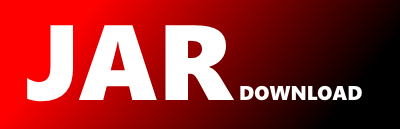
software.amazon.awssdk.services.workdocs.model.DeleteCustomMetadataRequest Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.workdocs.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class DeleteCustomMetadataRequest extends WorkDocsRequest implements
ToCopyableBuilder {
private static final SdkField AUTHENTICATION_TOKEN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AuthenticationToken").getter(getter(DeleteCustomMetadataRequest::authenticationToken))
.setter(setter(Builder::authenticationToken))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("Authentication").build()).build();
private static final SdkField RESOURCE_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ResourceId").getter(getter(DeleteCustomMetadataRequest::resourceId)).setter(setter(Builder::resourceId))
.traits(LocationTrait.builder().location(MarshallLocation.PATH).locationName("ResourceId").build()).build();
private static final SdkField VERSION_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("VersionId").getter(getter(DeleteCustomMetadataRequest::versionId)).setter(setter(Builder::versionId))
.traits(LocationTrait.builder().location(MarshallLocation.QUERY_PARAM).locationName("versionId").build()).build();
private static final SdkField> KEYS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Keys")
.getter(getter(DeleteCustomMetadataRequest::keys))
.setter(setter(Builder::keys))
.traits(LocationTrait.builder().location(MarshallLocation.QUERY_PARAM).locationName("keys").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField DELETE_ALL_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("DeleteAll").getter(getter(DeleteCustomMetadataRequest::deleteAll)).setter(setter(Builder::deleteAll))
.traits(LocationTrait.builder().location(MarshallLocation.QUERY_PARAM).locationName("deleteAll").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(AUTHENTICATION_TOKEN_FIELD,
RESOURCE_ID_FIELD, VERSION_ID_FIELD, KEYS_FIELD, DELETE_ALL_FIELD));
private final String authenticationToken;
private final String resourceId;
private final String versionId;
private final List keys;
private final Boolean deleteAll;
private DeleteCustomMetadataRequest(BuilderImpl builder) {
super(builder);
this.authenticationToken = builder.authenticationToken;
this.resourceId = builder.resourceId;
this.versionId = builder.versionId;
this.keys = builder.keys;
this.deleteAll = builder.deleteAll;
}
/**
*
* Amazon WorkDocs authentication token. Not required when using Amazon Web Services administrator credentials to
* access the API.
*
*
* @return Amazon WorkDocs authentication token. Not required when using Amazon Web Services administrator
* credentials to access the API.
*/
public final String authenticationToken() {
return authenticationToken;
}
/**
*
* The ID of the resource, either a document or folder.
*
*
* @return The ID of the resource, either a document or folder.
*/
public final String resourceId() {
return resourceId;
}
/**
*
* The ID of the version, if the custom metadata is being deleted from a document version.
*
*
* @return The ID of the version, if the custom metadata is being deleted from a document version.
*/
public final String versionId() {
return versionId;
}
/**
* For responses, this returns true if the service returned a value for the Keys property. This DOES NOT check that
* the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is useful
* because the SDK will never return a null collection or map, but you may need to differentiate between the service
* returning nothing (or null) and the service returning an empty collection or map. For requests, this returns true
* if a value for the property was specified in the request builder, and false if a value was not specified.
*/
public final boolean hasKeys() {
return keys != null && !(keys instanceof SdkAutoConstructList);
}
/**
*
* List of properties to remove.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasKeys} method.
*
*
* @return List of properties to remove.
*/
public final List keys() {
return keys;
}
/**
*
* Flag to indicate removal of all custom metadata properties from the specified resource.
*
*
* @return Flag to indicate removal of all custom metadata properties from the specified resource.
*/
public final Boolean deleteAll() {
return deleteAll;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(authenticationToken());
hashCode = 31 * hashCode + Objects.hashCode(resourceId());
hashCode = 31 * hashCode + Objects.hashCode(versionId());
hashCode = 31 * hashCode + Objects.hashCode(hasKeys() ? keys() : null);
hashCode = 31 * hashCode + Objects.hashCode(deleteAll());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof DeleteCustomMetadataRequest)) {
return false;
}
DeleteCustomMetadataRequest other = (DeleteCustomMetadataRequest) obj;
return Objects.equals(authenticationToken(), other.authenticationToken())
&& Objects.equals(resourceId(), other.resourceId()) && Objects.equals(versionId(), other.versionId())
&& hasKeys() == other.hasKeys() && Objects.equals(keys(), other.keys())
&& Objects.equals(deleteAll(), other.deleteAll());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("DeleteCustomMetadataRequest")
.add("AuthenticationToken", authenticationToken() == null ? null : "*** Sensitive Data Redacted ***")
.add("ResourceId", resourceId()).add("VersionId", versionId()).add("Keys", hasKeys() ? keys() : null)
.add("DeleteAll", deleteAll()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "AuthenticationToken":
return Optional.ofNullable(clazz.cast(authenticationToken()));
case "ResourceId":
return Optional.ofNullable(clazz.cast(resourceId()));
case "VersionId":
return Optional.ofNullable(clazz.cast(versionId()));
case "Keys":
return Optional.ofNullable(clazz.cast(keys()));
case "DeleteAll":
return Optional.ofNullable(clazz.cast(deleteAll()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function