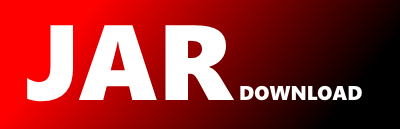
software.amazon.awssdk.services.workdocs.WorkDocsClient Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.workdocs;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkPublicApi;
import software.amazon.awssdk.annotations.ThreadSafe;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.core.SdkClient;
import software.amazon.awssdk.core.exception.SdkClientException;
import software.amazon.awssdk.regions.ServiceMetadata;
import software.amazon.awssdk.services.workdocs.model.AbortDocumentVersionUploadRequest;
import software.amazon.awssdk.services.workdocs.model.AbortDocumentVersionUploadResponse;
import software.amazon.awssdk.services.workdocs.model.ActivateUserRequest;
import software.amazon.awssdk.services.workdocs.model.ActivateUserResponse;
import software.amazon.awssdk.services.workdocs.model.AddResourcePermissionsRequest;
import software.amazon.awssdk.services.workdocs.model.AddResourcePermissionsResponse;
import software.amazon.awssdk.services.workdocs.model.ConcurrentModificationException;
import software.amazon.awssdk.services.workdocs.model.ConflictingOperationException;
import software.amazon.awssdk.services.workdocs.model.CreateCommentRequest;
import software.amazon.awssdk.services.workdocs.model.CreateCommentResponse;
import software.amazon.awssdk.services.workdocs.model.CreateCustomMetadataRequest;
import software.amazon.awssdk.services.workdocs.model.CreateCustomMetadataResponse;
import software.amazon.awssdk.services.workdocs.model.CreateFolderRequest;
import software.amazon.awssdk.services.workdocs.model.CreateFolderResponse;
import software.amazon.awssdk.services.workdocs.model.CreateLabelsRequest;
import software.amazon.awssdk.services.workdocs.model.CreateLabelsResponse;
import software.amazon.awssdk.services.workdocs.model.CreateNotificationSubscriptionRequest;
import software.amazon.awssdk.services.workdocs.model.CreateNotificationSubscriptionResponse;
import software.amazon.awssdk.services.workdocs.model.CreateUserRequest;
import software.amazon.awssdk.services.workdocs.model.CreateUserResponse;
import software.amazon.awssdk.services.workdocs.model.CustomMetadataLimitExceededException;
import software.amazon.awssdk.services.workdocs.model.DeactivateUserRequest;
import software.amazon.awssdk.services.workdocs.model.DeactivateUserResponse;
import software.amazon.awssdk.services.workdocs.model.DeactivatingLastSystemUserException;
import software.amazon.awssdk.services.workdocs.model.DeleteCommentRequest;
import software.amazon.awssdk.services.workdocs.model.DeleteCommentResponse;
import software.amazon.awssdk.services.workdocs.model.DeleteCustomMetadataRequest;
import software.amazon.awssdk.services.workdocs.model.DeleteCustomMetadataResponse;
import software.amazon.awssdk.services.workdocs.model.DeleteDocumentRequest;
import software.amazon.awssdk.services.workdocs.model.DeleteDocumentResponse;
import software.amazon.awssdk.services.workdocs.model.DeleteFolderContentsRequest;
import software.amazon.awssdk.services.workdocs.model.DeleteFolderContentsResponse;
import software.amazon.awssdk.services.workdocs.model.DeleteFolderRequest;
import software.amazon.awssdk.services.workdocs.model.DeleteFolderResponse;
import software.amazon.awssdk.services.workdocs.model.DeleteLabelsRequest;
import software.amazon.awssdk.services.workdocs.model.DeleteLabelsResponse;
import software.amazon.awssdk.services.workdocs.model.DeleteNotificationSubscriptionRequest;
import software.amazon.awssdk.services.workdocs.model.DeleteNotificationSubscriptionResponse;
import software.amazon.awssdk.services.workdocs.model.DeleteUserRequest;
import software.amazon.awssdk.services.workdocs.model.DeleteUserResponse;
import software.amazon.awssdk.services.workdocs.model.DescribeActivitiesRequest;
import software.amazon.awssdk.services.workdocs.model.DescribeActivitiesResponse;
import software.amazon.awssdk.services.workdocs.model.DescribeCommentsRequest;
import software.amazon.awssdk.services.workdocs.model.DescribeCommentsResponse;
import software.amazon.awssdk.services.workdocs.model.DescribeDocumentVersionsRequest;
import software.amazon.awssdk.services.workdocs.model.DescribeDocumentVersionsResponse;
import software.amazon.awssdk.services.workdocs.model.DescribeFolderContentsRequest;
import software.amazon.awssdk.services.workdocs.model.DescribeFolderContentsResponse;
import software.amazon.awssdk.services.workdocs.model.DescribeGroupsRequest;
import software.amazon.awssdk.services.workdocs.model.DescribeGroupsResponse;
import software.amazon.awssdk.services.workdocs.model.DescribeNotificationSubscriptionsRequest;
import software.amazon.awssdk.services.workdocs.model.DescribeNotificationSubscriptionsResponse;
import software.amazon.awssdk.services.workdocs.model.DescribeResourcePermissionsRequest;
import software.amazon.awssdk.services.workdocs.model.DescribeResourcePermissionsResponse;
import software.amazon.awssdk.services.workdocs.model.DescribeRootFoldersRequest;
import software.amazon.awssdk.services.workdocs.model.DescribeRootFoldersResponse;
import software.amazon.awssdk.services.workdocs.model.DescribeUsersRequest;
import software.amazon.awssdk.services.workdocs.model.DescribeUsersResponse;
import software.amazon.awssdk.services.workdocs.model.DocumentLockedForCommentsException;
import software.amazon.awssdk.services.workdocs.model.DraftUploadOutOfSyncException;
import software.amazon.awssdk.services.workdocs.model.EntityAlreadyExistsException;
import software.amazon.awssdk.services.workdocs.model.EntityNotExistsException;
import software.amazon.awssdk.services.workdocs.model.FailedDependencyException;
import software.amazon.awssdk.services.workdocs.model.GetCurrentUserRequest;
import software.amazon.awssdk.services.workdocs.model.GetCurrentUserResponse;
import software.amazon.awssdk.services.workdocs.model.GetDocumentPathRequest;
import software.amazon.awssdk.services.workdocs.model.GetDocumentPathResponse;
import software.amazon.awssdk.services.workdocs.model.GetDocumentRequest;
import software.amazon.awssdk.services.workdocs.model.GetDocumentResponse;
import software.amazon.awssdk.services.workdocs.model.GetDocumentVersionRequest;
import software.amazon.awssdk.services.workdocs.model.GetDocumentVersionResponse;
import software.amazon.awssdk.services.workdocs.model.GetFolderPathRequest;
import software.amazon.awssdk.services.workdocs.model.GetFolderPathResponse;
import software.amazon.awssdk.services.workdocs.model.GetFolderRequest;
import software.amazon.awssdk.services.workdocs.model.GetFolderResponse;
import software.amazon.awssdk.services.workdocs.model.GetResourcesRequest;
import software.amazon.awssdk.services.workdocs.model.GetResourcesResponse;
import software.amazon.awssdk.services.workdocs.model.IllegalUserStateException;
import software.amazon.awssdk.services.workdocs.model.InitiateDocumentVersionUploadRequest;
import software.amazon.awssdk.services.workdocs.model.InitiateDocumentVersionUploadResponse;
import software.amazon.awssdk.services.workdocs.model.InvalidArgumentException;
import software.amazon.awssdk.services.workdocs.model.InvalidCommentOperationException;
import software.amazon.awssdk.services.workdocs.model.InvalidOperationException;
import software.amazon.awssdk.services.workdocs.model.InvalidPasswordException;
import software.amazon.awssdk.services.workdocs.model.LimitExceededException;
import software.amazon.awssdk.services.workdocs.model.ProhibitedStateException;
import software.amazon.awssdk.services.workdocs.model.RemoveAllResourcePermissionsRequest;
import software.amazon.awssdk.services.workdocs.model.RemoveAllResourcePermissionsResponse;
import software.amazon.awssdk.services.workdocs.model.RemoveResourcePermissionRequest;
import software.amazon.awssdk.services.workdocs.model.RemoveResourcePermissionResponse;
import software.amazon.awssdk.services.workdocs.model.RequestedEntityTooLargeException;
import software.amazon.awssdk.services.workdocs.model.ResourceAlreadyCheckedOutException;
import software.amazon.awssdk.services.workdocs.model.ServiceUnavailableException;
import software.amazon.awssdk.services.workdocs.model.StorageLimitExceededException;
import software.amazon.awssdk.services.workdocs.model.StorageLimitWillExceedException;
import software.amazon.awssdk.services.workdocs.model.TooManyLabelsException;
import software.amazon.awssdk.services.workdocs.model.TooManySubscriptionsException;
import software.amazon.awssdk.services.workdocs.model.UnauthorizedOperationException;
import software.amazon.awssdk.services.workdocs.model.UnauthorizedResourceAccessException;
import software.amazon.awssdk.services.workdocs.model.UpdateDocumentRequest;
import software.amazon.awssdk.services.workdocs.model.UpdateDocumentResponse;
import software.amazon.awssdk.services.workdocs.model.UpdateDocumentVersionRequest;
import software.amazon.awssdk.services.workdocs.model.UpdateDocumentVersionResponse;
import software.amazon.awssdk.services.workdocs.model.UpdateFolderRequest;
import software.amazon.awssdk.services.workdocs.model.UpdateFolderResponse;
import software.amazon.awssdk.services.workdocs.model.UpdateUserRequest;
import software.amazon.awssdk.services.workdocs.model.UpdateUserResponse;
import software.amazon.awssdk.services.workdocs.model.WorkDocsException;
import software.amazon.awssdk.services.workdocs.paginators.DescribeDocumentVersionsIterable;
import software.amazon.awssdk.services.workdocs.paginators.DescribeFolderContentsIterable;
import software.amazon.awssdk.services.workdocs.paginators.DescribeUsersIterable;
/**
* Service client for accessing Amazon WorkDocs. This can be created using the static {@link #builder()} method.
*
*
* The WorkDocs API is designed for the following use cases:
*
*
* -
*
* File Migration: File migration applications are supported for users who want to migrate their files from an
* on-premises or off-premises file system or service. Users can insert files into a user directory structure, as well
* as allow for basic metadata changes, such as modifications to the permissions of files.
*
*
* -
*
* Security: Support security applications are supported for users who have additional security needs, such as antivirus
* or data loss prevention. The API actions, along with AWS CloudTrail, allow these applications to detect when changes
* occur in Amazon WorkDocs. Then, the application can take the necessary actions and replace the target file. If the
* target file violates the policy, the application can also choose to email the user.
*
*
* -
*
* eDiscovery/Analytics: General administrative applications are supported, such as eDiscovery and analytics. These
* applications can choose to mimic or record the actions in an Amazon WorkDocs site, along with AWS CloudTrail, to
* replicate data for eDiscovery, backup, or analytical applications.
*
*
*
*
* All Amazon WorkDocs API actions are Amazon authenticated and certificate-signed. They not only require the use of the
* AWS SDK, but also allow for the exclusive use of IAM users and roles to help facilitate access, trust, and permission
* policies. By creating a role and allowing an IAM user to access the Amazon WorkDocs site, the IAM user gains full
* administrative visibility into the entire Amazon WorkDocs site (or as set in the IAM policy). This includes, but is
* not limited to, the ability to modify file permissions and upload any file to any user. This allows developers to
* perform the three use cases above, as well as give users the ability to grant access on a selective basis using the
* IAM model.
*
*/
@Generated("software.amazon.awssdk:codegen")
@SdkPublicApi
@ThreadSafe
public interface WorkDocsClient extends SdkClient {
String SERVICE_NAME = "workdocs";
/**
* Value for looking up the service's metadata from the
* {@link software.amazon.awssdk.regions.ServiceMetadataProvider}.
*/
String SERVICE_METADATA_ID = "workdocs";
/**
* Create a {@link WorkDocsClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static WorkDocsClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link WorkDocsClient}.
*/
static WorkDocsClientBuilder builder() {
return new DefaultWorkDocsClientBuilder();
}
/**
*
* Aborts the upload of the specified document version that was previously initiated by
* InitiateDocumentVersionUpload. The client should make this call only when it no longer intends to upload
* the document version, or fails to do so.
*
*
* @param abortDocumentVersionUploadRequest
* @return Result of the AbortDocumentVersionUpload operation returned by the service.
* @throws EntityNotExistsException
* The resource does not exist.
* @throws ProhibitedStateException
* The specified document version is not in the INITIALIZED state.
* @throws UnauthorizedOperationException
* The operation is not permitted.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.AbortDocumentVersionUpload
* @see AWS API Documentation
*/
default AbortDocumentVersionUploadResponse abortDocumentVersionUpload(
AbortDocumentVersionUploadRequest abortDocumentVersionUploadRequest) throws EntityNotExistsException,
ProhibitedStateException, UnauthorizedOperationException, UnauthorizedResourceAccessException,
FailedDependencyException, ServiceUnavailableException, AwsServiceException, SdkClientException, WorkDocsException {
throw new UnsupportedOperationException();
}
/**
*
* Aborts the upload of the specified document version that was previously initiated by
* InitiateDocumentVersionUpload. The client should make this call only when it no longer intends to upload
* the document version, or fails to do so.
*
*
*
* This is a convenience which creates an instance of the {@link AbortDocumentVersionUploadRequest.Builder} avoiding
* the need to create one manually via {@link AbortDocumentVersionUploadRequest#builder()}
*
*
* @param abortDocumentVersionUploadRequest
* A {@link Consumer} that will call methods on {@link AbortDocumentVersionUploadRequest.Builder} to create a
* request.
* @return Result of the AbortDocumentVersionUpload operation returned by the service.
