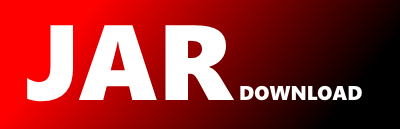
software.amazon.awssdk.services.workdocs.model.DocumentMetadata Maven / Gradle / Ivy
Show all versions of workdocs Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.workdocs.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Describes the document.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class DocumentMetadata implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField ID_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Id")
.getter(getter(DocumentMetadata::id)).setter(setter(Builder::id))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Id").build()).build();
private static final SdkField CREATOR_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("CreatorId").getter(getter(DocumentMetadata::creatorId)).setter(setter(Builder::creatorId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CreatorId").build()).build();
private static final SdkField PARENT_FOLDER_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ParentFolderId").getter(getter(DocumentMetadata::parentFolderId))
.setter(setter(Builder::parentFolderId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ParentFolderId").build()).build();
private static final SdkField CREATED_TIMESTAMP_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("CreatedTimestamp").getter(getter(DocumentMetadata::createdTimestamp))
.setter(setter(Builder::createdTimestamp))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CreatedTimestamp").build()).build();
private static final SdkField MODIFIED_TIMESTAMP_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("ModifiedTimestamp").getter(getter(DocumentMetadata::modifiedTimestamp))
.setter(setter(Builder::modifiedTimestamp))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ModifiedTimestamp").build()).build();
private static final SdkField LATEST_VERSION_METADATA_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("LatestVersionMetadata")
.getter(getter(DocumentMetadata::latestVersionMetadata)).setter(setter(Builder::latestVersionMetadata))
.constructor(DocumentVersionMetadata::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LatestVersionMetadata").build())
.build();
private static final SdkField RESOURCE_STATE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ResourceState").getter(getter(DocumentMetadata::resourceStateAsString))
.setter(setter(Builder::resourceState))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ResourceState").build()).build();
private static final SdkField> LABELS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Labels")
.getter(getter(DocumentMetadata::labels))
.setter(setter(Builder::labels))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Labels").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ID_FIELD, CREATOR_ID_FIELD,
PARENT_FOLDER_ID_FIELD, CREATED_TIMESTAMP_FIELD, MODIFIED_TIMESTAMP_FIELD, LATEST_VERSION_METADATA_FIELD,
RESOURCE_STATE_FIELD, LABELS_FIELD));
private static final long serialVersionUID = 1L;
private final String id;
private final String creatorId;
private final String parentFolderId;
private final Instant createdTimestamp;
private final Instant modifiedTimestamp;
private final DocumentVersionMetadata latestVersionMetadata;
private final String resourceState;
private final List labels;
private DocumentMetadata(BuilderImpl builder) {
this.id = builder.id;
this.creatorId = builder.creatorId;
this.parentFolderId = builder.parentFolderId;
this.createdTimestamp = builder.createdTimestamp;
this.modifiedTimestamp = builder.modifiedTimestamp;
this.latestVersionMetadata = builder.latestVersionMetadata;
this.resourceState = builder.resourceState;
this.labels = builder.labels;
}
/**
*
* The ID of the document.
*
*
* @return The ID of the document.
*/
public final String id() {
return id;
}
/**
*
* The ID of the creator.
*
*
* @return The ID of the creator.
*/
public final String creatorId() {
return creatorId;
}
/**
*
* The ID of the parent folder.
*
*
* @return The ID of the parent folder.
*/
public final String parentFolderId() {
return parentFolderId;
}
/**
*
* The time when the document was created.
*
*
* @return The time when the document was created.
*/
public final Instant createdTimestamp() {
return createdTimestamp;
}
/**
*
* The time when the document was updated.
*
*
* @return The time when the document was updated.
*/
public final Instant modifiedTimestamp() {
return modifiedTimestamp;
}
/**
*
* The latest version of the document.
*
*
* @return The latest version of the document.
*/
public final DocumentVersionMetadata latestVersionMetadata() {
return latestVersionMetadata;
}
/**
*
* The resource state.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #resourceState}
* will return {@link ResourceStateType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #resourceStateAsString}.
*
*
* @return The resource state.
* @see ResourceStateType
*/
public final ResourceStateType resourceState() {
return ResourceStateType.fromValue(resourceState);
}
/**
*
* The resource state.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #resourceState}
* will return {@link ResourceStateType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #resourceStateAsString}.
*
*
* @return The resource state.
* @see ResourceStateType
*/
public final String resourceStateAsString() {
return resourceState;
}
/**
* Returns true if the Labels property was specified by the sender (it may be empty), or false if the sender did not
* specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS service.
*/
public final boolean hasLabels() {
return labels != null && !(labels instanceof SdkAutoConstructList);
}
/**
*
* List of labels on the document.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasLabels()} to see if a value was sent in this field.
*
*
* @return List of labels on the document.
*/
public final List labels() {
return labels;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(id());
hashCode = 31 * hashCode + Objects.hashCode(creatorId());
hashCode = 31 * hashCode + Objects.hashCode(parentFolderId());
hashCode = 31 * hashCode + Objects.hashCode(createdTimestamp());
hashCode = 31 * hashCode + Objects.hashCode(modifiedTimestamp());
hashCode = 31 * hashCode + Objects.hashCode(latestVersionMetadata());
hashCode = 31 * hashCode + Objects.hashCode(resourceStateAsString());
hashCode = 31 * hashCode + Objects.hashCode(hasLabels() ? labels() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof DocumentMetadata)) {
return false;
}
DocumentMetadata other = (DocumentMetadata) obj;
return Objects.equals(id(), other.id()) && Objects.equals(creatorId(), other.creatorId())
&& Objects.equals(parentFolderId(), other.parentFolderId())
&& Objects.equals(createdTimestamp(), other.createdTimestamp())
&& Objects.equals(modifiedTimestamp(), other.modifiedTimestamp())
&& Objects.equals(latestVersionMetadata(), other.latestVersionMetadata())
&& Objects.equals(resourceStateAsString(), other.resourceStateAsString()) && hasLabels() == other.hasLabels()
&& Objects.equals(labels(), other.labels());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("DocumentMetadata").add("Id", id()).add("CreatorId", creatorId())
.add("ParentFolderId", parentFolderId()).add("CreatedTimestamp", createdTimestamp())
.add("ModifiedTimestamp", modifiedTimestamp()).add("LatestVersionMetadata", latestVersionMetadata())
.add("ResourceState", resourceStateAsString()).add("Labels", hasLabels() ? labels() : null).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Id":
return Optional.ofNullable(clazz.cast(id()));
case "CreatorId":
return Optional.ofNullable(clazz.cast(creatorId()));
case "ParentFolderId":
return Optional.ofNullable(clazz.cast(parentFolderId()));
case "CreatedTimestamp":
return Optional.ofNullable(clazz.cast(createdTimestamp()));
case "ModifiedTimestamp":
return Optional.ofNullable(clazz.cast(modifiedTimestamp()));
case "LatestVersionMetadata":
return Optional.ofNullable(clazz.cast(latestVersionMetadata()));
case "ResourceState":
return Optional.ofNullable(clazz.cast(resourceStateAsString()));
case "Labels":
return Optional.ofNullable(clazz.cast(labels()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function