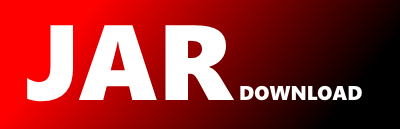
software.amazon.awssdk.services.workdocs.model.CreateUserRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of workdocs Show documentation
Show all versions of workdocs Show documentation
The AWS Java SDK for Amazon WorkDocs module holds the client classes that are used for
communicating with Amazon WorkDocs Service.
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.workdocs.model;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class CreateUserRequest extends WorkDocsRequest implements
ToCopyableBuilder {
private static final SdkField ORGANIZATION_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("OrganizationId").getter(getter(CreateUserRequest::organizationId))
.setter(setter(Builder::organizationId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OrganizationId").build()).build();
private static final SdkField USERNAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Username").getter(getter(CreateUserRequest::username)).setter(setter(Builder::username))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Username").build()).build();
private static final SdkField EMAIL_ADDRESS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("EmailAddress").getter(getter(CreateUserRequest::emailAddress)).setter(setter(Builder::emailAddress))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EmailAddress").build()).build();
private static final SdkField GIVEN_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("GivenName").getter(getter(CreateUserRequest::givenName)).setter(setter(Builder::givenName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("GivenName").build()).build();
private static final SdkField SURNAME_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Surname")
.getter(getter(CreateUserRequest::surname)).setter(setter(Builder::surname))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Surname").build()).build();
private static final SdkField PASSWORD_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Password").getter(getter(CreateUserRequest::password)).setter(setter(Builder::password))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Password").build()).build();
private static final SdkField TIME_ZONE_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TimeZoneId").getter(getter(CreateUserRequest::timeZoneId)).setter(setter(Builder::timeZoneId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TimeZoneId").build()).build();
private static final SdkField STORAGE_RULE_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("StorageRule")
.getter(getter(CreateUserRequest::storageRule)).setter(setter(Builder::storageRule))
.constructor(StorageRuleType::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StorageRule").build()).build();
private static final SdkField AUTHENTICATION_TOKEN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AuthenticationToken").getter(getter(CreateUserRequest::authenticationToken))
.setter(setter(Builder::authenticationToken))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("Authentication").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ORGANIZATION_ID_FIELD,
USERNAME_FIELD, EMAIL_ADDRESS_FIELD, GIVEN_NAME_FIELD, SURNAME_FIELD, PASSWORD_FIELD, TIME_ZONE_ID_FIELD,
STORAGE_RULE_FIELD, AUTHENTICATION_TOKEN_FIELD));
private static final Map> SDK_NAME_TO_FIELD = memberNameToFieldInitializer();
private final String organizationId;
private final String username;
private final String emailAddress;
private final String givenName;
private final String surname;
private final String password;
private final String timeZoneId;
private final StorageRuleType storageRule;
private final String authenticationToken;
private CreateUserRequest(BuilderImpl builder) {
super(builder);
this.organizationId = builder.organizationId;
this.username = builder.username;
this.emailAddress = builder.emailAddress;
this.givenName = builder.givenName;
this.surname = builder.surname;
this.password = builder.password;
this.timeZoneId = builder.timeZoneId;
this.storageRule = builder.storageRule;
this.authenticationToken = builder.authenticationToken;
}
/**
*
* The ID of the organization.
*
*
* @return The ID of the organization.
*/
public final String organizationId() {
return organizationId;
}
/**
*
* The login name of the user.
*
*
* @return The login name of the user.
*/
public final String username() {
return username;
}
/**
*
* The email address of the user.
*
*
* @return The email address of the user.
*/
public final String emailAddress() {
return emailAddress;
}
/**
*
* The given name of the user.
*
*
* @return The given name of the user.
*/
public final String givenName() {
return givenName;
}
/**
*
* The surname of the user.
*
*
* @return The surname of the user.
*/
public final String surname() {
return surname;
}
/**
*
* The password of the user.
*
*
* @return The password of the user.
*/
public final String password() {
return password;
}
/**
*
* The time zone ID of the user.