* @throws EntityNotExistsException
* The resource does not exist.
* @throws ProhibitedStateException
* The specified document version is not in the INITIALIZED state.
* @throws UnauthorizedOperationException
* The operation is not permitted.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.AbortDocumentVersionUpload
* @see AWS API Documentation
*/
default AbortDocumentVersionUploadResponse abortDocumentVersionUpload(
Consumer abortDocumentVersionUploadRequest)
throws EntityNotExistsException, ProhibitedStateException, UnauthorizedOperationException,
UnauthorizedResourceAccessException, FailedDependencyException, ServiceUnavailableException, AwsServiceException,
SdkClientException, WorkDocsException {
return abortDocumentVersionUpload(AbortDocumentVersionUploadRequest.builder()
.applyMutation(abortDocumentVersionUploadRequest).build());
}
/**
*
* Activates the specified user. Only active users can access Amazon WorkDocs.
*
*
* @param activateUserRequest
* @return Result of the ActivateUser operation returned by the service.
* @throws EntityNotExistsException
* The resource does not exist.
* @throws UnauthorizedOperationException
* The operation is not permitted.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.ActivateUser
* @see AWS API
* Documentation
*/
default ActivateUserResponse activateUser(ActivateUserRequest activateUserRequest) throws EntityNotExistsException,
UnauthorizedOperationException, UnauthorizedResourceAccessException, FailedDependencyException,
ServiceUnavailableException, AwsServiceException, SdkClientException, WorkDocsException {
throw new UnsupportedOperationException();
}
/**
*
* Activates the specified user. Only active users can access Amazon WorkDocs.
*
*
*
* This is a convenience which creates an instance of the {@link ActivateUserRequest.Builder} avoiding the need to
* create one manually via {@link ActivateUserRequest#builder()}
*
*
* @param activateUserRequest
* A {@link Consumer} that will call methods on {@link ActivateUserRequest.Builder} to create a request.
* @return Result of the ActivateUser operation returned by the service.
* @throws EntityNotExistsException
* The resource does not exist.
* @throws UnauthorizedOperationException
* The operation is not permitted.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.ActivateUser
* @see AWS API
* Documentation
*/
default ActivateUserResponse activateUser(Consumer activateUserRequest)
throws EntityNotExistsException, UnauthorizedOperationException, UnauthorizedResourceAccessException,
FailedDependencyException, ServiceUnavailableException, AwsServiceException, SdkClientException, WorkDocsException {
return activateUser(ActivateUserRequest.builder().applyMutation(activateUserRequest).build());
}
/**
*
* Creates a set of permissions for the specified folder or document. The resource permissions are overwritten if
* the principals already have different permissions.
*
*
* @param addResourcePermissionsRequest
* @return Result of the AddResourcePermissions operation returned by the service.
* @throws UnauthorizedOperationException
* The operation is not permitted.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.AddResourcePermissions
* @see AWS API Documentation
*/
default AddResourcePermissionsResponse addResourcePermissions(AddResourcePermissionsRequest addResourcePermissionsRequest)
throws UnauthorizedOperationException, UnauthorizedResourceAccessException, FailedDependencyException,
ServiceUnavailableException, AwsServiceException, SdkClientException, WorkDocsException {
throw new UnsupportedOperationException();
}
/**
*
* Creates a set of permissions for the specified folder or document. The resource permissions are overwritten if
* the principals already have different permissions.
*
*
*
* This is a convenience which creates an instance of the {@link AddResourcePermissionsRequest.Builder} avoiding the
* need to create one manually via {@link AddResourcePermissionsRequest#builder()}
*
*
* @param addResourcePermissionsRequest
* A {@link Consumer} that will call methods on {@link AddResourcePermissionsRequest.Builder} to create a
* request.
* @return Result of the AddResourcePermissions operation returned by the service.
* @throws UnauthorizedOperationException
* The operation is not permitted.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.AddResourcePermissions
* @see AWS API Documentation
*/
default AddResourcePermissionsResponse addResourcePermissions(
Consumer addResourcePermissionsRequest) throws UnauthorizedOperationException,
UnauthorizedResourceAccessException, FailedDependencyException, ServiceUnavailableException, AwsServiceException,
SdkClientException, WorkDocsException {
return addResourcePermissions(AddResourcePermissionsRequest.builder().applyMutation(addResourcePermissionsRequest)
.build());
}
/**
*
* Adds a new comment to the specified document version.
*
*
* @param createCommentRequest
* @return Result of the CreateComment operation returned by the service.
* @throws EntityNotExistsException
* The resource does not exist.
* @throws ProhibitedStateException
* The specified document version is not in the INITIALIZED state.
* @throws UnauthorizedOperationException
* The operation is not permitted.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws DocumentLockedForCommentsException
* This exception is thrown when the document is locked for comments and user tries to create or delete a
* comment on that document.
* @throws InvalidCommentOperationException
* The requested operation is not allowed on the specified comment object.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.CreateComment
* @see AWS API
* Documentation
*/
default CreateCommentResponse createComment(CreateCommentRequest createCommentRequest) throws EntityNotExistsException,
ProhibitedStateException, UnauthorizedOperationException, UnauthorizedResourceAccessException,
FailedDependencyException, ServiceUnavailableException, DocumentLockedForCommentsException,
InvalidCommentOperationException, AwsServiceException, SdkClientException, WorkDocsException {
throw new UnsupportedOperationException();
}
/**
*
* Adds a new comment to the specified document version.
*
*
*
* This is a convenience which creates an instance of the {@link CreateCommentRequest.Builder} avoiding the need to
* create one manually via {@link CreateCommentRequest#builder()}
*
*
* @param createCommentRequest
* A {@link Consumer} that will call methods on {@link CreateCommentRequest.Builder} to create a request.
* @return Result of the CreateComment operation returned by the service.
* @throws EntityNotExistsException
* The resource does not exist.
* @throws ProhibitedStateException
* The specified document version is not in the INITIALIZED state.
* @throws UnauthorizedOperationException
* The operation is not permitted.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws DocumentLockedForCommentsException
* This exception is thrown when the document is locked for comments and user tries to create or delete a
* comment on that document.
* @throws InvalidCommentOperationException
* The requested operation is not allowed on the specified comment object.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.CreateComment
* @see AWS API
* Documentation
*/
default CreateCommentResponse createComment(Consumer createCommentRequest)
throws EntityNotExistsException, ProhibitedStateException, UnauthorizedOperationException,
UnauthorizedResourceAccessException, FailedDependencyException, ServiceUnavailableException,
DocumentLockedForCommentsException, InvalidCommentOperationException, AwsServiceException, SdkClientException,
WorkDocsException {
return createComment(CreateCommentRequest.builder().applyMutation(createCommentRequest).build());
}
/**
*
* Adds one or more custom properties to the specified resource (a folder, document, or version).
*
*
* @param createCustomMetadataRequest
* @return Result of the CreateCustomMetadata operation returned by the service.
* @throws EntityNotExistsException
* The resource does not exist.
* @throws UnauthorizedOperationException
* The operation is not permitted.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws ProhibitedStateException
* The specified document version is not in the INITIALIZED state.
* @throws CustomMetadataLimitExceededException
* The limit has been reached on the number of custom properties for the specified resource.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.CreateCustomMetadata
* @see AWS
* API Documentation
*/
default CreateCustomMetadataResponse createCustomMetadata(CreateCustomMetadataRequest createCustomMetadataRequest)
throws EntityNotExistsException, UnauthorizedOperationException, UnauthorizedResourceAccessException,
ProhibitedStateException, CustomMetadataLimitExceededException, FailedDependencyException,
ServiceUnavailableException, AwsServiceException, SdkClientException, WorkDocsException {
throw new UnsupportedOperationException();
}
/**
*
* Adds one or more custom properties to the specified resource (a folder, document, or version).
*
*
*
* This is a convenience which creates an instance of the {@link CreateCustomMetadataRequest.Builder} avoiding the
* need to create one manually via {@link CreateCustomMetadataRequest#builder()}
*
*
* @param createCustomMetadataRequest
* A {@link Consumer} that will call methods on {@link CreateCustomMetadataRequest.Builder} to create a
* request.
* @return Result of the CreateCustomMetadata operation returned by the service.
* @throws EntityNotExistsException
* The resource does not exist.
* @throws UnauthorizedOperationException
* The operation is not permitted.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws ProhibitedStateException
* The specified document version is not in the INITIALIZED state.
* @throws CustomMetadataLimitExceededException
* The limit has been reached on the number of custom properties for the specified resource.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.CreateCustomMetadata
* @see AWS
* API Documentation
*/
default CreateCustomMetadataResponse createCustomMetadata(
Consumer createCustomMetadataRequest) throws EntityNotExistsException,
UnauthorizedOperationException, UnauthorizedResourceAccessException, ProhibitedStateException,
CustomMetadataLimitExceededException, FailedDependencyException, ServiceUnavailableException, AwsServiceException,
SdkClientException, WorkDocsException {
return createCustomMetadata(CreateCustomMetadataRequest.builder().applyMutation(createCustomMetadataRequest).build());
}
/**
*
* Creates a folder with the specified name and parent folder.
*
*
* @param createFolderRequest
* @return Result of the CreateFolder operation returned by the service.
* @throws EntityNotExistsException
* The resource does not exist.
* @throws EntityAlreadyExistsException
* The resource already exists.
* @throws ProhibitedStateException
* The specified document version is not in the INITIALIZED state.
* @throws ConflictingOperationException
* Another operation is in progress on the resource that conflicts with the current operation.
* @throws LimitExceededException
* The maximum of 100,000 folders under the parent folder has been exceeded.
* @throws UnauthorizedOperationException
* The operation is not permitted.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.CreateFolder
* @see AWS API
* Documentation
*/
default CreateFolderResponse createFolder(CreateFolderRequest createFolderRequest) throws EntityNotExistsException,
EntityAlreadyExistsException, ProhibitedStateException, ConflictingOperationException, LimitExceededException,
UnauthorizedOperationException, UnauthorizedResourceAccessException, FailedDependencyException,
ServiceUnavailableException, AwsServiceException, SdkClientException, WorkDocsException {
throw new UnsupportedOperationException();
}
/**
*
* Creates a folder with the specified name and parent folder.
*
*
*
* This is a convenience which creates an instance of the {@link CreateFolderRequest.Builder} avoiding the need to
* create one manually via {@link CreateFolderRequest#builder()}
*
*
* @param createFolderRequest
* A {@link Consumer} that will call methods on {@link CreateFolderRequest.Builder} to create a request.
* @return Result of the CreateFolder operation returned by the service.
* @throws EntityNotExistsException
* The resource does not exist.
* @throws EntityAlreadyExistsException
* The resource already exists.
* @throws ProhibitedStateException
* The specified document version is not in the INITIALIZED state.
* @throws ConflictingOperationException
* Another operation is in progress on the resource that conflicts with the current operation.
* @throws LimitExceededException
* The maximum of 100,000 folders under the parent folder has been exceeded.
* @throws UnauthorizedOperationException
* The operation is not permitted.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.CreateFolder
* @see AWS API
* Documentation
*/
default CreateFolderResponse createFolder(Consumer createFolderRequest)
throws EntityNotExistsException, EntityAlreadyExistsException, ProhibitedStateException,
ConflictingOperationException, LimitExceededException, UnauthorizedOperationException,
UnauthorizedResourceAccessException, FailedDependencyException, ServiceUnavailableException, AwsServiceException,
SdkClientException, WorkDocsException {
return createFolder(CreateFolderRequest.builder().applyMutation(createFolderRequest).build());
}
/**
*
* Adds the specified list of labels to the given resource (a document or folder)
*
*
* @param createLabelsRequest
* @return Result of the CreateLabels operation returned by the service.
* @throws EntityNotExistsException
* The resource does not exist.
* @throws UnauthorizedOperationException
* The operation is not permitted.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws TooManyLabelsException
* The limit has been reached on the number of labels for the specified resource.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.CreateLabels
* @see AWS API
* Documentation
*/
default CreateLabelsResponse createLabels(CreateLabelsRequest createLabelsRequest) throws EntityNotExistsException,
UnauthorizedOperationException, UnauthorizedResourceAccessException, FailedDependencyException,
ServiceUnavailableException, TooManyLabelsException, AwsServiceException, SdkClientException, WorkDocsException {
throw new UnsupportedOperationException();
}
/**
*
* Adds the specified list of labels to the given resource (a document or folder)
*
*
*
* This is a convenience which creates an instance of the {@link CreateLabelsRequest.Builder} avoiding the need to
* create one manually via {@link CreateLabelsRequest#builder()}
*
*
* @param createLabelsRequest
* A {@link Consumer} that will call methods on {@link CreateLabelsRequest.Builder} to create a request.
* @return Result of the CreateLabels operation returned by the service.
* @throws EntityNotExistsException
* The resource does not exist.
* @throws UnauthorizedOperationException
* The operation is not permitted.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws TooManyLabelsException
* The limit has been reached on the number of labels for the specified resource.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.CreateLabels
* @see AWS API
* Documentation
*/
default CreateLabelsResponse createLabels(Consumer createLabelsRequest)
throws EntityNotExistsException, UnauthorizedOperationException, UnauthorizedResourceAccessException,
FailedDependencyException, ServiceUnavailableException, TooManyLabelsException, AwsServiceException,
SdkClientException, WorkDocsException {
return createLabels(CreateLabelsRequest.builder().applyMutation(createLabelsRequest).build());
}
/**
*
* Configure Amazon WorkDocs to use Amazon SNS notifications. The endpoint receives a confirmation message, and must
* confirm the subscription.
*
*
* For more information, see Subscribe to
* Notifications in the Amazon WorkDocs Developer Guide.
