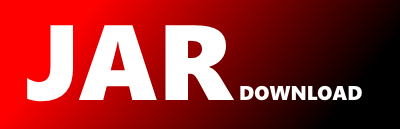
software.amazon.awssdk.services.workdocs.model.UpdateUserRequest Maven / Gradle / Ivy
Show all versions of workdocs Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.workdocs.model;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class UpdateUserRequest extends WorkDocsRequest implements
ToCopyableBuilder {
private static final SdkField AUTHENTICATION_TOKEN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AuthenticationToken").getter(getter(UpdateUserRequest::authenticationToken))
.setter(setter(Builder::authenticationToken))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("Authentication").build()).build();
private static final SdkField USER_ID_FIELD = SdkField. builder(MarshallingType.STRING).memberName("UserId")
.getter(getter(UpdateUserRequest::userId)).setter(setter(Builder::userId))
.traits(LocationTrait.builder().location(MarshallLocation.PATH).locationName("UserId").build()).build();
private static final SdkField GIVEN_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("GivenName").getter(getter(UpdateUserRequest::givenName)).setter(setter(Builder::givenName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("GivenName").build()).build();
private static final SdkField SURNAME_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Surname")
.getter(getter(UpdateUserRequest::surname)).setter(setter(Builder::surname))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Surname").build()).build();
private static final SdkField TYPE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Type")
.getter(getter(UpdateUserRequest::typeAsString)).setter(setter(Builder::type))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Type").build()).build();
private static final SdkField STORAGE_RULE_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("StorageRule")
.getter(getter(UpdateUserRequest::storageRule)).setter(setter(Builder::storageRule))
.constructor(StorageRuleType::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StorageRule").build()).build();
private static final SdkField TIME_ZONE_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TimeZoneId").getter(getter(UpdateUserRequest::timeZoneId)).setter(setter(Builder::timeZoneId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TimeZoneId").build()).build();
private static final SdkField LOCALE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Locale")
.getter(getter(UpdateUserRequest::localeAsString)).setter(setter(Builder::locale))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Locale").build()).build();
private static final SdkField GRANT_POWERUSER_PRIVILEGES_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("GrantPoweruserPrivileges").getter(getter(UpdateUserRequest::grantPoweruserPrivilegesAsString))
.setter(setter(Builder::grantPoweruserPrivileges))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("GrantPoweruserPrivileges").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(AUTHENTICATION_TOKEN_FIELD,
USER_ID_FIELD, GIVEN_NAME_FIELD, SURNAME_FIELD, TYPE_FIELD, STORAGE_RULE_FIELD, TIME_ZONE_ID_FIELD, LOCALE_FIELD,
GRANT_POWERUSER_PRIVILEGES_FIELD));
private final String authenticationToken;
private final String userId;
private final String givenName;
private final String surname;
private final String type;
private final StorageRuleType storageRule;
private final String timeZoneId;
private final String locale;
private final String grantPoweruserPrivileges;
private UpdateUserRequest(BuilderImpl builder) {
super(builder);
this.authenticationToken = builder.authenticationToken;
this.userId = builder.userId;
this.givenName = builder.givenName;
this.surname = builder.surname;
this.type = builder.type;
this.storageRule = builder.storageRule;
this.timeZoneId = builder.timeZoneId;
this.locale = builder.locale;
this.grantPoweruserPrivileges = builder.grantPoweruserPrivileges;
}
/**
*
* Amazon WorkDocs authentication token. Not required when using AWS administrator credentials to access the API.
*
*
* @return Amazon WorkDocs authentication token. Not required when using AWS administrator credentials to access the
* API.
*/
public String authenticationToken() {
return authenticationToken;
}
/**
*
* The ID of the user.
*
*
* @return The ID of the user.
*/
public String userId() {
return userId;
}
/**
*
* The given name of the user.
*
*
* @return The given name of the user.
*/
public String givenName() {
return givenName;
}
/**
*
* The surname of the user.
*
*
* @return The surname of the user.
*/
public String surname() {
return surname;
}
/**
*
* The type of the user.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #type} will return
* {@link UserType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #typeAsString}.
*
*
* @return The type of the user.
* @see UserType
*/
public UserType type() {
return UserType.fromValue(type);
}
/**
*
* The type of the user.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #type} will return
* {@link UserType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #typeAsString}.
*
*
* @return The type of the user.
