UpdateFolderRequest updateFolderRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates the specified attributes of the specified folder. The user must have access to both the folder and its
* parent folder, if applicable.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateFolderRequest.Builder} avoiding the need to
* create one manually via {@link UpdateFolderRequest#builder()}
*
*
* @param updateFolderRequest
* A {@link Consumer} that will call methods on {@link UpdateFolderRequest.Builder} to create a request.
* @return A Java Future containing the result of the UpdateFolder operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - EntityNotExistsException The resource does not exist.
* - EntityAlreadyExistsException The resource already exists.
* - ProhibitedStateException The specified document version is not in the INITIALIZED state.
* - ConflictingOperationException Another operation is in progress on the resource that conflicts with
* the current operation.
* - ConcurrentModificationException The resource hierarchy is changing.
* - LimitExceededException The maximum of 100,000 folders under the parent folder has been exceeded.
* - UnauthorizedOperationException The operation is not permitted.
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.UpdateFolder
* @see AWS API
* Documentation
*/
default CompletableFuture updateFolder(Consumer updateFolderRequest) {
return updateFolder(UpdateFolderRequest.builder().applyMutation(updateFolderRequest).build());
}
/**
*
* Updates the specified attributes of the specified user, and grants or revokes administrative privileges to the
* Amazon WorkDocs site.
*
*
* @param updateUserRequest
* @return A Java Future containing the result of the UpdateUser operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - EntityNotExistsException The resource does not exist.
* - UnauthorizedOperationException The operation is not permitted.
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - IllegalUserStateException The user is undergoing transfer of ownership.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - DeactivatingLastSystemUserException The last user in the organization is being deactivated.
* - InvalidArgumentException The pagination marker or limit fields are not valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.UpdateUser
* @see AWS API
* Documentation
*/
default CompletableFuture updateUser(UpdateUserRequest updateUserRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates the specified attributes of the specified user, and grants or revokes administrative privileges to the
* Amazon WorkDocs site.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateUserRequest.Builder} avoiding the need to
* create one manually via {@link UpdateUserRequest#builder()}
*
*
* @param updateUserRequest
* A {@link Consumer} that will call methods on {@link UpdateUserRequest.Builder} to create a request.
* @return A Java Future containing the result of the UpdateUser operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - EntityNotExistsException The resource does not exist.
* - UnauthorizedOperationException The operation is not permitted.
* - UnauthorizedResourceAccessException The caller does not have access to perform the action on the
* resource.
* - IllegalUserStateException The user is undergoing transfer of ownership.
* - FailedDependencyException The AWS Directory Service cannot reach an on-premises instance. Or a
* dependency under the control of the organization is failing, such as a connected Active Directory.
* - ServiceUnavailableException One or more of the dependencies is unavailable.
* - DeactivatingLastSystemUserException The last user in the organization is being deactivated.
* - InvalidArgumentException The pagination marker or limit fields are not valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkDocsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkDocsAsyncClient.UpdateUser
* @see AWS API
* Documentation
*/
default CompletableFuture updateUser(Consumer updateUserRequest) {
return updateUser(UpdateUserRequest.builder().applyMutation(updateUserRequest).build());
}
}