*
*
* @param createNotificationSubscriptionRequest
* @return Result of the CreateNotificationSubscription operation returned by the service.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws TooManySubscriptionsException
* You've reached the limit on the number of subscriptions for the WorkDocs instance.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.CreateNotificationSubscription
* @see AWS API Documentation
*/
default CreateNotificationSubscriptionResponse createNotificationSubscription(
CreateNotificationSubscriptionRequest createNotificationSubscriptionRequest)
throws UnauthorizedResourceAccessException, TooManySubscriptionsException, ServiceUnavailableException,
AwsServiceException, SdkClientException, WorkDocsException {
throw new UnsupportedOperationException();
}
/**
*
* Configure Amazon WorkDocs to use Amazon SNS notifications. The endpoint receives a confirmation message, and must
* confirm the subscription.
*
*
* For more information, see Subscribe to
* Notifications in the Amazon WorkDocs Developer Guide.
*
*
*
* This is a convenience which creates an instance of the {@link CreateNotificationSubscriptionRequest.Builder}
* avoiding the need to create one manually via {@link CreateNotificationSubscriptionRequest#builder()}
*
*
* @param createNotificationSubscriptionRequest
* A {@link Consumer} that will call methods on {@link CreateNotificationSubscriptionRequest.Builder} to
* create a request.
* @return Result of the CreateNotificationSubscription operation returned by the service.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws TooManySubscriptionsException
* You've reached the limit on the number of subscriptions for the WorkDocs instance.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.CreateNotificationSubscription
* @see AWS API Documentation
*/
default CreateNotificationSubscriptionResponse createNotificationSubscription(
Consumer createNotificationSubscriptionRequest)
throws UnauthorizedResourceAccessException, TooManySubscriptionsException, ServiceUnavailableException,
AwsServiceException, SdkClientException, WorkDocsException {
return createNotificationSubscription(CreateNotificationSubscriptionRequest.builder()
.applyMutation(createNotificationSubscriptionRequest).build());
}
/**
*
* Creates a user in a Simple AD or Microsoft AD directory. The status of a newly created user is "ACTIVE". New
* users can access Amazon WorkDocs.
*
*
* @param createUserRequest
* @return Result of the CreateUser operation returned by the service.
* @throws EntityAlreadyExistsException
* The resource already exists.
* @throws UnauthorizedOperationException
* The operation is not permitted.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.CreateUser
* @see AWS API
* Documentation
*/
default CreateUserResponse createUser(CreateUserRequest createUserRequest) throws EntityAlreadyExistsException,
UnauthorizedOperationException, UnauthorizedResourceAccessException, FailedDependencyException,
ServiceUnavailableException, AwsServiceException, SdkClientException, WorkDocsException {
throw new UnsupportedOperationException();
}
/**
*
* Creates a user in a Simple AD or Microsoft AD directory. The status of a newly created user is "ACTIVE". New
* users can access Amazon WorkDocs.
*
*
*
* This is a convenience which creates an instance of the {@link CreateUserRequest.Builder} avoiding the need to
* create one manually via {@link CreateUserRequest#builder()}
*
*
* @param createUserRequest
* A {@link Consumer} that will call methods on {@link CreateUserRequest.Builder} to create a request.
* @return Result of the CreateUser operation returned by the service.
* @throws EntityAlreadyExistsException
* The resource already exists.
* @throws UnauthorizedOperationException
* The operation is not permitted.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.CreateUser
* @see AWS API
* Documentation
*/
default CreateUserResponse createUser(Consumer createUserRequest)
throws EntityAlreadyExistsException, UnauthorizedOperationException, UnauthorizedResourceAccessException,
FailedDependencyException, ServiceUnavailableException, AwsServiceException, SdkClientException, WorkDocsException {
return createUser(CreateUserRequest.builder().applyMutation(createUserRequest).build());
}
/**
*
* Deactivates the specified user, which revokes the user's access to Amazon WorkDocs.
*
*
* @param deactivateUserRequest
* @return Result of the DeactivateUser operation returned by the service.
* @throws EntityNotExistsException
* The resource does not exist.
* @throws UnauthorizedOperationException
* The operation is not permitted.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.DeactivateUser
* @see AWS API
* Documentation
*/
default DeactivateUserResponse deactivateUser(DeactivateUserRequest deactivateUserRequest) throws EntityNotExistsException,
UnauthorizedOperationException, UnauthorizedResourceAccessException, FailedDependencyException,
ServiceUnavailableException, AwsServiceException, SdkClientException, WorkDocsException {
throw new UnsupportedOperationException();
}
/**
*
* Deactivates the specified user, which revokes the user's access to Amazon WorkDocs.
*
*
*
* This is a convenience which creates an instance of the {@link DeactivateUserRequest.Builder} avoiding the need to
* create one manually via {@link DeactivateUserRequest#builder()}
*
*
* @param deactivateUserRequest
* A {@link Consumer} that will call methods on {@link DeactivateUserRequest.Builder} to create a request.
* @return Result of the DeactivateUser operation returned by the service.
* @throws EntityNotExistsException
* The resource does not exist.
* @throws UnauthorizedOperationException
* The operation is not permitted.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.DeactivateUser
* @see AWS API
* Documentation
*/
default DeactivateUserResponse deactivateUser(Consumer deactivateUserRequest)
throws EntityNotExistsException, UnauthorizedOperationException, UnauthorizedResourceAccessException,
FailedDependencyException, ServiceUnavailableException, AwsServiceException, SdkClientException, WorkDocsException {
return deactivateUser(DeactivateUserRequest.builder().applyMutation(deactivateUserRequest).build());
}
/**
*
* Deletes the specified comment from the document version.
*
*
* @param deleteCommentRequest
* @return Result of the DeleteComment operation returned by the service.
* @throws EntityNotExistsException
* The resource does not exist.
* @throws ProhibitedStateException
* The specified document version is not in the INITIALIZED state.
* @throws UnauthorizedOperationException
* The operation is not permitted.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws DocumentLockedForCommentsException
* This exception is thrown when the document is locked for comments and user tries to create or delete a
* comment on that document.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.DeleteComment
* @see AWS API
* Documentation
*/
default DeleteCommentResponse deleteComment(DeleteCommentRequest deleteCommentRequest) throws EntityNotExistsException,
ProhibitedStateException, UnauthorizedOperationException, UnauthorizedResourceAccessException,
FailedDependencyException, ServiceUnavailableException, DocumentLockedForCommentsException, AwsServiceException,
SdkClientException, WorkDocsException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the specified comment from the document version.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteCommentRequest.Builder} avoiding the need to
* create one manually via {@link DeleteCommentRequest#builder()}
*
*
* @param deleteCommentRequest
* A {@link Consumer} that will call methods on {@link DeleteCommentRequest.Builder} to create a request.
* @return Result of the DeleteComment operation returned by the service.
* @throws EntityNotExistsException
* The resource does not exist.
* @throws ProhibitedStateException
* The specified document version is not in the INITIALIZED state.
* @throws UnauthorizedOperationException
* The operation is not permitted.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws DocumentLockedForCommentsException
* This exception is thrown when the document is locked for comments and user tries to create or delete a
* comment on that document.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.DeleteComment
* @see AWS API
* Documentation
*/
default DeleteCommentResponse deleteComment(Consumer deleteCommentRequest)
throws EntityNotExistsException, ProhibitedStateException, UnauthorizedOperationException,
UnauthorizedResourceAccessException, FailedDependencyException, ServiceUnavailableException,
DocumentLockedForCommentsException, AwsServiceException, SdkClientException, WorkDocsException {
return deleteComment(DeleteCommentRequest.builder().applyMutation(deleteCommentRequest).build());
}
/**
*
* Deletes custom metadata from the specified resource.
*
*
* @param deleteCustomMetadataRequest
* @return Result of the DeleteCustomMetadata operation returned by the service.
* @throws EntityNotExistsException
* The resource does not exist.
* @throws UnauthorizedOperationException
* The operation is not permitted.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws ProhibitedStateException
* The specified document version is not in the INITIALIZED state.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.DeleteCustomMetadata
* @see AWS
* API Documentation
*/
default DeleteCustomMetadataResponse deleteCustomMetadata(DeleteCustomMetadataRequest deleteCustomMetadataRequest)
throws EntityNotExistsException, UnauthorizedOperationException, UnauthorizedResourceAccessException,
ProhibitedStateException, FailedDependencyException, ServiceUnavailableException, AwsServiceException,
SdkClientException, WorkDocsException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes custom metadata from the specified resource.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteCustomMetadataRequest.Builder} avoiding the
* need to create one manually via {@link DeleteCustomMetadataRequest#builder()}
*
*
* @param deleteCustomMetadataRequest
* A {@link Consumer} that will call methods on {@link DeleteCustomMetadataRequest.Builder} to create a
* request.
* @return Result of the DeleteCustomMetadata operation returned by the service.
* @throws EntityNotExistsException
* The resource does not exist.
* @throws UnauthorizedOperationException
* The operation is not permitted.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws ProhibitedStateException
* The specified document version is not in the INITIALIZED state.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.DeleteCustomMetadata
* @see AWS
* API Documentation
*/
default DeleteCustomMetadataResponse deleteCustomMetadata(
Consumer deleteCustomMetadataRequest) throws EntityNotExistsException,
UnauthorizedOperationException, UnauthorizedResourceAccessException, ProhibitedStateException,
FailedDependencyException, ServiceUnavailableException, AwsServiceException, SdkClientException, WorkDocsException {
return deleteCustomMetadata(DeleteCustomMetadataRequest.builder().applyMutation(deleteCustomMetadataRequest).build());
}
/**
*
* Permanently deletes the specified document and its associated metadata.
*
*
* @param deleteDocumentRequest
* @return Result of the DeleteDocument operation returned by the service.
* @throws EntityNotExistsException
* The resource does not exist.
* @throws ProhibitedStateException
* The specified document version is not in the INITIALIZED state.
* @throws ConflictingOperationException
* Another operation is in progress on the resource that conflicts with the current operation.
* @throws ConcurrentModificationException
* The resource hierarchy is changing.
* @throws UnauthorizedOperationException
* The operation is not permitted.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.DeleteDocument
* @see AWS API
* Documentation
*/
default DeleteDocumentResponse deleteDocument(DeleteDocumentRequest deleteDocumentRequest) throws EntityNotExistsException,
ProhibitedStateException, ConflictingOperationException, ConcurrentModificationException,
UnauthorizedOperationException, UnauthorizedResourceAccessException, FailedDependencyException,
ServiceUnavailableException, AwsServiceException, SdkClientException, WorkDocsException {
throw new UnsupportedOperationException();
}
/**
*
* Permanently deletes the specified document and its associated metadata.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteDocumentRequest.Builder} avoiding the need to
* create one manually via {@link DeleteDocumentRequest#builder()}
*
*
* @param deleteDocumentRequest
* A {@link Consumer} that will call methods on {@link DeleteDocumentRequest.Builder} to create a request.
* @return Result of the DeleteDocument operation returned by the service.
* @throws EntityNotExistsException
* The resource does not exist.
* @throws ProhibitedStateException
* The specified document version is not in the INITIALIZED state.
* @throws ConflictingOperationException
* Another operation is in progress on the resource that conflicts with the current operation.
* @throws ConcurrentModificationException
* The resource hierarchy is changing.
* @throws UnauthorizedOperationException
* The operation is not permitted.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.DeleteDocument
* @see AWS API
* Documentation
*/
default DeleteDocumentResponse deleteDocument(Consumer deleteDocumentRequest)
throws EntityNotExistsException, ProhibitedStateException, ConflictingOperationException,
ConcurrentModificationException, UnauthorizedOperationException, UnauthorizedResourceAccessException,
FailedDependencyException, ServiceUnavailableException, AwsServiceException, SdkClientException, WorkDocsException {
return deleteDocument(DeleteDocumentRequest.builder().applyMutation(deleteDocumentRequest).build());
}
/**
*
* Permanently deletes the specified folder and its contents.
*
*
* @param deleteFolderRequest
* @return Result of the DeleteFolder operation returned by the service.
* @throws EntityNotExistsException
* The resource does not exist.
* @throws ProhibitedStateException
* The specified document version is not in the INITIALIZED state.
* @throws ConflictingOperationException
* Another operation is in progress on the resource that conflicts with the current operation.
* @throws ConcurrentModificationException
* The resource hierarchy is changing.
* @throws UnauthorizedOperationException
* The operation is not permitted.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.DeleteFolder
* @see AWS API
* Documentation
*/
default DeleteFolderResponse deleteFolder(DeleteFolderRequest deleteFolderRequest) throws EntityNotExistsException,
ProhibitedStateException, ConflictingOperationException, ConcurrentModificationException,
UnauthorizedOperationException, UnauthorizedResourceAccessException, FailedDependencyException,
ServiceUnavailableException, AwsServiceException, SdkClientException, WorkDocsException {
throw new UnsupportedOperationException();
}
/**
*
* Permanently deletes the specified folder and its contents.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteFolderRequest.Builder} avoiding the need to
* create one manually via {@link DeleteFolderRequest#builder()}
*
*
* @param deleteFolderRequest
* A {@link Consumer} that will call methods on {@link DeleteFolderRequest.Builder} to create a request.
* @return Result of the DeleteFolder operation returned by the service.
* @throws EntityNotExistsException
* The resource does not exist.
* @throws ProhibitedStateException
* The specified document version is not in the INITIALIZED state.
* @throws ConflictingOperationException
* Another operation is in progress on the resource that conflicts with the current operation.
* @throws ConcurrentModificationException
* The resource hierarchy is changing.