* @see UserType
*/
public String typeAsString() {
return type;
}
/**
*
* The amount of storage for the user.
*
*
* @return The amount of storage for the user.
*/
public StorageRuleType storageRule() {
return storageRule;
}
/**
*
* The time zone ID of the user.
*
*
* @return The time zone ID of the user.
*/
public String timeZoneId() {
return timeZoneId;
}
/**
*
* The locale of the user.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #locale} will
* return {@link LocaleType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #localeAsString}.
*
*
* @return The locale of the user.
* @see LocaleType
*/
public LocaleType locale() {
return LocaleType.fromValue(locale);
}
/**
*
* The locale of the user.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #locale} will
* return {@link LocaleType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #localeAsString}.
*
*
* @return The locale of the user.
* @see LocaleType
*/
public String localeAsString() {
return locale;
}
/**
*
* Boolean value to determine whether the user is granted Poweruser privileges.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #grantPoweruserPrivileges} will return {@link BooleanEnumType#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #grantPoweruserPrivilegesAsString}.
*
*
* @return Boolean value to determine whether the user is granted Poweruser privileges.
* @see BooleanEnumType
*/
public BooleanEnumType grantPoweruserPrivileges() {
return BooleanEnumType.fromValue(grantPoweruserPrivileges);
}
/**
*
* Boolean value to determine whether the user is granted Poweruser privileges.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #grantPoweruserPrivileges} will return {@link BooleanEnumType#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #grantPoweruserPrivilegesAsString}.
*
*
* @return Boolean value to determine whether the user is granted Poweruser privileges.
* @see BooleanEnumType
*/
public String grantPoweruserPrivilegesAsString() {
return grantPoweruserPrivileges;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(authenticationToken());
hashCode = 31 * hashCode + Objects.hashCode(userId());
hashCode = 31 * hashCode + Objects.hashCode(givenName());
hashCode = 31 * hashCode + Objects.hashCode(surname());
hashCode = 31 * hashCode + Objects.hashCode(typeAsString());
hashCode = 31 * hashCode + Objects.hashCode(storageRule());
hashCode = 31 * hashCode + Objects.hashCode(timeZoneId());
hashCode = 31 * hashCode + Objects.hashCode(localeAsString());
hashCode = 31 * hashCode + Objects.hashCode(grantPoweruserPrivilegesAsString());
return hashCode;
}
@Override
public boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof UpdateUserRequest)) {
return false;
}
UpdateUserRequest other = (UpdateUserRequest) obj;
return Objects.equals(authenticationToken(), other.authenticationToken()) && Objects.equals(userId(), other.userId())
&& Objects.equals(givenName(), other.givenName()) && Objects.equals(surname(), other.surname())
&& Objects.equals(typeAsString(), other.typeAsString()) && Objects.equals(storageRule(), other.storageRule())
&& Objects.equals(timeZoneId(), other.timeZoneId()) && Objects.equals(localeAsString(), other.localeAsString())
&& Objects.equals(grantPoweruserPrivilegesAsString(), other.grantPoweruserPrivilegesAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("UpdateUserRequest")
.add("AuthenticationToken", authenticationToken() == null ? null : "*** Sensitive Data Redacted ***")
.add("UserId", userId()).add("GivenName", givenName()).add("Surname", surname()).add("Type", typeAsString())
.add("StorageRule", storageRule()).add("TimeZoneId", timeZoneId()).add("Locale", localeAsString())
.add("GrantPoweruserPrivileges", grantPoweruserPrivilegesAsString()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "AuthenticationToken":
return Optional.ofNullable(clazz.cast(authenticationToken()));
case "UserId":
return Optional.ofNullable(clazz.cast(userId()));
case "GivenName":
return Optional.ofNullable(clazz.cast(givenName()));
case "Surname":
return Optional.ofNullable(clazz.cast(surname()));
case "Type":
return Optional.ofNullable(clazz.cast(typeAsString()));
case "StorageRule":
return Optional.ofNullable(clazz.cast(storageRule()));
case "TimeZoneId":
return Optional.ofNullable(clazz.cast(timeZoneId()));
case "Locale":
return Optional.ofNullable(clazz.cast(localeAsString()));
case "GrantPoweruserPrivileges":
return Optional.ofNullable(clazz.cast(grantPoweruserPrivilegesAsString()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function