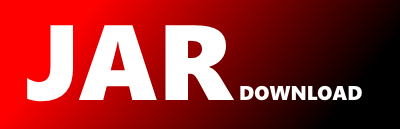
software.amazon.awssdk.services.workdocs.model.FolderMetadata Maven / Gradle / Ivy
Show all versions of workdocs Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.workdocs.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Describes a folder.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class FolderMetadata implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField ID_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Id")
.getter(getter(FolderMetadata::id)).setter(setter(Builder::id))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Id").build()).build();
private static final SdkField NAME_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Name")
.getter(getter(FolderMetadata::name)).setter(setter(Builder::name))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Name").build()).build();
private static final SdkField CREATOR_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("CreatorId").getter(getter(FolderMetadata::creatorId)).setter(setter(Builder::creatorId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CreatorId").build()).build();
private static final SdkField PARENT_FOLDER_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ParentFolderId").getter(getter(FolderMetadata::parentFolderId)).setter(setter(Builder::parentFolderId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ParentFolderId").build()).build();
private static final SdkField CREATED_TIMESTAMP_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("CreatedTimestamp").getter(getter(FolderMetadata::createdTimestamp))
.setter(setter(Builder::createdTimestamp))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CreatedTimestamp").build()).build();
private static final SdkField MODIFIED_TIMESTAMP_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("ModifiedTimestamp").getter(getter(FolderMetadata::modifiedTimestamp))
.setter(setter(Builder::modifiedTimestamp))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ModifiedTimestamp").build()).build();
private static final SdkField RESOURCE_STATE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ResourceState").getter(getter(FolderMetadata::resourceStateAsString))
.setter(setter(Builder::resourceState))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ResourceState").build()).build();
private static final SdkField SIGNATURE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Signature").getter(getter(FolderMetadata::signature)).setter(setter(Builder::signature))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Signature").build()).build();
private static final SdkField> LABELS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Labels")
.getter(getter(FolderMetadata::labels))
.setter(setter(Builder::labels))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Labels").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField SIZE_FIELD = SdkField. builder(MarshallingType.LONG).memberName("Size")
.getter(getter(FolderMetadata::size)).setter(setter(Builder::size))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Size").build()).build();
private static final SdkField LATEST_VERSION_SIZE_FIELD = SdkField. builder(MarshallingType.LONG)
.memberName("LatestVersionSize").getter(getter(FolderMetadata::latestVersionSize))
.setter(setter(Builder::latestVersionSize))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LatestVersionSize").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ID_FIELD, NAME_FIELD,
CREATOR_ID_FIELD, PARENT_FOLDER_ID_FIELD, CREATED_TIMESTAMP_FIELD, MODIFIED_TIMESTAMP_FIELD, RESOURCE_STATE_FIELD,
SIGNATURE_FIELD, LABELS_FIELD, SIZE_FIELD, LATEST_VERSION_SIZE_FIELD));
private static final Map> SDK_NAME_TO_FIELD = memberNameToFieldInitializer();
private static final long serialVersionUID = 1L;
private final String id;
private final String name;
private final String creatorId;
private final String parentFolderId;
private final Instant createdTimestamp;
private final Instant modifiedTimestamp;
private final String resourceState;
private final String signature;
private final List labels;
private final Long size;
private final Long latestVersionSize;
private FolderMetadata(BuilderImpl builder) {
this.id = builder.id;
this.name = builder.name;
this.creatorId = builder.creatorId;
this.parentFolderId = builder.parentFolderId;
this.createdTimestamp = builder.createdTimestamp;
this.modifiedTimestamp = builder.modifiedTimestamp;
this.resourceState = builder.resourceState;
this.signature = builder.signature;
this.labels = builder.labels;
this.size = builder.size;
this.latestVersionSize = builder.latestVersionSize;
}
/**
*
* The ID of the folder.
*
*
* @return The ID of the folder.
*/
public final String id() {
return id;
}
/**
*
* The name of the folder.
*
*
* @return The name of the folder.
*/
public final String name() {
return name;
}
/**
*
* The ID of the creator.
*
*
* @return The ID of the creator.
*/
public final String creatorId() {
return creatorId;
}
/**
*
* The ID of the parent folder.
*
*
* @return The ID of the parent folder.
*/
public final String parentFolderId() {
return parentFolderId;
}
/**
*
* The time when the folder was created.
*
*
* @return The time when the folder was created.
*/
public final Instant createdTimestamp() {
return createdTimestamp;
}
/**
*
* The time when the folder was updated.
*
*
* @return The time when the folder was updated.
*/
public final Instant modifiedTimestamp() {
return modifiedTimestamp;
}
/**
*
* The resource state of the folder.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #resourceState}
* will return {@link ResourceStateType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #resourceStateAsString}.
*
*
* @return The resource state of the folder.
* @see ResourceStateType
*/
public final ResourceStateType resourceState() {
return ResourceStateType.fromValue(resourceState);
}
/**
*
* The resource state of the folder.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #resourceState}
* will return {@link ResourceStateType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #resourceStateAsString}.