* @throws UnauthorizedOperationException
* The operation is not permitted.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.DeleteFolder
* @see AWS API
* Documentation
*/
default DeleteFolderResponse deleteFolder(Consumer deleteFolderRequest)
throws EntityNotExistsException, ProhibitedStateException, ConflictingOperationException,
ConcurrentModificationException, UnauthorizedOperationException, UnauthorizedResourceAccessException,
FailedDependencyException, ServiceUnavailableException, AwsServiceException, SdkClientException, WorkDocsException {
return deleteFolder(DeleteFolderRequest.builder().applyMutation(deleteFolderRequest).build());
}
/**
*
* Deletes the contents of the specified folder.
*
*
* @param deleteFolderContentsRequest
* @return Result of the DeleteFolderContents operation returned by the service.
* @throws EntityNotExistsException
* The resource does not exist.
* @throws ProhibitedStateException
* The specified document version is not in the INITIALIZED state.
* @throws ConflictingOperationException
* Another operation is in progress on the resource that conflicts with the current operation.
* @throws UnauthorizedOperationException
* The operation is not permitted.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.DeleteFolderContents
* @see AWS
* API Documentation
*/
default DeleteFolderContentsResponse deleteFolderContents(DeleteFolderContentsRequest deleteFolderContentsRequest)
throws EntityNotExistsException, ProhibitedStateException, ConflictingOperationException,
UnauthorizedOperationException, UnauthorizedResourceAccessException, FailedDependencyException,
ServiceUnavailableException, AwsServiceException, SdkClientException, WorkDocsException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the contents of the specified folder.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteFolderContentsRequest.Builder} avoiding the
* need to create one manually via {@link DeleteFolderContentsRequest#builder()}
*
*
* @param deleteFolderContentsRequest
* A {@link Consumer} that will call methods on {@link DeleteFolderContentsRequest.Builder} to create a
* request.
* @return Result of the DeleteFolderContents operation returned by the service.
* @throws EntityNotExistsException
* The resource does not exist.
* @throws ProhibitedStateException
* The specified document version is not in the INITIALIZED state.
* @throws ConflictingOperationException
* Another operation is in progress on the resource that conflicts with the current operation.
* @throws UnauthorizedOperationException
* The operation is not permitted.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.DeleteFolderContents
* @see AWS
* API Documentation
*/
default DeleteFolderContentsResponse deleteFolderContents(
Consumer deleteFolderContentsRequest) throws EntityNotExistsException,
ProhibitedStateException, ConflictingOperationException, UnauthorizedOperationException,
UnauthorizedResourceAccessException, FailedDependencyException, ServiceUnavailableException, AwsServiceException,
SdkClientException, WorkDocsException {
return deleteFolderContents(DeleteFolderContentsRequest.builder().applyMutation(deleteFolderContentsRequest).build());
}
/**
*
* Deletes the specified list of labels from a resource.
*
*
* @param deleteLabelsRequest
* @return Result of the DeleteLabels operation returned by the service.
* @throws EntityNotExistsException
* The resource does not exist.
* @throws UnauthorizedOperationException
* The operation is not permitted.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.DeleteLabels
* @see AWS API
* Documentation
*/
default DeleteLabelsResponse deleteLabels(DeleteLabelsRequest deleteLabelsRequest) throws EntityNotExistsException,
UnauthorizedOperationException, UnauthorizedResourceAccessException, FailedDependencyException,
ServiceUnavailableException, AwsServiceException, SdkClientException, WorkDocsException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the specified list of labels from a resource.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteLabelsRequest.Builder} avoiding the need to
* create one manually via {@link DeleteLabelsRequest#builder()}
*
*
* @param deleteLabelsRequest
* A {@link Consumer} that will call methods on {@link DeleteLabelsRequest.Builder} to create a request.
* @return Result of the DeleteLabels operation returned by the service.
* @throws EntityNotExistsException
* The resource does not exist.
* @throws UnauthorizedOperationException
* The operation is not permitted.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.DeleteLabels
* @see AWS API
* Documentation
*/
default DeleteLabelsResponse deleteLabels(Consumer deleteLabelsRequest)
throws EntityNotExistsException, UnauthorizedOperationException, UnauthorizedResourceAccessException,
FailedDependencyException, ServiceUnavailableException, AwsServiceException, SdkClientException, WorkDocsException {
return deleteLabels(DeleteLabelsRequest.builder().applyMutation(deleteLabelsRequest).build());
}
/**
*
* Deletes the specified subscription from the specified organization.
*
*
* @param deleteNotificationSubscriptionRequest
* @return Result of the DeleteNotificationSubscription operation returned by the service.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws EntityNotExistsException
* The resource does not exist.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws ProhibitedStateException
* The specified document version is not in the INITIALIZED state.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.DeleteNotificationSubscription
* @see AWS API Documentation
*/
default DeleteNotificationSubscriptionResponse deleteNotificationSubscription(
DeleteNotificationSubscriptionRequest deleteNotificationSubscriptionRequest)
throws UnauthorizedResourceAccessException, EntityNotExistsException, ServiceUnavailableException,
ProhibitedStateException, AwsServiceException, SdkClientException, WorkDocsException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the specified subscription from the specified organization.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteNotificationSubscriptionRequest.Builder}
* avoiding the need to create one manually via {@link DeleteNotificationSubscriptionRequest#builder()}
*
*
* @param deleteNotificationSubscriptionRequest
* A {@link Consumer} that will call methods on {@link DeleteNotificationSubscriptionRequest.Builder} to
* create a request.
* @return Result of the DeleteNotificationSubscription operation returned by the service.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws EntityNotExistsException
* The resource does not exist.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws ProhibitedStateException
* The specified document version is not in the INITIALIZED state.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.DeleteNotificationSubscription
* @see AWS API Documentation
*/
default DeleteNotificationSubscriptionResponse deleteNotificationSubscription(
Consumer deleteNotificationSubscriptionRequest)
throws UnauthorizedResourceAccessException, EntityNotExistsException, ServiceUnavailableException,
ProhibitedStateException, AwsServiceException, SdkClientException, WorkDocsException {
return deleteNotificationSubscription(DeleteNotificationSubscriptionRequest.builder()
.applyMutation(deleteNotificationSubscriptionRequest).build());
}
/**
*
* Deletes the specified user from a Simple AD or Microsoft AD directory.
*
*
* @param deleteUserRequest
* @return Result of the DeleteUser operation returned by the service.
* @throws EntityNotExistsException
* The resource does not exist.
* @throws UnauthorizedOperationException
* The operation is not permitted.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.DeleteUser
* @see AWS API
* Documentation
*/
default DeleteUserResponse deleteUser(DeleteUserRequest deleteUserRequest) throws EntityNotExistsException,
UnauthorizedOperationException, UnauthorizedResourceAccessException, FailedDependencyException,
ServiceUnavailableException, AwsServiceException, SdkClientException, WorkDocsException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the specified user from a Simple AD or Microsoft AD directory.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteUserRequest.Builder} avoiding the need to
* create one manually via {@link DeleteUserRequest#builder()}
*
*
* @param deleteUserRequest
* A {@link Consumer} that will call methods on {@link DeleteUserRequest.Builder} to create a request.
* @return Result of the DeleteUser operation returned by the service.
* @throws EntityNotExistsException
* The resource does not exist.
* @throws UnauthorizedOperationException
* The operation is not permitted.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.DeleteUser
* @see AWS API
* Documentation
*/
default DeleteUserResponse deleteUser(Consumer deleteUserRequest) throws EntityNotExistsException,
UnauthorizedOperationException, UnauthorizedResourceAccessException, FailedDependencyException,
ServiceUnavailableException, AwsServiceException, SdkClientException, WorkDocsException {
return deleteUser(DeleteUserRequest.builder().applyMutation(deleteUserRequest).build());
}
/**
*
* Describes the user activities in a specified time period.
*
*
* @param describeActivitiesRequest
* @return Result of the DescribeActivities operation returned by the service.
* @throws UnauthorizedOperationException
* The operation is not permitted.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws InvalidArgumentException
* The pagination marker or limit fields are not valid.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.DescribeActivities
* @see AWS
* API Documentation
*/
default DescribeActivitiesResponse describeActivities(DescribeActivitiesRequest describeActivitiesRequest)
throws UnauthorizedOperationException, UnauthorizedResourceAccessException, InvalidArgumentException,
FailedDependencyException, ServiceUnavailableException, AwsServiceException, SdkClientException, WorkDocsException {
throw new UnsupportedOperationException();
}
/**
*
* Describes the user activities in a specified time period.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeActivitiesRequest.Builder} avoiding the
* need to create one manually via {@link DescribeActivitiesRequest#builder()}
*
*
* @param describeActivitiesRequest
* A {@link Consumer} that will call methods on {@link DescribeActivitiesRequest.Builder} to create a
* request.
* @return Result of the DescribeActivities operation returned by the service.
* @throws UnauthorizedOperationException
* The operation is not permitted.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws InvalidArgumentException
* The pagination marker or limit fields are not valid.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.DescribeActivities
* @see AWS
* API Documentation
*/
default DescribeActivitiesResponse describeActivities(Consumer describeActivitiesRequest)
throws UnauthorizedOperationException, UnauthorizedResourceAccessException, InvalidArgumentException,
FailedDependencyException, ServiceUnavailableException, AwsServiceException, SdkClientException, WorkDocsException {
return describeActivities(DescribeActivitiesRequest.builder().applyMutation(describeActivitiesRequest).build());
}
/**
*
* List all the comments for the specified document version.
*
*
* @param describeCommentsRequest
* @return Result of the DescribeComments operation returned by the service.
* @throws EntityNotExistsException
* The resource does not exist.
* @throws ProhibitedStateException
* The specified document version is not in the INITIALIZED state.
* @throws UnauthorizedOperationException
* The operation is not permitted.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.DescribeComments
* @see AWS API
* Documentation
*/
default DescribeCommentsResponse describeComments(DescribeCommentsRequest describeCommentsRequest)
throws EntityNotExistsException, ProhibitedStateException, UnauthorizedOperationException,
UnauthorizedResourceAccessException, FailedDependencyException, ServiceUnavailableException, AwsServiceException,
SdkClientException, WorkDocsException {
throw new UnsupportedOperationException();
}
/**
*
* List all the comments for the specified document version.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeCommentsRequest.Builder} avoiding the need
* to create one manually via {@link DescribeCommentsRequest#builder()}
*
*
* @param describeCommentsRequest
* A {@link Consumer} that will call methods on {@link DescribeCommentsRequest.Builder} to create a request.
* @return Result of the DescribeComments operation returned by the service.
* @throws EntityNotExistsException
* The resource does not exist.
* @throws ProhibitedStateException
* The specified document version is not in the INITIALIZED state.
* @throws UnauthorizedOperationException
* The operation is not permitted.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.DescribeComments
* @see AWS API
* Documentation
*/
default DescribeCommentsResponse describeComments(Consumer describeCommentsRequest)
throws EntityNotExistsException, ProhibitedStateException, UnauthorizedOperationException,
UnauthorizedResourceAccessException, FailedDependencyException, ServiceUnavailableException, AwsServiceException,
SdkClientException, WorkDocsException {
return describeComments(DescribeCommentsRequest.builder().applyMutation(describeCommentsRequest).build());
}
/**
*
* Retrieves the document versions for the specified document.
*
*
* By default, only active versions are returned.
*
*
* @param describeDocumentVersionsRequest
* @return Result of the DescribeDocumentVersions operation returned by the service.
* @throws EntityNotExistsException
* The resource does not exist.
* @throws UnauthorizedOperationException
* The operation is not permitted.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws InvalidArgumentException
* The pagination marker or limit fields are not valid.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws ProhibitedStateException
* The specified document version is not in the INITIALIZED state.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.DescribeDocumentVersions
* @see AWS API Documentation
*/
default DescribeDocumentVersionsResponse describeDocumentVersions(
DescribeDocumentVersionsRequest describeDocumentVersionsRequest) throws EntityNotExistsException,
UnauthorizedOperationException, UnauthorizedResourceAccessException, InvalidArgumentException,
FailedDependencyException, ServiceUnavailableException, ProhibitedStateException, AwsServiceException,
SdkClientException, WorkDocsException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the document versions for the specified document.
*
*
* By default, only active versions are returned.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeDocumentVersionsRequest.Builder} avoiding
* the need to create one manually via {@link DescribeDocumentVersionsRequest#builder()}
*
*
* @param describeDocumentVersionsRequest
* A {@link Consumer} that will call methods on {@link DescribeDocumentVersionsRequest.Builder} to create a
* request.
* @return Result of the DescribeDocumentVersions operation returned by the service.
* @throws EntityNotExistsException
* The resource does not exist.
* @throws UnauthorizedOperationException
* The operation is not permitted.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws InvalidArgumentException
* The pagination marker or limit fields are not valid.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws ProhibitedStateException
* The specified document version is not in the INITIALIZED state.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.DescribeDocumentVersions
* @see AWS API Documentation
*/
default DescribeDocumentVersionsResponse describeDocumentVersions(
Consumer describeDocumentVersionsRequest) throws EntityNotExistsException,
UnauthorizedOperationException, UnauthorizedResourceAccessException, InvalidArgumentException,
FailedDependencyException, ServiceUnavailableException, ProhibitedStateException, AwsServiceException,
SdkClientException, WorkDocsException {
return describeDocumentVersions(DescribeDocumentVersionsRequest.builder().applyMutation(describeDocumentVersionsRequest)
.build());
}
/**
*
* Retrieves the document versions for the specified document.
*
*
* By default, only active versions are returned.
*
*
*
* This is a variant of
* {@link #describeDocumentVersions(software.amazon.awssdk.services.workdocs.model.DescribeDocumentVersionsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.workdocs.paginators.DescribeDocumentVersionsIterable responses = client.describeDocumentVersionsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.workdocs.paginators.DescribeDocumentVersionsIterable responses = client
* .describeDocumentVersionsPaginator(request);
* for (software.amazon.awssdk.services.workdocs.model.DescribeDocumentVersionsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.workdocs.paginators.DescribeDocumentVersionsIterable responses = client.describeDocumentVersionsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of Limit won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeDocumentVersions(software.amazon.awssdk.services.workdocs.model.DescribeDocumentVersionsRequest)}
* operation.
