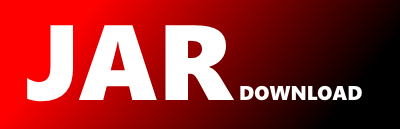
software.amazon.awssdk.services.workdocs.model.CreateCommentRequest Maven / Gradle / Ivy
Show all versions of workdocs Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.workdocs.model;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class CreateCommentRequest extends WorkDocsRequest implements
ToCopyableBuilder {
private static final SdkField AUTHENTICATION_TOKEN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AuthenticationToken").getter(getter(CreateCommentRequest::authenticationToken))
.setter(setter(Builder::authenticationToken))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("Authentication").build()).build();
private static final SdkField DOCUMENT_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("DocumentId").getter(getter(CreateCommentRequest::documentId)).setter(setter(Builder::documentId))
.traits(LocationTrait.builder().location(MarshallLocation.PATH).locationName("DocumentId").build()).build();
private static final SdkField VERSION_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("VersionId").getter(getter(CreateCommentRequest::versionId)).setter(setter(Builder::versionId))
.traits(LocationTrait.builder().location(MarshallLocation.PATH).locationName("VersionId").build()).build();
private static final SdkField PARENT_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ParentId").getter(getter(CreateCommentRequest::parentId)).setter(setter(Builder::parentId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ParentId").build()).build();
private static final SdkField THREAD_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ThreadId").getter(getter(CreateCommentRequest::threadId)).setter(setter(Builder::threadId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ThreadId").build()).build();
private static final SdkField TEXT_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Text")
.getter(getter(CreateCommentRequest::text)).setter(setter(Builder::text))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Text").build()).build();
private static final SdkField VISIBILITY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Visibility").getter(getter(CreateCommentRequest::visibilityAsString))
.setter(setter(Builder::visibility))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Visibility").build()).build();
private static final SdkField NOTIFY_COLLABORATORS_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("NotifyCollaborators").getter(getter(CreateCommentRequest::notifyCollaborators))
.setter(setter(Builder::notifyCollaborators))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NotifyCollaborators").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(AUTHENTICATION_TOKEN_FIELD,
DOCUMENT_ID_FIELD, VERSION_ID_FIELD, PARENT_ID_FIELD, THREAD_ID_FIELD, TEXT_FIELD, VISIBILITY_FIELD,
NOTIFY_COLLABORATORS_FIELD));
private final String authenticationToken;
private final String documentId;
private final String versionId;
private final String parentId;
private final String threadId;
private final String text;
private final String visibility;
private final Boolean notifyCollaborators;
private CreateCommentRequest(BuilderImpl builder) {
super(builder);
this.authenticationToken = builder.authenticationToken;
this.documentId = builder.documentId;
this.versionId = builder.versionId;
this.parentId = builder.parentId;
this.threadId = builder.threadId;
this.text = builder.text;
this.visibility = builder.visibility;
this.notifyCollaborators = builder.notifyCollaborators;
}
/**
*
* Amazon WorkDocs authentication token. Not required when using Amazon Web Services administrator credentials to
* access the API.
*
*
* @return Amazon WorkDocs authentication token. Not required when using Amazon Web Services administrator
* credentials to access the API.
*/
public final String authenticationToken() {
return authenticationToken;
}
/**
*
* The ID of the document.
*
*
* @return The ID of the document.
*/
public final String documentId() {
return documentId;
}
/**
*
* The ID of the document version.
*
*
* @return The ID of the document version.
*/
public final String versionId() {
return versionId;
}
/**
*
* The ID of the parent comment.
*
*
* @return The ID of the parent comment.
*/
public final String parentId() {
return parentId;
}
/**
*
* The ID of the root comment in the thread.
*
*
* @return The ID of the root comment in the thread.
*/
public final String threadId() {
return threadId;
}
/**
*
* The text of the comment.
*
*
* @return The text of the comment.
*/
public final String text() {
return text;
}
/**
*
* The visibility of the comment. Options are either PRIVATE, where the comment is visible only to the comment
* author and document owner and co-owners, or PUBLIC, where the comment is visible to document owners, co-owners,
* and contributors.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #visibility} will
* return {@link CommentVisibilityType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #visibilityAsString}.
*
*
* @return The visibility of the comment. Options are either PRIVATE, where the comment is visible only to the
* comment author and document owner and co-owners, or PUBLIC, where the comment is visible to document
* owners, co-owners, and contributors.
* @see CommentVisibilityType
*/
public final CommentVisibilityType visibility() {
return CommentVisibilityType.fromValue(visibility);
}
/**
*
* The visibility of the comment. Options are either PRIVATE, where the comment is visible only to the comment
* author and document owner and co-owners, or PUBLIC, where the comment is visible to document owners, co-owners,
* and contributors.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #visibility} will
* return {@link CommentVisibilityType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #visibilityAsString}.
*
*
* @return The visibility of the comment. Options are either PRIVATE, where the comment is visible only to the
* comment author and document owner and co-owners, or PUBLIC, where the comment is visible to document
* owners, co-owners, and contributors.
* @see CommentVisibilityType
*/
public final String visibilityAsString() {
return visibility;
}
/**
*
* Set this parameter to TRUE to send an email out to the document collaborators after the comment is created.
*
*
* @return Set this parameter to TRUE to send an email out to the document collaborators after the comment is
* created.
*/
public final Boolean notifyCollaborators() {
return notifyCollaborators;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(authenticationToken());
hashCode = 31 * hashCode + Objects.hashCode(documentId());
hashCode = 31 * hashCode + Objects.hashCode(versionId());
hashCode = 31 * hashCode + Objects.hashCode(parentId());
hashCode = 31 * hashCode + Objects.hashCode(threadId());
hashCode = 31 * hashCode + Objects.hashCode(text());
hashCode = 31 * hashCode + Objects.hashCode(visibilityAsString());
hashCode = 31 * hashCode + Objects.hashCode(notifyCollaborators());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CreateCommentRequest)) {
return false;
}
CreateCommentRequest other = (CreateCommentRequest) obj;
return Objects.equals(authenticationToken(), other.authenticationToken())
&& Objects.equals(documentId(), other.documentId()) && Objects.equals(versionId(), other.versionId())
&& Objects.equals(parentId(), other.parentId()) && Objects.equals(threadId(), other.threadId())
&& Objects.equals(text(), other.text()) && Objects.equals(visibilityAsString(), other.visibilityAsString())
&& Objects.equals(notifyCollaborators(), other.notifyCollaborators());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("CreateCommentRequest")
.add("AuthenticationToken", authenticationToken() == null ? null : "*** Sensitive Data Redacted ***")
.add("DocumentId", documentId()).add("VersionId", versionId()).add("ParentId", parentId())
.add("ThreadId", threadId()).add("Text", text() == null ? null : "*** Sensitive Data Redacted ***")
.add("Visibility", visibilityAsString()).add("NotifyCollaborators", notifyCollaborators()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "AuthenticationToken":
return Optional.ofNullable(clazz.cast(authenticationToken()));
case "DocumentId":
return Optional.ofNullable(clazz.cast(documentId()));
case "VersionId":
return Optional.ofNullable(clazz.cast(versionId()));
case "ParentId":
return Optional.ofNullable(clazz.cast(parentId()));
case "ThreadId":
return Optional.ofNullable(clazz.cast(threadId()));
case "Text":
return Optional.ofNullable(clazz.cast(text()));
case "Visibility":
return Optional.ofNullable(clazz.cast(visibilityAsString()));
case "NotifyCollaborators":
return Optional.ofNullable(clazz.cast(notifyCollaborators()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function