*
*
* @return The time zone ID of the user.
*/
public final String timeZoneId() {
return timeZoneId;
}
/**
*
* The amount of storage for the user.
*
*
* @return The amount of storage for the user.
*/
public final StorageRuleType storageRule() {
return storageRule;
}
/**
*
* Amazon WorkDocs authentication token. Not required when using Amazon Web Services administrator credentials to
* access the API.
*
*
* @return Amazon WorkDocs authentication token. Not required when using Amazon Web Services administrator
* credentials to access the API.
*/
public final String authenticationToken() {
return authenticationToken;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(organizationId());
hashCode = 31 * hashCode + Objects.hashCode(username());
hashCode = 31 * hashCode + Objects.hashCode(emailAddress());
hashCode = 31 * hashCode + Objects.hashCode(givenName());
hashCode = 31 * hashCode + Objects.hashCode(surname());
hashCode = 31 * hashCode + Objects.hashCode(password());
hashCode = 31 * hashCode + Objects.hashCode(timeZoneId());
hashCode = 31 * hashCode + Objects.hashCode(storageRule());
hashCode = 31 * hashCode + Objects.hashCode(authenticationToken());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CreateUserRequest)) {
return false;
}
CreateUserRequest other = (CreateUserRequest) obj;
return Objects.equals(organizationId(), other.organizationId()) && Objects.equals(username(), other.username())
&& Objects.equals(emailAddress(), other.emailAddress()) && Objects.equals(givenName(), other.givenName())
&& Objects.equals(surname(), other.surname()) && Objects.equals(password(), other.password())
&& Objects.equals(timeZoneId(), other.timeZoneId()) && Objects.equals(storageRule(), other.storageRule())
&& Objects.equals(authenticationToken(), other.authenticationToken());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("CreateUserRequest").add("OrganizationId", organizationId())
.add("Username", username() == null ? null : "*** Sensitive Data Redacted ***")
.add("EmailAddress", emailAddress() == null ? null : "*** Sensitive Data Redacted ***")
.add("GivenName", givenName() == null ? null : "*** Sensitive Data Redacted ***")
.add("Surname", surname() == null ? null : "*** Sensitive Data Redacted ***")
.add("Password", password() == null ? null : "*** Sensitive Data Redacted ***").add("TimeZoneId", timeZoneId())
.add("StorageRule", storageRule())
.add("AuthenticationToken", authenticationToken() == null ? null : "*** Sensitive Data Redacted ***").build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "OrganizationId":
return Optional.ofNullable(clazz.cast(organizationId()));
case "Username":
return Optional.ofNullable(clazz.cast(username()));
case "EmailAddress":
return Optional.ofNullable(clazz.cast(emailAddress()));
case "GivenName":
return Optional.ofNullable(clazz.cast(givenName()));
case "Surname":
return Optional.ofNullable(clazz.cast(surname()));
case "Password":
return Optional.ofNullable(clazz.cast(password()));
case "TimeZoneId":
return Optional.ofNullable(clazz.cast(timeZoneId()));
case "StorageRule":
return Optional.ofNullable(clazz.cast(storageRule()));
case "AuthenticationToken":
return Optional.ofNullable(clazz.cast(authenticationToken()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Map> memberNameToFieldInitializer() {
Map> map = new HashMap<>();
map.put("OrganizationId", ORGANIZATION_ID_FIELD);
map.put("Username", USERNAME_FIELD);
map.put("EmailAddress", EMAIL_ADDRESS_FIELD);
map.put("GivenName", GIVEN_NAME_FIELD);
map.put("Surname", SURNAME_FIELD);
map.put("Password", PASSWORD_FIELD);
map.put("TimeZoneId", TIME_ZONE_ID_FIELD);
map.put("StorageRule", STORAGE_RULE_FIELD);
map.put("Authentication", AUTHENTICATION_TOKEN_FIELD);
return Collections.unmodifiableMap(map);
}
private static Function
© 2015 - 2025 Weber Informatics LLC | Privacy Policy