*
*
* @param describeDocumentVersionsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws EntityNotExistsException
* The resource does not exist.
* @throws UnauthorizedOperationException
* The operation is not permitted.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws InvalidArgumentException
* The pagination marker or limit fields are not valid.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws ProhibitedStateException
* The specified document version is not in the INITIALIZED state.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.DescribeDocumentVersions
* @see AWS API Documentation
*/
default DescribeDocumentVersionsIterable describeDocumentVersionsPaginator(
DescribeDocumentVersionsRequest describeDocumentVersionsRequest) throws EntityNotExistsException,
UnauthorizedOperationException, UnauthorizedResourceAccessException, InvalidArgumentException,
FailedDependencyException, ServiceUnavailableException, ProhibitedStateException, AwsServiceException,
SdkClientException, WorkDocsException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the document versions for the specified document.
*
*
* By default, only active versions are returned.
*
*
*
* This is a variant of
* {@link #describeDocumentVersions(software.amazon.awssdk.services.workdocs.model.DescribeDocumentVersionsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.workdocs.paginators.DescribeDocumentVersionsIterable responses = client.describeDocumentVersionsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.workdocs.paginators.DescribeDocumentVersionsIterable responses = client
* .describeDocumentVersionsPaginator(request);
* for (software.amazon.awssdk.services.workdocs.model.DescribeDocumentVersionsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.workdocs.paginators.DescribeDocumentVersionsIterable responses = client.describeDocumentVersionsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of Limit won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeDocumentVersions(software.amazon.awssdk.services.workdocs.model.DescribeDocumentVersionsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link DescribeDocumentVersionsRequest.Builder} avoiding
* the need to create one manually via {@link DescribeDocumentVersionsRequest#builder()}
*
*
* @param describeDocumentVersionsRequest
* A {@link Consumer} that will call methods on {@link DescribeDocumentVersionsRequest.Builder} to create a
* request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws EntityNotExistsException
* The resource does not exist.
* @throws UnauthorizedOperationException
* The operation is not permitted.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws InvalidArgumentException
* The pagination marker or limit fields are not valid.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws ProhibitedStateException
* The specified document version is not in the INITIALIZED state.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.DescribeDocumentVersions
* @see AWS API Documentation
*/
default DescribeDocumentVersionsIterable describeDocumentVersionsPaginator(
Consumer describeDocumentVersionsRequest) throws EntityNotExistsException,
UnauthorizedOperationException, UnauthorizedResourceAccessException, InvalidArgumentException,
FailedDependencyException, ServiceUnavailableException, ProhibitedStateException, AwsServiceException,
SdkClientException, WorkDocsException {
return describeDocumentVersionsPaginator(DescribeDocumentVersionsRequest.builder()
.applyMutation(describeDocumentVersionsRequest).build());
}
/**
*
* Describes the contents of the specified folder, including its documents and subfolders.
*
*
* By default, Amazon WorkDocs returns the first 100 active document and folder metadata items. If there are more
* results, the response includes a marker that you can use to request the next set of results. You can also request
* initialized documents.
*
*
* @param describeFolderContentsRequest
* @return Result of the DescribeFolderContents operation returned by the service.
* @throws EntityNotExistsException
* The resource does not exist.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws InvalidArgumentException
* The pagination marker or limit fields are not valid.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws ProhibitedStateException
* The specified document version is not in the INITIALIZED state.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.DescribeFolderContents
* @see AWS API Documentation
*/
default DescribeFolderContentsResponse describeFolderContents(DescribeFolderContentsRequest describeFolderContentsRequest)
throws EntityNotExistsException, UnauthorizedResourceAccessException, InvalidArgumentException,
FailedDependencyException, ServiceUnavailableException, ProhibitedStateException, AwsServiceException,
SdkClientException, WorkDocsException {
throw new UnsupportedOperationException();
}
/**
*
* Describes the contents of the specified folder, including its documents and subfolders.
*
*
* By default, Amazon WorkDocs returns the first 100 active document and folder metadata items. If there are more
* results, the response includes a marker that you can use to request the next set of results. You can also request
* initialized documents.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeFolderContentsRequest.Builder} avoiding the
* need to create one manually via {@link DescribeFolderContentsRequest#builder()}
*
*
* @param describeFolderContentsRequest
* A {@link Consumer} that will call methods on {@link DescribeFolderContentsRequest.Builder} to create a
* request.
* @return Result of the DescribeFolderContents operation returned by the service.
* @throws EntityNotExistsException
* The resource does not exist.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws InvalidArgumentException
* The pagination marker or limit fields are not valid.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws ProhibitedStateException
* The specified document version is not in the INITIALIZED state.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.DescribeFolderContents
* @see AWS API Documentation
*/
default DescribeFolderContentsResponse describeFolderContents(
Consumer describeFolderContentsRequest) throws EntityNotExistsException,
UnauthorizedResourceAccessException, InvalidArgumentException, FailedDependencyException,
ServiceUnavailableException, ProhibitedStateException, AwsServiceException, SdkClientException, WorkDocsException {
return describeFolderContents(DescribeFolderContentsRequest.builder().applyMutation(describeFolderContentsRequest)
.build());
}
/**
*
* Describes the contents of the specified folder, including its documents and subfolders.
*
*
* By default, Amazon WorkDocs returns the first 100 active document and folder metadata items. If there are more
* results, the response includes a marker that you can use to request the next set of results. You can also request
* initialized documents.
*
*
*
* This is a variant of
* {@link #describeFolderContents(software.amazon.awssdk.services.workdocs.model.DescribeFolderContentsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.workdocs.paginators.DescribeFolderContentsIterable responses = client.describeFolderContentsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.workdocs.paginators.DescribeFolderContentsIterable responses = client
* .describeFolderContentsPaginator(request);
* for (software.amazon.awssdk.services.workdocs.model.DescribeFolderContentsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.workdocs.paginators.DescribeFolderContentsIterable responses = client.describeFolderContentsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of Limit won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeFolderContents(software.amazon.awssdk.services.workdocs.model.DescribeFolderContentsRequest)}
* operation.
*
*
* @param describeFolderContentsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws EntityNotExistsException
* The resource does not exist.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws InvalidArgumentException
* The pagination marker or limit fields are not valid.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws ProhibitedStateException
* The specified document version is not in the INITIALIZED state.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.DescribeFolderContents
* @see AWS API Documentation
*/
default DescribeFolderContentsIterable describeFolderContentsPaginator(
DescribeFolderContentsRequest describeFolderContentsRequest) throws EntityNotExistsException,
UnauthorizedResourceAccessException, InvalidArgumentException, FailedDependencyException,
ServiceUnavailableException, ProhibitedStateException, AwsServiceException, SdkClientException, WorkDocsException {
throw new UnsupportedOperationException();
}
/**
*
* Describes the contents of the specified folder, including its documents and subfolders.
*
*
* By default, Amazon WorkDocs returns the first 100 active document and folder metadata items. If there are more
* results, the response includes a marker that you can use to request the next set of results. You can also request
* initialized documents.
*
*
*
* This is a variant of
* {@link #describeFolderContents(software.amazon.awssdk.services.workdocs.model.DescribeFolderContentsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.workdocs.paginators.DescribeFolderContentsIterable responses = client.describeFolderContentsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.workdocs.paginators.DescribeFolderContentsIterable responses = client
* .describeFolderContentsPaginator(request);
* for (software.amazon.awssdk.services.workdocs.model.DescribeFolderContentsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.workdocs.paginators.DescribeFolderContentsIterable responses = client.describeFolderContentsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of Limit won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeFolderContents(software.amazon.awssdk.services.workdocs.model.DescribeFolderContentsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link DescribeFolderContentsRequest.Builder} avoiding the
* need to create one manually via {@link DescribeFolderContentsRequest#builder()}
*
*
* @param describeFolderContentsRequest
* A {@link Consumer} that will call methods on {@link DescribeFolderContentsRequest.Builder} to create a
* request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws EntityNotExistsException
* The resource does not exist.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws InvalidArgumentException
* The pagination marker or limit fields are not valid.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws ProhibitedStateException
* The specified document version is not in the INITIALIZED state.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.DescribeFolderContents
* @see AWS API Documentation
*/
default DescribeFolderContentsIterable describeFolderContentsPaginator(
Consumer describeFolderContentsRequest) throws EntityNotExistsException,
UnauthorizedResourceAccessException, InvalidArgumentException, FailedDependencyException,
ServiceUnavailableException, ProhibitedStateException, AwsServiceException, SdkClientException, WorkDocsException {
return describeFolderContentsPaginator(DescribeFolderContentsRequest.builder()
.applyMutation(describeFolderContentsRequest).build());
}
/**
*
* Describes the groups specified by the query. Groups are defined by the underlying Active Directory.
*
*
* @param describeGroupsRequest
* @return Result of the DescribeGroups operation returned by the service.
* @throws UnauthorizedOperationException
* The operation is not permitted.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.DescribeGroups
* @see AWS API
* Documentation
*/
default DescribeGroupsResponse describeGroups(DescribeGroupsRequest describeGroupsRequest)
throws UnauthorizedOperationException, UnauthorizedResourceAccessException, FailedDependencyException,
ServiceUnavailableException, AwsServiceException, SdkClientException, WorkDocsException {
throw new UnsupportedOperationException();
}
/**
*
* Describes the groups specified by the query. Groups are defined by the underlying Active Directory.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeGroupsRequest.Builder} avoiding the need to
* create one manually via {@link DescribeGroupsRequest#builder()}
*
*
* @param describeGroupsRequest
* A {@link Consumer} that will call methods on {@link DescribeGroupsRequest.Builder} to create a request.
* @return Result of the DescribeGroups operation returned by the service.
* @throws UnauthorizedOperationException
* The operation is not permitted.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.DescribeGroups
* @see AWS API
* Documentation
*/
default DescribeGroupsResponse describeGroups(Consumer describeGroupsRequest)
throws UnauthorizedOperationException, UnauthorizedResourceAccessException, FailedDependencyException,
ServiceUnavailableException, AwsServiceException, SdkClientException, WorkDocsException {
return describeGroups(DescribeGroupsRequest.builder().applyMutation(describeGroupsRequest).build());
}
/**
*
* Lists the specified notification subscriptions.
*
*
* @param describeNotificationSubscriptionsRequest
* @return Result of the DescribeNotificationSubscriptions operation returned by the service.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws EntityNotExistsException
* The resource does not exist.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.DescribeNotificationSubscriptions
* @see AWS API Documentation
*/
default DescribeNotificationSubscriptionsResponse describeNotificationSubscriptions(
DescribeNotificationSubscriptionsRequest describeNotificationSubscriptionsRequest)
throws UnauthorizedResourceAccessException, EntityNotExistsException, ServiceUnavailableException,
AwsServiceException, SdkClientException, WorkDocsException {
throw new UnsupportedOperationException();
}
/**
*
* Lists the specified notification subscriptions.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeNotificationSubscriptionsRequest.Builder}
* avoiding the need to create one manually via {@link DescribeNotificationSubscriptionsRequest#builder()}
*
*
* @param describeNotificationSubscriptionsRequest
* A {@link Consumer} that will call methods on {@link DescribeNotificationSubscriptionsRequest.Builder} to
* create a request.
* @return Result of the DescribeNotificationSubscriptions operation returned by the service.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws EntityNotExistsException
* The resource does not exist.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.DescribeNotificationSubscriptions
* @see AWS API Documentation
*/
default DescribeNotificationSubscriptionsResponse describeNotificationSubscriptions(
Consumer describeNotificationSubscriptionsRequest)
throws UnauthorizedResourceAccessException, EntityNotExistsException, ServiceUnavailableException,
AwsServiceException, SdkClientException, WorkDocsException {
return describeNotificationSubscriptions(DescribeNotificationSubscriptionsRequest.builder()
.applyMutation(describeNotificationSubscriptionsRequest).build());
}
/**
*
* Describes the permissions of a specified resource.
*
*
* @param describeResourcePermissionsRequest
* @return Result of the DescribeResourcePermissions operation returned by the service.
* @throws UnauthorizedOperationException
* The operation is not permitted.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.DescribeResourcePermissions
* @see AWS API Documentation
*/
default DescribeResourcePermissionsResponse describeResourcePermissions(
DescribeResourcePermissionsRequest describeResourcePermissionsRequest) throws UnauthorizedOperationException,
UnauthorizedResourceAccessException, FailedDependencyException, ServiceUnavailableException, AwsServiceException,
SdkClientException, WorkDocsException {
throw new UnsupportedOperationException();
}
/**
*
* Describes the permissions of a specified resource.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeResourcePermissionsRequest.Builder}
* avoiding the need to create one manually via {@link DescribeResourcePermissionsRequest#builder()}
*
*
* @param describeResourcePermissionsRequest
* A {@link Consumer} that will call methods on {@link DescribeResourcePermissionsRequest.Builder} to create
* a request.
* @return Result of the DescribeResourcePermissions operation returned by the service.
* @throws UnauthorizedOperationException
* The operation is not permitted.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.DescribeResourcePermissions
* @see AWS API Documentation
*/
default DescribeResourcePermissionsResponse describeResourcePermissions(
Consumer describeResourcePermissionsRequest)
throws UnauthorizedOperationException, UnauthorizedResourceAccessException, FailedDependencyException,
ServiceUnavailableException, AwsServiceException, SdkClientException, WorkDocsException {
return describeResourcePermissions(DescribeResourcePermissionsRequest.builder()
.applyMutation(describeResourcePermissionsRequest).build());
}
/**
*
* Describes the current user's special folders; the RootFolder
and the RecycleBin
.