*
*
* @return The resource state of the folder.
* @see ResourceStateType
*/
public final String resourceStateAsString() {
return resourceState;
}
/**
*
* The unique identifier created from the subfolders and documents of the folder.
*
*
* @return The unique identifier created from the subfolders and documents of the folder.
*/
public final String signature() {
return signature;
}
/**
* For responses, this returns true if the service returned a value for the Labels property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasLabels() {
return labels != null && !(labels instanceof SdkAutoConstructList);
}
/**
*
* List of labels on the folder.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasLabels} method.
*
*
* @return List of labels on the folder.
*/
public final List labels() {
return labels;
}
/**
*
* The size of the folder metadata.
*
*
* @return The size of the folder metadata.
*/
public final Long size() {
return size;
}
/**
*
* The size of the latest version of the folder metadata.
*
*
* @return The size of the latest version of the folder metadata.
*/
public final Long latestVersionSize() {
return latestVersionSize;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(id());
hashCode = 31 * hashCode + Objects.hashCode(name());
hashCode = 31 * hashCode + Objects.hashCode(creatorId());
hashCode = 31 * hashCode + Objects.hashCode(parentFolderId());
hashCode = 31 * hashCode + Objects.hashCode(createdTimestamp());
hashCode = 31 * hashCode + Objects.hashCode(modifiedTimestamp());
hashCode = 31 * hashCode + Objects.hashCode(resourceStateAsString());
hashCode = 31 * hashCode + Objects.hashCode(signature());
hashCode = 31 * hashCode + Objects.hashCode(hasLabels() ? labels() : null);
hashCode = 31 * hashCode + Objects.hashCode(size());
hashCode = 31 * hashCode + Objects.hashCode(latestVersionSize());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof FolderMetadata)) {
return false;
}
FolderMetadata other = (FolderMetadata) obj;
return Objects.equals(id(), other.id()) && Objects.equals(name(), other.name())
&& Objects.equals(creatorId(), other.creatorId()) && Objects.equals(parentFolderId(), other.parentFolderId())
&& Objects.equals(createdTimestamp(), other.createdTimestamp())
&& Objects.equals(modifiedTimestamp(), other.modifiedTimestamp())
&& Objects.equals(resourceStateAsString(), other.resourceStateAsString())
&& Objects.equals(signature(), other.signature()) && hasLabels() == other.hasLabels()
&& Objects.equals(labels(), other.labels()) && Objects.equals(size(), other.size())
&& Objects.equals(latestVersionSize(), other.latestVersionSize());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("FolderMetadata").add("Id", id())
.add("Name", name() == null ? null : "*** Sensitive Data Redacted ***").add("CreatorId", creatorId())
.add("ParentFolderId", parentFolderId()).add("CreatedTimestamp", createdTimestamp())
.add("ModifiedTimestamp", modifiedTimestamp()).add("ResourceState", resourceStateAsString())
.add("Signature", signature()).add("Labels", hasLabels() ? labels() : null).add("Size", size())
.add("LatestVersionSize", latestVersionSize()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Id":
return Optional.ofNullable(clazz.cast(id()));
case "Name":
return Optional.ofNullable(clazz.cast(name()));
case "CreatorId":
return Optional.ofNullable(clazz.cast(creatorId()));
case "ParentFolderId":
return Optional.ofNullable(clazz.cast(parentFolderId()));
case "CreatedTimestamp":
return Optional.ofNullable(clazz.cast(createdTimestamp()));
case "ModifiedTimestamp":
return Optional.ofNullable(clazz.cast(modifiedTimestamp()));
case "ResourceState":
return Optional.ofNullable(clazz.cast(resourceStateAsString()));
case "Signature":
return Optional.ofNullable(clazz.cast(signature()));
case "Labels":
return Optional.ofNullable(clazz.cast(labels()));
case "Size":
return Optional.ofNullable(clazz.cast(size()));
case "LatestVersionSize":
return Optional.ofNullable(clazz.cast(latestVersionSize()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Map> memberNameToFieldInitializer() {
Map> map = new HashMap<>();
map.put("Id", ID_FIELD);
map.put("Name", NAME_FIELD);
map.put("CreatorId", CREATOR_ID_FIELD);
map.put("ParentFolderId", PARENT_FOLDER_ID_FIELD);
map.put("CreatedTimestamp", CREATED_TIMESTAMP_FIELD);
map.put("ModifiedTimestamp", MODIFIED_TIMESTAMP_FIELD);
map.put("ResourceState", RESOURCE_STATE_FIELD);
map.put("Signature", SIGNATURE_FIELD);
map.put("Labels", LABELS_FIELD);
map.put("Size", SIZE_FIELD);
map.put("LatestVersionSize", LATEST_VERSION_SIZE_FIELD);
return Collections.unmodifiableMap(map);
}
private static Function