* RootFolder
is the root of user's files and folders and RecycleBin
is the root of
* recycled items. This is not a valid action for SigV4 (administrative API) clients.
*
*
* This action requires an authentication token. To get an authentication token, register an application with Amazon
* WorkDocs. For more information, see Authentication and Access
* Control for User Applications in the Amazon WorkDocs Developer Guide.
*
*
* @param describeRootFoldersRequest
* @return Result of the DescribeRootFolders operation returned by the service.
* @throws UnauthorizedOperationException
* The operation is not permitted.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws InvalidArgumentException
* The pagination marker or limit fields are not valid.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.DescribeRootFolders
* @see AWS
* API Documentation
*/
default DescribeRootFoldersResponse describeRootFolders(DescribeRootFoldersRequest describeRootFoldersRequest)
throws UnauthorizedOperationException, UnauthorizedResourceAccessException, InvalidArgumentException,
FailedDependencyException, ServiceUnavailableException, AwsServiceException, SdkClientException, WorkDocsException {
throw new UnsupportedOperationException();
}
/**
*
* Describes the current user's special folders; the RootFolder
and the RecycleBin
.
* RootFolder
is the root of user's files and folders and RecycleBin
is the root of
* recycled items. This is not a valid action for SigV4 (administrative API) clients.
*
*
* This action requires an authentication token. To get an authentication token, register an application with Amazon
* WorkDocs. For more information, see Authentication and Access
* Control for User Applications in the Amazon WorkDocs Developer Guide.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeRootFoldersRequest.Builder} avoiding the
* need to create one manually via {@link DescribeRootFoldersRequest#builder()}
*
*
* @param describeRootFoldersRequest
* A {@link Consumer} that will call methods on {@link DescribeRootFoldersRequest.Builder} to create a
* request.
* @return Result of the DescribeRootFolders operation returned by the service.
* @throws UnauthorizedOperationException
* The operation is not permitted.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws InvalidArgumentException
* The pagination marker or limit fields are not valid.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.DescribeRootFolders
* @see AWS
* API Documentation
*/
default DescribeRootFoldersResponse describeRootFolders(
Consumer describeRootFoldersRequest) throws UnauthorizedOperationException,
UnauthorizedResourceAccessException, InvalidArgumentException, FailedDependencyException,
ServiceUnavailableException, AwsServiceException, SdkClientException, WorkDocsException {
return describeRootFolders(DescribeRootFoldersRequest.builder().applyMutation(describeRootFoldersRequest).build());
}
/**
*
* Describes the specified users. You can describe all users or filter the results (for example, by status or
* organization).
*
*
* By default, Amazon WorkDocs returns the first 24 active or pending users. If there are more results, the response
* includes a marker that you can use to request the next set of results.
*
*
* @param describeUsersRequest
* @return Result of the DescribeUsers operation returned by the service.
* @throws EntityNotExistsException
* The resource does not exist.
* @throws UnauthorizedOperationException
* The operation is not permitted.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws InvalidArgumentException
* The pagination marker or limit fields are not valid.
* @throws RequestedEntityTooLargeException
* The response is too large to return. The request must include a filter to reduce the size of the
* response.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.DescribeUsers
* @see AWS API
* Documentation
*/
default DescribeUsersResponse describeUsers(DescribeUsersRequest describeUsersRequest) throws EntityNotExistsException,
UnauthorizedOperationException, UnauthorizedResourceAccessException, FailedDependencyException,
ServiceUnavailableException, InvalidArgumentException, RequestedEntityTooLargeException, AwsServiceException,
SdkClientException, WorkDocsException {
throw new UnsupportedOperationException();
}
/**
*
* Describes the specified users. You can describe all users or filter the results (for example, by status or
* organization).
*
*
* By default, Amazon WorkDocs returns the first 24 active or pending users. If there are more results, the response
* includes a marker that you can use to request the next set of results.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeUsersRequest.Builder} avoiding the need to
* create one manually via {@link DescribeUsersRequest#builder()}
*
*
* @param describeUsersRequest
* A {@link Consumer} that will call methods on {@link DescribeUsersRequest.Builder} to create a request.
* @return Result of the DescribeUsers operation returned by the service.
* @throws EntityNotExistsException
* The resource does not exist.
* @throws UnauthorizedOperationException
* The operation is not permitted.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws InvalidArgumentException
* The pagination marker or limit fields are not valid.
* @throws RequestedEntityTooLargeException
* The response is too large to return. The request must include a filter to reduce the size of the
* response.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.DescribeUsers
* @see AWS API
* Documentation
*/
default DescribeUsersResponse describeUsers(Consumer describeUsersRequest)
throws EntityNotExistsException, UnauthorizedOperationException, UnauthorizedResourceAccessException,
FailedDependencyException, ServiceUnavailableException, InvalidArgumentException, RequestedEntityTooLargeException,
AwsServiceException, SdkClientException, WorkDocsException {
return describeUsers(DescribeUsersRequest.builder().applyMutation(describeUsersRequest).build());
}
/**
*
* Describes the specified users. You can describe all users or filter the results (for example, by status or
* organization).
*
*
* By default, Amazon WorkDocs returns the first 24 active or pending users. If there are more results, the response
* includes a marker that you can use to request the next set of results.
*
*
*
* This is a variant of {@link #describeUsers(software.amazon.awssdk.services.workdocs.model.DescribeUsersRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.workdocs.paginators.DescribeUsersIterable responses = client.describeUsersPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.workdocs.paginators.DescribeUsersIterable responses = client.describeUsersPaginator(request);
* for (software.amazon.awssdk.services.workdocs.model.DescribeUsersResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.workdocs.paginators.DescribeUsersIterable responses = client.describeUsersPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of Limit won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeUsers(software.amazon.awssdk.services.workdocs.model.DescribeUsersRequest)} operation.
*
*
* @param describeUsersRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws EntityNotExistsException
* The resource does not exist.
* @throws UnauthorizedOperationException
* The operation is not permitted.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws InvalidArgumentException
* The pagination marker or limit fields are not valid.
* @throws RequestedEntityTooLargeException
* The response is too large to return. The request must include a filter to reduce the size of the
* response.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.DescribeUsers
* @see AWS API
* Documentation
*/
default DescribeUsersIterable describeUsersPaginator(DescribeUsersRequest describeUsersRequest)
throws EntityNotExistsException, UnauthorizedOperationException, UnauthorizedResourceAccessException,
FailedDependencyException, ServiceUnavailableException, InvalidArgumentException, RequestedEntityTooLargeException,
AwsServiceException, SdkClientException, WorkDocsException {
throw new UnsupportedOperationException();
}
/**
*
* Describes the specified users. You can describe all users or filter the results (for example, by status or
* organization).
*
*
* By default, Amazon WorkDocs returns the first 24 active or pending users. If there are more results, the response
* includes a marker that you can use to request the next set of results.
*
*
*
* This is a variant of {@link #describeUsers(software.amazon.awssdk.services.workdocs.model.DescribeUsersRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.workdocs.paginators.DescribeUsersIterable responses = client.describeUsersPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.workdocs.paginators.DescribeUsersIterable responses = client.describeUsersPaginator(request);
* for (software.amazon.awssdk.services.workdocs.model.DescribeUsersResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.workdocs.paginators.DescribeUsersIterable responses = client.describeUsersPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of Limit won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeUsers(software.amazon.awssdk.services.workdocs.model.DescribeUsersRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link DescribeUsersRequest.Builder} avoiding the need to
* create one manually via {@link DescribeUsersRequest#builder()}
*
*
* @param describeUsersRequest
* A {@link Consumer} that will call methods on {@link DescribeUsersRequest.Builder} to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws EntityNotExistsException
* The resource does not exist.
* @throws UnauthorizedOperationException
* The operation is not permitted.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws InvalidArgumentException
* The pagination marker or limit fields are not valid.
* @throws RequestedEntityTooLargeException
* The response is too large to return. The request must include a filter to reduce the size of the
* response.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.DescribeUsers
* @see AWS API
* Documentation
*/
default DescribeUsersIterable describeUsersPaginator(Consumer describeUsersRequest)
throws EntityNotExistsException, UnauthorizedOperationException, UnauthorizedResourceAccessException,
FailedDependencyException, ServiceUnavailableException, InvalidArgumentException, RequestedEntityTooLargeException,
AwsServiceException, SdkClientException, WorkDocsException {
return describeUsersPaginator(DescribeUsersRequest.builder().applyMutation(describeUsersRequest).build());
}
/**
*
* Retrieves details of the current user for whom the authentication token was generated. This is not a valid action
* for SigV4 (administrative API) clients.
*
*
* This action requires an authentication token. To get an authentication token, register an application with Amazon
* WorkDocs. For more information, see Authentication and Access
* Control for User Applications in the Amazon WorkDocs Developer Guide.
*
*
* @param getCurrentUserRequest
* @return Result of the GetCurrentUser operation returned by the service.
* @throws EntityNotExistsException
* The resource does not exist.
* @throws UnauthorizedOperationException
* The operation is not permitted.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.GetCurrentUser
* @see AWS API
* Documentation
*/
default GetCurrentUserResponse getCurrentUser(GetCurrentUserRequest getCurrentUserRequest) throws EntityNotExistsException,
UnauthorizedOperationException, UnauthorizedResourceAccessException, FailedDependencyException,
ServiceUnavailableException, AwsServiceException, SdkClientException, WorkDocsException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves details of the current user for whom the authentication token was generated. This is not a valid action
* for SigV4 (administrative API) clients.
*
*
* This action requires an authentication token. To get an authentication token, register an application with Amazon
* WorkDocs. For more information, see Authentication and Access
* Control for User Applications in the Amazon WorkDocs Developer Guide.
*
*
*
* This is a convenience which creates an instance of the {@link GetCurrentUserRequest.Builder} avoiding the need to
* create one manually via {@link GetCurrentUserRequest#builder()}
*
*
* @param getCurrentUserRequest
* A {@link Consumer} that will call methods on {@link GetCurrentUserRequest.Builder} to create a request.
* @return Result of the GetCurrentUser operation returned by the service.
* @throws EntityNotExistsException
* The resource does not exist.
* @throws UnauthorizedOperationException
* The operation is not permitted.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.GetCurrentUser
* @see AWS API
* Documentation
*/
default GetCurrentUserResponse getCurrentUser(Consumer getCurrentUserRequest)
throws EntityNotExistsException, UnauthorizedOperationException, UnauthorizedResourceAccessException,
FailedDependencyException, ServiceUnavailableException, AwsServiceException, SdkClientException, WorkDocsException {
return getCurrentUser(GetCurrentUserRequest.builder().applyMutation(getCurrentUserRequest).build());
}
/**
*
* Retrieves details of a document.
*
*
* @param getDocumentRequest
* @return Result of the GetDocument operation returned by the service.
* @throws EntityNotExistsException
* The resource does not exist.
* @throws UnauthorizedOperationException
* The operation is not permitted.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws InvalidArgumentException
* The pagination marker or limit fields are not valid.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws InvalidPasswordException
* The password is invalid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.GetDocument
* @see AWS API
* Documentation
*/
default GetDocumentResponse getDocument(GetDocumentRequest getDocumentRequest) throws EntityNotExistsException,
UnauthorizedOperationException, UnauthorizedResourceAccessException, InvalidArgumentException,
FailedDependencyException, ServiceUnavailableException, InvalidPasswordException, AwsServiceException,
SdkClientException, WorkDocsException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves details of a document.
*
*
*
* This is a convenience which creates an instance of the {@link GetDocumentRequest.Builder} avoiding the need to
* create one manually via {@link GetDocumentRequest#builder()}
*
*
* @param getDocumentRequest
* A {@link Consumer} that will call methods on {@link GetDocumentRequest.Builder} to create a request.
* @return Result of the GetDocument operation returned by the service.
* @throws EntityNotExistsException
* The resource does not exist.
* @throws UnauthorizedOperationException
* The operation is not permitted.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws InvalidArgumentException
* The pagination marker or limit fields are not valid.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws InvalidPasswordException
* The password is invalid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.GetDocument
* @see AWS API
* Documentation
*/
default GetDocumentResponse getDocument(Consumer getDocumentRequest)
throws EntityNotExistsException, UnauthorizedOperationException, UnauthorizedResourceAccessException,
InvalidArgumentException, FailedDependencyException, ServiceUnavailableException, InvalidPasswordException,
AwsServiceException, SdkClientException, WorkDocsException {
return getDocument(GetDocumentRequest.builder().applyMutation(getDocumentRequest).build());
}
/**
*
* Retrieves the path information (the hierarchy from the root folder) for the requested document.
*
*
* By default, Amazon WorkDocs returns a maximum of 100 levels upwards from the requested document and only includes
* the IDs of the parent folders in the path. You can limit the maximum number of levels. You can also request the
* names of the parent folders.
*
*
* @param getDocumentPathRequest
* @return Result of the GetDocumentPath operation returned by the service.
* @throws EntityNotExistsException
* The resource does not exist.
* @throws UnauthorizedOperationException
* The operation is not permitted.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.GetDocumentPath
* @see AWS API
* Documentation
*/
default GetDocumentPathResponse getDocumentPath(GetDocumentPathRequest getDocumentPathRequest)
throws EntityNotExistsException, UnauthorizedOperationException, UnauthorizedResourceAccessException,
FailedDependencyException, ServiceUnavailableException, AwsServiceException, SdkClientException, WorkDocsException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the path information (the hierarchy from the root folder) for the requested document.
*
*
* By default, Amazon WorkDocs returns a maximum of 100 levels upwards from the requested document and only includes
* the IDs of the parent folders in the path. You can limit the maximum number of levels. You can also request the
* names of the parent folders.
*
*
*
* This is a convenience which creates an instance of the {@link GetDocumentPathRequest.Builder} avoiding the need
* to create one manually via {@link GetDocumentPathRequest#builder()}
*
*
* @param getDocumentPathRequest
* A {@link Consumer} that will call methods on {@link GetDocumentPathRequest.Builder} to create a request.
* @return Result of the GetDocumentPath operation returned by the service.
* @throws EntityNotExistsException
* The resource does not exist.
* @throws UnauthorizedOperationException
* The operation is not permitted.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.GetDocumentPath
* @see AWS API
* Documentation
*/
default GetDocumentPathResponse getDocumentPath(Consumer getDocumentPathRequest)
throws EntityNotExistsException, UnauthorizedOperationException, UnauthorizedResourceAccessException,
FailedDependencyException, ServiceUnavailableException, AwsServiceException, SdkClientException, WorkDocsException {
return getDocumentPath(GetDocumentPathRequest.builder().applyMutation(getDocumentPathRequest).build());
}
/**
*
* Retrieves version metadata for the specified document.
*
*
* @param getDocumentVersionRequest
* @return Result of the GetDocumentVersion operation returned by the service.
* @throws EntityNotExistsException
* The resource does not exist.
* @throws UnauthorizedOperationException
* The operation is not permitted.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws ProhibitedStateException
* The specified document version is not in the INITIALIZED state.
* @throws InvalidPasswordException
* The password is invalid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.GetDocumentVersion
* @see AWS
* API Documentation
*/
default GetDocumentVersionResponse getDocumentVersion(GetDocumentVersionRequest getDocumentVersionRequest)
throws EntityNotExistsException, UnauthorizedOperationException, UnauthorizedResourceAccessException,
FailedDependencyException, ServiceUnavailableException, ProhibitedStateException, InvalidPasswordException,
AwsServiceException, SdkClientException, WorkDocsException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves version metadata for the specified document.
*
*
*
* This is a convenience which creates an instance of the {@link GetDocumentVersionRequest.Builder} avoiding the
* need to create one manually via {@link GetDocumentVersionRequest#builder()}
*
*
* @param getDocumentVersionRequest
* A {@link Consumer} that will call methods on {@link GetDocumentVersionRequest.Builder} to create a
* request.
* @return Result of the GetDocumentVersion operation returned by the service.
* @throws EntityNotExistsException
* The resource does not exist.
* @throws UnauthorizedOperationException
* The operation is not permitted.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws ProhibitedStateException
* The specified document version is not in the INITIALIZED state.
* @throws InvalidPasswordException
* The password is invalid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.GetDocumentVersion
* @see AWS
* API Documentation
*/
default GetDocumentVersionResponse getDocumentVersion(Consumer getDocumentVersionRequest)
throws EntityNotExistsException, UnauthorizedOperationException, UnauthorizedResourceAccessException,
FailedDependencyException, ServiceUnavailableException, ProhibitedStateException, InvalidPasswordException,
AwsServiceException, SdkClientException, WorkDocsException {
return getDocumentVersion(GetDocumentVersionRequest.builder().applyMutation(getDocumentVersionRequest).build());
}
/**
*
* Retrieves the metadata of the specified folder.
*
*
* @param getFolderRequest
* @return Result of the GetFolder operation returned by the service.
* @throws EntityNotExistsException
* The resource does not exist.
* @throws UnauthorizedOperationException
* The operation is not permitted.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws InvalidArgumentException
* The pagination marker or limit fields are not valid.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws ProhibitedStateException
* The specified document version is not in the INITIALIZED state.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.GetFolder
* @see AWS API
* Documentation
*/
default GetFolderResponse getFolder(GetFolderRequest getFolderRequest) throws EntityNotExistsException,
UnauthorizedOperationException, UnauthorizedResourceAccessException, InvalidArgumentException,
FailedDependencyException, ServiceUnavailableException, ProhibitedStateException, AwsServiceException,
SdkClientException, WorkDocsException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the metadata of the specified folder.
*
*
*
* This is a convenience which creates an instance of the {@link GetFolderRequest.Builder} avoiding the need to
* create one manually via {@link GetFolderRequest#builder()}
*
*
* @param getFolderRequest
* A {@link Consumer} that will call methods on {@link GetFolderRequest.Builder} to create a request.
* @return Result of the GetFolder operation returned by the service.
* @throws EntityNotExistsException
* The resource does not exist.
* @throws UnauthorizedOperationException
* The operation is not permitted.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws InvalidArgumentException
* The pagination marker or limit fields are not valid.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws ProhibitedStateException
* The specified document version is not in the INITIALIZED state.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.GetFolder
* @see AWS API
* Documentation
*/
default GetFolderResponse getFolder(Consumer getFolderRequest) throws EntityNotExistsException,
UnauthorizedOperationException, UnauthorizedResourceAccessException, InvalidArgumentException,
FailedDependencyException, ServiceUnavailableException, ProhibitedStateException, AwsServiceException,
SdkClientException, WorkDocsException {
return getFolder(GetFolderRequest.builder().applyMutation(getFolderRequest).build());
}
/**
*
* Retrieves the path information (the hierarchy from the root folder) for the specified folder.
*
*
* By default, Amazon WorkDocs returns a maximum of 100 levels upwards from the requested folder and only includes
* the IDs of the parent folders in the path. You can limit the maximum number of levels. You can also request the
* parent folder names.
*
*
* @param getFolderPathRequest
* @return Result of the GetFolderPath operation returned by the service.
* @throws EntityNotExistsException
* The resource does not exist.
* @throws UnauthorizedOperationException
* The operation is not permitted.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.GetFolderPath
* @see AWS API
* Documentation
*/
default GetFolderPathResponse getFolderPath(GetFolderPathRequest getFolderPathRequest) throws EntityNotExistsException,
UnauthorizedOperationException, UnauthorizedResourceAccessException, FailedDependencyException,
ServiceUnavailableException, AwsServiceException, SdkClientException, WorkDocsException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the path information (the hierarchy from the root folder) for the specified folder.
*
*
* By default, Amazon WorkDocs returns a maximum of 100 levels upwards from the requested folder and only includes
* the IDs of the parent folders in the path. You can limit the maximum number of levels. You can also request the
* parent folder names.
*
*
*
* This is a convenience which creates an instance of the {@link GetFolderPathRequest.Builder} avoiding the need to
* create one manually via {@link GetFolderPathRequest#builder()}
*
*
* @param getFolderPathRequest
* A {@link Consumer} that will call methods on {@link GetFolderPathRequest.Builder} to create a request.
* @return Result of the GetFolderPath operation returned by the service.
* @throws EntityNotExistsException
* The resource does not exist.
* @throws UnauthorizedOperationException
* The operation is not permitted.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.GetFolderPath
* @see AWS API
* Documentation
*/
default GetFolderPathResponse getFolderPath(Consumer getFolderPathRequest)
throws EntityNotExistsException, UnauthorizedOperationException, UnauthorizedResourceAccessException,
FailedDependencyException, ServiceUnavailableException, AwsServiceException, SdkClientException, WorkDocsException {
return getFolderPath(GetFolderPathRequest.builder().applyMutation(getFolderPathRequest).build());
}
/**
*
* Retrieves a collection of resources, including folders and documents. The only CollectionType
* supported is SHARED_WITH_ME
.
*
*
* @param getResourcesRequest
* @return Result of the GetResources operation returned by the service.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws UnauthorizedOperationException
* The operation is not permitted.
* @throws InvalidArgumentException
* The pagination marker or limit fields are not valid.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.GetResources
* @see AWS API
* Documentation
*/
default GetResourcesResponse getResources(GetResourcesRequest getResourcesRequest)
throws UnauthorizedResourceAccessException, UnauthorizedOperationException, InvalidArgumentException,
FailedDependencyException, ServiceUnavailableException, AwsServiceException, SdkClientException, WorkDocsException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves a collection of resources, including folders and documents. The only CollectionType
* supported is SHARED_WITH_ME
.
*
*
*
* This is a convenience which creates an instance of the {@link GetResourcesRequest.Builder} avoiding the need to
* create one manually via {@link GetResourcesRequest#builder()}
*
*
* @param getResourcesRequest
* A {@link Consumer} that will call methods on {@link GetResourcesRequest.Builder} to create a request.
* @return Result of the GetResources operation returned by the service.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws UnauthorizedOperationException
* The operation is not permitted.
* @throws InvalidArgumentException
* The pagination marker or limit fields are not valid.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.GetResources
* @see AWS API
* Documentation
*/
default GetResourcesResponse getResources(Consumer getResourcesRequest)
throws UnauthorizedResourceAccessException, UnauthorizedOperationException, InvalidArgumentException,
FailedDependencyException, ServiceUnavailableException, AwsServiceException, SdkClientException, WorkDocsException {
return getResources(GetResourcesRequest.builder().applyMutation(getResourcesRequest).build());
}
/**
*
* Creates a new document object and version object.
*
*
* The client specifies the parent folder ID and name of the document to upload. The ID is optionally specified when
* creating a new version of an existing document. This is the first step to upload a document. Next, upload the
* document to the URL returned from the call, and then call UpdateDocumentVersion.
*
*
* To cancel the document upload, call AbortDocumentVersionUpload.
*
*
* @param initiateDocumentVersionUploadRequest
* @return Result of the InitiateDocumentVersionUpload operation returned by the service.
* @throws EntityNotExistsException
* The resource does not exist.
* @throws EntityAlreadyExistsException
* The resource already exists.
* @throws StorageLimitExceededException
* The storage limit has been exceeded.
* @throws StorageLimitWillExceedException
* The storage limit will be exceeded.
* @throws ProhibitedStateException
* The specified document version is not in the INITIALIZED state.
* @throws UnauthorizedOperationException
* The operation is not permitted.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws DraftUploadOutOfSyncException
* This exception is thrown when a valid checkout ID is not presented on document version upload calls for a
* document that has been checked out from Web client.
* @throws ResourceAlreadyCheckedOutException
* The resource is already checked out.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.InitiateDocumentVersionUpload
* @see AWS API Documentation
*/
default InitiateDocumentVersionUploadResponse initiateDocumentVersionUpload(
InitiateDocumentVersionUploadRequest initiateDocumentVersionUploadRequest) throws EntityNotExistsException,
EntityAlreadyExistsException, StorageLimitExceededException, StorageLimitWillExceedException,
ProhibitedStateException, UnauthorizedOperationException, UnauthorizedResourceAccessException,
FailedDependencyException, ServiceUnavailableException, DraftUploadOutOfSyncException,
ResourceAlreadyCheckedOutException, AwsServiceException, SdkClientException, WorkDocsException {
throw new UnsupportedOperationException();
}
/**
*
* Creates a new document object and version object.
*
*
* The client specifies the parent folder ID and name of the document to upload. The ID is optionally specified when
* creating a new version of an existing document. This is the first step to upload a document. Next, upload the
* document to the URL returned from the call, and then call UpdateDocumentVersion.
*
*
* To cancel the document upload, call AbortDocumentVersionUpload.
*
*
*
* This is a convenience which creates an instance of the {@link InitiateDocumentVersionUploadRequest.Builder}
* avoiding the need to create one manually via {@link InitiateDocumentVersionUploadRequest#builder()}
*
*
* @param initiateDocumentVersionUploadRequest
* A {@link Consumer} that will call methods on {@link InitiateDocumentVersionUploadRequest.Builder} to
* create a request.
* @return Result of the InitiateDocumentVersionUpload operation returned by the service.
* @throws EntityNotExistsException
* The resource does not exist.
* @throws EntityAlreadyExistsException
* The resource already exists.
* @throws StorageLimitExceededException
* The storage limit has been exceeded.
* @throws StorageLimitWillExceedException
* The storage limit will be exceeded.
* @throws ProhibitedStateException
* The specified document version is not in the INITIALIZED state.
* @throws UnauthorizedOperationException
* The operation is not permitted.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws DraftUploadOutOfSyncException
* This exception is thrown when a valid checkout ID is not presented on document version upload calls for a
* document that has been checked out from Web client.
* @throws ResourceAlreadyCheckedOutException
* The resource is already checked out.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.InitiateDocumentVersionUpload
* @see AWS API Documentation
*/
default InitiateDocumentVersionUploadResponse initiateDocumentVersionUpload(
Consumer initiateDocumentVersionUploadRequest)
throws EntityNotExistsException, EntityAlreadyExistsException, StorageLimitExceededException,
StorageLimitWillExceedException, ProhibitedStateException, UnauthorizedOperationException,
UnauthorizedResourceAccessException, FailedDependencyException, ServiceUnavailableException,
DraftUploadOutOfSyncException, ResourceAlreadyCheckedOutException, AwsServiceException, SdkClientException,
WorkDocsException {
return initiateDocumentVersionUpload(InitiateDocumentVersionUploadRequest.builder()
.applyMutation(initiateDocumentVersionUploadRequest).build());
}
/**
*
* Removes all the permissions from the specified resource.
*
*
* @param removeAllResourcePermissionsRequest
* @return Result of the RemoveAllResourcePermissions operation returned by the service.
* @throws UnauthorizedOperationException
* The operation is not permitted.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.RemoveAllResourcePermissions
* @see AWS API Documentation
*/
default RemoveAllResourcePermissionsResponse removeAllResourcePermissions(
RemoveAllResourcePermissionsRequest removeAllResourcePermissionsRequest) throws UnauthorizedOperationException,
UnauthorizedResourceAccessException, FailedDependencyException, ServiceUnavailableException, AwsServiceException,
SdkClientException, WorkDocsException {
throw new UnsupportedOperationException();
}
/**
*
* Removes all the permissions from the specified resource.
*
*
*
* This is a convenience which creates an instance of the {@link RemoveAllResourcePermissionsRequest.Builder}
* avoiding the need to create one manually via {@link RemoveAllResourcePermissionsRequest#builder()}
*
*
* @param removeAllResourcePermissionsRequest
* A {@link Consumer} that will call methods on {@link RemoveAllResourcePermissionsRequest.Builder} to create
* a request.
* @return Result of the RemoveAllResourcePermissions operation returned by the service.
* @throws UnauthorizedOperationException
* The operation is not permitted.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.RemoveAllResourcePermissions
* @see AWS API Documentation
*/
default RemoveAllResourcePermissionsResponse removeAllResourcePermissions(
Consumer removeAllResourcePermissionsRequest)
throws UnauthorizedOperationException, UnauthorizedResourceAccessException, FailedDependencyException,
ServiceUnavailableException, AwsServiceException, SdkClientException, WorkDocsException {
return removeAllResourcePermissions(RemoveAllResourcePermissionsRequest.builder()
.applyMutation(removeAllResourcePermissionsRequest).build());
}
/**
*
* Removes the permission for the specified principal from the specified resource.
*
*
* @param removeResourcePermissionRequest
* @return Result of the RemoveResourcePermission operation returned by the service.
* @throws UnauthorizedOperationException
* The operation is not permitted.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.RemoveResourcePermission
* @see AWS API Documentation
*/
default RemoveResourcePermissionResponse removeResourcePermission(
RemoveResourcePermissionRequest removeResourcePermissionRequest) throws UnauthorizedOperationException,
UnauthorizedResourceAccessException, FailedDependencyException, ServiceUnavailableException, AwsServiceException,
SdkClientException, WorkDocsException {
throw new UnsupportedOperationException();
}
/**
*
* Removes the permission for the specified principal from the specified resource.
*
*
*
* This is a convenience which creates an instance of the {@link RemoveResourcePermissionRequest.Builder} avoiding
* the need to create one manually via {@link RemoveResourcePermissionRequest#builder()}
*
*
* @param removeResourcePermissionRequest
* A {@link Consumer} that will call methods on {@link RemoveResourcePermissionRequest.Builder} to create a
* request.
* @return Result of the RemoveResourcePermission operation returned by the service.
* @throws UnauthorizedOperationException
* The operation is not permitted.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.RemoveResourcePermission
* @see AWS API Documentation
*/
default RemoveResourcePermissionResponse removeResourcePermission(
Consumer removeResourcePermissionRequest)
throws UnauthorizedOperationException, UnauthorizedResourceAccessException, FailedDependencyException,
ServiceUnavailableException, AwsServiceException, SdkClientException, WorkDocsException {
return removeResourcePermission(RemoveResourcePermissionRequest.builder().applyMutation(removeResourcePermissionRequest)
.build());
}
/**
*
* Updates the specified attributes of a document. The user must have access to both the document and its parent
* folder, if applicable.
*
*
* @param updateDocumentRequest
* @return Result of the UpdateDocument operation returned by the service.
* @throws EntityNotExistsException
* The resource does not exist.
* @throws EntityAlreadyExistsException
* The resource already exists.
* @throws LimitExceededException
* The maximum of 100,000 folders under the parent folder has been exceeded.
* @throws ProhibitedStateException
* The specified document version is not in the INITIALIZED state.
* @throws ConflictingOperationException
* Another operation is in progress on the resource that conflicts with the current operation.
* @throws ConcurrentModificationException
* The resource hierarchy is changing.
* @throws UnauthorizedOperationException
* The operation is not permitted.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.UpdateDocument
* @see AWS API
* Documentation
*/
default UpdateDocumentResponse updateDocument(UpdateDocumentRequest updateDocumentRequest) throws EntityNotExistsException,
EntityAlreadyExistsException, LimitExceededException, ProhibitedStateException, ConflictingOperationException,
ConcurrentModificationException, UnauthorizedOperationException, UnauthorizedResourceAccessException,
FailedDependencyException, ServiceUnavailableException, AwsServiceException, SdkClientException, WorkDocsException {
throw new UnsupportedOperationException();
}
/**
*
* Updates the specified attributes of a document. The user must have access to both the document and its parent
* folder, if applicable.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateDocumentRequest.Builder} avoiding the need to
* create one manually via {@link UpdateDocumentRequest#builder()}
*
*
* @param updateDocumentRequest
* A {@link Consumer} that will call methods on {@link UpdateDocumentRequest.Builder} to create a request.
* @return Result of the UpdateDocument operation returned by the service.
* @throws EntityNotExistsException
* The resource does not exist.
* @throws EntityAlreadyExistsException
* The resource already exists.
* @throws LimitExceededException
* The maximum of 100,000 folders under the parent folder has been exceeded.
* @throws ProhibitedStateException
* The specified document version is not in the INITIALIZED state.
* @throws ConflictingOperationException
* Another operation is in progress on the resource that conflicts with the current operation.
* @throws ConcurrentModificationException
* The resource hierarchy is changing.
* @throws UnauthorizedOperationException
* The operation is not permitted.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.UpdateDocument
* @see AWS API
* Documentation
*/
default UpdateDocumentResponse updateDocument(Consumer updateDocumentRequest)
throws EntityNotExistsException, EntityAlreadyExistsException, LimitExceededException, ProhibitedStateException,
ConflictingOperationException, ConcurrentModificationException, UnauthorizedOperationException,
UnauthorizedResourceAccessException, FailedDependencyException, ServiceUnavailableException, AwsServiceException,
SdkClientException, WorkDocsException {
return updateDocument(UpdateDocumentRequest.builder().applyMutation(updateDocumentRequest).build());
}
/**
*
* Changes the status of the document version to ACTIVE.
*
*
* Amazon WorkDocs also sets its document container to ACTIVE. This is the last step in a document upload, after the
* client uploads the document to an S3-presigned URL returned by InitiateDocumentVersionUpload.
*
*
* @param updateDocumentVersionRequest
* @return Result of the UpdateDocumentVersion operation returned by the service.
* @throws EntityNotExistsException
* The resource does not exist.
* @throws ProhibitedStateException
* The specified document version is not in the INITIALIZED state.
* @throws ConcurrentModificationException
* The resource hierarchy is changing.
* @throws InvalidOperationException
* The operation is invalid.
* @throws UnauthorizedOperationException
* The operation is not permitted.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.UpdateDocumentVersion
* @see AWS API Documentation
*/
default UpdateDocumentVersionResponse updateDocumentVersion(UpdateDocumentVersionRequest updateDocumentVersionRequest)
throws EntityNotExistsException, ProhibitedStateException, ConcurrentModificationException,
InvalidOperationException, UnauthorizedOperationException, UnauthorizedResourceAccessException,
FailedDependencyException, ServiceUnavailableException, AwsServiceException, SdkClientException, WorkDocsException {
throw new UnsupportedOperationException();
}
/**
*
* Changes the status of the document version to ACTIVE.
*
*
* Amazon WorkDocs also sets its document container to ACTIVE. This is the last step in a document upload, after the
* client uploads the document to an S3-presigned URL returned by InitiateDocumentVersionUpload.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateDocumentVersionRequest.Builder} avoiding the
* need to create one manually via {@link UpdateDocumentVersionRequest#builder()}
*
*
* @param updateDocumentVersionRequest
* A {@link Consumer} that will call methods on {@link UpdateDocumentVersionRequest.Builder} to create a
* request.
* @return Result of the UpdateDocumentVersion operation returned by the service.
* @throws EntityNotExistsException
* The resource does not exist.
* @throws ProhibitedStateException
* The specified document version is not in the INITIALIZED state.
* @throws ConcurrentModificationException
* The resource hierarchy is changing.
* @throws InvalidOperationException
* The operation is invalid.
* @throws UnauthorizedOperationException
* The operation is not permitted.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.UpdateDocumentVersion
* @see AWS API Documentation
*/
default UpdateDocumentVersionResponse updateDocumentVersion(
Consumer updateDocumentVersionRequest) throws EntityNotExistsException,
ProhibitedStateException, ConcurrentModificationException, InvalidOperationException, UnauthorizedOperationException,
UnauthorizedResourceAccessException, FailedDependencyException, ServiceUnavailableException, AwsServiceException,
SdkClientException, WorkDocsException {
return updateDocumentVersion(UpdateDocumentVersionRequest.builder().applyMutation(updateDocumentVersionRequest).build());
}
/**
*
* Updates the specified attributes of the specified folder. The user must have access to both the folder and its
* parent folder, if applicable.
*
*
* @param updateFolderRequest
* @return Result of the UpdateFolder operation returned by the service.
* @throws EntityNotExistsException
* The resource does not exist.
* @throws EntityAlreadyExistsException
* The resource already exists.
* @throws ProhibitedStateException
* The specified document version is not in the INITIALIZED state.
* @throws ConflictingOperationException
* Another operation is in progress on the resource that conflicts with the current operation.
* @throws ConcurrentModificationException
* The resource hierarchy is changing.
* @throws LimitExceededException
* The maximum of 100,000 folders under the parent folder has been exceeded.
* @throws UnauthorizedOperationException
* The operation is not permitted.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.UpdateFolder
* @see AWS API
* Documentation
*/
default UpdateFolderResponse updateFolder(UpdateFolderRequest updateFolderRequest) throws EntityNotExistsException,
EntityAlreadyExistsException, ProhibitedStateException, ConflictingOperationException,
ConcurrentModificationException, LimitExceededException, UnauthorizedOperationException,
UnauthorizedResourceAccessException, FailedDependencyException, ServiceUnavailableException, AwsServiceException,
SdkClientException, WorkDocsException {
throw new UnsupportedOperationException();
}
/**
*
* Updates the specified attributes of the specified folder. The user must have access to both the folder and its
* parent folder, if applicable.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateFolderRequest.Builder} avoiding the need to
* create one manually via {@link UpdateFolderRequest#builder()}
*
*
* @param updateFolderRequest
* A {@link Consumer} that will call methods on {@link UpdateFolderRequest.Builder} to create a request.
* @return Result of the UpdateFolder operation returned by the service.
* @throws EntityNotExistsException
* The resource does not exist.
* @throws EntityAlreadyExistsException
* The resource already exists.
* @throws ProhibitedStateException
* The specified document version is not in the INITIALIZED state.
* @throws ConflictingOperationException
* Another operation is in progress on the resource that conflicts with the current operation.
* @throws ConcurrentModificationException
* The resource hierarchy is changing.
* @throws LimitExceededException
* The maximum of 100,000 folders under the parent folder has been exceeded.
* @throws UnauthorizedOperationException
* The operation is not permitted.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.UpdateFolder
* @see AWS API
* Documentation
*/
default UpdateFolderResponse updateFolder(Consumer updateFolderRequest)
throws EntityNotExistsException, EntityAlreadyExistsException, ProhibitedStateException,
ConflictingOperationException, ConcurrentModificationException, LimitExceededException,
UnauthorizedOperationException, UnauthorizedResourceAccessException, FailedDependencyException,
ServiceUnavailableException, AwsServiceException, SdkClientException, WorkDocsException {
return updateFolder(UpdateFolderRequest.builder().applyMutation(updateFolderRequest).build());
}
/**
*
* Updates the specified attributes of the specified user, and grants or revokes administrative privileges to the
* Amazon WorkDocs site.
*
*
* @param updateUserRequest
* @return Result of the UpdateUser operation returned by the service.
* @throws EntityNotExistsException
* The resource does not exist.
* @throws UnauthorizedOperationException
* The operation is not permitted.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws IllegalUserStateException
* The user is undergoing transfer of ownership.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws DeactivatingLastSystemUserException
* The last user in the organization is being deactivated.
* @throws InvalidArgumentException
* The pagination marker or limit fields are not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.UpdateUser
* @see AWS API
* Documentation
*/
default UpdateUserResponse updateUser(UpdateUserRequest updateUserRequest) throws EntityNotExistsException,
UnauthorizedOperationException, UnauthorizedResourceAccessException, IllegalUserStateException,
FailedDependencyException, ServiceUnavailableException, DeactivatingLastSystemUserException,
InvalidArgumentException, AwsServiceException, SdkClientException, WorkDocsException {
throw new UnsupportedOperationException();
}
/**
*
* Updates the specified attributes of the specified user, and grants or revokes administrative privileges to the
* Amazon WorkDocs site.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateUserRequest.Builder} avoiding the need to
* create one manually via {@link UpdateUserRequest#builder()}
*
*
* @param updateUserRequest
* A {@link Consumer} that will call methods on {@link UpdateUserRequest.Builder} to create a request.
* @return Result of the UpdateUser operation returned by the service.
* @throws EntityNotExistsException
* The resource does not exist.
* @throws UnauthorizedOperationException
* The operation is not permitted.
* @throws UnauthorizedResourceAccessException
* The caller does not have access to perform the action on the resource.
* @throws IllegalUserStateException
* The user is undergoing transfer of ownership.
* @throws FailedDependencyException
* The AWS Directory Service cannot reach an on-premises instance. Or a dependency under the control of the
* organization is failing, such as a connected Active Directory.
* @throws ServiceUnavailableException
* One or more of the dependencies is unavailable.
* @throws DeactivatingLastSystemUserException
* The last user in the organization is being deactivated.
* @throws InvalidArgumentException
* The pagination marker or limit fields are not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws WorkDocsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample WorkDocsClient.UpdateUser
* @see AWS API
* Documentation
*/
default UpdateUserResponse updateUser(Consumer updateUserRequest) throws EntityNotExistsException,
UnauthorizedOperationException, UnauthorizedResourceAccessException, IllegalUserStateException,
FailedDependencyException, ServiceUnavailableException, DeactivatingLastSystemUserException,
InvalidArgumentException, AwsServiceException, SdkClientException, WorkDocsException {
return updateUser(UpdateUserRequest.builder().applyMutation(updateUserRequest).build());
}
static ServiceMetadata serviceMetadata() {
return ServiceMetadata.of(SERVICE_METADATA_ID);
}
}